<?php
/*
* This file is part of the Symfony package.
*
* (c) Fabien Potencier <fabien@symfony.com>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Symfony\Component\Mime;
use Symfony\Component\Mime\Exception\LogicException;
/**
* Manages MIME types and file extensions.
*
* For MIME type guessing, you can register custom guessers
* by calling the registerGuesser() method.
* Custom guessers are always called before any default ones:
*
* $guesser = new MimeTypes();
* $guesser->registerGuesser(new MyCustomMimeTypeGuesser());
*
* If you want to change the order of the default guessers, just re-register your
* preferred one as a custom one. The last registered guesser is preferred over
* previously registered ones.
*
* Re-registering a built-in guesser also allows you to configure it:
*
* $guesser = new MimeTypes();
* $guesser->registerGuesser(new FileinfoMimeTypeGuesser('/path/to/magic/file'));
*
* @author Fabien Potencier <fabien@symfony.com>
*/
final class MimeTypes implements MimeTypesInterface
{
private $extensions = [];
private $mimeTypes = [];
/**
* @var MimeTypeGuesserInterface[]
*/
private $guessers = [];
private static $default;
public function __construct(array $map = [])
{
foreach ($map as $mimeType => $extensions) {
$this->extensions[$mimeType] = $extensions;
foreach ($extensions as $extension) {
$this->mimeTypes[$extension][] = $mimeType;
}
}
$this->registerGuesser(new FileBinaryMimeTypeGuesser());
$this->registerGuesser(new FileinfoMimeTypeGuesser());
}
public static function setDefault(self $default)
{
self::$default = $default;
}
public static function getDefault(): self
{
return self::$default ?? self::$default = new self();
}
/**
* Registers a MIME type guesser.
*
* The last registered guesser has precedence over the other ones.
*/
public function registerGuesser(MimeTypeGuesserInterface $guesser)
{
array_unshift($this->guessers, $guesser);
}
/**
* {@inheritdoc}
*/
public function getExtensions(string $mimeType): array
{
if ($this->extensions) {
$extensions = $this->extensions[$mimeType] ?? $this->extensions[$lcMimeType = strtolower($mimeType)] ?? null;
}
return $extensions ?? self::$map[$mimeType] ?? self::$map[$lcMimeType ?? strtolower($mimeType)] ?? [];
}
/**
* {@inheritdoc}
*/
public function getMimeTypes(string $ext): array
{
if ($this->mimeTypes) {
$mimeTypes = $this->mimeTypes[$ext] ?? $this->mimeTypes[$lcExt = strtolower($ext)] ?? null;
}
return $mimeTypes ?? self::$reverseMap[$ext] ?? self::$reverseMap[$lcExt ?? strtolower($ext)] ?? [];
}
/**
* {@inheritdoc}
*/
public function isGuesserSupported(): bool
{
foreach ($this->guessers as $guesser) {
if ($guesser->isGuesserSupported()) {
return true;
}
}
return false;
}
/**
* {@inheritdoc}
*
* The file is passed to each registered MIME type guesser in reverse order
* of their registration (last registered is queried first). Once a guesser
* returns a value that is not null, this method terminates and returns the
* value.
*/
public function guessMimeType(string $path): ?string
{
foreach ($this->guessers as $guesser) {
if (!$guesser->isGuesserSupported()) {
continue;
}
if (null !== $mimeType = $guesser->guessMimeType($path)) {
return $mimeType;
}
}
if (!$this->isGuesserSupported()) {
throw new LogicException('Unable to guess the MIME type as no guessers are available (have you enabled the php_fileinfo extension?).');
}
return null;
}
/**
* A map of MIME types and their default extensions.
*
* Updated from upstream on 2019-01-16
*
* @see Resources/bin/update_mime_types.php
*/
private static $map = [
'application/acrobat' => ['pdf'],
'application/andrew-inset' => ['ez'],
'application/annodex' => ['anx'],
'application/applixware' => ['aw'],
'application/atom+xml' => ['atom'],
'application/atomcat+xml' => ['atomcat'],
'application/atomsvc+xml' => ['atomsvc'],
'application/ccxml+xml' => ['ccxml'],
'application/cdmi-capability' => ['cdmia'],
'application/cdmi-container' => ['cdmic'],
'application/cdmi-domain' => ['cdmid'],
'application/cdmi-object' => ['cdmio'],
'application/cdmi-queue' => ['cdmiq'],
'application/cdr' => ['cdr'],
'application/coreldraw' => ['cdr'],
'application/csv' => ['csv'],
'application/cu-seeme' => ['cu'],
'application/davmount+xml' => ['davmount'],
'application/dbase' => ['dbf'],
'application/dbf' => ['dbf'],
'application/dicom' => ['dcm'],
'application/docbook+xml' => ['dbk', 'docbook'],
'application/dssc+der' => ['dssc'],
'application/dssc+xml' => ['xdssc'],
'application/ecmascript' => ['ecma', 'es'],
'application/emf' => ['emf'],
'application/emma+xml' => ['emma'],
'application/epub+zip' => ['epub'],
'application/exi' => ['exi'],
'application/font-tdpfr' => ['pfr'],
'application/font-woff' => ['woff'],
'application/futuresplash' => ['swf', 'spl'],
'application/geo+json' => ['geojson', 'geo.json'],
'application/gml+xml' => ['gml'],
'application/gnunet-directory' => ['gnd'],
'application/gpx' => ['gpx'],
'application/gpx+xml' => ['gpx'],
'application/gxf' => ['gxf'],
'application/gzip' => ['gz'],
'application/hyperstudio' => ['stk'],
'application/ico' => ['ico'],
'application/ics' => ['vcs', 'ics'],
'application/illustrator' => ['ai'],
'application/inkml+xml' => ['ink', 'inkml'],
'application/ipfix' => ['ipfix'],
'application/java' => ['class'],
'application/java-archive' => ['jar'],
'application/java-byte-code' => ['class'],
'application/java-serialized-object' => ['ser'],
'application/java-vm' => ['class'],
'application/javascript' => ['js', 'jsm', 'mjs'],
'application/jrd+json' => ['jrd'],
'application/json' => ['json'],
'application/json-patch+json' => ['json-patch'],
'application/jsonml+json' => ['jsonml'],
'application/ld+json' => ['jsonld'],
'application/lost+xml' => ['lostxml'],
'application/lotus123' => ['123', 'wk1', 'wk3', 'wk4', 'wks'],
'application/m3u' => ['m3u', 'm3u8', 'vlc'],
'application/mac-binhex40' => ['hqx'],
'application/mac-compactpro' => ['cpt'],
'application/mads+xml' => ['mads'],
'application/marc' => ['mrc'],
'application/marcxml+xml' => ['mrcx'],
'application/mathematica' => ['ma', 'nb', 'mb'],
'application/mathml+xml' => ['mathml', 'mml'],
'application/mbox' => ['mbox'],
'application/mdb' => ['mdb'],
'application/mediaservercontrol+xml' => ['mscml'],
'application/metalink+xml' => ['metalink'],
'application/metalink4+xml' => ['meta4'],
'application/mets+xml' => ['mets'],
'application/mods+xml' => ['mods'],
'application/mp21' => ['m21', 'mp21'],
'application/mp4' => ['mp4s'],
'application/ms-tnef' => ['tnef', 'tnf'],
'application/msaccess' => ['mdb'],
'application/msexcel' => ['xls', 'xlc', 'xll', 'xlm', 'xlw', 'xla', 'xlt', 'xld'],
'a
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
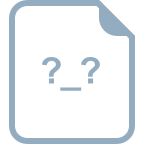
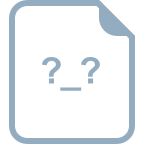
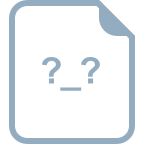
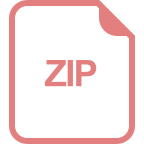
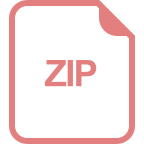
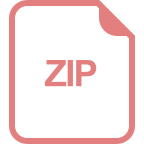
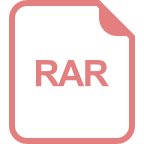
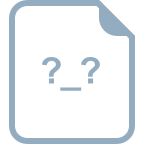
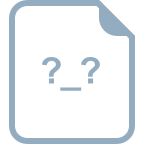
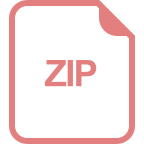
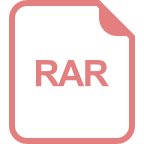
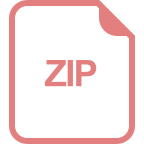
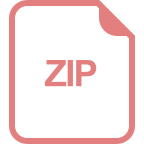
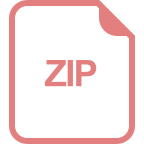
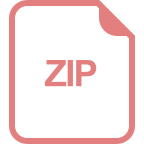
收起资源包目录

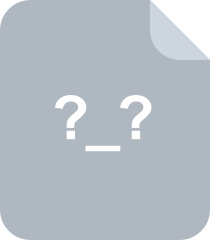
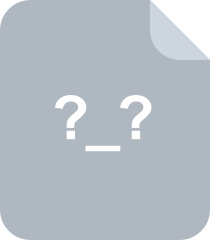
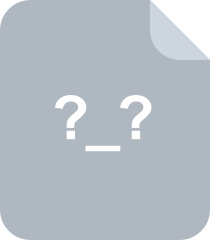
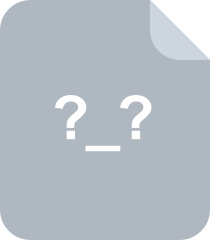
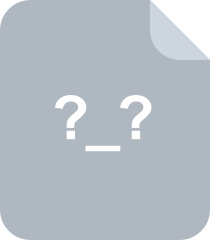
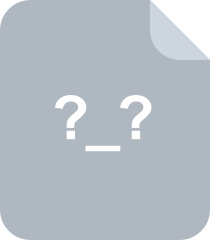
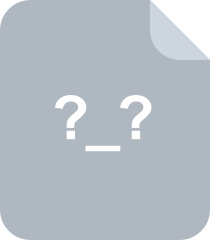
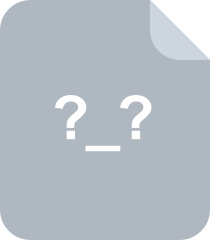
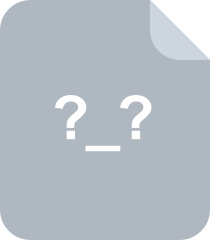
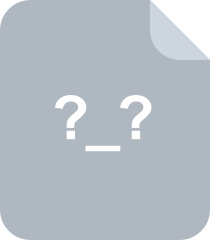
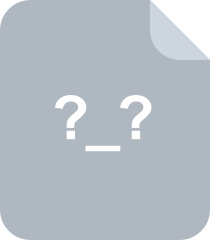
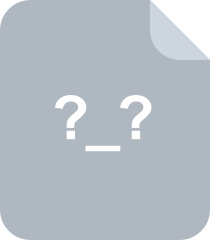
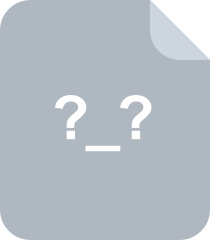
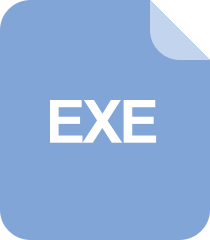
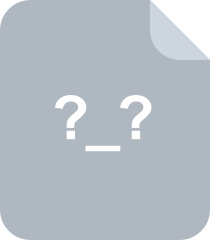
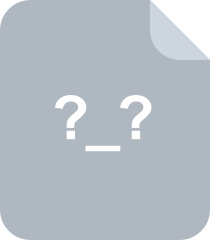
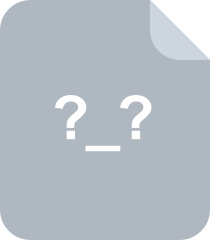
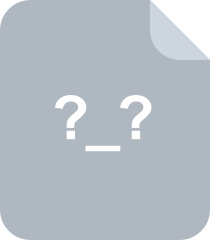
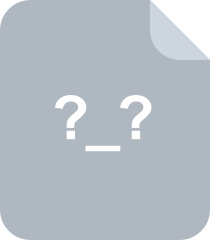
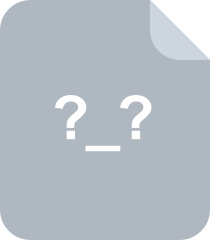
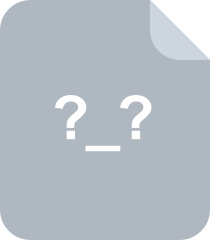
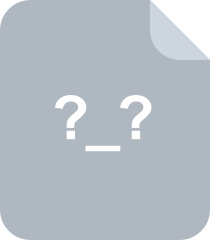
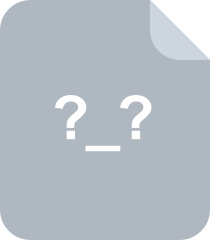
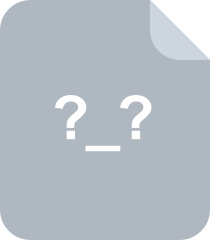
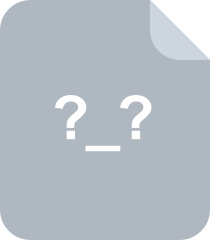
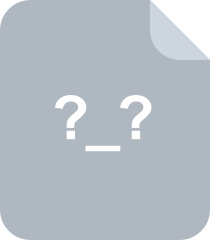
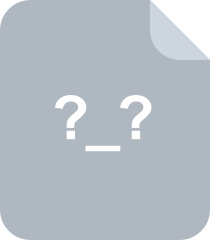
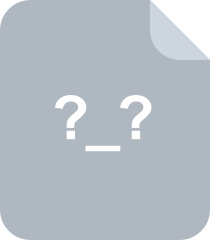
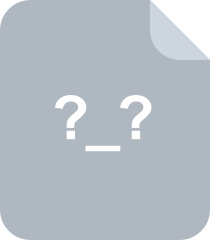
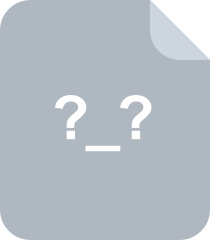
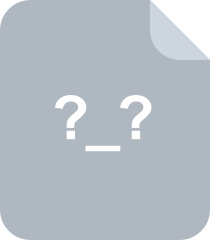
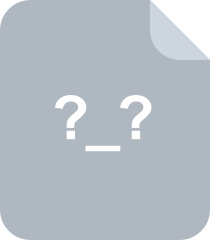
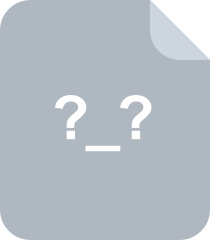
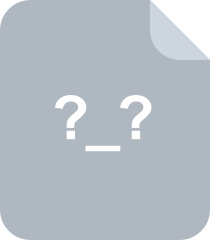
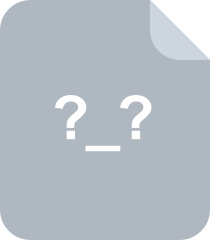
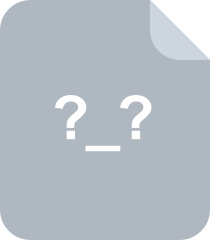
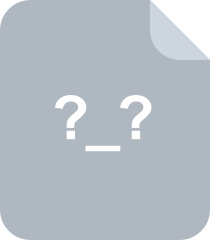
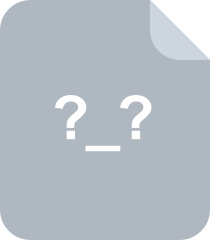
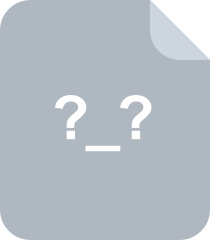
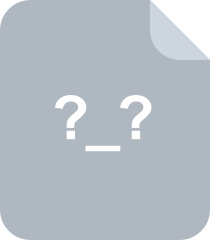
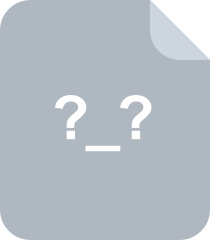
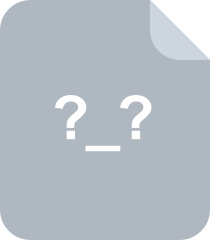
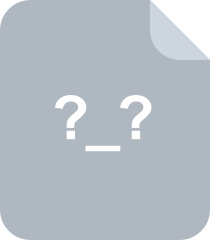
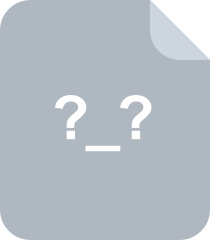
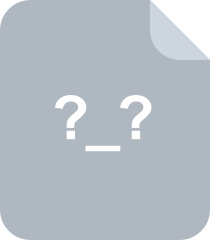
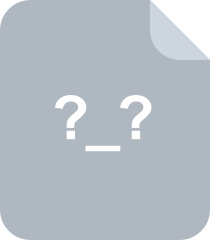
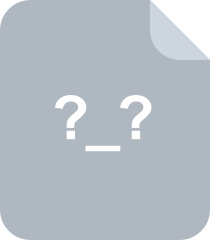
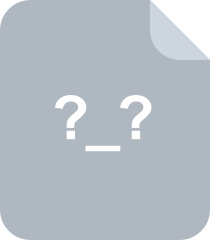
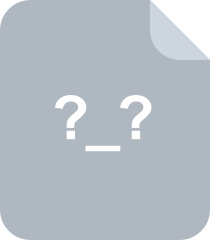
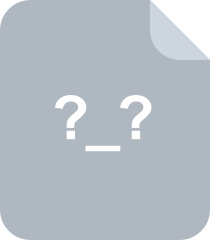
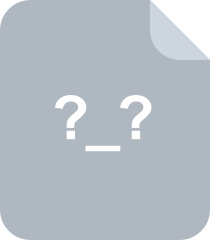
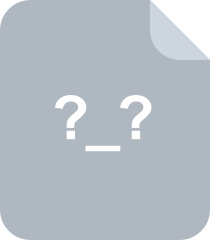
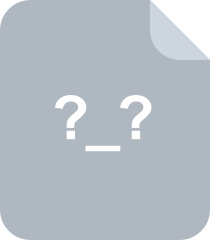
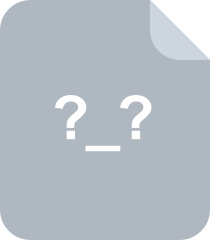
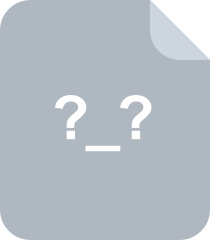
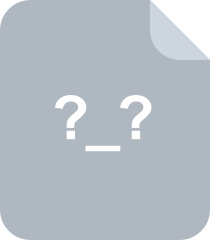
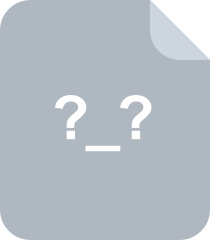
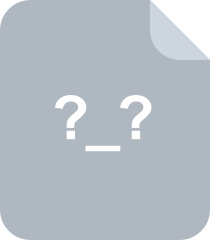
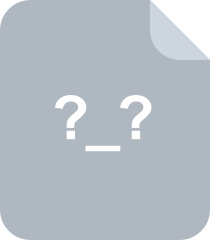
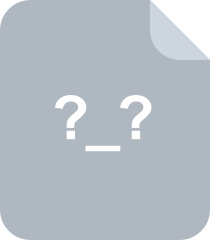
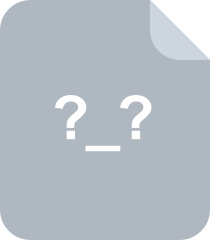
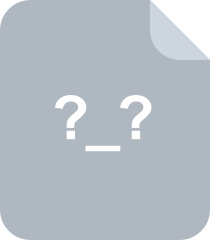
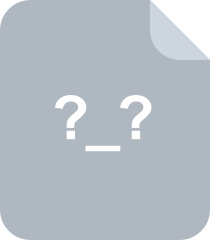
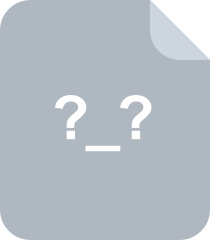
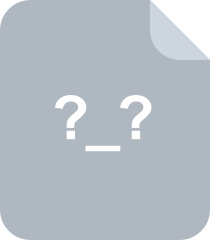
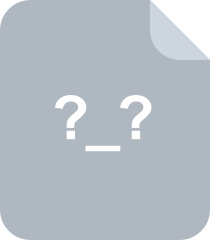
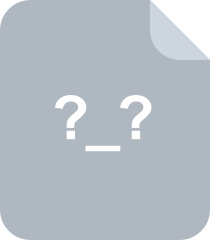
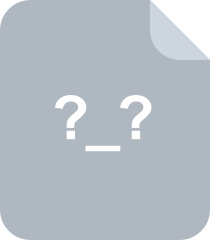
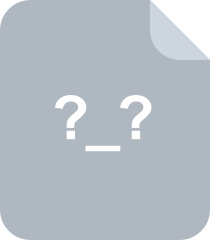
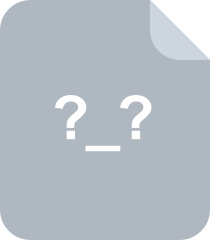
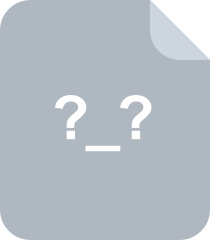
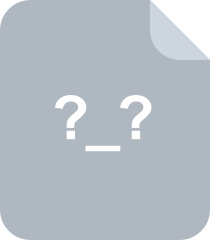
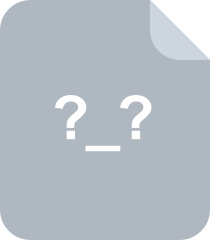
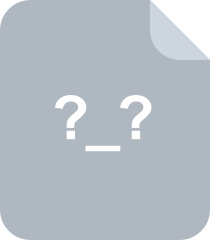
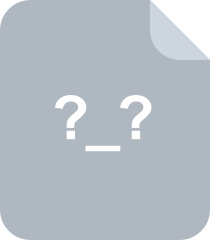
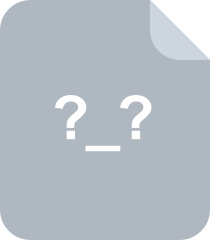
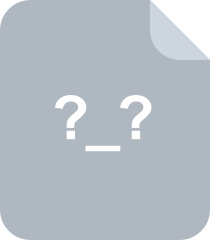
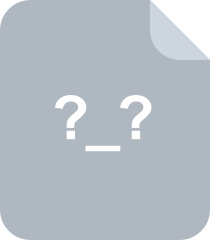
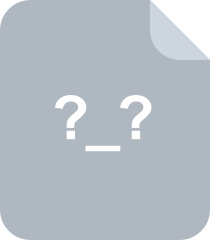
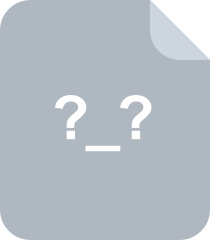
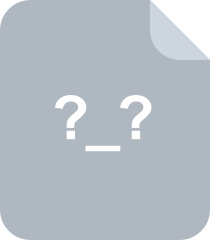
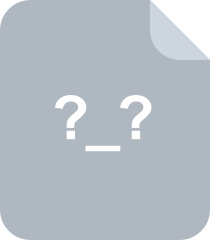
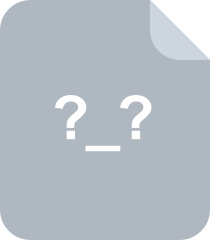
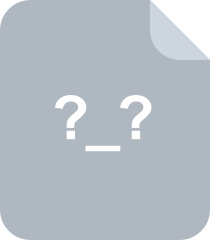
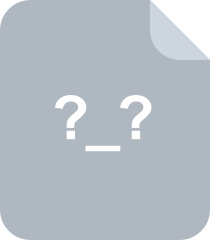
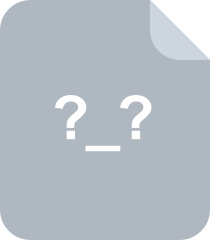
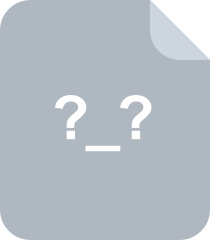
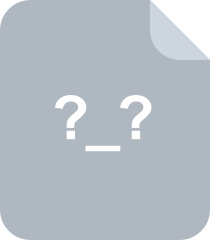
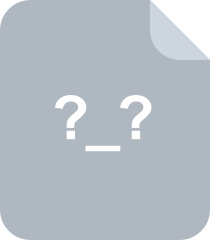
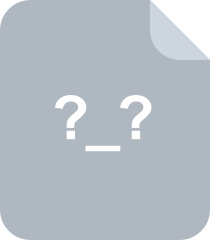
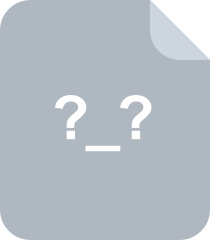
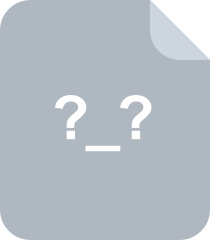
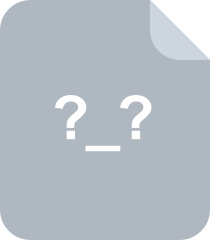
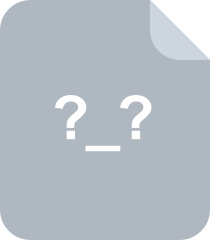
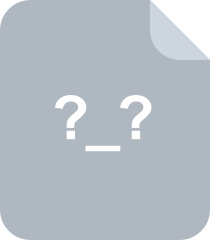
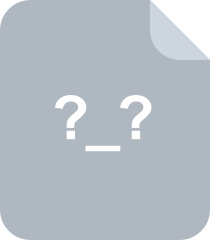
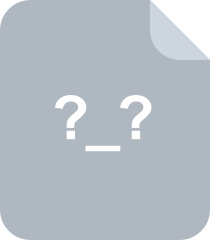
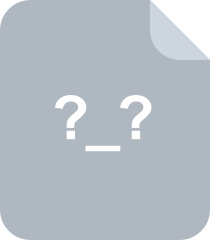
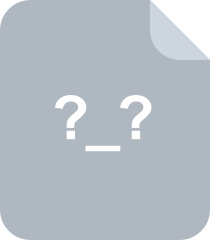
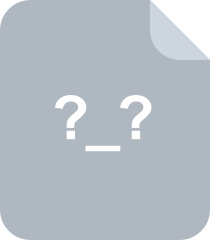
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
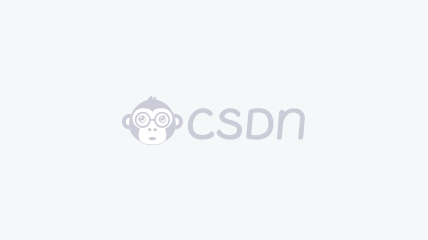
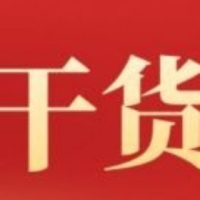
毕业_设计
- 粉丝: 1991
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

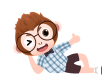
最新资源
- 基于Python爬虫的中国疫情数据分析与可视化毕设项目.zip
- 开源自己绘制的V851S核心板和底板
- 微信群成员重复对比.py
- Linux、File System、Linux基本常用命令
- miniconda的python2.7的环境安装包
- unity物体的旋转,缩放与拖拽
- Python开发的IP归属地批量查询工具
- Kotlin编程语言入门详解及核心知识点
- PeakVue山顶风景独好-毕业设计课程专属资源-JDK1.8
- 香蕉、包子、焦炭、水果沙拉、手、托盘、水瓶检测29-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


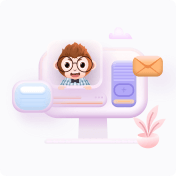
安全验证
文档复制为VIP权益,开通VIP直接复制
