<?php
/**
* Smarty Internal Plugin Templateparser
*
* This is the template parser.
* It is generated from the internal.templateparser.y file
* @package Smarty
* @subpackage Compiler
* @author Uwe Tews
*/
class TP_yyToken implements ArrayAccess
{
public $string = '';
public $metadata = array();
function __construct($s, $m = array())
{
if ($s instanceof TP_yyToken) {
$this->string = $s->string;
$this->metadata = $s->metadata;
} else {
$this->string = (string) $s;
if ($m instanceof TP_yyToken) {
$this->metadata = $m->metadata;
} elseif (is_array($m)) {
$this->metadata = $m;
}
}
}
function __toString()
{
return $this->_string;
}
function offsetExists($offset)
{
return isset($this->metadata[$offset]);
}
function offsetGet($offset)
{
return $this->metadata[$offset];
}
function offsetSet($offset, $value)
{
if ($offset === null) {
if (isset($value[0])) {
$x = ($value instanceof TP_yyToken) ?
$value->metadata : $value;
$this->metadata = array_merge($this->metadata, $x);
return;
}
$offset = count($this->metadata);
}
if ($value === null) {
return;
}
if ($value instanceof TP_yyToken) {
if ($value->metadata) {
$this->metadata[$offset] = $value->metadata;
}
} elseif ($value) {
$this->metadata[$offset] = $value;
}
}
function offsetUnset($offset)
{
unset($this->metadata[$offset]);
}
}
class TP_yyStackEntry
{
public $stateno; /* The state-number */
public $major; /* The major token value. This is the code
** number for the token at this stack level */
public $minor; /* The user-supplied minor token value. This
** is the value of the token */
};
#line 12 "smarty_internal_templateparser.y"
class Smarty_Internal_Templateparser#line 79 "smarty_internal_templateparser.php"
{
#line 14 "smarty_internal_templateparser.y"
const Err1 = "Security error: Call to private object member not allowed";
const Err2 = "Security error: Call to dynamic object member not allowed";
// states whether the parse was successful or not
public $successful = true;
public $retvalue = 0;
private $lex;
private $internalError = false;
function __construct($lex, $compiler) {
// set instance object
self::instance($this);
$this->lex = $lex;
$this->compiler = $compiler;
$this->smarty = $this->compiler->smarty;
$this->template = $this->compiler->template;
$this->compiler->has_variable_string = false;
$this->compiler->prefix_code = array();
$this->prefix_number = 0;
$this->block_nesting_level = 0;
if ($this->security = isset($this->smarty->security_policy)) {
$this->php_handling = $this->smarty->security_policy->php_handling;
} else {
$this->php_handling = $this->smarty->php_handling;
}
$this->is_xml = false;
$this->asp_tags = (ini_get('asp_tags') != '0');
$this->current_buffer = $this->root_buffer = new _smarty_template_buffer($this);
}
public static function &instance($new_instance = null)
{
static $instance = null;
if (isset($new_instance) && is_object($new_instance))
$instance = $new_instance;
return $instance;
}
public static function escape_start_tag($tag_text) {
$tag = preg_replace('/\A<\?(.*)\z/', '<<?php ?>?\1', $tag_text, -1 , $count); //Escape tag
assert($tag !== false && $count === 1);
return $tag;
}
public static function escape_end_tag($tag_text) {
assert($tag_text === '?>');
return '?<?php ?>>';
}
#line 132 "smarty_internal_templateparser.php"
const TP_VERT = 1;
const TP_COLON = 2;
const TP_COMMENT = 3;
const TP_PHPSTARTTAG = 4;
const TP_PHPENDTAG = 5;
const TP_ASPSTARTTAG = 6;
const TP_ASPENDTAG = 7;
const TP_FAKEPHPSTARTTAG = 8;
const TP_XMLTAG = 9;
const TP_OTHER = 10;
const TP_LINEBREAK = 11;
const TP_LITERALSTART = 12;
const TP_LITERALEND = 13;
const TP_LITERAL = 14;
const TP_LDEL = 15;
const TP_RDEL = 16;
const TP_DOLLAR = 17;
const TP_ID = 18;
const TP_EQUAL = 19;
const TP_PTR = 20;
const TP_LDELIF = 21;
const TP_SPACE = 22;
const TP_LDELFOR = 23;
const TP_SEMICOLON = 24;
const TP_INCDEC = 25;
const TP_TO = 26;
const TP_STEP = 27;
const TP_LDELFOREACH = 28;
const TP_AS = 29;
const TP_APTR = 30;
const TP_SMARTYBLOCKCHILD = 31;
const TP_LDELSLASH = 32;
const TP_INTEGER = 33;
const TP_COMMA = 34;
const TP_MATH = 35;
const TP_UNIMATH = 36;
const TP_ANDSYM = 37;
const TP_ISIN = 38;
const TP_ISDIVBY = 39;
const TP_ISNOTDIVBY = 40;
const TP_ISEVEN = 41;
const TP_ISNOTEVEN = 42;
const TP_ISEVENBY = 43;
const TP_ISNOTEVENBY = 44;
const TP_ISODD = 45;
const TP_ISNOTODD = 46;
const TP_ISODDBY = 47;
const TP_ISNOTODDBY = 48;
const TP_INSTANCEOF = 49;
const TP_OPENP = 50;
const TP_CLOSEP = 51;
const TP_QMARK = 52;
const TP_NOT = 53;
const TP_TYPECAST = 54;
const TP_HEX = 55;
const TP_DOT = 56;
const TP_SINGLEQUOTESTRING = 57;
const TP_DOUBLECOLON = 58;
const TP_AT = 59;
const TP_HATCH = 60;
const TP_OPENB = 61;
const TP_CLOSEB = 62;
const TP_EQUALS = 63;
const TP_NOTEQUALS = 64;
const TP_GREATERTHAN = 65;
const TP_LESSTHAN = 66;
const TP_GREATEREQUAL = 67;
const TP_LESSEQUAL = 68;
const TP_IDENTITY = 69;
const TP_NONEIDENTITY = 70;
const TP_MOD = 71;
const TP_LAND = 72;
const TP_LOR = 73;
const TP_LXOR = 74;
const TP_QUOTE = 75;
const TP_BACKTICK = 76;
const TP_DOLLARID = 77;
const YY_NO_ACTION = 572;
const YY_ACCEPT_ACTION = 571;
cons
没有合适的资源?快使用搜索试试~ 我知道了~
ASP实例开发源码-东光生活网(东光地方生活信息门户站) 科讯内核 v4.0.zip
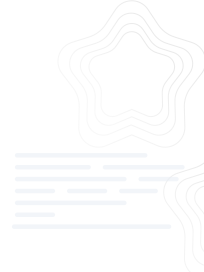
共1691个文件
gif:679个
php:298个
html:226个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 6 浏览量
2022-11-15
20:05:50
上传
评论
收藏 8.18MB ZIP 举报
温馨提示
ASP实例开发源码—东光生活网(东光地方生活信息门户站) 科讯内核 v4.0.zip ASP实例开发源码—东光生活网(东光地方生活信息门户站) 科讯内核 v4.0.zip ASP实例开发源码—东光生活网(东光地方生活信息门户站) 科讯内核 v4.0.zip
资源推荐
资源详情
资源评论
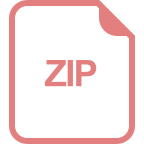
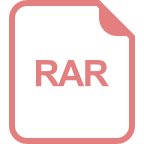
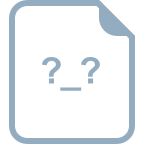
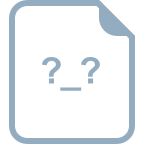
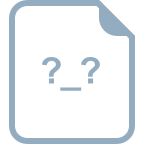
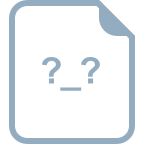
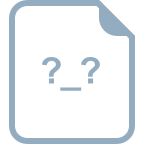
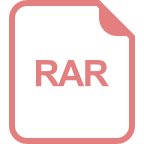
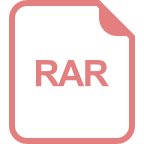
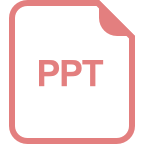
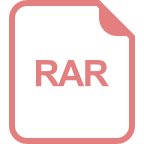
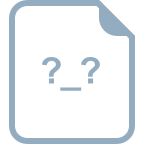
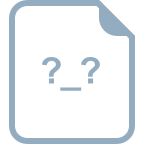
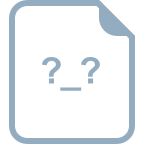
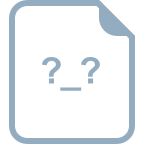
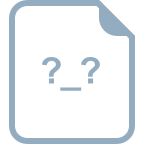
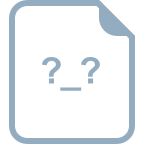
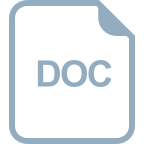
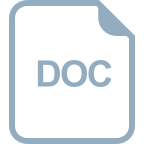
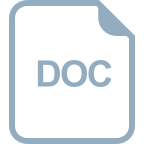
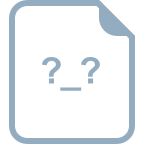
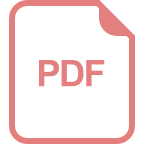
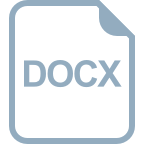
收起资源包目录

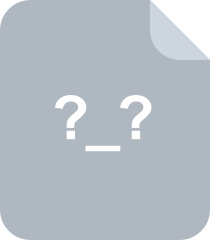
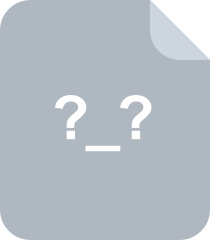
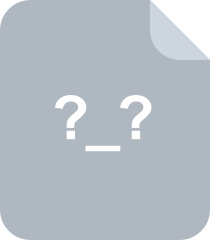
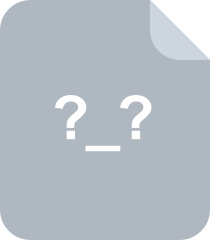
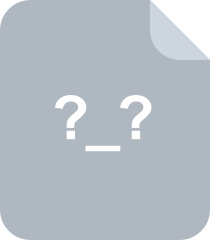
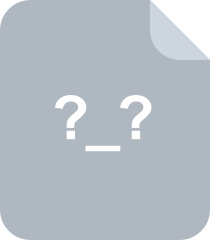
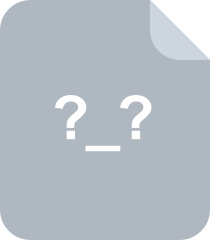
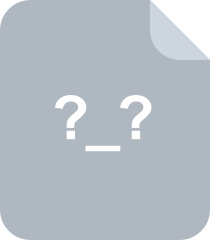
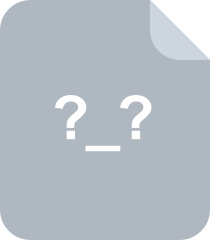
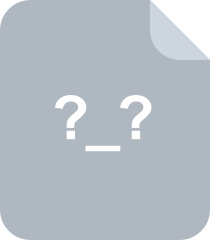
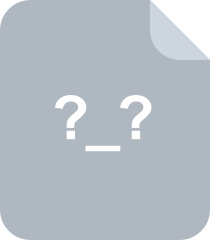
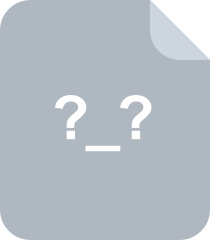
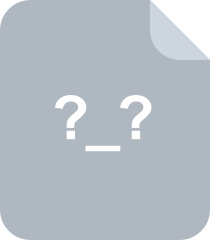
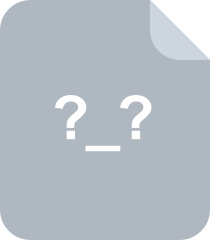
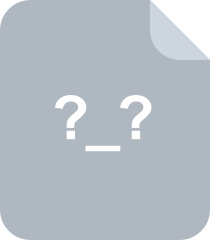
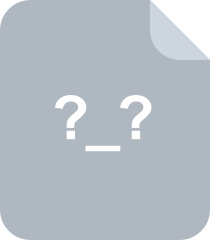
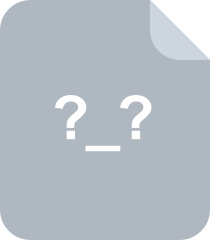
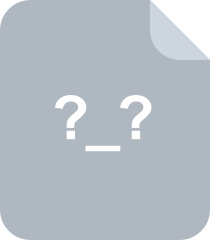
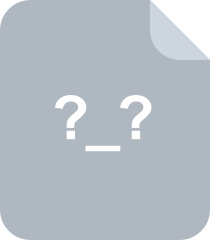
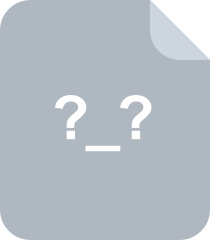
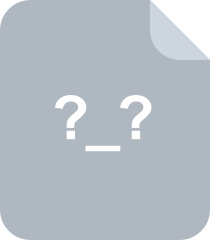
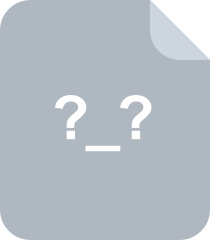
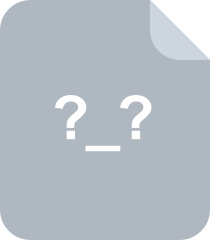
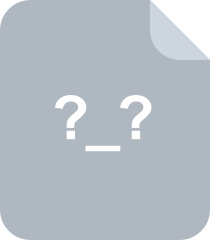
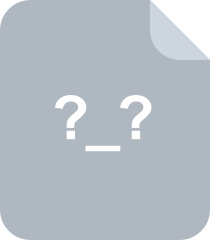
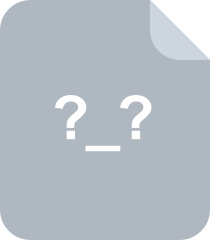
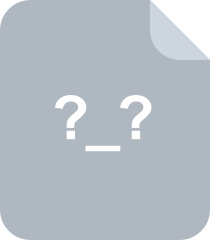
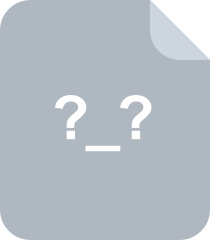
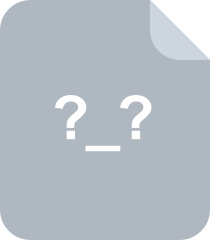
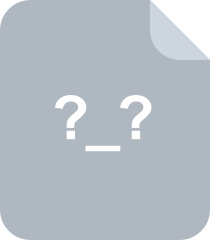
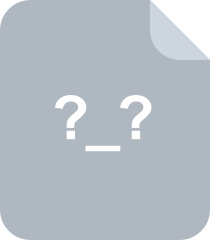
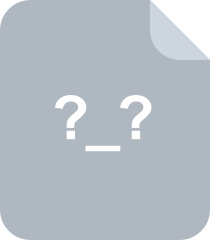
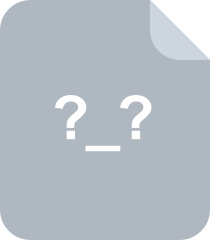
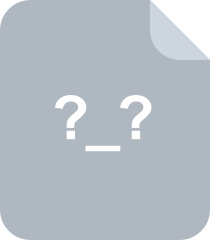
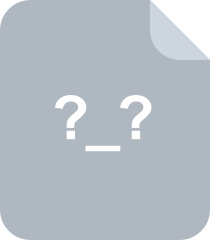
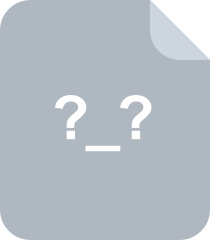
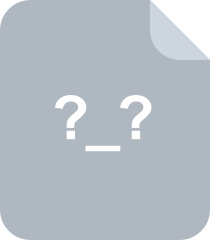
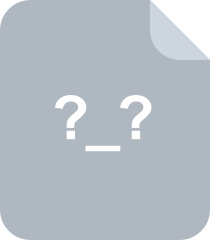
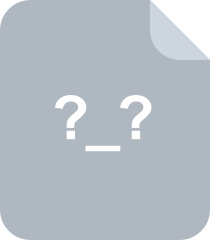
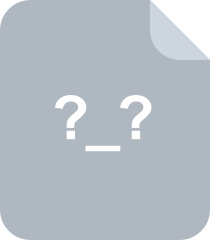
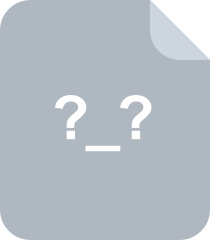
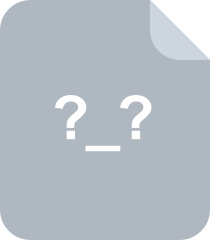
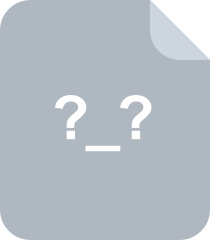
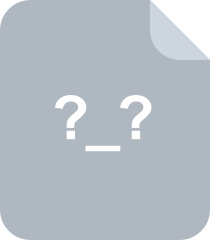
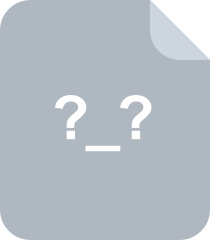
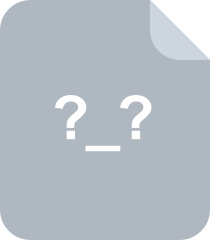
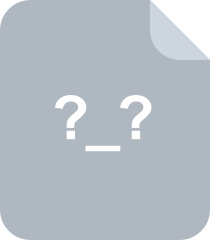
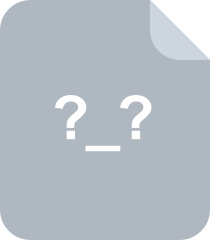
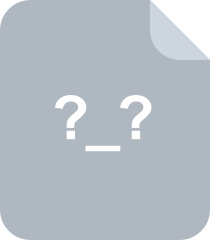
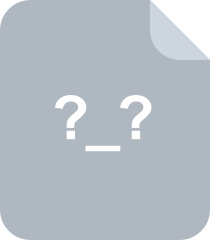
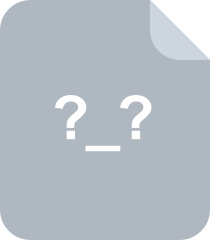
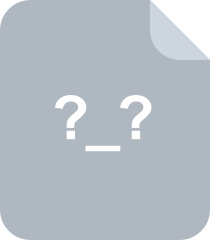
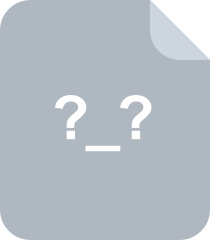
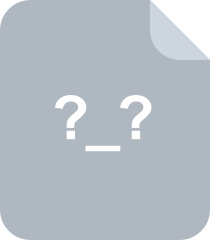
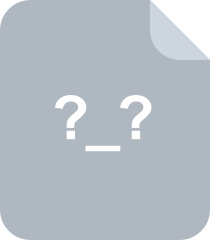
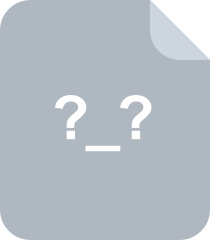
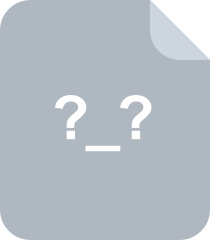
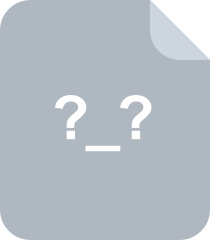
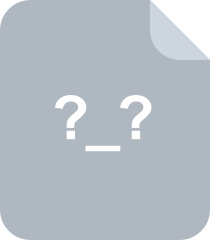
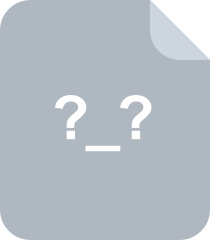
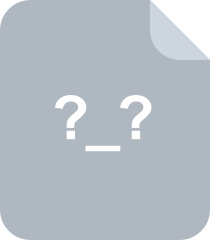
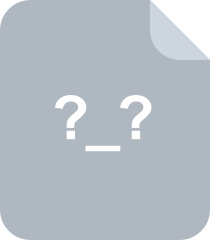
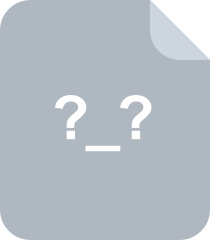
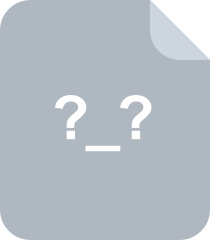
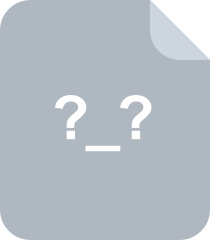
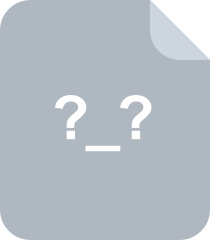
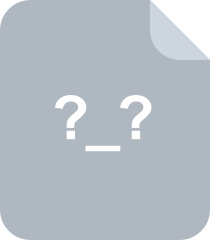
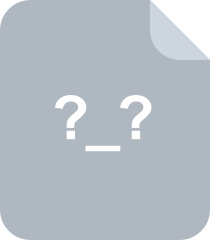
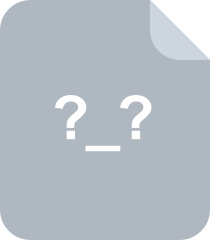
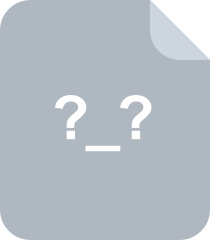
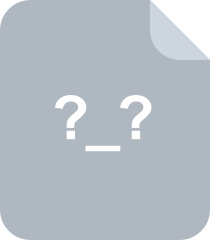
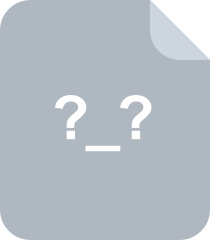
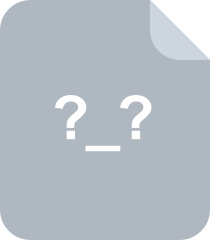
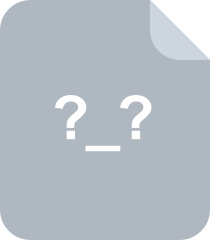
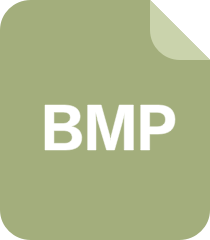
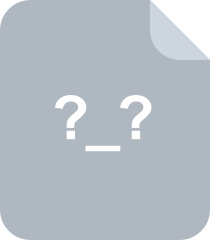
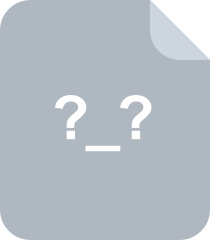
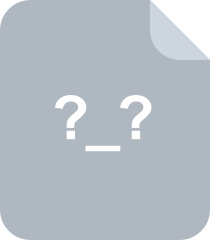
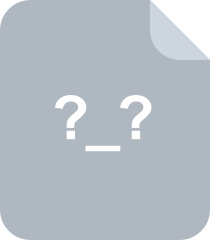
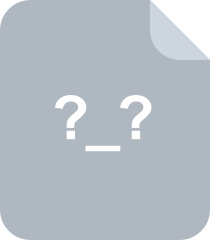
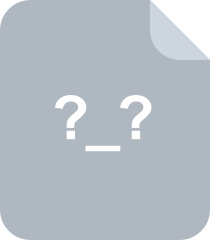
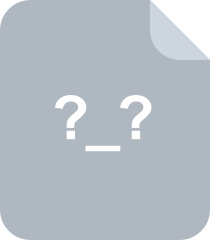
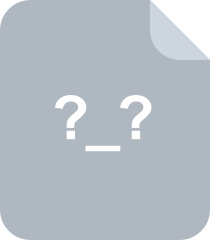
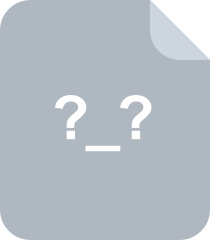
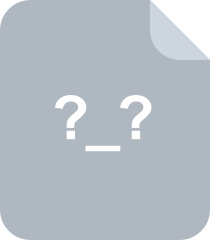
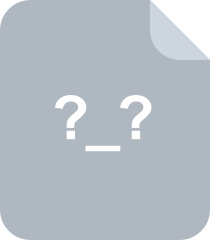
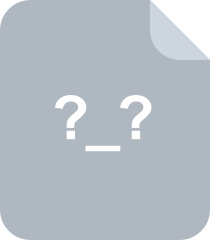
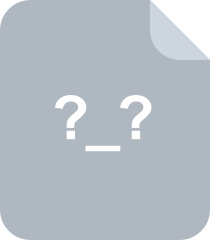
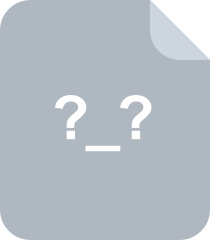
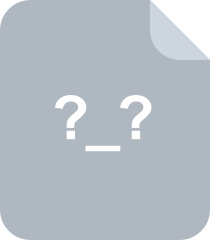
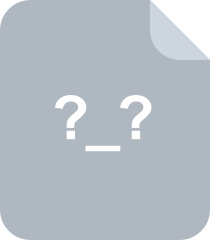
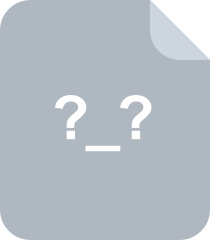
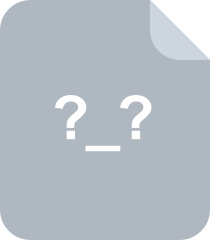
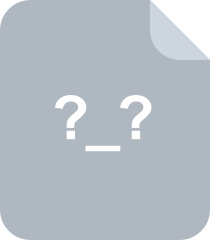
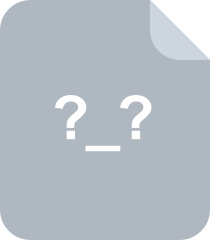
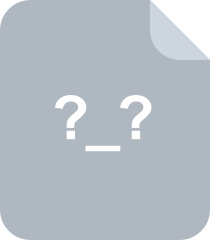
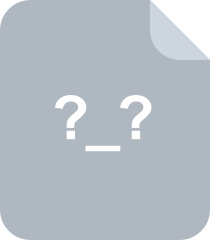
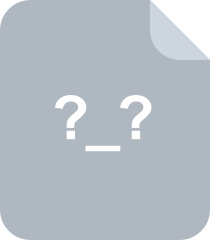
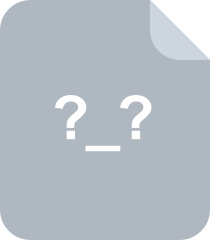
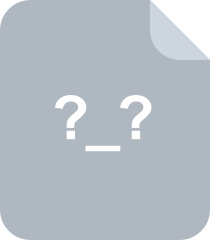
共 1691 条
- 1
- 2
- 3
- 4
- 5
- 6
- 17
资源评论
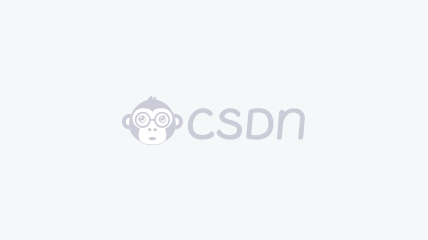
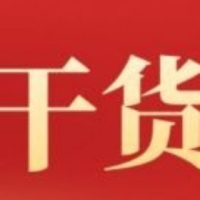
毕业_设计
- 粉丝: 1974
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

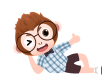
安全验证
文档复制为VIP权益,开通VIP直接复制
