import numpy as np
import random
# 线性数据集、过原点
def get_beans(counts):
xs = np.random.rand(counts)
xs = np.sort(xs)
ys = [1.2 * x + np.random.rand() / 10 for x in xs]
return xs, ys
# 线性数据集、过原点 带正负号
def get_beans2(counts):
xs = np.random.rand(counts) * 10 - 5
xs = np.sort(xs)
ys = [2.3 * x + np.random.rand() for x in xs]
return xs, ys
# 线性数据集、带截距
def get_beans3(counts):
xs = np.random.rand(counts)
xs = np.sort(xs)
ys = np.array([(0.7 * x + (0.5 - np.random.rand()) / 5 + 0.5) for x in xs])
return xs, ys
#
def get_beans4(counts):
xs = np.random.rand(counts)
xs = np.sort(xs)
ys = np.zeros(counts)
for i in range(counts):
x = xs[i]
yi = 0.7 * x + (0.5 - np.random.rand()) / 50 + 0.5
if yi > 0.8:
ys[i] = 1
return xs, ys
def get_beans5(counts):
xs = np.random.rand(counts) * 2
xs = np.sort(xs)
ys = np.zeros(counts)
for i in range(counts):
x = xs[i]
yi = 0.7 * x + (0.5 - np.random.rand()) / 50 + 0.5
if yi > 0.8 and yi < 1.4:
ys[i] = 1
return xs, ys
def get_beans6(counts):
xs = np.random.rand(counts, 2) * 2
ys = np.zeros(counts)
for i in range(counts):
x = xs[i]
if (x[0] - 0.5 * x[1] - 0.1) > 0:
ys[i] = 1
return xs, ys
def get_beans7(counts):
xs = np.random.rand(counts, 2) * 2
ys = np.zeros(counts)
for i in range(counts):
x = xs[i]
if (np.power(x[0] - 1, 2) + np.power(x[1] - 0.3, 2)) < 0.5:
ys[i] = 1
return xs, ys
# 定义函数get_house_prices,参数为样本数量counts
def get_house_prices(counts):
# 生成counts个随机数表示房屋的面积,并赋值给sizes(假设面积在50到200平方米之间)
sizes = np.random.uniform(50, 200, counts)
# 对sizes进行排序
sizes = np.sort(sizes)
# 生成prices,其中每个值为3000倍的sizes加上一个在0到50000之间的随机数
prices = [3000 * size + np.random.uniform(0, 50000) for size in sizes]
# 返回sizes和prices
return sizes, prices
def get_beans8(counts):
posX, posY = genSpiral(int(counts / 2), 0, 1)
negX, negY = genSpiral(int(counts / 2), np.pi, 0)
X = np.vstack((posX, negX))
Y = np.hstack((posY, negY))
return X, Y
def genSpiral(counts, deltaT, label):
X = np.zeros((counts, 2))
Y = np.zeros(counts)
for i in range(counts):
r = i / counts * 5
t = 1.75 * i / counts * 2 * np.pi + deltaT;
x1 = r * np.sin(t) + random.uniform(-0.1, 0.1)
x2 = r * np.cos(t) + random.uniform(-0.1, 0.1)
X[i] = np.array([x1, x2])
Y[i] = label
return X, Y
def dist(a, b):
dx = a['x'] - b['x'];
dy = a['y'] - b['y'];
return np.sqrt(dx * dx + dy * dy);
def getCircleLabel(p, center):
radius = 1;
if dist(p, center) < (radius * 0.5):
return 1
else:
return 0
def randUniform(a=-1, b=1):
return np.random.rand() * (b - a) + a;
def classifyCircleData(numSamples=100, noise=0):
points = [];
Y = []
X = []
radius = 1;
num = int(numSamples / 2)
for i in range(num):
r = randUniform(0, radius * 0.5);
angle = randUniform(0, 2 * np.pi);
x = r * np.sin(angle);
y = r * np.cos(angle);
noiseX = randUniform(-radius, radius) * noise;
noiseY = randUniform(-radius, radius) * noise;
label = getCircleLabel({'x': x + noiseX, 'y': y + noiseY}, {'x': 0, 'y': 0});
X.append([x + 1, y + 1])
Y.append(label)
for i in range(num):
r = randUniform(radius * 0.7, radius);
angle = randUniform(0, 2 * np.pi);
x = r * np.sin(angle);
y = r * np.cos(angle);
noiseX = randUniform(-radius, radius) * noise;
noiseY = randUniform(-radius, radius) * noise;
label = getCircleLabel({'x': x + noiseX, 'y': y + noiseY}, {'x': 0, 'y': 0});
X.append([x + 1, y + 1])
Y.append(label)
X = np.array(X)
Y = np.array(Y)
return X, Y
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
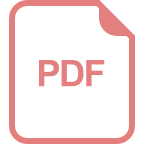
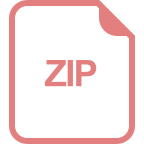
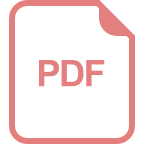
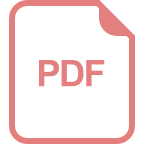
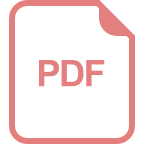
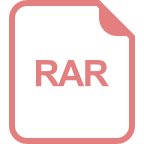
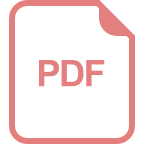
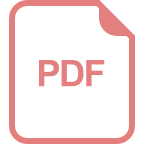
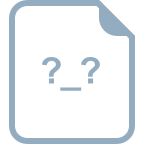
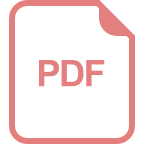
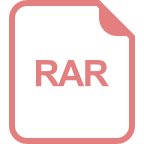
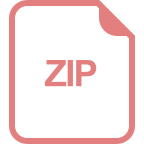
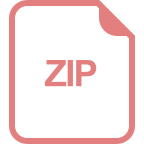
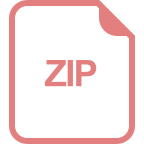
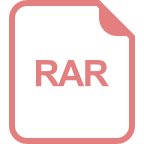
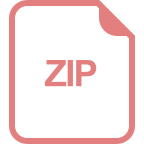
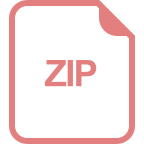
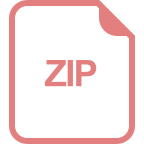
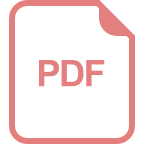
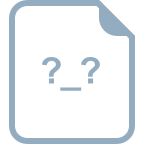
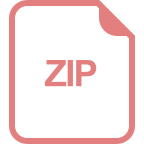
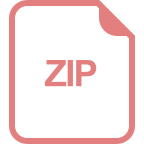
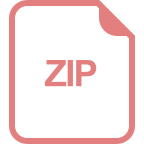
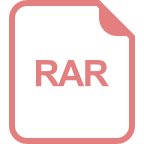
收起资源包目录


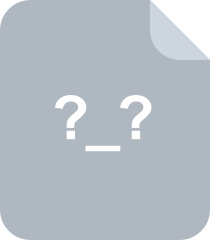
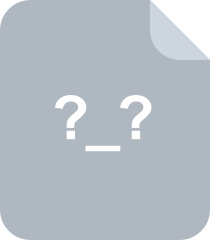
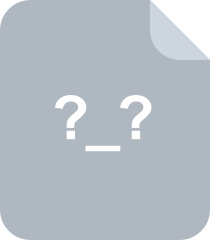
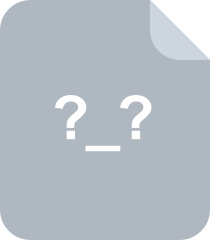

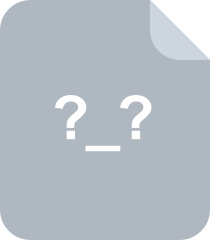
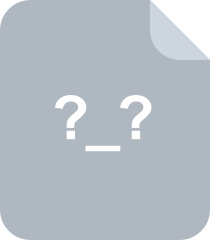
共 6 条
- 1
资源评论
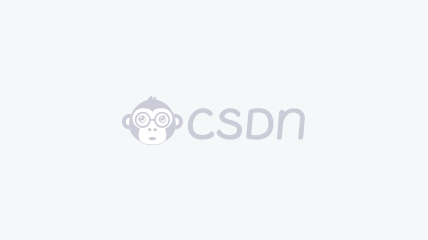

物联通信社区
- 粉丝: 27
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

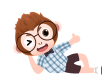
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


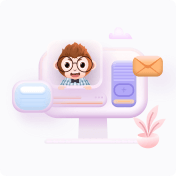
安全验证
文档复制为VIP权益,开通VIP直接复制
