# MPU9250
Arduino library for communicating with the [MPU-9250](https://www.invensense.com/products/motion-tracking/9-axis/mpu-9250/) and MPU-9255 nine-axis Inertial Measurement Units (IMU).
# Description
The InvenSense MPU-9250 is a System in Package (SiP) that combines two chips: the MPU-6500 three-axis gyroscope and three-axis accelerometer; and the AK8963 three-axis magnetometer. The MPU-9250 supports I2C, up to 400 kHz, and SPI communication, up to 1 MHz for register setup and 20 MHz for data reading. The following selectable full scale sensor ranges are available:
| Gyroscope Full Scale Range | Accelerometer Full Scale Range | Magnetometer Full Scale Range |
| --- | --- | --- |
| +/- 250 (deg/s) | +/- 2 (g) | +/- 4800 (uT) |
| +/- 500 (deg/s) | +/- 4 (g) | |
| +/- 1000 (deg/s) | +/- 8 (g) | |
| +/- 2000 (deg/s) | +/- 16 (g) | |
The MPU-9250 samples the gyroscopes, accelerometers, and magnetometers with 16 bit analog to digital converters. It also features programmable digital filters, a precision clock, an embedded temperature sensor, programmable interrupts (including wake on motion), and a 512 byte FIFO buffer.
# Usage
This library supports both I2C and SPI commmunication with the MPU-9250.
## Installation
Simply clone or download this library into your Arduino/libraries folder.
## Function Description
This library supports both I2C and SPI communication with the MPU-9250. The *MPU9250* object declaration is overloaded with different declarations for I2C and SPI communication. All other functions remain the same. Additionally, a derived class, *MPU250FIFO*, is included, which provides FIFO setup and data collection functionality in addition to all of the functionality included in the base *MPU9250* class.
## MPU9250 Class
### I2C Object Declaration
**MPU9250(TwoWire &bus,uint8_t address)**
An MPU9250 object should be declared, specifying the I2C bus and MPU-9250 I2C address. The MPU-9250 I2C address will be 0x68 if the AD0 pin is grounded or 0x69 if the AD0 pin is pulled high. For example, the following code declares an MPU9250 object called *IMU* with an MPU-9250 sensor located on I2C bus 0 with a sensor address of 0x68 (AD0 grounded).
```C++
MPU9250 IMU(Wire,0x68);
```
### SPI Object Declaratioon
**MPU9250(SPIClass &bus,uint8_t csPin)**
An MPU9250 object should be declared, specifying the SPI bus and chip select pin used. Multiple MPU-9250 or other SPI objects could be used on the same SPI bus, each with their own chip select pin. The chip select pin can be any available digital pin. For example, the following code declares an MPU9250 object called *IMU* with an MPU-9250 sensor located on SPI bus 0 with chip select pin 10.
```C++
MPU9250 IMU(SPI,10);
```
### Common Setup Functions
The following functions are used to setup the MPU-9250 sensor. These should be called once before data collection, typically this is done in the Arduino *void setup()* function. The *begin* function should always be used. Optionally, the *setAccelRange* and *setGyroRange*, *setDlpfBandwidth*, and *setSrd* functions can be used to set the accelerometer and gyroscope full scale ranges, DLPF bandwidth, and SRD to values other than default. The *enableDataReadyInterrupt* and *disableDataReadyInterrupt* control whether the MPU-9250 generates an interrupt on data ready. The *enableWakeOnMotion* puts the MPU-9250 into a low power mode and enables an interrupt when motion detected is above a given threshold. Finally, *enableFifo* sets up and enables the FIFO buffer. These functions are described in detail, below.
**int begin()**
This should be called in your setup function. It initializes communication with the MPU-9250, sets up the sensor for reading data, and estimates the gyro bias, which is removed from the sensor data. This function returns a positive value on a successful initialization and returns a negative value on an unsuccesful initialization. If unsuccessful, please check your wiring or try resetting power to the sensor. The following is an example of setting up the MPU-9250.
```C++
int status;
status = IMU.begin();
```
#### Configuration Functions
**(optional) int setAccelRange(AccelRange range)**
This function sets the accelerometer full scale range to the given value. By default, if this function is not called, a full scale range of +/- 16 g will be used. The enumerated accelerometer full scale ranges are:
| Accelerometer Name | Accelerometer Full Scale Range |
| ------------------ | ------------------------------ |
| ACCEL_RANGE_2G | +/- 2 (g) |
| ACCEL_RANGE_4G | +/- 4 (g) |
| ACCEL_RANGE_8G | +/- 8 (g) |
| ACCEL_RANGE_16G | +/- 16 (g) |
This function returns a positive value on success and a negative value on failure. Please see the *Advanced_I2C example*. The following is an example of selecting an accelerometer full scale range of +/- 8g.
```C++
status = IMU.setAccelRange(MPU9250::ACCEL_RANGE_8G);
```
**(optional) int setGyroRange(GyroRange range)**
This function sets the gyroscope full scale range to the given value. By default, if this function is not called, a full scale range of +/- 2000 deg/s will be used. The enumerated gyroscope full scale ranges are:
| Gyroscope Name | Gyroscope Full Scale Range |
| ------------------ | -------------------------- |
| GYRO_RANGE_250DPS | +/- 250 (deg/s) |
| GYRO_RANGE_500DPS | +/- 500 (deg/s) |
| GYRO_RANGE_1000DPS | +/- 1000 (deg/s) |
| GYRO_RANGE_2000DPS | +/- 2000 (deg/s) |
This function returns a positive value on success and a negative value on failure. Please see the *Advanced_I2C example*. The following is an example of selecting an gyroscope full scale range of +/- 250 deg/s.
```C++
status = IMU.setGyroRange(MPU9250::GYRO_RANGE_250DPS);
```
**(optional) int setDlpfBandwidth(DlpfBandwidth bandwidth)**
This is an optional function to set the programmable Digital Low Pass Filter (DLPF) bandwidth. By default, if this function is not called, a DLPF bandwidth of 184 Hz is used. The following DLPF bandwidths are supported:
| Bandwidth Name | DLPF Bandwidth | Gyroscope Delay | Accelerometer Delay | Temperature Bandwidth | Temperature Delay |
| --- | --- | --- | --- | --- | --- |
| DLPF_BANDWIDTH_184HZ | 184 Hz | 2.9 ms | 5.8 ms | 188 Hz | 1.9 ms |
| DLPF_BANDWIDTH_92HZ | 92 Hz | 3.9 ms | 7.8 ms | 98 Hz | 2.8 ms |
| DLPF_BANDWIDTH_41HZ | 41 Hz | 5.9 ms | 11.8 ms | 42 Hz | 4.8 ms |
| DLPF_BANDWIDTH_20HZ | 20 Hz | 9.9 ms | 19.8 ms | 20 Hz | 8.3 ms |
| DLPF_BANDWIDTH_10HZ | 10 Hz | 17.85 ms | 35.7 ms | 10 Hz | 13.4 ms |
| DLPF_BANDWIDTH_5HZ | 5 Hz | 33.48 ms | 66.96 ms | 5 Hz | 18.6 ms |
This function returns a positive value on success and a negative value on failure. Please see the *Advanced_I2C example*. The following is an example of selecting a DLPF bandwidth of 20 Hz.
```C++
status = IMU.setDlpfBandwidth(MPU9250::DLPF_BANDWIDTH_20HZ);
```
**(optional) int setSrd(uint8_t srd)**
This is an optional function to set the data output rate. The data output rate is set by a sample rate divider, *uint8_t SRD*. The data output rate is then given by:
*Data Output Rate = 1000 / (1 + SRD)*
By default, if this function is not called, an SRD of 0 is used resulting in a data output rate of 1000 Hz. This allows the data output rate for the gyroscopes, accelerometers, and temperature sensor to be set between 3.9 Hz and 1000 Hz. Note that data should be read at or above the selected rate. In order to prevent aliasing, the data should be sampled at twice the frequency of the DLPF bandwidth or higher. For example, this means for a DLPF bandwidth set to 41 Hz, the data output rate and data collection should be at frequencies of 82 Hz or higher.
The magnetometer is fixed to an output rate of:

t0_54coder
- 粉丝: 3171
- 资源: 5642
最新资源
- 西安电子科技大学在线评测系统(xdoj)题目分级解析及维护机制
- Simulink加速自适应与经典AUTOSAR平台中的SOA软件开发技术解析及应用
- 多种渠道合法获取开源及商业软件源码的方法汇总
- 中国30各省份的人均GDP(元/人)(2013-2022年)
- 太原理工大学javaee实践教学:从校内项目到学科竞赛及校企合作的全面覆盖
- 新能源动力电池ccd自动检测机sw21可编辑全套技术资料100%好用.zip
- 获取开源及商业软件源码的技术路径与渠道
- 西安电子科技大学在线评测系统(XDOJ)的题目难度分析及维护机制解析
- 中国30个省份第二产业结构占比(%)(2013-2022年)
- 太原理工大学javaee高校JavaEE实践项目:从校内课程到校企合作的应用案例解析及实施途径
- PPPOE实训拓扑.docx
- 树莓派双线寻迹,opencv+python
- 中国30个省份的城镇化率(%)(2013-2022年)
- 车用驱动电机原理与控制基础-P143公式(6-39)
- 2023-04-06-项目笔记 - 第三百六十九阶段 - 4.4.2.367全局变量的作用域-367 -2025.01.05
- 2023-04-06-项目笔记 - 第三百六十九阶段 - 4.4.2.367全局变量的作用域-367 -2025.01.05
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


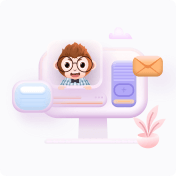