Uniform
=======
Sexy form elements with jQuery. Now with HTML5 attributes!
Version 2.1.1
Works well with jQuery 1.6+, but we've received patches and heard that this works with jQuery 1.3.
Licensed under the [MIT License](http://www.opensource.org/licenses/mit-license.php)
Installation
------------
Installation of Uniform is quite simple. First, make sure you have jQuery installed. Then you’ll want to link to the jquery.uniform.js file and uniform.default.css in the head area of your page. Here's what your `<head>` tag contents should probably contain:
<!-- Make sure your CSS file is listed before jQuery -->
<link rel="stylesheet" href="uniform.default.css" media="screen" />
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.8/jquery.min.js"></script>
<script src="jquery.uniform.js"></script>
This relies upon a copy of jquery.uniform.js, uniform.default.css and the various images all being available on your webserver.
Basic usage
-----------
Using Uniform is easy. Simply tell it what elements to style:
// Style all <select> elements
$("select").uniform();
To "uniform" all possible form elements, just do something like this. Things that can't get styled appropriately will be skipped by Uniform.
// Style everything
$("select, input, a.button, button").uniform();
You can exclude elements too by using more jQuery selectors or methods:
// Avoid styling some elements
$("select").not(".skip_these").uniform(); // Method 1
$('select[class!="skip_these"]').uniform(); // Method 2
A complete set of tags in the HEAD section of your site can therefore look like this:
<!-- Make sure your CSS file is listed before jQuery -->
<link rel="stylesheet" href="uniform.default.css" media="screen" />
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.8/jquery.min.js"></script>
<script src="jquery.uniform.js"></script>
<script type='text/javascript'>
// On load, style typical form elements
$(function () {
$("select, input, button").uniform();
});
</script>
Extra parameters
----------------
You can pass in extra parameters to control certain aspects of Uniform. To pass in parameters, use syntax like what is seen here. This only changes the settings for the elements that are actually uniformed in this particular call.
$("select").uniform({
param1: value,
param2: value,
param3: value
});
There is a separate listing of global defaults. You access them by using the `defaults` property. *Note: This property name changed in v2.0.*
$.uniform.defaults.checkedClass = "uniformCheckedClass";
$.uniform.defaults.fileBtnHtml = "Pick a file";
Uniform v1.x had a bug where setting values in the call to `.uniform()` also potentially reset the defaults and redrew other uniformed objects with new settings. As of version 2.0.0 the global defaults are now completely separate from the settings passed to every `.uniform()` call. Extra parameters defined when instantiating Uniform are not global and can't be recalled from `$.uniform.defaults` later.
### activeClass (string)
*Default:* "active"
Sets the class given to elements when they are active (pressed).
$("select").uniform({activeClass: 'myActiveClass'});
### autoHide (boolean)
*Default:* true
If this option is set to true, Uniform will hide the new elements if the existing elements are currently hidden using `display: none`.
If you want to show a select or checkbox you'll need to show the new Uniform div instead of the child element.
### buttonClass (string)
*Default:* "button"
Sets the class given to a button that's been Uniformed
$("input[type=button]").uniform({buttonClass: 'myBtnClass'});
### checkboxClass (string)
*Default:* "checker"
Sets the class given to the wrapper div for checkbox elements.
$(":checkbox").uniform({checkboxClass: 'myCheckClass'});
### checkedClass (string)
*Default:* "checked"
Sets the class given to elements when they are checked (radios and checkboxes).
$(":radio, :checkbox").uniform({checkedClass: 'myCheckedClass'});
### disabledClass (string)
*Default:* "disabled"
Sets the class given to elements when they are disabled.
$("select").uniform({disabledClass: 'myDisabledClass'});
### eventNamespace (string)
*Default:* ".uniform"
Binds events using this namespace with jQuery. Useful if you want to unbind them later. Shouldn't probably need to be changed unless it conflicts with other code.
$("select").uniform({eventNamespace: '.uniformEvents'});
### fileButtonClass (string)
*Default:* "action"
Sets the class given to div inside a file upload container that acts as the "Choose file" button.
$(":file").uniform({fileButtonClass: 'myFileBtnClass'});
### fileButtonHtml (string)
*Default:* "Choose File"
Sets the text written on the action button inside a file upload input.
$(":file").uniform({fileButtonHtml: 'Choose …'});
### fileClass (string)
*Default:* "uploader"
Sets the class given to the wrapper div for file upload elements.
$(":file").uniform({fileClass: 'myFileClass'});
### fileDefaultHtml (string)
*Default:* "No file selected"
Sets the text written in the filename div of a file upload input when there is no file selected.
$(":file").uniform({fileDefaultHtml: 'Select a file please'});
### filenameClass (string)
*Default:* "filename"
Sets the class given to div inside a file upload container that spits out the filename.
$(":file").uniform({filenameClass: 'myFilenameClass'});
### focusClass (string)
*Default:* "focus"
Sets the class given to elements when they are focused.
$("select").uniform({focusClass: 'myFocusClass'});
### hoverClass (string)
*Default:* "hover"
Sets the class given to elements when they are currently hovered.
$("select").uniform({hoverClass: 'myHoverClass'});
### idPrefix (string)
*Default:* "uniform"
If useID is set to true, this string is prefixed to element ID’s and attached to the container div of each Uniformed element. If you have a checkbox with the ID of "remember-me" the container div would have the ID "uniform-remember-me".
$("select").uniform({idPrefix: 'container'});
### inputAddTypeAsClass (boolean)
*Default:* true
When true, `<input>` elements will get a class applied that is equal to their "type" attribute.
$("input").uniform({inputAddTypeAsClass: true});
### inputClass (string)
*Default:* "uniform-input"
Applies this class to all input elements when they get uniformed.
$("input").uniform({inputClass: "inputElement"});
### radioClass (string)
*Default:* "radio"
Sets the class given to the wrapper div for radio elements.
$(":radio").uniform({radioClass: 'myRadioClass'});
### resetDefaultHtml (string)
*Default:* "Reset"
This text is what's shown on form reset buttons. It is very similar to submitDefaultHtml.
$("input[type='reset']).uniform({resetDefaultHtml: "Clear"});
### resetSelector (boolean/string)
*Default:* false
This parameter allows you to use a jQuery-style selector to point to a "reset" button in your form if you have one. Use false if you have no "reset" button, or a selector string that points to the reset button if you have one.
$("select").uniform({resetSelector: 'input[type="reset"]'});
### selectAutoWidth (boolean)
*Default:* true
If this option is set to true, Uniform will try to fit the select width to the actual content. When false, it forces the selects to all be the width that was specified in the theme.
When using auto widths, the size of the element is detected, then wrapped by Uniform and expanded to fit the wrapping.
If you want to specify a size of a select element and then have Uniform wrap it appropriately, there will be some difficulty. The size of the element needs to get detected and then will be changed by Uniform. For this to happen, it is suggested you do one of these solutions when you have issues.
* Set a custom inline width for the element (`<select style="width
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
探索未来设计的数字前沿,这份精心设计的“web前端网站源码”无疑是您不可错过的创意宝藏。它巧妙融合了现代网页设计的灵动,打造出一个既展现专业实力又兼顾用户体验的在线空间。 该源码采用了最前沿的HTML5、CSS3及JavaScript技术,确保网站在不同设备上均能完美呈现,从桌面大屏到手机小屏,都能享受流畅无阻的浏览体验。独特的响应式设计,让信息架构清晰明了,无论是产品展示、企业介绍还是新闻动态,都能以最佳布局吸引访客目光。 通过这份源码,您可以轻松搭建起具备高度互动性和用户友好性的网站。它不仅是您企业线上形象的闪亮名片,更是吸引潜在客户、提升品牌影响力的有力工具。立即拥有,开启您的数字化之旅,让每一行代码都成为推动行业进步的力量!
资源推荐
资源详情
资源评论
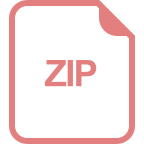
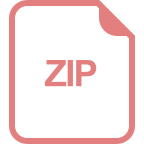
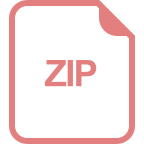
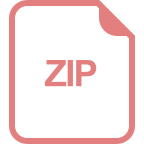
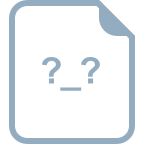
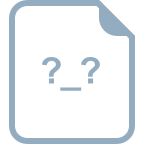
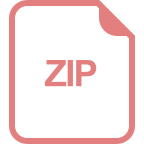
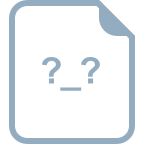
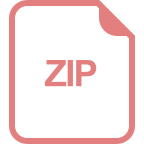
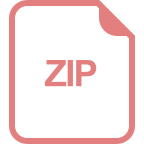
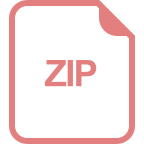
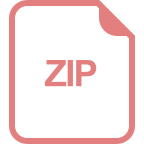
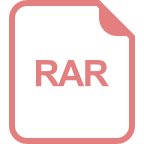
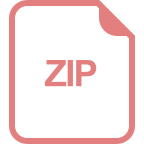
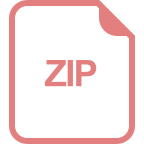
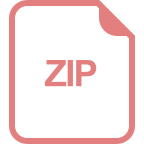
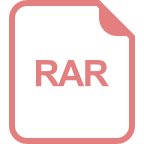
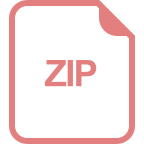
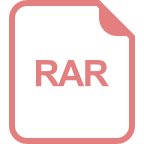
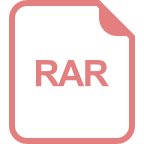
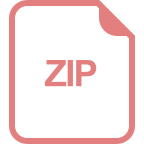
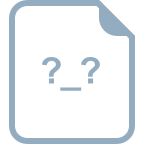
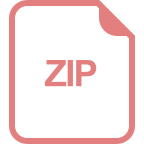
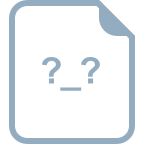
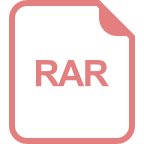
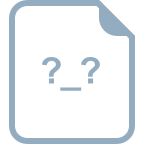
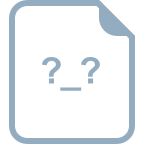
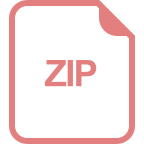
收起资源包目录

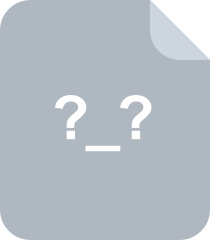
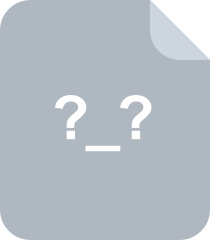
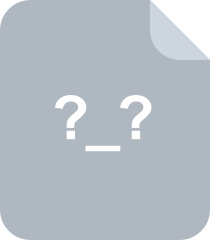
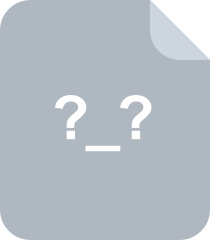
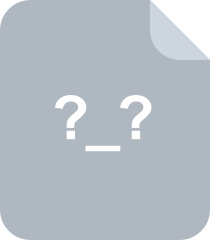
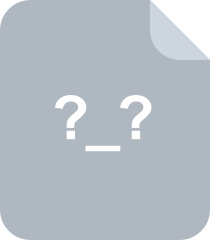
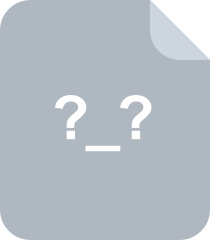
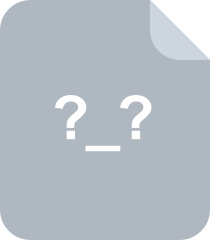
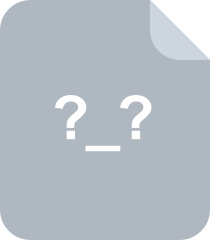
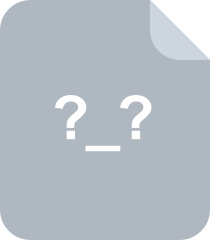
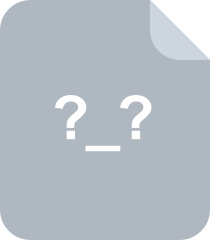
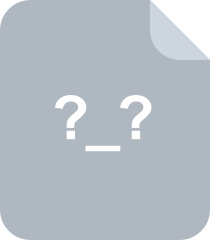
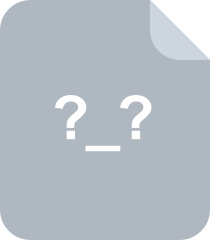
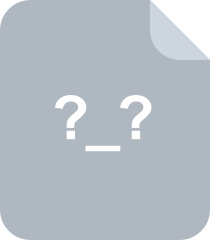
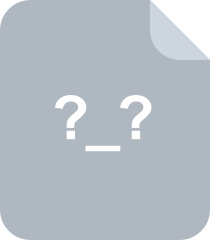
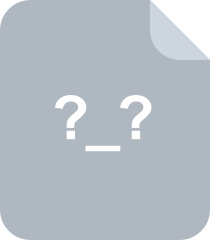
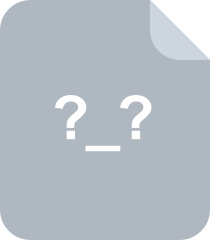
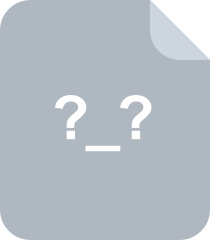
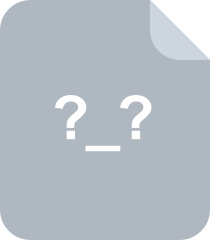
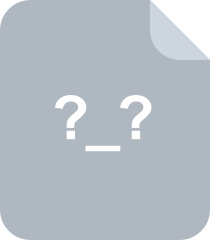
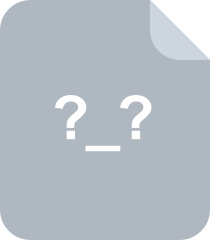
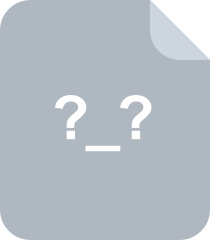
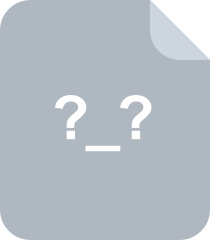
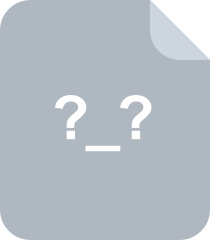
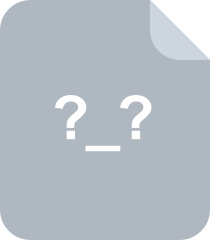
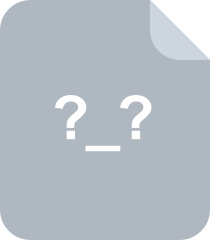
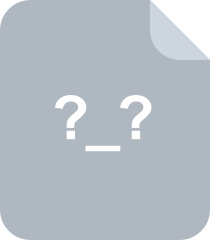
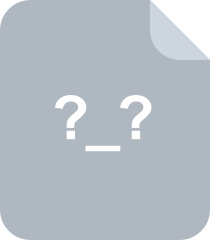
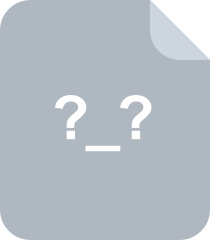
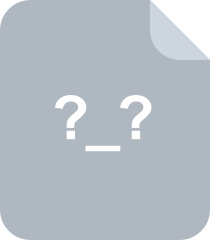
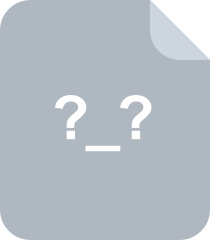
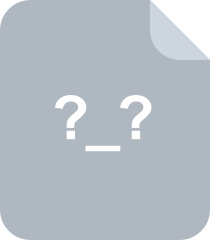
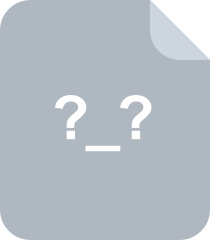
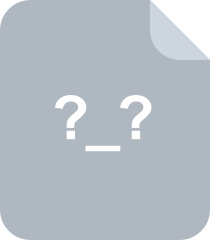
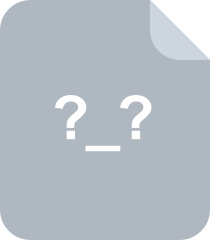
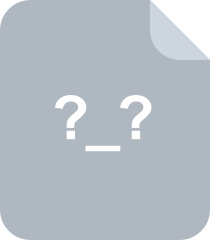
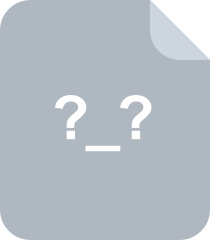
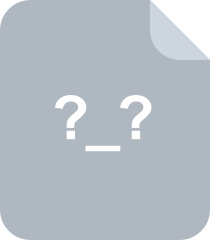
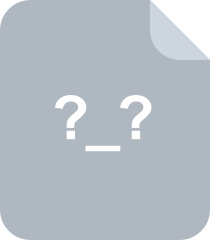
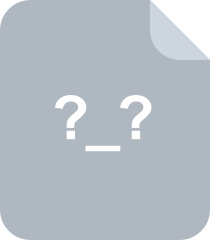
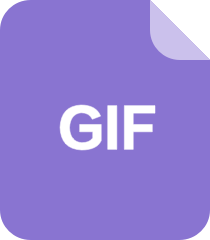
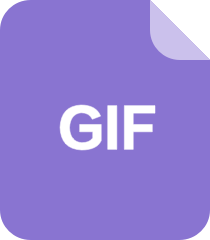
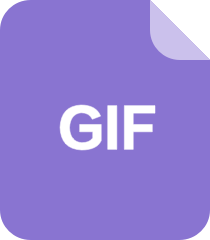
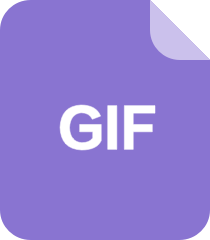
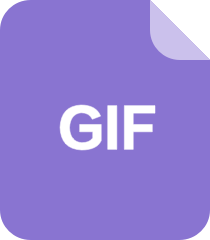
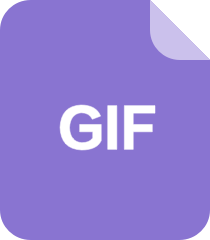
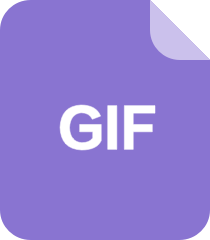
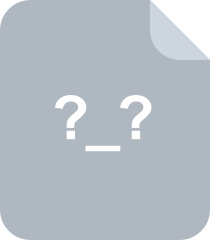
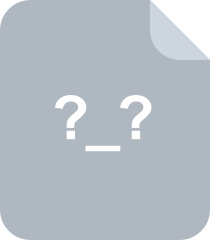
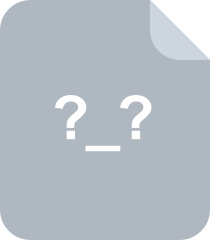
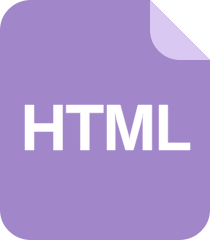
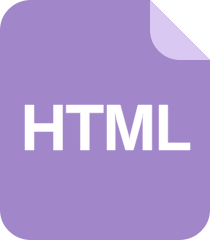
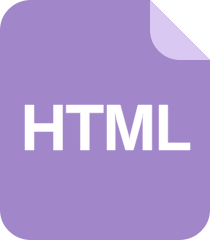
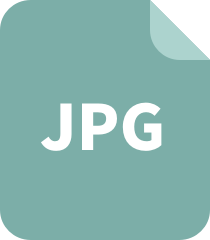
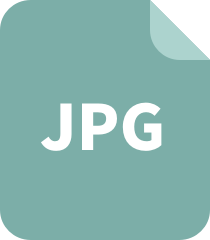
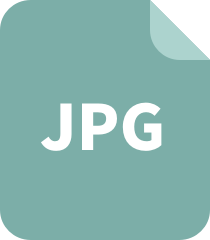
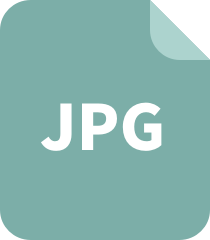
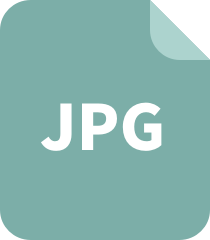
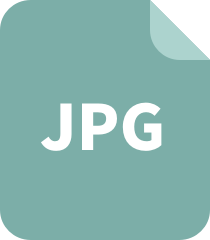
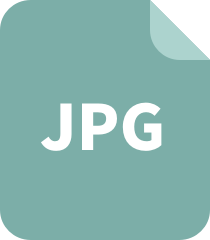
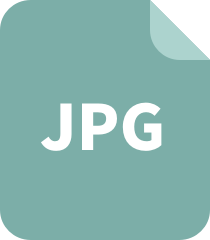
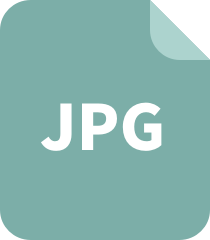
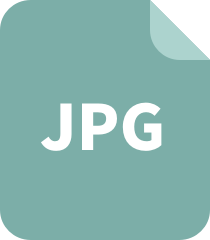
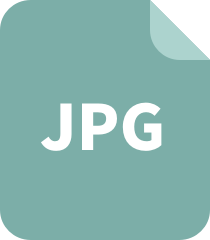
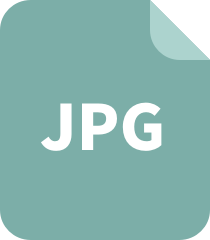
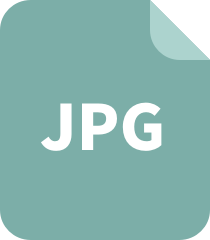
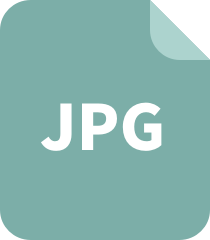
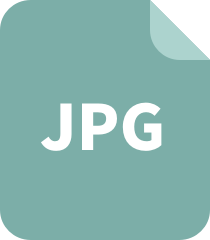
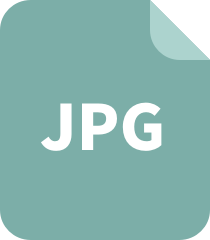
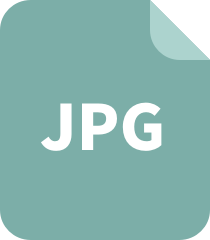
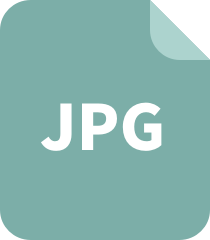
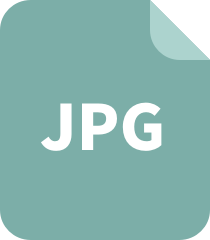
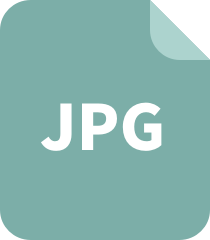
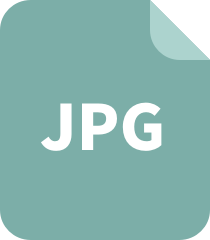
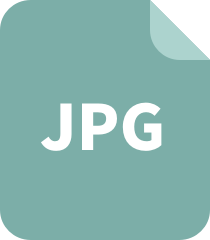
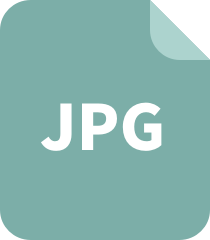
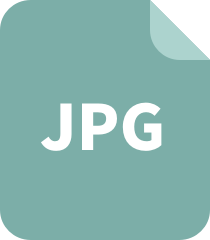
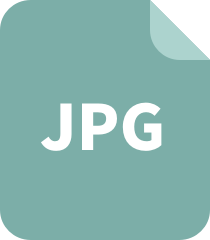
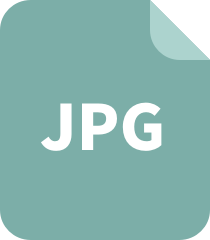
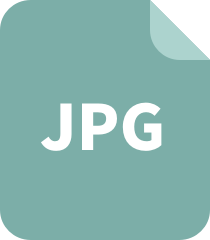
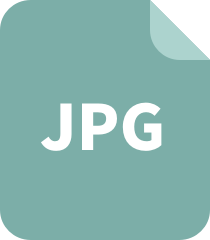
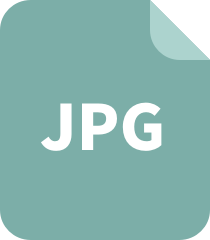
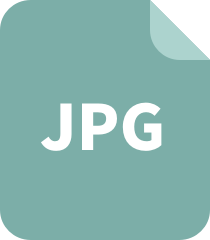
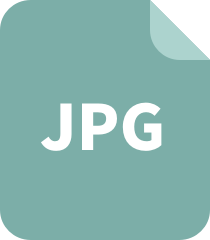
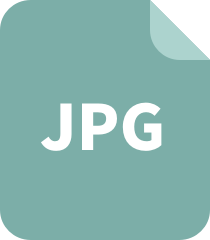
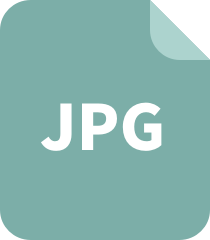
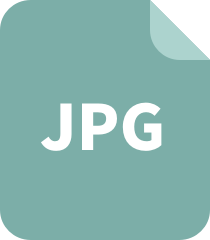
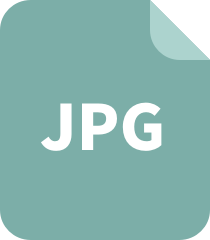
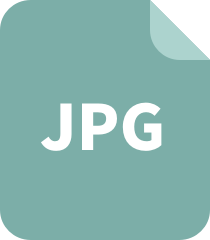
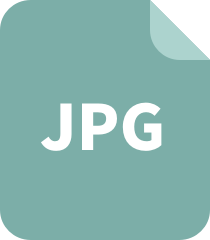
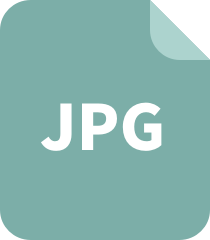
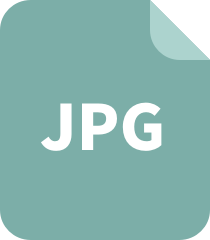
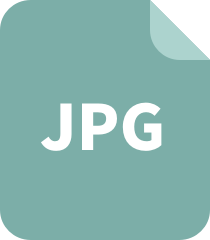
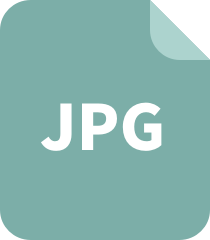
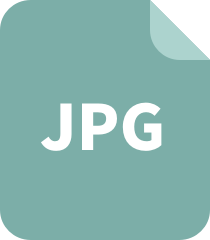
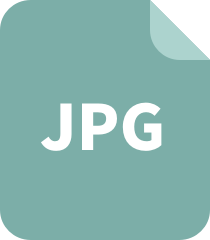
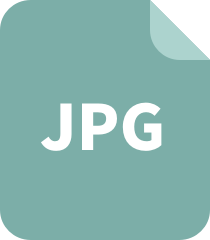
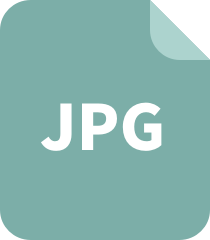
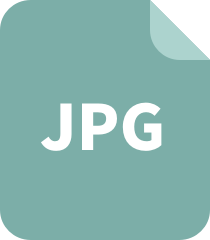
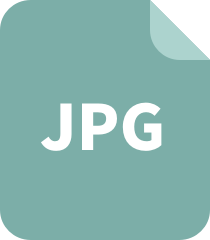
共 409 条
- 1
- 2
- 3
- 4
- 5
资源评论
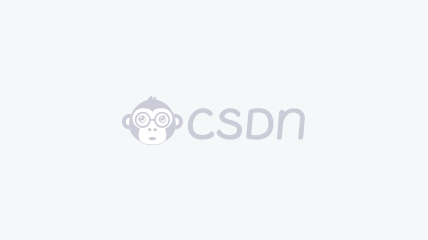

master_chenchengg
- 粉丝: 1w+
- 资源: 2157
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

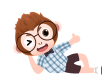
最新资源
- 从XML生成可与Ajax共同使用的JSON中文WORD版最新版本
- silverlight通过WebService连接数据库中文WORD版最新版本
- 使用NetBeans连接SQLserver2008数据库教程中文WORD版最新版本
- XPath实例中文WORD版最新版本
- XPath语法规则中文WORD版最新版本
- XPath入门教程中文WORD版最新版本
- ORACLE数据库管理系统体系结构中文WORD版最新版本
- Sybase数据库安装以及新建数据库中文WORD版最新版本
- tomcat6.0配置oracle数据库连接池中文WORD版最新版本
- hibernate连接oracle数据库中文WORD版最新版本
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


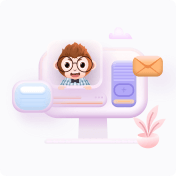
安全验证
文档复制为VIP权益,开通VIP直接复制
