<?php
/**
* Smarty Internal Plugin Templateparser
*
* This is the template parser.
* It is generated from the internal.templateparser.y file
* @package Smarty
* @subpackage Compiler
* @author Uwe Tews
*/
class TP_yyToken implements ArrayAccess
{
public $string = '';
public $metadata = array();
function __construct($s, $m = array())
{
if ($s instanceof TP_yyToken) {
$this->string = $s->string;
$this->metadata = $s->metadata;
} else {
$this->string = (string) $s;
if ($m instanceof TP_yyToken) {
$this->metadata = $m->metadata;
} elseif (is_array($m)) {
$this->metadata = $m;
}
}
}
function __toString()
{
return $this->_string;
}
function offsetExists($offset)
{
return isset($this->metadata[$offset]);
}
function offsetGet($offset)
{
return $this->metadata[$offset];
}
function offsetSet($offset, $value)
{
if ($offset === null) {
if (isset($value[0])) {
$x = ($value instanceof TP_yyToken) ?
$value->metadata : $value;
$this->metadata = array_merge($this->metadata, $x);
return;
}
$offset = count($this->metadata);
}
if ($value === null) {
return;
}
if ($value instanceof TP_yyToken) {
if ($value->metadata) {
$this->metadata[$offset] = $value->metadata;
}
} elseif ($value) {
$this->metadata[$offset] = $value;
}
}
function offsetUnset($offset)
{
unset($this->metadata[$offset]);
}
}
class TP_yyStackEntry
{
public $stateno; /* The state-number */
public $major; /* The major token value. This is the code
** number for the token at this stack level */
public $minor; /* The user-supplied minor token value. This
** is the value of the token */
};
#line 12 "smarty_internal_templateparser.y"
class Smarty_Internal_Templateparser#line 79 "smarty_internal_templateparser.php"
{
#line 14 "smarty_internal_templateparser.y"
const Err1 = "Security error: Call to private object member not allowed";
const Err2 = "Security error: Call to dynamic object member not allowed";
const Err3 = "PHP in template not allowed. Use SmartyBC to enable it";
// states whether the parse was successful or not
public $successful = true;
public $retvalue = 0;
private $lex;
private $internalError = false;
function __construct($lex, $compiler) {
$this->lex = $lex;
$this->compiler = $compiler;
$this->smarty = $this->compiler->smarty;
$this->template = $this->compiler->template;
$this->compiler->has_variable_string = false;
$this->compiler->prefix_code = array();
$this->prefix_number = 0;
$this->block_nesting_level = 0;
if ($this->security = isset($this->smarty->security_policy)) {
$this->php_handling = $this->smarty->security_policy->php_handling;
} else {
$this->php_handling = $this->smarty->php_handling;
}
$this->is_xml = false;
$this->asp_tags = (ini_get('asp_tags') != '0');
$this->current_buffer = $this->root_buffer = new _smarty_template_buffer($this);
}
public static function escape_start_tag($tag_text) {
$tag = preg_replace('/\A<\?(.*)\z/', '<<?php ?>?\1', $tag_text, -1 , $count); //Escape tag
return $tag;
}
public static function escape_end_tag($tag_text) {
return '?<?php ?>>';
}
public function compileVariable($variable) {
if (strpos($variable,'(') == 0) {
// not a variable variable
$var = trim($variable,'\'');
$this->compiler->tag_nocache=$this->compiler->tag_nocache|$this->template->getVariable($var, null, true, false)->nocache;
$this->template->properties['variables'][$var] = $this->compiler->tag_nocache|$this->compiler->nocache;
}
// return '(isset($_smarty_tpl->tpl_vars['. $variable .'])?$_smarty_tpl->tpl_vars['. $variable .']->value:$_smarty_tpl->getVariable('. $variable .')->value)';
return '$_smarty_tpl->tpl_vars['. $variable .']->value';
}
#line 131 "smarty_internal_templateparser.php"
const TP_VERT = 1;
const TP_COLON = 2;
const TP_COMMENT = 3;
const TP_PHPSTARTTAG = 4;
const TP_PHPENDTAG = 5;
const TP_ASPSTARTTAG = 6;
const TP_ASPENDTAG = 7;
const TP_FAKEPHPSTARTTAG = 8;
const TP_XMLTAG = 9;
const TP_OTHER = 10;
const TP_LINEBREAK = 11;
const TP_LITERALSTART = 12;
const TP_LITERALEND = 13;
const TP_LITERAL = 14;
const TP_LDEL = 15;
const TP_RDEL = 16;
const TP_DOLLAR = 17;
const TP_ID = 18;
const TP_EQUAL = 19;
const TP_PTR = 20;
const TP_LDELIF = 21;
const TP_LDELFOR = 22;
const TP_SEMICOLON = 23;
const TP_INCDEC = 24;
const TP_TO = 25;
const TP_STEP = 26;
const TP_LDELFOREACH = 27;
const TP_SPACE = 28;
const TP_AS = 29;
const TP_APTR = 30;
const TP_LDELSETFILTER = 31;
const TP_SMARTYBLOCKCHILD = 32;
const TP_LDELSLASH = 33;
const TP_INTEGER = 34;
const TP_COMMA = 35;
const TP_OPENP = 36;
const TP_CLOSEP = 37;
const TP_MATH = 38;
const TP_UNIMATH = 39;
const TP_ANDSYM = 40;
const TP_ISIN = 41;
const TP_ISDIVBY = 42;
const TP_ISNOTDIVBY = 43;
const TP_ISEVEN = 44;
const TP_ISNOTEVEN = 45;
const TP_ISEVENBY = 46;
const TP_ISNOTEVENBY = 47;
const TP_ISODD = 48;
const TP_ISNOTODD = 49;
const TP_ISODDBY = 50;
const TP_ISNOTODDBY = 51;
const TP_INSTANCEOF = 52;
const TP_QMARK = 53;
const TP_NOT = 54;
const TP_TYPECAST = 55;
const TP_HEX = 56;
const TP_DOT = 57;
const TP_SINGLEQUOTESTRING = 58;
const TP_DOUBLECOLON = 59;
const TP_AT = 60;
const TP_HATCH = 61;
const TP_OPENB = 62;
const TP_CLOSEB = 63;
const TP_EQUALS = 64;
const TP_NOTEQUALS = 65;
const TP_GREATERTHAN = 66;
const TP_LESSTHAN
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
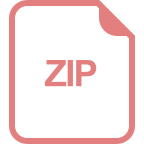
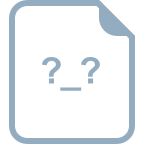
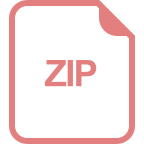
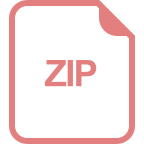
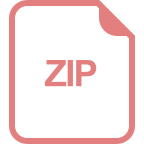
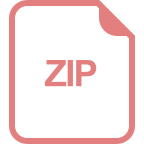
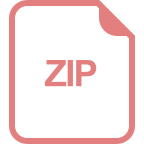
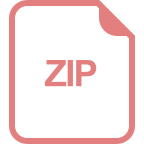
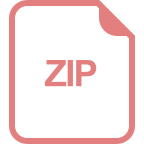
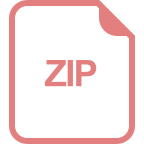
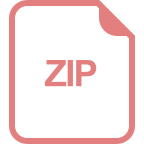
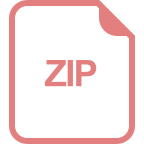
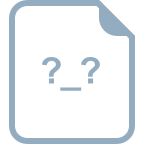
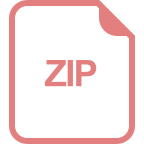
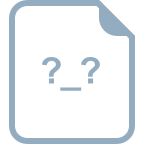
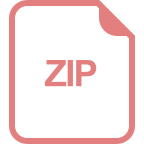
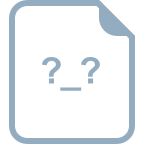
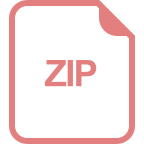
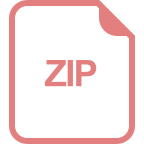
收起资源包目录

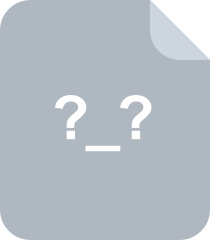
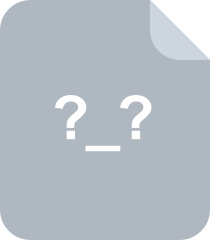
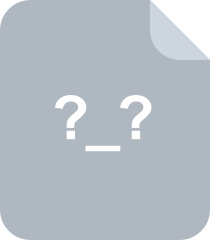
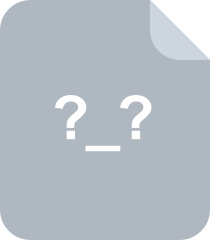
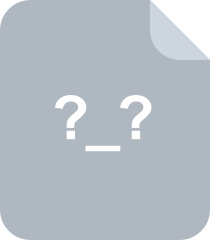
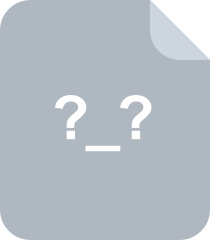
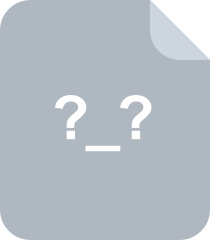
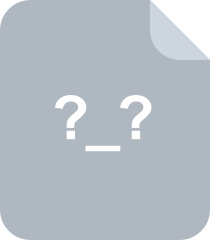
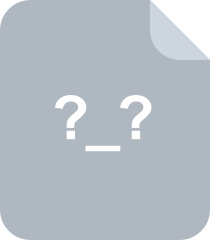
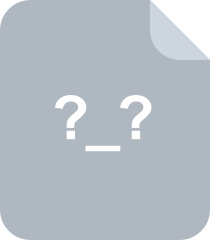
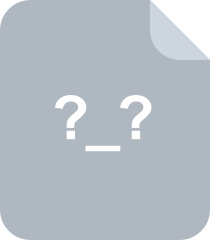
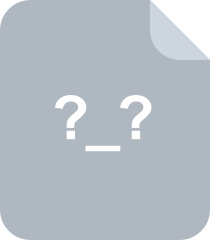
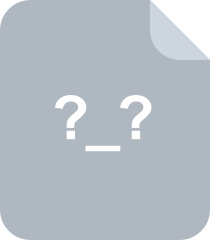
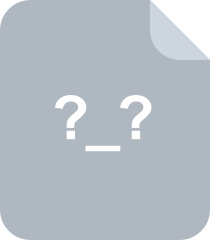
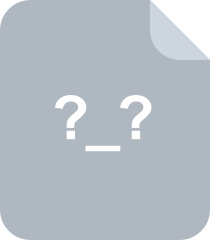
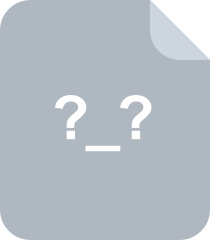
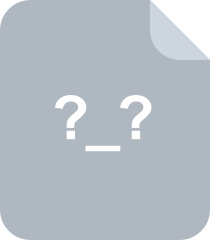
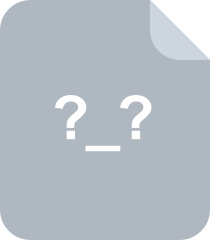
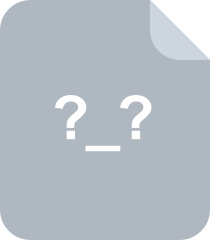
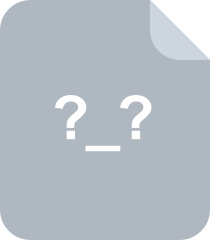
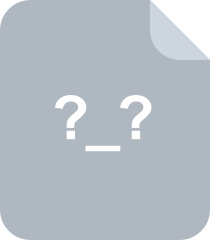
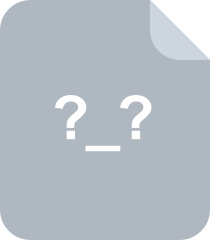
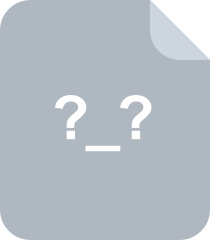
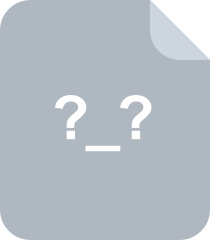
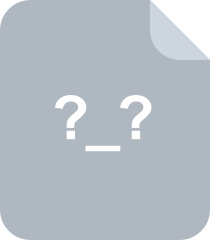
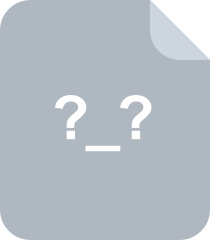
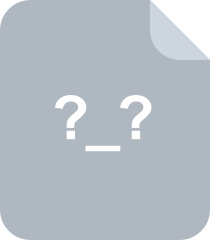
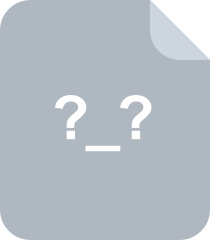
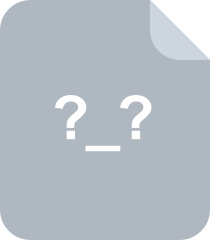
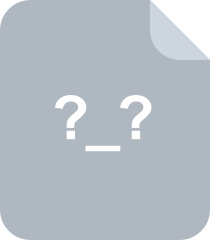
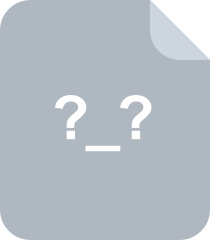
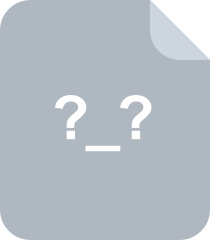
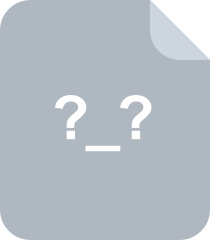
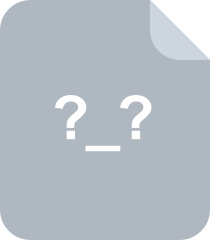
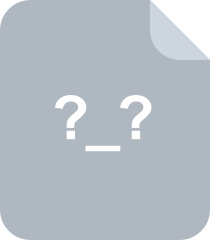
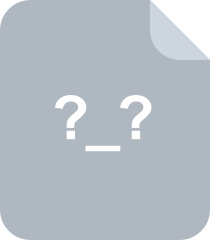
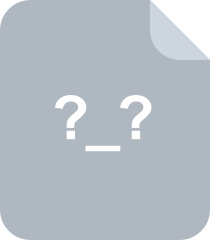
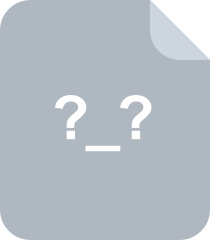
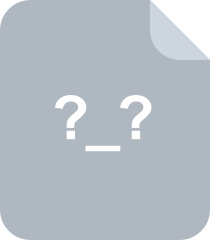
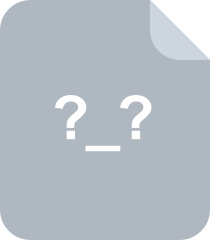
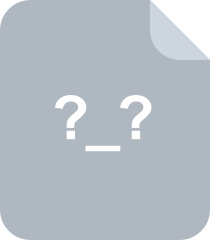
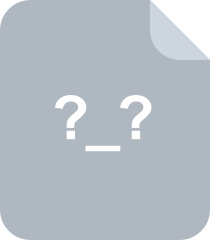
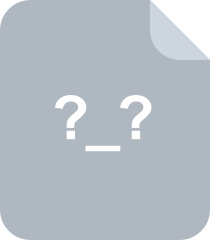
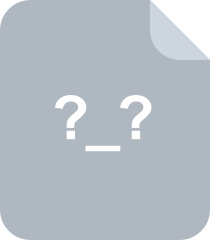
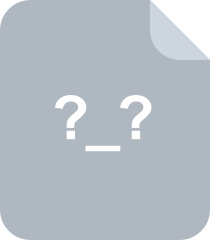
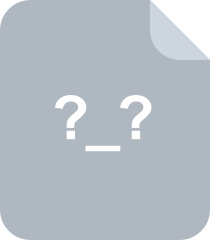
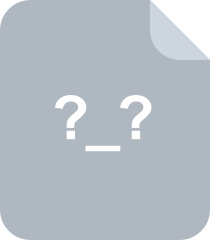
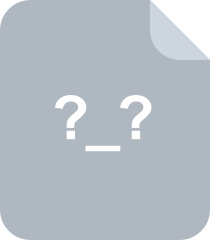
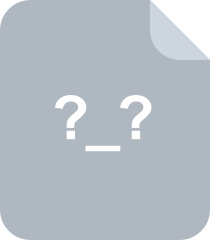
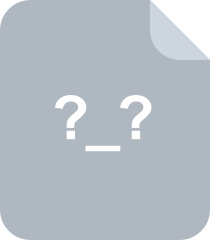
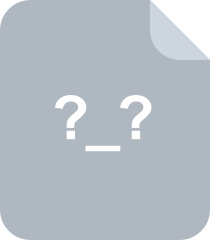
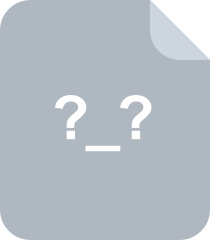
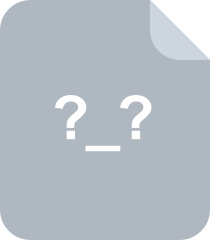
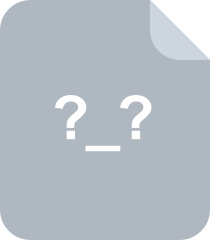
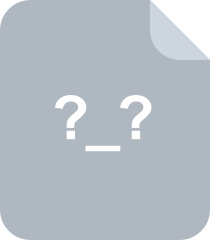
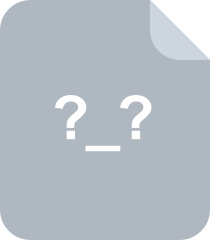
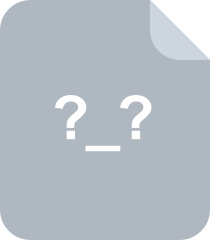
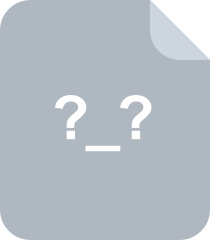
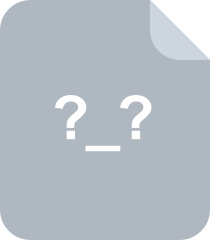
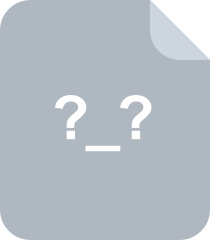
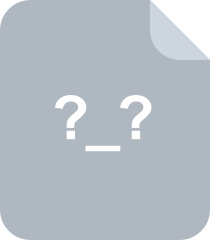
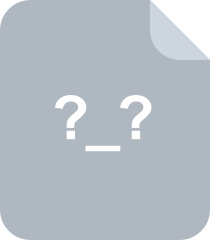
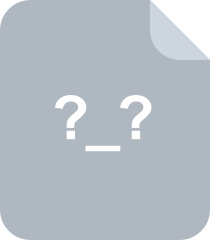
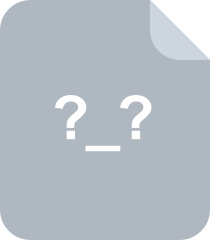
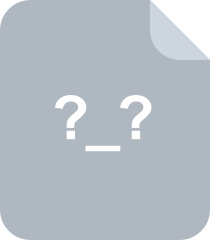
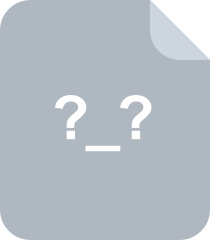
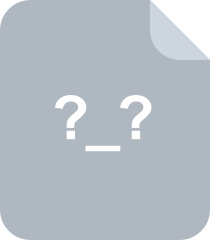
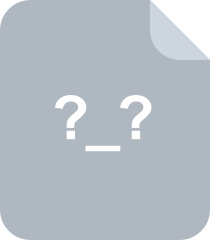
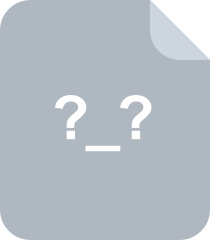
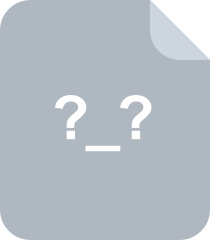
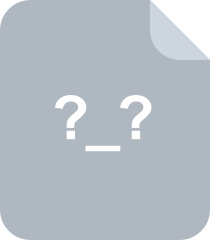
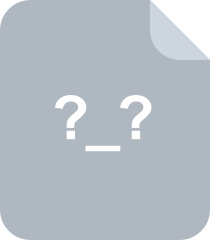
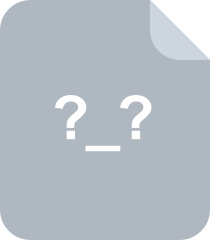
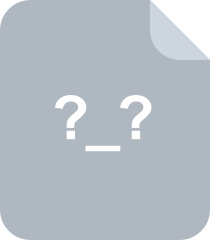
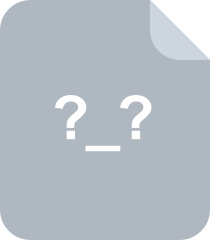
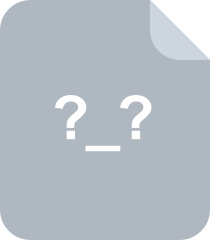
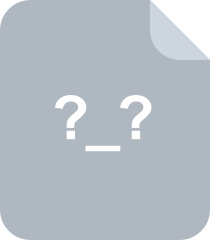
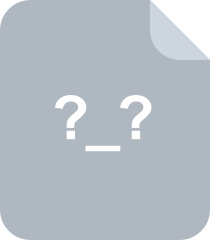
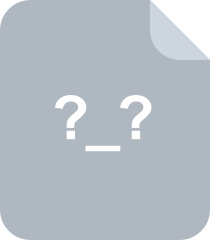
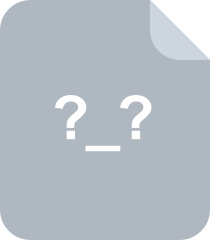
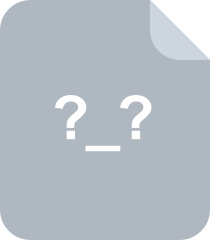
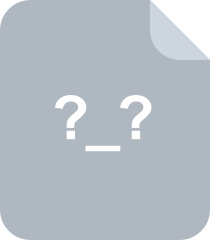
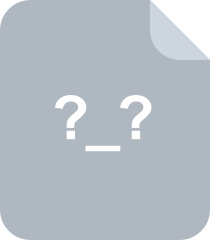
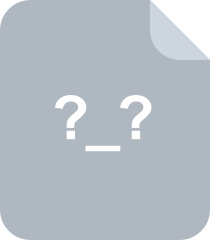
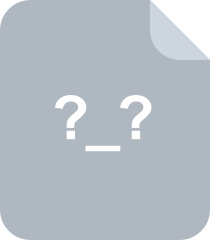
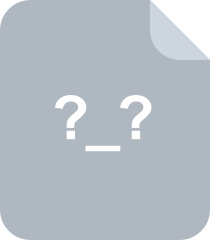
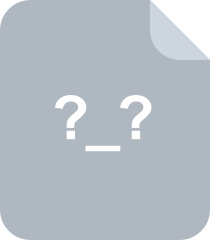
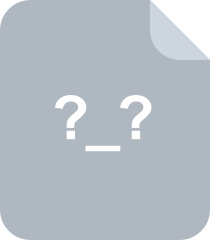
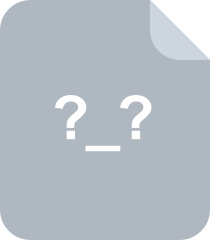
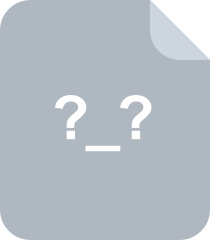
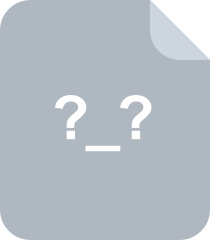
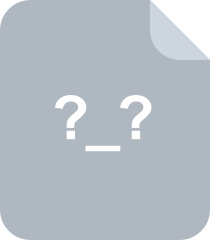
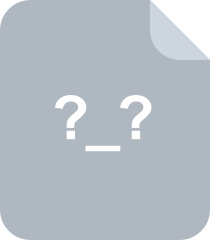
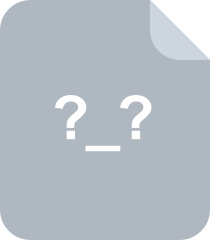
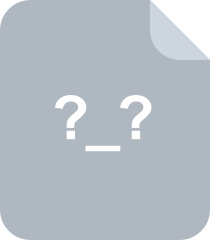
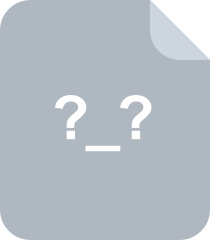
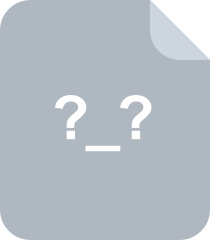
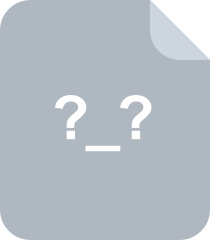
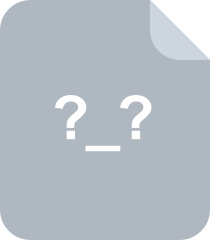
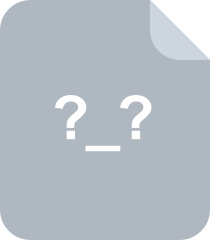
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
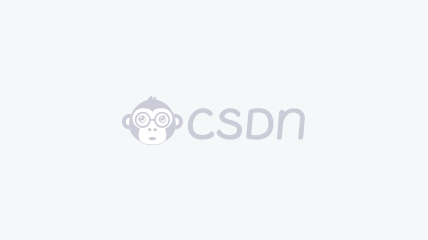
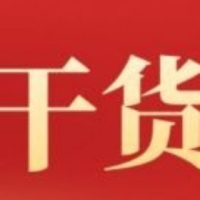
毕业_设计
- 粉丝: 1992
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

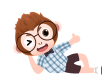
最新资源
- 【全年行事历】团建活动计划表.xlsx
- 【全年行事历】团建行程安排表-xx山.xlsx
- 【全年行事历】团建活动策划方案.docx
- 【全年行事历】团建开销费用分析.xlsx
- 【全年行事历】团建活动物料清单.xlsx
- 【全年行事历】团建文化衫尺码统计表.xlsx
- 【全年行事历】团建医药箱常备药清单.docx
- 【全年行事历】小型公司活动全年活动行事历.xlsx
- 【全年行事历】员工野外拓展活动方案.docx
- 四足机器人机械结构设计PDF
- 06-公司团建活动申请表.docx
- 03-团建活动策划方案.docx
- 07-团建活动采购预算清单.xlsx
- 08-团建日程计划表.xlsx
- 09-财务公司月度团建支出表.xlsx
- T-SQL查询高级SQLServer索引中的碎片和填充因子word文档doc格式最新版本
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


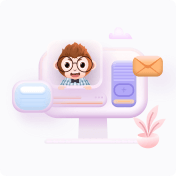
安全验证
文档复制为VIP权益,开通VIP直接复制
