package com.example.app_mqtt;
import androidx.appcompat.app.AppCompatActivity;
import android.annotation.SuppressLint;
import android.os.AsyncTask;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.CompoundButton;
import android.widget.Switch;
import android.widget.TextView;
import org.eclipse.paho.client.mqttv3.IMqttDeliveryToken;
import org.eclipse.paho.client.mqttv3.MqttCallback;
import org.eclipse.paho.client.mqttv3.MqttClient;
import org.eclipse.paho.client.mqttv3.MqttConnectOptions;
import org.eclipse.paho.client.mqttv3.MqttException;
import org.eclipse.paho.client.mqttv3.MqttMessage;
import org.eclipse.paho.client.mqttv3.MqttPersistenceException;
import org.eclipse.paho.client.mqttv3.MqttTopic;
import org.eclipse.paho.client.mqttv3.persist.MemoryPersistence;
import org.w3c.dom.Text;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.TimeUnit;
public class MainActivity extends AppCompatActivity {
private String productKey="h8iz2jQxPhW";
private String deviceName="AppContent";
private String deviceSecret="3b38d82aa26f77db1aaf1fa18a362035";
//tpioc 上传定义
final String led_up = "LED_STATE_UPDATA";
final String door_up = "DOOR_STATE_UPDATA";
final String curtin_up = "CURTIN_STATE_UPDATA";
final String window_up = "WINDOW_STATE_UPDATA";
final String fan_up = "FAN_STATE_UPDATA";
final String lighbelt_up = "LightBelt_STATE_UPDATA";
final String updata_up = "UPDATA_STATE_UPDATA";//用于手动更新数据
//tpioc 接收定义
final String temp_re = "TEMP_STATE_RECEIVE";
final String hum_re = "HUM_STATE_RECEIVE";
final String smoke_re = "SMOKE_STATE_RECEIVE";
final String rain_re = "RAIN_STATE_RECEIVE";
final String entry_re = "EntryNotice_STATE_RECEIVE";
final String led_re = "LED_STATE_RECEIVE";
final String door_re = "DOOR_STATE_RECEIVE";
final String curtin_re = "CURTIN_STATE_RECEIVE";
final String window_re = "WINDOW_STATE_RECEIVE";
final String fan_re = "FAN_STATE_RECEIVE";
final String lighbelt_re = "LightBelt_STATE_RECEIVE";
private MqttClient mqttClient=null;
private MqttConnectOptions connOpts= null;
private static MemoryPersistence memoryPersistence = null;
private TextView notice_text,temp_text,hum_text,Text_text;
private Switch lamp_switch,door_switch,fan_switch,window_switch,curtain_switch,LightBelt_switch;
private Button btu_updata,but_connect;
private String[] notice_data = new String[3];//通知消息缓存
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
notice_text = findViewById(R.id.notice_text);
temp_text = findViewById(R.id.temp_text);
hum_text = findViewById(R.id.hum_text);
lamp_switch = findViewById(R.id.lamp_switch);
door_switch = findViewById(R.id.door_switch);
fan_switch = findViewById(R.id.fan_switch);
window_switch = findViewById(R.id.window_switch);
curtain_switch = findViewById(R.id.curtain_switch);
LightBelt_switch = findViewById(R.id.LightBelt_switch);
btu_updata = findViewById(R.id.btu_updata);
but_connect = findViewById(R.id.btu_connect);
Text_text = findViewById(R.id.Test_test);
notice_data[0]=notice_data[1]=notice_data[2]="";
control_response();
but_connect.setText("Not connected");
but_connect.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
if(but_connect.getText().equals("Not connected")){
MqttInit();
but_connect.setText("connected");
}else{
mqtt_close();
but_connect.setText("Not connected");
}
}
});
MqttInit();//连接mqtt 并订阅主题
}
/*
initAliyunIoTClient :用户将三个参数转换为用户的账号和密码
*/
private void initAliyunIoTClient(String productKey,String deviceName,String deviceSecret) {
try {
String clientId = "12345"+ System.currentTimeMillis();
Map<String, String> params = new HashMap<String, String>(16);
params.put("productKey", productKey);
params.put("deviceName", deviceName);
params.put("clientId", clientId);
String timestamp = String.valueOf(System.currentTimeMillis());
params.put("timestamp", timestamp);
// cn-shanghai
String targetServer ="tcp://"+ productKey + ".iot-as-mqtt.cn-shanghai.aliyuncs.com:1883";
String mqttclientId = clientId + "|securemode=3,signmethod=hmacsha1,timestamp=" + timestamp + "|";
String mqttUsername = deviceName + "&" + productKey;
String mqttPassword = AliyunIoTSignUtil.sign(params, deviceSecret, "hmacsha1");
connectMqtt(targetServer, mqttclientId, mqttUsername, mqttPassword);
} catch (Exception e) {
e.printStackTrace();
}
}
/*
connectMqtt:用于连接MQTT
*/
public void connectMqtt(String url, String clientId, String mqttUsername, String mqttPassword) throws Exception {
MemoryPersistence persistence = new MemoryPersistence();
mqttClient = new MqttClient(url, clientId, persistence);
connOpts = new MqttConnectOptions();
// MQTT 3.1.1
connOpts.setMqttVersion(4);
connOpts.setAutomaticReconnect(true);
connOpts.setCleanSession(false);
connOpts.setUserName(mqttUsername);
connOpts.setPassword(mqttPassword.toCharArray());
connOpts.setKeepAliveInterval(60);
mqttClient.connect(connOpts);
memoryPersistence =new MemoryPersistence();
}
/*
发布主题
函数参数: pubTopic:要发布的主题
payload: 发的信息
说明:这里发送的信息 应该为数字字符串 没有做判断
*/
private void postDeviceProperties1(String pubTopic ,String payload) {
if(mqttClient.isConnected()) {
try {
MqttMessage message = new MqttMessage();
message.setQos(1);//可靠发送
MqttTopic topic = mqttClient.getTopic("/" + productKey + "/" + deviceName + "/" + "user" + "/" + pubTopic);
message.setPayload(payload.getBytes());
if (null != topic) {
topic.publish(message);
}
} catch (Exception e) {
e.printStackTrace();
}
}else{
reConnect();
}
}
private void MqttInit(){
initAliyunIoTClient(productKey,deviceName,deviceSecret);
do{
} while(!mqttClient.isConnected());
SubscribeTopic_init();
//设置回调函数
mqttClient.setCallback(new MqttCallback() {
@Override
public void connectionLost(Throwable throwable) {
}
@Override
public void messageArrived(String s, MqttMessage mqttMessage) throws Exception {
ReData_Handler(s,new String(mqttMessage.getPayload()));
}
@Override
public void deliveryComplete(IMqttDeliveryToken iMqttDeliveryToken) {
try {
iMqttDeliveryToken.waitForCompletion();
} catch (MqttException e) {
e.printStackTrace();
}
}
});
}
//关闭连接
private void mqtt_close(){
if(null != memoryPersistence) {
try {
memoryPersistence.close();
} catch (MqttPersistenceException e) {
e.printStackTrace();
}
}
if(mq
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
<项目介绍> 毕业设计-基于Stm32实现的智能家居控制系统源码+文档说明+博客说明+接线图 - 不懂运行,下载完可以私聊问,可远程教学 该资源内项目源码是个人的毕设,代码都测试ok,都是运行成功后才上传资源,答辩评审平均分达到96分,放心下载使用! 1、该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的,请放心下载使用! 2、本项目适合计算机相关专业(如计科、人工智能、通信工程、自动化、电子信息等)的在校学生、老师或者企业员工下载学习,也适合小白学习进阶,当然也可作为毕设项目、课程设计、作业、项目初期立项演示等。 3、如果基础还行,也可在此代码基础上进行修改,以实现其他功能,也可用于毕设、课设、作业等。 下载后请首先打开README.md文件(如有),仅供学习参考, 切勿用于商业用途。 --------
资源推荐
资源详情
资源评论
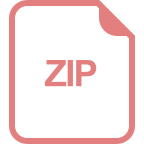
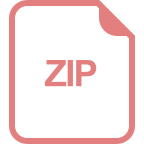
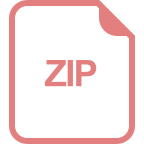
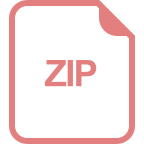
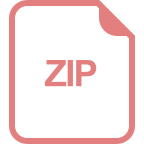
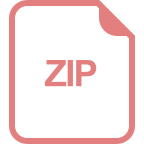
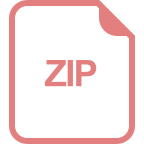
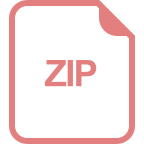
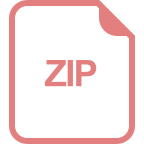
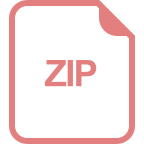
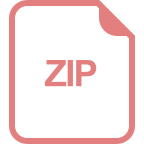
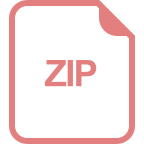
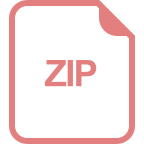
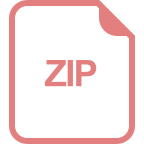
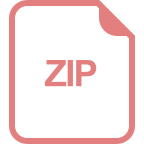
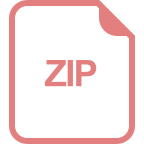
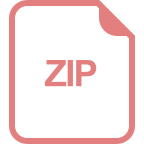
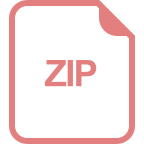
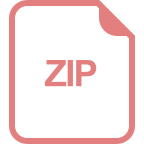
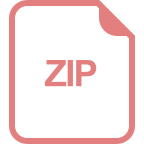
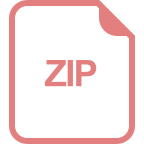
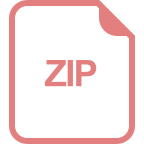
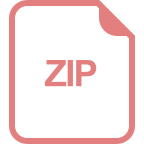
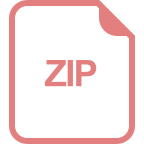
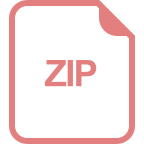
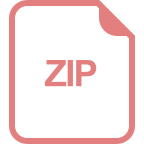
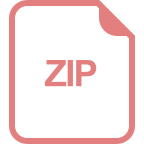
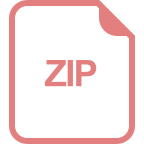
收起资源包目录

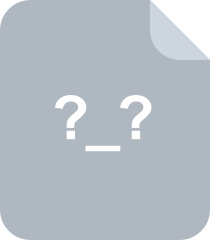
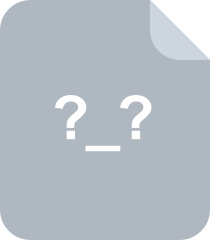
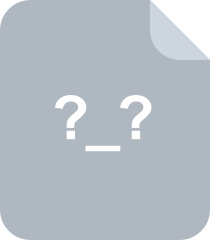
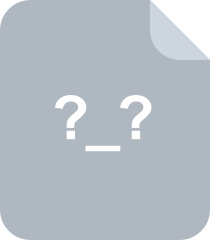
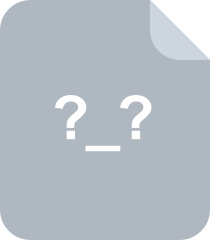
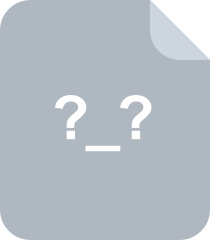
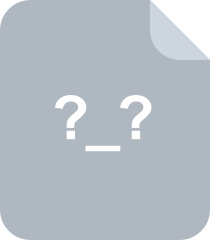
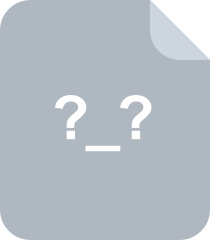
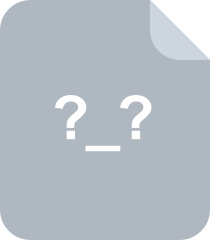
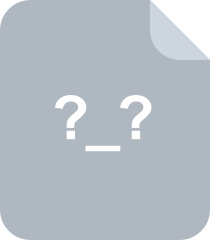
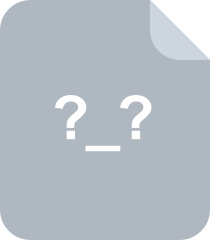
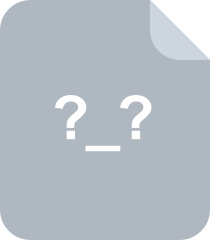
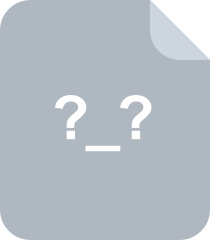
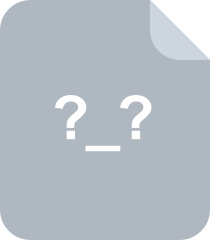
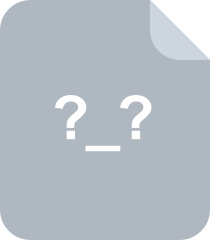
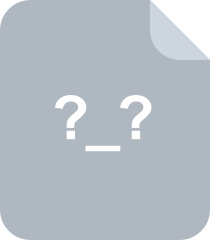
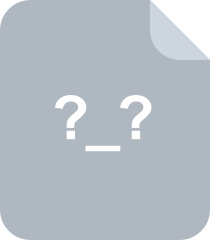
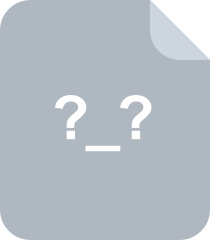
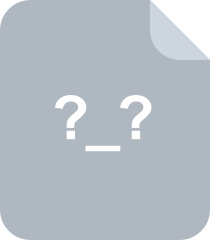
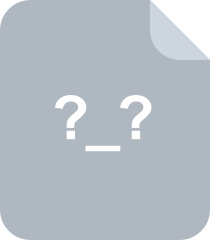
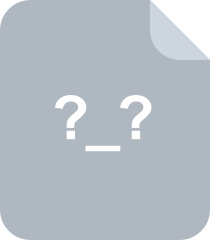
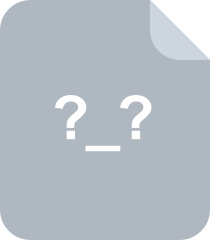
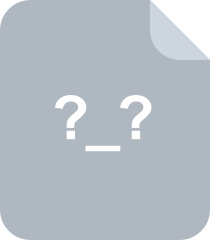
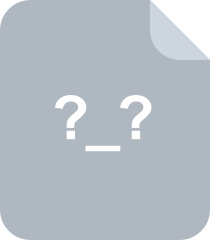
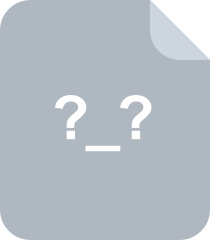
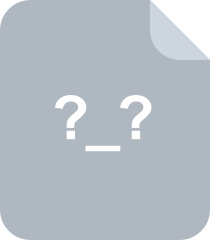
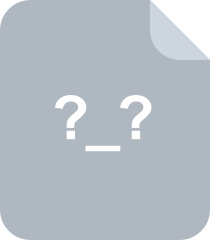
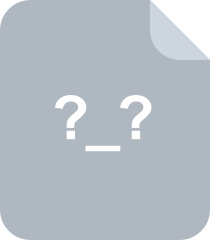
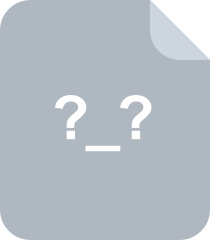
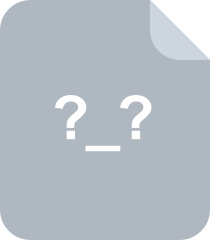
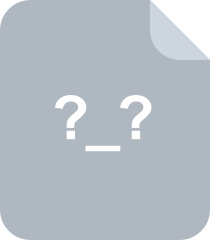
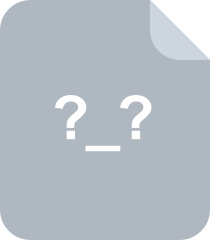
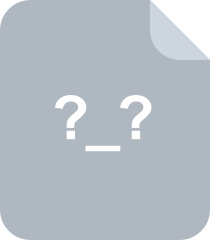
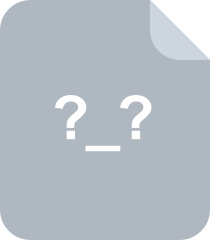
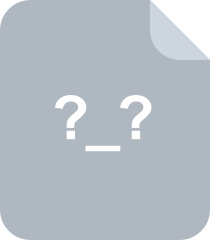
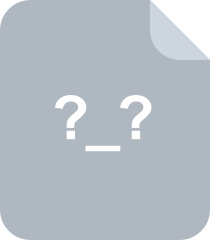
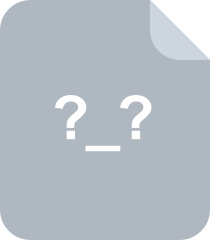
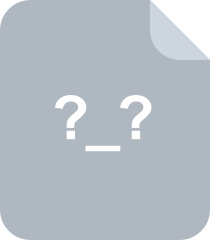
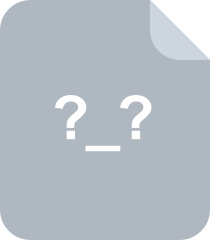
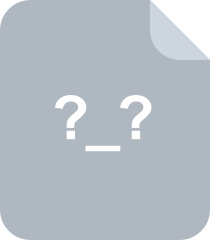
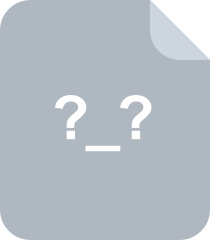
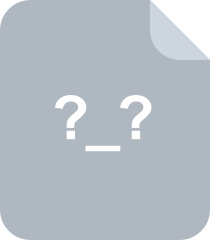
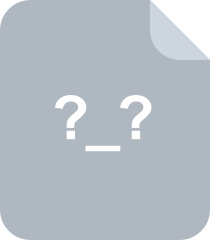
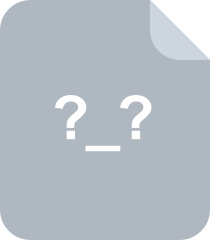
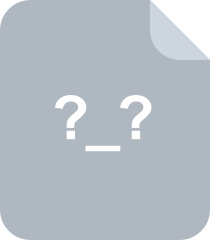
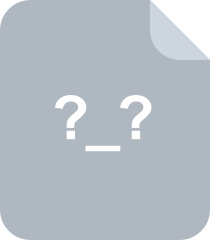
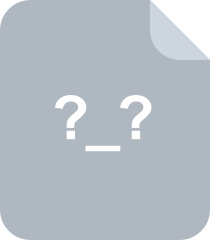
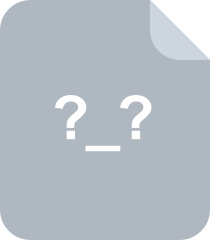
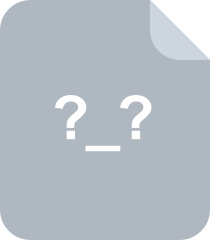
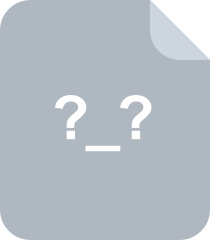
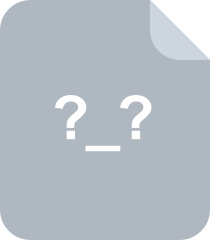
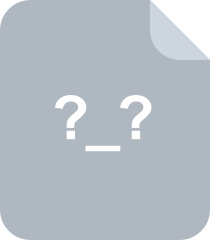
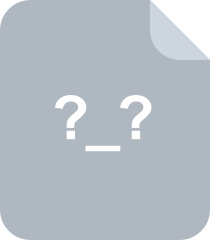
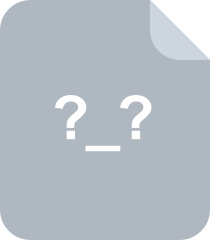
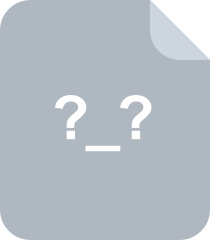
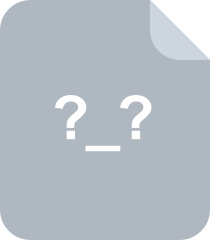
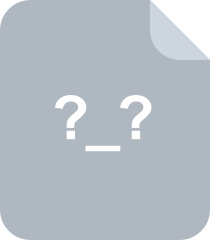
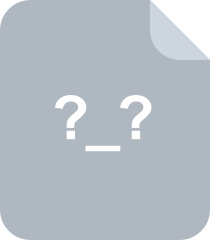
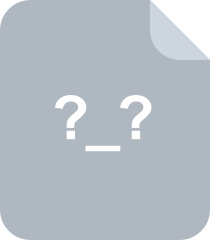
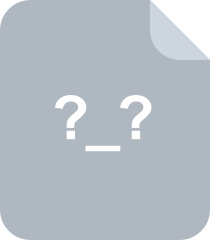
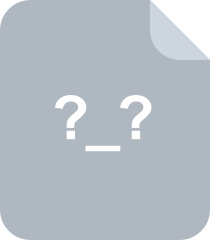
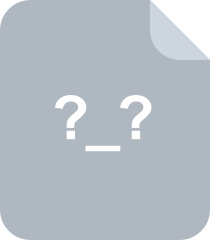
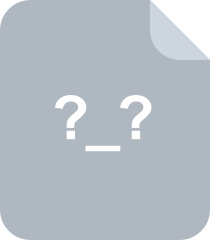
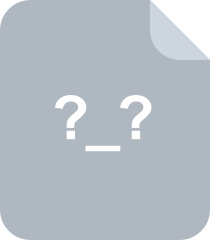
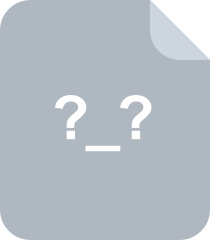
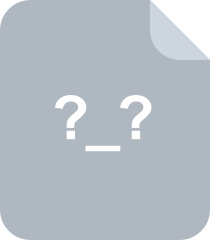
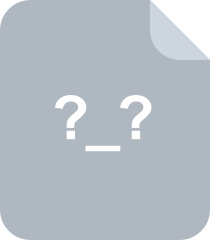
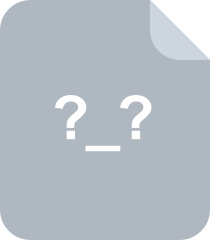
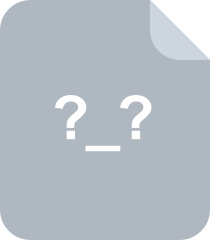
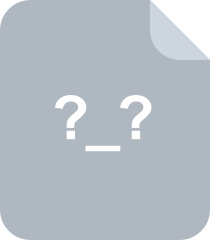
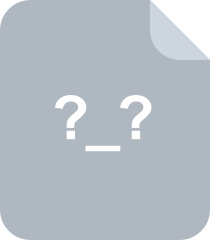
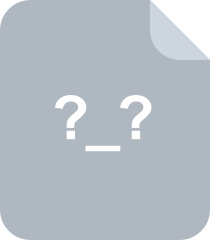
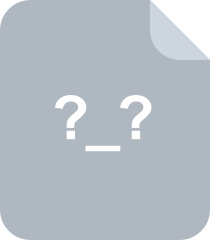
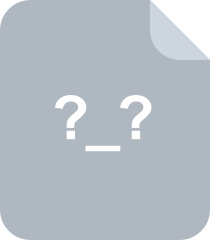
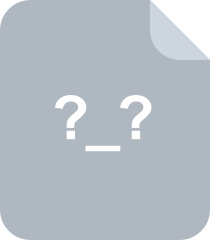
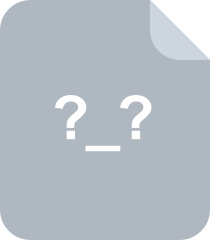
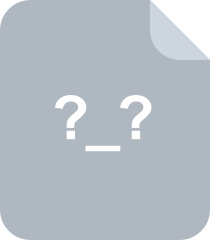
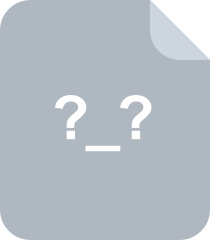
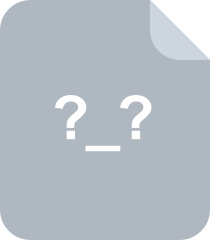
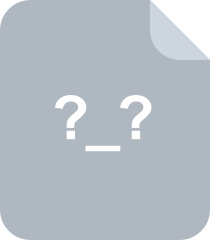
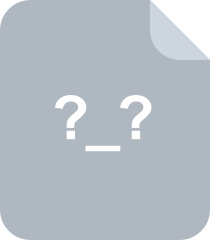
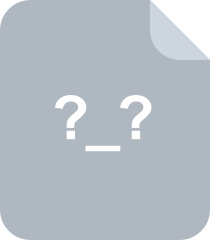
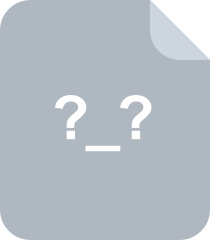
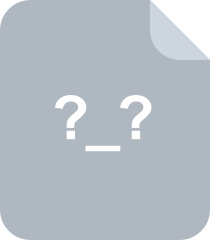
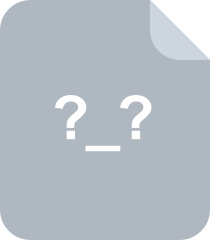
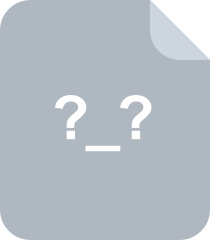
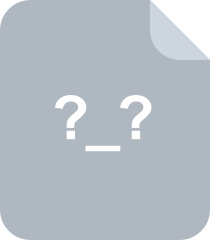
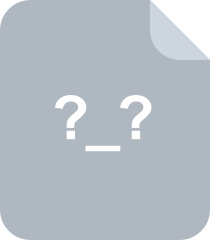
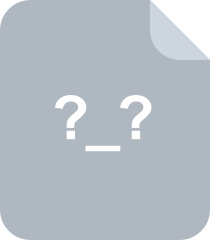
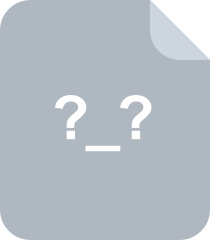
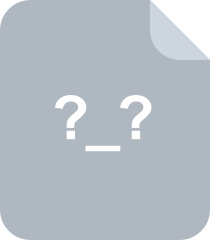
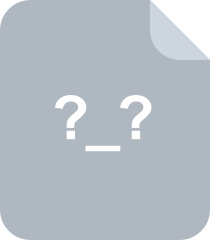
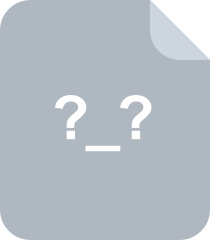
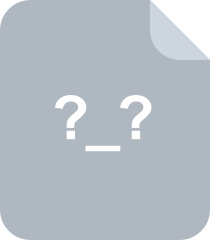
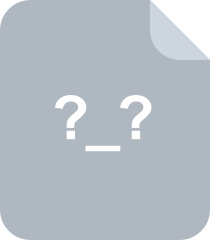
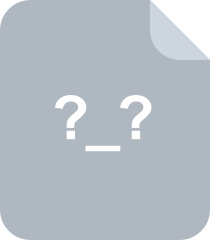
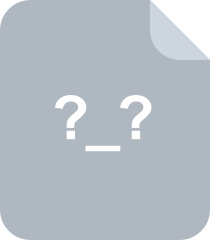
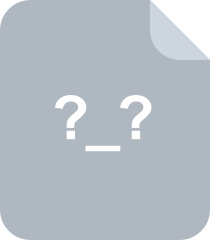
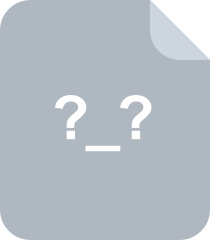
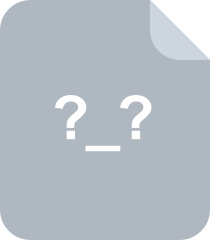
共 706 条
- 1
- 2
- 3
- 4
- 5
- 6
- 8
资源评论
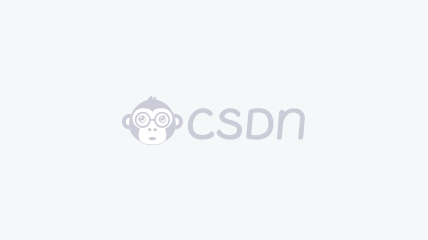

机智的程序员zero
- 粉丝: 2416
- 资源: 4812
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

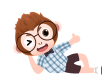
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


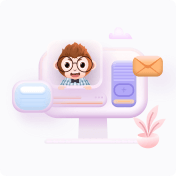
安全验证
文档复制为VIP权益,开通VIP直接复制
