package com.example.controller;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.example.Service.CustomerService;
import com.example.pojo.Customer;
import com.example.pojo.Insure;
import com.example.pojo.Report;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.*;
@RestController //说明当前类是控制层的类,返回的数据是json
@CrossOrigin //在服务器端支持跨域访问
@RequestMapping("/customer") //设置当前类的请求路径
public class CustomerController {
@Autowired
private CustomerService customerService;
/*
* 响应方法:
* 处理前端显示客户信息的请求方法--显示分页
* */
@RequestMapping("getOnePage") //设置当前方法的请求路径
public Map<String,Object> getOnePage(Integer page,Integer limit){
IPage<Customer> onePage= customerService.getOnePage(page, limit);
//将查到的信息构造成的map格式,以便前端使用
HashMap<String,Object> map=new HashMap<>();
map.put("code",0);
map.put("msg","");
map.put("count",onePage.getTotal()); //getTotal()获取onePage中所有的记录数
map.put("data",onePage.getRecords()); //getRecords()获取onePage中所有记录
return map;
}
@RequestMapping("getOnePagecus_name") //设置当前方法的请求路径
public Map<String,Object> getOnePagecus_name(Integer page,Integer limit,String cus_name){
IPage<Customer> onePage= customerService.getOnePagecus_name(page, limit,cus_name);
//将查到的信息构造成的map格式,以便前端使用
HashMap<String,Object> map=new HashMap<>();
map.put("code",0);
map.put("msg","");
map.put("count",onePage.getTotal()); //getTotal()获取onePage中所有的记录数
map.put("data",onePage.getRecords()); //getRecords()获取onePage中所有记录
return map;
}
@RequestMapping("getOnePagecus_industry") //设置当前方法的请求路径
public Map<String,Object> getOnePagecus_industry(Integer page,Integer limit,Integer cus_industry){
IPage<Customer> onePage= customerService.getOnePagecus_industry(page, limit,cus_industry);
//将查到的信息构造成的map格式,以便前端使用
HashMap<String,Object> map=new HashMap<>();
map.put("code",0);
map.put("msg","");
map.put("count",onePage.getTotal()); //getTotal()获取onePage中所有的记录数
map.put("data",onePage.getRecords()); //getRecords()获取onePage中所有记录
return map;
}
@RequestMapping("getOnePagecus_region") //设置当前方法的请求路径
public Map<String,Object> getOnePagecus_region(Integer page,Integer limit,Integer cus_region){
IPage<Customer> onePage= customerService.getOnePagecus_region(page, limit,cus_region);
//将查到的信息构造成的map格式,以便前端使用
HashMap<String,Object> map=new HashMap<>();
map.put("code",0);
map.put("msg","");
map.put("count",onePage.getTotal()); //getTotal()获取onePage中所有的记录数
map.put("data",onePage.getRecords()); //getRecords()获取onePage中所有记录
return map;
}
@RequestMapping("getOnePagecus_satisfy") //设置当前方法的请求路径
public Map<String,Object> getOnePagecus_satisfy(Integer page,Integer limit,Integer cus_satisfy){
IPage<Customer> onePage= customerService.getOnePagecus_satisfy(page, limit,cus_satisfy);
//将查到的信息构造成的map格式,以便前端使用
HashMap<String,Object> map=new HashMap<>();
map.put("code",0);
map.put("msg","");
map.put("count",onePage.getTotal()); //getTotal()获取onePage中所有的记录数
map.put("data",onePage.getRecords()); //getRecords()获取onePage中所有记录
return map;
}
/*
* 响应前端修改客户信息请求
* */
@RequestMapping("/update")
public Map<String,Object> update(Customer customer){
//1.调用service中的修改方法,并获取修改结果
Boolean flag = customerService.update(customer);
//2.构造返回的数据--修改成功或失败
HashMap<String, Object> map = new HashMap<>();
if(flag){
map.put("msg","修改成功");
}
else {
map.put("msg","修改失败");
}
return map;
}
//响应前端的添加客户信息的请求
@RequestMapping("/add")
public Map<String,Object> add(Customer customer){
//1.调用service中的修改方法,并获取修改结果
Boolean flag = customerService.add(customer);
//2.构造返回的数据--修改成功或失败
HashMap<String, Object> map = new HashMap<>();
if(flag){
map.put("msg","添加成功");
}
else {
map.put("msg","添加失败");
}
return map;
}
/*
* 响应前端删除客户信息的请求
* */
@RequestMapping("/delete")
public Map<String,Object> delete(Integer [] ids){
//Arrays.asList(ids)将ids数组转化为List<Integer>
Boolean flag = customerService.delete(Arrays.asList(ids));
HashMap<String, Object> map = new HashMap<>();
if(flag){
map.put("msg","删除成功");
}
else {
map.put("msg","删除失败");
}
return map;
}
@RequestMapping("/deleteid")
public Map<String,Object> deleteid(Integer cus_id){
//Arrays.asList(ids)将ids数组转化为List<Integer>
Boolean flag = customerService.deleteid(cus_id);
HashMap<String, Object> map = new HashMap<>();
if(flag){
map.put("msg","删除成功");
}
else {
map.put("msg","删除失败");
}
return map;
}
@RequestMapping("/getCusliushi")
public Map<String,Object> getCusliushi(){//流失客户
List<Customer> List = customerService.getCusliushi();
HashMap<String, Object> map = new HashMap<>();
if(!List.isEmpty()) {
map.put("code", 0); //正确
map.put("msg","");
map.put("data", List);
}
else {
map.put("code",1); //错误
map.put("msg", "沒有流失客戶信息");
}
return map;
}
@RequestMapping("/getCuscommon")
public Map<String,Object> getCuscommon(){//正常客户
List<Customer> List = customerService.getCuscommon();
HashMap<String, Object> map = new HashMap<>();
if(!List.isEmpty()) {
map.put("code", 0); //正确
map.put("msg","");
map.put("data", List);
}
else {
map.put("code",1); //错误
map.put("msg", "沒有该信息");
}
return map;
}
/*
* 响应获取客户地区及其客户数量的方法
* */
@RequestMapping("/getCusNumByRegion")
public Map<String,Object> getCusNumByRegion(){
//1.获取reportlist
//2.将reportlist中的item和num分别存放到xdata和ydata中
List<Report> reportList = customerService.getCusNumByRegion();
ArrayList<String> xdata = new ArrayList<>();
ArrayList<Integer> ydata = new ArrayList<>();
for(Report report:reportList){//for循环读取reportList里的数据
String item = report.getItem();
switch (item){
case "1":
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
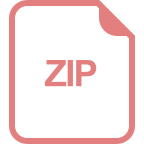
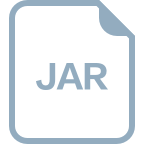
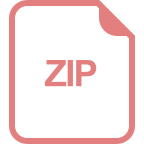
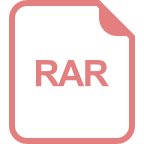
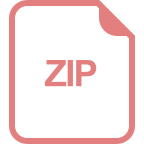
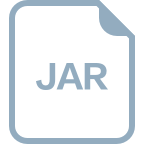
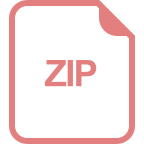
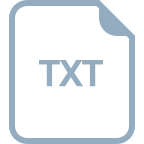
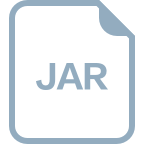
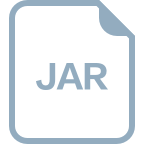
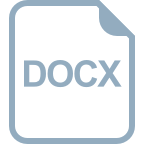
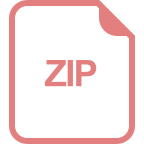
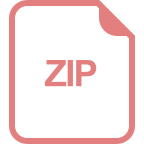
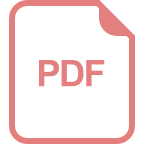
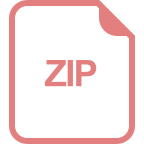
收起资源包目录

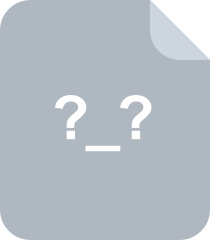
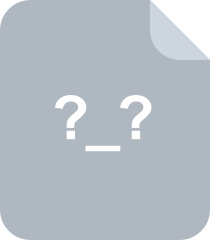
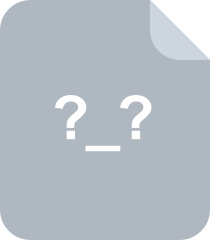
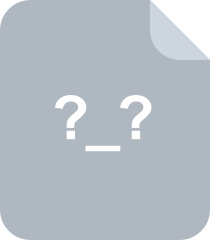
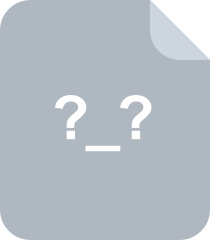
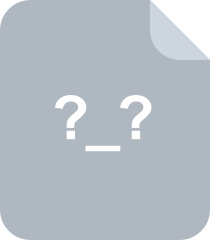
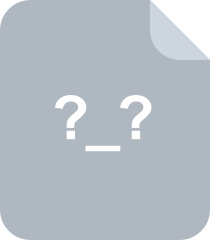
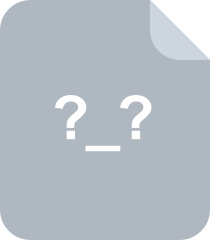
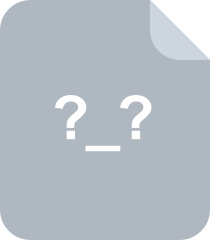
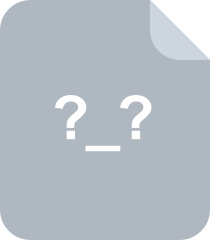
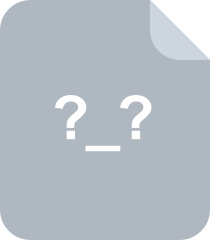
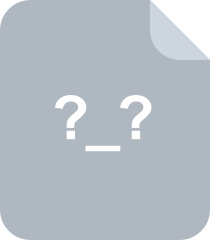
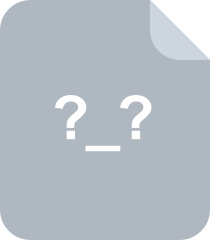
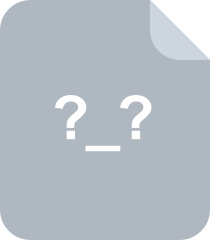
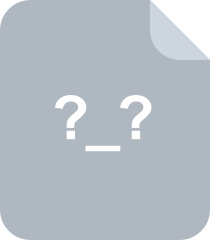
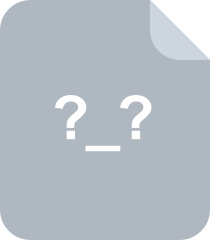
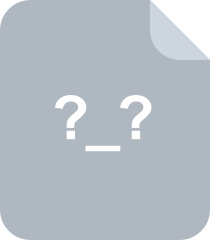
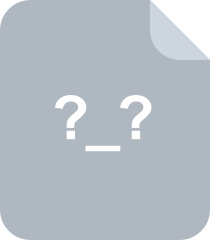
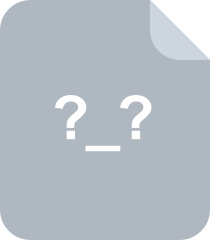
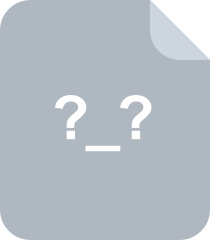
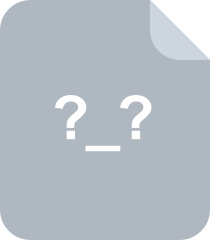
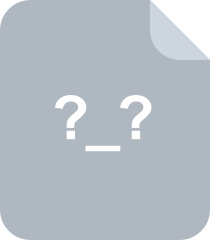
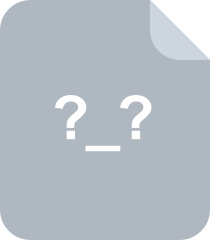
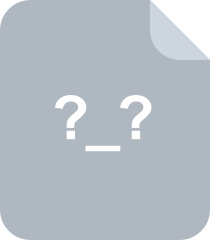
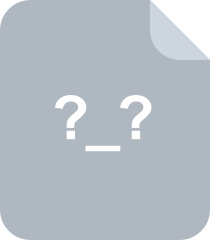
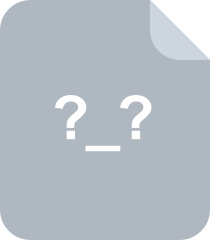
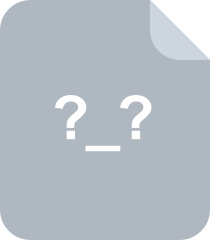
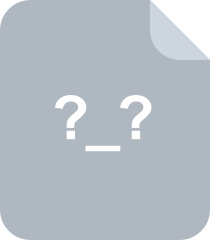
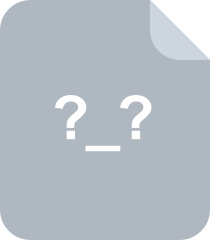
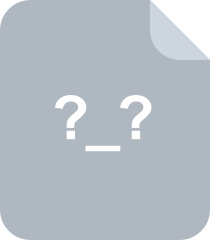
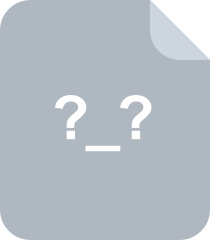
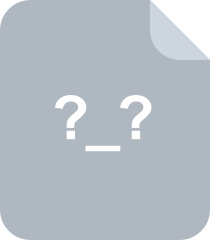
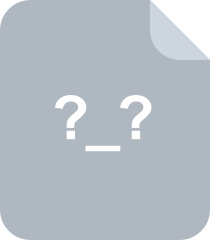
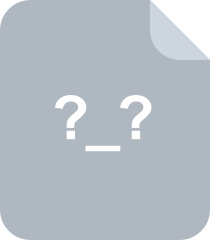
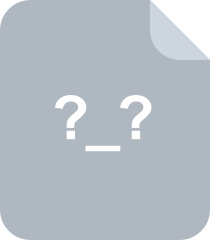
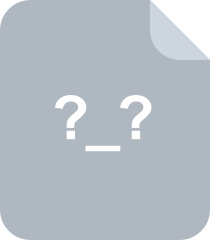
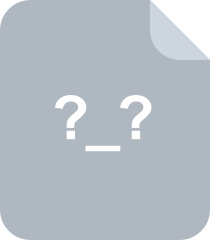
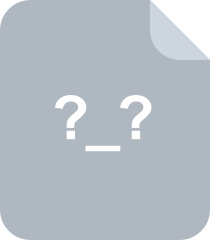
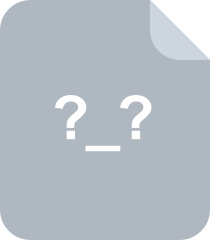
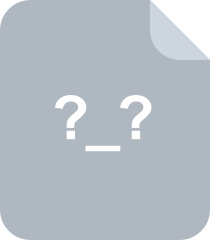
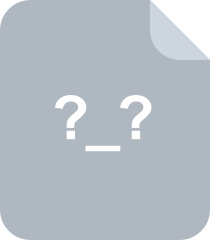
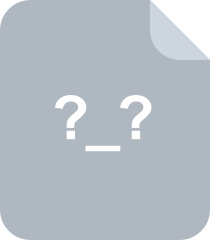
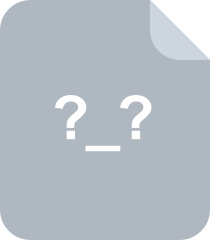
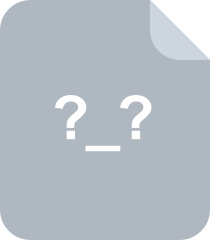
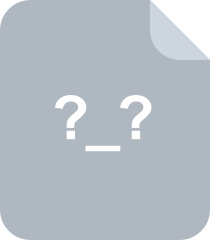
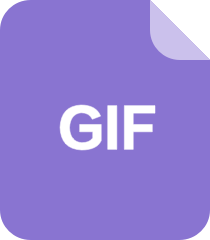
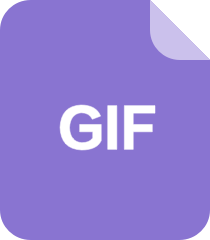
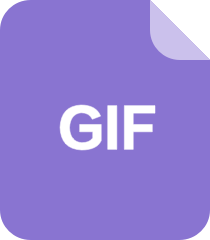
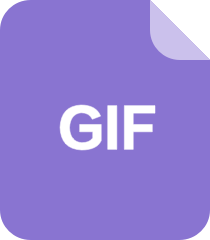
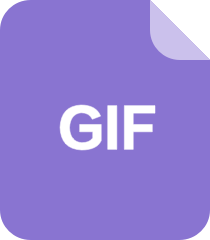
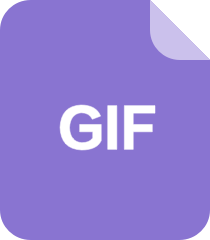
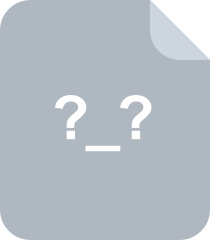
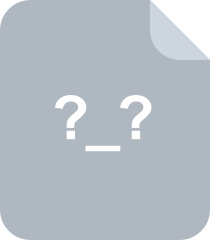
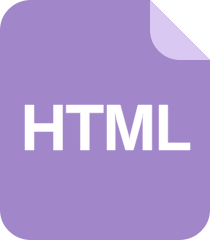
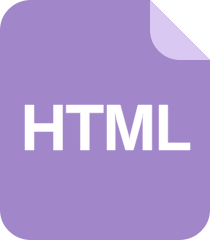
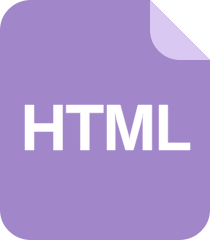
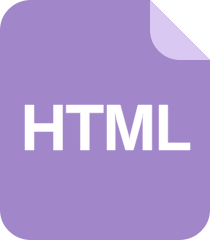
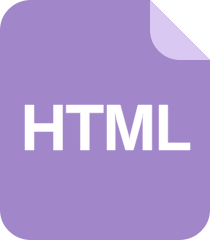
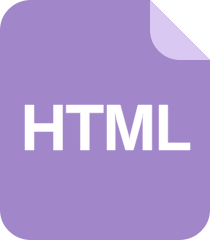
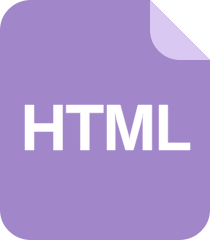
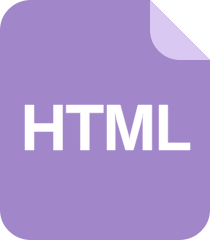
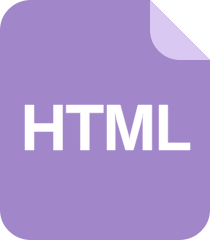
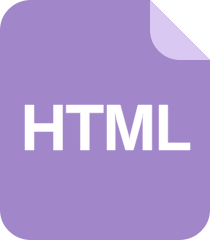
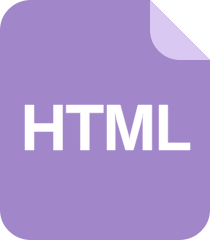
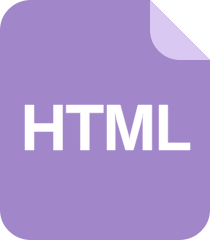
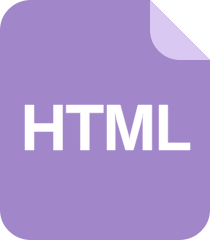
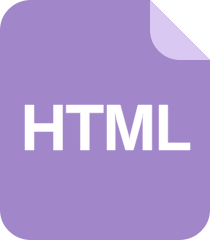
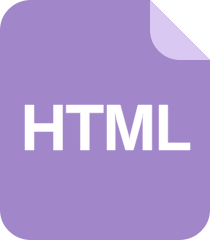
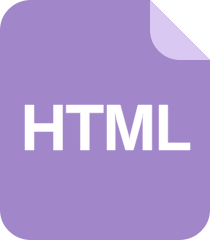
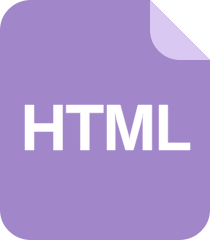
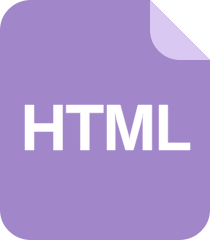
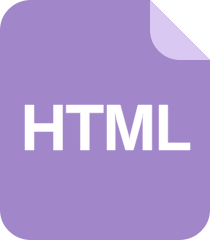
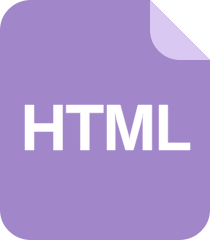
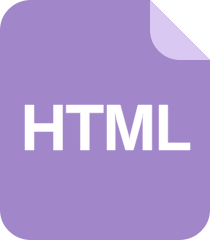
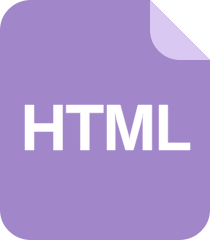
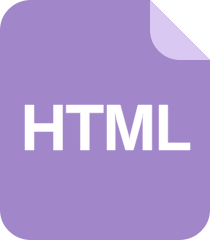
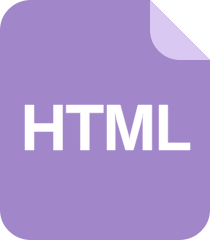
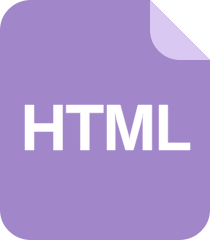
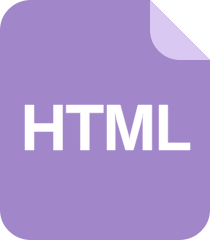
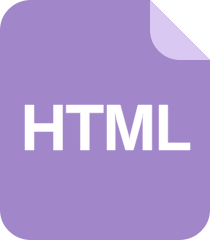
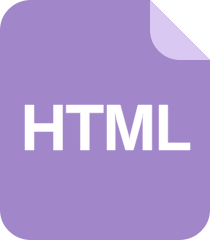
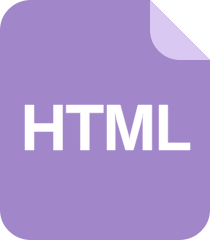
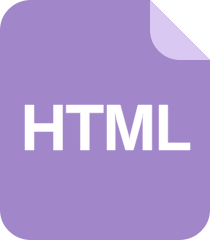
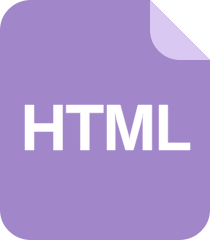
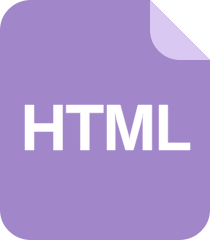
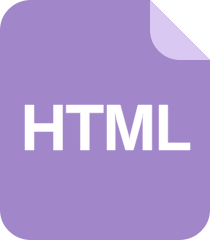
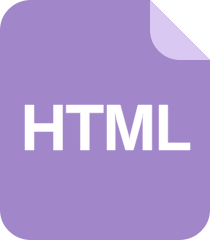
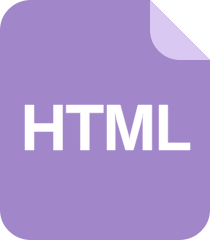
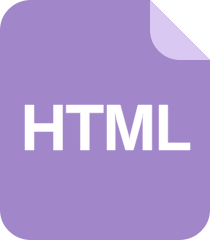
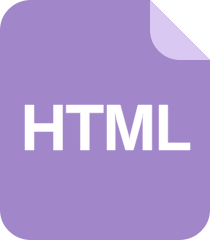
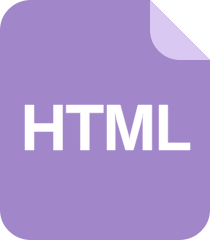
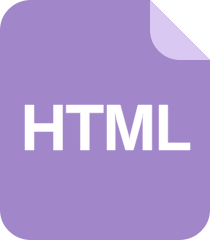
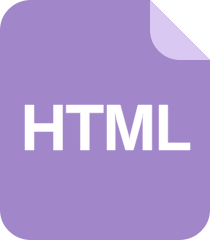
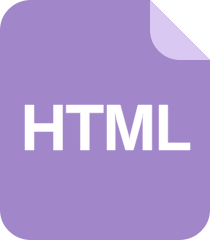
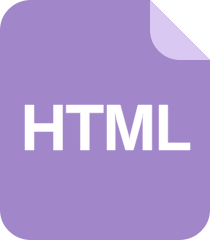
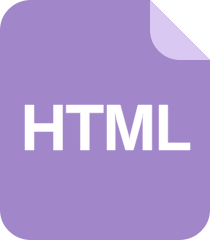
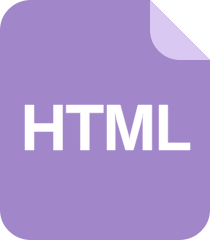
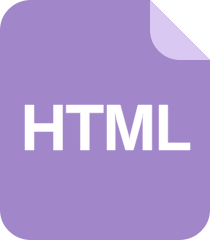
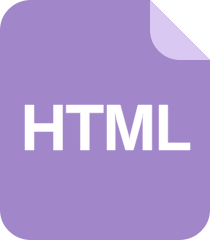
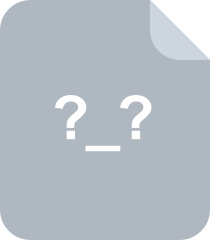
共 310 条
- 1
- 2
- 3
- 4
资源评论
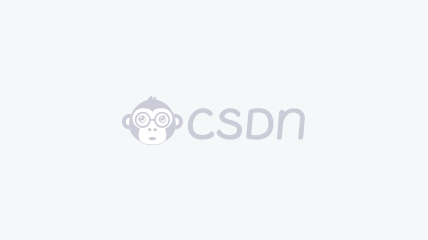

m0_69748516
- 粉丝: 1
- 资源: 10
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

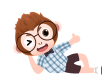
安全验证
文档复制为VIP权益,开通VIP直接复制
