/************************************************************************
* Copyright (C) 2021 Chenyang https://wcy-dt.github.io *
* *
* @file bankdb.cpp *
* @brief Bankdb simulates a database for the project. The speed of *
* this database is slow for that we must ensure the safety *
* of every deal. *
* @author Chenyang *
* @date 2021 - 07 *
************************************************************************/
#include "bankdb.h"
#include <QDebug>
#include <QDialog>
#include <QMessageBox>
#include <fstream>
#include <map>
#include <time.h>
using namespace std;
/**
* @brief initialization and read from the file
*/
bankdb::bankdb()
{
GetFile();
}
/**
* @brief read from the file which stores the data
*/
void bankdb::GetFile()
{
vAccount.clear();
mAccount.clear();
mMoney.clear();
int iNumOfAccounts; /// number of accounts
int iNumOfFlow; /// number of flows
ifstream iFile("bankdb.db", ios::binary);
if (!iFile) /// the file not exist
{
iNumOfAccounts = 0; /// zero account
WriteFile();
return;
}
iFile >> iNumOfAccounts;
for (int i = 0; i < iNumOfAccounts; i++)
{
accountInfo tmpAccount; /// get a record, and pushback to vector
string strTmpString; /// for the convenience of infile
/**
* @note here i use a lot of getline(), for that spaces may exist in the strings
* getline(iFile, strTmpString) is for jump over ENTER
*/
iFile >> tmpAccount.strNumber;
getline(iFile, strTmpString);
getline(iFile, tmpAccount.strName);
getline(iFile, tmpAccount.strPasswd);
getline(iFile, tmpAccount.strAddress);
iFile >> tmpAccount.iType >> tmpAccount.dInterest >> tmpAccount.bLost >> tmpAccount.tLostTime;
getline(iFile, strTmpString);
getline(iFile, tmpAccount.strOperator);
iFile >> iNumOfFlow;
mMoney[tmpAccount.strNumber] = 0; /// initialize the money in the account
for (int j = 0; j < iNumOfFlow; j++)
{
flowInfo tmpFlow; /// get a flow, and pushback to vector
iFile >> tmpFlow.tTime >> tmpFlow.dMoney >> tmpFlow.iOperationType;
getline(iFile, strTmpString);
getline(iFile, tmpFlow.strOperator);
if (tmpFlow.iOperationType == 0) /// deposit
mMoney[tmpAccount.strNumber] += tmpFlow.dMoney;
else /// withdraw
mMoney[tmpAccount.strNumber] -= tmpFlow.dMoney;
tmpAccount.vFlow.push_back(tmpFlow);
}
mAccount[tmpAccount.strNumber] = vAccount.size(); /// map account number to the index
/// @see bankdb.h
vAccount.push_back(tmpAccount);
}
}
/**
* @brief write data to the file
*/
void bankdb::WriteFile()
{
int iNumOfAccounts = vAccount.size();
/**
* @note use trunc for that it is not much slower than find where to insert or edit
*/
ofstream oFile("bankdb.db", ios::trunc | ios::binary);
oFile << iNumOfAccounts << "\n";
for (int i = 0; i < iNumOfAccounts; i++)
{
accountInfo tmpAccount = vAccount[i];
int iNumOfFlow = tmpAccount.vFlow.size();
oFile << tmpAccount.strNumber << "\n"
<< tmpAccount.strName << "\n"
<< tmpAccount.strPasswd << "\n"
<< tmpAccount.strAddress << "\n"
<< tmpAccount.iType << "\n"
<< tmpAccount.dInterest << "\n"
<< tmpAccount.bLost << "\n"
<< tmpAccount.tLostTime << "\n"
<< tmpAccount.strOperator << "\n"
<< iNumOfFlow << "\n";
for (int j = 0; j < iNumOfFlow; j++)
{
flowInfo tmpFlow = tmpAccount.vFlow[j];
oFile << tmpFlow.tTime << "\n"
<< tmpFlow.dMoney << "\n"
<< tmpFlow.iOperationType << "\n"
<< tmpFlow.strOperator << "\n";
}
}
}
/**
* @brief add an account
*
* @param [in] strNum number of the account
* @param [in] strNam name of the account owner
* @param [in] strPas password of the account
* @param [in] strAdd address of the account owner
* @param [in] iType type of the account
* @param [in] dInt interest of the account
* @param [in] strOpe operator of the deal
*
* requirments of these params @see bankdb.h
*/
void bankdb::AddAccount(string strNum, string strNam, string strPas, string strAdd, int iTyp, double dInt, string strOpe)
{
accountInfo tmpAccountInfo;
tmpAccountInfo.strNumber = strNum;
tmpAccountInfo.strName = strNam;
tmpAccountInfo.strPasswd = strPas;
tmpAccountInfo.strAddress = strAdd;
tmpAccountInfo.iType = iTyp;
tmpAccountInfo.dInterest = dInt;
tmpAccountInfo.bLost = false;
tmpAccountInfo.tLostTime = time(nullptr);
tmpAccountInfo.strOperator = strOpe;
vAccount.push_back(tmpAccountInfo);
vAccount[vAccount.size() - 1].vFlow.clear();
/**
* @note do not delete Getfile()!
* i do not think it is necessary, but without it the program has bugs
* it is to refresh the memory
*/
WriteFile();
GetFile();
}
/**
* @brief edit an account
*
* @param [in] strNum number of the account [unchangable]
* @param [in] strNam name of the account owner
* @param [in] strAdd address of the account owner
* @param [in] iType type of the account
* @param [in] dInt interest of the account
*
* requirments of these params @see bankdb.h
*/
void bankdb::EditAccount(string strNum, string strNam, string strAdd, int iTyp, double dInt)
{
vAccount[mAccount[strNum]].strName = strNam;
vAccount[mAccount[strNum]].strAddress = strAdd;
vAccount[mAccount[strNum]].iType = iTyp;
vAccount[mAccount[strNum]].dInterest = dInt;
WriteFile();
GetFile();
}
/**
* @brief edit password
*
* @param [in] strNum number of the account [unchangable]
* @param [in] strPas password of the account
*
* requirments of these params @see bankdb.h
*/
void bankdb::EditPasswd(string strNum, string strPas)
{
vAccount[mAccount[strNum]].strPasswd = strPas;
WriteFile();
}
/**
* @brief marked as lost
*
* @param [in] strNum number of the account [unchangable]
* @param [in] tTim time of the operation
*
* requirments of these params @see bankdb.h
*/
void bankdb::SetLost(string strNum, time_t tTim)
{
vAccount[mAccount[strNum]].bLost = true;
vAccount[mAccount[strNum]].tLostTime = tTim;
WriteFile();
}
void bankdb::RidLost(string strNum)
{
vAccount[mAccount[strNum]].bLost = false;
vAccount[mAccount[strNum]].tLostTime = 0;
WriteFile();
}
/**
* @brief judge whether the account exists
*
* @param [in] strNum number of the account
*
* @retval true finded
* false not finded
*/
bool bankdb::ExistAccount(string strNumber)
{
/**
* @note a common way to find sth. in a map
*/
map<string, int>::iterator iter;
iter = mAccount.find(strNumber);
return (iter != mAccount.end());
}
/**
* @brief judge whether the account number matches the password
*
* @param [in] strNumber number of the account
* @param [in] strPasswd password of the account
*
* @retval true match
* false not match
*/
bool bankdb::CheckAccount(string strNumber, string strPasswd)
{
if (!ExistAccount(strNumber)) /// the account does not exist
return false;
return (vAccount[mAccount[strNumber]].strPasswd == strPasswd);
}
/**
* @brief get name by the account number
*
* @param [in] strNum number of the account
*
* @return the name @see bandb.h
*/
strin
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
软件开发设计:PHP、QT、应用软件开发、系统软件开发、移动应用开发、网站开发C++、Java、python、web、C#等语言的项目开发与学习资料 硬件与设备:单片机、EDA、proteus、RTOS、包括计算机硬件、服务器、网络设备、存储设备、移动设备等 操作系统:LInux、IOS、树莓派、安卓开发、微机操作系统、网络操作系统、分布式操作系统等。此外,还有嵌入式操作系统、智能操作系统等。 网络与通信:数据传输、信号处理、网络协议、网络与通信硬件、网络安全网络与通信是一个非常广泛的领域,它涉及到计算机科学、电子工程、数学等多个学科的知识。 云计算与大数据:数据集、包括云计算平台、大数据分析、人工智能、机器学习等,云计算是一种基于互联网的计算方式,通过这种方式,共享的软硬件资源和信息可以按需提供给计算机和其他设备。
资源推荐
资源详情
资源评论
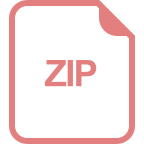
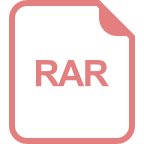
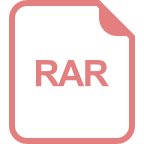
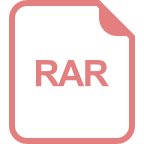
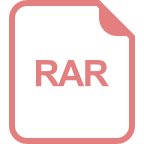
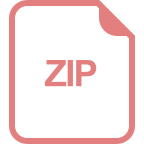
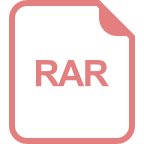
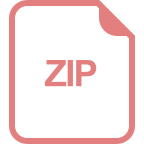
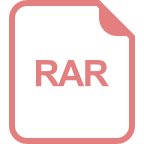
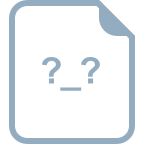
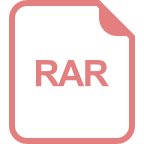
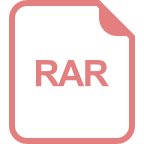
收起资源包目录


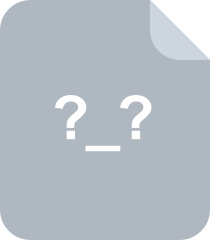
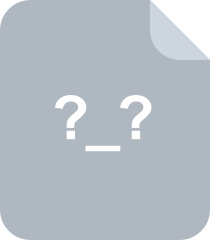
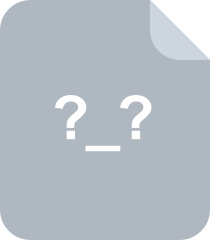
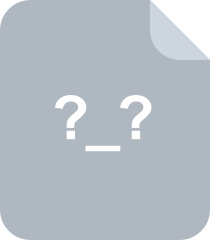
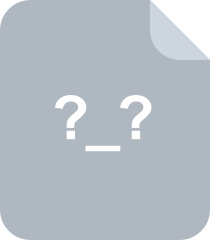
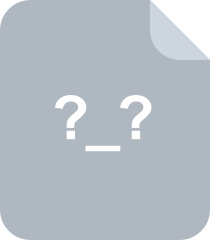
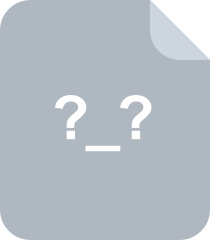
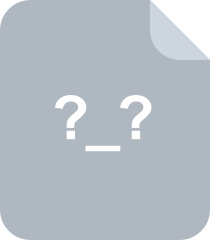
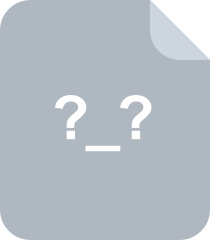
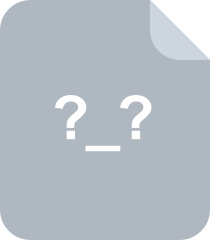
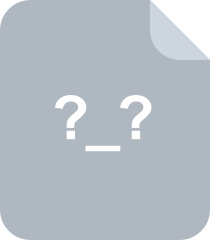
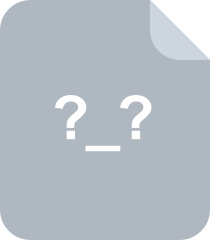
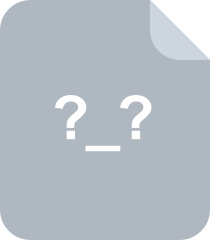
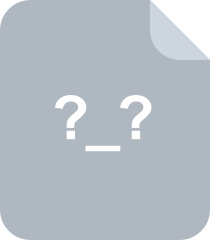
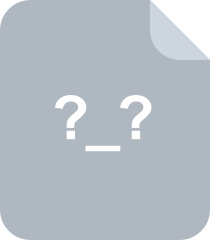
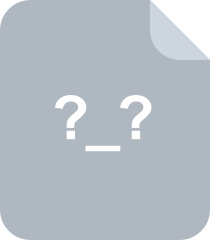
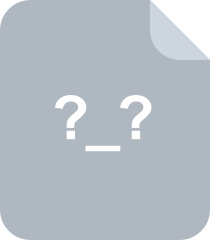
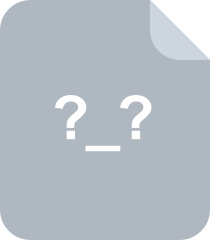
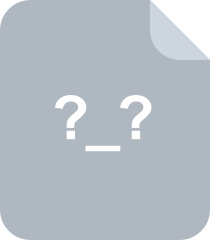
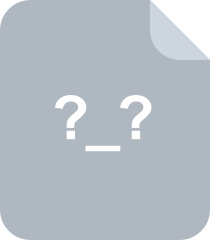
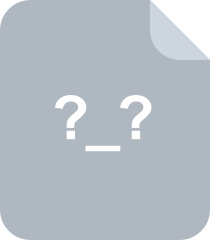
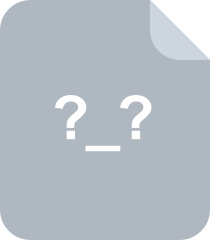
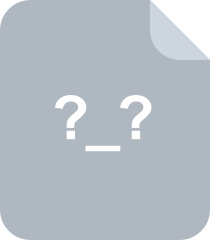
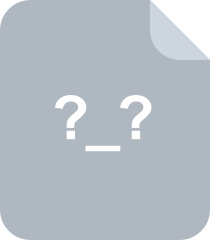
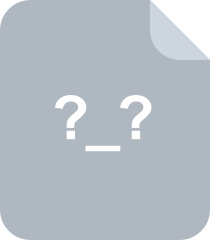
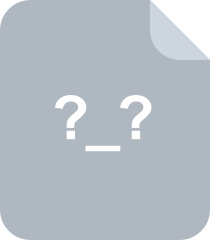
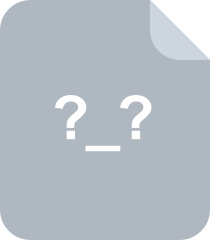
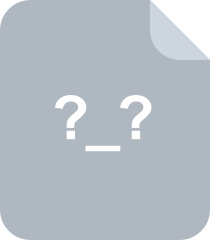
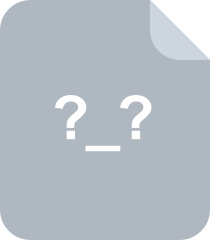
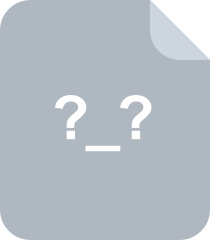
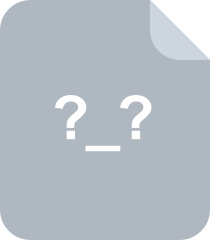
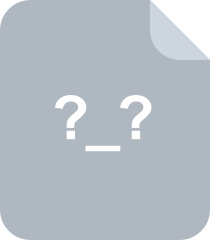
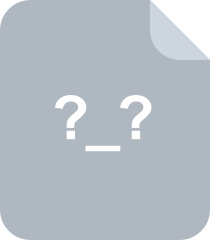
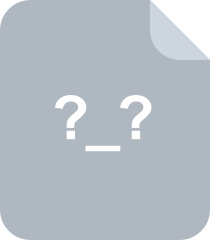
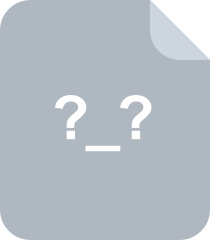
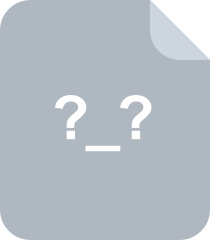
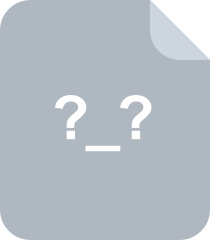
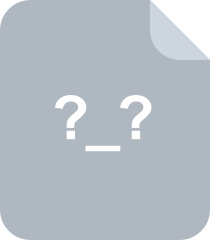
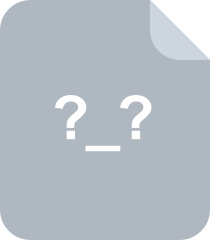
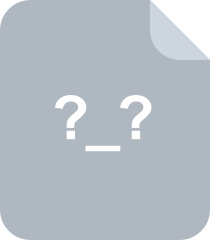
共 40 条
- 1
资源评论
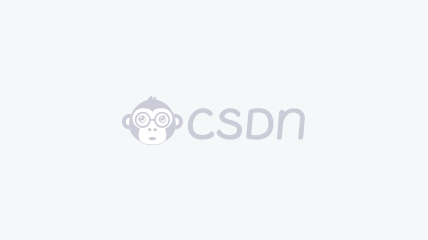

妄北y
- 粉丝: 1w+
- 资源: 1万+

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

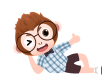
最新资源
- [咒术回战][21][咒术甲子园][中文][1080P].mp4
- SpringBoot框架示例:整合SpringMVC、MyBatis、安全框架Shiro、页面布局框架Sitemesh.zip
- SpringBoot集成thumbnailator图片压缩.zip
- SpringBoot发送邮件神器,只需简单配置即可,支持自定义模板.zip
- SpringBoot的定时调用的加强工具,实现定时任务动态管理,后续加入可视化管理、调度日志、集群任务统一管理.zip
- springboot-starter-gemini 一个基于gemini提供的springboot sdk.zip
- springboot+vue实现简单的前后端分离.zip
- Springboot、SpringCloud开发脚手架,集合各种常用框架使用案例.zip
- SpringBoot 全家桶 - 本项目对目前Web开发中常用的各个技术,通过和SpringBoot的集成.zip
- 随机森林RF葡萄酒分类,随机森林二分类(代码完整,数据齐全)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


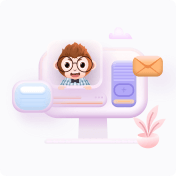
安全验证
文档复制为VIP权益,开通VIP直接复制
