package cn.sjxy.webserver;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.ServerSocket;
import java.net.Socket;
//为了提高服务的效率,把没有用户的请求都包装成一个线程对象
class Tomcat implements Runnable{
private Socket socket;
public Tomcat(Socket socket) {//传递为用户服务的客服对象
this.socket=socket;
}
@Override
public void run() {
//获取输入输出流对象
InputStream in=null;
OutputStream out=null;
try {
in=this.socket.getInputStream();
out=this.socket.getOutputStream();
if(in!=null) {
byte[] buff=new byte[1024];
int len=in.read(buff);
String request=new String(buff,0,len);
//截取该请求的字符串(请求头信息,HTTP)
request=request.substring(0, request.indexOf("\r\n"));
request=request.split(" ")[1];
request=request.substring(1);
System.out.println(request);
//在服务器端查找该请求的资源是否存在,如果存在则输出给客户端,如果不存在给客户一个响应提示信息
//把该资源包装成一个文件
File file=new File("src/"+request);
if(file.exists()) {
//则把该字符以流的形式输出给客户端
FileInputStream fout=new FileInputStream(file);
while((len=fout.read(buff))>0) {
//把读取出的数据写出给客户端
out.write(buff, 0, len);
}
//释放资源
fout.close();
}else {
//给客户端一个输出提示信息
if(out!=null) {
String info="<h1><font color='red'>对不起,您请求的网络资源不存在!</font></h1>";
out.write(info.getBytes());
}
}
}
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally {
if(in!=null) {
try {
in.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
if(out!=null) {
try {
out.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
}
public class TomcatMoni {
public static void main(String[] args) {
// 创建一个服务端的ServerSocket
try {
ServerSocket server=new ServerSocket(8080);
while(true) {//模拟不停的为用户请求服务
//获取为客户请求服务的一个对应Socket
Socket client=server.accept();
//把该socket包装成一个线程
new Thread(new Tomcat(client)).start();
// //获取用户的请求(输入流对象)
// InputStream in=client.getInputStream();
// //获取一个响应的输出流对象(为用户提供输出服务)
// OutputStream out=client.getOutputStream();
// //首先读取用户的请求信息
// byte[] buff=new byte[1024];
// int len=in.read(buff);
// //把读取到的字节数据转换成字符串
// String request=new String(buff,0,len);
// //字符串的截取
// request=request.substring(0, request.indexOf("\r\n"));
// request=request.split(" ")[1];
// request=request.substring(1);
// System.out.println(request);
// //在服务器端查找该请求的资源是否存在,如果存在则输出给客户端,如果不存在给客户一个响应提示信息
// //把该资源包装成一个文件
// File file=new File("src/"+request);
// if(file.exists()) {
// //表示资源存在
// }else {//表示资源不存在
// //给客户端一个输出提示信息
// if(out!=null) {
// String info="<h1><font color='red'>对不起,您请求的网络资源不存在!</font></h1>";
// out.write(info.getBytes());
// }
//
// }
// out.close();
// in.close();
}
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
第一章代码.rar
需积分: 0 151 浏览量
2024-02-29
12:05:52
上传
评论
收藏 4.92MB RAR 举报

m0_75089027
- 粉丝: 0
- 资源: 1
最新资源
- 10Eclipse项目源码.jpg
- 大屏可视化数据课程项目
- Maven 快速入门指南:安装和配置方法详解
- STM32物信息通过MQTT协议上传云平台
- STM32物信息通过MQTT协议上传云平台
- 基于Selenium的Java爬虫实战(内含谷歌浏览器Chrom和Chromedriver版本122.0.6260.0)
- 基于Selenium的Java爬虫实战(内含谷歌浏览器Chrom和Chromedriver版本122.0.6259.0)
- 基于Selenium的Java爬虫实战(内含谷歌浏览器Chrom和Chromedriver版本122.0.6258.0)
- 基于Selenium的Java爬虫实战(内含谷歌浏览器Chrom和Chromedriver版本122.0.6257.0)
- Screenshot_2024_0614_022736.png
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


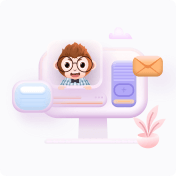