/*
Copyright (c) 2007-2009, Yusuke Yamamoto
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
* Neither the name of the Yusuke Yamamoto nor the
names of its contributors may be used to endorse or promote products
derived from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY Yusuke Yamamoto ``AS IS'' AND ANY
EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
DISCLAIMED. IN NO EVENT SHALL Yusuke Yamamoto BE LIABLE FOR ANY
DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package weibo4android;
import java.io.File;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.LinkedList;
import java.util.List;
import java.util.Locale;
import java.util.TimeZone;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import weibo4android.http.AccessToken;
import weibo4android.http.HttpClient;
import weibo4android.http.ImageItem;
import weibo4android.http.PostParameter;
import weibo4android.http.RequestToken;
import weibo4android.http.Response;
import weibo4android.org.json.JSONException;
import weibo4android.org.json.JSONObject;
/**
* A java reporesentation of the <a href="http://open.t.sina.com.cn/wiki/">Weibo API</a>
*/
public class Weibo extends WeiboSupport implements java.io.Serializable {
public static String CONSUMER_KEY = "1757896691";
public static String CONSUMER_SECRET = "3f3ad33ef527d040afe737cb02ed4886";
private String baseURL = Configuration.getScheme() + "api.t.sina.com.cn/";
private String searchBaseURL = Configuration.getScheme() + "api.t.sina.com.cn/";
private static final long serialVersionUID = -1486360080128882436L;
public Weibo() {
super();
format.setTimeZone(TimeZone.getTimeZone("GMT"));
http.setRequestTokenURL(Configuration.getScheme() + "api.t.sina.com.cn/oauth/request_token");
http.setAuthorizationURL(Configuration.getScheme() + "api.t.sina.com.cn/oauth/authorize");
http.setAccessTokenURL(Configuration.getScheme() + "api.t.sina.com.cn/oauth/access_token");
}
/**
* Sets token information
* @param token
* @param tokenSecret
*/
public void setToken(String token, String tokenSecret) {
http.setToken(token, tokenSecret);
}
public Weibo(String baseURL) {
this();
this.baseURL = baseURL;
}
public Weibo(String id, String password) {
this();
setUserId(id);
setPassword(password);
}
public Weibo(String id, String password, String baseURL) {
this();
setUserId(id);
setPassword(password);
this.baseURL = baseURL;
}
/**
* Sets the base URL
*
* @param baseURL String the base URL
*/
public void setBaseURL(String baseURL) {
this.baseURL = baseURL;
}
/**
* Returns the base URL
*
* @return the base URL
*/
public String getBaseURL() {
return this.baseURL;
}
/**
* Sets the search base URL
*
* @param searchBaseURL the search base URL
* @since Weibo4J 1.1220
*/
public void setSearchBaseURL(String searchBaseURL) {
this.searchBaseURL = searchBaseURL;
}
/**
* Returns the search base url
* @return search base url
* @since Weibo4J 1.1220
*/
public String getSearchBaseURL(){
return this.searchBaseURL;
}
/**
*
* @param consumerKey OAuth consumer key
* @param consumerSecret OAuth consumer secret
* @since Weibo4J 1.1220
*/
public synchronized void setOAuthConsumer(String consumerKey, String consumerSecret){
this.http.setOAuthConsumer(consumerKey, consumerSecret);
}
/**
* Retrieves a request token
* @return generated request token.
* @throws WeiboException when Weibo service or network is unavailable
* @since Weibo4J 1.1220
* @see <a href="http://oauth.net/core/1.0/#auth_step1">OAuth Core 1.0 - 6.1. Obtaining an Unauthorized Request Token</a>
*/
public RequestToken getOAuthRequestToken() throws WeiboException {
return http.getOAuthRequestToken();
}
public RequestToken getOAuthRequestToken(String callback_url) throws WeiboException {
return http.getOauthRequestToken(callback_url);
}
/**
* Retrieves an access token assosiated with the supplied request token.
* @param requestToken the request token
* @return access token associsted with the supplied request token.
* @throws WeiboException when Weibo service or network is unavailable, or the user has not authorized
* @see <a href="http://open.t.sina.com.cn/wiki/index.php/Oauth/access_token">Oauth/access token </a>
* @see <a href="http://oauth.net/core/1.0/#auth_step2">OAuth Core 1.0 - 6.2. Obtaining User Authorization</a>
* @since Weibo4J 1.1220
*/
public synchronized AccessToken getOAuthAccessToken(RequestToken requestToken) throws WeiboException {
return http.getOAuthAccessToken(requestToken);
}
/**
* Retrieves an access token assosiated with the supplied request token and sets userId.
* @param requestToken the request token
* @param pin pin
* @return access token associsted with the supplied request token.
* @throws WeiboException when Weibo service or network is unavailable, or the user has not authorized
* @see <a href="http://open.t.sina.com.cn/wiki/index.php/Oauth/access_token">Oauth/access token </a>
* @see <a href="http://oauth.net/core/1.0/#auth_step2">OAuth Core 1.0 - 6.2. Obtaining User Authorization</a>
* @since Weibo4J 1.1220
*/
public synchronized AccessToken getOAuthAccessToken(RequestToken requestToken, String pin) throws WeiboException {
AccessToken accessToken = http.getOAuthAccessToken(requestToken, pin);
setUserId(accessToken.getScreenName());
return accessToken;
}
/**
* Retrieves an access token assosiated with the supplied request token and sets userId.
* @param token request token
* @param tokenSecret request token secret
* @return access token associsted with the supplied request token.
* @throws WeiboException when Weibo service or network is unavailable, or the user has not authorized
* @see <a href="http://open.t.sina.com.cn/wiki/index.php/Oauth/access_token">Oauth/access token </a>
* @see <a href="http://oauth.net/core/1.0/#auth_step2">OAuth Core 1.0 - 6.2. Obtaining User Authorization</a>
* @since Weibo4J 1.1220
*/
public synchronized AccessToken getOAuthAccessToken(String token, String tokenSecret) throws WeiboException {
AccessToken accessToken = http.getOAuthAccessToken(token, tokenSecret);
setUserId(accessToken.getScreenName());
return accessToken;
}
/**
* Retrieves an access token assosiated with the supplied request token.
* @param token request token
* @param tokenSecret request token secret
* @param oauth_v
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
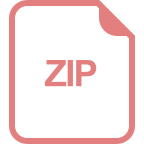
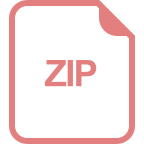
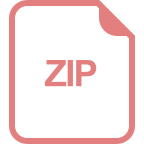
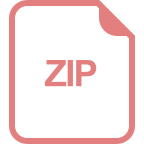
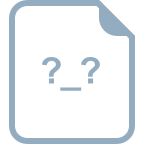
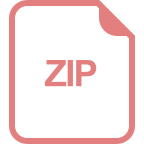
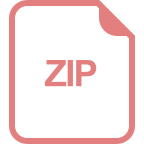
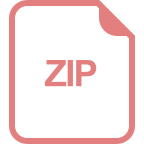
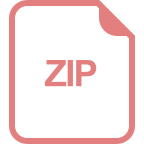
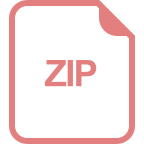
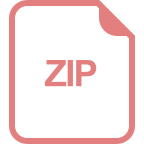
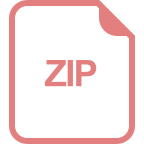
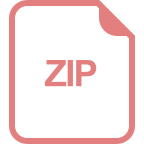
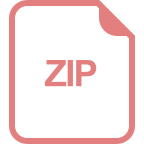
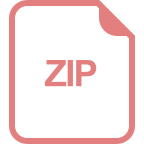
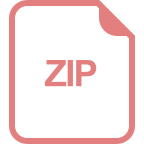
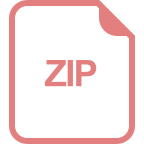
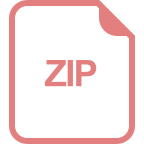
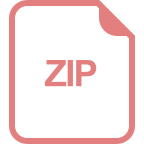
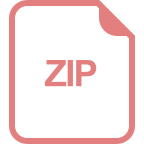
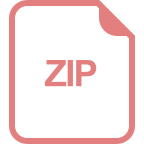
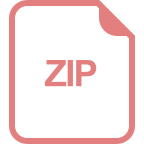
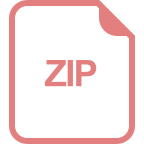
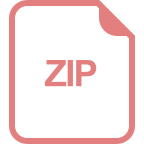
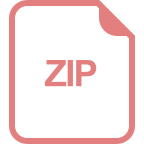
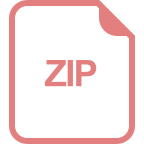
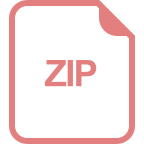
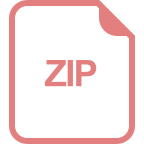
收起资源包目录

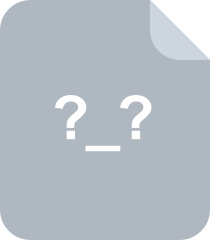
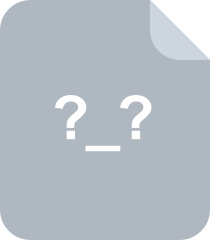
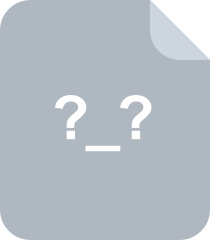
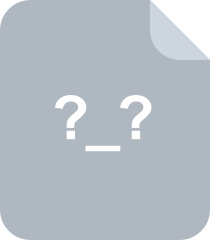
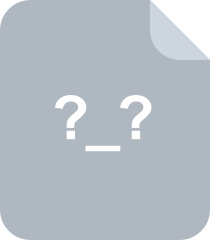
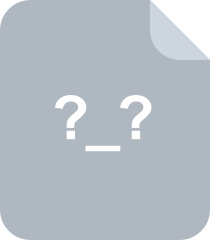
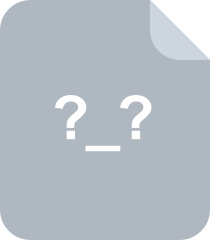
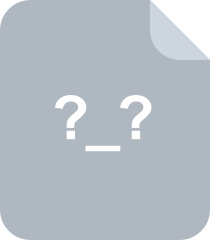
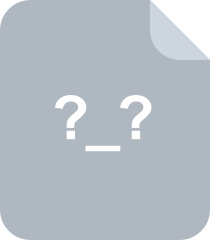
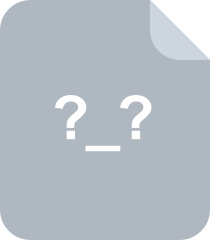
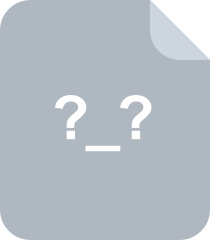
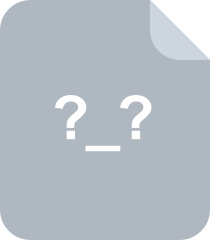
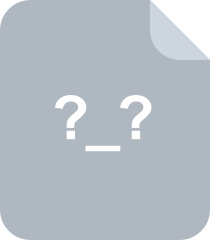
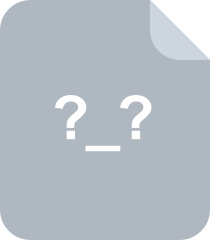
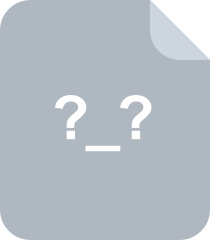
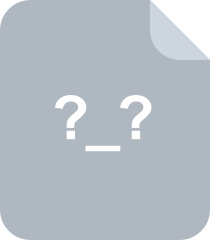
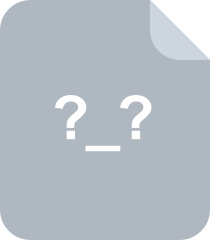
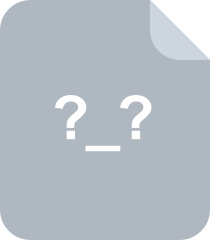
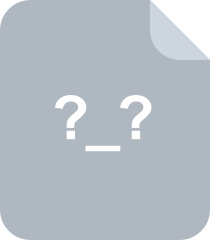
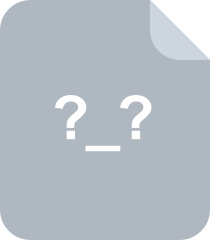
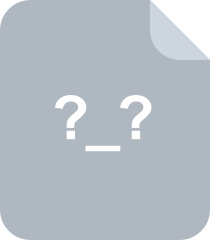
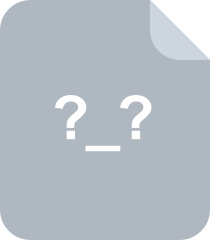
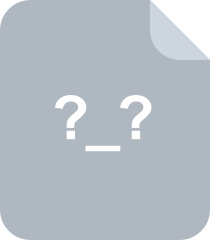
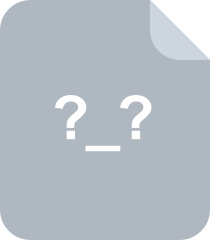
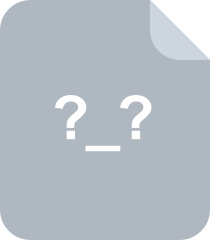
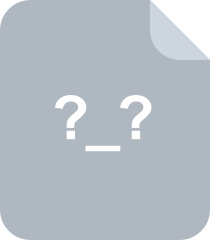
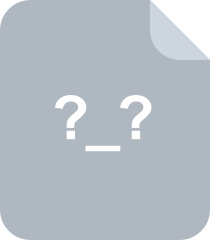
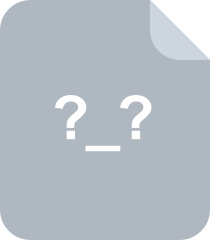
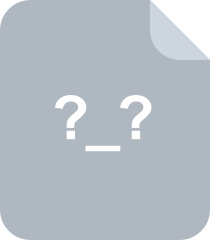
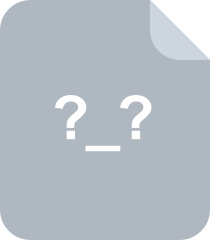
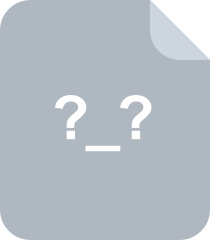
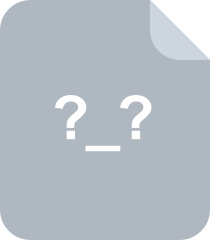
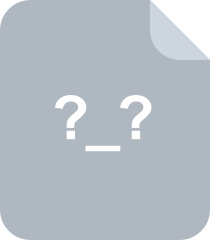
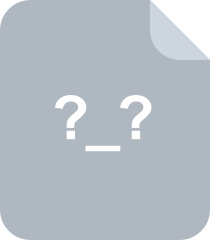
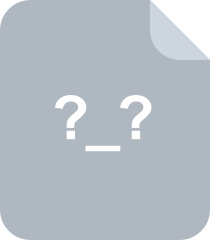
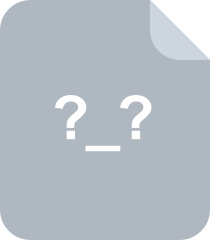
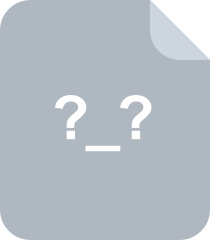
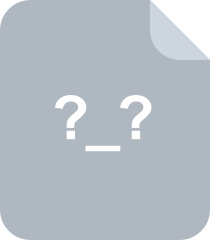
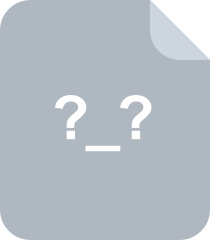
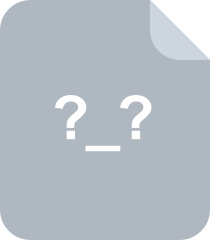
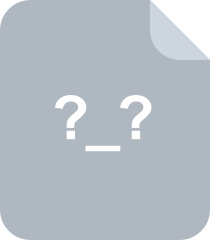
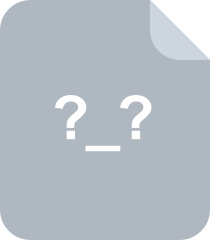
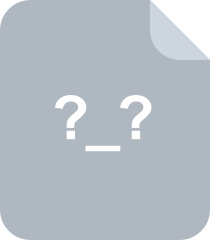
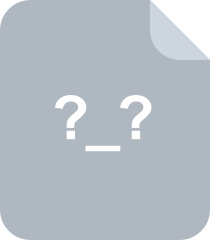
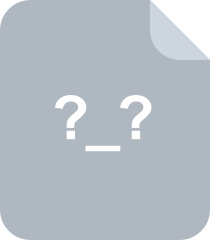
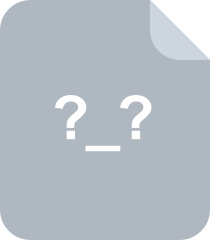
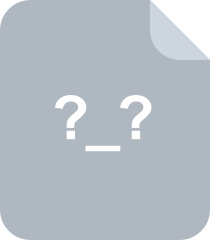
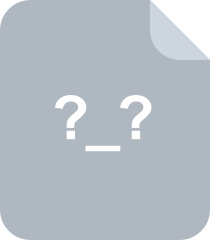
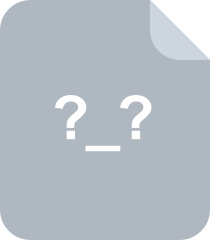
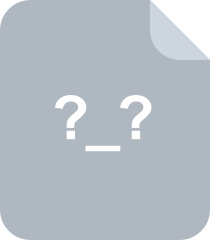
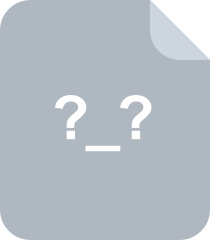
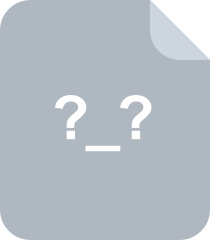
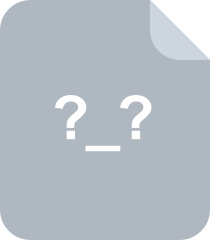
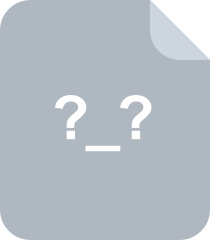
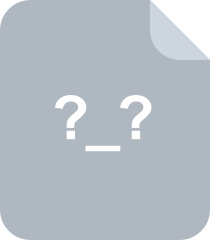
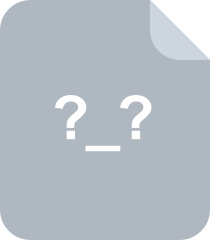
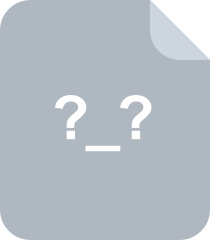
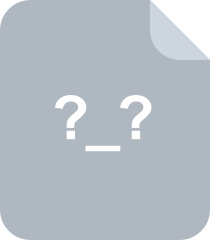
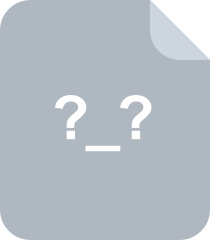
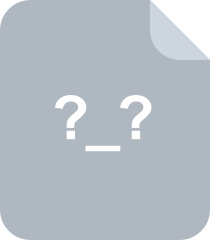
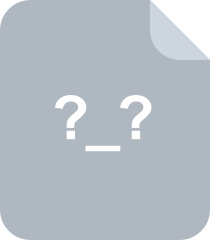
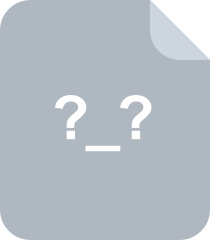
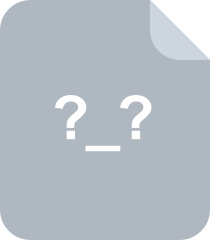
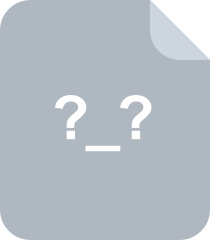
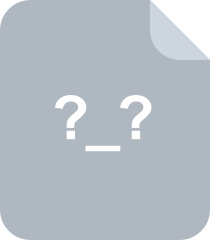
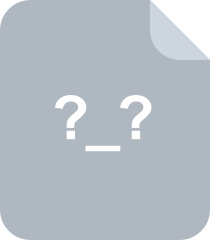
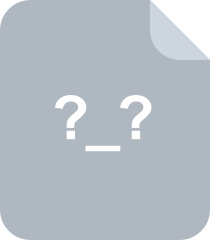
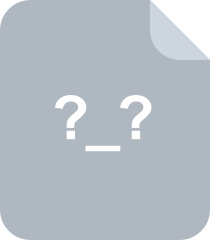
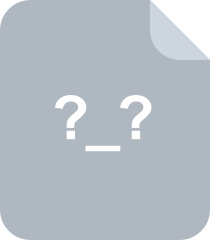
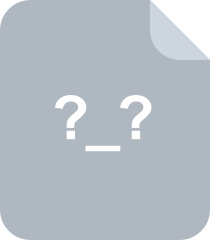
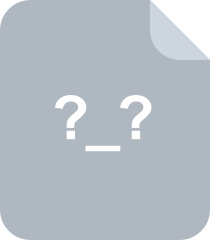
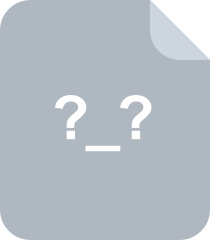
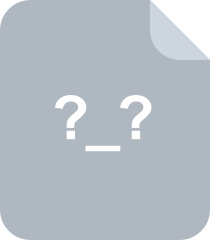
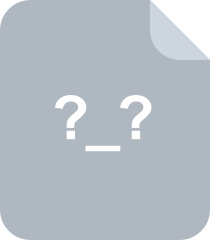
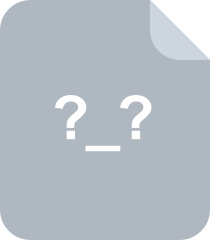
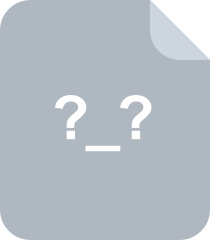
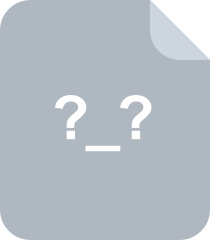
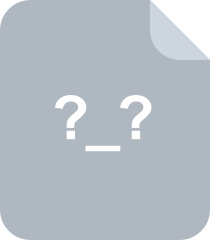
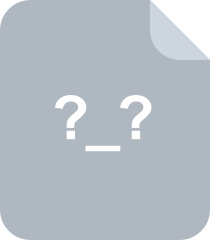
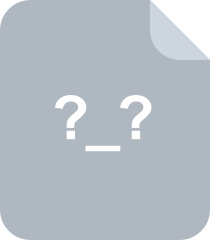
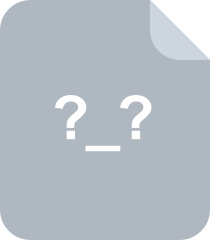
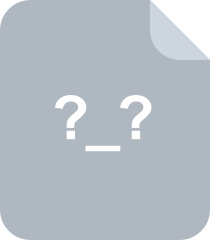
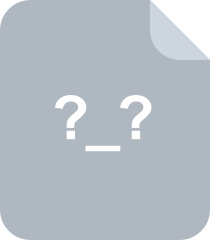
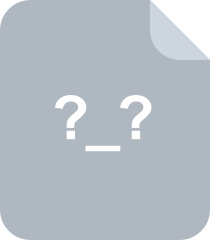
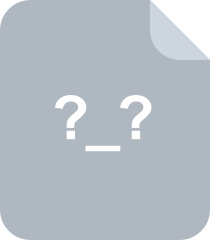
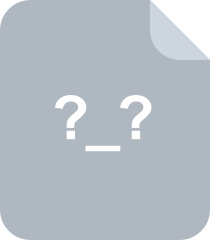
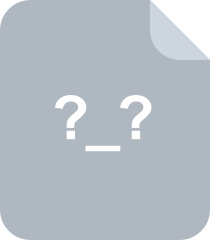
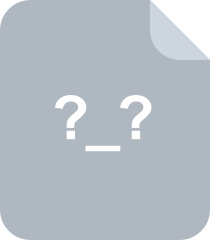
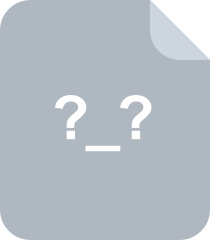
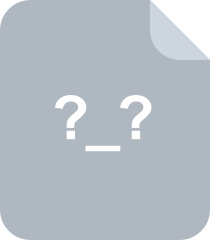
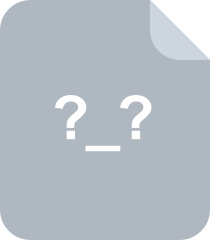
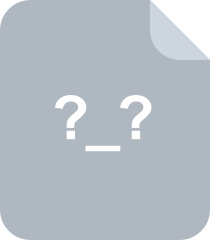
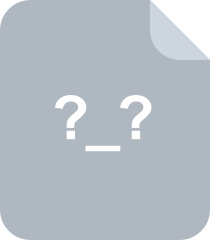
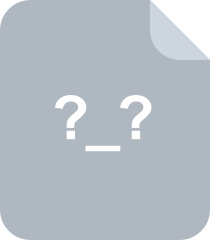
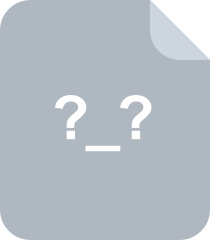
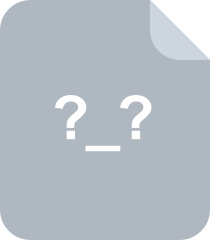
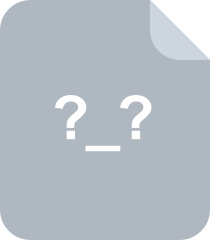
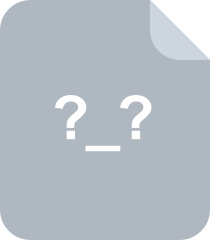
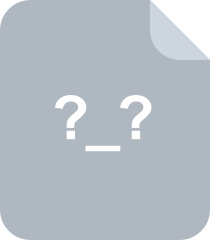
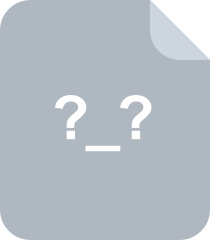
共 1254 条
- 1
- 2
- 3
- 4
- 5
- 6
- 13
资源评论
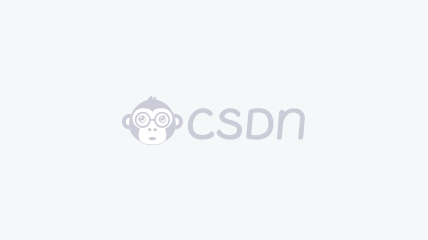

俊星学长
- 粉丝: 3240
- 资源: 486
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

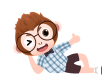
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


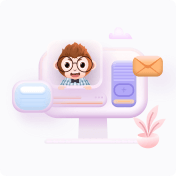
安全验证
文档复制为VIP权益,开通VIP直接复制
