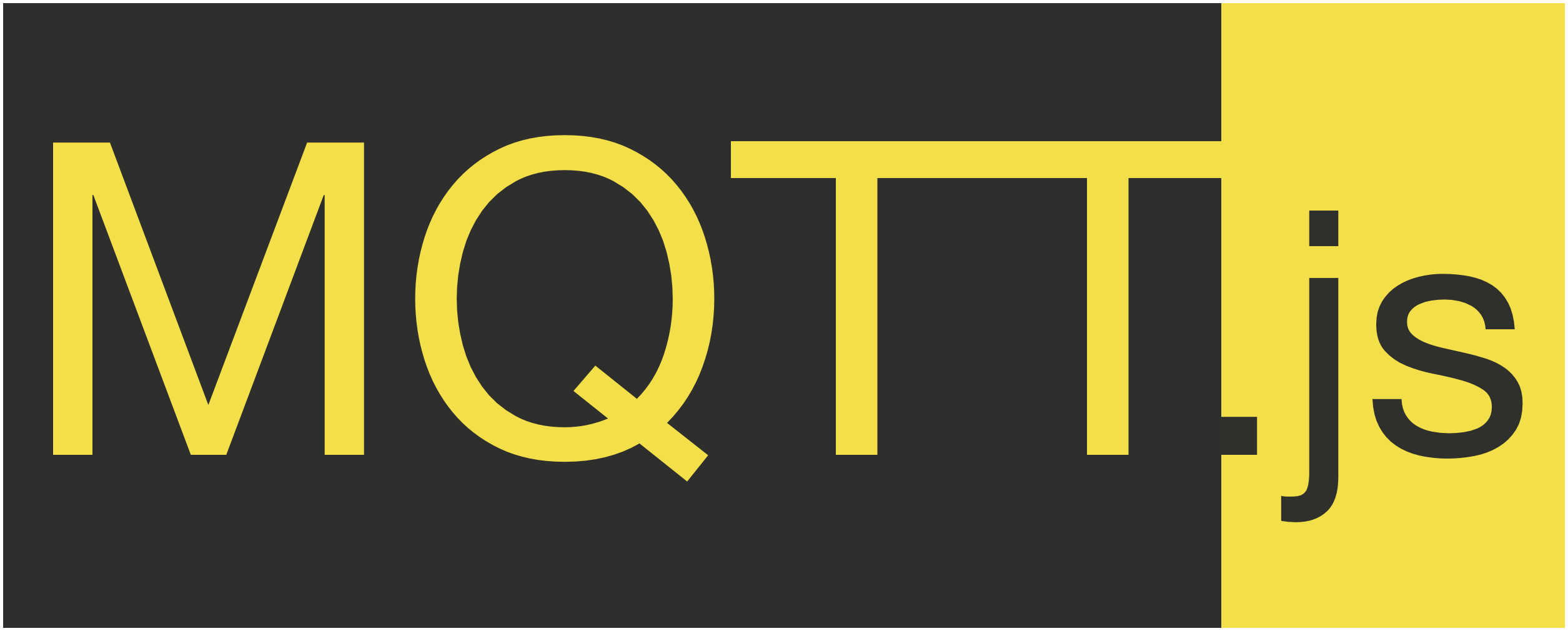
=======
MQTT.js is a client library for the [MQTT](http://mqtt.org/) protocol, written
in JavaScript. And this is a fork of original [MQTT.js](https://github.com/mqttjs/MQTT.js) for Ruff that currently supports only TCP connection.
* [Installation](#install)
* [Example](#example)
* [API](#api)
* [License](#license)
<a name="install"></a>
## Installation
```sh
rap install mqtt
```
<a name="example"></a>
## Example
For the sake of simplicity, let's put the subscriber and the publisher in the same file:
```js
var mqtt = require('mqtt');
var client = mqtt.connect('mqtt://test.mosquitto.org');
client.on('connect', function () {
client.subscribe('presence');
client.publish('presence', 'Hello Ruff!');
});
client.on('message', function (topic, message) {
// message is Buffer
console.log('topic:', topic);
console.log('message:', message.toString());
client.end();
});
```
### Output
```
topic: presense
message: Hello Ruff!
```
<a name="api"></a>
## API
* <a href="#connect"><code>mqtt.<b>connect()</b></code></a>
* <a href="#client"><code>mqtt.<b>Client()</b></code></a>
* <a href="#publish"><code>mqtt.Client#<b>publish()</b></code></a>
* <a href="#subscribe"><code>mqtt.Client#<b>subscribe()</b></code></a>
* <a href="#unsubscribe"><code>mqtt.Client#<b>unsubscribe()</b></code></a>
* <a href="#end"><code>mqtt.Client#<b>end()</b></code></a>
* <a href="#handleMessage"><code>mqtt.Client#<b>handleMessage()</b></code></a>
* <a href="#store"><code>mqtt.<b>Store()</b></code></a>
* <a href="#put"><code>mqtt.Store#<b>put()</b></code></a>
* <a href="#del"><code>mqtt.Store#<b>del()</b></code></a>
* <a href="#createStream"><code>mqtt.Store#<b>createStream()</b></code></a>
* <a href="#close"><code>mqtt.Store#<b>close()</b></code></a>
---
<a name="connect"></a>
### mqtt.connect([url], options)
Connects to the broker specified by the given url and options and
returns a [Client](#client).
The URL can be on the following protocols: 'mqtt', 'mqtts', 'tcp',
'tls', 'ws', 'wss'. The URL can also be an object as returned by
[`URL.parse()`](http://nodejs.org/api/url.html#url_url_parse_urlstr_parsequerystring_slashesdenotehost),
in that case the two objects are merged, i.e. you can pass a single
object with both the URL and the connect options.
You can also specify a `servers` options with content: `[{ host:
'localhost', port: 1883 }, ... ]`, in that case that array is iterated
at every connect.
For all MQTT-related options, see the [Client](#client)
constructor.
---
<a name="client"></a>
### mqtt.Client(streamBuilder, options)
The `Client` class wraps a client connection to an
MQTT broker over an arbitrary transport method (TCP only in this fork).
`Client` automatically handles the following:
* Regular server pings
* QoS flow
* Automatic reconnections
* Start publishing before being connected
The arguments are:
* `streamBuilder` is a function that returns a subclass of the `Stream` class that supports
the `connect` event. Typically a `net.Socket`.
* `options` is the client connection options (see: the [connect packet](https://github.com/mcollina/mqtt-packet#connect)). Defaults:
* `keepalive`: `10` seconds, set to `0` to disable
* `reschedulePings`: reschedule ping messages after sending packets (default `true`)
* `clientId`: `'mqttjs_' + Math.random().toString(16).substr(2, 8)`
* `protocolId`: `'MQTT'`
* `protocolVersion`: `4`
* `clean`: `true`, set to false to receive QoS 1 and 2 messages while
offline
* `reconnectPeriod`: `1000` milliseconds, interval between two
reconnections
* `connectTimeout`: `30 * 1000` milliseconds, time to wait before a
CONNACK is received
* `username`: the username required by your broker, if any
* `password`: the password required by your broker, if any
* `incomingStore`: a [Store](#store) for the incoming packets
* `outgoingStore`: a [Store](#store) for the outgoing packets
* `queueQoSZero`: if connection is broken, queue outgoing QoS zero messages (default `true`)
* `will`: a message that will sent by the broker automatically when
the client disconnect badly. The format is:
* `topic`: the topic to publish
* `payload`: the message to publish
* `qos`: the QoS
* `retain`: the retain flag
If you are connecting to a broker that supports only MQTT 3.1 (not
3.1.1 compliant), you should pass these additional options:
```js
{
protocolId: 'MQIsdp',
protocolVersion: 3
}
```
This is confirmed on RabbitMQ 3.2.4, and on Mosquitto < 1.3. Mosquitto
version 1.3 and 1.4 works fine without those.
#### Event `'connect'`
`function(connack) {}`
Emitted on successful (re)connection (i.e. connack rc=0).
* `connack` received connack packet. When `clean` connection option is `false` and server has a previous session
for `clientId` connection option, then `connack.sessionPresent` flag is `true`. When that is the case,
you may rely on stored session and prefer not to send subscribe commands for the client.
#### Event `'reconnect'`
`function() {}`
Emitted when a reconnect starts.
#### Event `'close'`
`function() {}`
Emitted after a disconnection.
#### Event `'offline'`
`function() {}`
Emitted when the client goes offline.
#### Event `'error'`
`function(error) {}`
Emitted when the client cannot connect (i.e. connack rc != 0) or when a
parsing error occurs.
### Event `'message'`
`function(topic, message, packet) {}`
Emitted when the client receives a publish packet
* `topic` topic of the received packet
* `message` payload of the received packet
* `packet` received packet, as defined in
[mqtt-packet](https://github.com/mcollina/mqtt-packet#publish)
---
<a name="publish"></a>
### mqtt.Client#publish(topic, message, [options], [callback])
Publish a message to a topic
* `topic` is the topic to publish to, `String`
* `message` is the message to publish, `Buffer` or `String`
* `options` is the options to publish with, including:
* `qos` QoS level, `Number`, default `0`
* `retain` retain flag, `Boolean`, default `false`
* `callback` callback fired when the QoS handling completes,
or at the next tick if QoS 0.
---
<a name="subscribe"></a>
### mqtt.Client#subscribe(topic/topic array/topic object, [options], [callback])
Subscribe to a topic or topics
* `topic` is a `String` topic to subscribe to or an `Array` of
topics to subscribe to. It can also be an object, it has as object
keys the topic name and as value the QoS, like `{'test1': 0, 'test2': 1}`.
* `options` is the options to subscribe with, including:
* `qos` qos subscription level, default 0
* `callback` - `function(err, granted)`
callback fired on suback where:
* `err` a subscription error
* `granted` is an array of `{topic, qos}` where:
* `topic` is a subscribed to topic
* `qos` is the granted qos level on it
---
<a name="unsubscribe"></a>
### mqtt.Client#unsubscribe(topic/topic array, [options], [callback])
Unsubscribe from a topic or topics
* `topic` is a `String` topic or an array of topics to unsubscribe from
* `callback` fired on unsuback
---
<a name="end"></a>
### mqtt.Client#end([force], [cb])
Close the client, accepts the following options:
* `force`: passing it to true will close the client right away, without
waiting for the in-flight messages to be acked. This parameter is
optional.
* `cb`: will be called when the client is closed. This parameter is
optional.
---
<a name="handleMessage"></a>
### mqtt.Client#handleMessage(packet, callback)
Handle messages with backpressure support, one at a time.
Override at will, but __always call `callback`__, or the client
will hang.
---
<a name="store"></a>
### mqtt.Store()
In-memory implementation of the message store.
Another implementaion is
[mqtt-level-store](http://npm.im/mqtt-level-store) which uses
[Level-browserify](http://npm.im/level-browserify) to store the infl


妄北y
- 粉丝: 2w+
- 资源: 1万+
最新资源
- 基于java+springboot+vue+mysql的智慧旅游系统 源码+数据库+论文(高分毕业设计).zip
- 基于java+springboot+vue+mysql的中文社区交流平台 源码+数据库+论文(高分毕业设计).zip
- 基于java+springboot+vue+mysql的中医养生系统 源码+数据库+论文(高分毕业设计).zip
- 双机并联自适应阻抗下垂控制仿真 复现一篇核心期刊参考文献 (看图) 图一:双机并联整体仿真图 图二:自适应控制模块,有功频率下垂、无功电压下垂以及加入的自适应阻抗下垂控制策略 图三:两台逆变输出的有
- java作业管理系统设计(源码)
- 新型内置式永磁同步电机设计 随着能源问题的日益突出,环境污染越来越严重,全社会都在提倡绿色环保清洁能源,汽车行业也在朝着节能和环保的理念发展,特别是纯电动汽车作为最清洁的能源汽车是我国新能源汽车发展的
- Windows版本包含nginx-http-flv-module的nginx推流
- 苹果WWDC 2024:苹果开启AI新纪元,引入个人化AI系统Apple intelligence.pdf
- 基于java+ssm+mysql+微信小程序的教学辅助小程序 源码+数据库+论文(高分毕业设计).zip
- 基于java+ssm+mysql+微信小程序的购物系统 源码+数据库+论文(高分毕业设计).zip
- 基于java+ssm+mysql+微信小程序的家庭记账本系统 源码+数据库+论文(高分毕业设计).zip
- 基于java+ssm+mysql+微信小程序的校园水电费管理小程序 源码+数据库+论文(高分毕业设计).zip
- 风光储并网发电系统仿真模型(共直流式) 共直流母线式风光储:风力发电+光伏发电+储能+三相逆变并网 ①光伏Boost:采用电导增量法来实现光伏板最大功率跟踪 ②风机:拓扑采用三相整流电路,控制采用MP
- SpringBoot2 + Java8 实现的考试后台管理系统
- javaC语言试题生成与考试系统(源代码+论文)
- 整车十四自由度simulink模型(仿真+说明文档+参考文献) 资料:仿真+说明文档+参考文献 数据齐全,含说明文档,建模清晰可用,其中十四自由度模型可以控制四个车轮转向和转矩,包括纵向,横向,横摆
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


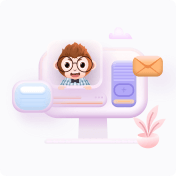