const Article = require('../models/article.model')
const {
handleSuccess,
handleError
} = require('../utils/handle')
const authIsVerified = require('../utils/auth')
const request = require('request')
const config = require('../config/index')
class ArticleController {
// 获取文章列表
static async getArts (ctx) {
const {
current_page = 1,
page_size = 10,
keyword = '',
state = 1,
publish = 1,
tag,
type,
date,
hot } = ctx.query
// 过滤条件
const options = {
sort: { create_at: -1 },
page: Number(current_page),
limit: Number(page_size),
populate: ['tag'],
select: '-content'
}
// 参数
const querys = {}
// 关键词查询
if (keyword) {
const keywordReg = new RegExp(keyword)
querys['$or'] = [
{ 'title': keywordReg },
{ 'content': keywordReg },
{ 'description': keywordReg }
]
}
// 按照state查询
if (['1', '2'].includes(state)) {
querys.state = state
}
// 按照公开程度查询
if (['1', '2'].includes(publish)) {
querys.publish = publish
}
// 按照类型程度查询
if (['1', '2', '3'].includes(type)) {
querys.type = type
}
// 按热度排行
if (hot) {
options.sort = {
'meta.views': -1,
'meta.likes': -1,
'meta.comments': -1
}
}
// 时间查询
if (date) {
const getDate = new Date(date)
if(!Object.is(getDate.toString(), 'Invalid Date')) {
querys.create_at = {
"$gte": new Date((getDate / 1000 - 60 * 60 * 8) * 1000),
"$lt": new Date((getDate / 1000 + 60 * 60 * 16) * 1000)
}
}
}
if (tag) querys.tag = tag
// 如果是前台请求,则重置公开状态和发布状态
if (!authIsVerified(ctx.request)) {
querys.state = 1
querys.publish = 1
}
// 查询
const result = await Article
.paginate(querys, options)
.catch(err => ctx.throw(500, '服务器内部错误'))
if (result) {
handleSuccess({
ctx,
result: {
pagination: {
total: result.total,
current_page: result.page,
total_page: result.pages,
page_size: result.limit
},
list: result.docs
},
message: '列表数据获取成功!'
})
} else handleError({ ctx, message: '获取列表数据失败'})
}
// 添加文章
static async postArt (ctx) {
const res = Article.createArticle(ctx.request.body)
if (res) {
handleSuccess({ ctx, message: '添加文章成功' })
// 百度 seo push
// request.post({
// url: `http://data.zz.baidu.com/urls?site=${config.BAIDU.site}&token=${config.BAIDU.token}`,
// headers: { 'Content-Type': 'text/plain' },
// body: `${config.INFO.site}/article/${res._id}`
// }, (error, response, body) => {
// console.log('推送结果:', body)
// })
} else handleError({ ctx, message: '添加文章失败' })
}
// 根据文章id 获取内容
static async getArt (ctx) {
const _id = ctx.params.id
if (!_id) {
handleError({ ctx, message: '无效参数' })
return false
}
const res = await Article
.findById(_id)
.populate('tag')
.catch(err => ctx.throw(500, '服务器内部错误'))
if (res) {
// 每次请求,views 都增加一次
res.meta.views += 1
res.save()
handleSuccess({ ctx, message: '文章获取成功', result: res })
} else handleError({ ctx, message: '获取文章失败' })
}
// 删除文章
static async deleteArt (ctx) {
const _id = ctx.params.id
if (!_id) {
handleError({ ctx, message: '无效参数' })
return false
}
const res = await Article
.findByIdAndRemove(_id)
.catch(err => ctx.throw(500, '服务器内部错误'))
if (res) {
handleSuccess({ ctx, message: '删除文章成功' })
// 百度推送
request.post({
url: `http://data.zz.baidu.com/del?site=${config.BAIDU.site}&token=${config.BAIDU.token}`,
headers: { 'Content-Type': 'text/plain' },
body: `${config.INFO.site}/article/${_id}`
}, (error, response, body) => {
console.log('百度删除结果:', body);
})
} else handleError({ ctx, message: '删除文章失败' })
}
// 修改文章
static async putArt (ctx) {
const _id = ctx.params.id
const { title, keyword, tag } = ctx.request.body
delete ctx.request.body.create_at
delete ctx.request.body.update_at
delete ctx.request.body.meta
if (!_id) {
handleError({ ctx, message: '无效参数' })
return false
}
if(!title || !keyword) {
handleError({ ctx, message: 'title, keyword必填' })
return false
}
const res = await Article
.findByIdAndUpdate(_id, ctx.request.body)
.catch(err => ctx.throw(500, '服务器内部错误'))
if (res) {
handleSuccess({ ctx, message: '更新文章成功' })
// 百度推送
// request.post({
// url: `http://data.zz.baidu.com/update?site=${config.BAIDU.site}&token=${config.BAIDU.token}`,
// headers: { 'Content-Type': 'text/plain' },
// body: `${config.INFO.site}/article/${_id}`
// }, (error, response, body) => {
// console.log('百度删除结果:', body);
// })
} else handleError({ ctx, message: '更新文章失败' })
}
// 修改文章状态
static async patchArt (ctx) {
const _id = ctx.params.id
const { state, publish } = ctx.request.body
const querys = {}
if (state) querys.state = state
if (publish) querys.publish = publish
if (!_id) {
handleError({ ctx, message: '无效参数'})
return false
}
const res = await Article
.findByIdAndUpdate(_id, querys)
.catch(err => ctx.throw(500, '服务器内部错误'))
if (res) handleSuccess({ ctx, message: '更新文章状态成功'})
else handleError({ ctx, message: '更新文章状态失败' })
}
// 文章归档
static async getAllArts (ctx) {
const current_page = 1
const page_size = 10000
// 过滤条件
const options = {
sort: { create_at: -1 },
page: Number(current_page),
limit: Number(page_size),
populate: ['tag'],
select: '-content'
}
// 参数
const querys = {
state: 1,
publish: 1
}
// 查询
const article = await Article.aggregate([
{ $match: { state: 1, publish: 1 } },
{
$project: {
year: { $year: '$create_at' },
month: { $month: '$create_at' },
title: 1,
create_at: 1


妄北y
- 粉丝: 2w+
- 资源: 1万+
最新资源
- 储能变流器三相并网电压矢量控制控制(双向充放电) 0.0~0.7s:储能向电网供电50kW 0.7 ~1.2s:电网向电池充电50kW 0.7秒电池充电切放电,电网380AC,母线电压800V,电池
- 高频注入仿真pmsm 无感控制 解决0速转矩输出问题 插入式永磁同步电机,凸极,高频注入 MATLAB simulink仿真,供研究学习
- 机械设计饺子机sw18可编辑非常好的设计图纸100%好用.zip
- 氢电混合储能系统仿真(光伏,锂电池,燃料电池) 储能共直流母线 光伏储能共交流母线 储能由氢燃料电池锂电池组成 直流母线电压稳定在800v 考虑光伏故障下系统的运行特性
- 机械设计立式超声波焊接设备sw17非常好的设计图纸100%好用.zip
- 磁链观测器(仿真+闭环代码+参考文档) 1.仿真采用simulink搭建,2018b版本 2.代码采用Keil软件编译,思路参考vesc中使用的方法,自己编写的代码能够实现0速闭环启动,并且标注有大量
- 纯Java常用方法工具类
- Ceph一些介绍和使用说明.zip
- 电气仿真 分布式电源接入对配电网的影响 分布式电源是双馈感应发电机(DFIG)实现了变速变桨控制风力涡轮机模型 (lunwen5)参考lunwen可提供
- 机械设计精密电子自动除尘生产线sw17可编辑非常好的设计图纸100%好用.zip
- 机械设计快走丝电火花线切割机床(毕设ug8+cad+说明书)非常好的设计图纸100%好用.zip
- 激光熔覆仿真comsol通过激光进行熔覆工艺进行仿真,对温度与应力进行研究 采用COMSOL中的固体传热等物理场进行耦合仿真 对激光熔覆工艺完成后的温度分布与应力分布以云图形式输出,并研究某一点温度与
- CSS3.0博客学习配套完整参考手册 v4.2.4
- 全新版本码支付个人免签支付系统源码
- 西门子变频器 SINAMICS STARTER V5.6 HF2 软件 STARTER V56 STARTERV56HF2-cd-2.zip.003
- 纯java安全线程池工具类
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


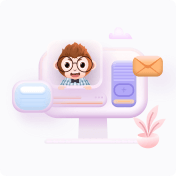