[](https://supportukrainenow.org/)

# PHPMailer – A full-featured email creation and transfer class for PHP
[](https://github.com/PHPMailer/PHPMailer/actions)
[](https://codecov.io/gh/PHPMailer/PHPMailer)
[](https://packagist.org/packages/phpmailer/phpmailer)
[](https://packagist.org/packages/phpmailer/phpmailer)
[](https://packagist.org/packages/phpmailer/phpmailer)
[](https://phpmailer.github.io/PHPMailer/)
## Features
- Probably the world's most popular code for sending email from PHP!
- Used by many open-source projects: WordPress, Drupal, 1CRM, SugarCRM, Yii, Joomla! and many more
- Integrated SMTP support – send without a local mail server
- Send emails with multiple To, CC, BCC and Reply-to addresses
- Multipart/alternative emails for mail clients that do not read HTML email
- Add attachments, including inline
- Support for UTF-8 content and 8bit, base64, binary, and quoted-printable encodings
- SMTP authentication with LOGIN, PLAIN, CRAM-MD5, and XOAUTH2 mechanisms over SMTPS and SMTP+STARTTLS transports
- Validates email addresses automatically
- Protects against header injection attacks
- Error messages in over 50 languages!
- DKIM and S/MIME signing support
- Compatible with PHP 5.5 and later, including PHP 8.1
- Namespaced to prevent name clashes
- Much more!
## Why you might need it
Many PHP developers need to send email from their code. The only PHP function that supports this directly is [`mail()`](https://www.php.net/manual/en/function.mail.php). However, it does not provide any assistance for making use of popular features such as encryption, authentication, HTML messages, and attachments.
Formatting email correctly is surprisingly difficult. There are myriad overlapping (and conflicting) standards, requiring tight adherence to horribly complicated formatting and encoding rules – the vast majority of code that you'll find online that uses the `mail()` function directly is just plain wrong, if not unsafe!
The PHP `mail()` function usually sends via a local mail server, typically fronted by a `sendmail` binary on Linux, BSD, and macOS platforms, however, Windows usually doesn't include a local mail server; PHPMailer's integrated SMTP client allows email sending on all platforms without needing a local mail server. Be aware though, that the `mail()` function should be avoided when possible; it's both faster and [safer](https://exploitbox.io/paper/Pwning-PHP-Mail-Function-For-Fun-And-RCE.html) to use SMTP to localhost.
*Please* don't be tempted to do it yourself – if you don't use PHPMailer, there are many other excellent libraries that
you should look at before rolling your own. Try [SwiftMailer](https://swiftmailer.symfony.com/)
, [Laminas/Mail](https://docs.laminas.dev/laminas-mail/), [ZetaComponents](https://github.com/zetacomponents/Mail) etc.
## License
This software is distributed under the [LGPL 2.1](http://www.gnu.org/licenses/lgpl-2.1.html) license, along with the [GPL Cooperation Commitment](https://gplcc.github.io/gplcc/). Please read [LICENSE](https://github.com/PHPMailer/PHPMailer/blob/master/LICENSE) for information on the software availability and distribution.
## Installation & loading
PHPMailer is available on [Packagist](https://packagist.org/packages/phpmailer/phpmailer) (using semantic versioning), and installation via [Composer](https://getcomposer.org) is the recommended way to install PHPMailer. Just add this line to your `composer.json` file:
```json
"phpmailer/phpmailer": "^6.5"
```
or run
```sh
composer require phpmailer/phpmailer
```
Note that the `vendor` folder and the `vendor/autoload.php` script are generated by Composer; they are not part of PHPMailer.
If you want to use the Gmail XOAUTH2 authentication class, you will also need to add a dependency on the `league/oauth2-client` package in your `composer.json`.
Alternatively, if you're not using Composer, you
can [download PHPMailer as a zip file](https://github.com/PHPMailer/PHPMailer/archive/master.zip), (note that docs and examples are not included in the zip file), then copy the contents of the PHPMailer folder into one of the `include_path` directories specified in your PHP configuration and load each class file manually:
```php
<?php
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\Exception;
require 'path/to/PHPMailer/src/Exception.php';
require 'path/to/PHPMailer/src/PHPMailer.php';
require 'path/to/PHPMailer/src/SMTP.php';
```
If you're not using the `SMTP` class explicitly (you're probably not), you don't need a `use` line for the SMTP class. Even if you're not using exceptions, you do still need to load the `Exception` class as it is used internally.
## Legacy versions
PHPMailer 5.2 (which is compatible with PHP 5.0 — 7.0) is no longer supported, even for security updates. You will find the latest version of 5.2 in the [5.2-stable branch](https://github.com/PHPMailer/PHPMailer/tree/5.2-stable). If you're using PHP 5.5 or later (which you should be), switch to the 6.x releases.
### Upgrading from 5.2
The biggest changes are that source files are now in the `src/` folder, and PHPMailer now declares the namespace `PHPMailer\PHPMailer`. This has several important effects – [read the upgrade guide](https://github.com/PHPMailer/PHPMailer/tree/master/UPGRADING.md) for more details.
### Minimal installation
While installing the entire package manually or with Composer is simple, convenient, and reliable, you may want to include only vital files in your project. At the very least you will need [src/PHPMailer.php](https://github.com/PHPMailer/PHPMailer/tree/master/src/PHPMailer.php). If you're using SMTP, you'll need [src/SMTP.php](https://github.com/PHPMailer/PHPMailer/tree/master/src/SMTP.php), and if you're using POP-before SMTP (*very* unlikely!), you'll need [src/POP3.php](https://github.com/PHPMailer/PHPMailer/tree/master/src/POP3.php). You can skip the [language](https://github.com/PHPMailer/PHPMailer/tree/master/language/) folder if you're not showing errors to users and can make do with English-only errors. If you're using XOAUTH2 you will need [src/OAuth.php](https://github.com/PHPMailer/PHPMailer/tree/master/src/OAuth.php) as well as the Composer dependencies for the services you wish to authenticate with. Really, it's much easier to use Composer!
## A Simple Example
```php
<?php
//Import PHPMailer classes into the global namespace
//These must be at the top of your script, not inside a function
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\SMTP;
use PHPMailer\PHPMailer\Exception;
//Load Composer's autoloader
require 'vendor/autoload.php';
//Create an instance; passing `true` enables exceptions
$mail = new PHPMailer(true);
try {
//Server settings
$mail->SMTPDebug = SMTP::DEBUG_SERVER; //Enable verbose debug output
$mail->isSMTP(); //Send using SMTP
$mail->Host = 'smtp.example.com'; //Set the SMTP server to send through
$mail->SMTPAuth = true; //Enable SMTP authentication
$mail->Username = 'user@example.com'; //SMTP username
$mail->Password = 'secret'; //SMTP password
$mail->SMTPSecure = PHPMailer::ENCRYPTION_SMTPS;
没有合适的资源?快使用搜索试试~ 我知道了~
高级版CRM客户关系管理系统源码手机版crm跟单销售公司订单合同办公erp客户管理
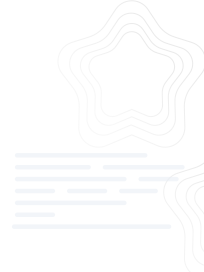
共2000个文件
php:338个
js:309个
html:241个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 20 浏览量
2024-12-24
10:39:52
上传
评论
收藏 29.29MB ZIP 举报
温馨提示
一款非常好用的客户关系管理系统源码,CRM客户系统,支持pc+wap手机端,随时随地办公, 支持批量删除客户,抢客户,客户公海,客户线索,客户来源,客户地区, 业绩管理,合同管理等强大功能,满足各行业CRM客户关系管理的需求。 本套源码采用PHP+MySQL进行开发,性能稳定可靠。数据存取集中控制,避免了数据泄漏的可能。 采用加密数据传递参数,保护系统数据安全。多级的权限控制,完善的密码验证与登录机制更加强了系统安全性, 功能强大,安装简单,使用方便,附有完整的图文安装修改教程。 功能列表: 客户管理,线索管理,客户管理,线索、客户池(公海),联系人管理,自定义场景视图, 多条件高级搜索,信息导入导出,沟通日志,跟进记录,客户提醒,客户置顶 客户转移、分享,到期回收, 客户管理数限制,客户领取周期管理,商机管理,商机视图,商机进度,沟通日志, 商机置顶,商机分组,多条件高级搜索,批量导入,批量删除…… 多条件高级搜索: 财务管理,应收款,回款单,应付款,付款单,发票管理,银行卡管理,回款进度,多条件高级搜索 数据分析: 线索数据分析,客户数据分析,客户过程分析,客
资源推荐
资源详情
资源评论
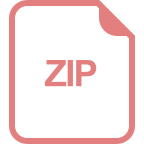
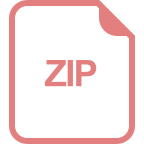
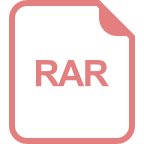
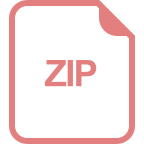
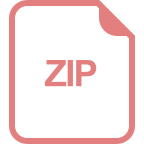
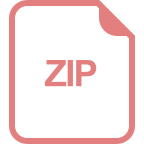
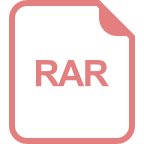
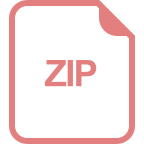
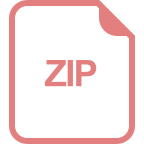
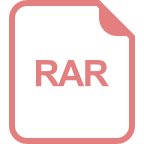
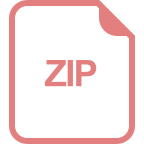
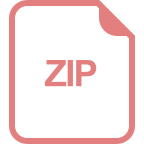
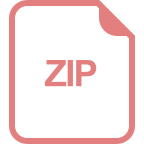
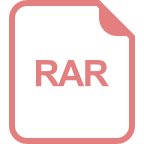
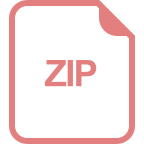
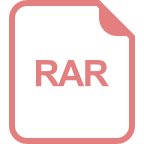
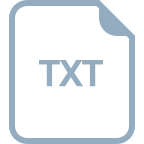
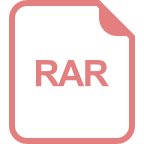
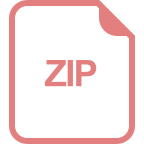
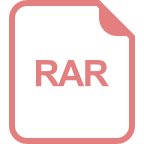
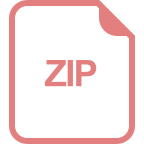
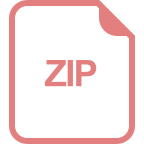
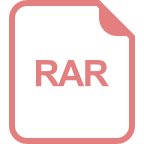
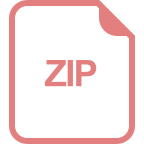
收起资源包目录

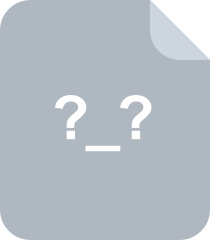
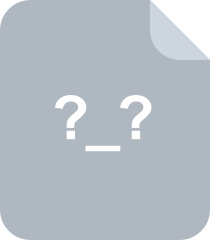
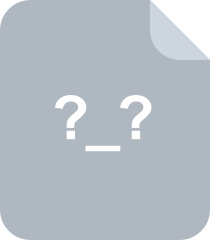
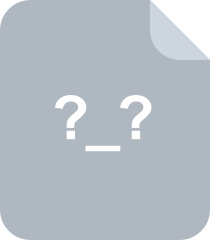
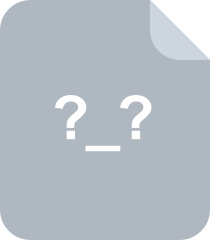
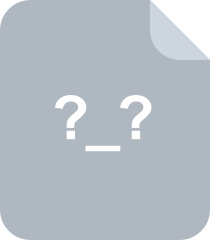
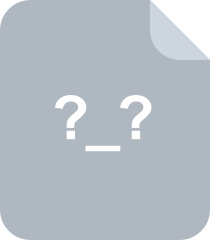
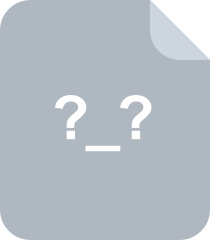
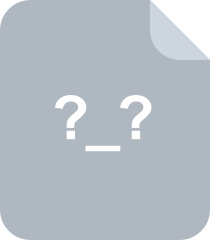
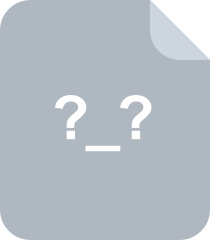
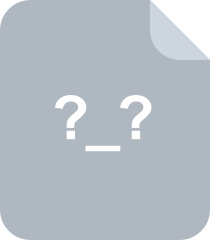
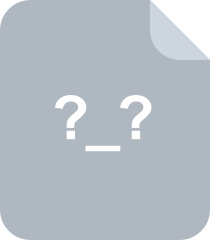
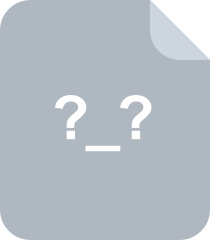
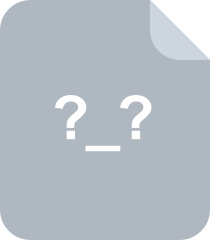
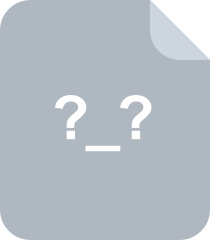
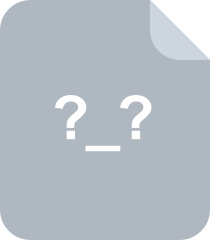
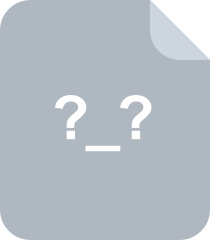
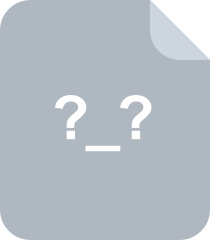
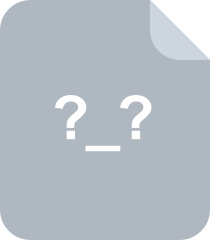
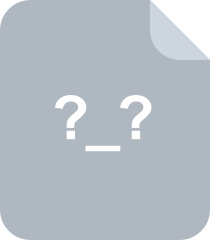
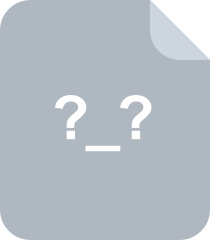
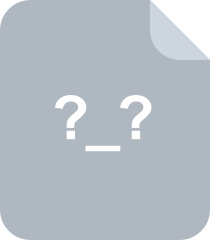
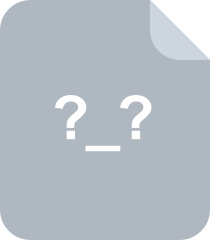
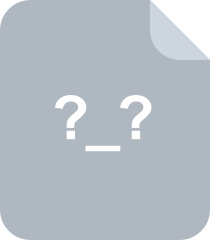
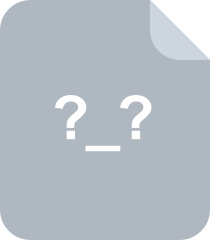
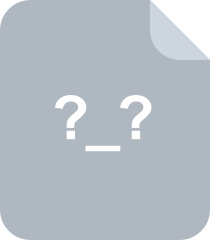
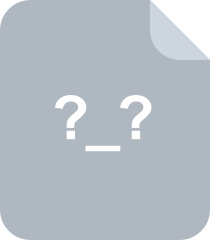
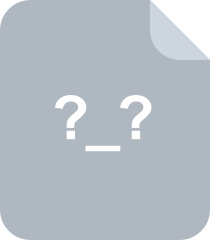
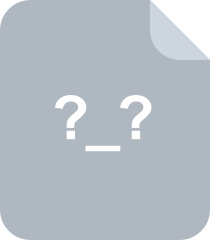
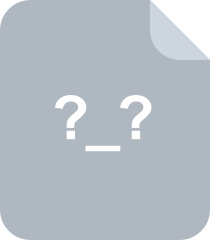
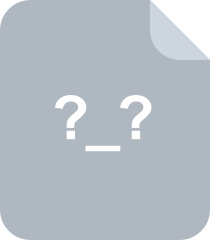
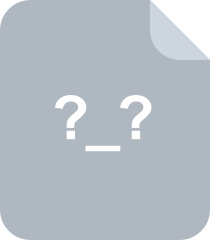
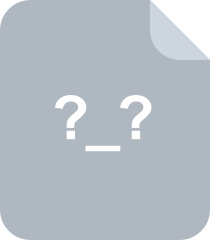
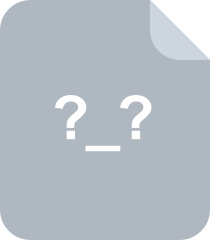
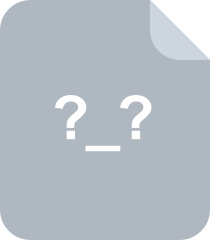
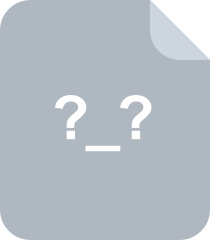
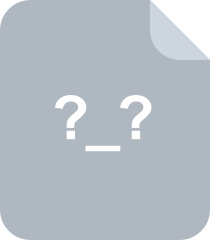
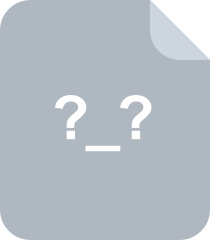
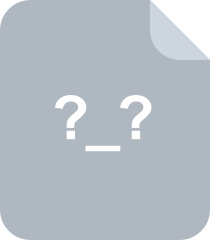
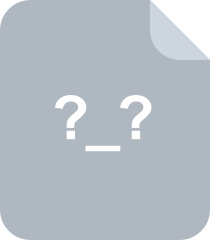
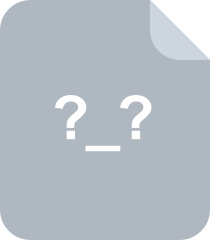
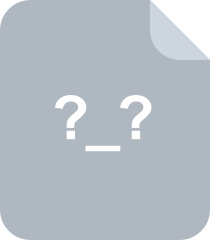
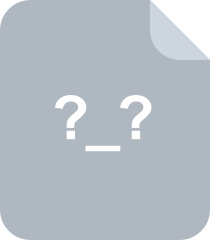
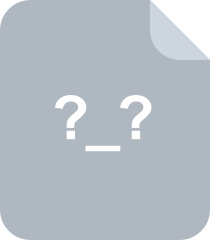
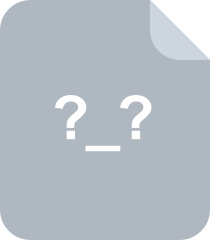
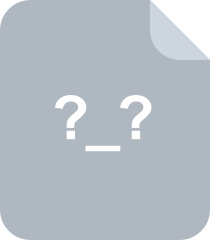
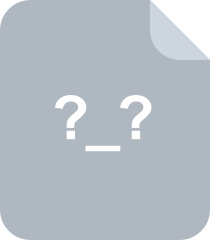
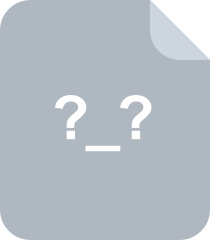
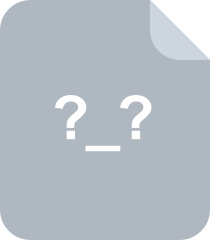
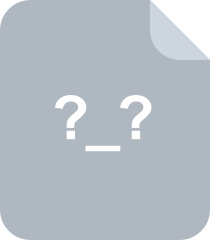
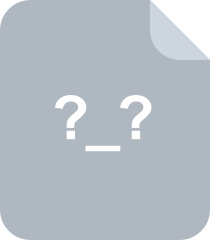
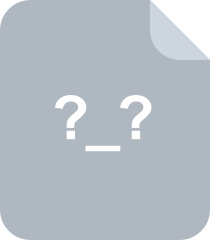
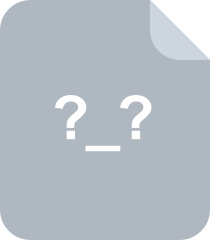
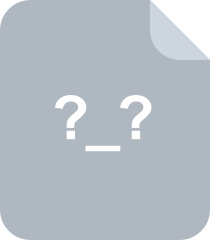
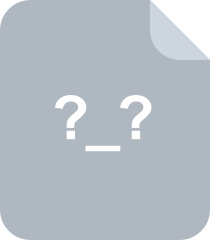
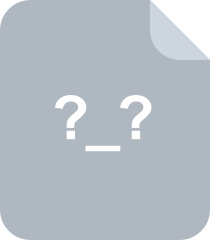
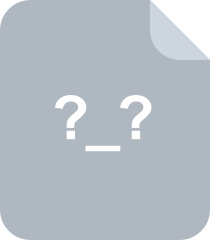
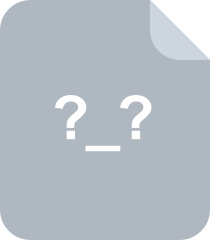
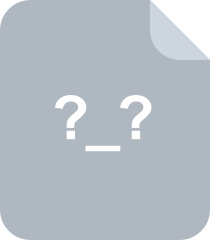
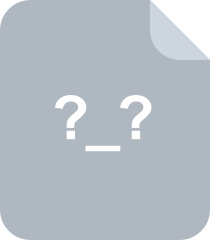
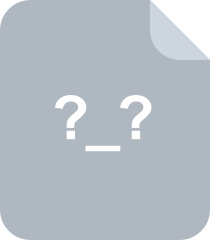
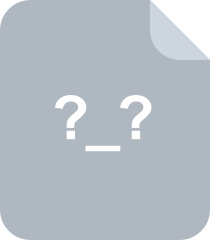
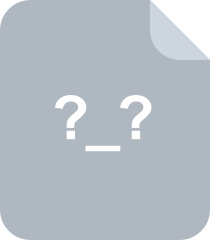
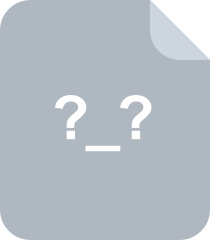
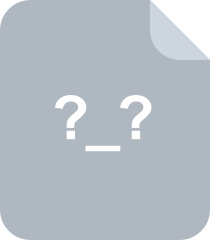
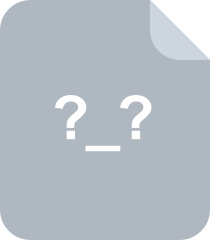
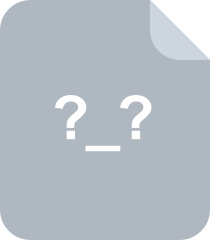
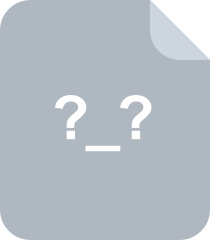
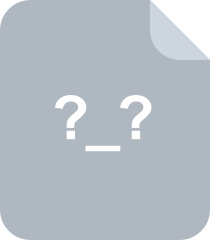
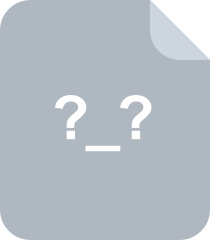
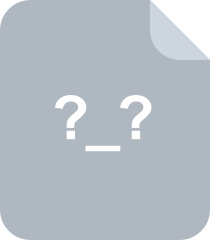
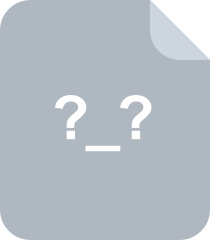
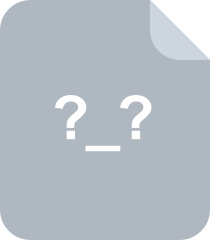
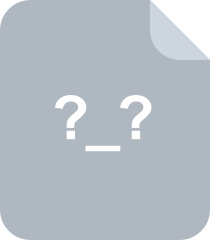
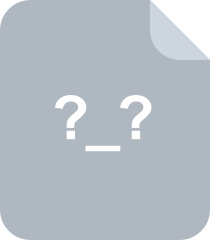
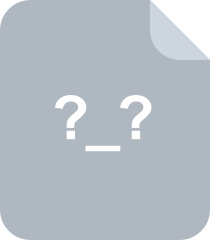
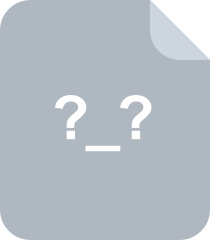
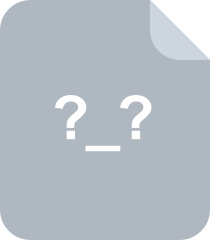
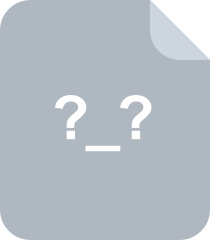
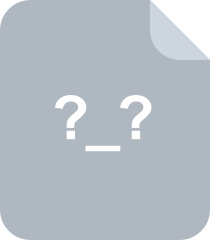
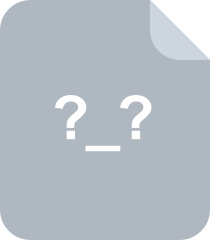
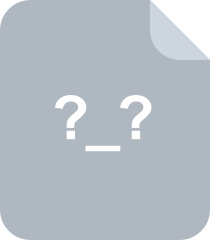
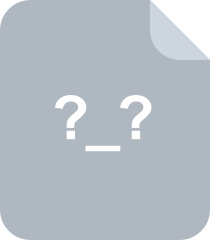
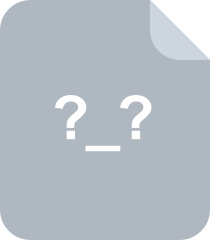
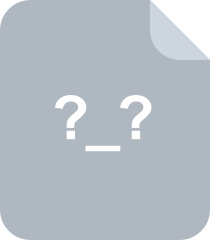
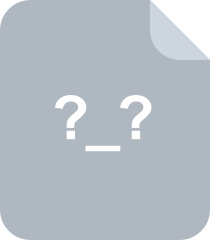
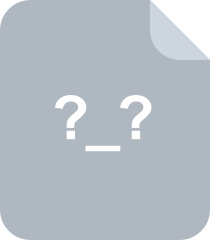
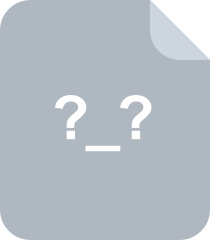
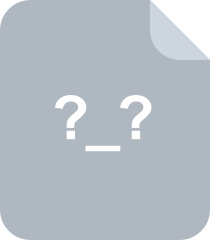
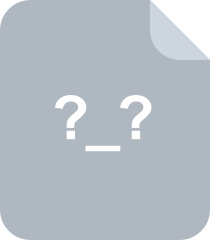
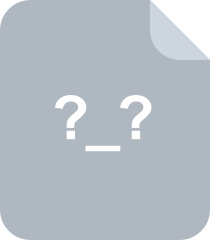
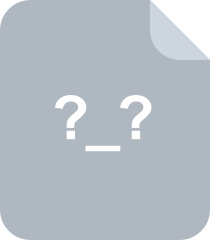
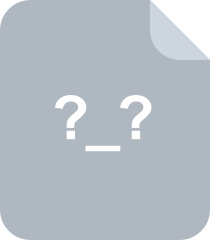
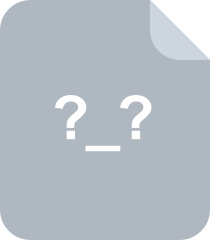
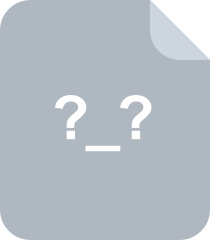
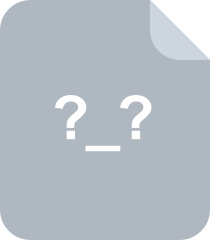
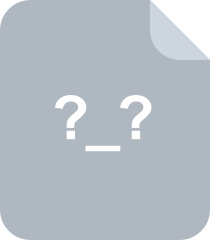
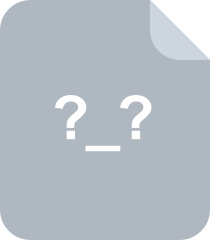
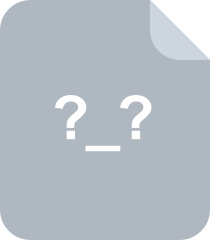
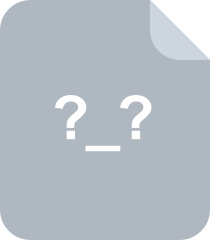
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
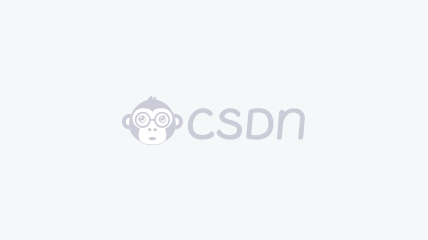

希希分享
- 粉丝: 6902
- 资源: 3821
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

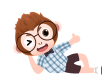
最新资源
- (174538016)downloading-Python基于深度学习和opencv的车牌识别系统.zip
- okio-2.8.0工具包
- (175360432)2储能的微电网优化调度问题
- (175396234)python实现车牌识别的示例代码.pdf
- okhttp-4.9.3工具包
- (175683250)微信小程序完美购物车抛物线(飞入效果)+ 回到顶部
- (175919248)基于python的深度学习车牌识别系统源码数据库论文.docx
- 项目费用管理看板.xlsx
- 【SOP】视频号思维导图.pdf
- 企业员工30天考勤表.xlsx
- 65个思维模型地图.pdf
- (176101808)西门子S7-1500PLC与西门子V90 PN伺服通讯控制项 西门子S7-1500PLC与西门子V90 PN伺服通讯控制项目程序
- 基于 Qt 4 + Mysql数据库成员管理系统,详细文档+全部资料+高分项目.zip
- 毕业设计-基于Qt Qwidget的学生管理系统,详细文档+全部资料+高分项目.zip
- 基于 Qt 的快递管理系统 CMake 版本详细文档+全部资料+高分项目.zip
- 基于 Qt 的机械臂操作系统 —— Arduino、四轴桌面电动机械臂、Qt 开发上位机、USB 串口通信详细文档+全部资料+高分项目.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


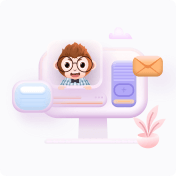
安全验证
文档复制为VIP权益,开通VIP直接复制
