package com.javaeye.sample.security.Impl;
import java.util.Collection;
import java.util.Iterator;
import java.util.Map;
import javax.servlet.ServletContext;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.security.ConfigAttributeDefinition;
import org.springframework.security.ConfigAttributeEditor;
import org.springframework.security.intercept.web.FilterInvocation;
import org.springframework.security.intercept.web.FilterInvocationDefinitionSource;
import org.springframework.security.util.AntUrlPathMatcher;
import org.springframework.security.util.RegexUrlPathMatcher;
import org.springframework.security.util.UrlMatcher;
/**
* 对资源进行认证
* @author hejianming
*
*/
public class SecureResourceFilterInvocationDefinitionSource implements
FilterInvocationDefinitionSource, InitializingBean {
private UrlMatcher urlMatcher;
private boolean useAntPath = true;
private boolean lowercaseComparisons = true;
/**
* @param useAntPath the useAntPath to set
*/
public void setUseAntPath(boolean useAntPath) {
this.useAntPath = useAntPath;
}
/**
* @param lowercaseComparisons
*/
public void setLowercaseComparisons(boolean lowercaseComparisons) {
this.lowercaseComparisons = lowercaseComparisons;
}
/* (non-Javadoc)
* @see org.springframework.beans.factory.InitializingBean#afterPropertiesSet()
*/
public void afterPropertiesSet() throws Exception {
// default url matcher will be RegexUrlPathMatcher
this.urlMatcher = new RegexUrlPathMatcher();
if (useAntPath) { // change the implementation if required
this.urlMatcher = new AntUrlPathMatcher();
}
// Only change from the defaults if the attribute has been set
if ("true".equals(lowercaseComparisons)) {
if (!this.useAntPath) {
((RegexUrlPathMatcher) this.urlMatcher).setRequiresLowerCaseUrl(true);
}
} else if ("false".equals(lowercaseComparisons)) {
if (this.useAntPath) {
((AntUrlPathMatcher) this.urlMatcher).setRequiresLowerCaseUrl(false);
}
}
}
/* (non-Javadoc)
* @see org.springframework.security.intercept.ObjectDefinitionSource#getAttributes(java.lang.Object)
*/
public ConfigAttributeDefinition getAttributes(Object filter) throws IllegalArgumentException {
FilterInvocation filterInvocation = (FilterInvocation) filter;
String requestURI = filterInvocation.getRequestUrl();
Map<String, String> urlAuthorities = this.getUrlAuthorities(filterInvocation);
String grantedAuthorities = null;
if(urlAuthorities!=null){
for(Iterator<Map.Entry<String, String>> iter = urlAuthorities.entrySet().iterator(); iter.hasNext();) {
Map.Entry<String, String> entry = iter.next();
String url = entry.getKey();
if(urlMatcher.pathMatchesUrl(url, requestURI)) {
grantedAuthorities = entry.getValue();
break;
}
}
}
if(grantedAuthorities != null) {
ConfigAttributeEditor configAttrEditor = new ConfigAttributeEditor();
configAttrEditor.setAsText(grantedAuthorities);
return (ConfigAttributeDefinition) configAttrEditor.getValue();
}
return null;
}
/* (non-Javadoc)
* @see org.springframework.security.intercept.ObjectDefinitionSource#getConfigAttributeDefinitions()
*/
@SuppressWarnings("unchecked")
public Collection getConfigAttributeDefinitions() {
return null;
}
/* (non-Javadoc)
* @see org.springframework.security.intercept.ObjectDefinitionSource#supports(java.lang.Class)
*/
@SuppressWarnings("unchecked")
public boolean supports(Class clazz) {
return true;
}
/**
*
* @param filterInvocation
* @return
*/
@SuppressWarnings("unchecked")
private Map<String, String> getUrlAuthorities(FilterInvocation filterInvocation) {
ServletContext servletContext = filterInvocation.getHttpRequest().getSession().getServletContext();
return (Map<String, String>)servletContext.getAttribute("urlAuthorities");
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
Spring Security 2.0 安全框架实例v1.1
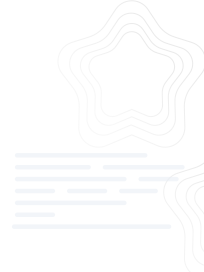
共60个文件
java:12个
class:12个
properties:10个


温馨提示
Spring Security 为基于J2EE 的企业应用软件提供了一套全面的安全解决方案。正如你在本手册中看到的 那样,我们尝试为您提供一套好用,高可配置的安全系统。 安全问题是一个不断变化的目标,更重要的是寻求一种全面的,系统化的解决方案。 在安全领域我们建议 你采取“分层安全”,这样让每一层确保本身尽可能的安全,并为其他层提供额外的安全保障。 每层自身越是“紧 密”,你的程序就会越鲁棒越安全。
资源推荐
资源详情
资源评论
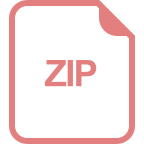
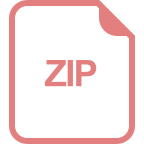
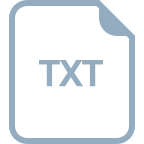
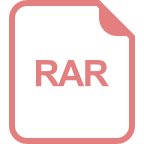
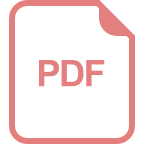
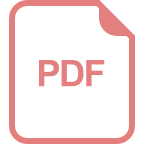
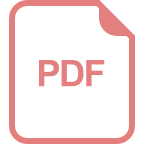
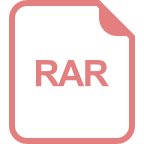
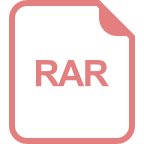
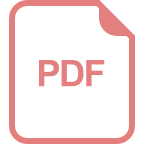
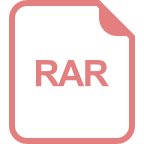
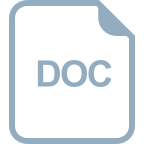
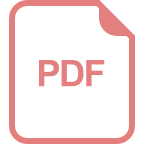
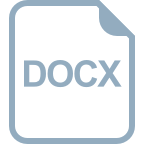
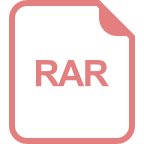
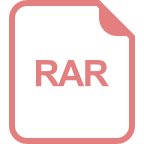
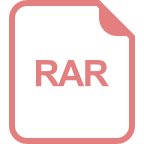
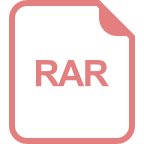
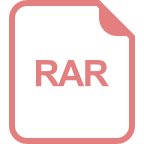
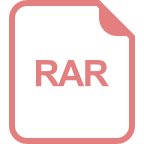
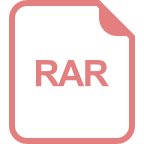
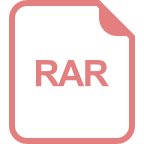
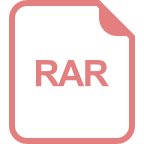
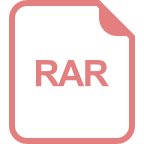
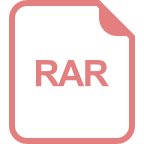
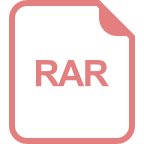
收起资源包目录


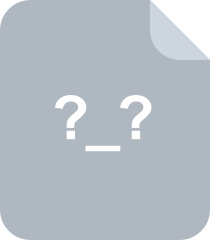
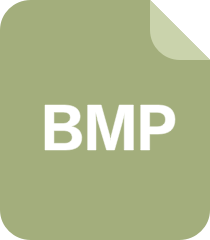

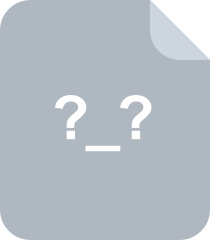
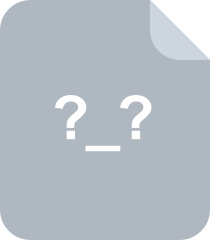
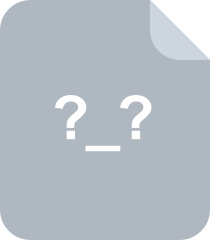
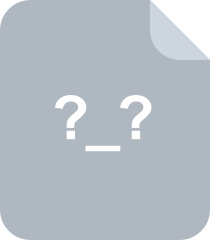
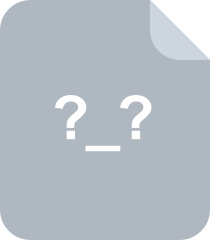
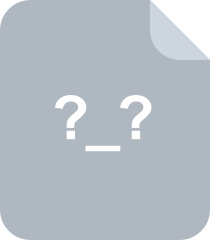
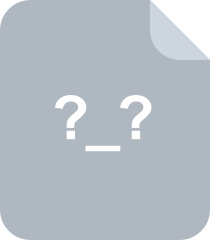

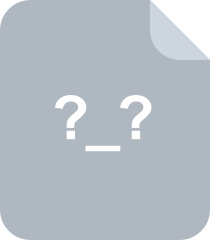
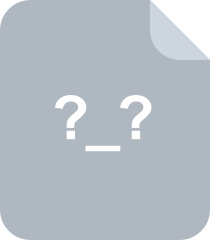
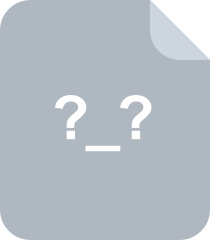






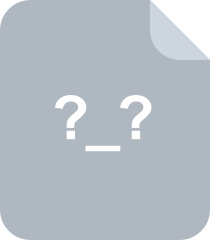

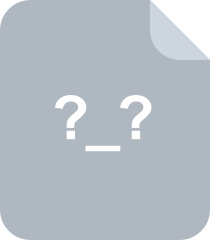
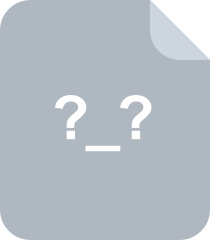


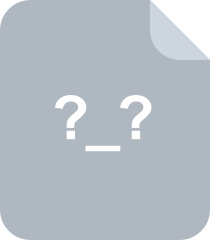
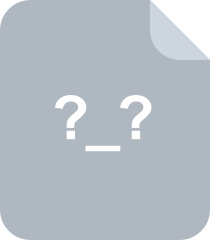
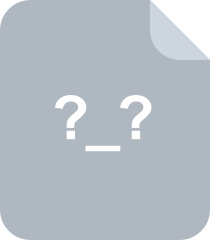

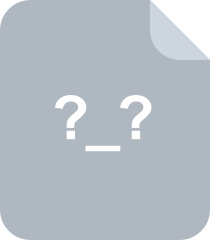

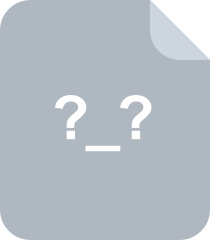
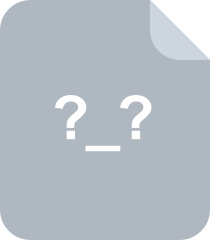
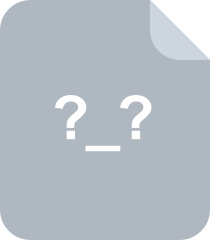


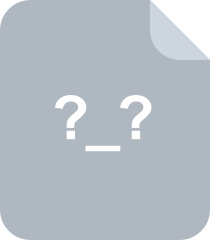

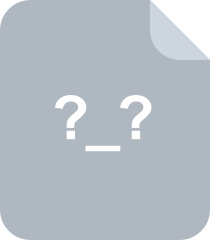
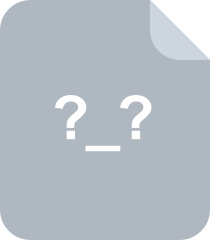
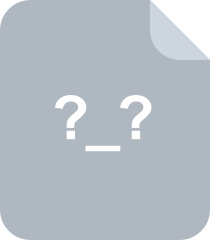
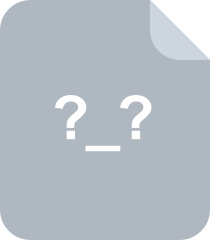
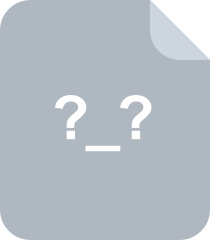
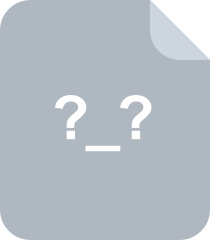
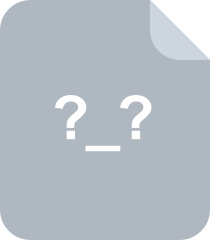


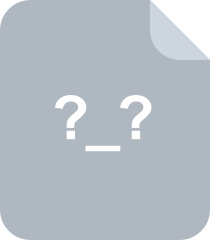

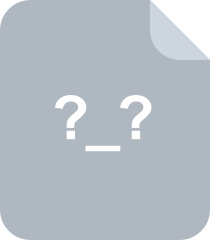


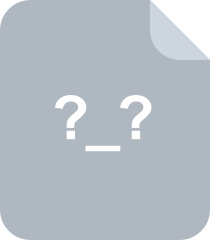
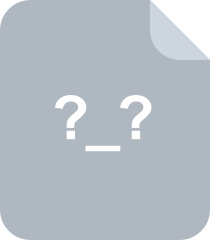
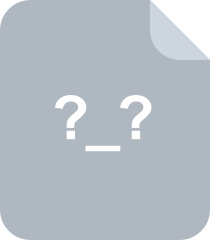






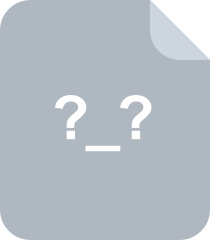

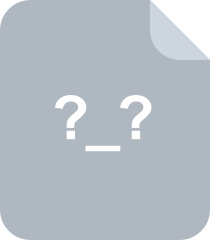
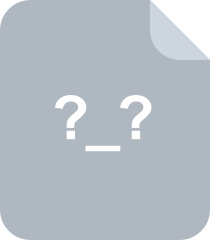


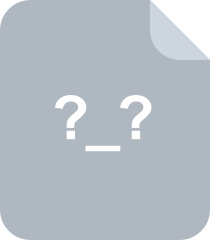
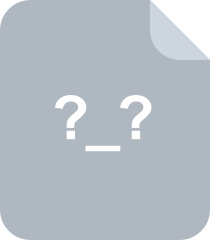
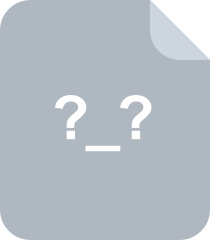

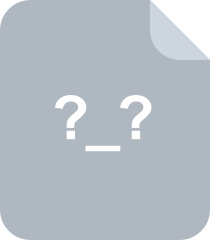

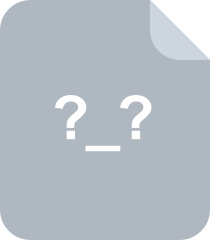
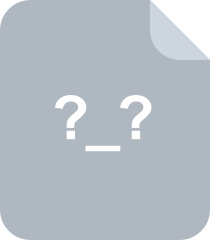
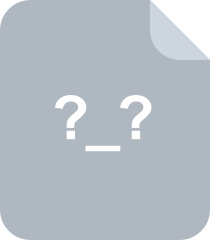


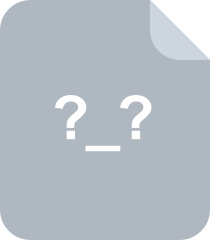

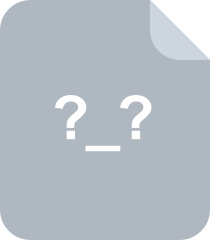
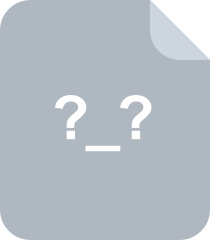
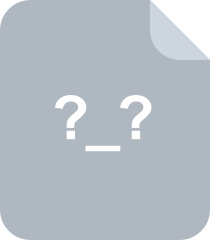
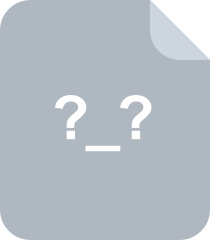
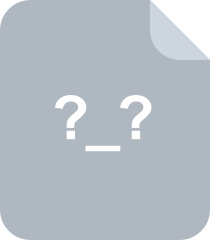
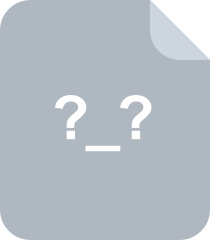
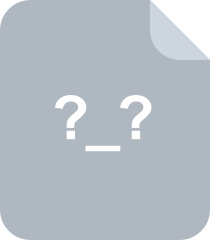
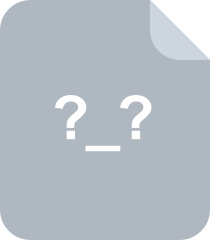

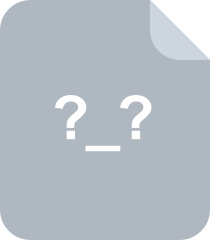
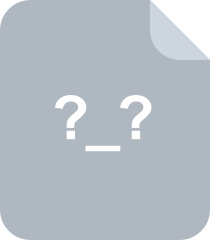

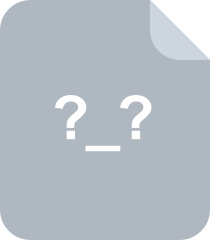

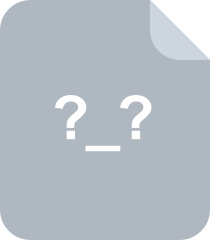

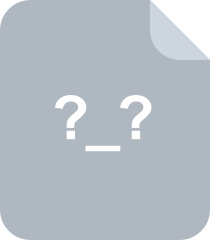
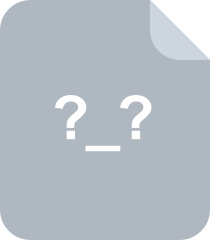
共 60 条
- 1
资源评论
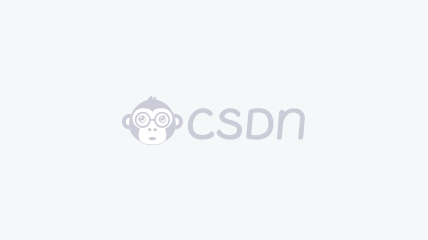
- 紫苏水釉2014-01-14不建议下载,内容根本不行

mark_2000
- 粉丝: 3
- 资源: 21
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

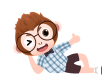
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


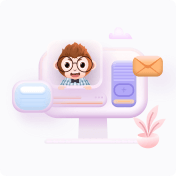
安全验证
文档复制为VIP权益,开通VIP直接复制
