import java.awt.Image;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
import org.opencv.core.Core;
import org.opencv.core.CvType;
import org.opencv.core.Mat;
import org.opencv.core.MatOfRect;
import org.opencv.core.Point;
import org.opencv.core.Rect;
import org.opencv.core.Scalar;
import org.opencv.highgui.Highgui;
import org.opencv.objdetect.CascadeClassifier;
import com.sun.javafx.css.FontFace;
import sun.font.FontScaler;
public class FaceRecognit {
private Mat image;
private CascadeClassifier classifier;
private MatOfRect matOfRect;
public FaceRecognit() {
super();
// TODO Auto-generated constructor stub
classifier = new CascadeClassifier(getClass().getResource("lbpcascade_frontalface.xml").getPath().substring(1));
}
public void writeImage(Mat frame){
image=frame.clone();
matOfRect = new MatOfRect();
classifier.detectMultiScale(image, matOfRect);
for (Rect rect : matOfRect.toArray()) {
//Core.rectangle(image, new Point(rect.x, rect.y), new Point(rect.x + rect.width, rect.y + rect.height), new Scalar(0, 255, 0));
Core.circle(image, new Point(rect.x+rect.width/2, rect.y+rect.height/2), rect.width/2, new Scalar(0, 255, 0));
}
Core.putText(image, "Author:MarksLin@HFUT_XC", new Point(10, 25),2,1, new Scalar(0, 255, 0));
Core.putText(image, "Student ID:2012217166", new Point(10, 60),2,1, new Scalar(0, 255, 0));
String filename = "face.png";
Highgui.imwrite(filename, image);
}
@SuppressWarnings("deprecation")
public Image readImage(){
Image img=null;
try {
File file=new File(System.getProperty("user.dir")+"\\face.png");
img=ImageIO.read(file.toURL());
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return img;
}
}

MarksLin
- 粉丝: 2
- 资源: 6
最新资源
- 计算机视觉领域中YOLOv8实时目标检测算法及其应用
- 折半查找算法实现与分析
- 不同操作系统下Anaconda的安装流程与使用介绍
- 使用界面布局的例子,包括QFormLayout、QGridLayout、QHBoxLayout、QVBoxLayout及一个简易登录界面
- Go语言开发版本 fuxiaohei.me
- 基于 ESP8266 利用 IRext 开源红外库实现万能红外遥控,已对接 MQTT 协议,可轻松接入 HomeAssistant bomb详细文档+全部资料.zip
- 基于 Eclipse Vert.x 和 Apache Ignite 实现的 MQTT 协议服务器详细文档+全部资料.zip
- 基于 go 语言实现的 mqtt 服务器详细文档+全部资料.zip
- 基于 javafx, netty, mqtt 协议实现的聊天客户端,需要联合 mqttx 一起使用详细文档+全部资料.zip
- 基于 MQTT 设计的物联网平台详细文档+全部资料.zip
- 基于 mqttv3.1.1 协议,使用 netty 实现的极简 mqtt 客户端详细文档+全部资料.zip
- 基于 MQTT协议 物联网 智能家居 管理平台详细文档+全部资料.zip
- 基于 Rust、Mqtt 实现 IM 客户端详细文档+全部资料.zip
- 基于Android的MQTT客户端工具详细文档+全部资料.zip
- 基于 STM32 的 MQTT 远程继电器网关详细文档+全部资料.zip
- 基于C#、WPF、Prism、MaterialDesign、HandyControl开发的通讯调试工具,,支持Modbus Rtu调试、Mqtt调试详细文档+全部资料.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


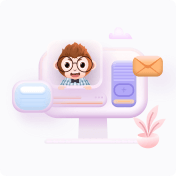
评论5