package aos.framework.core.utils;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileWriter;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.StringReader;
import java.io.StringWriter;
import java.io.UnsupportedEncodingException;
import java.net.InetAddress;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLEncoder;
import java.net.UnknownHostException;
import java.security.CodeSource;
import java.security.ProtectionDomain;
import java.sql.Timestamp;
import java.text.ParseException;
import java.text.ParsePosition;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Collection;
import java.util.Date;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Random;
import org.apache.commons.beanutils.BeanUtils;
import org.apache.commons.beanutils.BeanUtilsBean;
import org.apache.commons.beanutils.ConvertUtils;
import org.apache.commons.collections.ListUtils;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.collect.Lists;
import aos.framework.core.exception.AOSException;
import aos.framework.core.typewrap.Dto;
import aos.framework.core.typewrap.Dtos;
import aos.framework.core.typewrap.utils.TypeConvertUtil;
import aos.framework.core.velocity.VelocityHelper;
/**
* <b>辅助工具类</b>
*
* @author xiongchun
* @since 1.0
* @date 2009-1-22
*/
@SuppressWarnings("all")
public class AOSUtils {
private static Logger log = LoggerFactory.getLogger(AOSUtils.class);
/**
* 判断对象是否Empty(null或元素为0)<br>
* 实用于对如下对象做判断:String Collection及其子类 Map及其子类
*
* @param pObj
* 待检查对象
* @return boolean 返回的布尔值
*/
public static boolean isEmpty(Object pObj) {
if (pObj == null)
return true;
if (pObj == "")
return true;
if (pObj instanceof String) {
if (((String) pObj).length() == 0) {
return true;
}
} else if (pObj instanceof Collection) {
if (((Collection) pObj).size() == 0) {
return true;
}
} else if (pObj instanceof Map) {
if (((Map) pObj).size() == 0) {
return true;
}
}
return false;
}
/**
* 判断对象是否为NotEmpty(!null或元素>0)<br>
* 实用于对如下对象做判断:String Collection及其子类 Map及其子类
*
* @param pObj
* 待检查对象
* @return boolean 返回的布尔值
*/
public static boolean isNotEmpty(Object pObj) {
if (pObj == null)
return false;
if (pObj == "")
return false;
if (pObj instanceof String) {
if (((String) pObj).length() == 0) {
return false;
}
} else if (pObj instanceof Collection) {
if (((Collection) pObj).size() == 0) {
return false;
}
} else if (pObj instanceof Map) {
if (((Map) pObj).size() == 0) {
return false;
}
}
return true;
}
/**
* Java对象之间属性值拷贝(Dto、Map、JavaBean)
*
* @param pFromObj
* Bean源对象
* @param pToObj
* Bean目标对象
*/
public static void copyProperties(Object pFromObj, Object pToObj) {
if (pToObj != null) {
try {
// 支持属性空值复制
BeanUtilsBean.getInstance().getConvertUtils().register(false, true, 0);
// 日期类型复制
BeanUtilsDateConverter converter = new BeanUtilsDateConverter();
ConvertUtils.register(converter, java.util.Date.class);
ConvertUtils.register(converter, java.sql.Date.class);
BeanUtils.copyProperties(pToObj, pFromObj);
} catch (Exception e) {
throw new AOSException("JavaBean之间的属性值拷贝发生错误", e);
}
}
}
/**
* 将JavaBean对象中的属性值拷贝到Dto对象
*
* @param pFromObj
* JavaBean对象源
* @param pToDto
* Dto目标对象
*/
public static void copyProperties(Object pFromObj, Dto pToDto) {
if (pToDto != null) {
try {
pToDto.putAll(BeanUtils.describe(pFromObj));
pToDto.remove("class");
} catch (Exception e) {
throw new AOSException("将JavaBean属性值拷贝到Dto对象发生错误", e);
}
}
}
/**
* 将传入的身份证号码进行校验,并返回一个对应的18位身份证
*
* @param personIDCode
* 身份证号码
* @return String 十八位身份证号码
* @throws 无效的身份证号
*/
public static String getFixedPersonIDCode(String personIDCode) {
if (personIDCode == null)
throw new AOSException("输入的身份证号无效,请检查");
if (personIDCode.length() == 18) {
if (isIdentity(personIDCode))
return personIDCode;
else
throw new AOSException("输入的身份证号无效,请检查");
} else if (personIDCode.length() == 15)
return fixPersonIDCodeWithCheck(personIDCode);
else
throw new AOSException("输入的身份证号无效,请检查");
}
/**
* 修补15位居民身份证号码为18位,并校验15位身份证有效性
*
* @param personIDCode
* 十五位身份证号码
* @return String 十八位身份证号码
* @throws 无效的身份证号
*/
public static String fixPersonIDCodeWithCheck(String personIDCode) {
if (personIDCode == null || personIDCode.trim().length() != 15)
throw new AOSException("输入的身份证号不足15位,请检查");
if (!isIdentity(personIDCode))
throw new AOSException("输入的身份证号无效,请检查");
return fixPersonIDCodeWithoutCheck(personIDCode);
}
/**
* 修补15位居民身份证号码为18位,不校验身份证有效性
*
* @param personIDCode
* 十五位身份证号码
* @return 十八位身份证号码
* @throws 身份证号参数不是15位
*/
public static String fixPersonIDCodeWithoutCheck(String personIDCode) {
String id17 = null;
if(personIDCode != null && personIDCode.length() == 17)
id17 = personIDCode;
else if (personIDCode != null || personIDCode.trim().length() == 15)
id17 = personIDCode.substring(0, 6) + "19" + personIDCode.substring(6, 15); // 15位身份证补'19'
else
throw new AOSException("输入的身份证号不足15位,请检查");
char[] code = { '1', '0', 'X', '9', '8', '7', '6', '5', '4', '3', '2' }; // 11个校验码字符
int[] factor = { 7, 9, 10, 5, 8, 4, 2, 1, 6, 3, 7, 9, 10, 5, 8, 4, 2, 1 }; // 18个加权因子
int[] idcd = new int[18];
int sum; // 根据公式 ∑(ai×Wi) 计算
int remainder; // 第18位校验码
for (int i = 0; i < 17; i++) {
idcd[i] = Integer.parseInt(id17.substring(i, i + 1));
}
sum = 0;
for (int i = 0; i < 17; i++) {
sum = sum + idcd[i] * factor[i];
}
remainder = sum % 11;
String lastCheckBit = String.valueOf(code[remainder]);
return id17 + lastCheckBit;
}
/**
* 判断是否是有效的18位或15位居民身份证号码
*
* @param identity
* 18位或15位居民身份证号码
* @return 是否为有效的身份证号码
*/
public static boolean isIdentity(String identity) {
if (identity == null)
return false;
if (identity.length() == 18 || identity.length() == 15) {
String id15 = null;
String id17 = null;
if (identity.length() == 18){
id17 = identity.substring(0, 17);
id15 = identity.substring(0, 6) + identity.substring(8, 17);
}
else
id15 = identity;
try {
Long.parseLong(id15); // 校验是否为数字字符串
String birthday = "19" + id15.substring(6, 12);
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMdd");
sdf.parse(birthday); // 校验出生日期
if (identity.length() == 18 && !fixPersonIDCodeWithoutCheck(id17).equals(identity))
return false; // 校验18位身份证
} catch (Exception e) {
return false;
}
return true;
} else
return false;
}
/**
* 从身份证号中获取出生日期,身份证号可以为15位或18位
*
* @p
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
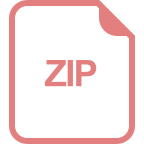
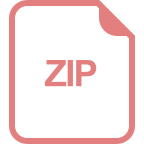
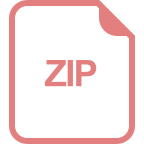
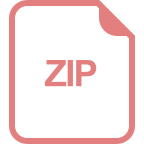
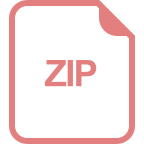
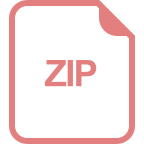
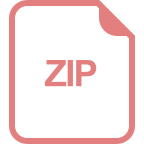
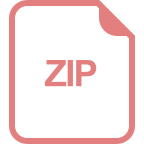
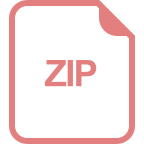
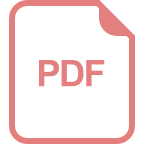
收起资源包目录

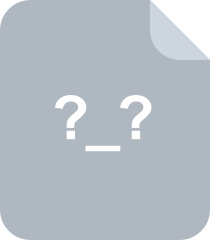
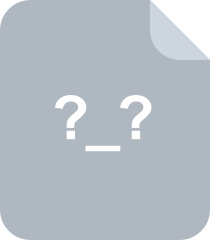
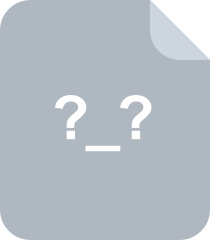
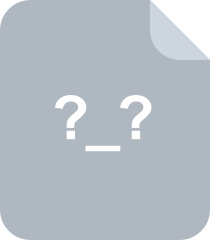
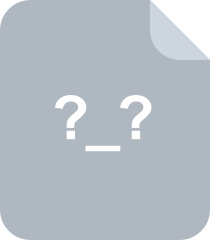
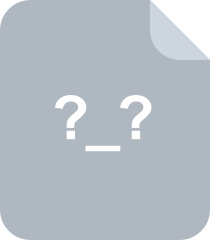
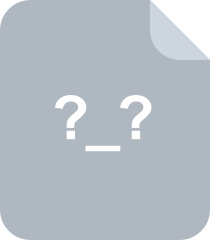
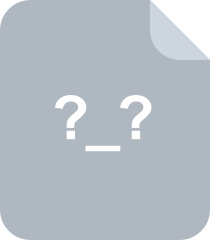
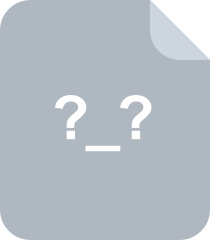
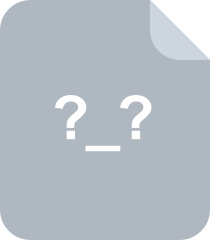
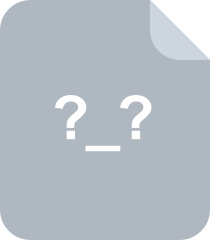
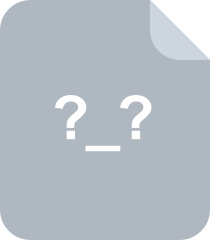
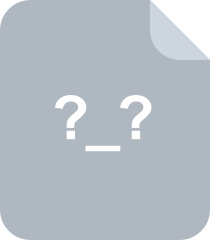
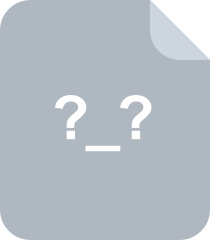
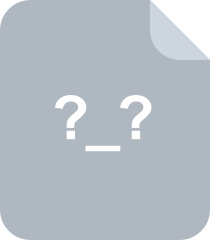
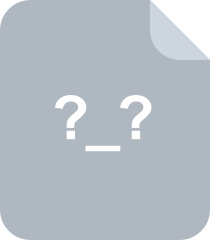
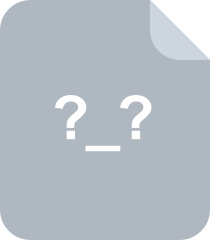
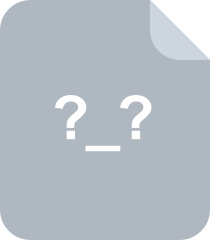
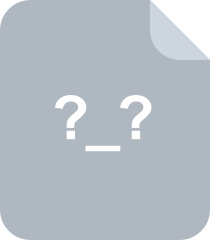
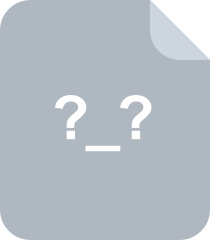
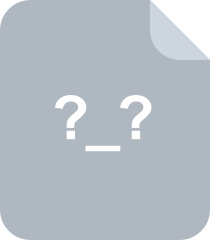
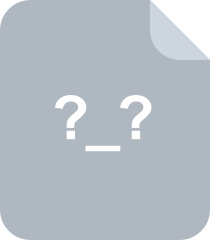
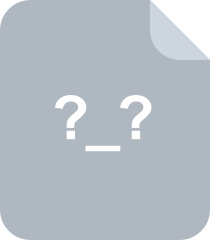
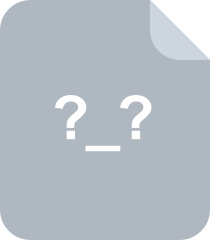
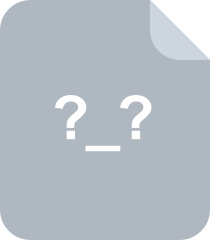
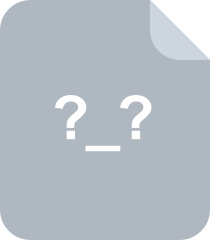
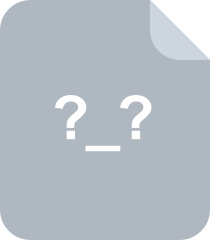
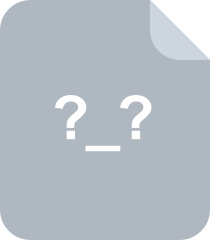
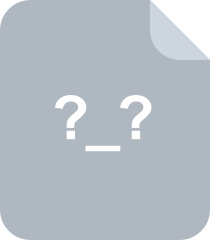
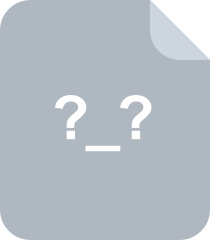
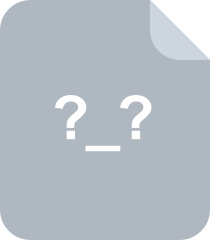
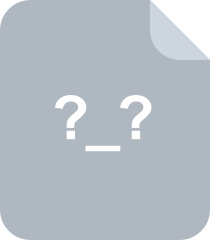
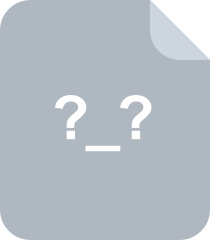
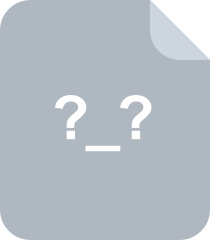
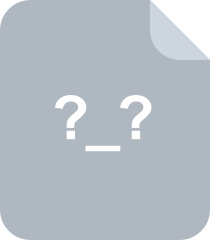
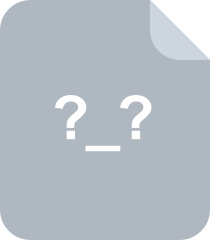
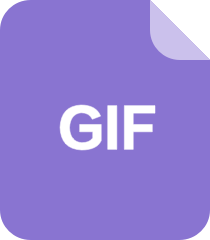
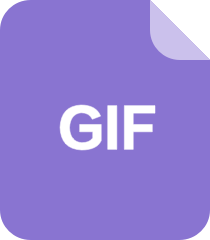
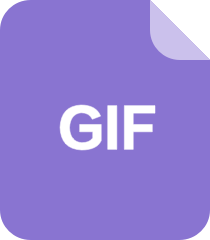
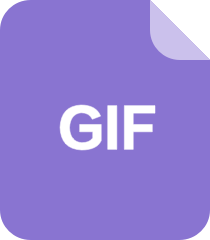
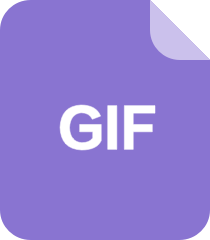
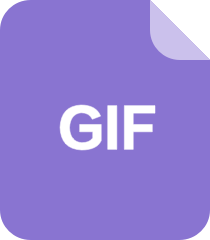
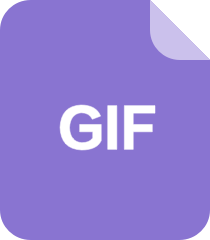
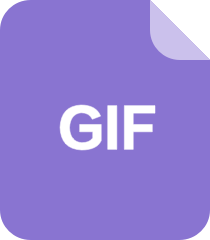
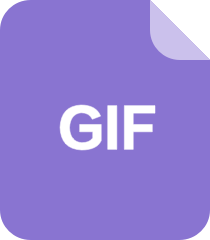
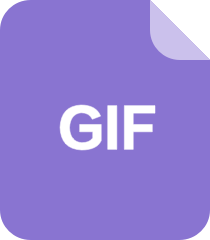
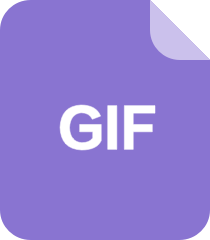
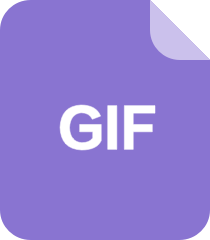
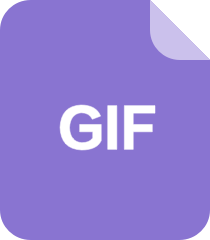
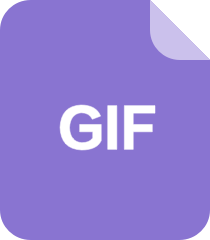
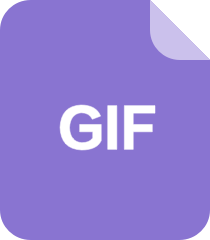
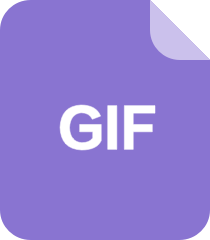
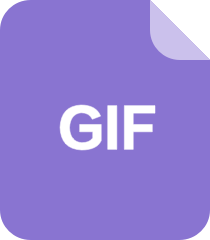
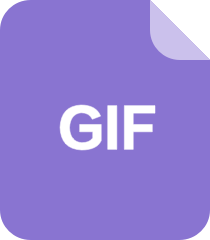
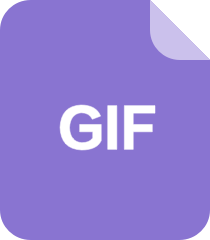
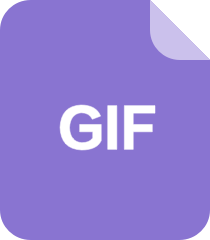
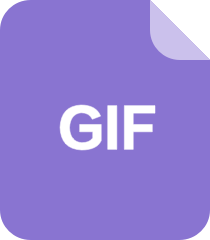
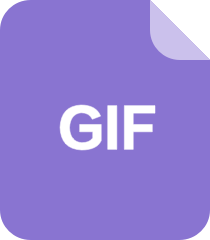
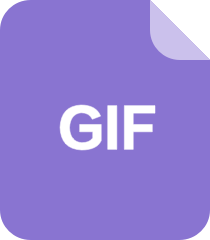
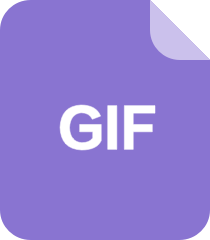
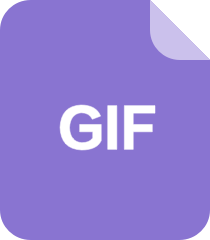
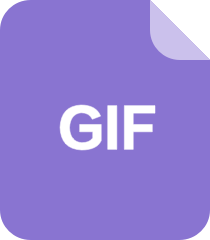
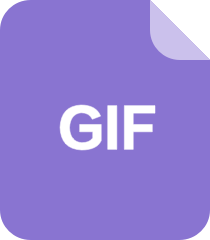
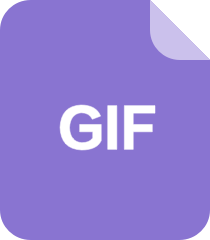
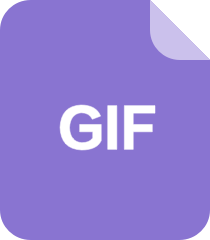
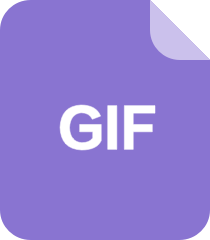
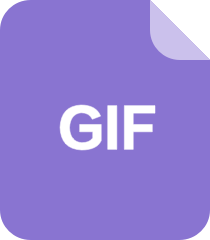
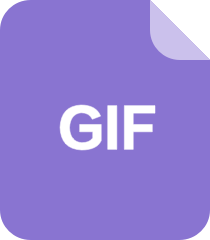
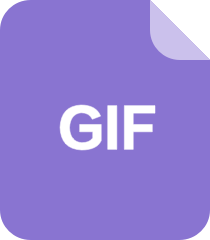
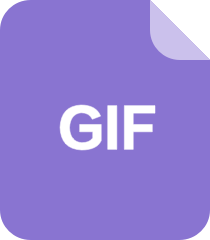
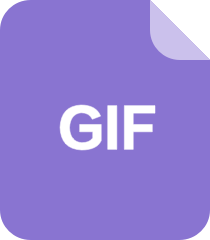
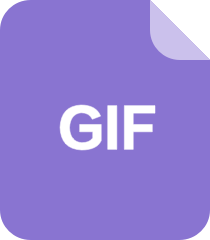
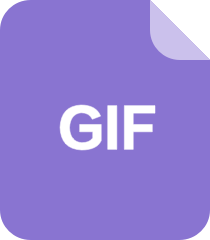
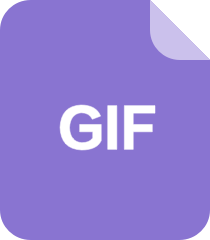
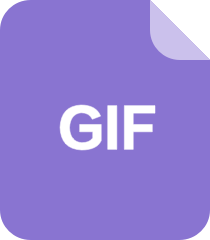
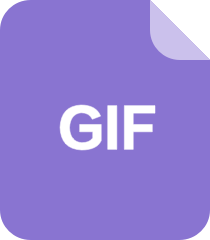
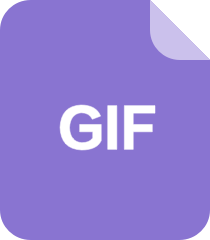
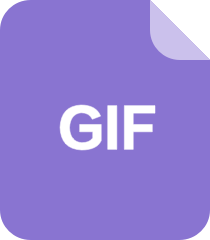
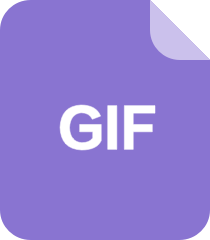
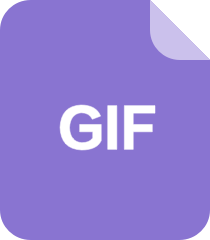
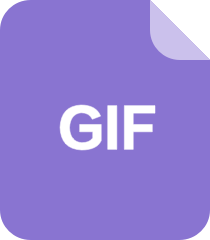
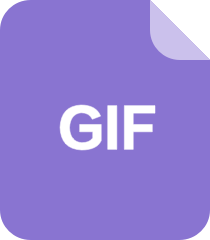
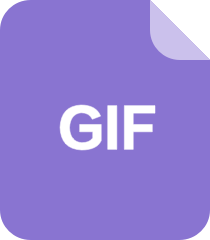
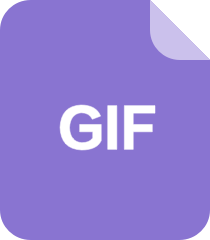
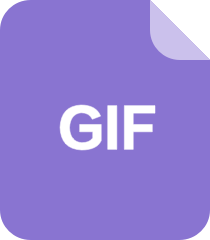
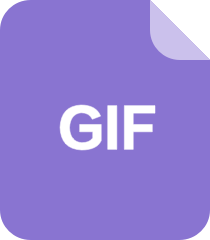
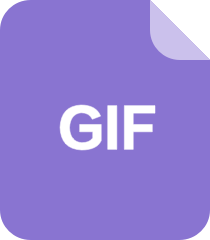
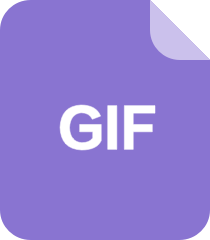
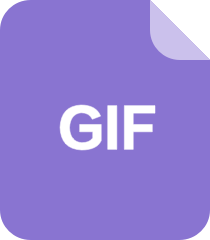
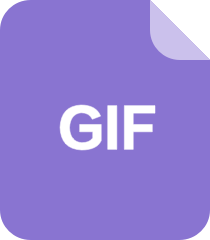
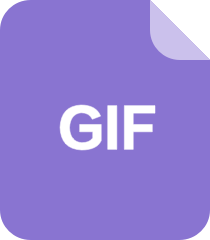
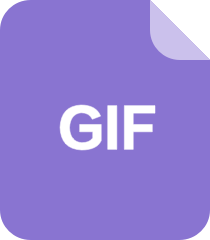
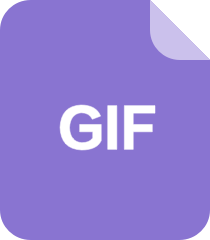
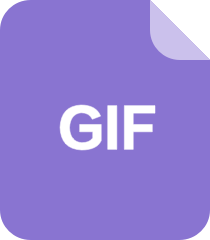
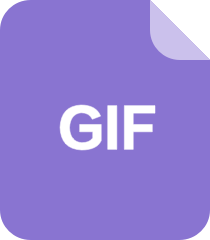
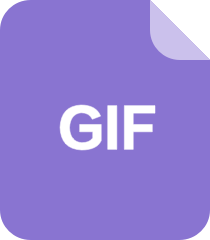
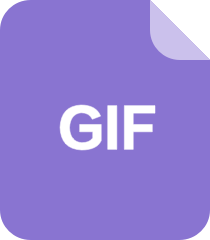
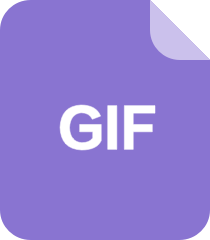
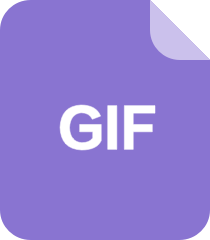
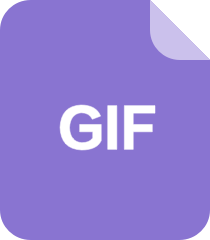
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
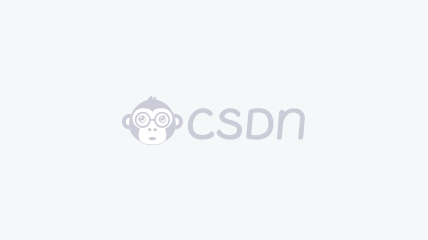

m0_72731342
- 粉丝: 2
- 资源: 1832
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

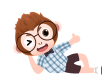
最新资源
- settings.code-profile
- 网络安全涉及到保护网络系统的硬件、软件及其数据免受破坏、更改和泄露
- maven-项目管理和构建自动化工具的概要介绍与分析
- 企业官网模板html,公司官网模板,单页bootstrap官网模板
- 基于SpringBoot的学生成绩可视化分析系统源代码+数据库,学生成绩管理,考试发布,可视化数据分析,辅助教学,提高教学质量
- qt-online-installer-x64-4.8.0.zip
- 树状图控件,用来展示一个树状图形,数据结构类似TreeView 在企业信息系统里经常会用到,比如公司组织架构,产品BOM"
- 布尔诺理工大学的VHDL课程
- PHP的概要介绍与分析
- Tensorflow安装的概要介绍与分析
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


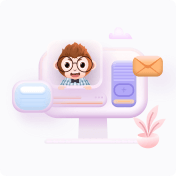
安全验证
文档复制为VIP权益,开通VIP直接复制
