# property-information
[![Build][build-badge]][build]
[![Coverage][coverage-badge]][coverage]
[![Downloads][downloads-badge]][downloads]
[![Size][size-badge]][size]
Info for properties and attributes on the web-platform (HTML, SVG, ARIA, XML,
XMLNS, XLink).
This package follows a sensible naming scheme as defined by [hast][].
## Install
[npm][]:
```sh
npm install property-information
```
## Contents
* [Use](#use)
* [API](#api)
* [`propertyInformation.find(schema, name)`](#propertyinformationfindschema-name)
* [`propertyInformation.normalize(name)`](#propertyinformationnormalizename)
* [`propertyInformation.html`](#propertyinformationhtml)
* [`propertyInformation.svg`](#propertyinformationsvg)
* [`hastToReact`](#hasttoreact)
* [Support](#support)
* [Related](#related)
* [License](#license)
## Use
```js
var info = require('property-information')
console.log(info.find(info.html, 'className'))
// Or: info.find(info.html, 'class')
console.log(info.find(info.svg, 'horiz-adv-x'))
// Or: info.find(info.svg, 'horizAdvX')
console.log(info.find(info.svg, 'xlink:arcrole'))
// Or: info.find(info.svg, 'xLinkArcRole')
console.log(info.find(info.html, 'xmlLang'))
// Or: info.find(info.html, 'xml:lang')
console.log(info.find(info.html, 'ariaValueNow'))
// Or: info.find(info.html, 'aria-valuenow')
```
Yields:
```js
{ space: 'html',
attribute: 'class',
property: 'className',
spaceSeparated: true }
{ space: 'svg',
attribute: 'horiz-adv-x',
property: 'horizAdvX',
number: true }
{ space: 'xlink', attribute: 'xlink:arcrole', property: 'xLinkArcrole' }
{ space: 'xml', attribute: 'xml:lang', property: 'xmlLang' }
{ attribute: 'aria-valuenow', property: 'ariaValueNow', number: true }
```
## API
### `propertyInformation.find(schema, name)`
Look up info on a property.
In most cases, the given `schema` contains info on the property.
All standard, most legacy, and some non-standard properties are supported.
For these cases, the returned [`Info`][info] has hints about value of the
property.
`name` can be a [valid data attribute or property][data], in which case an
[`Info`][info] object with the correctly cased `attribute` and `property` is
returned.
`name` can be an unknown attribute, in which case an [`Info`][info] object
with `attribute` and `property` set to the given name is returned.
It is not recommended to provide unsupported legacy or recently specced
properties.
#### Parameters
* `schema` ([`Schema`][schema])
— Either `propertyInformation.html` or `propertyInformation.svg`
* `name` (`string`)
— An attribute-like or property-like name that is passed through
[`normalize`][normalize] to find the correct info
#### Returns
[`Info`][info].
#### Note
`find` can be accessed directly from `require('property-information/find')` as
well.
#### Example
Aside from the aforementioned example, which shows known HTML, SVG, XML, XLink,
and ARIA support, data properties, and attributes are also supported:
```js
console.log(info.find(info.html, 'data-date-of-birth'))
// Or: info.find(info.html, 'dataDateOfBirth')
```
Yields:
```js
{ attribute: 'data-date-of-birth', property: 'dataDateOfBirth' }
```
Unknown values are passed through untouched:
```js
console.log(info.find(info.html, 'un-Known'))
```
Yields:
```js
{ attribute: 'un-Known', property: 'un-Known' }
```
### `propertyInformation.normalize(name)`
Get the cleaned case-insensitive form of an attribute or a property.
#### Parameters
* `name` (`string`) — An attribute-like or property-like name
#### Returns
`string` that can be used to look up the properly cased property in a
[`Schema`][schema].
#### Note
`normalize` can be accessed directly from
`require('property-information/normalize')` as well.
#### Example
```js
info.html.normal[info.normalize('for')] // => 'htmlFor'
info.svg.normal[info.normalize('VIEWBOX')] // => 'viewBox'
info.html.normal[info.normalize('unknown')] // => undefined
info.html.normal[info.normalize('accept-charset')] // => 'acceptCharset'
```
### `propertyInformation.html`
### `propertyInformation.svg`
[`Schema`][schema] for either HTML or SVG, containing info on properties from
the primary space (HTML or SVG) and related embedded spaces (ARIA, XML, XMLNS,
XLink).
#### Note
`html` and `svg` can be accessed directly from
`require('property-information/html')` and `require('property-information/svg')`
as well.
#### Example
```js
console.log(info.html.property.htmlFor)
console.log(info.svg.property.viewBox)
console.log(info.html.property.unknown)
```
Yields:
```js
{ space: 'html',
attribute: 'for',
property: 'htmlFor',
spaceSeparated: true }
{ space: 'svg', attribute: 'viewBox', property: 'viewBox' }
undefined
```
#### `Schema`
A schema for a primary space.
* `space` (`'html'` or `'svg'`) — Primary space of the schema
* `normal` (`Object.<string>`) — Object mapping normalized attributes and
properties to properly cased properties
* `property` ([`Object.<Info>`][info]) — Object mapping properties to info
#### `Info`
Info on a property.
* `space` (`'html'`, `'svg'`, `'xml'`, `'xlink'`, `'xmlns'`, optional)
— [Space][namespace] of the property
* `attribute` (`string`) — Attribute name for the property that could be used
in markup (for example: `'aria-describedby'`, `'allowfullscreen'`,
`'xml:lang'`, `'for'`, or `'charoff'`)
* `property` (`string`) — JavaScript-style camel-cased name, based on the
DOM, but sometimes different (for example: `'ariaDescribedBy'`,
`'allowFullScreen'`, `'xmlLang'`, `'htmlFor'`, `'charOff'`)
* `boolean` (`boolean`) — The property is `boolean`.
The default value of this property is false, so it can be omitted
* `booleanish` (`boolean`) — The property is a `boolean`.
The default value of this property is something other than false, so
`false` must persist.
The value can hold a string (as is the case with `ariaChecked` and its
`'mixed'` value)
* `overloadedBoolean` (`boolean`) — The property is `boolean`.
The default value of this property is false, so it can be omitted.
The value can hold a string (as is the case with `download` as its value
reflects the name to use for the downloaded file)
* `number` (`boolean`) — The property is `number`.
These values can sometimes hold a string
* `spaceSeparated` (`boolean`) — The property is a list separated by spaces
(for example, `className`)
* `commaSeparated` (`boolean`) — The property is a list separated by commas
(for example, `srcSet`)
* `commaOrSpaceSeparated` (`boolean`) — The property is a list separated by
commas or spaces (for example, `strokeDashArray`)
* `mustUseProperty` (`boolean`) — If a DOM is used, setting the property
should be used for the change to take effect (this is true only for
`'checked'`, `'multiple'`, `'muted'`, and `'selected'`)
* `defined` (`boolean`) — The property is [defined by a space](#support).
This is true for values in HTML (including data and ARIA), SVG, XML,
XMLNS, and XLink.
These values can only be accessed through `find`.
### `hastToReact`
> Accessible through `require('property-information/hast-to-react.json')`
[hast][] is close to [React][], but differs in a couple of cases.
To get a React property from a hast property, check if it is in
[`hast-to-react`][hast-to-react] (`Object.<string>`), if it is, then use the
corresponding value, otherwise, use the hast property.
## Support
<!--list start-->
| Property | Attribute | Space |
| ---------------------------- | ------------------------------ | ------------- |
| `aLink` | `alink` | `html` |
| `abbr` | `abbr` | `html` |
| `about` | `about` | `svg` |
| `accentHeight`


妄北y
- 粉丝: 2w+
- 资源: 1万+
最新资源
- daily-interview-master整理的面经,内容包括机器学习,CV,NLP
- Oracle 11g安装步骤详谈.docx
- 第十四届蓝桥杯单片机组国二代码库.zip
- Java学习路径:从基础到高级的企业级开发指南
- 博客管理系统的前台代码
- 1998-2022年各地级市第二产业占GDP比重数据/地级市第二产业占比数据(全市).xlsx
- 管家婆辉煌食品版TOP13.3
- OpenCV for Unity 2.3.4
- 关于DeepSeek的全网相关资源整理及实用指南
- ollama部署包+deepseek部署指南+deepseek技术文档
- 基于STM32F103系列芯片的OTA远程升级方案:WiFi连接,稳定可靠的BIN文件升级流程,基于STM32F103系列芯片的OTA远程升级方案:WiFi连接,稳定可靠的BIN文件升级流程,stm3
- 管家婆辉煌食品版TOP13.32
- 项目到期后自动关闭11
- 基于PLC的85#三菱组态王药片装瓶自动控制系统的设计与实现,基于PLC与三菱组态王的自动控制系统在药片装瓶过程中的应用与优化,85#三菱组态王基于PLC的药片装瓶自动控制系统 ,三菱组态王
- 基于MATLAB的图像中值滤波代码
- 沈阳日立笔试题(4).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


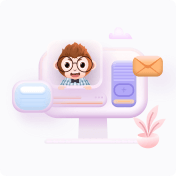