# ESPAsyncWebServer
[](https://travis-ci.org/me-no-dev/ESPAsyncWebServer)  [](https://www.codacy.com/manual/me-no-dev/ESPAsyncWebServer?utm_source=github.com&utm_medium=referral&utm_content=me-no-dev/ESPAsyncWebServer&utm_campaign=Badge_Grade)
For help and support [](https://gitter.im/me-no-dev/ESPAsyncWebServer?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
Async HTTP and WebSocket Server for ESP8266 Arduino
For ESP8266 it requires [ESPAsyncTCP](https://github.com/me-no-dev/ESPAsyncTCP)
To use this library you might need to have the latest git versions of [ESP8266](https://github.com/esp8266/Arduino) Arduino Core
For ESP32 it requires [AsyncTCP](https://github.com/me-no-dev/AsyncTCP) to work
To use this library you might need to have the latest git versions of [ESP32](https://github.com/espressif/arduino-esp32) Arduino Core
## Table of contents
- [ESPAsyncWebServer](#espasyncwebserver)
- [Table of contents](#table-of-contents)
- [Installation](#installation)
- [Using PlatformIO](#using-platformio)
- [Why should you care](#why-should-you-care)
- [Important things to remember](#important-things-to-remember)
- [Principles of operation](#principles-of-operation)
- [The Async Web server](#the-async-web-server)
- [Request Life Cycle](#request-life-cycle)
- [Rewrites and how do they work](#rewrites-and-how-do-they-work)
- [Handlers and how do they work](#handlers-and-how-do-they-work)
- [Responses and how do they work](#responses-and-how-do-they-work)
- [Template processing](#template-processing)
- [Libraries and projects that use AsyncWebServer](#libraries-and-projects-that-use-asyncwebserver)
- [Request Variables](#request-variables)
- [Common Variables](#common-variables)
- [Headers](#headers)
- [GET, POST and FILE parameters](#get-post-and-file-parameters)
- [FILE Upload handling](#file-upload-handling)
- [Body data handling](#body-data-handling)
- [JSON body handling with ArduinoJson](#json-body-handling-with-arduinojson)
- [Responses](#responses)
- [Redirect to another URL](#redirect-to-another-url)
- [Basic response with HTTP Code](#basic-response-with-http-code)
- [Basic response with HTTP Code and extra headers](#basic-response-with-http-code-and-extra-headers)
- [Basic response with string content](#basic-response-with-string-content)
- [Basic response with string content and extra headers](#basic-response-with-string-content-and-extra-headers)
- [Send large webpage from PROGMEM](#send-large-webpage-from-progmem)
- [Send large webpage from PROGMEM and extra headers](#send-large-webpage-from-progmem-and-extra-headers)
- [Send large webpage from PROGMEM containing templates](#send-large-webpage-from-progmem-containing-templates)
- [Send large webpage from PROGMEM containing templates and extra headers](#send-large-webpage-from-progmem-containing-templates-and-extra-headers)
- [Send binary content from PROGMEM](#send-binary-content-from-progmem)
- [Respond with content coming from a Stream](#respond-with-content-coming-from-a-stream)
- [Respond with content coming from a Stream and extra headers](#respond-with-content-coming-from-a-stream-and-extra-headers)
- [Respond with content coming from a Stream containing templates](#respond-with-content-coming-from-a-stream-containing-templates)
- [Respond with content coming from a Stream containing templates and extra headers](#respond-with-content-coming-from-a-stream-containing-templates-and-extra-headers)
- [Respond with content coming from a File](#respond-with-content-coming-from-a-file)
- [Respond with content coming from a File and extra headers](#respond-with-content-coming-from-a-file-and-extra-headers)
- [Respond with content coming from a File containing templates](#respond-with-content-coming-from-a-file-containing-templates)
- [Respond with content using a callback](#respond-with-content-using-a-callback)
- [Respond with content using a callback and extra headers](#respond-with-content-using-a-callback-and-extra-headers)
- [Respond with content using a callback containing templates](#respond-with-content-using-a-callback-containing-templates)
- [Respond with content using a callback containing templates and extra headers](#respond-with-content-using-a-callback-containing-templates-and-extra-headers)
- [Chunked Response](#chunked-response)
- [Chunked Response containing templates](#chunked-response-containing-templates)
- [Print to response](#print-to-response)
- [ArduinoJson Basic Response](#arduinojson-basic-response)
- [ArduinoJson Advanced Response](#arduinojson-advanced-response)
- [Serving static files](#serving-static-files)
- [Serving specific file by name](#serving-specific-file-by-name)
- [Serving files in directory](#serving-files-in-directory)
- [Serving static files with authentication](#serving-static-files-with-authentication)
- [Specifying Cache-Control header](#specifying-cache-control-header)
- [Specifying Date-Modified header](#specifying-date-modified-header)
- [Specifying Template Processor callback](#specifying-template-processor-callback)
- [Param Rewrite With Matching](#param-rewrite-with-matching)
- [Using filters](#using-filters)
- [Serve different site files in AP mode](#serve-different-site-files-in-ap-mode)
- [Rewrite to different index on AP](#rewrite-to-different-index-on-ap)
- [Serving different hosts](#serving-different-hosts)
- [Determine interface inside callbacks](#determine-interface-inside-callbacks)
- [Bad Responses](#bad-responses)
- [Respond with content using a callback without content length to HTTP/1.0 clients](#respond-with-content-using-a-callback-without-content-length-to-http10-clients)
- [Async WebSocket Plugin](#async-websocket-plugin)
- [Async WebSocket Event](#async-websocket-event)
- [Methods for sending data to a socket client](#methods-for-sending-data-to-a-socket-client)
- [Direct access to web socket message buffer](#direct-access-to-web-socket-message-buffer)
- [Limiting the number of web socket clients](#limiting-the-number-of-web-socket-clients)
- [Async Event Source Plugin](#async-event-source-plugin)
- [Setup Event Source on the server](#setup-event-source-on-the-server)
- [Setup Event Source in the browser](#setup-event-source-in-the-browser)
- [Scanning for available WiFi Networks](#scanning-for-available-wifi-networks)
- [Remove handlers and rewrites](#remove-handlers-and-rewrites)
- [Setting up the server](#setting-up-the-server)
- [Setup global and class functions as request handlers](#setup-global-and-class-functions-as-request-handlers)
- [Methods for controlling websocket connections](#methods-for-controlling-websocket-connections)
- [Adding Default Headers](#adding-default-headers)
- [Path variable](#path-variable)
## Installation
### Using PlatformIO
[PlatformIO](http://platformio.org) is an open source ecosystem for IoT development with cross platform build system, library manager and full support for Espressif ESP8266/ESP32 development. It works on the popular host OS: Mac OS X, Windows, Linux 32/64, Linux ARM (like Raspberry Pi, BeagleBone, CubieBoard).
1. Install [PlatformIO IDE](http://platformio.org/platformio-ide)
2. Create new project using "PlatformIO Home > New Project"
3. Update dev/platform to stagin
没有合适的资源?快使用搜索试试~ 我知道了~
(源码)基于C++编程语言的住宅环境传感器监控系统.zip
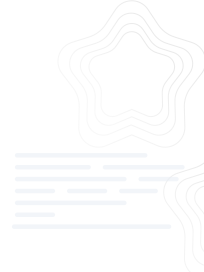
共128个文件
h:25个
cpp:23个
sh:9个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 54 浏览量
2024-11-16
13:08:25
上传
评论
收藏 467KB ZIP 举报
温馨提示
# 基于C++编程语言的住宅环境传感器监控系统 ## 项目简介 该项目是一个基于C++编程语言的住宅环境监控系统,通过ESP32或其他类似的微控制器,实现对住宅环境参数的监控,如土壤湿度、环境温度和湿度等。系统支持通过MQTT协议将传感器数据发布到远程服务器,并通过Web界面实现动态配置和更新。 ## 主要特性和功能 1. 传感器数据处理通过传感器库读取土壤湿度、环境温度和湿度等数据,并对其进行有效性检查和处理。 2. MQTT通信使用MQTT协议将传感器数据发布到远程服务器,以便进行远程监控和控制。 3. Web配置界面在设备处于访问点(AP)模式时,通过Web服务器提供动态配置界面,允许用户通过表单输入WiFi网络的SSID、密码以及MQTT服务器的地址和端口号等信息。 4. 深度睡眠模式在空闲状态下,ESP设备进入深度睡眠模式以降低功耗。 5. OTA更新支持通过OverTheAir(OTA)更新固件和文件系统。
资源推荐
资源详情
资源评论
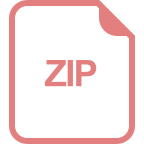
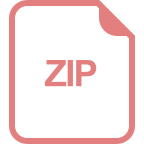
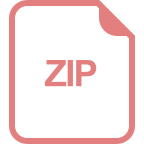
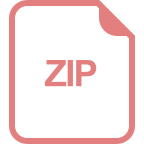
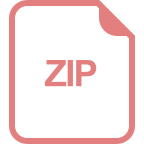
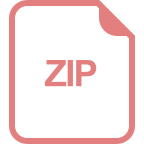
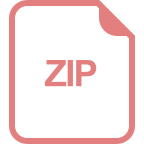
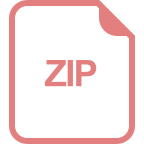
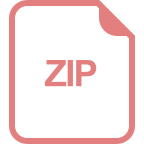
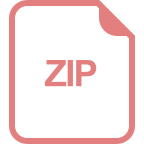
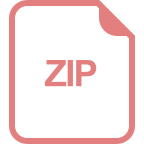
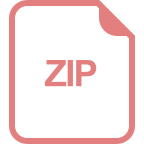
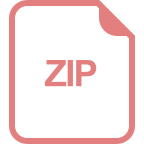
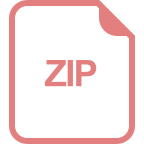
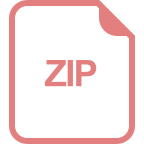
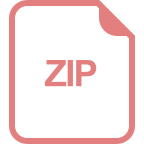
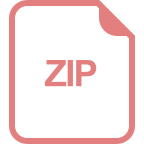
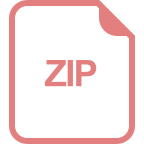
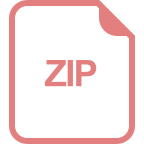
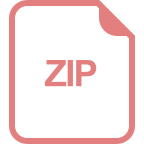
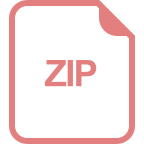
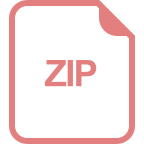
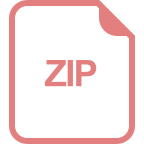
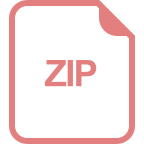
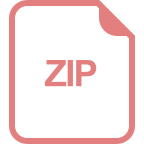
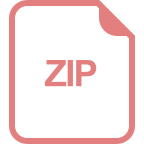
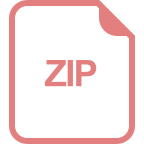
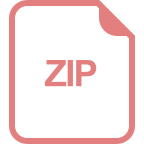
收起资源包目录

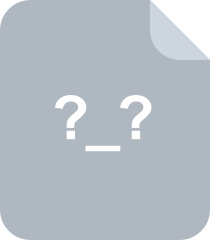
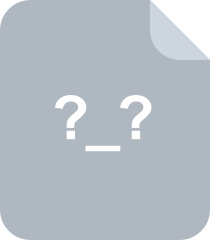
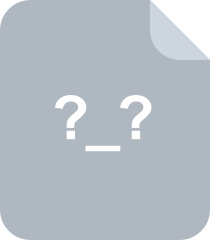
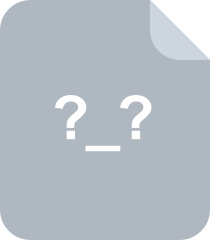
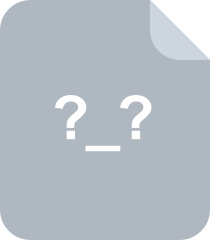
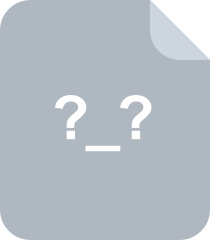
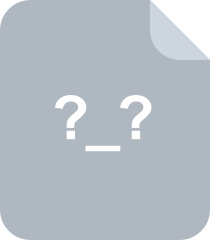
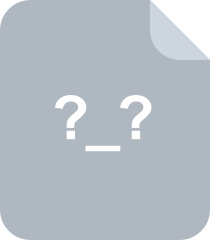
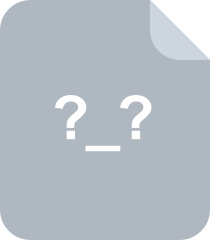
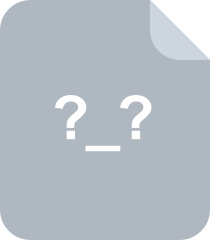
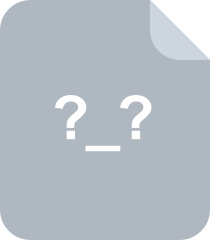
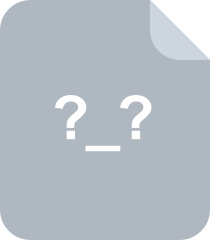
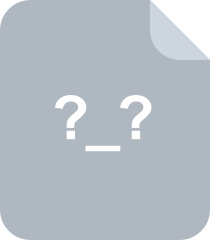
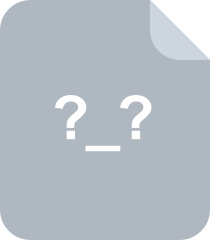
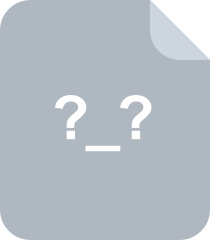
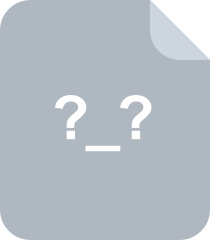
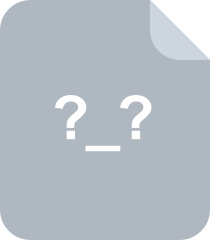
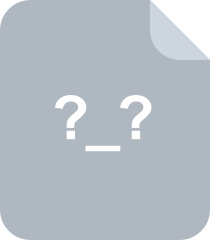
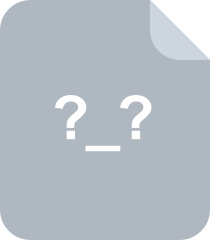
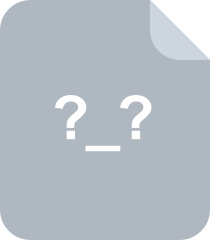
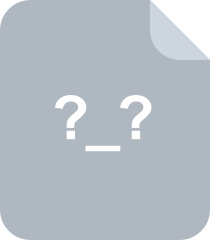
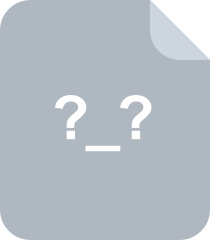
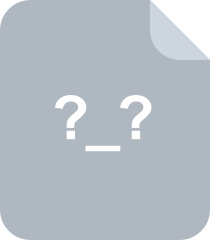
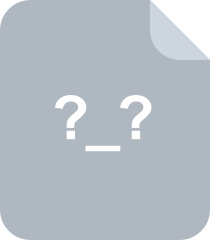
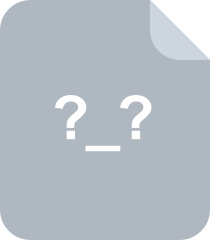
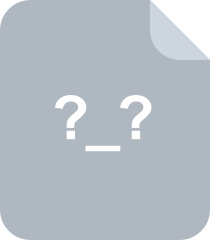
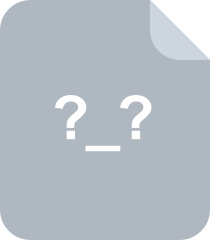
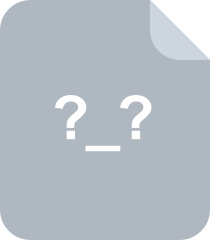
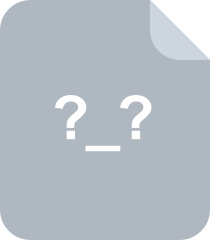
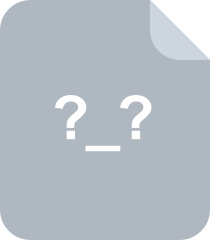
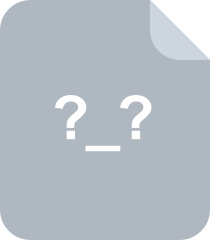
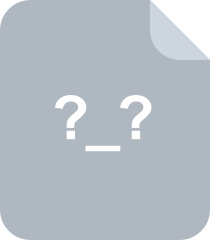
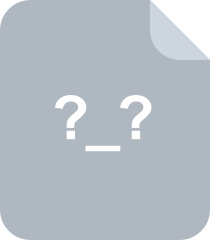






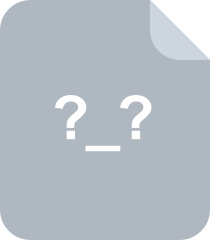
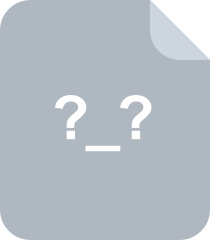
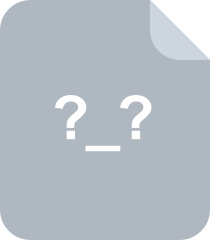
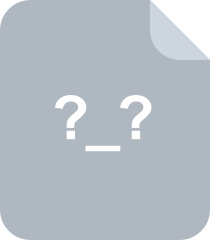
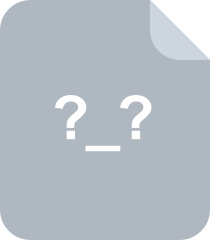
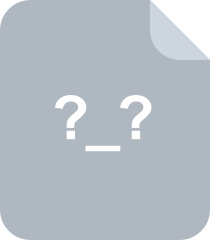
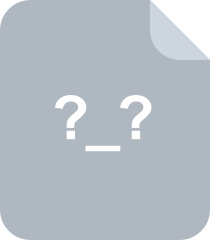
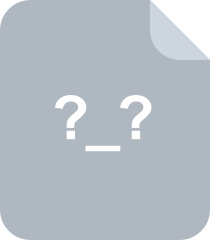
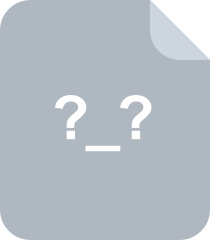
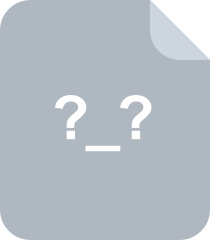
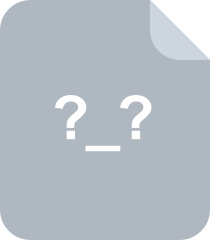
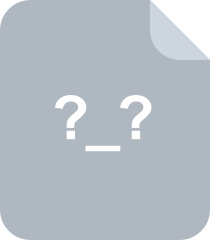
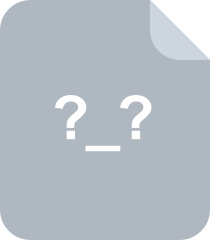
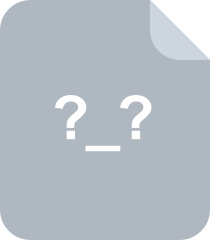
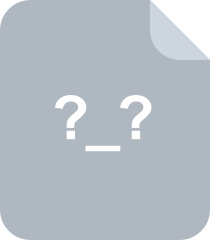
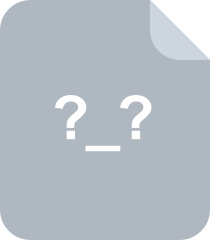
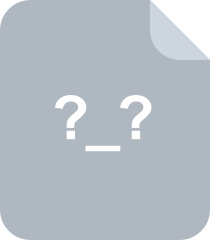
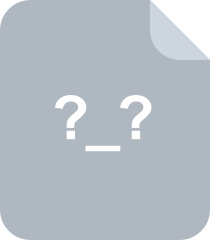
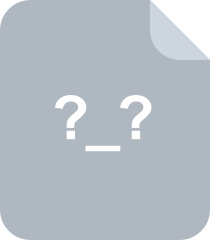
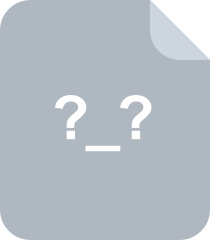
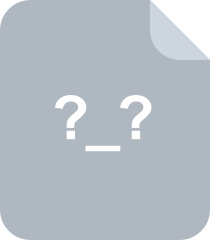
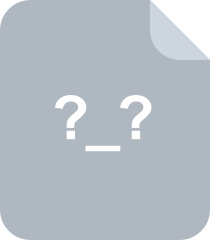
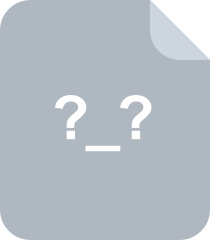
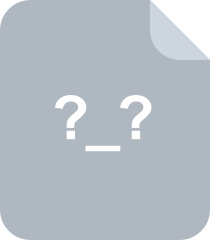
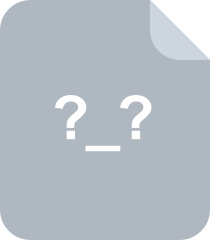
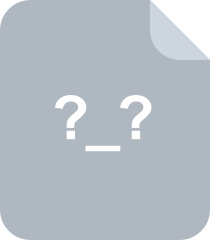
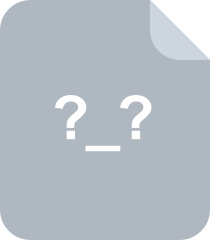
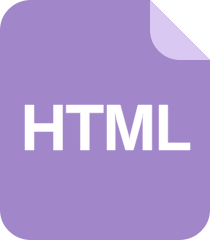
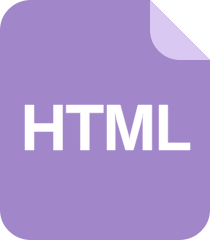
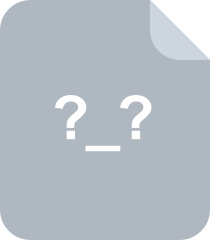
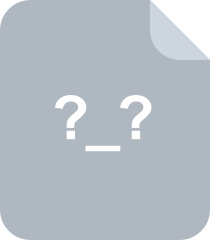
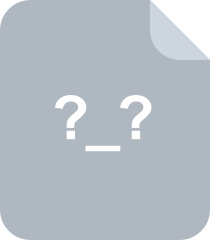
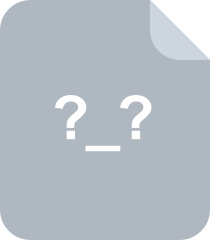
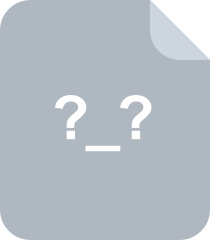
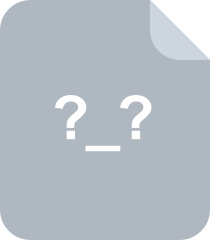
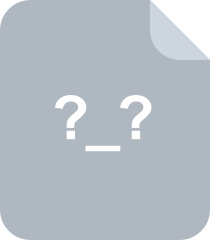
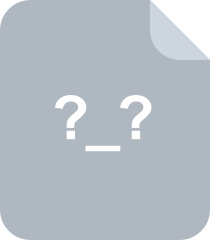
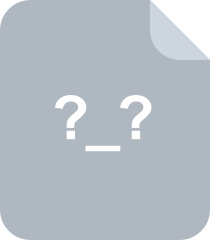
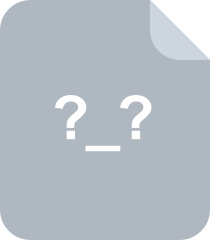
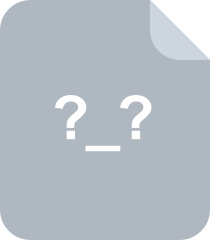
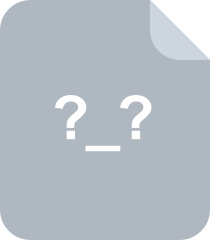
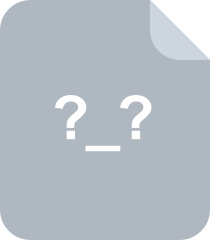
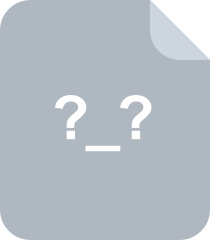
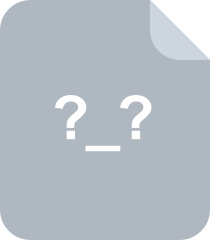
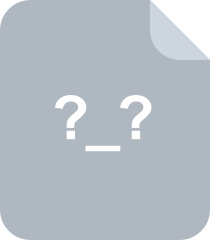
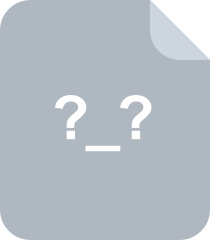
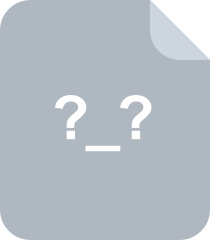
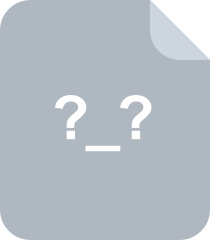
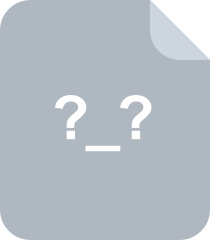
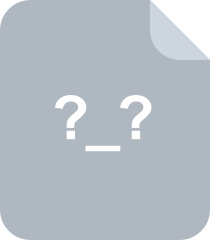
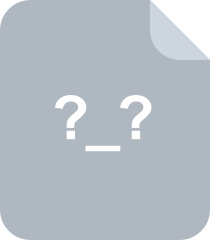
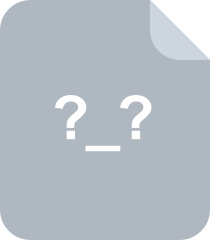
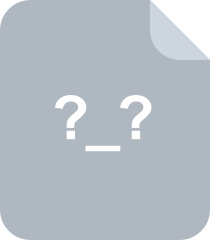
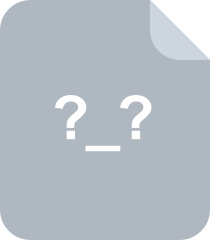
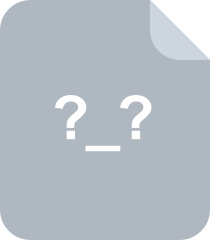
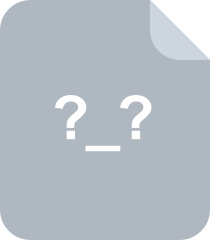
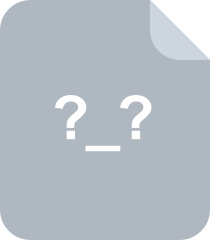
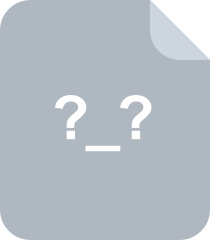
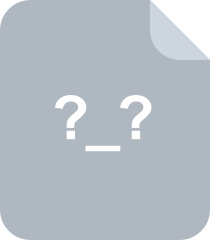
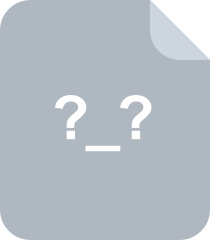
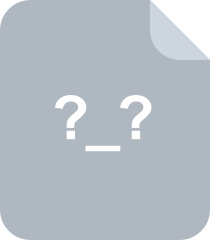
共 128 条
- 1
- 2
资源评论
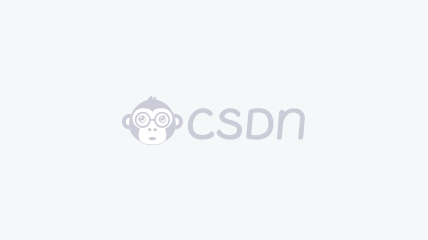

t0_54coder
- 粉丝: 2389
- 资源: 2808

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

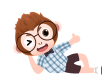
最新资源
- (源码)基于gRPC和Zookeeper的GirafKV分布式键值存储系统.zip
- javaEE企业级B2C商城源码带文档数据库 MySQL源码类型 WebForm
- (源码)基于Spark2.x和Flume的实时新闻分析系统.zip
- (源码)基于C#的礼服管控系统.zip
- R语言数据去重与匹配:20种常用函数详解及实战示例
- (源码)基于SpringCloudAlibaba的系统管理平台.zip
- java企业级维修订单系统源码数据库 MySQL源码类型 WebForm
- (源码)基于ESP32S2和腾讯云的物联网设备管理系统.zip
- DENON天龙回音壁功放DHT-S514维修手册dpf
- (源码)基于Arduino和Firebase的物联网称重监测系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


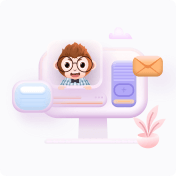
安全验证
文档复制为VIP权益,开通VIP直接复制
