<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1,
minimum-scale=1,maximum-scale=1,user-scalable=no" />
<!--引入CSS代码-->
<style>
* {
margin: 0;
padding: 0;
}
a {
list-style: none;
}
li {
list-style: none;
}
.lunbo {
width: 100%;
}
.content {
width: 800px;
height: 300px;
margin: 20px auto;
position: relative;
background: green;
}
#item {
width: 100%;
height: 100%;
}
.item {
position: absolute;
opacity: 0;
transition: all 1s;
width: 100%;
height: 100%;
}
.item.active {
opacity: 1;
}
img {
width: 100%;
height: 100%;
display: block;
}
#btn-left {
width: 30px;
height: 69px;
font-size: 30px;
color: white;
background-color: rgba(0, 0, 0, 0.4);
line-height: 69px;
padding-left: 5px;
z-index: 10;
/*始终显示在图片的上层*/
position: absolute;
left: 0;
top: 50%;
transform: translateY(-60%);
/*使按钮向上偏移居中对齐*/
cursor: pointer;
opacity: 0;
/*平时隐藏*/
}
.lunbo:hover #btn-left {
/*鼠标滑入,显示图标*/
opacity: 1;
}
#btn-right {
width: 26px;
height: 69px;
font-size: 30px;
color: white;
background-color: rgba(0, 0, 0, 0.4);
line-height: 69px;
padding-left: 5px;
z-index: 10;
position: absolute;
right: 0;
top: 50%;
cursor: pointer;
opacity: 0;
transform: translateY(-60%);
}
.lunbo:hover #btn-right {
opacity: 1;
}
#circle {
height: 20px;
display: flex;
position: absolute;
bottom: 35px;
right: 25px;
}
.circle {
width: 10px;
height: 10px;
border-radius: 10px;
border: 2px solid white;
background-color: black;
cursor: pointer;
margin: 5px;
}
.white {
background-color: #FFFFFF;
}
</style>
<!--引入Js代码-->
<title>Js实现轮播图</title>
</head>
<body>
<div class="lunbo">
<div class="content">
<ul id="item">
<li class="item">
<a href="#"><img src="img/1.jpg"></a>
</li>
<li class="item">
<a href="#"><img src="img/2.jpg"></a>
</li>
<li class="item">
<a href="#"><img src="img/3.jpg"></a>
</li>
<li class="item">
<a href="#"><img src="img/4.jpg"></a>
</li>
<li class="item">
<a href="#"><img src="img/5.jpg"></a>
</li>
</ul>
<div id="btn-left">
< </div>
<div id="btn-right">></div>
<ul id="circle">
<li class="circle"></li>
<li class="circle"></li>
<li class="circle"></li>
<li class="circle"></li>
<li class="circle"></li>
</ul>
</div>
</div>
<script>
window.onload = function() {
var index = 0
var itemul = document.getElementById("item")
var itens = itemul.getElementsByTagName("li")
var left = document.getElementById("btn-left")
var right = document.getElementById("btn-right")
var circleul = document.getElementById("circle")
var circles = circleul.getElementsByTagName("li")
itens[index].style.opacity = 1
circles[index].style.backgroundColor = "#fff"
//圆点点击事件
for (var i = 0; i < circles.length; i++) {
circles[i].index = i
circles[i].onclick = function() {
this.style.backgroundColor = "#fff" //设置选择的圆点为白色
console.log(this.index)
itens[this.index].style.opacity = 1
if (index != 0) {
itens[index].style.opacity = 0 //把之前的图片设置为透明
circles[index].style.backgroundColor = "black"
}
circles[index].style.backgroundColor = "black"
index = this.index
}
}
left.onclick = function() {
circles[index].style.backgroundColor = "black" //上一次的圆标设置为黑色
itens[index].style.opacity = 0 //把之前的图片设置为透明
if (index == 0) {
index = 4
itens[index].style.opacity = 1 //把当前的图片设置为不透明
circles[index].style.backgroundColor = "#fff" //当前的圆标设置为白色
} else {
index--
itens[index].style.opacity = 1 //把当前的图片设置为不透明
circles[index].style.backgroundColor = "#fff" //当前的圆标设置为白色
}
}
right.onclick = function() {
circles[index].style.backgroundColor = "black" //上一次的圆标设置为黑色
itens[index].style.opacity = 0 //把之前的图片设置为透明
if (index == 4) {
index = 0
itens[index].style.opacity = 1 //把当前的图片设置为不透明
circles[index].style.backgroundColor = "#fff" //当前的圆标设置为白色
} else {
index++
itens[index].style.opacity = 1 //把当前的图片设置为不透明
circles[index].style.backgroundColor = "#fff" //当前的圆标设置为白色
}
console.log(index)
}
for (var i = 0; i < itens.length; i++) {
itens[i].index = i
itens[i].onmouseover = function() {
}
}
var intTime = setInterval(clock, 2000)
function clock() {
circles[index].style.backgroundColor = "black" //上一次的圆标设置为黑色
itens[index].style.opacity = 0 //把之前的图片设置为透明
if (index == 4) {
index = 0
} else {
index++
}
circles[index].style.backgroundColor = "#fff" //当前的圆标设置为白色
itens[index].style.opacity = 1 //把当前的图片设置为不透明
}
itemul.onmouseout = function() {
intTime = setInterval(clock, 2000)
}
itemul.onmouseover = function(e) {
clearInterval(intTime)
}
circleul.onmouseout = function() {
intTime = setInterval(clock, 2000)
}
circleul.onmouseover = function(e) {
clearInterval(intTime)
}
left.onmouseout = function() {
intTime = setInterval(clock, 2000)
}
left.onmouseover = function(e) {
clearInterval(intTime)
}
right.onmouseout = function() {
intTime = setInterval(clock, 2000)
}
right.onmouseover = function(e) {
clearInterval(intTime)
}
}
</script>
</body>
</html>

河里的鲨鱼
- 粉丝: 6
- 资源: 4
最新资源
- 1960-2023年世界各国相关指标数据集指标
- 吉林大学计算机网络期末复习(自用)
- 图像分割数据集:自动驾驶车道线虚线、实线语义分割数据集(3类分割,约1300张数据和标签)
- 微信小程序云课堂平台的系统设计与功能实现研究
- 心力衰竭临床记录,python实现代码 数据和展示
- 新年计算机资源整合:优化生活环境与自我提升
- 2025最新AI源码开源版,chat开源版,AI翻译,语音图生
- 基于 DenseUnet 对自动驾驶车道线虚线、实线图像分割实战【包含代码+完整数据集】
- 计算机专业学习路径:资源推荐、学习策略与实践指导
- 运营版【白娘子传奇】手游新手架设教程视频+最新完整手工端
- LSSVM,SSA-LSSVM,VMD-LSSVM,VMD-SSA-LSSVM四种算法做短期电力负荷预测,做对比 结果分析-lssvm 均方根误差(RMSE):0.79172 平均绝对误差(MAE)
- ASP.NET实现的班级特色网站(源码+论文).zip
- Boost二级升压光伏并网结构,Simulink建模,MPPT最大功率点追踪,扰动观察法采用功率反馈方式,若ΔP>0,说明电压调整的方向正确,可以继续按原方向进行“干扰”;若ΔP<0,说明电压调整的方
- 基于Java的在线图书商城系统开发与实现-涵盖前后端与数据库设计
- 质心侧偏角相平面simulink程序 横摆角速度相平面simulink程序 相平面法 “质心侧偏角-横摆角速度” 可根据不同的速度,路面附着系数和前轮轮转角生成相平面 包含m文件,slx文件以及相关参
- Java编程环境下的医院信息管理系统的开发设计与应用实践
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


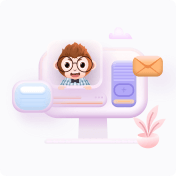