import os
import struct
import numpy as np
import csv
# 读入数据,加载下载的MNIST数据是,需要这样读出
def load_mnist(path, kind='train'):
"""Load MNIST data from `path`"""
labels_path = os.path.join(path,
'%s-labels.idx1-ubyte'
% kind)
images_path = os.path.join(path,
'%s-images.idx3-ubyte'
% kind)
with open(labels_path, 'rb') as lbpath:
magic, n = struct.unpack('>II',
lbpath.read(8))
labels = np.fromfile(lbpath,
dtype=np.uint8)
with open(images_path, 'rb') as imgpath:
magic, num, rows, cols = struct.unpack('>IIII',
imgpath.read(16))
images = np.fromfile(imgpath,
dtype=np.uint8).reshape(len(labels), 28, 28)
return images, labels # image的大小是60000*28*28
# 将数据从原来的数据中读出,并写入CSV,分为6个CSV写入
# 把一个数据集拆分为6个
# 数据集的格式为:一张图片:28*28 + 1
# 返回数据集名称张量
def makeCSVFiles(path, kind="train"):
data_train_path = os.path.join(path, "data_train")
labels_path = os.path.join(path,
'%s-labels.idx1-ubyte'
% kind)
images_path = os.path.join(path,
'%s-images.idx3-ubyte'
% kind)
with open(labels_path, 'rb') as lbpath:
magic, n = struct.unpack('>II',
lbpath.read(8))
labels = np.fromfile(lbpath,
dtype=np.uint8)
with open(images_path, 'rb') as imgpath:
magic, num, rows, cols = struct.unpack('>IIII',
imgpath.read(16))
images = np.fromfile(imgpath,
dtype=np.uint8).reshape(len(labels), 784)
#打开第一个CSV
file = open(os.path.join(data_train_path, ('mnist_batch0' + ".csv")),'w',newline='')
for i in range(len(labels)):
example = []
example.extend(images[i])
example.append(labels[i])
# with file as f:
writer = csv.writer(file)
writer.writerow(example)
# print("example:"+str(example))
if i % 10000 == 0:
print("i:"+str(i))
index = int(i / 10000)
file.close()
# 存入文件中,第10000-20000个存入另一个CSV
file = open(os.path.join(data_train_path, ('mnist_batch' + str(index) + ".csv")),'w',newline='')
file.close()
# makeCSVFiles(path="data")
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于python实现的CNN卷积神经网络手写数字识别实验源码+数据集(高分毕业设计).zip该项目是个人高分毕业设计项目源码,已获导师指导认可通过,都经过严格调试,确保可以运行!放心下载使用。 基于python实现的CNN卷积神经网络手写数字识别实验源码+数据集(高分毕业设计).zip该项目是个人高分毕业设计项目源码,已获导师指导认可通过,都经过严格调试,确保可以运行!放心下载使用。 基于python实现的CNN卷积神经网络手写数字识别实验源码+数据集(高分毕业设计).zip该项目是个人高分毕业设计项目源码,已获导师指导认可通过,都经过严格调试,确保可以运行!放心下载使用。 基于python实现的CNN卷积神经网络手写数字识别实验源码+数据集(高分毕业设计).zip该项目是个人高分毕业设计项目源码,已获导师指导认可通过,都经过严格调试,确保可以运行!放心下载使用。 基于python实现的CNN卷积神经网络手写数字识别实验源码+数据集(高分毕业设计).zip该项目是个人高分毕业设计项目源码,已获导师指导认可通过,都经过严格调试,确保可以运行!放心下载使用。 基于python实现的CN
资源推荐
资源详情
资源评论
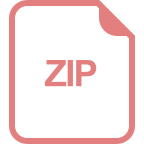
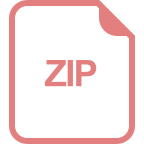
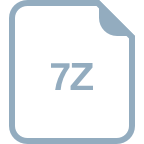
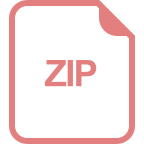
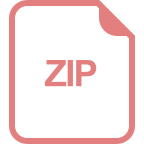
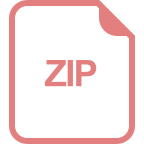
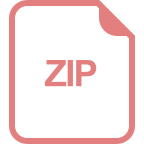
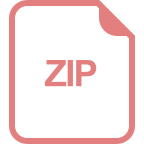
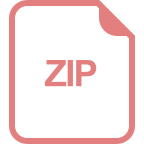
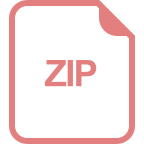
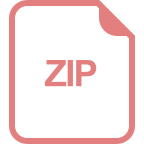
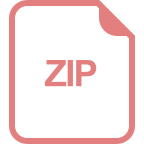
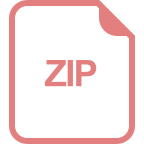
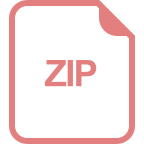
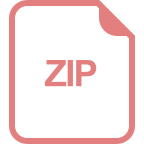
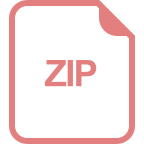
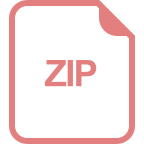
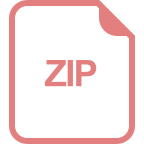
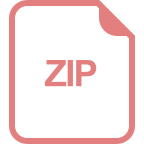
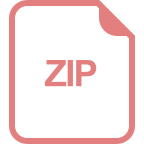
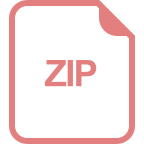
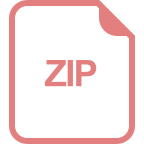
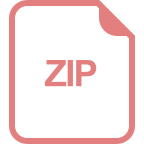
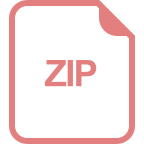
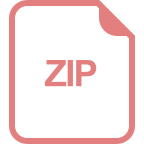
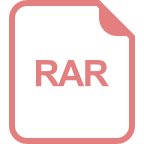
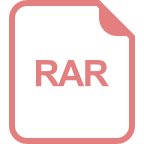
收起资源包目录





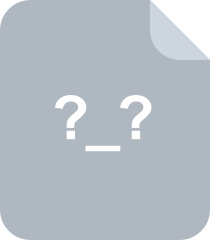
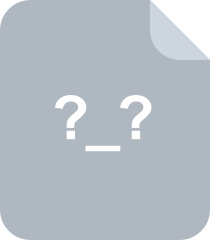
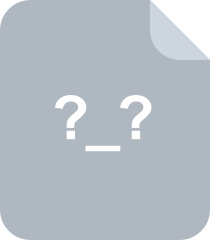

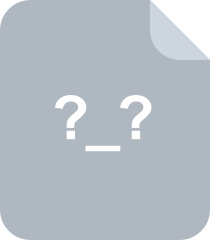
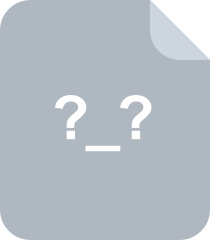
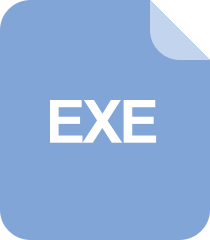
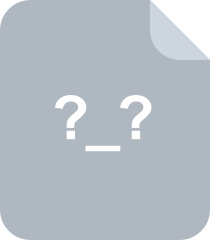
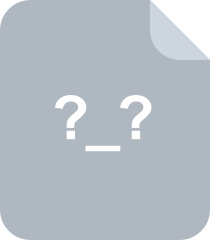
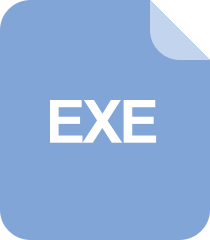
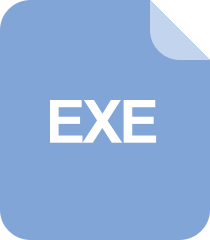
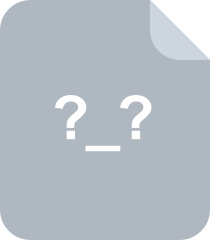
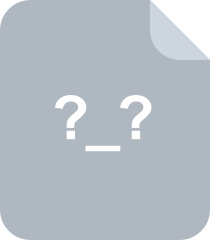
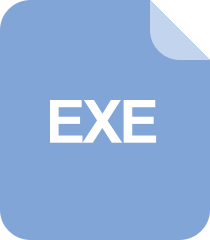
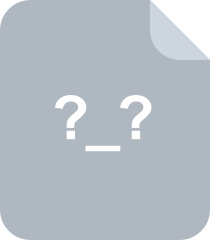
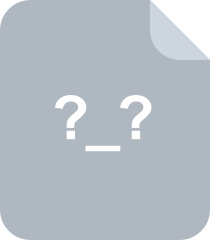
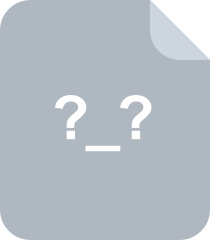
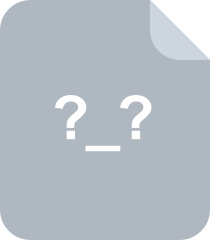
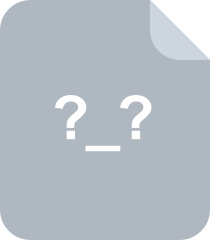
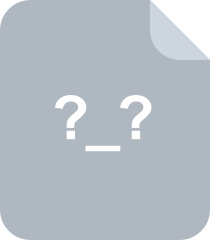
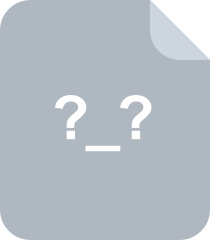
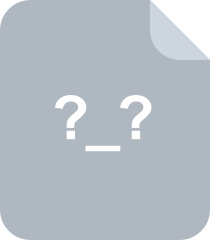
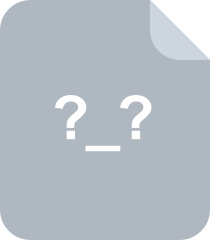
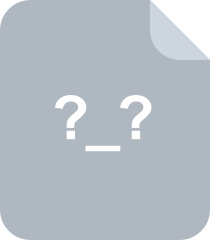
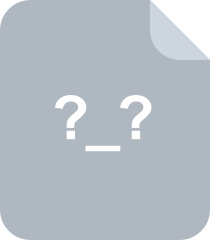
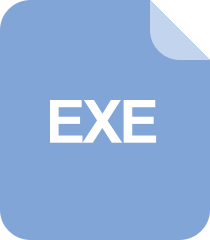
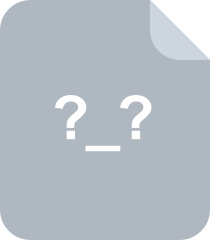
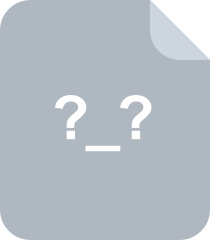
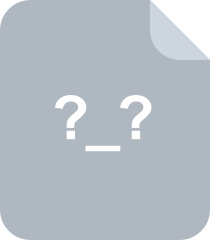
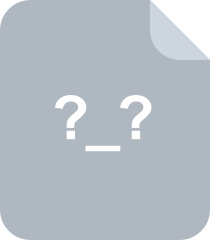
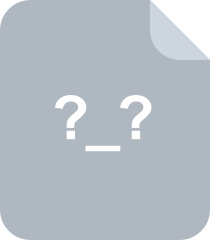
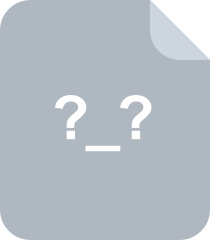
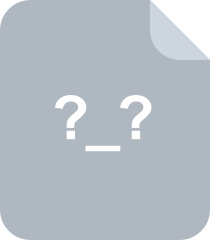
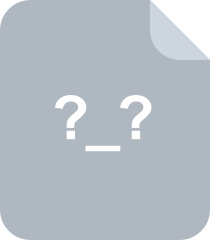
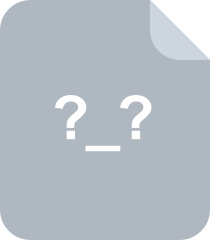
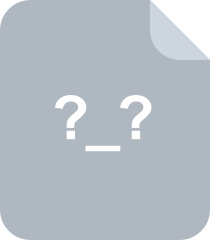
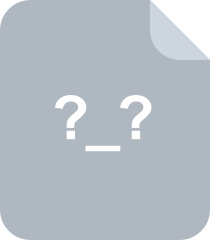
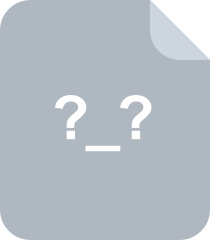
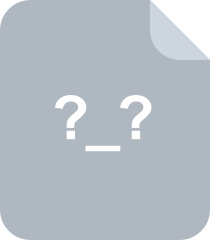
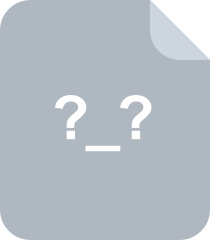
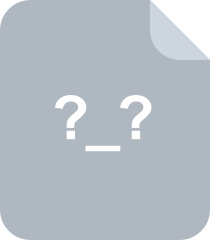
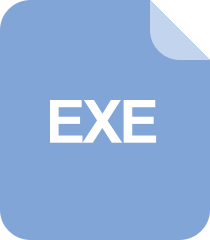
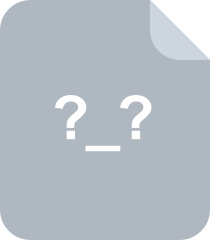
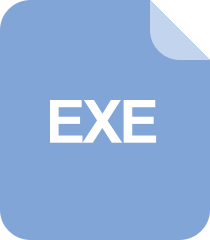
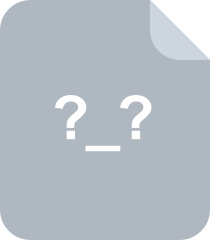
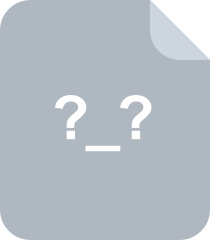
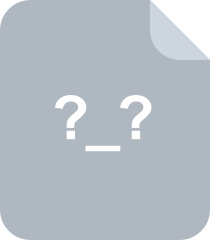
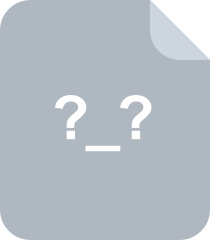
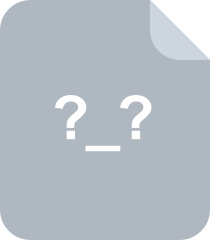



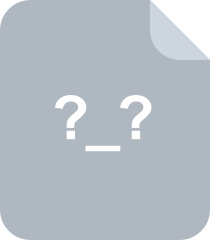

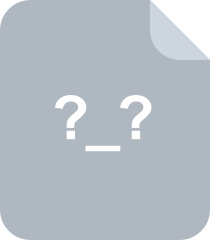
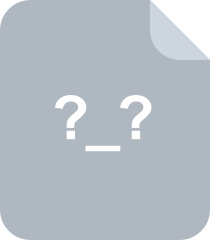

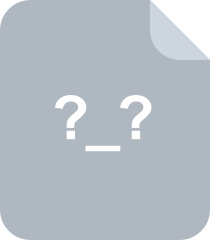

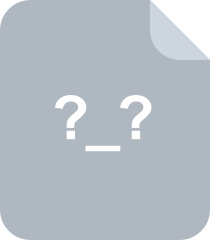


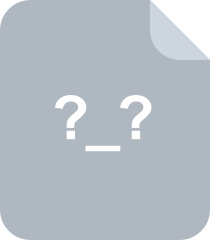
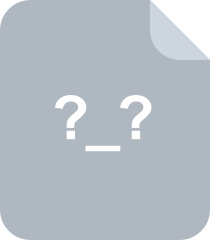
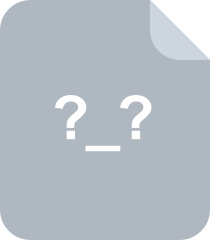
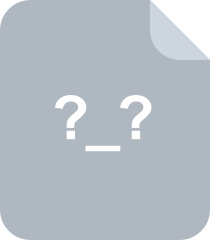
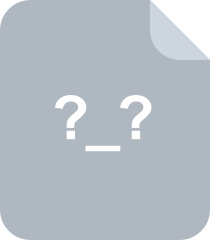
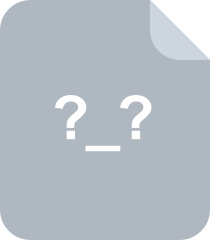


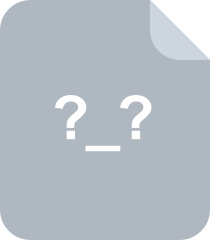
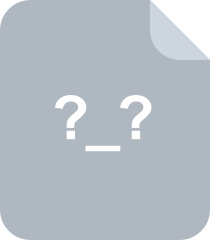

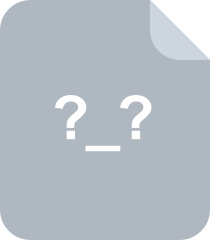
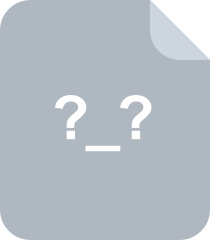
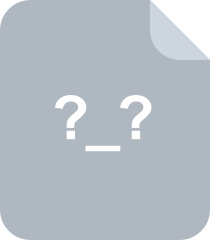
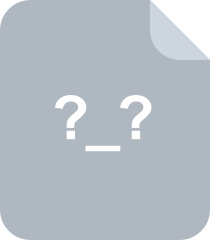
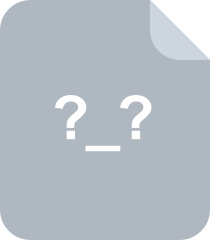

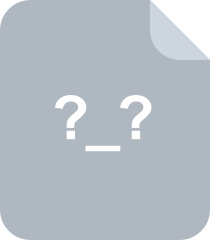
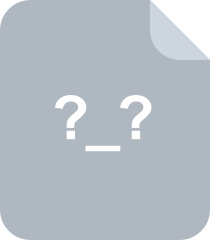
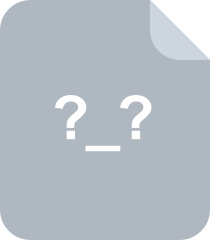
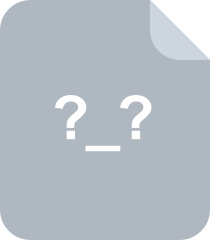
共 74 条
- 1
资源评论
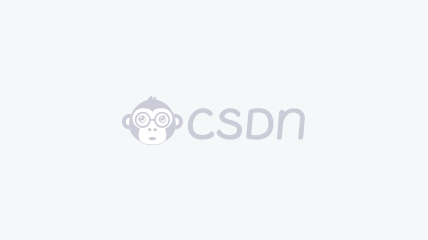
- jianzong890762024-01-13资源很实用,对我启发很大,有很好的参考价值,内容详细。
- Asdf_smy2024-06-18这个资源对我启发很大,受益匪浅,学到了很多,谢谢分享~
- hmm_66581402024-03-11资源不错,很实用,内容全面,介绍详细,很好用,谢谢分享。

盈梓的博客
- 粉丝: 9287
- 资源: 2203
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

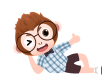
最新资源
- 适用于 Raspberry Pi 的 Adafruit 库代码.zip
- 章节2:编程基本概念之python程序的构成
- 适用于 Python 的 LINE 消息 API SDK.zip
- 宝塔面板安装及关键网络安全设置指南
- 适用于 Python 的 AWS 开发工具包.zip
- 适用于 Python 3 的 Django LDAP 用户身份验证后端 .zip
- 基于PBL-CDIO的材料成型及控制工程课程设计实践与改革
- JQuerymobilea4中文手册CHM版最新版本
- 适用于 Python 2 和 3 以及 PyPy (ws4py 0.5.1) 的 WebSocket 客户端和服务器库.zip
- 适用于 AWS 的 Python 无服务器微框架.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


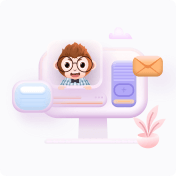
安全验证
文档复制为VIP权益,开通VIP直接复制
