
## DateTools
DateTools was written to streamline date and time handling in Objective-C. Classes and concepts from other languages served as an inspiration for DateTools, especially the [DateTime](http://msdn.microsoft.com/en-us/library/system.datetime(v=vs.110).aspx) structure and [Time Period Library](Time Period Library) for .NET. Through these classes and others, DateTools removes the boilerplate required to access date components, handles more nuanced date comparisons, and serves as the foundation for entirely new concepts like Time Periods and their collections.
[](https://travis-ci.org/MatthewYork/DateTools)
[](http://cocoapods.org/?q=datetools)
####Featured In
<table>
<tr>
<td align="center">
<a href="https://itunes.apple.com/hk/app/yahoo-livetext-video-messenger/id995121875?mt=8"><img src="http://a4.mzstatic.com/us/r30/Purple2/v4/7c/1b/11/7c1b11f3-2a73-b655-751e-b21b2e8bc6f7/icon100x100.png" /></a>
</td>
<td align="center">
<a href="https://itunes.apple.com/app/id547436543"><img src="http://a5.mzstatic.com/us/r30/Purple5/v4/1f/7b/a5/1f7ba545-038e-353e-18a0-b6472eef1913/icon100x100.jpeg" /></a>
</td>
<td align="center">
<a href="https://itunes.apple.com/us/app/aldi-usa/id429396645?mt=8"><img src="http://a4.mzstatic.com/us/r30/Purple7/v4/a7/63/20/a76320db-2de4-62ad-b620-efaab8a179dc/icon100x100.jpeg" /></a>
</td>
<td align="center">
<a href="https://itunes.apple.com/us/app/guidebook/id428713847?mt=8"><img src="http://a5.mzstatic.com/us/r30/Purple7/v4/e4/af/db/e4afdbc1-9ceb-c403-4d06-299e7e693120/icon100x100.png" /></a>
</td>
<td align="center">
<a href="https://itunes.apple.com/us/app/pitch-locator-pro/id964965940?mt=8"><img src="http://a2.mzstatic.com/us/r30/Purple7/v4/39/ed/24/39ed248b-afab-ce8d-4276-35ba0459ac60/icon100x100.png" /></a>
</td>
<tr>
<td align="center">Yahoo! Livetext</td>
<td align="center">My Disney Experience</td>
<td align="center">ALDI</td>
<td align="center">Guidebook</td>
<td align="center">Pitch Locator Pro</td>
</tr>
</tr>
</table>
## Installation
**Cocoapods**
<code>pod 'DateTools'</code>
**Manual Installation**
All the classes required for DateTools are located in the DateTools folder in the root of this repository. They are listed below:
* <code>DateTools.h</code>
* <code>NSDate+DateTools.{h,m}</code>
* <code>DTConstants.h</code>
* <code>DTError.{h,m}</code>
* <code>DTTimePeriod.{h,m}</code>
* <code>DTTimePeriodGroup.{h,m}</code>
* <code>DTTimePeriodCollection.{h,m}</code>
* <code>DTTimePeriodChain.{h,m}</code>
The following bundle is necessary if you would like to support internationalization or would like to use the "Time Ago" functionality. You can add localizations at the `Localizations` subheading under `Info` in the `Project` menu.
* <code>DateTools.bundle</code>
<code>DateTools.h</code> contains the headers for all the other files. Import this if you want to link to the entire framework.
## Table of Contents
* [**NSDate+DateTools**](#nsdate-datetools)
* [Time Ago](#time-ago)
* [Date Components](#date-components)
* [Date Editing](#date-editing)
* [Date Comparison](#date-comparison)
* [Formatted Date Strings](#formatted-date-strings)
* [**Time Periods**](#time-periods)
* [Initialization](#initialization)
* [Time Period Info](#time-period-info)
* [Manipulation](#manipulation)
* [Relationships](#relationships)
* [**Time Period Groups**](#time-period-groups)
* [Time Period Collections](#time-period-collections)
* [Time Period Chains](#time-period-chains)
* [**Unit Tests**](#unit-tests)
* [**Credits**](#credits)
* [**License**](#license)
##NSDate+DateTools
One of the missions of DateTools was to make NSDate feel more complete. There are many other languages that allow direct access to information about dates from their date classes, but NSDate (sadly) does not. It safely works only in the Unix time offsets through the <code>timeIntervalSince...</code> methods for building dates and remains calendar agnostic. But that's not <i>always</i> what we want to do. Sometimes, we want to work with dates based on their date components (like year, month, day, etc) at a more abstract level. This is where DateTools comes in.
####Time Ago
No date library would be complete without the ability to quickly make an NSString based on how much earlier a date is than now. DateTools has you covered. These "time ago" strings come in a long and short form, with the latter closely resembling Twitter. You can get these strings like so:
```objc
NSDate *timeAgoDate = [NSDate dateWithTimeIntervalSinceNow:-4];
NSLog(@"Time Ago: %@", timeAgoDate.timeAgoSinceNow);
NSLog(@"Time Ago: %@", timeAgoDate.shortTimeAgoSinceNow);
//Output:
//Time Ago: 4 seconds ago
//Time Ago: 4s
```
Assuming you have added the localization to your project, `DateTools` currently supports the following languages:
- ar (Arabic)
- bg (Bulgarian)
- ca (Catalan)
- zh_Hans (Chinese Simplified)
- zh_Hant (Chinese Traditional)
- cs (Czech)
- da (Danish)
- nl (Dutch)
- en (English)
- fi (Finnish)
- fr (French)
- de (German)
- gre (Greek)
- gu (Gujarati)
- he (Hebrew)
- hi (Hindi)
- hu (Hungarian)
- is (Icelandic)
- id (Indonesian)
- it (Italian)
- ja (Japanese)
- ko (Korean)
- lv (Latvian)
- ms (Malay)
- nb (Norwegian)
- pl (Polish)
- pt (Portuguese)
- ro (Romanian)
- ru (Russian)
- sl (Slovenian)
- es (Spanish)
- sv (Swedish)
- th (Thai)
- tr (Turkish)
- uk (Ukrainian)
- vi (Vietnamese)
- cy (Welsh)
- hr (Croatian)
If you know a language not listed here, please consider submitting a translation. [Localization codes by language](http://stackoverflow.com/questions/3040677/locale-codes-for-iphone-lproj-folders).
This project is user driven (by people like you). Pull requests close faster than issues (merged or rejected).
Thanks to Kevin Lawler for his work on [NSDate+TimeAgo](https://github.com/kevinlawler/NSDate-TimeAgo), which has been officially merged into this library.
####Date Components
There is a lot of boilerplate associated with getting date components from an NSDate. You have to set up a calendar, use the desired flags for the components you want, and finally extract them out of the calendar.
With DateTools, this:
```objc
//Create calendar
NSCalendar *calendar = [[NSCalendar alloc] initWithCalendarIdentifier:NSGregorianCalendar];
unitFlags = NSYearCalendarUnit | NSMonthCalendarUnit;
NSDateComponents *dateComponents = [calendar components:unitFlags fromDate:date];
//Get components
NSInteger year = dateComponents.year;
NSInteger month = dateComponents.month;
```
...becomes this:
```objc
NSInteger year = date.year;
NSInteger month = date.month;
```
And if you would like to use a non-Gregorian calendar, that option is available as well.
```objc
NSInteger day = [date dayWithCalendar:calendar];
```
If you would like to override the default calendar that DateTools uses, simply change it in the <code>defaultCalendar</code> method of <code>NSDate+DateTools.m</code>.
####Date Editing
The date editing methods in NSDate+DateTools makes it easy to shift a date earlier or later by adding and subtracting date components. For instance, if you would like a date that is 1 year later from a given date, simply call the method <code>dateByAddingYears</code>.
With DateTools, this:
```objc
//Create calendar
NSCalendar *calendar = [[NSCalendar alloc] initWithCalendarIdentifier:[NSDate defaultCalendar]];
NSDateComponents *components = [[NSDateComponents alloc] init];
//Make changes
[components setYear:1];
//Get new date with updated year
NSDate *newDate = [calendar dateByAddingComponents:components toDate:date options:0];
```
...becomes this:
```objc
NSDate *newDate = [date dateByAddingYears:1];
```
Subtraction of date components is
没有合适的资源?快使用搜索试试~ 我知道了~
待办事项提醒应用程序ToDoList
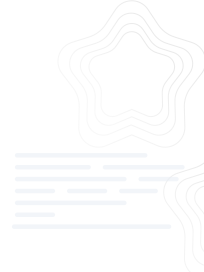
共391个文件
h:176个
m:73个
strings:46个

需积分: 0 0 下载量 81 浏览量
2023-06-11
09:22:49
上传
评论
收藏 548KB ZIP 举报
温馨提示
主要功能点 添加一个基于本地通知的提醒任务 设置提醒的开始日期,结束日期,触发提醒的时间点以及提醒的频次 如何将日期、触发时间点、提醒频次组合成一个有效的本地通知 声明一个UILocalNotification对象 使用NSDateComponents拼接触发通知的日期和时间点 设置UILocalNotification对象的fireDate属性,即,触发通知的具体日期时间 设置UILocalNotification对象的repeatInterval属性,即,触发通知的频率 最后使用[[UIApplication sharedApplication] scheduleLocalNotification:myLocalNotification]配置通知
资源推荐
资源详情
资源评论
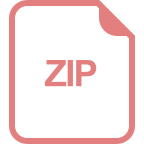
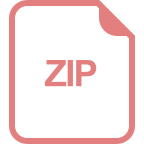
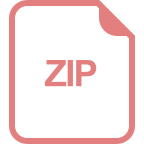
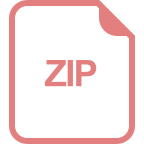
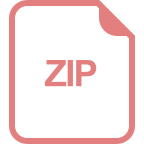
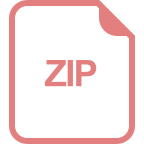
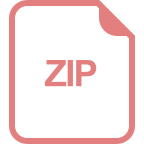
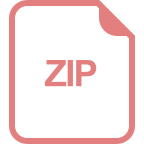
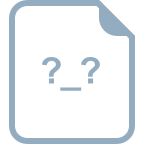
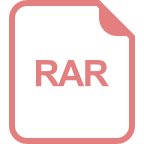
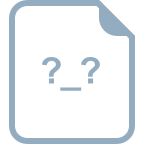
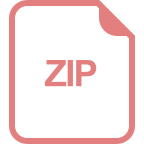
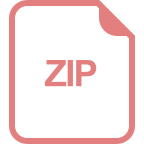
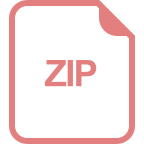
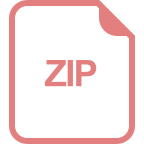
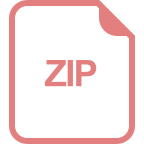
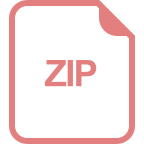
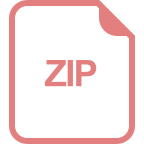
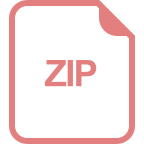
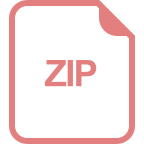
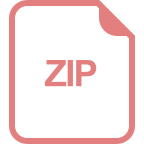
收起资源包目录

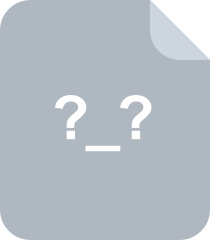
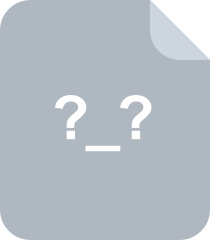
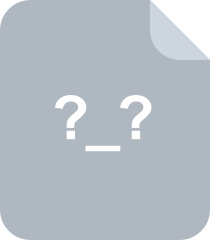
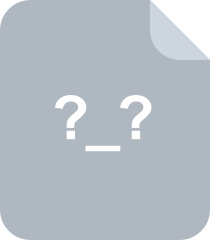
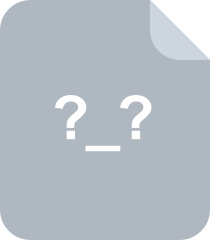
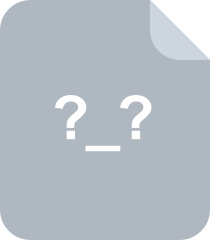
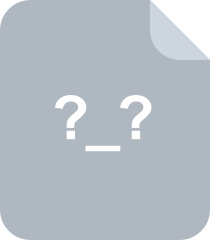
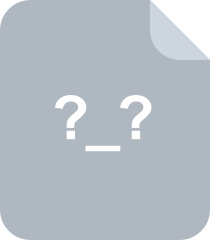
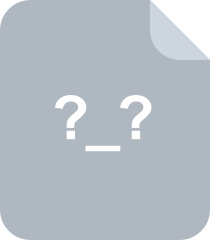
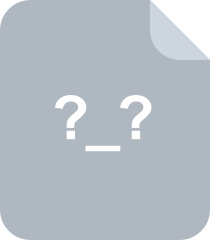
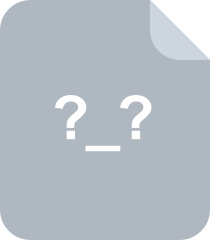
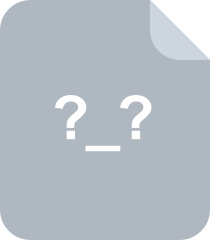
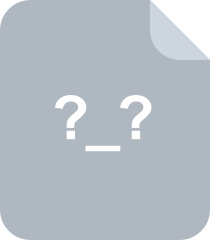
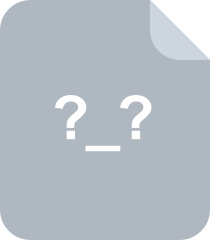
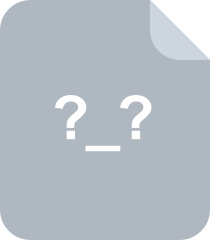
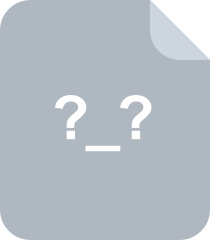
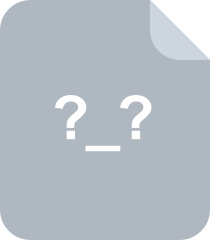
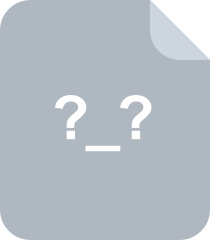
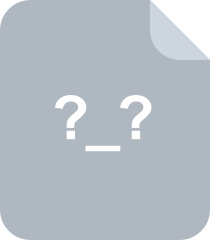
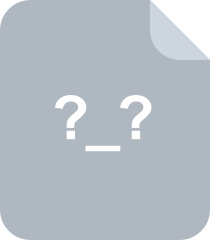
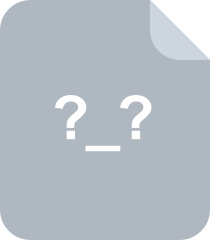
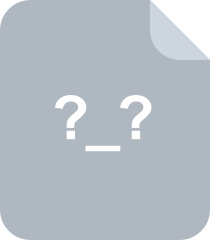
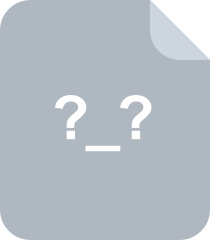
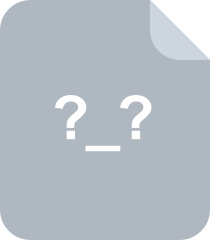
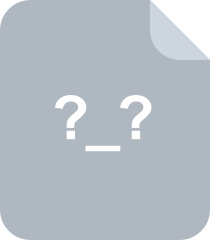
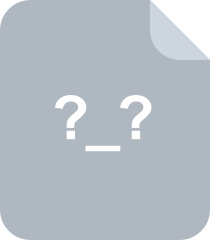
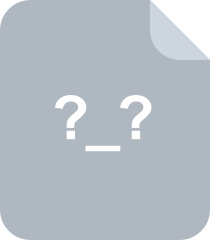
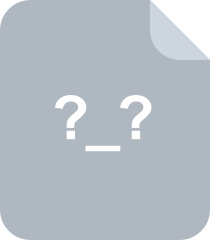
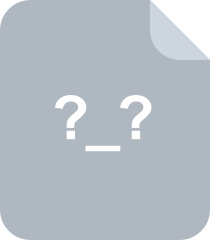
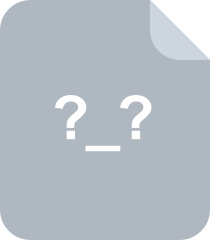
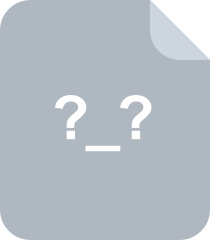
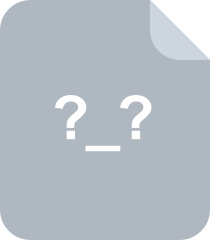
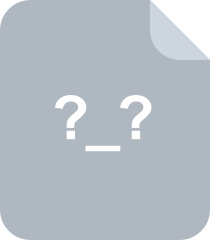
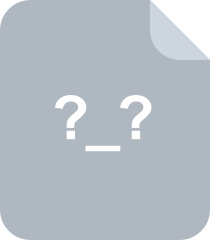
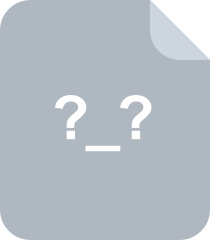
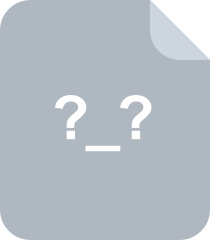
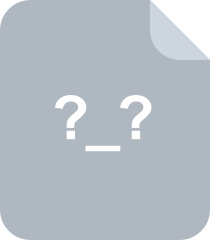
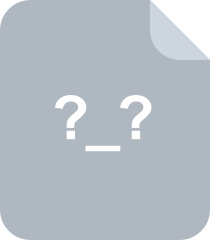
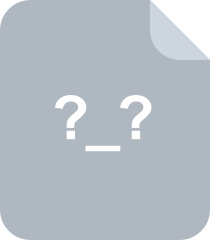
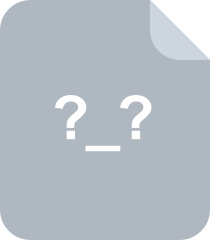
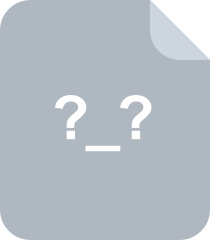
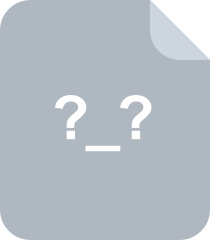
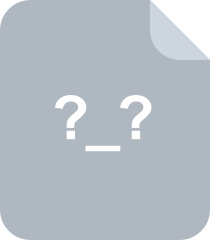
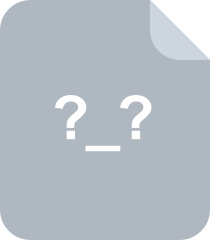
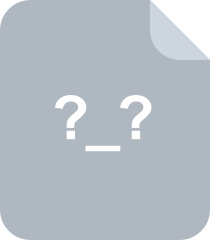
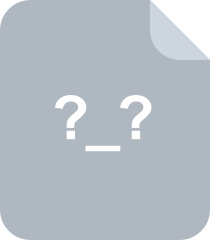
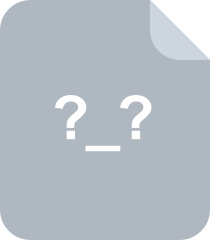
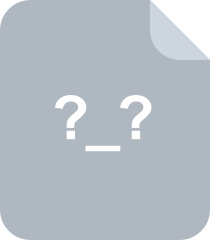
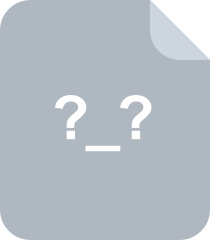
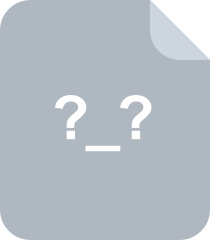
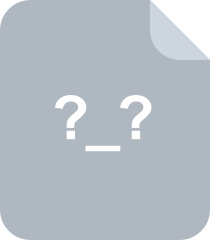
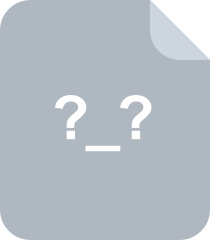
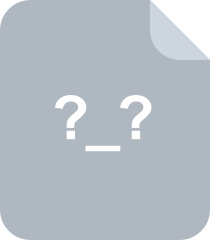
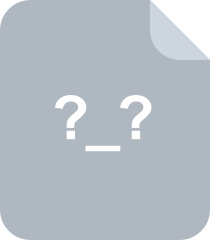
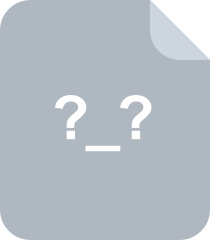
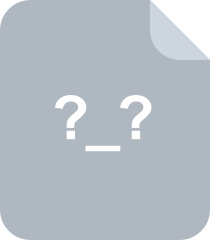
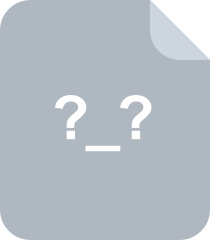
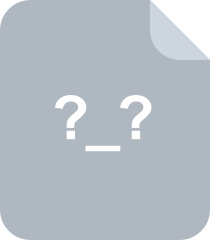
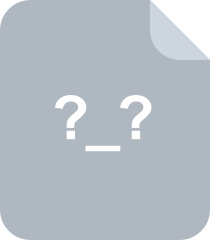
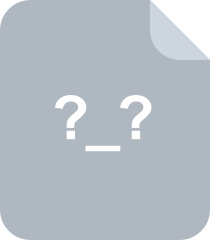
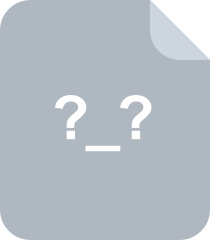
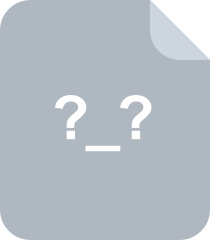
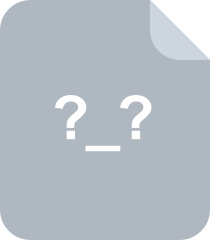
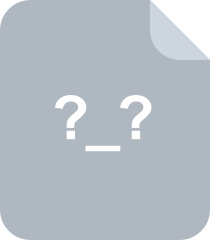
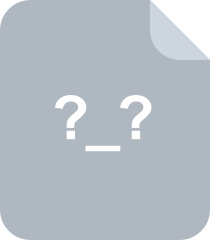
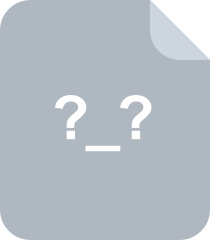
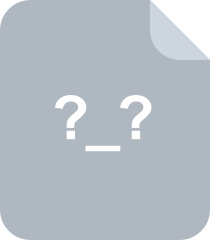
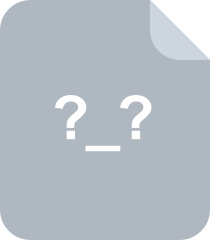
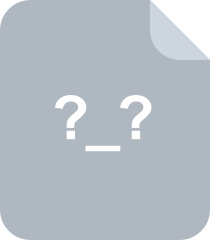
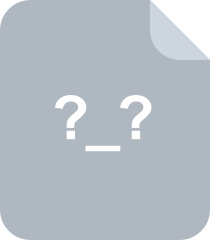
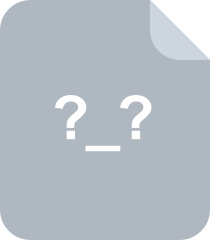
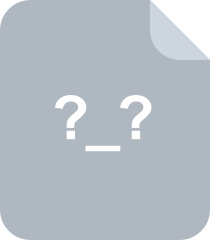
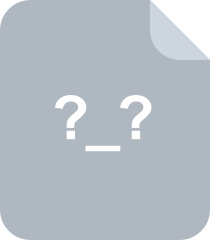
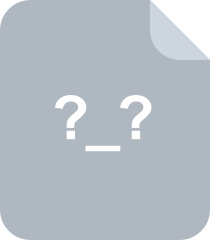
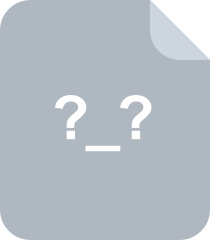
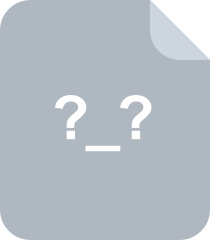
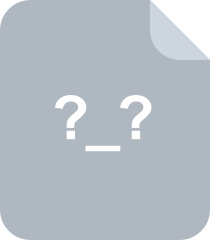
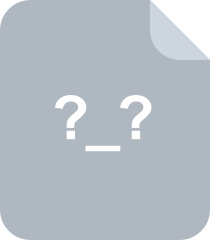
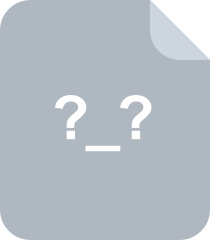
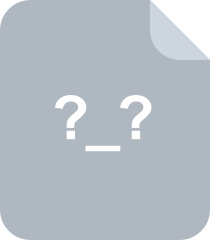
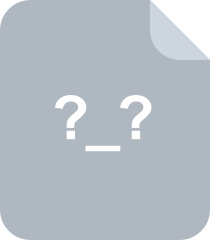
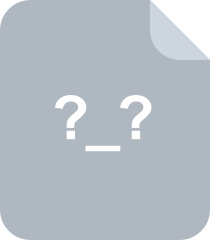
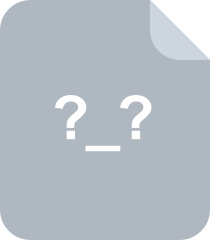
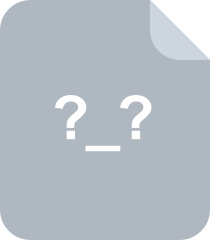
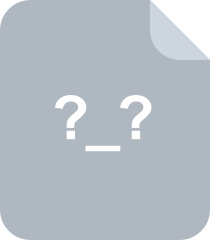
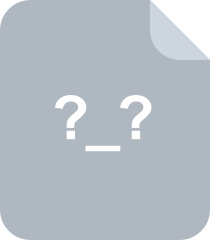
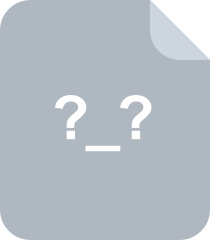
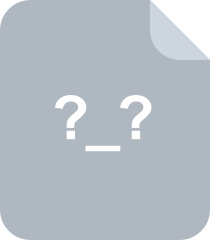
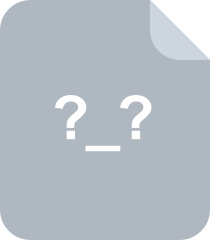
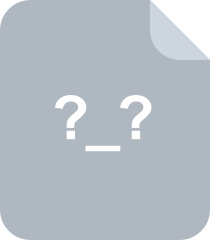
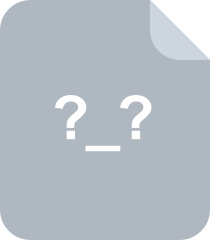
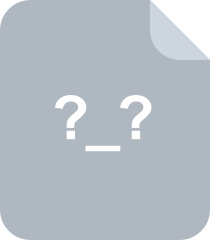
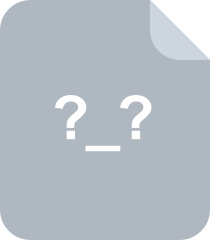
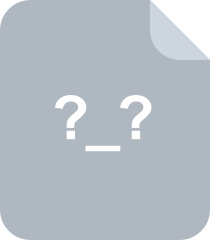
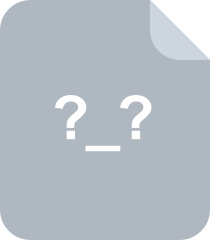
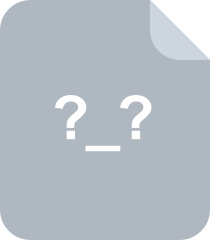
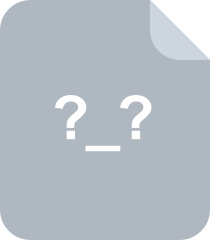
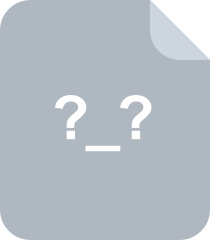
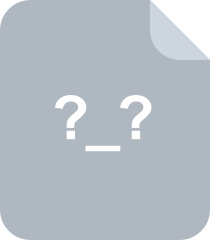
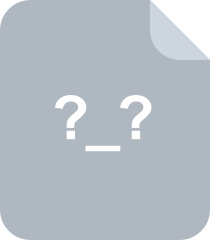
共 391 条
- 1
- 2
- 3
- 4
资源评论
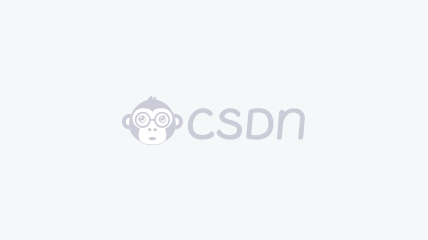

Coder_Kevin_Vans
- 粉丝: 622
- 资源: 73
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

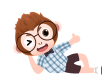
最新资源
- 【Unity 插件】Photon Multiplayer Template (For Game Creator 2)
- 【字幕SRT翻译器】+【支持9种语言】+【大模型翻译,效果一级棒】+【永久不过期】
- PHP站长导航资源网站导航系统源码修复版
- 消息队列中间件RabbitMQ的CentOS环境下安装与配置指南
- yolov6n.onnx
- 高级系统架构设计师下午试题模拟题6套试题.pdf
- 科技公司员工转正评估表.xlsx
- 微观企业劳动力生产率数据(1999-2023年).txt
- CCF大数据竞赛-垃圾短信基于文本内容的识别项目源码(高分项目)
- Linux环境下Nginx服务器的源码安装与自动启动配置指南
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


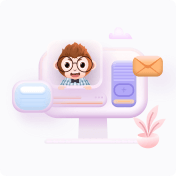
安全验证
文档复制为VIP权益,开通VIP直接复制
