<?php
/**
* Main WordPress Formatting API.
*
* Handles many functions for formatting output.
*
* @package WordPress
*/
/**
* Replaces common plain text characters with formatted entities.
*
* Returns given text with transformations of quotes into smart quotes, apostrophes,
* dashes, ellipses, the trademark symbol, and the multiplication symbol.
*
* As an example,
*
* 'cause today's effort makes it worth tomorrow's "holiday" ...
*
* Becomes:
*
* ’cause today’s effort makes it worth tomorrow’s “holiday” …
*
* Code within certain HTML blocks are skipped.
*
* Do not use this function before the {@see 'init'} action hook; everything will break.
*
* @since 0.71
*
* @global array $wp_cockneyreplace Array of formatted entities for certain common phrases.
* @global array $shortcode_tags
*
* @param string $text The text to be formatted.
* @param bool $reset Set to true for unit testing. Translated patterns will reset.
* @return string The string replaced with HTML entities.
*/
function wptexturize( $text, $reset = false ) {
global $wp_cockneyreplace, $shortcode_tags;
static $static_characters = null,
$static_replacements = null,
$dynamic_characters = null,
$dynamic_replacements = null,
$default_no_texturize_tags = null,
$default_no_texturize_shortcodes = null,
$run_texturize = true,
$apos = null,
$prime = null,
$double_prime = null,
$opening_quote = null,
$closing_quote = null,
$opening_single_quote = null,
$closing_single_quote = null,
$open_q_flag = '<!--oq-->',
$open_sq_flag = '<!--osq-->',
$apos_flag = '<!--apos-->';
// If there's nothing to do, just stop.
if ( empty( $text ) || false === $run_texturize ) {
return $text;
}
// Set up static variables. Run once only.
if ( $reset || ! isset( $static_characters ) ) {
/**
* Filters whether to skip running wptexturize().
*
* Returning false from the filter will effectively short-circuit wptexturize()
* and return the original text passed to the function instead.
*
* The filter runs only once, the first time wptexturize() is called.
*
* @since 4.0.0
*
* @see wptexturize()
*
* @param bool $run_texturize Whether to short-circuit wptexturize().
*/
$run_texturize = apply_filters( 'run_wptexturize', $run_texturize );
if ( false === $run_texturize ) {
return $text;
}
/* translators: Opening curly double quote. */
$opening_quote = _x( '“', 'opening curly double quote' );
/* translators: Closing curly double quote. */
$closing_quote = _x( '”', 'closing curly double quote' );
/* translators: Apostrophe, for example in 'cause or can't. */
$apos = _x( '’', 'apostrophe' );
/* translators: Prime, for example in 9' (nine feet). */
$prime = _x( '′', 'prime' );
/* translators: Double prime, for example in 9" (nine inches). */
$double_prime = _x( '″', 'double prime' );
/* translators: Opening curly single quote. */
$opening_single_quote = _x( '‘', 'opening curly single quote' );
/* translators: Closing curly single quote. */
$closing_single_quote = _x( '’', 'closing curly single quote' );
/* translators: En dash. */
$en_dash = _x( '–', 'en dash' );
/* translators: Em dash. */
$em_dash = _x( '—', 'em dash' );
$default_no_texturize_tags = array( 'pre', 'code', 'kbd', 'style', 'script', 'tt' );
$default_no_texturize_shortcodes = array( 'code' );
// If a plugin has provided an autocorrect array, use it.
if ( isset( $wp_cockneyreplace ) ) {
$cockney = array_keys( $wp_cockneyreplace );
$cockneyreplace = array_values( $wp_cockneyreplace );
} else {
/*
* translators: This is a comma-separated list of words that defy the syntax of quotations in normal use,
* for example... 'We do not have enough words yet'... is a typical quoted phrase. But when we write
* lines of code 'til we have enough of 'em, then we need to insert apostrophes instead of quotes.
*/
$cockney = explode(
',',
_x(
"'tain't,'twere,'twas,'tis,'twill,'til,'bout,'nuff,'round,'cause,'em",
'Comma-separated list of words to texturize in your language'
)
);
$cockneyreplace = explode(
',',
_x(
'’tain’t,’twere,’twas,’tis,’twill,’til,’bout,’nuff,’round,’cause,’em',
'Comma-separated list of replacement words in your language'
)
);
}
$static_characters = array_merge( array( '...', '``', '\'\'', ' (tm)' ), $cockney );
$static_replacements = array_merge( array( '…', $opening_quote, $closing_quote, ' ™' ), $cockneyreplace );
// Pattern-based replacements of characters.
// Sort the remaining patterns into several arrays for performance tuning.
$dynamic_characters = array(
'apos' => array(),
'quote' => array(),
'dash' => array(),
);
$dynamic_replacements = array(
'apos' => array(),
'quote' => array(),
'dash' => array(),
);
$dynamic = array();
$spaces = wp_spaces_regexp();
// '99' and '99" are ambiguous among other patterns; assume it's an abbreviated year at the end of a quotation.
if ( "'" !== $apos || "'" !== $closing_single_quote ) {
$dynamic[ '/\'(\d\d)\'(?=\Z|[.,:;!?)}\-\]]|>|' . $spaces . ')/' ] = $apos_flag . '$1' . $closing_single_quote;
}
if ( "'" !== $apos || '"' !== $closing_quote ) {
$dynamic[ '/\'(\d\d)"(?=\Z|[.,:;!?)}\-\]]|>|' . $spaces . ')/' ] = $apos_flag . '$1' . $closing_quote;
}
// '99 '99s '99's (apostrophe) But never '9 or '99% or '999 or '99.0.
if ( "'" !== $apos ) {
$dynamic['/\'(?=\d\d(?:\Z|(?![%\d]|[.,]\d)))/'] = $apos_flag;
}
// Quoted numbers like '0.42'.
if ( "'" !== $opening_single_quote && "'" !== $closing_single_quote ) {
$dynamic[ '/(?<=\A|' . $spaces . ')\'(\d[.,\d]*)\'/' ] = $open_sq_flag . '$1' . $closing_single_quote;
}
// Single quote at start, or preceded by (, {, <, [, ", -, or spaces.
if ( "'" !== $opening_single_quote ) {
$dynamic[ '/(?<=\A|[([{"\-]|<|' . $spaces . ')\'/' ] = $open_sq_flag;
}
// Apostrophe in a word. No spaces, double apostrophes, or other punctuation.
if ( "'" !== $apos ) {
$dynamic[ '/(?<!' . $spaces . ')\'(?!\Z|[.,:;!?"\'(){}[\]\-]|&[lg]t;|' . $spaces . ')/' ] = $apos_flag;
}
$dynamic_characters['apos'] = array_keys( $dynamic );
$dynamic_replacements['apos'] = array_values( $dynamic );
$dynamic = array();
// Quoted numbers like "42".
if ( '"' !== $opening_quote && '"' !== $closing_quote ) {
$dynamic[ '/(?<=\A|' . $spaces . ')"(\d[.,\d]*)"/' ] = $open_q_flag . '$1' . $closing_quote;
}
// Double quote at start, or preceded by (, {, <, [, -, or spaces, and not followed by spaces.
if ( '"' !== $opening_quote ) {
$dynamic[ '/(?<=\A|[([{\-]|<|' . $spaces . ')"(?!' . $spaces . ')/' ] = $open_q_flag;
}
$dynamic_characters['quote'] = array_keys( $dynamic );
$dynamic_replacements['quote'] = array_values( $dynamic );
$dynamic = array();
// Dashes and spaces.
$dynamic['/---/'] = $em_dash;
$dynamic[ '/(?<=^|' . $spaces . ')--(?=$|' . $spaces . ')/' ] = $em_dash;
$dynamic['/(?<!xn)--/'] = $en_dash;
$dynamic[ '/(?<=^|' . $spaces . ')-(?=$|' . $spaces . ')/' ] = $en_dash;
$dynamic_characters['dash'] = array_keys( $dynamic );
$dynamic_replacements['dash'] = array_values( $dynamic );
}
// Must do this every time in case plugins use these filters in a context sensitive manner.
/**
* Filters the list of HTML elements not to texturize.
*
* @since 2.8.
没有合适的资源?快使用搜索试试~ 我知道了~
资源共享网整站源码WordPress CorePress 主题丨带会员充值插件
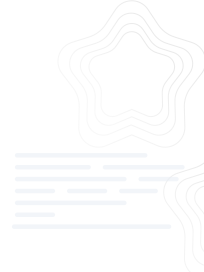
共2000个文件
php:1899个
css:630个
js:545个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 45 浏览量
2022-02-02
14:38:30
上传
评论
收藏 18.92MB ZIP 举报
温馨提示
WordPress高颜值,高性能CorePress主题,极致优化,专为极客,主题体积小于2m,代码精,功能全,颜值高,兼容好。响应式设计开启用户中心,接管WordPress自带登录页面,注册页面,找回密码页面,同时自定义个人中心设置页面,支持修改昵称,签名,修改密码,绑定账号。 环境要求: PHP版本5.6以上,WordPress 5.0+ 推荐使用宝塔建站 安装教程: 1.源码上传服务器根目录 2.修改 http://你的域名/wp-config.php 数据库信息 3.访问安装 http://你的域名/wp-admin/install.php 4.登录后台 http://你的域名/wp-admin 5.在后台 - 外观 - 点击 - CorePress 启用
资源推荐
资源详情
资源评论
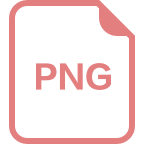
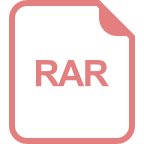
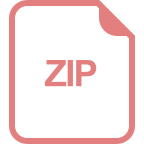
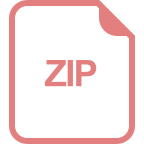
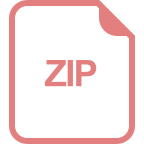
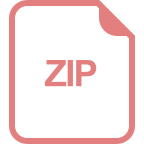
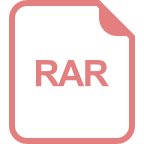
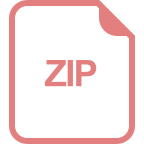
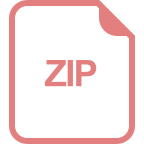
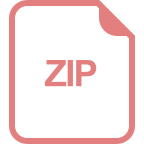
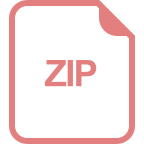
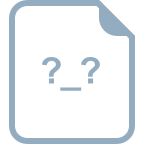
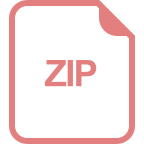
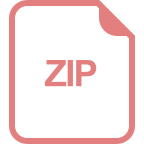
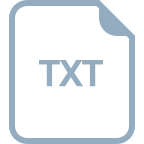
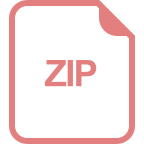
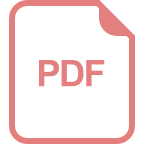
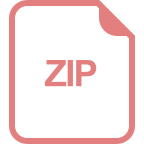
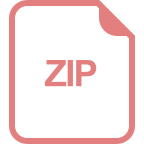
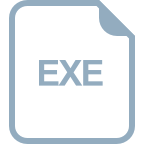
收起资源包目录

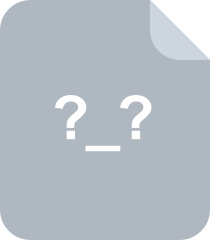
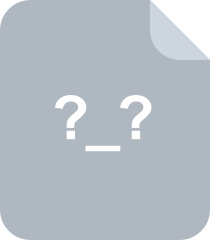
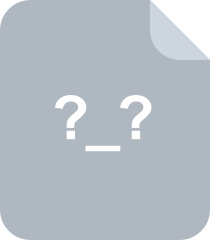
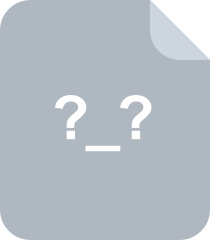
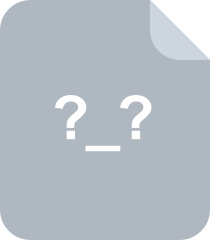
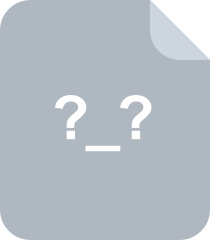
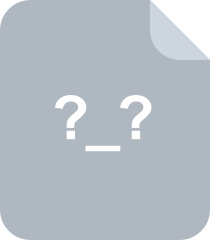
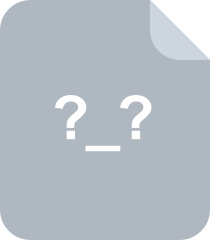
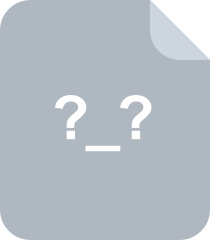
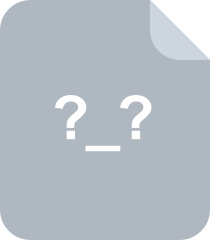
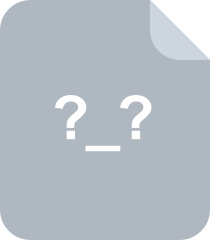
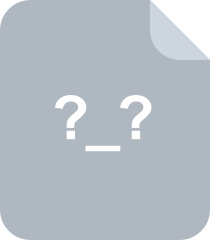
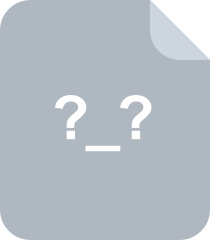
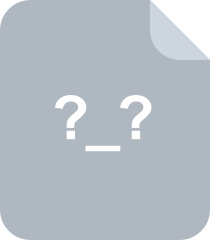
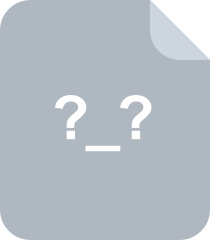
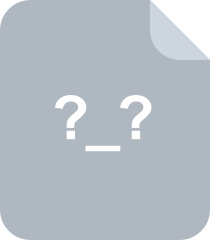
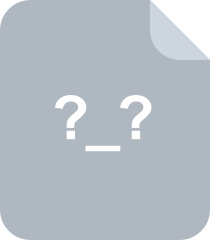
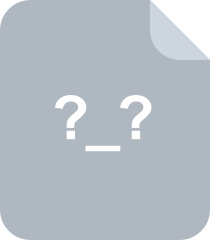
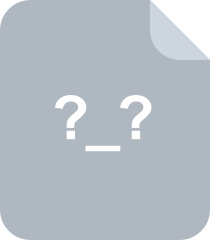
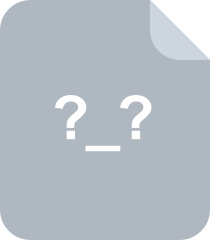
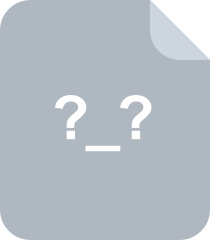
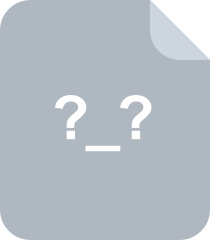
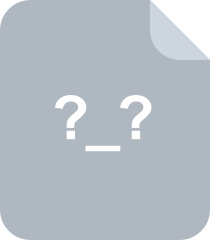
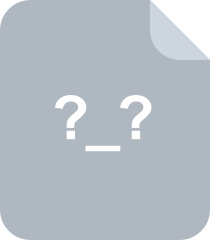
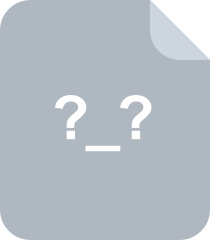
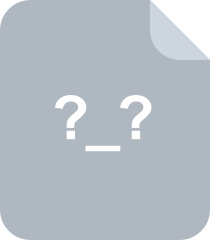
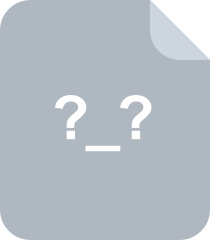
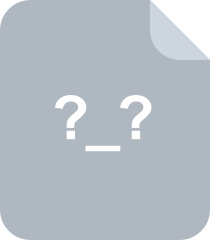
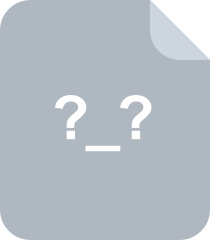
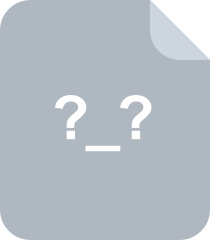
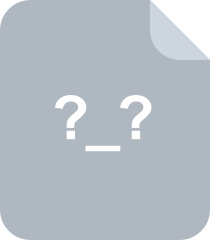
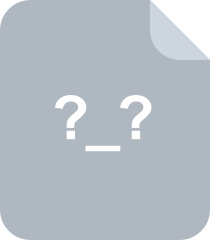
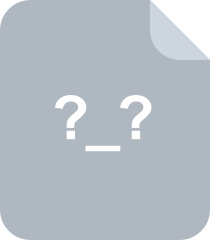
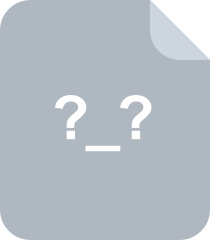
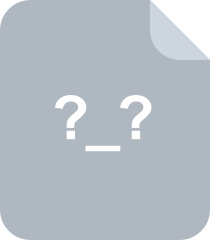
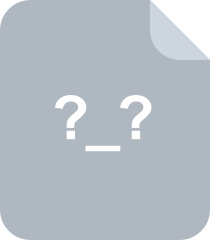
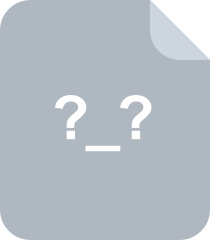
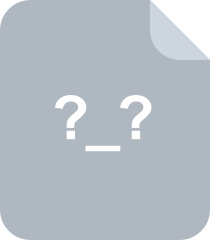
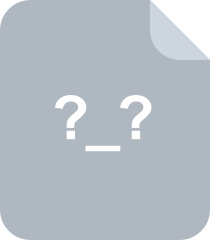
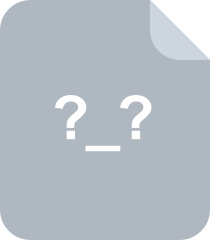
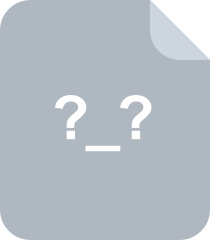
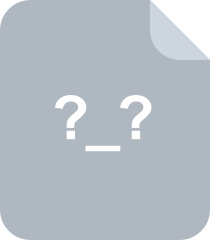
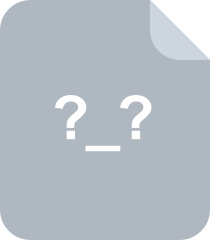
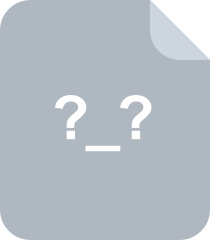
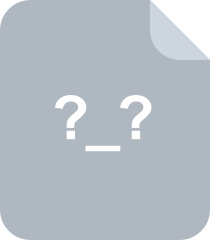
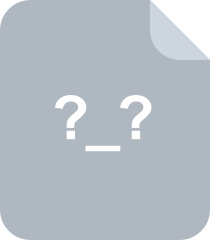
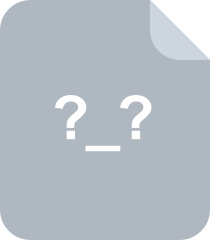
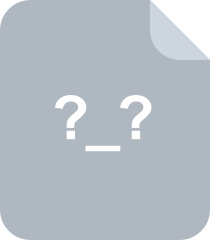
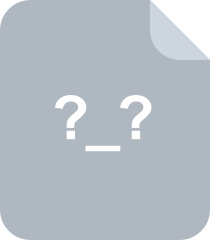
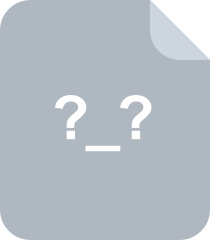
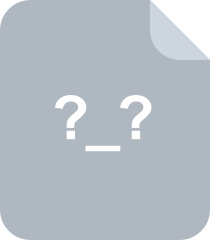
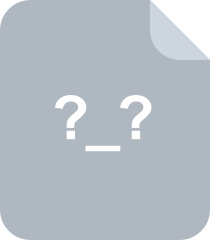
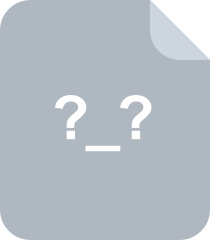
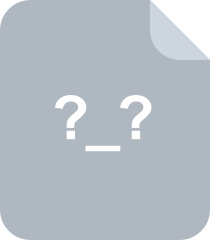
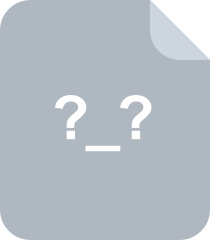
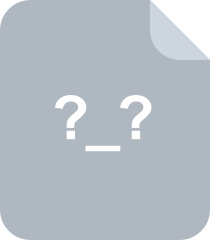
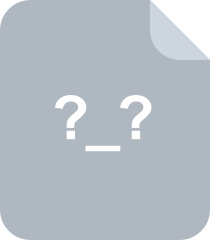
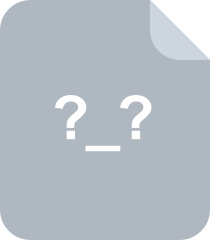
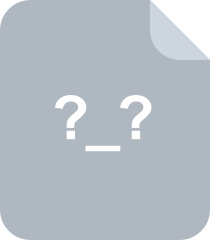
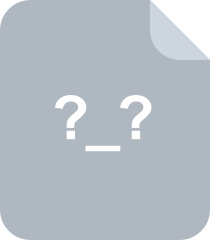
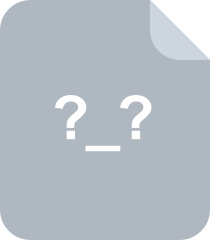
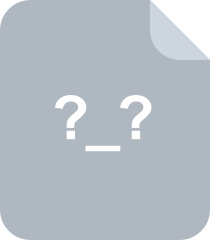
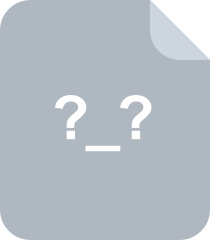
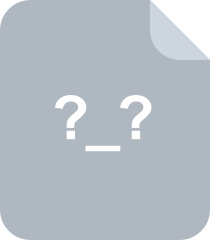
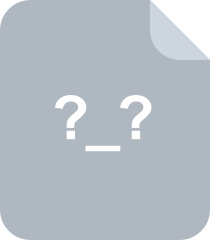
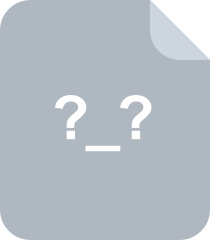
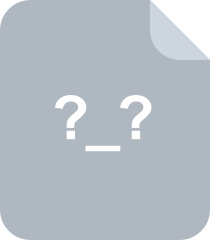
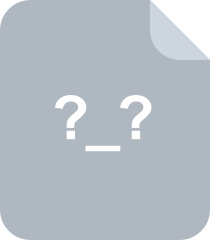
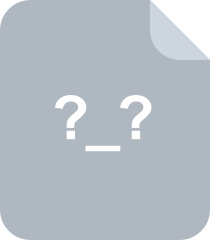
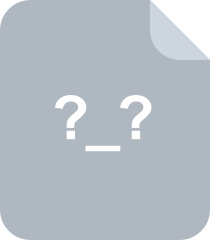
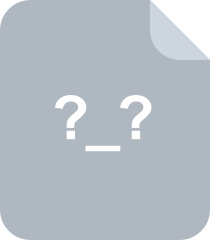
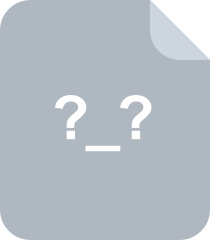
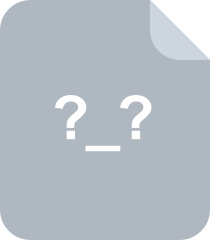
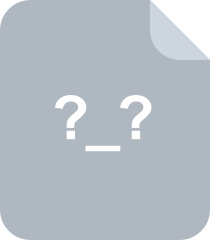
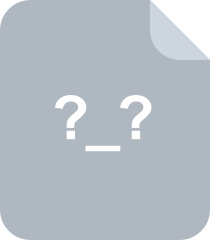
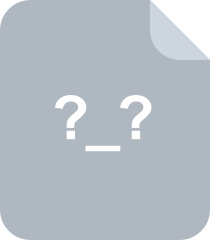
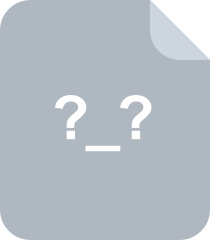
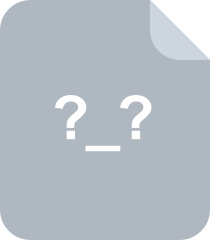
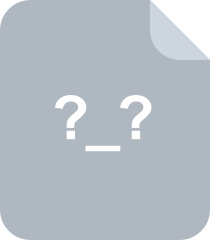
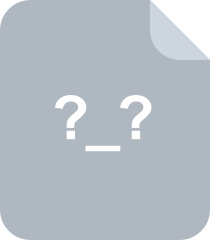
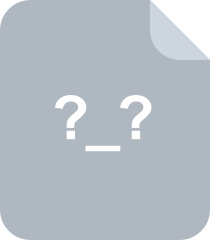
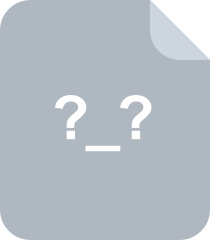
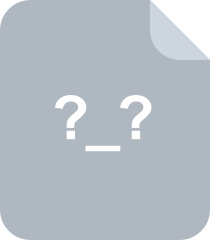
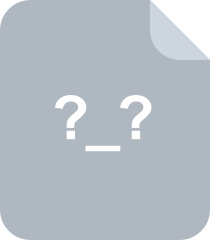
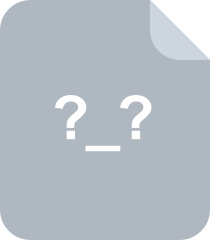
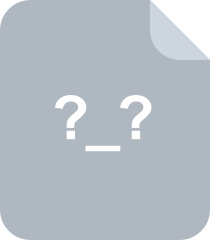
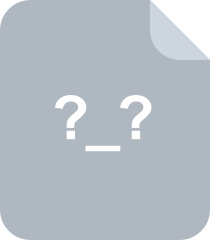
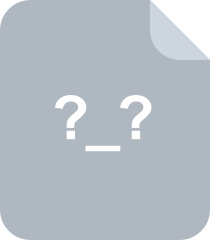
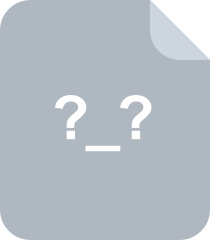
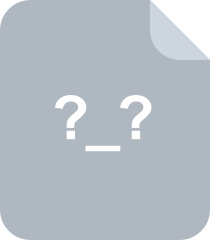
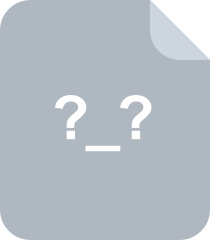
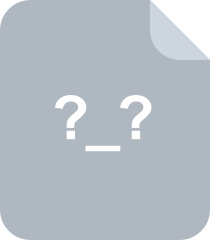
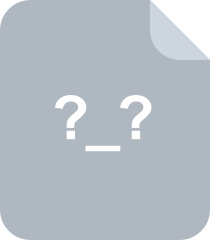
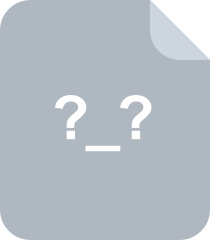
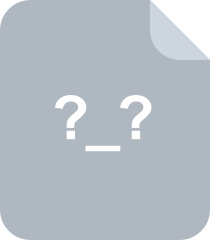
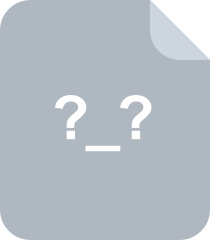
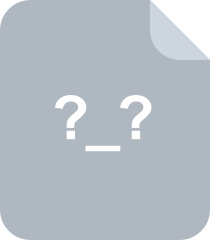
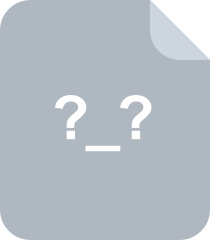
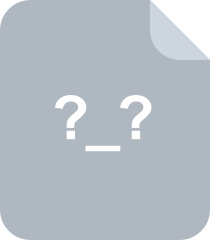
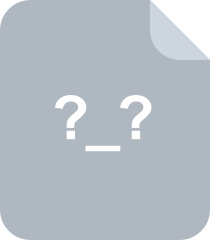
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
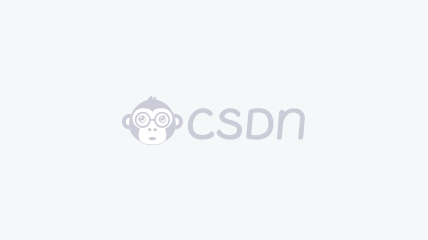

软希源码
- 粉丝: 4237
- 资源: 3591
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

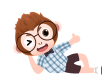
最新资源
- 基于Matlab人脸肤色定理的教师人数统计+源代码+全部数据+文档说明+详细注释+使用说明+截图(高分课程设计)
- 基于Matlab霍夫曼变换的表盘读数识别+源代码+全部数据+文档说明+详细注释+使用说明+截图(高分课程设计)
- 基于Matlab火灾烟雾检测源码带GUI界面+源代码+全部数据+文档说明+详细注释+使用说明+截图(高分课程设计)
- 基于Matlab的恶劣天气交通标志识别系统+源代码+全部数据+文档说明+详细注释+使用说明+截图(高分课程设计)
- 基于MATLAB的霍夫曼变换的表盘示数识别+源代码+全部数据+文档说明+详细注释+使用说明+截图(高分课程设计)
- 基于Matlab的车道线识别系统 +源代码+全部数据+文档说明+详细注释+使用说明+截图(高分课程设计)
- 基于MATLAB的教室人数统计系统带Gui界面+源代码+全部数据+文档说明+详细注释+使用说明+截图(高分课程设计)
- 基于MATLAB的教室人数统计系统带Gui界面+源代码+全部数据+文档说明+详细注释+使用说明+截图(高分课程设计)
- 基于MATLAB 的霍夫曼变换答题卡识别源码+全部数据+文档说明+详细注释+使用说明+截图(高分课程设计)
- 基于Matlab+bp神经网络的神经网络汉字识别系统+源代码+全部数据+文档说明+详细注释+使用说明+截图(高分课程设计)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


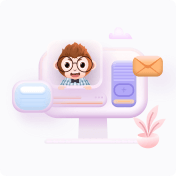
安全验证
文档复制为VIP权益,开通VIP直接复制
