#define _GNU_SOURCE
#include <errno.h>
#include <fcntl.h>
#include <stdbool.h>
#include <stdint.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/mman.h>
#include <time.h>
#include <unistd.h>
#include <xf86drm.h>
#include <xf86drmMode.h>
#include <fcntl.h>
#include <stdio.h>
#include <sys/ioctl.h>
int disableCloseScreen()//关闭电源管理10s关闭显示器功能
{
int f0;
f0 = open("/dev/tty0", O_RDWR);
write(f0, "\033[9;0]", 8);
close(f0);
return 0;
}
struct buffer_object {
uint32_t width;
uint32_t height;
uint32_t pitch;
uint32_t handle;
uint32_t size;
uint8_t *vaddr;
uint32_t fb_id;
};
struct buffer_object buf;
static int modeset_create_fb(int fd, struct buffer_object *bo)
{
struct drm_mode_create_dumb create = {};
struct drm_mode_map_dumb map = {};
/* create a dumb-buffer, the pixel format is XRGB888 */
create.width = bo->width;
create.height = bo->height;
create.bpp = 32;
drmIoctl(fd, DRM_IOCTL_MODE_CREATE_DUMB, &create);
/* bind the dumb-buffer to an FB object */
bo->pitch = create.pitch;
bo->size = create.size;
bo->handle = create.handle;
drmModeAddFB(fd, bo->width, bo->height, 24, 32, bo->pitch,
bo->handle, &bo->fb_id);
/* map the dumb-buffer to userspace */
map.handle = create.handle;
drmIoctl(fd, DRM_IOCTL_MODE_MAP_DUMB, &map);
bo->vaddr = mmap(0, create.size, PROT_READ | PROT_WRITE,
MAP_SHARED, fd, map.offset);
/* initialize the dumb-buffer with white-color */
memset(bo->vaddr, 0xff, bo->size);
return 0;
}
static void modeset_destroy_fb(int fd, struct buffer_object *bo)
{
struct drm_mode_destroy_dumb destroy = {};
drmModeRmFB(fd, bo->fb_id);
munmap(bo->vaddr, bo->size);
destroy.handle = bo->handle;
drmIoctl(fd, DRM_IOCTL_MODE_DESTROY_DUMB, &destroy);
}
unsigned char buftmp1[1920*1200*3];
unsigned char buftmp2[1920*1200*4];
unsigned int bufosd[10][16*25];
void readosdcnt()
{
int i=0,k=0,j=0;
unsigned char b;
char bmpstr[10][20] = {
"bmp09/s0.bmp",
"bmp09/s1.bmp",
"bmp09/s2.bmp",
"bmp09/s3.bmp",
"bmp09/s4.bmp",
"bmp09/s5.bmp",
"bmp09/s6.bmp",
"bmp09/s7.bmp",
"bmp09/s8.bmp",
"bmp09/s9.bmp"
};
FILE *fp;
unsigned char tmp[16*25*3];
memset(bufosd,0,16*25*40);
for(i=0;i<10;i++)
{
fp = fopen(bmpstr[i],"rb+");
fread(tmp,54,1,fp);
fread(tmp,16*25*3,1,fp);
close(fp);
//
for(k=0;k<25;k++)
{
for(j=0;j<16;j++)
{
b = tmp[((24-k)*16+j)*3+1];
// if(b>0x80)
bufosd[i][k*16+j] = b;
// else
// bufosd[i][k*16+j] = 0;
}
}
}
}
//static int osdcnt=0;
static int osdcnt1=0;
void showBmp(char *filename,int w,int h)
{//填充bmp到framebuf
int x,y,cnt[5],osdcnt;
FILE *fp;
fp = fopen(filename,"rb");
fread(buftmp1,54,1,fp);
fread(buftmp1,w*h*3,1,fp);
fclose(fp);
osdcnt = osdcnt1;
for(y=0;y<h;y++)
{
for(x=0;x<w;x++)
{
buftmp2[ (y*w+x)*4 +0 ] =buftmp1[((h-y-1)*w+x)*3 +0];
buftmp2[ (y*w+x)*4 +1 ] =buftmp1[((h-y-1)*w+x)*3 +1];
buftmp2[ (y*w+x)*4 +2 ] =buftmp1[((h-y-1)*w+x)*3 +2];
}
}
printf("\nshowbmp:%dx%d ,%d,%d\n",w,h,buf.width,osdcnt);
//set osdcnt
cnt[0] = osdcnt%10; osdcnt = osdcnt/10;
cnt[1] = osdcnt%10; osdcnt = osdcnt/10;
cnt[2] = osdcnt%10; osdcnt = osdcnt/10;
cnt[3] = osdcnt%10; osdcnt = osdcnt/10;
cnt[4] = osdcnt%10;
for(y=0;y<25;y++)
{
for(x=0;x<16*5;x++)
{
//unsigned int bufosd[10][16*25];
buftmp2[ (y*w+x)*4 +0 ] =bufosd[cnt[4-x/16]][y*16+x%16];
buftmp2[ (y*w+x)*4 +1 ] =bufosd[cnt[4-x/16]][y*16+x%16];
buftmp2[ (y*w+x)*4 +2 ] =bufosd[cnt[4-x/16]][y*16+x%16];
}
}
//
memcpy(buf.vaddr,buftmp2,w*h*4);
}
void show4Screen(int w,int h)
{//四分屏
int x,y;
for(y=0;y<h;y++)
{
for(x=0;x<w;x++)
{
if(y<h/2)
{
//
if(x<w/2)
{
*(unsigned int *)(buf.vaddr+ (y*w+x)*4 ) = 0x00FFFFFF;
}
else
{
*(unsigned int *)(buf.vaddr+ (y*w+x)*4 ) = 0x000000FF;//b
}
}
else
{
//
if(x<w/2)
{
*(unsigned int *)(buf.vaddr+ (y*w+x)*4 ) = 0x0000FF00;//g
}
else
{
*(unsigned int *)(buf.vaddr+ (y*w+x)*4 ) = 0x00ff0000;//r
}
}
}
}
}
#include <unistd.h>
#include <stdio.h>
#include <dirent.h>
#include <string.h>
#include <sys/stat.h>
#include <stdlib.h>
char currBmpFile[100][128];
char dir[7][20] = {
"bmp1920/",
"bmp1600/",
"bmp1600/",
"bmp1400/",
"bmp1280/",
"bmp1300/",//define
"bmp1024/"
};
int PrintDir(int index, int depth)
{//遍历bmp目录下的bmp文件
DIR *dp;
int curcnt=0;
struct dirent *entry;
struct stat statbuf;
memset(currBmpFile,0,strlen(currBmpFile));
if ((dp = opendir(dir[index])) == NULL)
{
fprintf(stderr, "Cannot open directory: %s\n", dir);
return;
}
chdir(dir);
while ((entry = readdir(dp)) != NULL)
{
lstat(entry->d_name, &statbuf);
if (S_ISDIR(statbuf.st_mode))
{
if (strcmp(".", entry->d_name) == 0 || strcmp("..", entry->d_name) == 0)
continue;
}
else
{
printf("%s\n",entry->d_name);
strcpy(currBmpFile[curcnt++],entry->d_name);
}
}
chdir("..");
closedir(dp);
return curcnt;
}
unsigned int timing[][10]=
{
{800,840,968,1056,600,601,605,628,40000,60},
{1024,1048,1184,1344,768,771,777,628,65000,60},
{1600,1664,1856,2160,1200,1201,1204,1250,162000,60},
{1680,1728,1760,1840,1050,1053,1059,1080,119000,60},
};
#define S_1920X1080 0
#define S_1600X1200 1
#define S_1600X128M 2
#define S_1400X1050 3
#define S_1280X1024 4
#define S_1300X768 5
#define S_1024X768 6
uint32_t tmIndex[7][2]={
{1920,1080},
{1600,1200},
{1600,1200},//128M
{1400,1050},
{1280,1024},
{1300,1000},//define
{1024,768}
};
int dispMOde=6;//S_1024X768;//当前分辨率设置
//int dispMOde=S_1400X1050;//当前分辨率设置
//int dispMOde=S_1024X768;//当前分辨率设置
int picMode()
{
int fd=0,i,ret,index=0;
drmModeConnector *conn;
drmModeRes *res;
uint32_t conn_id;
uint32_t crtc_id;
uint32_t plane_id;
drmModePlaneRes *plane_res;
fd = open("/dev/dri/card0", O_RDWR | O_CLOEXEC);
if (fd < 0)
{
ret = -errno;
printf("open err\n");
return ret;
}
//检查DRM的能力
uint64_t has_dumb;
if (drmGetCap(fd, DRM_CAP_DUMB_BUFFER, &has_dumb) < 0 || !has_dumb)
{
printf("drm device '%s' does not support dumb buffers\n");
close(fd);
return -EOPNOTSUPP;
}
//检索Resource
res = drmModeGetResources(fd);
if(!res)
{
printf("cannot retrieve DRM resources\n");
return 0;
}
crtc_id = res->crtcs[0];
conn_id = res->connectors[0];
//add 2021-1015
drmSetClientCap(fd, DRM_CLIENT_CAP_UNIVERSAL_PLANES, 1);
plane_res = drmModeGetPlaneResources(fd);
plane_id = plane_res->planes[2];
//
//printf("aaa \n");
conn = drmModeGetConnector(fd, conn_id);
if(!conn)
{
printf("cannot retrieve DRM connector\n");
return 0;
}
buf.width = conn->modes[dispMOde+2].hdisplay;
buf.height = conn->modes[dispMOde+2].vdisplay;
//printf("bbb \n");
//hdisplay, hsync_start, hsync_end, htotal, vdisplay, vsync_start, vsync_end, vtotal,clock, vrefresh
// 800x600 60 800 840 968 1056 600 601 605 628
#if 1 //获取Connector
printf("connID:%d crtcID:%d count_modes:%d %d x %d\n",conn_id,crtc_id,co

毛毛虫的爹
- 粉丝: 2525
- 资源: 81
最新资源
- 使用 GSD (DirectX Hook Library) 绘制十字线.zip
- 使用 Graphic, DirectX, OpenGL 进行全屏拍摄.zip
- jd-gui-windows-1.6.6 java反编译工具
- 经典分子模拟教程 《The art of molucular dynamics simulation》作者: D.C. Rapaport 出版社:Cambridge Universi
- InputTip - 根据输入法中英文状态切换鼠标样式的小工具
- 使用 Dx3D9 Sprite 对象的 DirectX 2D 引擎.zip
- C code for "The art of molecular dynamics simulation"
- 国外版剪映 特效无限用,无需登录
- 使用 DX12 编写的基于物理的渲染器,具有基于图像的照明、经典的延迟和平铺照明方法.zip
- windows命令行curl命令工具
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


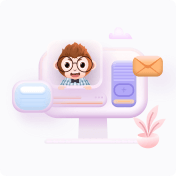