<?php
/**
* @link http://www.yiiframework.com/
* @copyright Copyright (c) 2008 Yii Software LLC
* @license http://www.yiiframework.com/license/
*/
namespace yii\helpers;
use Yii;
use yii\base\InvalidArgumentException;
use yii\base\Model;
use yii\db\ActiveRecordInterface;
use yii\validators\StringValidator;
use yii\web\Request;
/**
* BaseHtml provides concrete implementation for [[Html]].
*
* Do not use BaseHtml. Use [[Html]] instead.
*
* @author Qiang Xue <qiang.xue@gmail.com>
* @since 2.0
*/
class BaseHtml
{
/**
* @var string Regular expression used for attribute name validation.
* @since 2.0.12
*/
public static $attributeRegex = '/(^|.*\])([\w\.\+]+)(\[.*|$)/u';
/**
* @var array list of void elements (element name => 1)
* @see http://www.w3.org/TR/html-markup/syntax.html#void-element
*/
public static $voidElements = [
'area' => 1,
'base' => 1,
'br' => 1,
'col' => 1,
'command' => 1,
'embed' => 1,
'hr' => 1,
'img' => 1,
'input' => 1,
'keygen' => 1,
'link' => 1,
'meta' => 1,
'param' => 1,
'source' => 1,
'track' => 1,
'wbr' => 1,
];
/**
* @var array the preferred order of attributes in a tag. This mainly affects the order of the attributes
* that are rendered by [[renderTagAttributes()]].
*/
public static $attributeOrder = [
'type',
'id',
'class',
'name',
'value',
'href',
'src',
'srcset',
'form',
'action',
'method',
'selected',
'checked',
'readonly',
'disabled',
'multiple',
'size',
'maxlength',
'width',
'height',
'rows',
'cols',
'alt',
'title',
'rel',
'media',
];
/**
* @var array list of tag attributes that should be specially handled when their values are of array type.
* In particular, if the value of the `data` attribute is `['name' => 'xyz', 'age' => 13]`, two attributes
* will be generated instead of one: `data-name="xyz" data-age="13"`.
* @since 2.0.3
*/
public static $dataAttributes = ['aria', 'data', 'data-ng', 'ng'];
/**
* Encodes special characters into HTML entities.
* The [[\yii\base\Application::charset|application charset]] will be used for encoding.
* @param string $content the content to be encoded
* @param bool $doubleEncode whether to encode HTML entities in `$content`. If false,
* HTML entities in `$content` will not be further encoded.
* @return string the encoded content
* @see decode()
* @see https://secure.php.net/manual/en/function.htmlspecialchars.php
*/
public static function encode($content, $doubleEncode = true)
{
return htmlspecialchars($content, ENT_QUOTES | ENT_SUBSTITUTE, Yii::$app ? Yii::$app->charset : 'UTF-8', $doubleEncode);
}
/**
* Decodes special HTML entities back to the corresponding characters.
* This is the opposite of [[encode()]].
* @param string $content the content to be decoded
* @return string the decoded content
* @see encode()
* @see https://secure.php.net/manual/en/function.htmlspecialchars-decode.php
*/
public static function decode($content)
{
return htmlspecialchars_decode($content, ENT_QUOTES);
}
/**
* Generates a complete HTML tag.
* @param string|bool|null $name the tag name. If $name is `null` or `false`, the corresponding content will be rendered without any tag.
* @param string $content the content to be enclosed between the start and end tags. It will not be HTML-encoded.
* If this is coming from end users, you should consider [[encode()]] it to prevent XSS attacks.
* @param array $options the HTML tag attributes (HTML options) in terms of name-value pairs.
* These will be rendered as the attributes of the resulting tag. The values will be HTML-encoded using [[encode()]].
* If a value is null, the corresponding attribute will not be rendered.
*
* For example when using `['class' => 'my-class', 'target' => '_blank', 'value' => null]` it will result in the
* html attributes rendered like this: `class="my-class" target="_blank"`.
*
* See [[renderTagAttributes()]] for details on how attributes are being rendered.
*
* @return string the generated HTML tag
* @see beginTag()
* @see endTag()
*/
public static function tag($name, $content = '', $options = [])
{
if ($name === null || $name === false) {
return $content;
}
$html = "<$name" . static::renderTagAttributes($options) . '>';
return isset(static::$voidElements[strtolower($name)]) ? $html : "$html$content</$name>";
}
/**
* Generates a start tag.
* @param string|bool|null $name the tag name. If $name is `null` or `false`, the corresponding content will be rendered without any tag.
* @param array $options the tag options in terms of name-value pairs. These will be rendered as
* the attributes of the resulting tag. The values will be HTML-encoded using [[encode()]].
* If a value is null, the corresponding attribute will not be rendered.
* See [[renderTagAttributes()]] for details on how attributes are being rendered.
* @return string the generated start tag
* @see endTag()
* @see tag()
*/
public static function beginTag($name, $options = [])
{
if ($name === null || $name === false) {
return '';
}
return "<$name" . static::renderTagAttributes($options) . '>';
}
/**
* Generates an end tag.
* @param string|bool|null $name the tag name. If $name is `null` or `false`, the corresponding content will be rendered without any tag.
* @return string the generated end tag
* @see beginTag()
* @see tag()
*/
public static function endTag($name)
{
if ($name === null || $name === false) {
return '';
}
return "</$name>";
}
/**
* Generates a style tag.
* @param string $content the style content
* @param array $options the tag options in terms of name-value pairs. These will be rendered as
* the attributes of the resulting tag. The values will be HTML-encoded using [[encode()]].
* If a value is null, the corresponding attribute will not be rendered.
* See [[renderTagAttributes()]] for details on how attributes are being rendered.
* @return string the generated style tag
*/
public static function style($content, $options = [])
{
return static::tag('style', $content, $options);
}
/**
* Generates a script tag.
* @param string $content the script content
* @param array $options the tag options in terms of name-value pairs. These will be rendered as
* the attributes of the resulting tag. The values will be HTML-encoded using [[encode()]].
* If a value is null, the corresponding attribute will not be rendered.
* See [[renderTagAttributes()]] for details on how attributes are being rendered.
* @return string the generated script tag
*/
public static function script($content, $options = [])
{
return static::tag('script', $content, $options);
}
/**
* Generates a link tag that refers to an external CSS file.
* @param array|string $url the URL of the external CSS file. This parameter will be processed by [[Url::to()]].
* @param array $options the tag options in terms of name-value pairs. The following options are specially handled:
*
* - condition: specifies the conditional comments for IE, e.g., `lt IE 9`. When this is specified,
* the generated `link` tag will be enclosed within the conditional comment
没有合适的资源?快使用搜索试试~ 我知道了~
PHP实例开发源码-Yii Framework php框架.zip
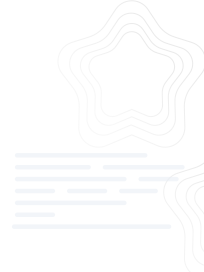
共2325个文件
php:996个
md:842个
png:261个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 153 浏览量
2022-11-21
21:36:56
上传
评论
收藏 19.03MB ZIP 举报
温馨提示
PHP实例开发源码—Yii Framework php框架.zip PHP实例开发源码—Yii Framework php框架.zip PHP实例开发源码—Yii Framework php框架.zip
资源推荐
资源详情
资源评论
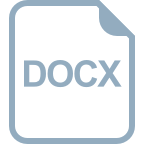
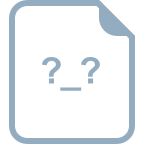
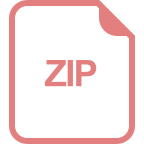
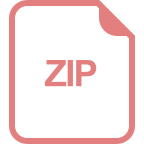
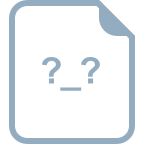
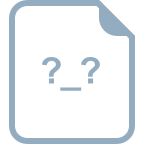
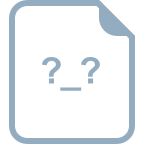
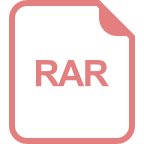
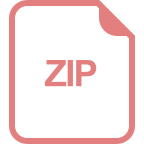
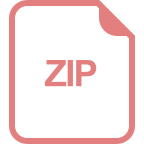
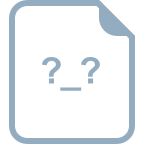
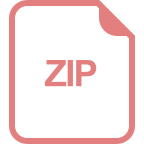
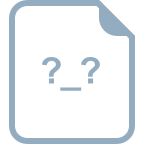
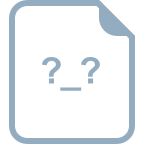
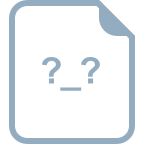
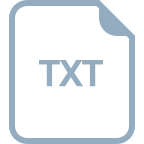
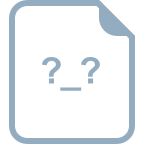
收起资源包目录

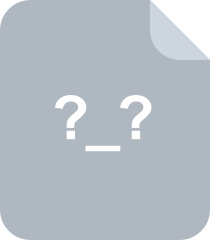
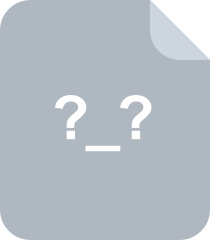
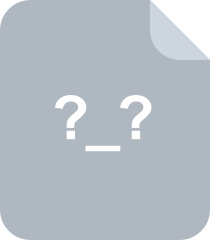
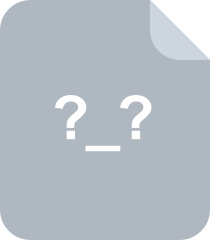
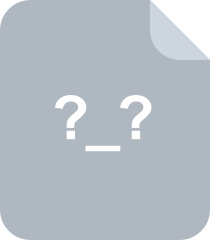
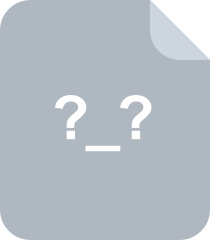
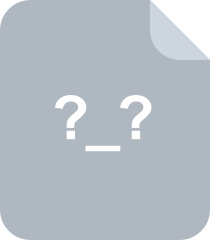
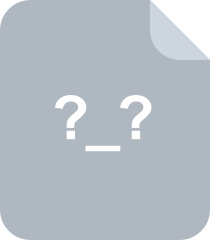
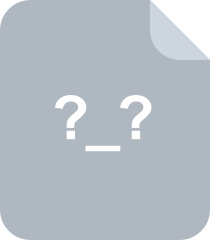
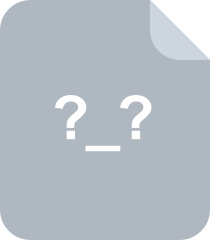
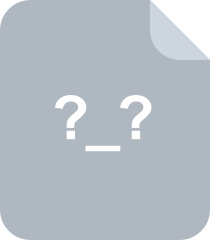
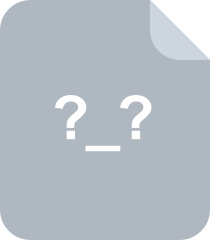
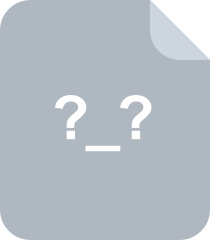
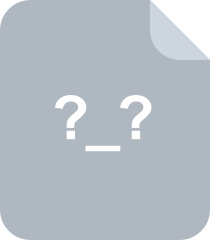
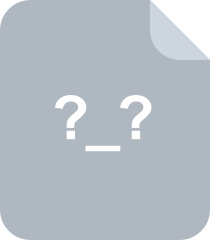
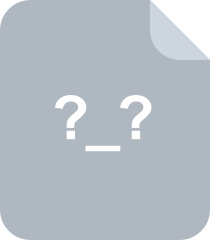
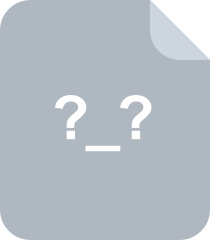
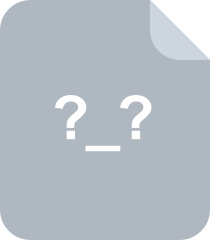
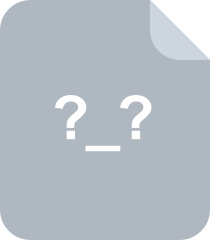
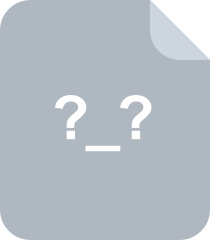
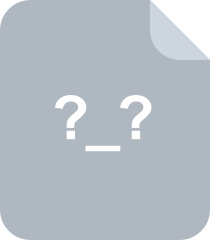
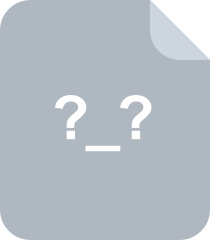
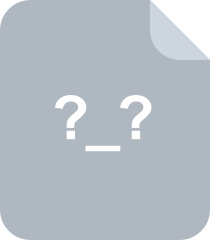
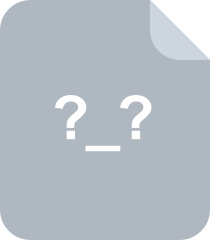
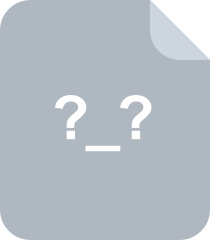
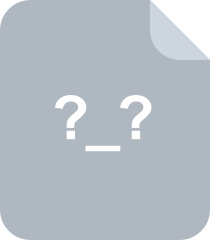
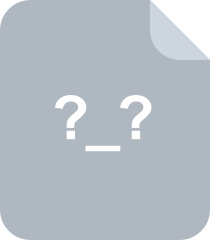
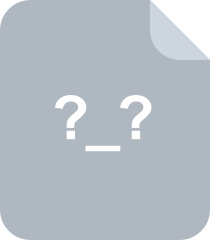
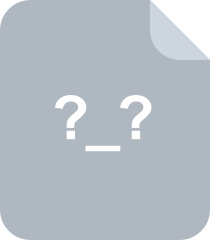
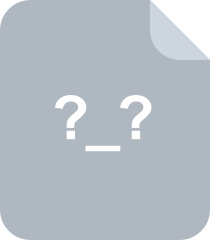
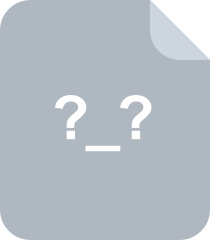
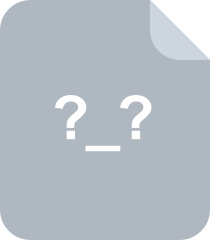
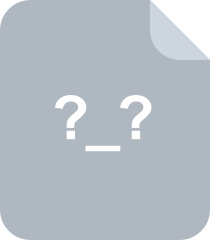
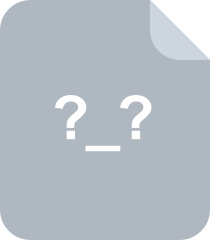
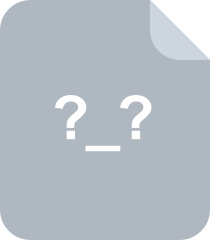
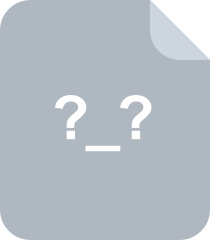
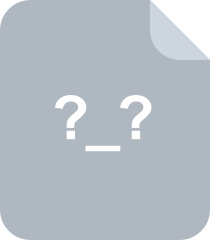
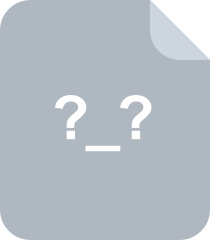
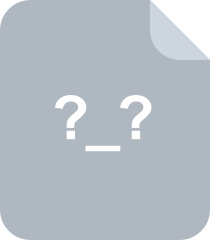
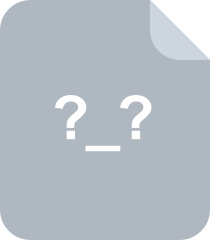
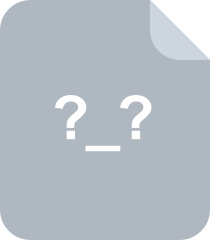
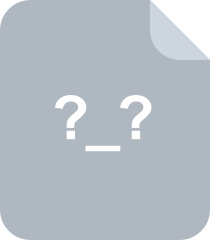
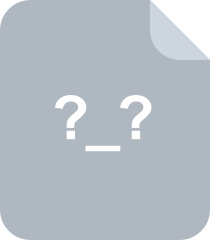
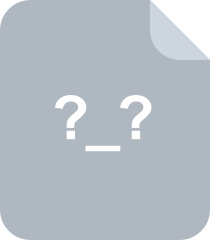
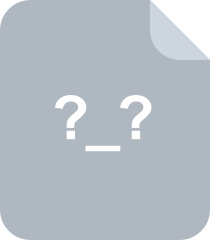
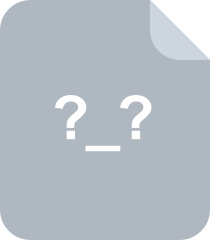
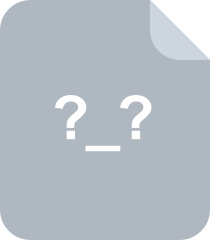
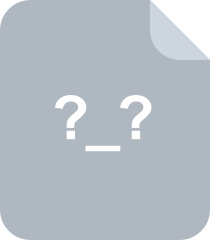
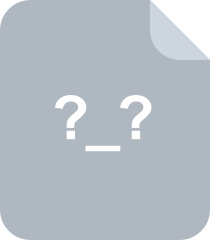
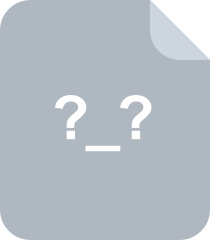
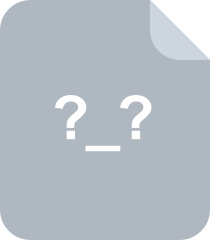
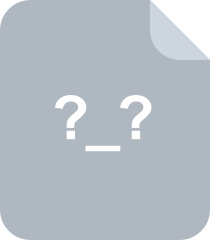
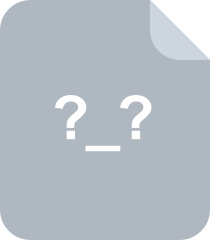
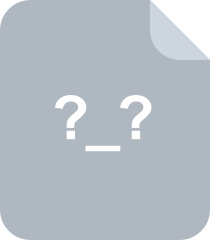
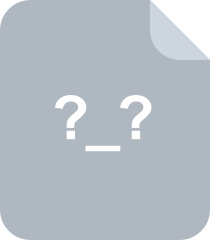
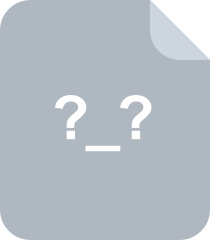
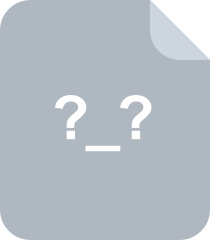
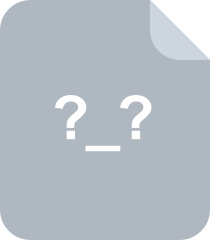
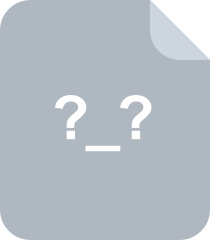
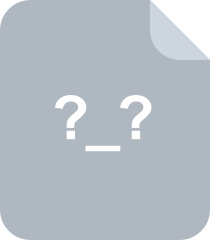
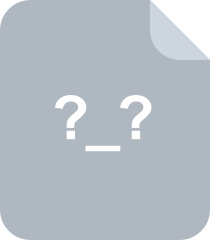
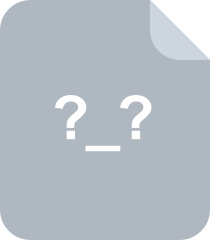
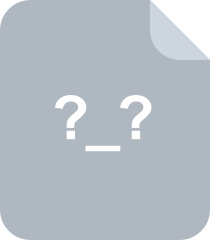
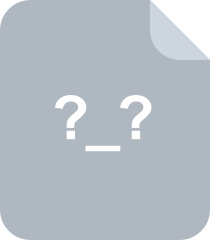
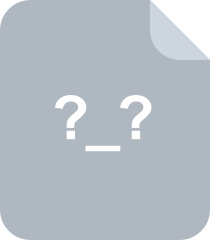
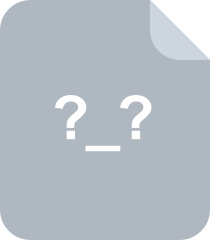
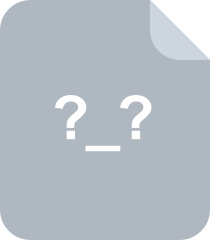
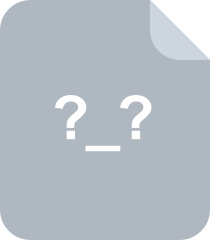
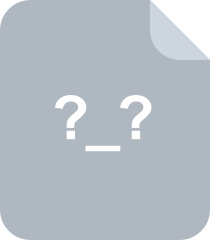
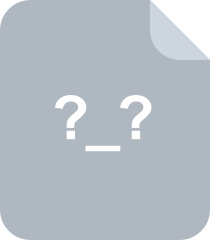
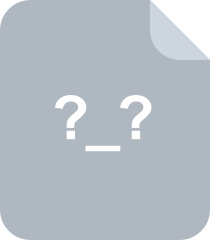
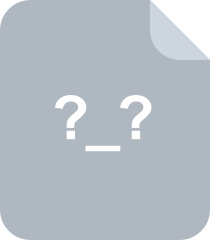
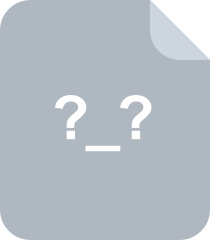
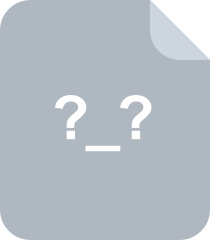
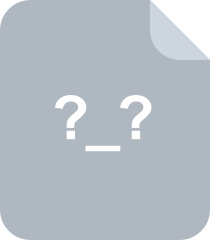
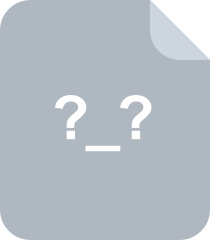
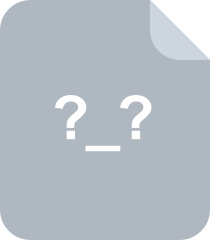
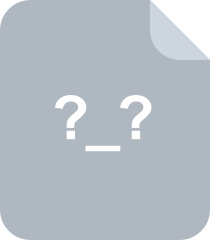
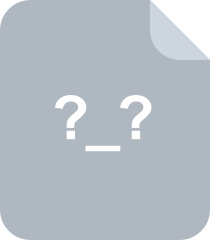
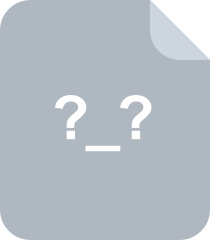
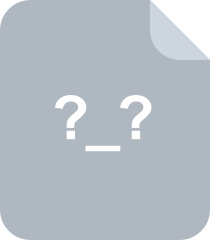
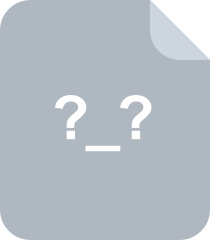
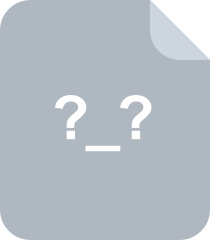
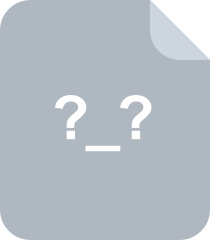
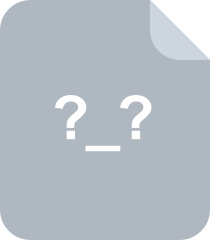
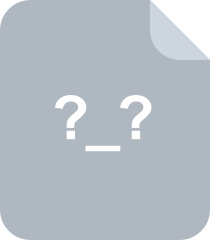
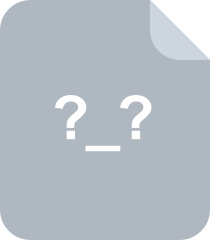
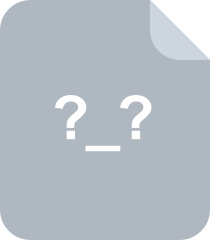
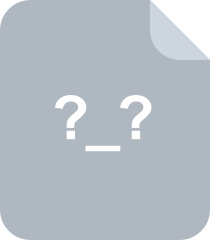
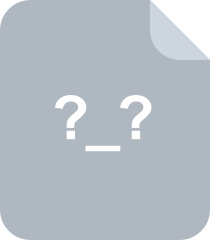
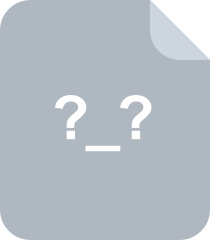
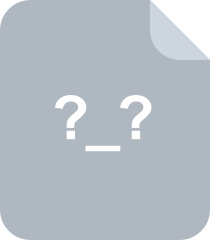
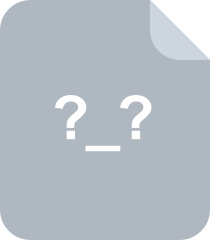
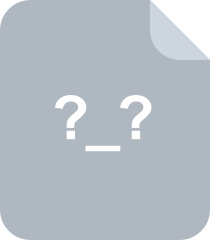
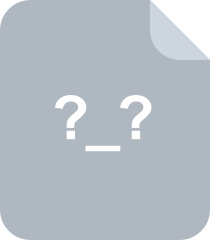
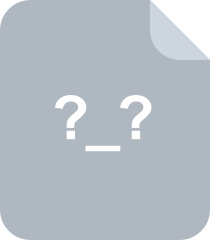
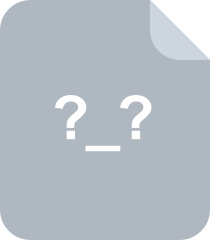
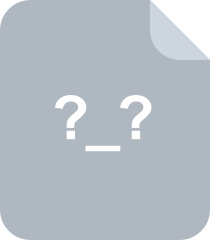
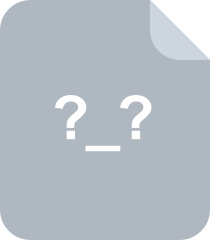
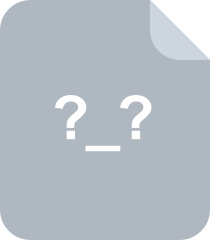
共 2325 条
- 1
- 2
- 3
- 4
- 5
- 6
- 24
资源评论
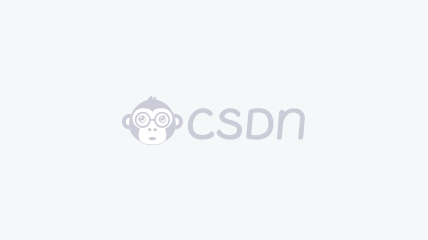
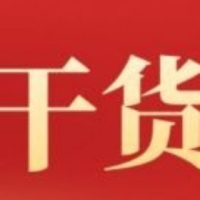
毕业_设计
- 粉丝: 1922
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

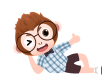
安全验证
文档复制为VIP权益,开通VIP直接复制
