# PyTorch-Deep-Learning
## 1.Overview
**Requirements:**
* Algebra + Probability
* Python
**Relationship:**

**Polular deep learning frameworks:**
* Theano/Tensor Flow
* Caffe/Caffe2
* Torch/PyTorch
## 2.线性模型
**Linear Model:** $\hat{y} = x * w + b$
**Training Loss(Error):** $loss = (\hat{y}-y)^2 = (x * w - y)^2$
**Mean Square Error:** $cost = \frac{1}{N}\sum\limits^{N}_{n=1}(\hat{y_n}-y_n)^2$
## 3.梯度下降算法
**Optimization Problem:** $w^* = \underset{w}{arg min cost}(w)$
### Gradient Descent
**Gradient:** $\frac{\partial cost}{\partial w} = \frac{1}{N}\sum\limits^{N}_{n=1}2\cdot x_n\cdot (x_n \cdot w-y_n)$
**Update:** $w = w - \alpha \frac{\partial cost}{\partial w} = w - \alpha \frac{1}{N}\sum\limits^{N}_{n=1}2\cdot x_n\cdot (x_n \cdot w-y_n)$
### Stochastic Gradient Descent
**Gradient:** $\frac{\partial loss_n}{\partial w} = 2\cdot x_n\cdot (x_n \cdot w-y_n)$
**Update:** $w = w - \alpha \frac{\partial loss_n}{\partial w} = w - \alpha \cdot 2\cdot x_n\cdot (x_n \cdot w-y_n)$
Update weight by every grad of sample of train set. It is possible to jump over local optimum point when compared with GD algorithm. But the time complexity is larger.
## 4.反向传播

### Tensor in PyTorch
**Definition of TensorFlow:** A tensor is a generalization of vectors and matrices to potentially higher dimensions.
* 点——标量(scalar)——零阶张量
* 线——向量(vector)——一阶张量
* 面——矩阵(matrix)——二阶张量
* 体——张量(tensor)
In PyTorch, ***Tensor*** is the important component in constructing dynamic computational graph. Actually, it is a class object.
It contains ***data*** and ***grad***, which storage the value of node and gradient w.r.t(abbreviation:with respect to) loss respectively.

## 5.用PyTorch实现线性回归
In PyTorch, the computational graph is mini-batch fashion.
**Steps:**
1. Prepare dataset
2. Design model using Class
inherit from nn.module
3. Construct loss and optimizer
using PyTorch API
4. Training cycle
forward, backward, update
**Different Optimizer in Linear Regression:**
* torch.optim.Adagrad
* torch.optim.Adam
* torch.optim.Adamax
* torch.optim.ASGD
* torch.optim.LBFGS
* torch.optim.RMSprop
* torch.optim.Rprop
* torch.optim.SGD
## 6.Logistics回归
**Logistic Function:**
$\sigma(x) = \frac{1}{1 + e^{-x}}$
**Loss Function:**
$loss = -(ylog\hat{y} + (1-y)log(1-\hat{y}))$
**Binary Classification Error:**
$cost = -\frac{1}{N}\sum\limits_{n=1}^N y_nlog\hat{y_n}+(1-y_n)log(1-\hat{y_n})$
**Other Sigmoid Function:**

## 7.处理多维特征的输入
## 8.加载数据集
## 9.多分类问题
## 10.卷积神经网络(基础篇)
## 11.卷积神经网络(高级篇)
## 12.循环神经网络(基础篇)
## 13.循环神经网络(高级篇)
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【项目资源】: 包含前端、后端、移动开发、操作系统、人工智能、物联网、信息化管理、数据库、硬件开发、大数据、课程资源、音视频、网站开发等各种技术项目的源码。 包括STM32、ESP8266、PHP、QT、Linux、iOS、C++、Java、python、web、C#、EDA、proteus、RTOS等项目的源码。 【项目质量】: 所有源码都经过严格测试,可以直接运行。 功能在确认正常工作后才上传。 【适用人群】: 适用于希望学习不同技术领域的小白或进阶学习者。 可作为毕设项目、课程设计、大作业、工程实训或初期项目立项。 【附加价值】: 项目具有较高的学习借鉴价值,也可直接拿来修改复刻。 对于有一定基础或热衷于研究的人来说,可以在这些基础代码上进行修改和扩展,实现其他功能。 【沟通交流】: 有任何使用上的问题,欢迎随时与博主沟通,博主会及时解答。 鼓励下载和使用,并欢迎大家互相学习,共同进步。
资源推荐
资源详情
资源评论
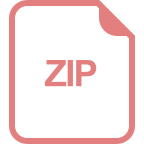
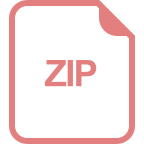
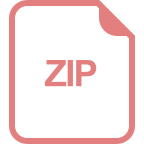
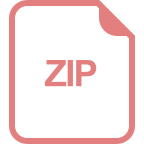
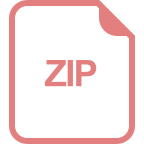
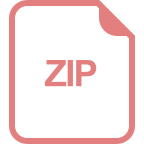
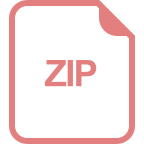
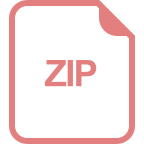
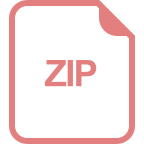
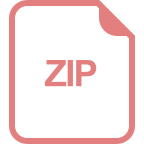
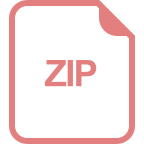
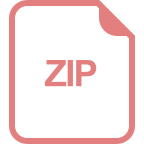
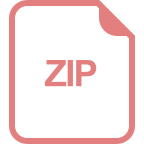
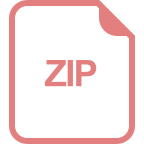
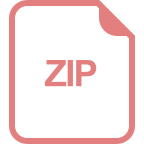
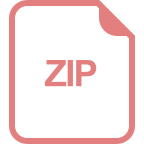
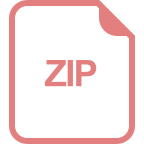
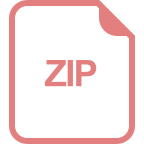
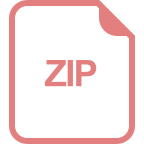
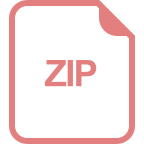
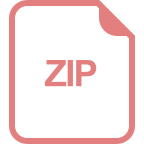
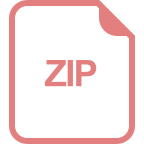
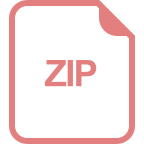
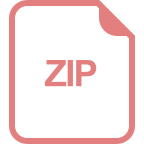
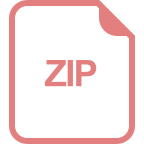
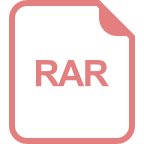
收起资源包目录


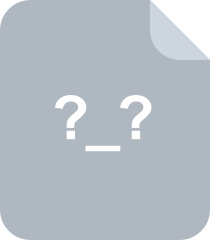



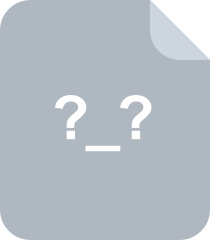
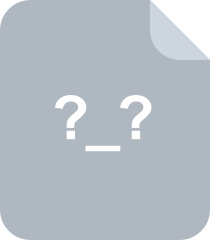
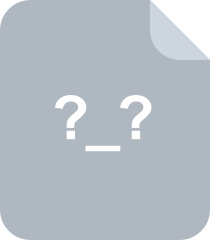
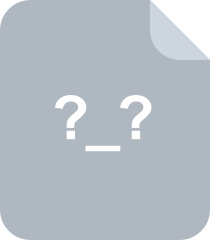
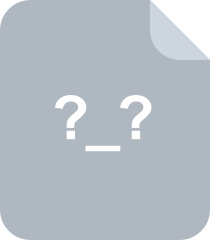
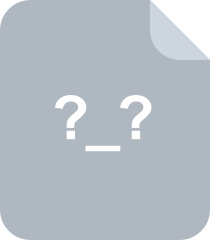

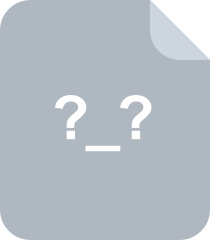
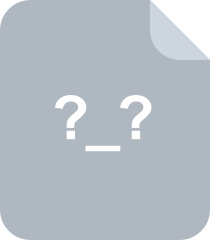
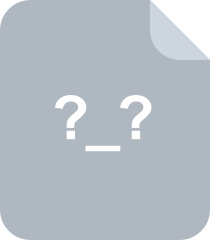
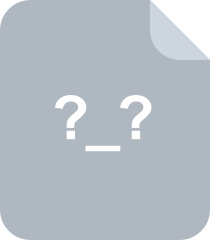
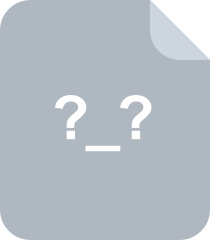
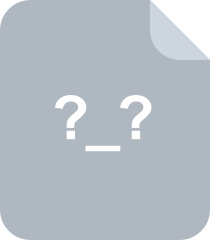
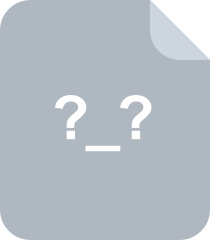
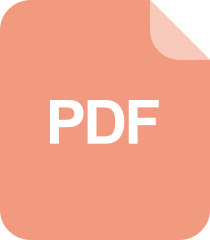
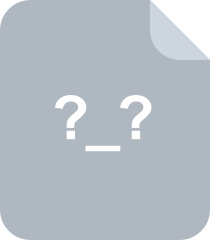
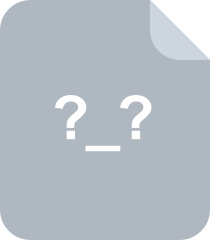
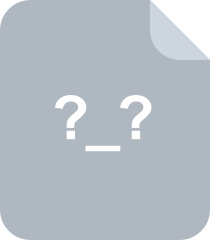
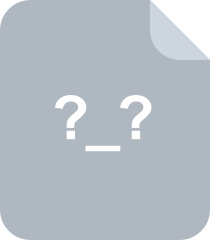

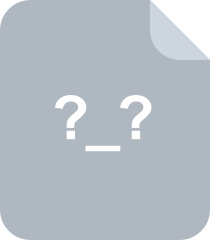
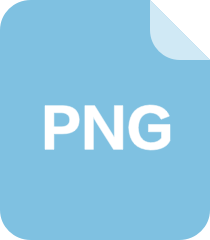
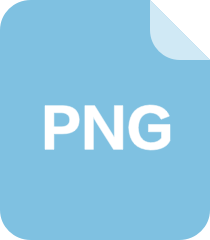
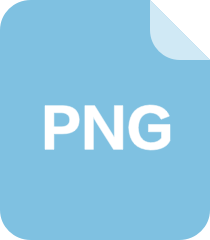


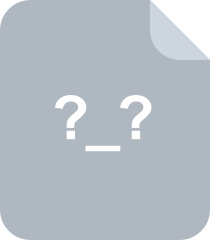
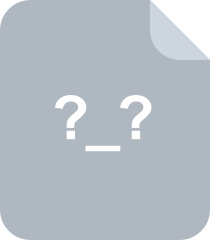
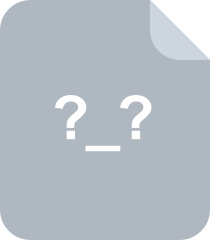
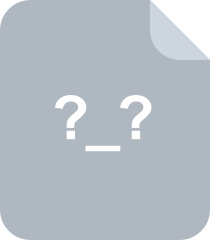
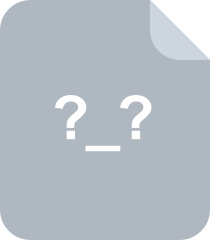
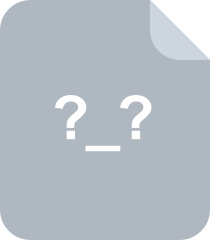

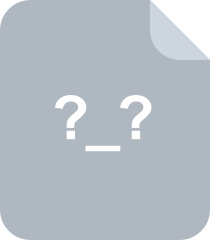
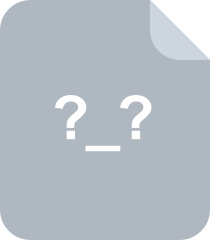
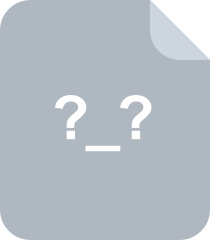
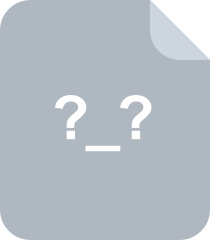
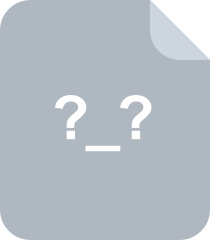
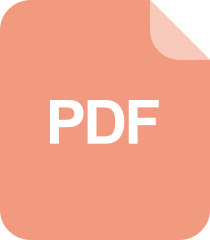
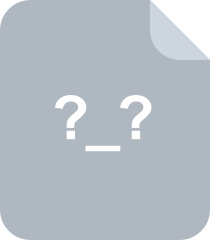
共 36 条
- 1
资源评论
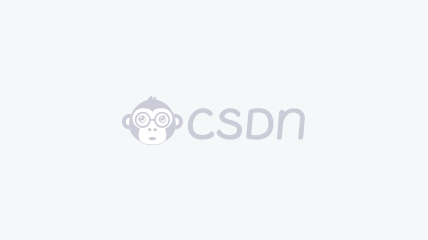


普通网友
- 粉丝: 1w+
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

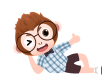
最新资源
- C语言-leetcode题解之28-implement-strstr.c
- C语言-leetcode题解之27-remove-element.c
- C语言-leetcode题解之26-remove-duplicates-from-sorted-array.c
- C语言-leetcode题解之24-swap-nodes-in-pairs.c
- C语言-leetcode题解之22-generate-parentheses.c
- C语言-leetcode题解之21-merge-two-sorted-lists.c
- java-leetcode题解之Online Stock Span.java
- java-leetcode题解之Online Majority Element In Subarray.java
- java-leetcode题解之Odd Even Jump.java
- 计算机毕业设计:python+爬虫+cnki网站爬
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


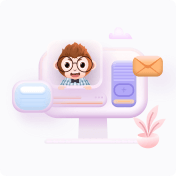
安全验证
文档复制为VIP权益,开通VIP直接复制
