package com.nenusoftware.onlineexam.service.paperdetail.impl;
import com.alibaba.fastjson.JSONArray;
import com.nenusoftware.onlineexam.entity.answer.Answer;
import com.nenusoftware.onlineexam.entity.paper.Paper;
import com.nenusoftware.onlineexam.entity.paperdetail.PaperDetail;
import com.nenusoftware.onlineexam.entity.score.Score;
import com.nenusoftware.onlineexam.entity.wrong.Wrong;
import com.nenusoftware.onlineexam.mapper.paperdetail.PaperDetailMapper;
import com.nenusoftware.onlineexam.service.paper.PaperService;
import com.nenusoftware.onlineexam.service.paperdetail.PaperDetailService;
import com.nenusoftware.onlineexam.service.score.ScoreService;
import com.nenusoftware.onlineexam.service.wrong.WrongService;
import org.apache.ibatis.annotations.Param;
import org.springframework.stereotype.Service;
import javax.annotation.Resource;
import java.util.Collections;
import java.util.List;
/**
* @Author:kongyy
* @Description: PaperDetailService的底层实现方法
* @Date: 10:33 2020/6/16
*/
@Service
public class PaperDetailServiceImpl implements PaperDetailService {
@Resource
PaperDetailMapper paperDetailMapper;
@Resource
PaperService paperService;
@Resource
ScoreService scoreService;
@Resource
WrongService wrongService;
/**
* 列出所有试卷详细信息
* @return 返回List形式的试卷详细信息
* @throws Exception 抛出错误类型
*/
@Override
public List<PaperDetail> listAllPaperDetail() throws Exception{
List<PaperDetail> paperDetailList = Collections.emptyList();
paperDetailList = paperDetailMapper.listAllPaperDetail();
for (PaperDetail detail : paperDetailList) {
PaperDetail paperDetail = new PaperDetail();
paperDetail = detail;
if("简答题".equals(paperDetail.getExerciseType())){
String str, str1, str2, str3;
str1 = paperDetail.getAnswer();
str2 = paperDetail.getAnswer2();
str3 = paperDetail.getAnswer3();
str = "得分点为:" + str1 + " " + str2 + " " + str3;
paperDetail.setAnswer(str);
}
}
return paperDetailList;
}
/**
* 根据试卷编号列出试卷详细信息
* @param paperId 试卷编号
* @return 返回List形式的试卷详细信息
* @throws Exception 抛出错误类型
*/
@Override
public List<PaperDetail> listPaperDetailByPaperId(int paperId) throws Exception{
List<PaperDetail> paperDetailList = Collections.emptyList();
paperDetailList = paperDetailMapper.listPaperDetailByPaperId(paperId);
for (PaperDetail detail : paperDetailList) {
PaperDetail paperDetail = new PaperDetail();
paperDetail = detail;
String str, str1, str2, str3;
str1 = paperDetail.getAnswer();
str2 = paperDetail.getAnswer2();
str3 = paperDetail.getAnswer3();
str = "关键字为:" + str1 + " " + str2 + " " + str3;
paperDetail.setAnswer(str);
}
return paperDetailList;
}
/**
* 增加试卷详细信息
* @param paperDetail 试卷详细信息实体
* @throws Exception 抛出错误类型
*/
@Override
public void addPaperDetail(PaperDetail paperDetail) throws Exception {
paperDetailMapper.addPaperDetail(paperDetail);
}
/**
* 删除试卷详细信息
* @param paperDetailId 试卷详细信息编号
* @return 删除成功返回true,删除失败返回false
* @throws Exception 抛出错误类型
*/
@Override
public boolean deletePaperDetail(int paperDetailId) throws Exception{
return paperDetailMapper.deletePaperDetail(paperDetailId);
}
/**
* 修改试卷详细信息
* @param paperDetail 试卷详细信息实体
* @throws Exception 抛出错误类型
*/
@Override
public void updatePaperDetail(PaperDetail paperDetail) throws Exception{
paperDetailMapper.updatePaperDetail(paperDetail);
}
/**
* 模糊查询(查询试卷题目的内容或类型)
* @param keyWord 输入查询的关键字
* @return 返回List形式的试卷详细信息
* @throws Exception 抛出错误类型
*/
@Override
public List<PaperDetail> queryPaperDetail(String keyWord) throws Exception{
List<PaperDetail> paperDetaillist = Collections.emptyList();
keyWord = "%" + keyWord + "%";
paperDetaillist = paperDetailMapper.queryPaperDetail(keyWord);
return paperDetaillist;
}
/**
* 根据题目类型列出试卷详细信息
* @param exerciseType 题目类型
* @return 返回List形式的试卷详细信息
* @throws Exception 抛出错误类型
*/
@Override
public List<PaperDetail> queryExerciseByTypes(String exerciseType) throws Exception{
List<PaperDetail> paperDetailList = Collections.emptyList();
paperDetailList = paperDetailMapper.queryExerciseByTypes(exerciseType);
for (PaperDetail detail : paperDetailList) {
PaperDetail paperDetail = new PaperDetail();
paperDetail = detail;
if("简答题".equals(paperDetail.getExerciseType())){
String str, str1, str2, str3;
str1 = paperDetail.getAnswer();
str2 = paperDetail.getAnswer2();
str3 = paperDetail.getAnswer3();
str = "得分点为:" + str1 + " " + str2 + " " + str3;
paperDetail.setAnswer(str);
}
}
return paperDetailList;
}
/**
* 根据试卷Id查询题目选项
* @param paperDetailId 试卷详细信息编号
* @return 返回List形式的试卷详细信息
* @throws Exception 抛出错误类型
*/
@Override
public List<PaperDetail> queryExerciseItemsById(int paperDetailId) throws Exception{
List<PaperDetail> paperDetailItemsList = Collections.emptyList();
paperDetailItemsList = paperDetailMapper.queryExerciseItemsById(paperDetailId);
return paperDetailItemsList;
}
/**
* 根据试题内容获取题目编号
* @param content 试题内容
* @return 题目编号
* @throws Exception 抛出错误类型
*/
@Override
public PaperDetail queryIdByContent(@Param("content") String content) throws Exception{
return paperDetailMapper.queryIdByContent(content);
}
/**
* 根据题目的id来查找题目
* @param paperDetailId 题目id
* @return 返回查找到的PaperDetail对象
* @throws Exception 抛出错误类型
*/
@Override
public PaperDetail queryQuestion(int paperDetailId) throws Exception{
List<PaperDetail> paperDetailItemsList = Collections.emptyList();
PaperDetail paperDetail = new PaperDetail();
paperDetailItemsList = paperDetailMapper.queryQuestion(paperDetailId);
paperDetail = paperDetailItemsList.get(0);
return paperDetail;
}
/**
*根据学生做题信息,计算学生得了多少分
* @param jsonArray 学生做题信息,其中包含两项信息,学生答案:answer和这道题目的id:paperDetailId
* @param userId 学生的id
* @return 返回学生得的分数
* @throws Exception 抛出错误类型
*/
@Override
public int judgeQuestion(JSONArray jsonArray, int userId) throws Exception{
int result = 0;
String paperIdStr = jsonArray.getJSONObject(0).getString("paperId");
int paperId = Integer.parseInt(paperIdStr);
try{
for(int i=0;i<jsonArray.size();i++){
Answer answer = new Answer();
PaperDetail paperDetail = new PaperDetail();
String paperDetailIdStr = jsonArray.getJSONObject(i).getString("paperDetailId");
String solution = json
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
java毕业设计SpringBoot+Vue前后端分离的在线考试系统源码+数据库+文档说明(高分项目),本资源中的源码都是经过本地编译过可运行的,评审分达到98分,资源项目的难度比较适中,内容都是经过助教老师审定过的能够满足学习、毕业设计、期末大作业和课程设计使用需求,如果有需要的话可以放心下载使用。 java毕业设计SpringBoot+Vue前后端分离的在线考试系统源码+数据库+文档说明(高分项目)java毕业设计SpringBoot+Vue前后端分离的在线考试系统源码+数据库+文档说明(高分项目)java毕业设计SpringBoot+Vue前后端分离的在线考试系统源码+数据库+文档说明(高分项目)java毕业设计SpringBoot+Vue前后端分离的在线考试系统源码+数据库+文档说明(高分项目)java毕业设计SpringBoot+Vue前后端分离的在线考试系统源码+数据库+文档说明(高分项目)java毕业设计本资源中的源码都是经过本地编译过可运行的,评审分达到98分,资源项目的难度比较适中,内容都是经过助教老师审定过的能够满足学习、毕业设计、期末大作业和课程设计使用需求。
资源推荐
资源详情
资源评论
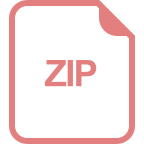
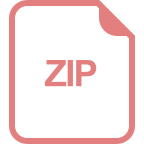
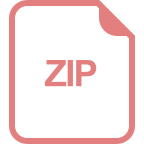
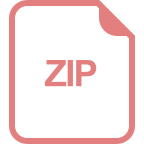
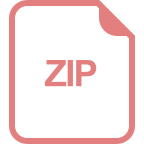
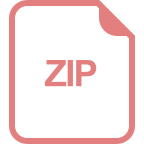
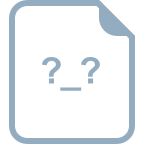
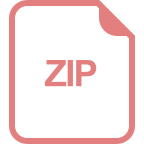
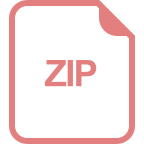
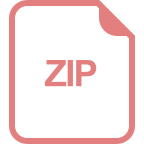
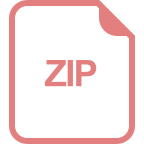
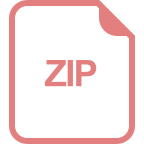
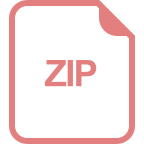
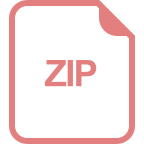
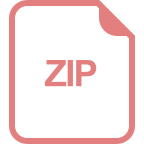
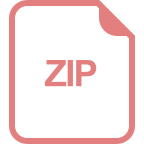
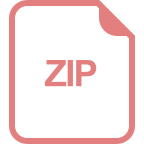
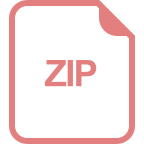
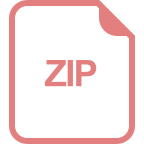
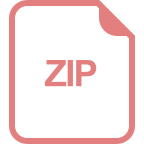
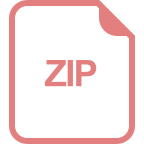
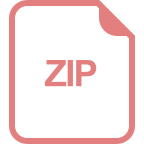
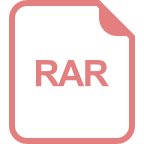
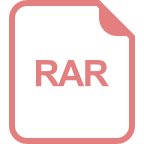
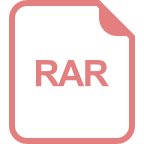
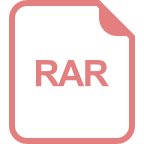
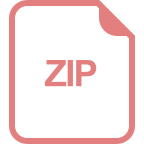
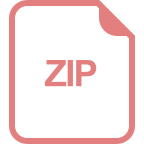
收起资源包目录

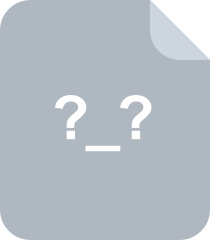
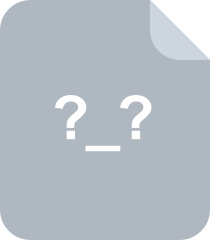
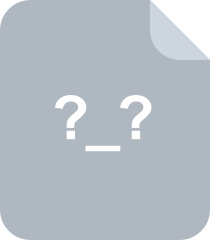
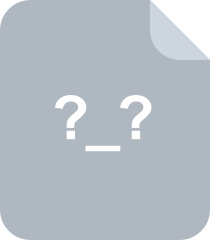
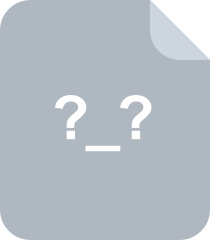
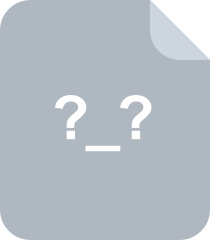
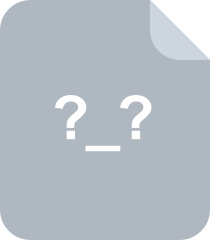
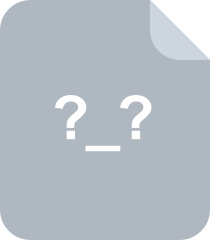
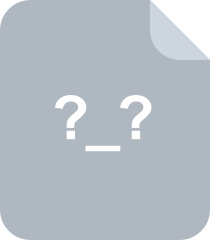
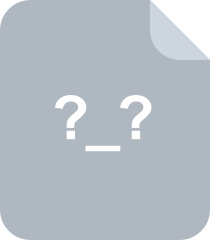
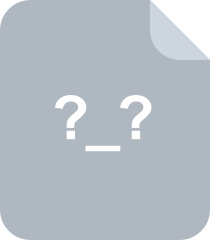
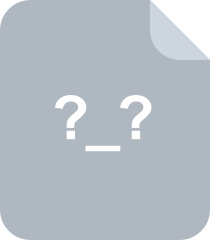
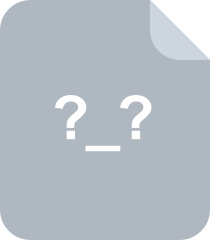
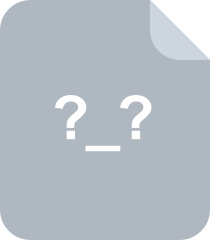
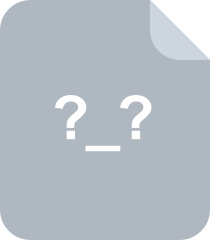
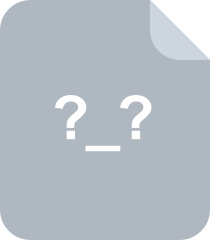
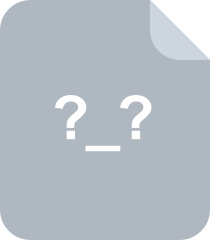
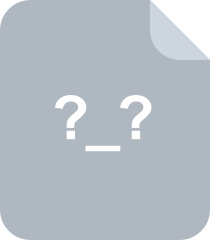
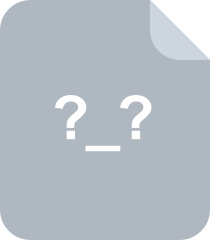
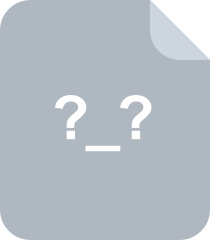
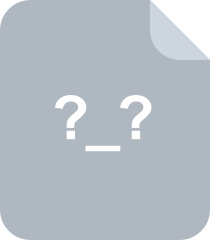
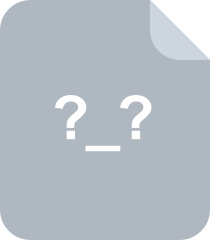
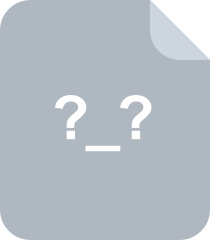
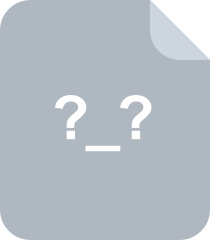
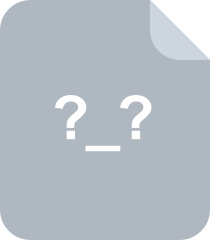
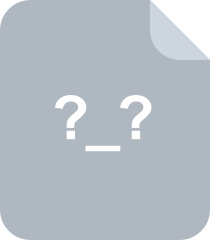
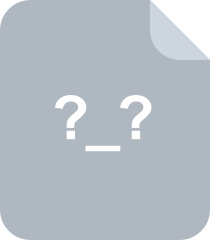
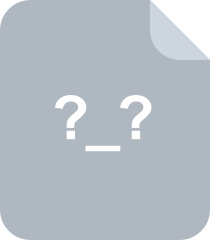
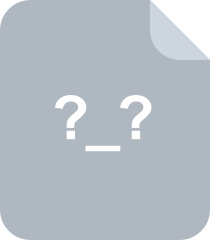
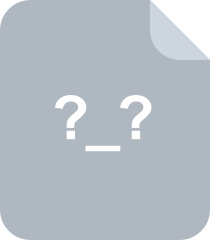
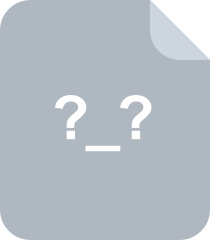
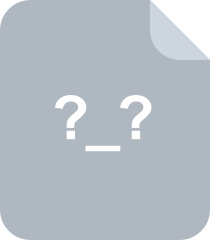
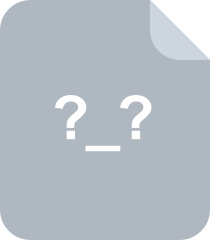
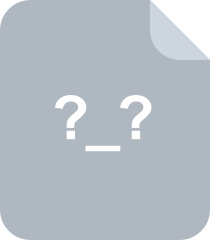
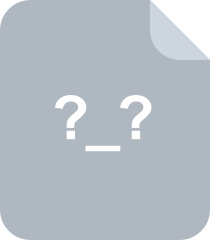
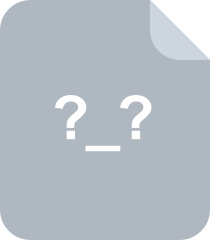
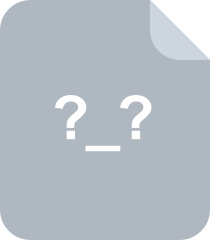
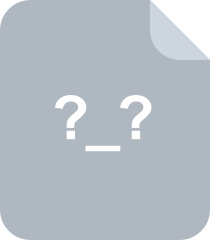
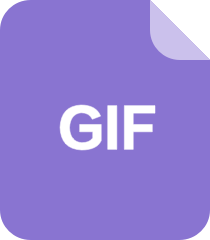
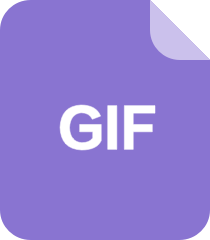
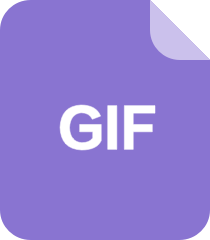
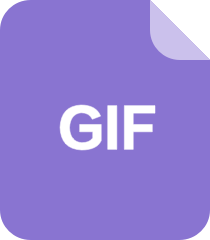
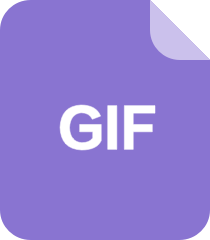
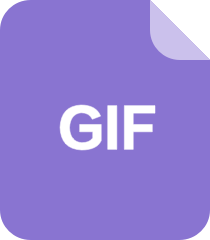
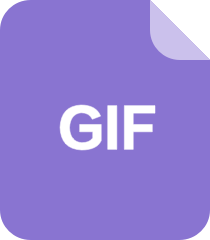
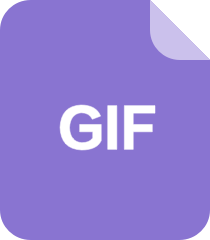
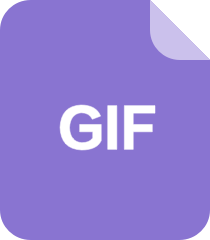
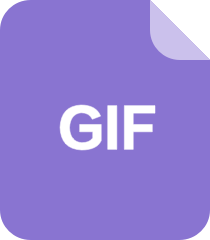
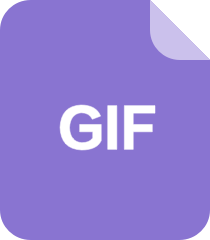
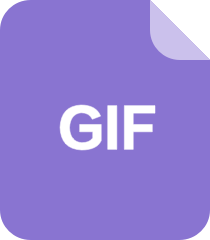
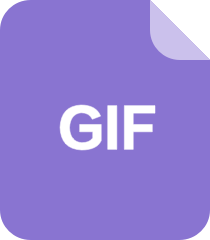
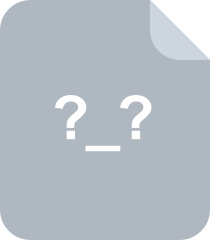
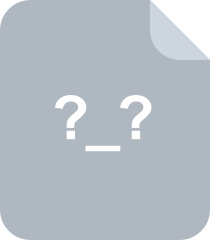
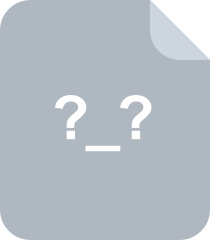
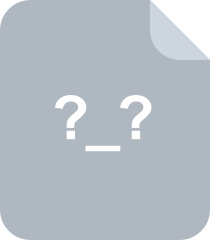
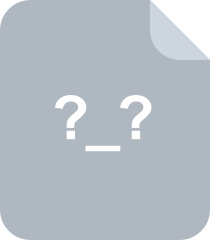
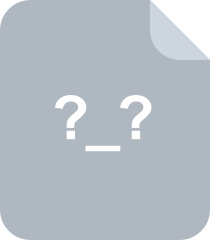
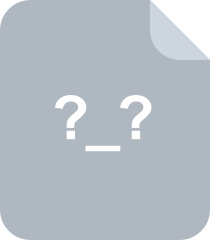
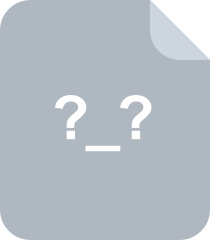
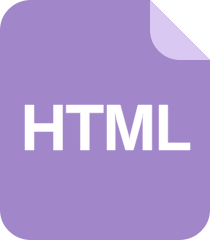
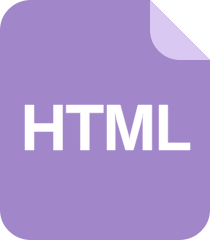
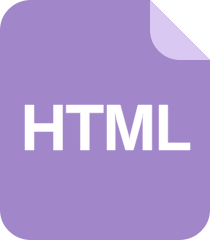
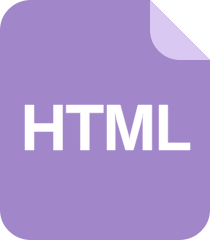
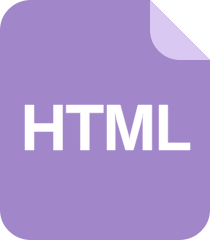
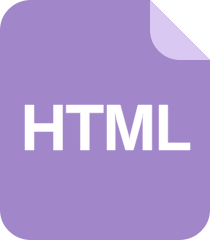
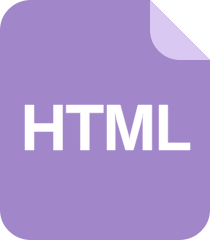
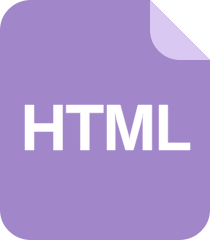
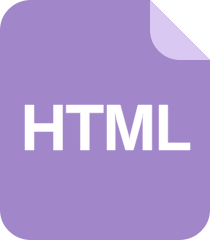
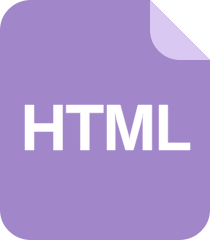
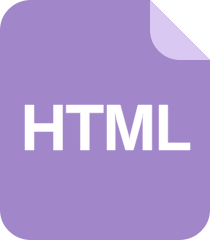
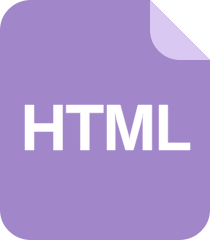
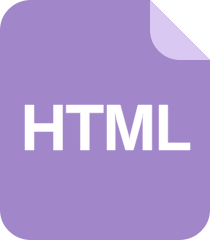
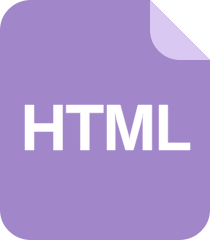
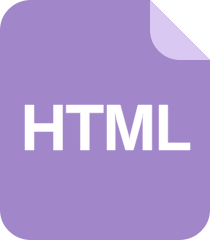
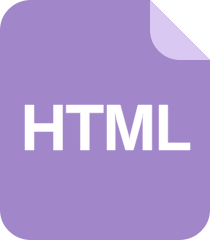
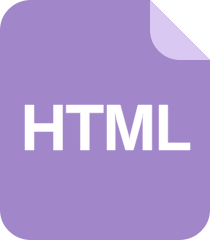
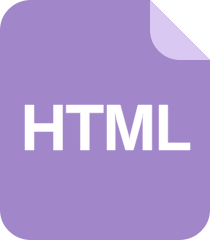
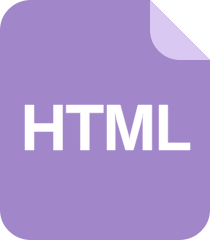
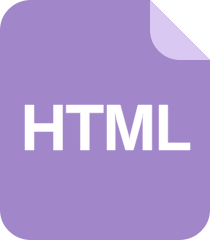
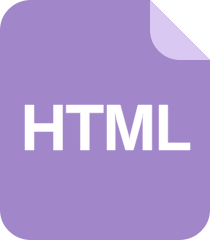
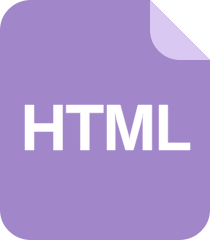
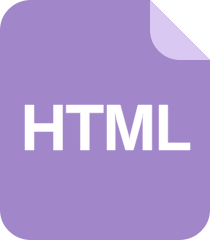
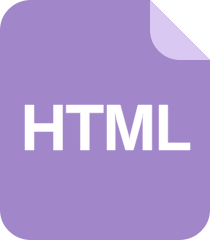
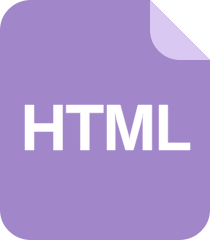
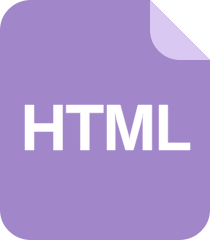
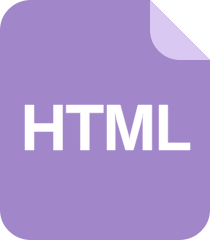
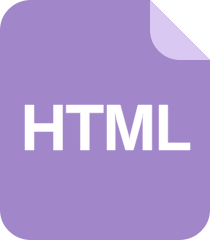
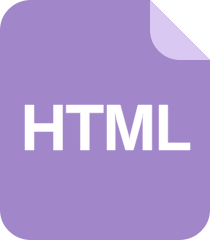
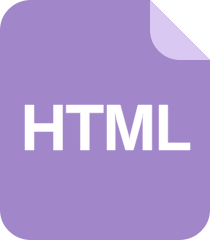
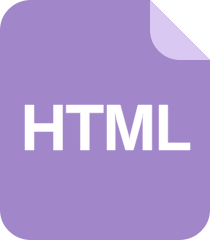
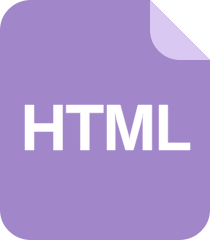
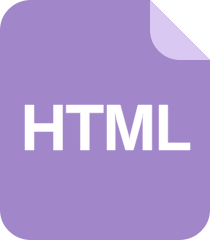
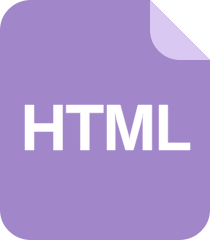
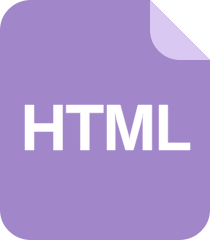
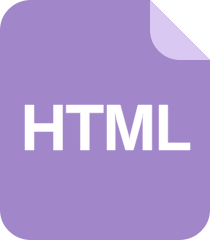
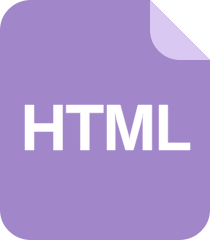
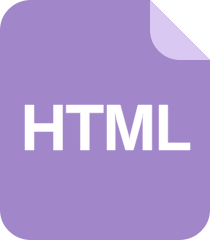
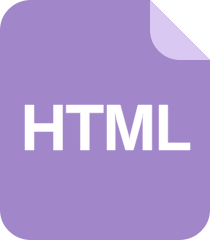
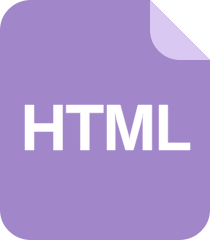
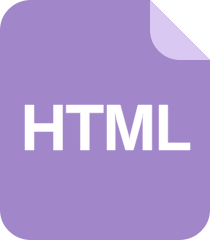
共 1214 条
- 1
- 2
- 3
- 4
- 5
- 6
- 13
资源评论
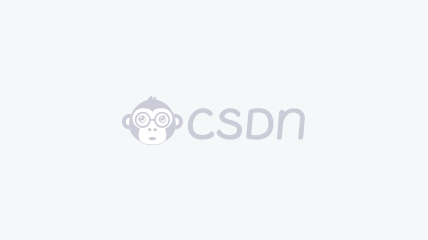

盈梓的博客
- 粉丝: 9341
- 资源: 2248
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

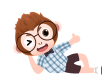
最新资源
- Delphi 12 控件之Unit-EchartsMapNew.pas
- delphi 12 控件之Dart-Products-Incl-Keygen-Patch-v1.0.0.6-By-DFoX.rar
- 开发工具+JDK+corretto-1.8.0-422.zip
- 晶体管的分类,由浅入深
- JAVAssm+bootstrap的零食商城源码数据库 MySQL源码类型 WebForm
- 前端vue+前端koa,全栈式开发bilibili首页.zip
- PHP在线文档管理系统源码数据库 MySQL源码类型 WebForm
- BAY06_0072_20241129_024710_114.cfg
- 基于Javaweb的用户笔记管理系统
- 基于SpringBoot+Vue的超市管理系统
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


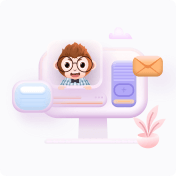
安全验证
文档复制为VIP权益,开通VIP直接复制
