# Ono (斧)
**A sensible way to deal with XML & HTML for iOS & Mac OS X**
XML support in Cocoa isn't great (unless, of course, the XML in question is a `.plist`). `NSXMLParser` forces a cumbersome delegate model, which is extremely inconvenient to implement. `NSXMLDocument` is a bit nicer to use, but only works on Mac OS X, and has a large memory footprint.
**Ono makes working with XML & HTML as nice as JSON.**
Whether your app needs to interface with a XML-RPC webservice, scrape a website, or parse an RSS feed, Ono will make your day a whole lot less terrible.
> Ono (斧) means "axe", in homage to [Nokogiri](http://nokogiri.org) (鋸), which means "saw".
> Using [AFNetworking](https://github.com/AFNetworking/AFNetworking)? Easily integrate Ono into your networking stack with [AFOnoResponseSerializer](https://github.com/AFNetworking/AFOnoResponseSerializer).
## Features
- Simple, modern API following standard Objective-C conventions, including extensive use of blocks and `NSFastEnumeration`
- Extremely performant document parsing and traversal, powered by `libxml2`
- Support for both [XPath](http://en.wikipedia.org/wiki/XPath) and [CSS](http://en.wikipedia.org/wiki/Cascading_Style_Sheets) queries
- Automatic conversion of date and number values
- Correct, common-sense handling of XML namespaces for elements and attributes
- Ability to load HTML and XML documents from either `NSString` or `NSData`
- Full documentation
- Comprehensive test suite
## Installation
[CocoaPods](http://cocoapods.org) is the recommended method of installing Ono. Simply add the following line to your `Podfile`:
#### Podfile
```ruby
pod 'Ono'
```
## Usage
```objective-c
#import "Ono.h"
NSData *data = ...;
NSError *error;
ONOXMLDocument *document = [ONOXMLDocument XMLDocumentWithData:data error:&error];
for (ONOXMLElement *element in document.rootElement.children) {
NSLog(@"%@: %@", element.tag, element.attributes);
}
// Support for Namespaces
NSString *author = [[document.rootElement firstChildWithTag:@"creator" inNamespace:@"dc"] stringValue];
// Automatic Conversion for Number & Date Values
NSDate *date = [[document.rootElement firstChildWithTag:@"created_at"] dateValue]; // ISO 8601 Timestamp
NSInteger numberOfWords = [[[document.rootElement firstChildWithTag:@"word_count"] numberValue] integerValue];
BOOL isPublished = [[[document.rootElement firstChildWithTag:@"is_published"] numberValue] boolValue];
// Convenient Accessors for Attributes
NSString *unit = [document.rootElement firstChildWithTag:@"Length"][@"unit"];
NSDictionary *authorAttributes = [[document.rootElement firstChildWithTag:@"author"] attributes];
// Support for XPath & CSS Queries
[document enumerateElementsWithXPath:@"//Content" usingBlock:^(ONOXMLElement *element, NSUInteger idx, BOOL *stop) {
NSLog(@"%@", element);
}];
```
## Demo
Build and run the example project in Xcode to see `Ono` in action.
## Requirements
Ono is compatible with iOS 5 and higher, as well as Mac OS X 10.7 and higher. It requires the `libxml2` library, which is included automatically when installed with CocoaPods, or can be added manually by adding "libxml2.dylib" to the target's "Link Binary With Libraries" build phase.
### Contact
[Mattt Thompson](http://github.com/mattt)
[@mattt](https://twitter.com/mattt)
## License
Ono is available under the MIT license. See the LICENSE file for more info.
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
软件开发设计:PHP、QT、应用软件开发、系统软件开发、移动应用开发、网站开发C++、Java、python、web、C#等语言的项目开发与学习资料 硬件与设备:单片机、EDA、proteus、RTOS、包括计算机硬件、服务器、网络设备、存储设备、移动设备等 操作系统:LInux、IOS、树莓派、安卓开发、微机操作系统、网络操作系统、分布式操作系统等。此外,还有嵌入式操作系统、智能操作系统等。 网络与通信:数据传输、信号处理、网络协议、网络与通信硬件、网络安全网络与通信是一个非常广泛的领域,它涉及到计算机科学、电子工程、数学等多个学科的知识。 云计算与大数据:数据集、包括云计算平台、大数据分析、人工智能、机器学习等,云计算是一种基于互联网的计算方式,通过这种方式,共享的软硬件资源和信息可以按需提供给计算机和其他设备。
资源推荐
资源详情
资源评论
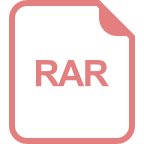
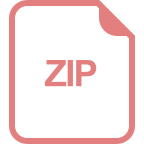
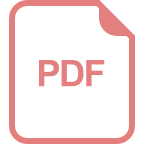
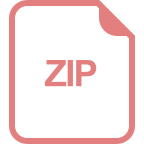
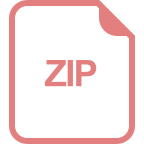
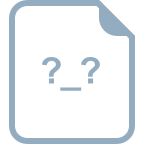
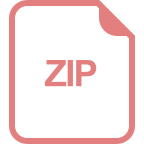
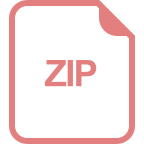
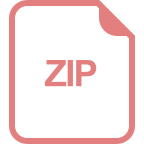
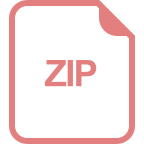
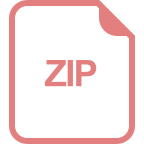
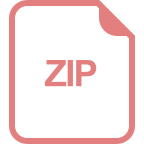
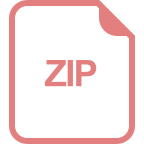
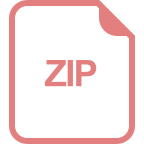
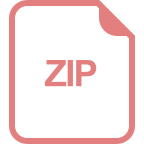
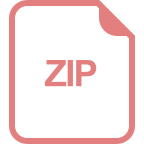
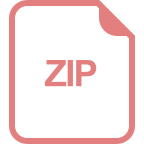
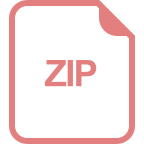
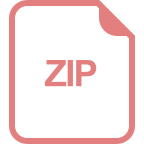
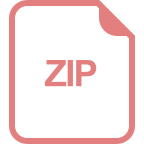
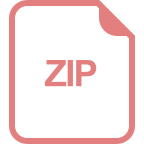
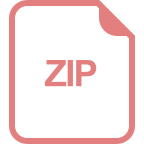
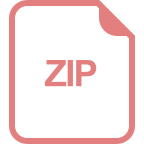
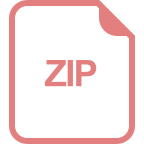
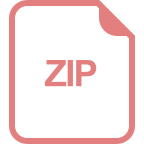
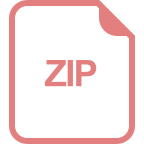
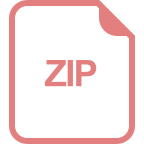
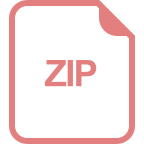
收起资源包目录


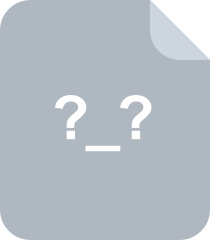
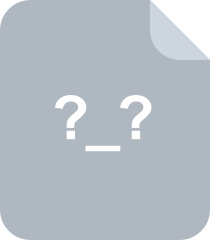

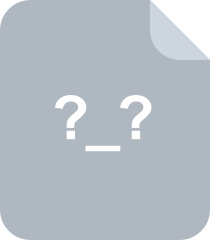
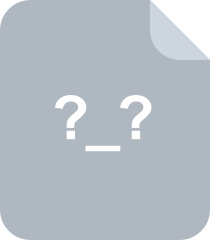

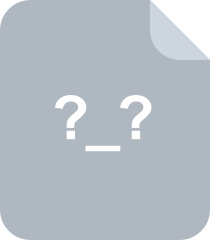
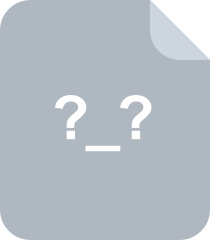


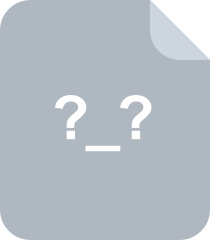



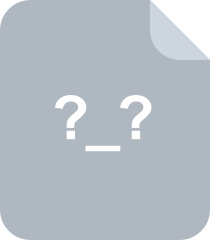
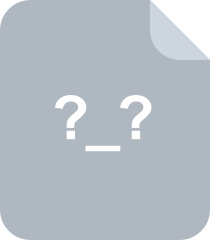
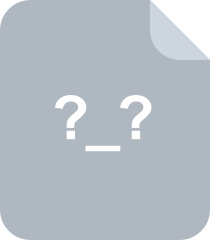
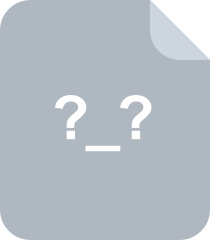



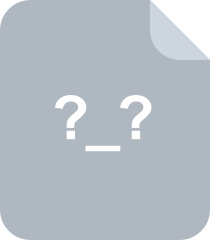
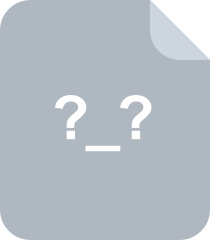


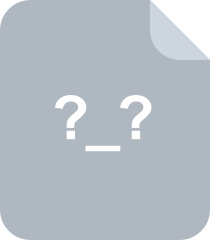
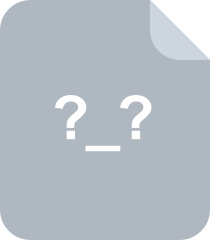


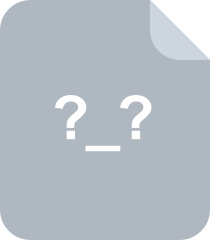
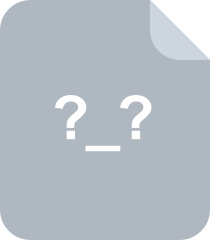
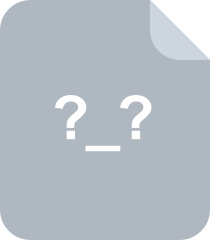
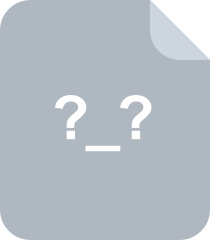
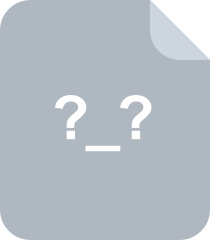
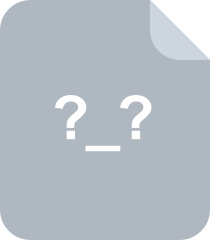
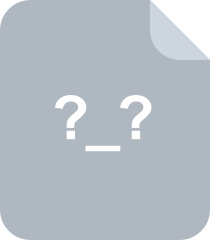

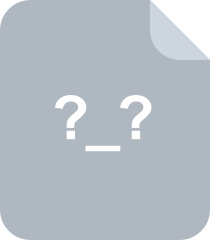
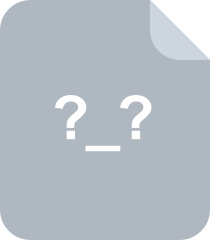
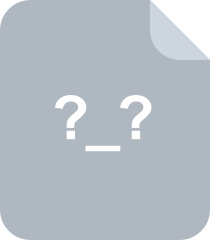


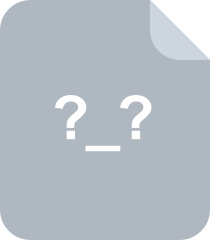
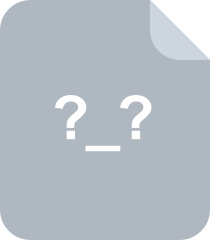
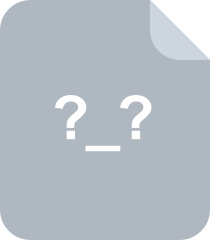
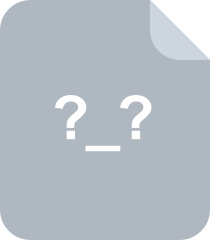
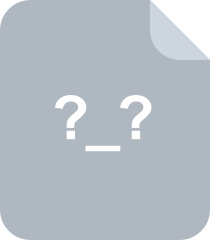

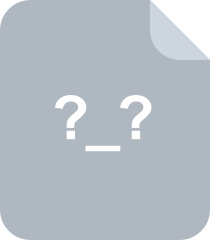
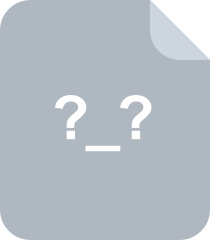
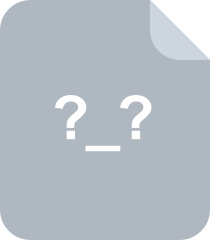
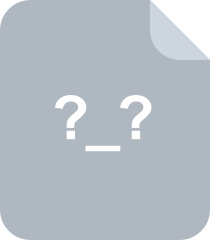
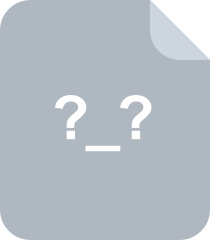

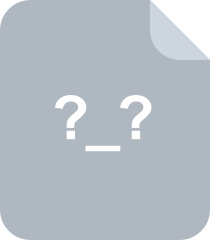
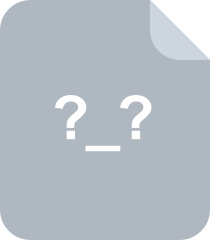
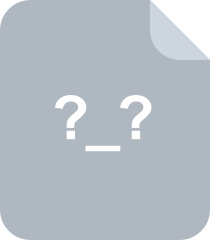
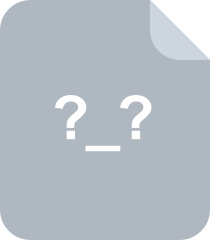
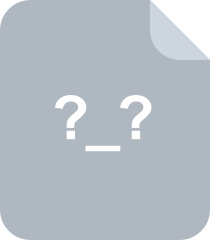
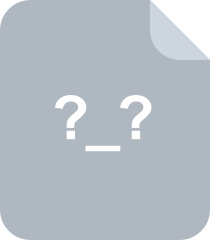
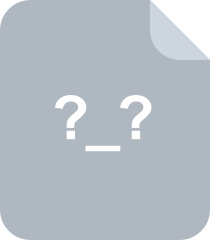
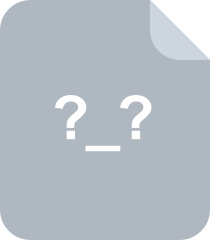
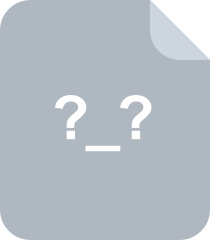
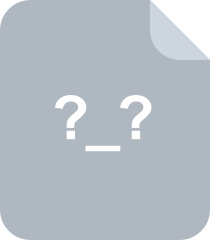
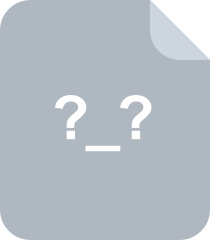


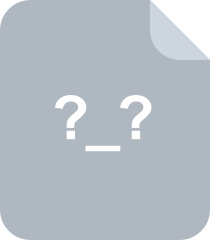
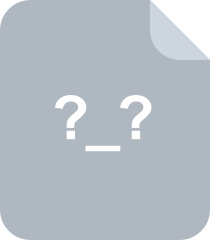

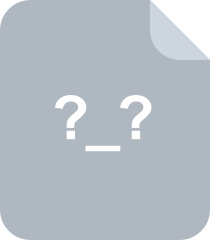



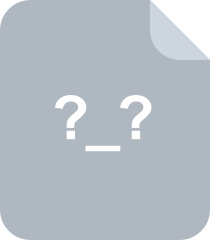
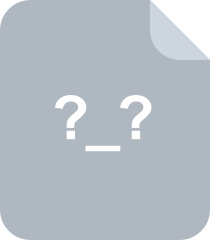
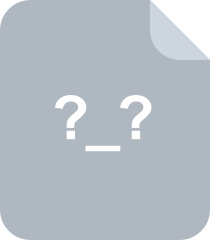

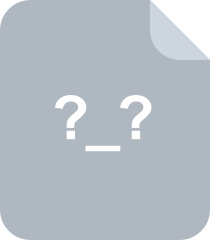



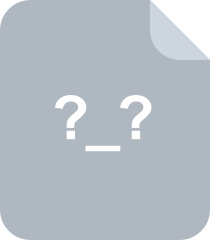
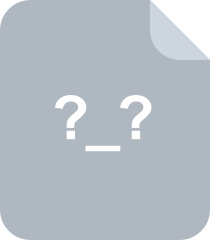

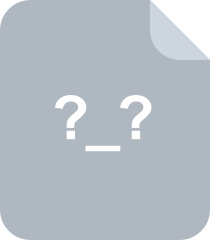
共 56 条
- 1
资源评论
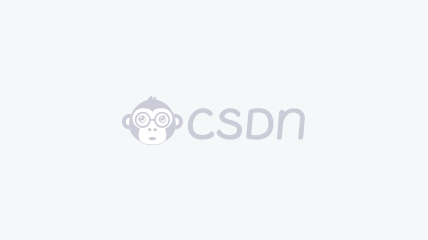


妄北y
- 粉丝: 2w+
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

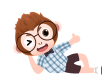
最新资源
- 毕设和企业适用springboot企业知识管理平台类及在线音乐平台源码+论文+视频.zip
- 毕设和企业适用springboot企业知识管理平台类及智慧社区管理平台源码+论文+视频.zip
- 毕设和企业适用springboot人才招聘类及企业资源规划平台源码+论文+视频.zip
- 毕设和企业适用springboot人才招聘类及社区服务平台源码+论文+视频.zip
- 毕设和企业适用springboot人才招聘类及食品配送管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业资源规划类及AI数据标注平台源码+论文+视频.zip
- 毕设和企业适用springboot企业资源规划类及车载智能管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业资源规划类及大数据实时处理系统源码+论文+视频.zip
- 毕设和企业适用springboot人才招聘类及视频内容管理平台源码+论文+视频.zip
- 毕设和企业适用springboot汽车管理类及网络营销平台源码+论文+视频.zip
- 毕设和企业适用springboot汽车管理类及物流信息平台源码+论文+视频.zip
- 毕设和企业适用springboot汽车管理类及销售管理平台源码+论文+视频.zip
- 毕设和企业适用springboot汽车管理类及物流追踪系统源码+论文+视频.zip
- 毕设和企业适用springboot汽车管理类及消费品管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业资源规划类及个性化广告平台源码+论文+视频.zip
- 毕设和企业适用springboot企业资源规划类及教育评价系统源码+论文+视频.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


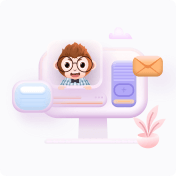
安全验证
文档复制为VIP权益,开通VIP直接复制
