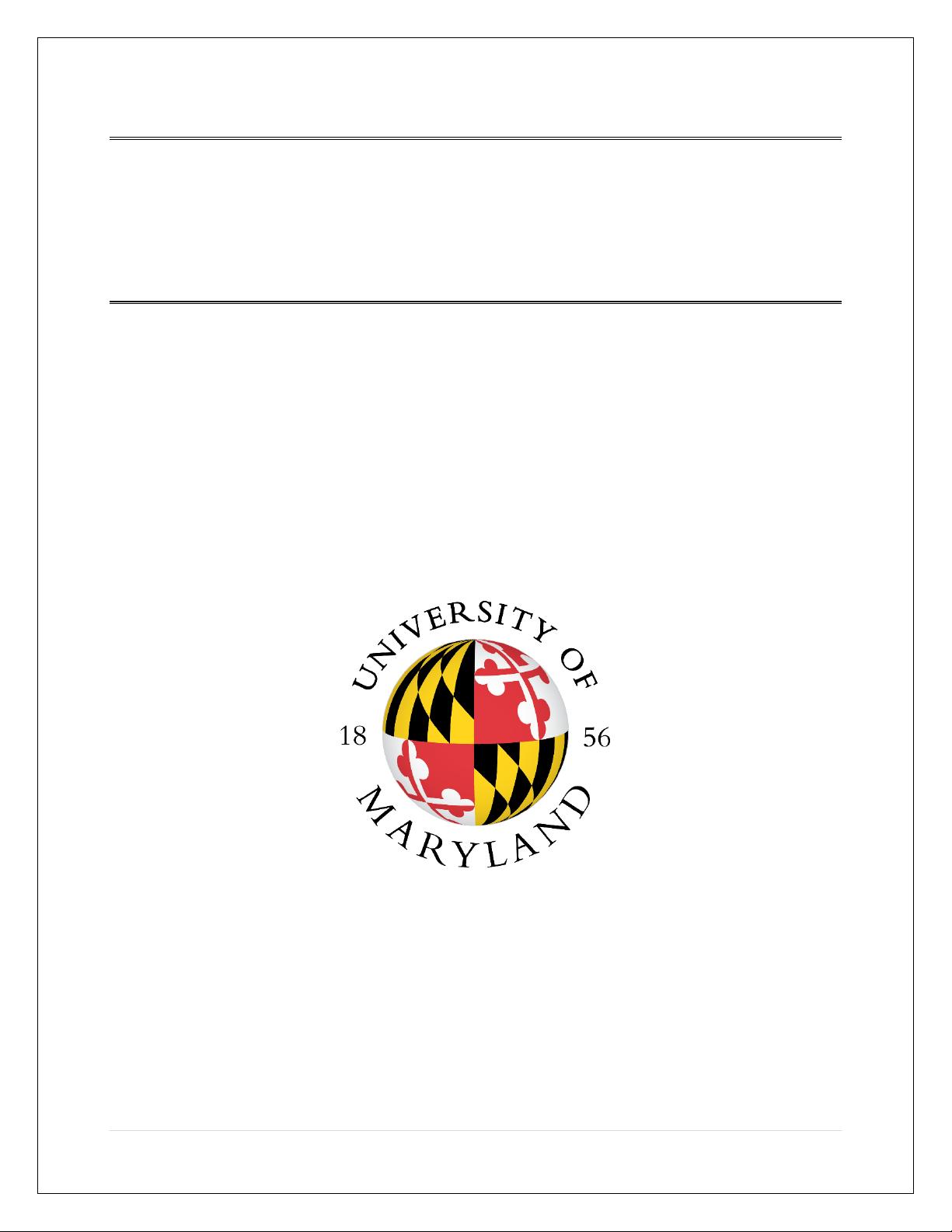
Project 1: Lane Detection Yash Shah
1 | P a g e
Project 1: Lane Detection
ENPM673: Perception for Autonomous Robots
Project Report
Guide: Prof. Cornelia Fermuller
Yash Shah
UID: 115710498
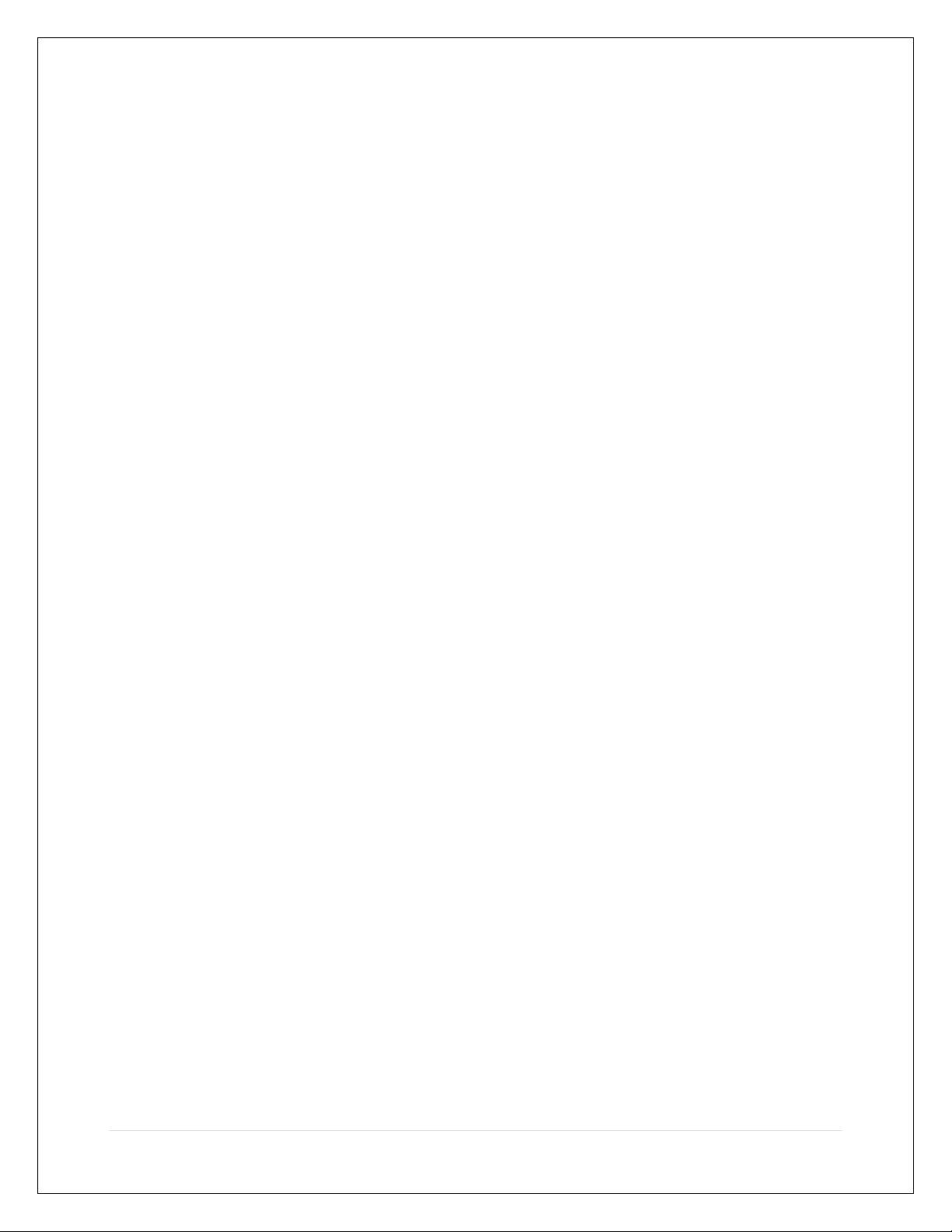
Project 1: Lane Detection Yash Shah
2 | P a g e
Contents
Lane Detection .................................................................................................. 2
Pre-processing the Image .................................................................................. 4
Edge Detection .................................................................................................. 7
Extraxtion of Region of Interest ......................................................................... 8
Hough Transform ............................................................................................ 14
Extrapolation of Lines...................................................................................... 15
Turn Prediction ............................................................................................... 16
Conclusion………………………………………………………………………………………………………17
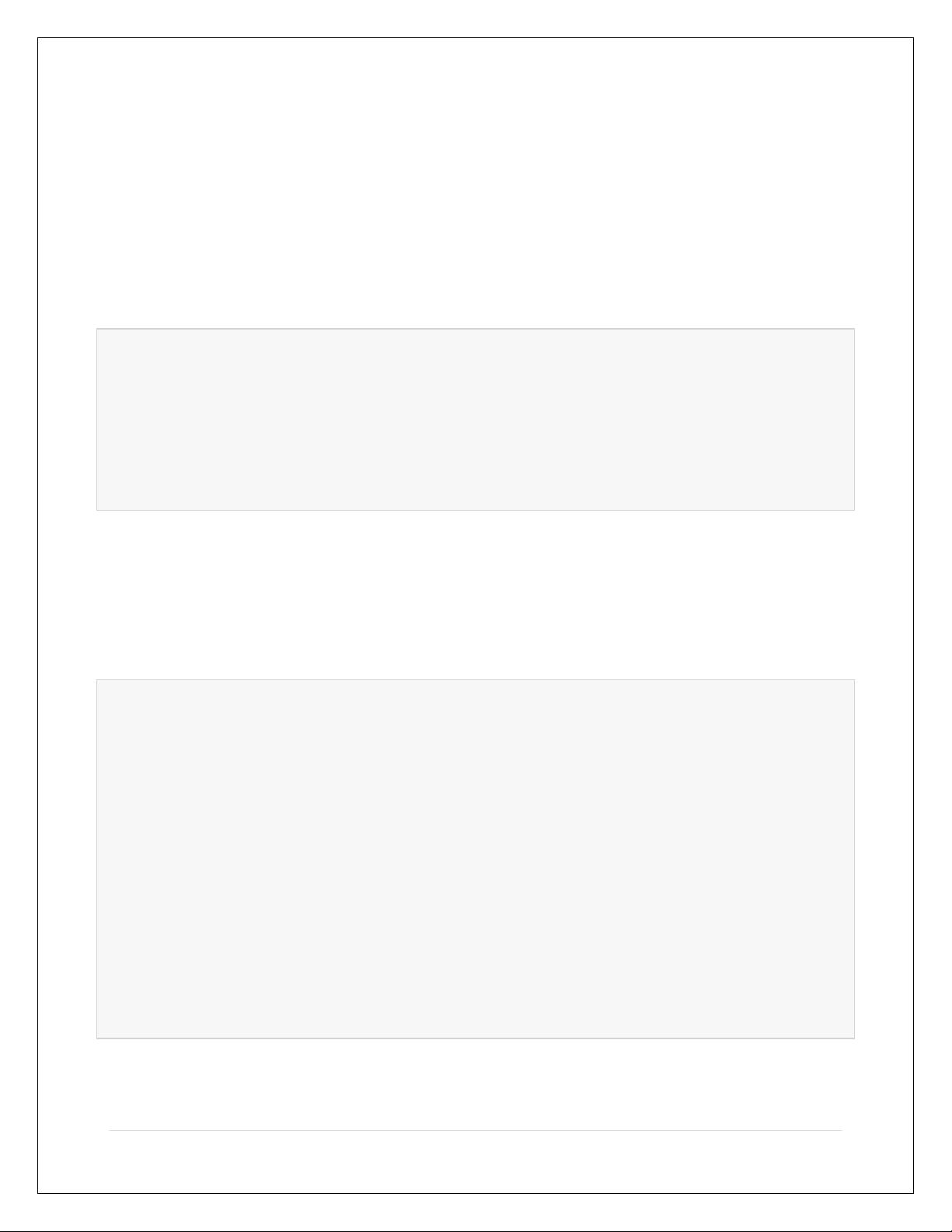
Project 1: Lane Detection Yash Shah
3 | P a g e
Lane Detection
In this project, MATLAB is used as an Image Processing Tool to detect Lanes on the road. The
following techniques are used for lane detection.
• Color Masking
• Canny Edge Detection
• Region of Interest Selection
• Hough Transform Line detection
%==========================================================================
% MATLAB code for Project 1 (Perception Class)
% Written by Yash Shah (115710498)
% email ID: ysshah95@umd.edu
%==========================================================================
Pre-processing the Image
The first step is to import the video file and initialize the variables to be use din the code. Some
variables are also imported from the .mat file to be used in the code.
Initializing the Code
clc
close all
clear all
%-------------------------Importing the Video File-------------------------
VideoFile = VideoReader('project_video.mp4');
%-------------------Loading Region of Interest Variables-------------------
load('roi_variables', 'c', 'r');
%-----------------Defining Variables for saving Video File-----------------
Output_Video=VideoWriter('Result_Yash');
Output_Video.FrameRate= 25;
open(Output_Video);
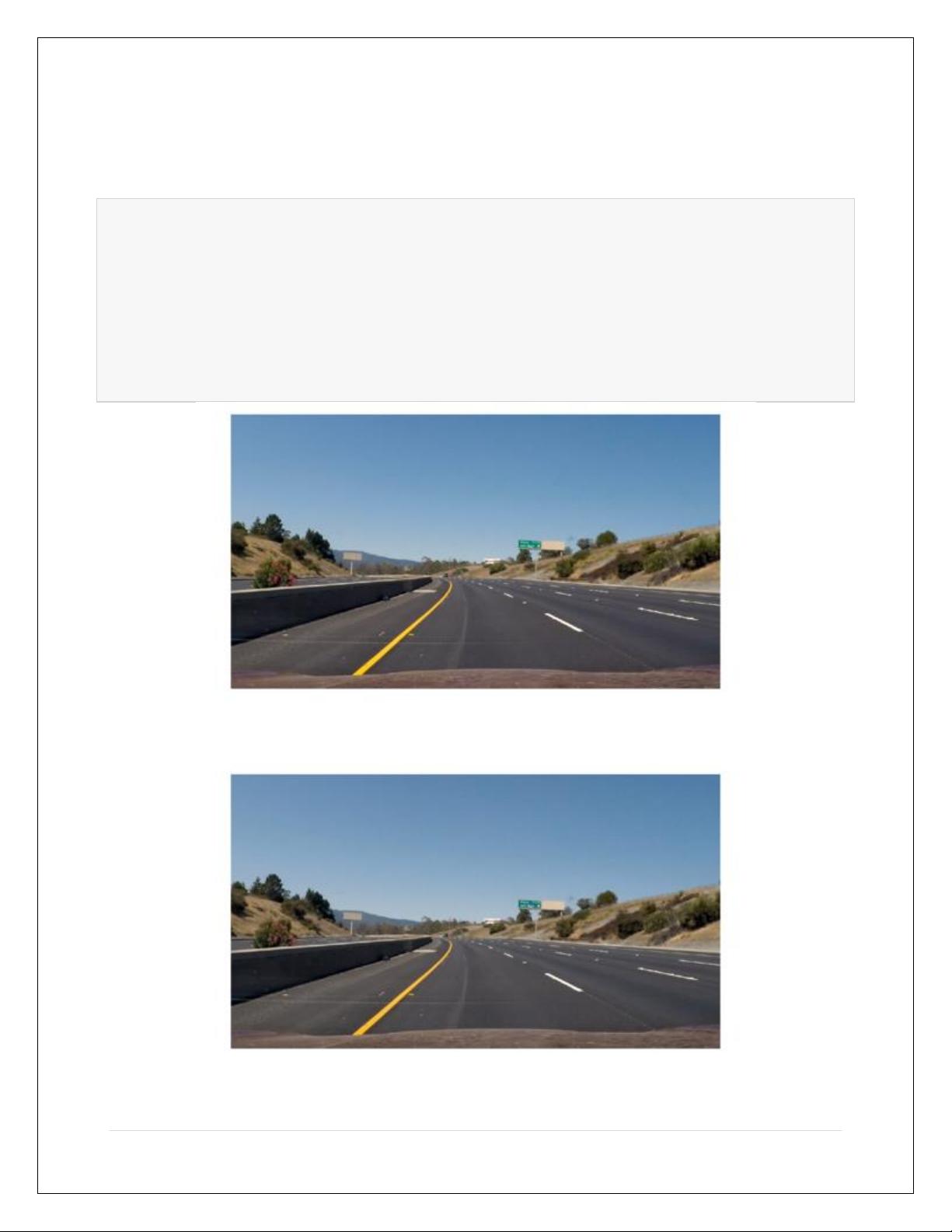
Project 1: Lane Detection Yash Shah
4 | P a g e
Initializing the loop to take frames one by one
First the frame is read and them filtered using a Gaussian Filter.
while hasFrame(VideoFile)
%------------------Reading each frame from Video File------------------
frame = readFrame(VideoFile);
figure('Name','Original Image'), imshow(frame);
frame = imgaussfilt3(frame);
figure('Name','Filtered Image'), imshow(frame);
Fig 1: Original Image
Fig 2: Filtered Image
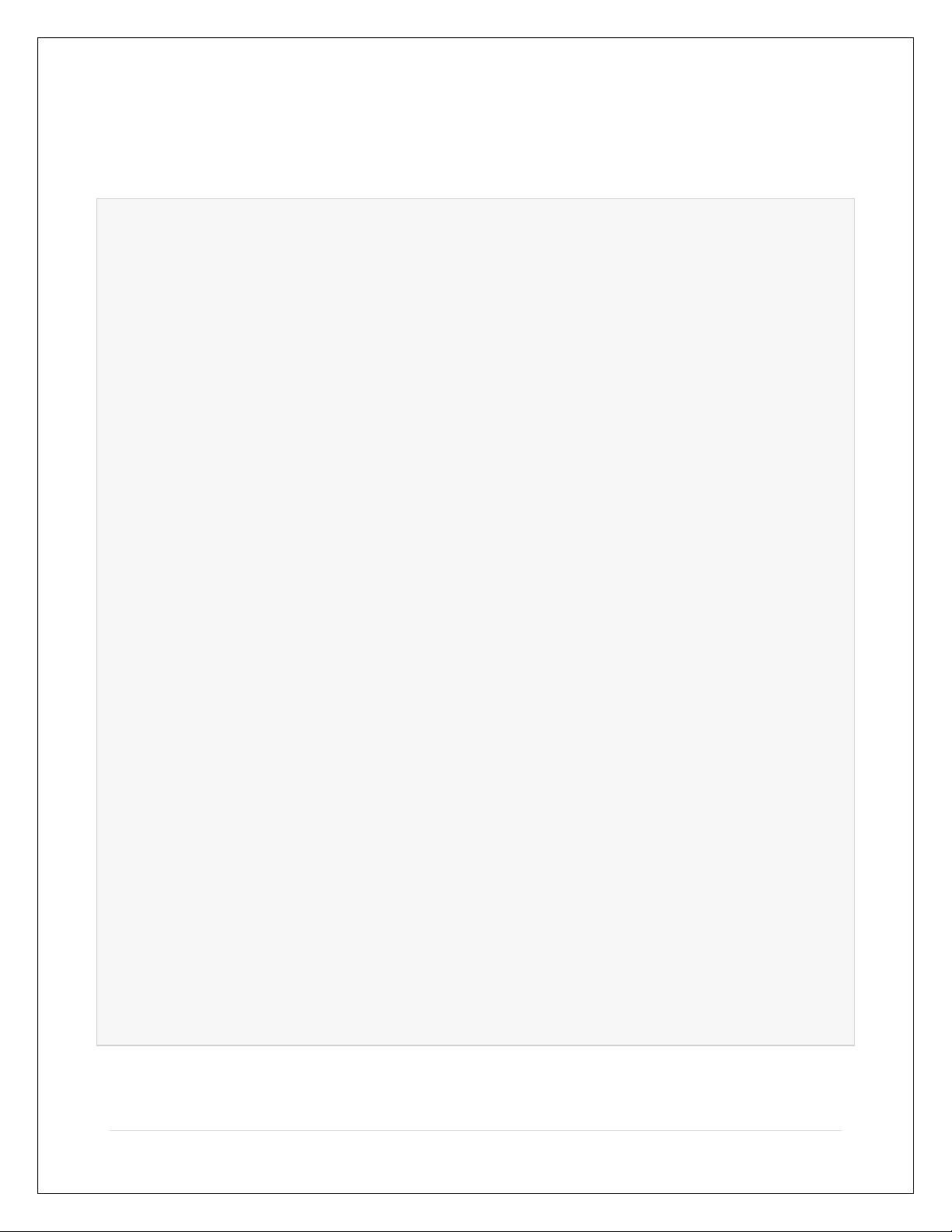
Project 1: Lane Detection Yash Shah
5 | P a g e
Masking the image for White and Yellow Color
The frame is masked with yellow and white color to detect the lane lines perfectly.
%--------------Define Thresholds for masking Yellow Color--------------
%----------------------Define thresholds for 'Hue'---------------------
channel1MinY = 130;
channel1MaxY = 255;
%------------------Define thresholds for 'Saturation'------------------
channel2MinY = 130;
channel2MaxY = 255;
%---------------------Define thresholds for 'Value'--------------------
channel3MinY = 0;
channel3MaxY = 130;
%-----------Create mask based on chosen histogram thresholds-----------
Yellow=((frame(:,:,1)>=channel1MinY)|(frame(:,:,1)<=channel1MaxY))& ...
(frame(:,:,2)>=channel2MinY)&(frame(:,:,2)<=channel2MaxY)&...
(frame(:,:,3)>=channel3MinY)&(frame(:,:,3)<=channel3MaxY);
figure('Name','Yellow Mask'), imshow(Yellow);
%--------------Define Thresholds for masking White Color---------------
%----------------------Define thresholds for 'Hue'---------------------
channel1MinW = 200;
channel1MaxW = 255;
%------------------Define thresholds for 'Saturation'------------------
channel2MinW = 200;
channel2MaxW = 255;
%---------------------Define thresholds for 'Value'--------------------
channel3MinW = 200;
channel3MaxW = 255;
%-----------Create mask based on chosen histogram thresholds-----------
White=((frame(:,:,1)>=channel1MinW)|(frame(:,:,1)<=channel1MaxW))&...
(frame(:,:,2)>=channel2MinW)&(frame(:,:,2)<=channel2MaxW)& ...
(frame(:,:,3)>=channel3MinW)&(frame(:,:,3)<=channel3MaxW);
figure('Name','White Mask'), imshow(White);
- 1
- 2
前往页