Jsp结合Velocity实现依据Word模板文件生成对应数据文件
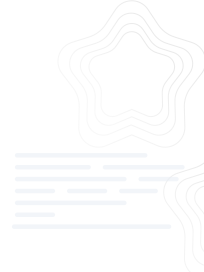


在IT行业中,有时候我们需要将动态数据插入到预设的文档模板中,生成定制化的输出文件。在这种场景下,"Jsp结合Velocity实现依据Word模板文件生成对应数据文件"的技术方案显得尤为实用。JSP(JavaServer Pages)是用于构建动态Web应用的服务器端技术,而Velocity则是一个强大的模板引擎,它允许开发者将Java代码与HTML或Word模板无缝集成,从而实现动态内容的生成。 **JSP** 是一种基于Java的视图技术,它允许开发者在HTML页面中嵌入Java代码来处理动态内容。通过JSP,我们可以从后端服务器获取数据,并将其呈现给前端用户。JSP的核心概念包括JSP指令、脚本元素、表达式和自定义标签等,这些元素帮助开发者创建动态网页。 **Velocity** 是Apache软件基金会的一个开源项目,它提供了一个简单易用的模板语言,用于分离业务逻辑和表现层。Velocity模板语言(VTL)允许开发者在模板中引用Java对象,而无需直接写Java代码。这样,开发者可以专注于内容和布局,而不是关注如何控制流程或处理异常。 **使用JSP和Velocity结合操作Word模板** 的过程通常包括以下步骤: 1. **创建Word模板**:你需要创建一个包含静态文本和占位符的Word文档。占位符是特定格式的文本,例如 `${variable}`,它们将在运行时被动态数据替换。 2. **整合Velocity**:在JSP中,你需要引入Velocity库,并设置Velocity上下文。上下文是存放要传递给模板的数据结构,你可以将Java对象或变量放入其中。 3. **读取模板文件**:使用Velocity的`ResourceLoader`加载Word模板文件,这通常涉及到文件系统的操作或者使用URL。 4. **填充数据**:在上下文中添加你需要的数据,例如数据库查询结果、服务器配置信息等。 5. **渲染模板**:使用Velocity的`Template`和`VelocityContext`对象,将数据合并到模板中,生成新的Word文档。 6. **保存或输出文件**:将生成的Word文档保存到服务器磁盘或直接作为HTTP响应发送给客户端。 这种技术的应用场景广泛,如批量生成报告、个性化合同生成、邮件模板等,尤其在需要大量动态生成Word文档的场景下,它能显著提高效率并减少人工错误。 在提供的`VelocityDemo`文件中,可能包含了实现这个功能的示例代码,包括JSP页面、Velocity模板文件、以及相关的Java后台处理类。通过研究这个示例,你可以更好地理解如何将JSP和Velocity结合,实现在Word模板上的动态数据注入。不过,具体的实现细节需要查看源代码才能详细了解。
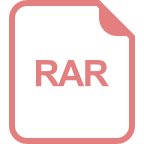
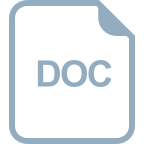
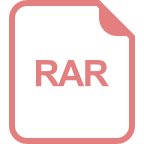
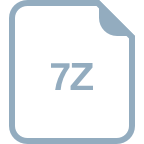
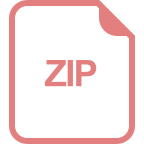
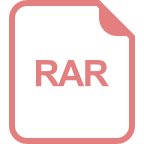
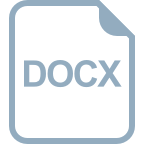
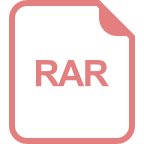
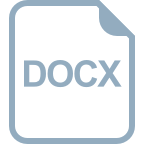
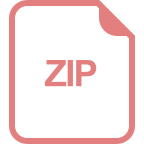
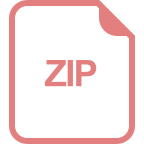
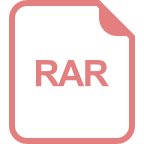
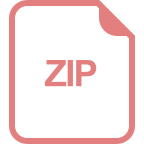
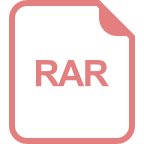
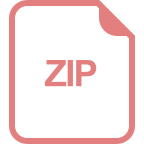
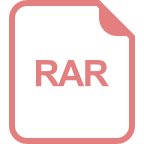
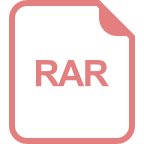
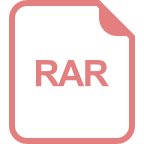
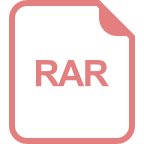
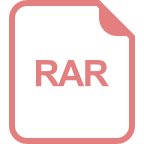
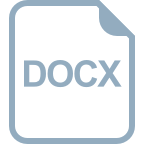
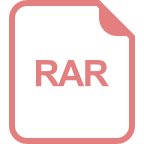


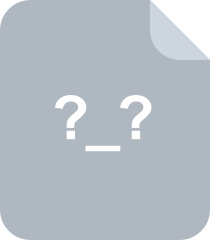
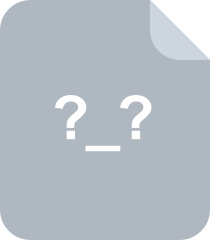


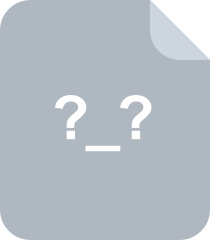
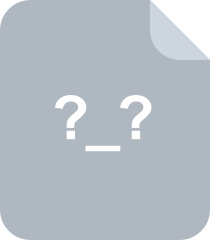

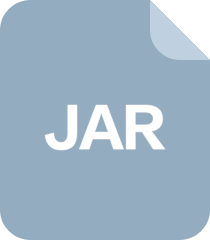
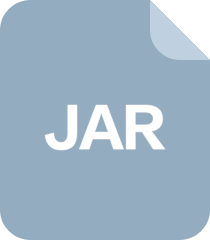
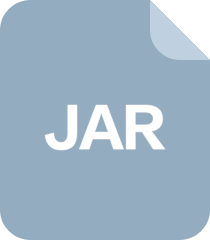




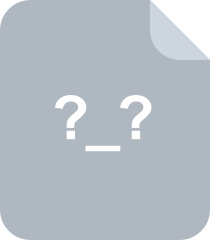
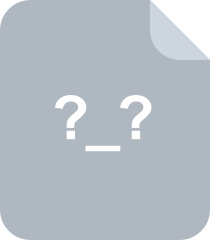

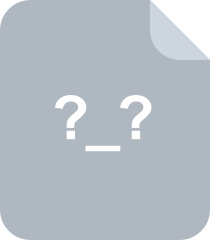
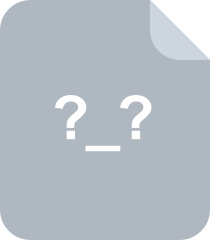

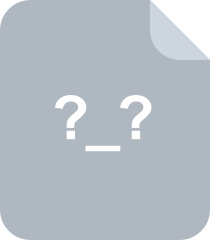





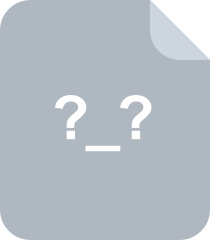
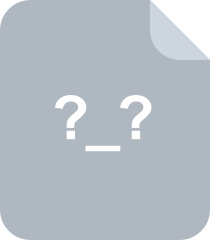
- 1

- 粉丝: 176
- 资源: 68
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

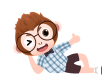
最新资源
- 脉冲注入法,持续注入,启动低速运行过程中注入,电感法,ipd,力矩保持,无霍尔无感方案,媲美有霍尔效果 bldc控制器方案,无刷电机 提供源码,原理图
- Matlab Simulink#直驱永磁风电机组并网仿真模型 基于永磁直驱式风机并网仿真模型 采用背靠背双PWM变流器,先整流,再逆变 不仅实现电机侧的有功、无功功率的解耦控制和转速调节,而且能实
- 157389节奏盒子地狱模式第三阶段7.apk
- 操作系统实验ucore lab3
- DG储能选址定容模型matlab 程序采用改进粒子群算法,考虑时序性得到分布式和储能的选址定容模型,程序运行可靠 这段程序是一个改进的粒子群算法,主要用于解决电力系统中的优化问题 下面我将对程序进行详
- final_work_job1(1).sql
- 区块链与联邦学习结合:FedChain项目详细复现指南
- 西门子S7 和 S7 Plus 协议开发示例
- 模块化多电平变流器 MMC 的VSG控制 同步发电机控制 MATLAB–Simulink仿真模型 5电平三相MMC,采用VSG控制 受端接可编辑三相交流源,直流侧接无穷大电源提供调频能量 设置频率
- 基于小程序的智慧校园管理系统源代码(java+小程序+mysql+LW).zip

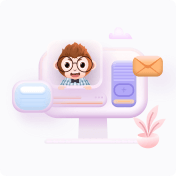

- 1
- 2
- 3
- 4
前往页