Complex复数类
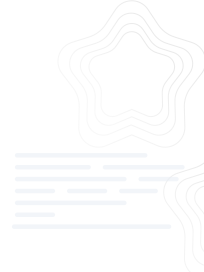

在C++编程中,"Complex复数类"是用于处理复数数据类型的一种自定义类。复数由实部和虚部组成,通常表示为`a + bi`,其中`a`是实部,`b`是虚部,`i`是虚数单位,其平方等于-1。创建复数类可以帮助我们更方便地进行复数相关的计算,如加法、减法、乘法等。 我们来看一下`c.h`头文件,这是定义复数类的接口部分。在这个头文件中,通常会包含类的声明,包括类名`Complex`、数据成员(实部和虚部)以及相关的成员函数声明,如构造函数、析构函数、访问器(getter)和修改器(setter)、复数运算方法等。例如: ```cpp class Complex { public: // 构造函数 Complex(double real = 0.0, double imaginary = 0.0); // 赋值运算符 Complex& operator=(const Complex& other); // 加法运算符 Complex operator+(const Complex& other) const; // 减法运算符 Complex operator-(const Complex& other) const; // 乘法运算符 Complex operator*(const Complex& other) const; // 访问器 double getReal() const; double getImaginary() const; // 修改器 void setReal(double real); void setImaginary(double imaginary); private: double real; // 实部 double imaginary; // 虚部 }; ``` 接下来,`c.cpp`文件是实现复数类的源代码,它包含了类的成员函数的具体实现。比如,构造函数用于初始化复数对象,赋值运算符用于复数之间的赋值,而加减乘运算符重载则实现了复数的算术运算。这里可能的实现如下: ```cpp #include "c.h" // 构造函数 Complex::Complex(double r, double i) : real(r), imaginary(i) {} // 赋值运算符 Complex& Complex::operator=(const Complex& other) { if (this != &other) { real = other.real; imaginary = other.imaginary; } return *this; } // 加法运算符 Complex Complex::operator+(const Complex& other) const { return Complex(real + other.real, imaginary + other.imaginary); } // 减法运算符 Complex Complex::operator-(const Complex& other) const { return Complex(real - other.real, imaginary - other.imaginary); } // 乘法运算符 Complex Complex::operator*(const Complex& other) const { double new_real = real * other.real - imaginary * other.imaginary; double new_imaginary = real * other.imaginary + imaginary * other.real; return Complex(new_real, new_imaginary); } // 访问器 double Complex::getReal() const { return real; } double Complex::getImaginary() const { return imaginary; } // 修改器 void Complex::setReal(double r) { real = r; } void Complex::setImaginary(double i) { imaginary = i; } ``` `test.cpp`文件是用来测试`Complex`类功能的。它包含了主函数`main`,通过创建`Complex`对象并进行各种操作来验证类的功能是否正常。例如: ```cpp #include "c.h" #include <iostream> int main() { Complex c1(3, 4); Complex c2(1, -2); std::cout << "c1 = (" << c1.getReal() << ", " << c1.getImaginary() << ")\n"; std::cout << "c2 = (" << c2.getReal() << ", " << c2.getImaginary() << ")\n"; Complex c3 = c1 + c2; std::cout << "c1 + c2 = (" << c3.getReal() << ", " << c3.getImaginary() << ")\n"; c1 = c2; std::cout << "After assignment: c1 = (" << c1.getReal() << ", " << c1.getImaginary() << ")\n"; return 0; } ``` 通过编译和运行`test.cpp`,我们可以验证`Complex`类是否正确实现了复数的存储、加减乘运算以及赋值操作。这个例子展示了如何在C++中通过面向对象的方式处理复数,使得代码更加模块化和易于维护。同时,接口与实现的分离使得代码更具可读性和可扩展性。
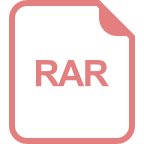
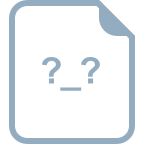
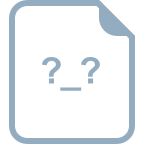
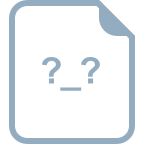
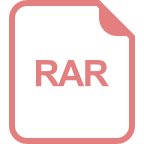
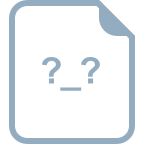
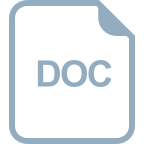
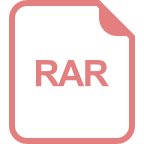
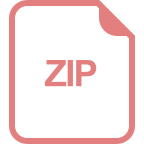
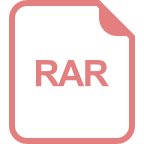
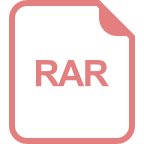
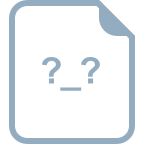
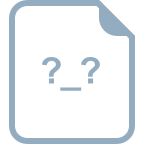
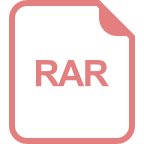
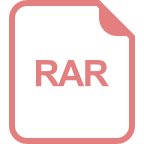
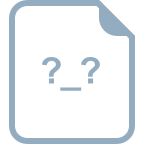
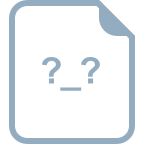
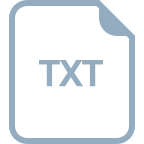
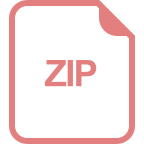
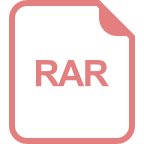
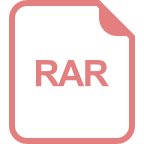
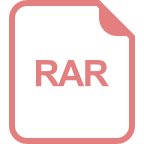
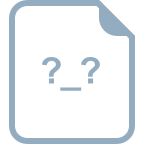

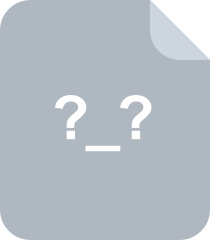
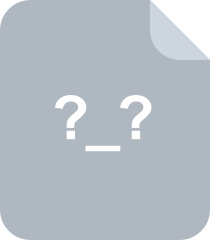
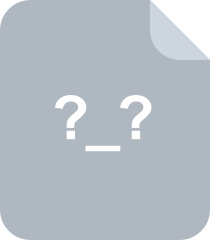
- 1
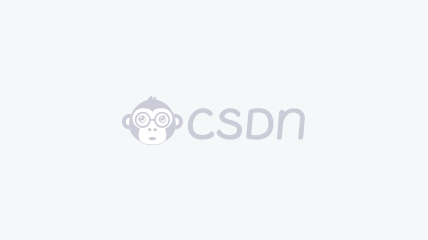

- 粉丝: 12
- 资源: 7
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

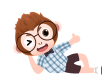
最新资源
- NOI 全国青少年信息学奥林匹克竞赛(官网)-2024.11.05.pdf
- 【Unity抢劫和犯罪题材的低多边形3D资源包】POLYGON Heist - Low Poly 3D Art
- 网络安全是一个广泛的领域,涉及的知识和技能非常多样.docx
- 用Python实现,PySide构建GUI界面的“井字棋”游戏 具备学习功能(源码)
- 系统测试报告模板 测试目的、测试依据、测试准备、测试内容、测试结果及分析、总结
- 雷柏2.4G无线鼠标键盘对码软件V3.1
- Python基础入门-待办事项列表.pdf
- 240301031刘炳炎咖啡网站导航.psd
- 数据集【YOLO目标检测】道路油污检测数据集 170 张,YOLO/VOC格式标注!
- 基于Robot FrameWork框架的自动化测试

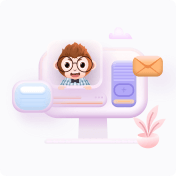
