/*
这段代码是一个使用Java编写的类,名为`CommentExample`,它定义了一个查询评论(Comment)数据的标准。该类通过`Criteria`类来构建查询条件,用于数据库查询。
该类的主要部分如下:
1. `orderByClause`:用于排序的SQL语句片段。
2. `distinct`:是否进行去重查询的布尔值。
3. `oredCriteria`:一个`Criteria`对象的列表,用于存储查询条件。
`CommentExample`类提供了多种方法来设置和获取这些属性,并提供了`or()`和`createCriteria()`方法用于构建查询条件。
`Criteria`类是一个内部类,用于存储查询条件。它包含了一个`condition`字符串(表示查询条件)、一个`value`对象(表示查询条件中的值)、一个`secondValue`对象(表示在`between`查询中的第二个值)、以及一个`typeHandler`字符串(表示值的类型处理器)。
此外,`Criteria`类还包含了一些静态方法,如`andIdIsNull()`、`andIdEqualTo(Long value)`等,这些方法用于创建特定的查询条件。
整体来说,这段代码是一个用于构建查询评论数据的标准类,它可以通过添加多个`Criteria`对象来构建复杂的查询条件。
*/
package com.xw.community.model;
import java.util.ArrayList;
import java.util.List;
public class CommentExample {
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table comment
*
* @mbg.generated Sat Mar 28 18:06:26 CST 2020
*/
protected String orderByClause;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table comment
*
* @mbg.generated Sat Mar 28 18:06:26 CST 2020
*/
protected boolean distinct;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table comment
*
* @mbg.generated Sat Mar 28 18:06:26 CST 2020
*/
protected List<Criteria> oredCriteria;
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table comment
*
* @mbg.generated Sat Mar 28 18:06:26 CST 2020
*/
public CommentExample() {
oredCriteria = new ArrayList<>();
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table comment
*
* @mbg.generated Sat Mar 28 18:06:26 CST 2020
*/
public void setOrderByClause(String orderByClause) {
this.orderByClause = orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table comment
*
* @mbg.generated Sat Mar 28 18:06:26 CST 2020
*/
public String getOrderByClause() {
return orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table comment
*
* @mbg.generated Sat Mar 28 18:06:26 CST 2020
*/
public void setDistinct(boolean distinct) {
this.distinct = distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table comment
*
* @mbg.generated Sat Mar 28 18:06:26 CST 2020
*/
public boolean isDistinct() {
return distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table comment
*
* @mbg.generated Sat Mar 28 18:06:26 CST 2020
*/
public List<Criteria> getOredCriteria() {
return oredCriteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table comment
*
* @mbg.generated Sat Mar 28 18:06:26 CST 2020
*/
public void or(Criteria criteria) {
oredCriteria.add(criteria);
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table comment
*
* @mbg.generated Sat Mar 28 18:06:26 CST 2020
*/
public Criteria or() {
Criteria criteria = createCriteriaInternal();
oredCriteria.add(criteria);
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table comment
*
* @mbg.generated Sat Mar 28 18:06:26 CST 2020
*/
public Criteria createCriteria() {
Criteria criteria = createCriteriaInternal();
if (oredCriteria.size() == 0) {
oredCriteria.add(criteria);
}
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table comment
*
* @mbg.generated Sat Mar 28 18:06:26 CST 2020
*/
protected Criteria createCriteriaInternal() {
Criteria criteria = new Criteria();
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table comment
*
* @mbg.generated Sat Mar 28 18:06:26 CST 2020
*/
public void clear() {
oredCriteria.clear();
orderByClause = null;
distinct = false;
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table comment
*
* @mbg.generated Sat Mar 28 18:06:26 CST 2020
*/
protected abstract static class GeneratedCriteria {
protected List<Criterion> criteria;
protected GeneratedCriteria() {
super();
criteria = new ArrayList<>();
}
public boolean isValid() {
return criteria.size() > 0;
}
public List<Criterion> getAllCriteria() {
return criteria;
}
public List<Criterion> getCriteria() {
return criteria;
}
protected void addCriterion(String condition) {
if (condition == null) {
throw new RuntimeException("Value for condition cannot be null");
}
criteria.add(new Criterion(condition));
}
protected void addCriterion(String condition, Object value, String property) {
if (value == null) {
throw new RuntimeException("Value for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value));
}
protected void addCriterion(String condition, Object value1, Object value2, String property) {
if (value1 == null || value2 == null) {
throw new RuntimeException("Between values for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value1, value2));
}
public Criteria andIdIsNull() {
addCriterion("id is null");
return (Criteria) this;
}
public Criteria andIdIsNotNull() {
addCriterion("id is not null");
return (Criteria) this;
}
public Criteria andIdEqualTo(Long value) {
addCriterion("id =", value, "id");
return (Criteria) this;
}
public Criteria andIdNotEqualTo(Long value) {
addCriterion("id <>", value, "id");
return (Criteria) this;
}
public Criteria andIdGreaterThan(Long value) {
addCriterion("id >", value, "id");
return (Criteria) this;
}
public Criteria andIdGreaterThanOrEqualTo(Long value) {
addCriterion("id >=", value, "id");
return (Criteria) this;
}
public Criteria andIdLessThan(Long value) {
addCriterion("id <", valu
没有合适的资源?快使用搜索试试~ 我知道了~
(源码)基于Spring Boot框架的问答社区系统.zip
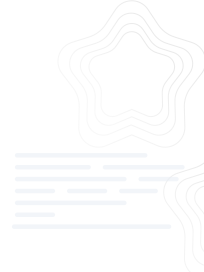
共485个文件
js:202个
html:107个
css:56个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 27 浏览量
2024-11-13
19:00:00
上传
评论
收藏 2.41MB ZIP 举报
温馨提示
# 基于Spring Boot框架的问答社区系统 ## 项目简介 本项目是一个基于Spring Boot框架开发的问答社区系统。用户可以通过Gitee的第三方登录功能进行登录,登录后可以进行提问、回答问题、搜索问题、查看个人提问和收到的回复等操作。系统支持Markdown编辑器,方便用户编辑和查看内容,并实现了图片上传功能。此外,系统还支持二级回复和未读通知提示功能。 ## 项目的主要特性和功能 1. 用户登录与授权 支持Gitee第三方登录。 用户登录后可以进行提问、回答和查看个人相关内容。 2. 提问与回答 用户可以添加和修改提问,提问内容支持Markdown格式。 用户可以回复问题,支持二级回复。 3. 搜索与查看 用户可以搜索问题。 用户可以查看个人提问和收到的回复。 4. 通知与提醒 用户收到的回复会有未读提示。 通知数量会在通知处显示。
资源推荐
资源详情
资源评论
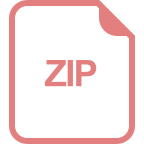
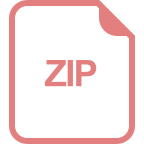
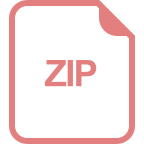
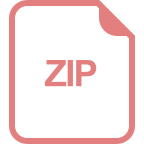
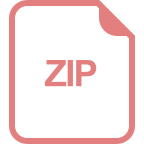
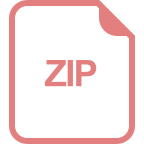
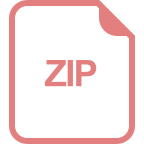
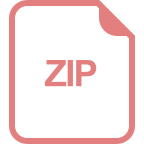
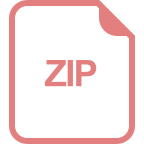
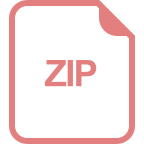
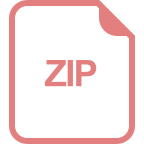
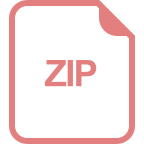
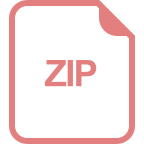
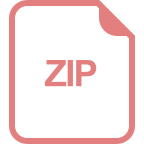
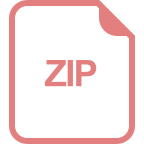
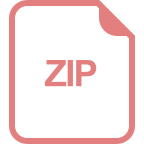
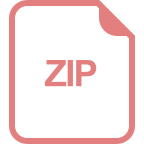
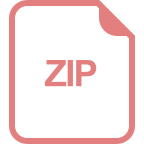
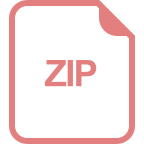
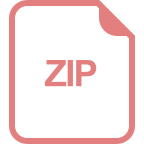
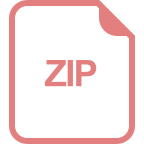
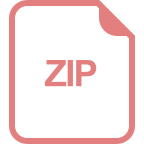
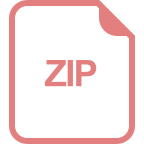
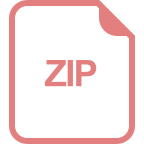
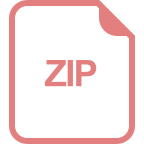
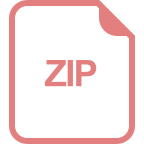
收起资源包目录

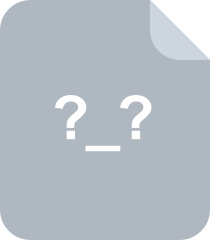
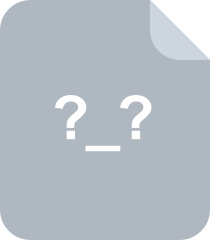
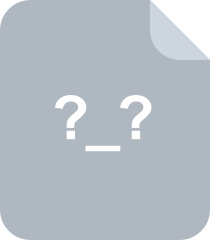
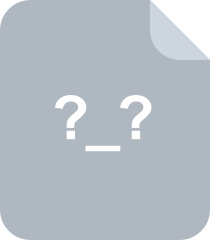
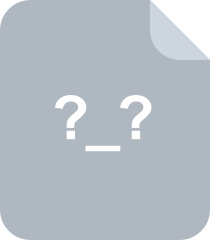
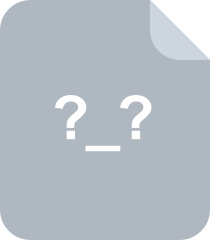
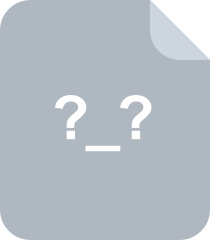
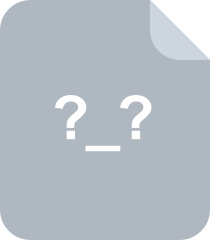
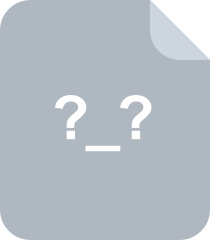
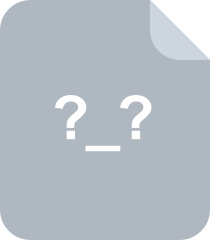
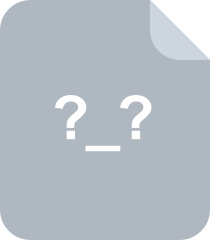
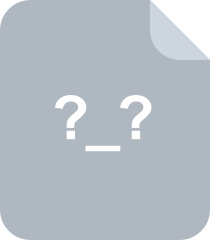
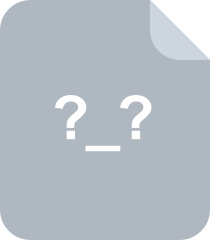
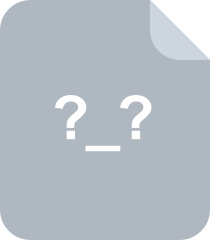
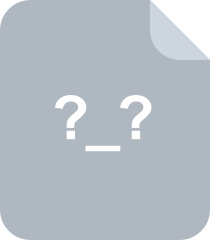
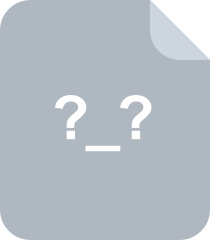
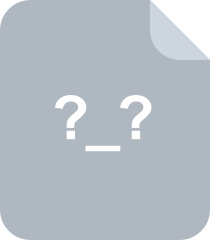
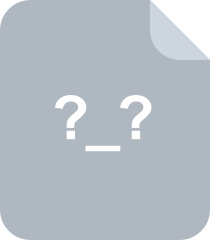
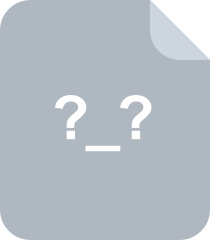
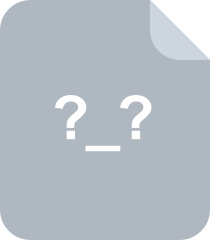
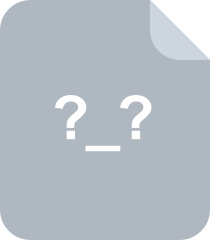
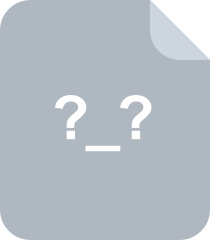
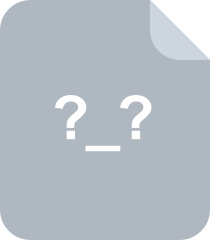
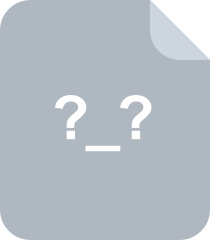
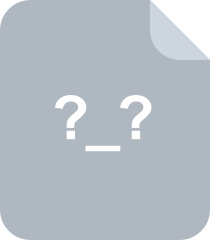
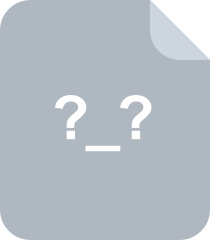
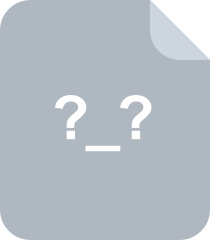
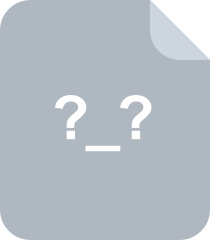
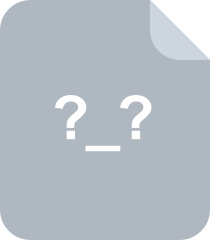
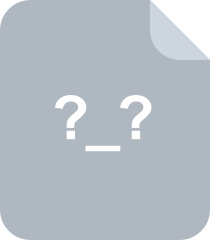
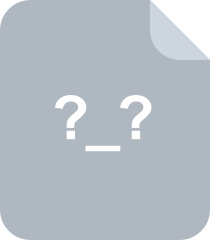
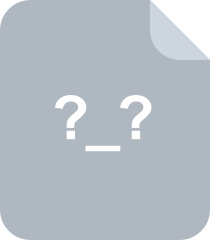
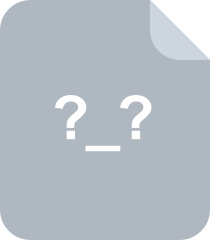
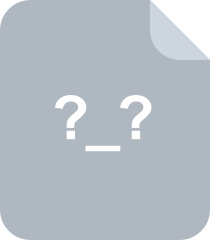
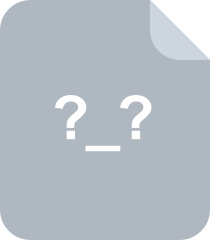
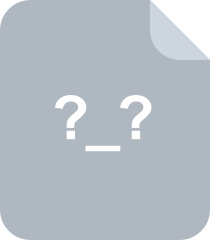
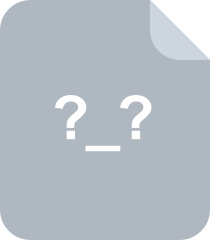
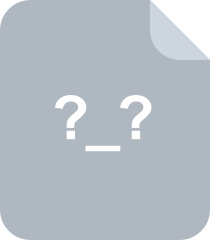
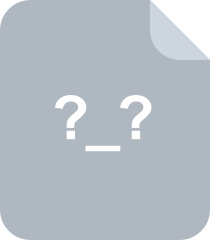
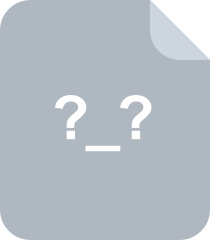
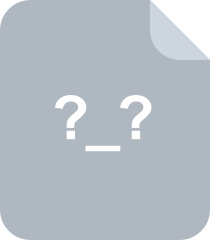
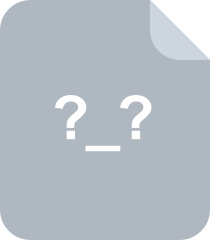
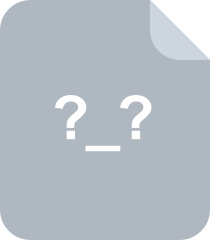
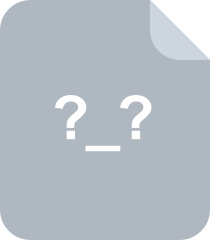
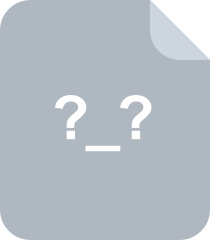
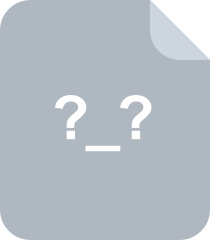
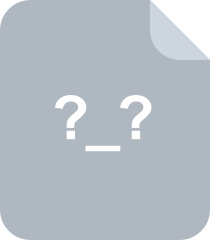
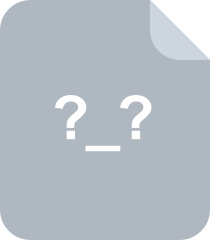
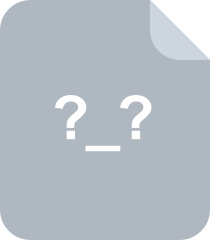
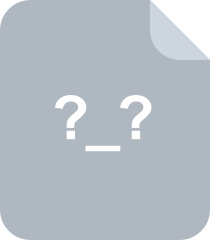
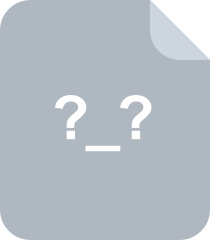
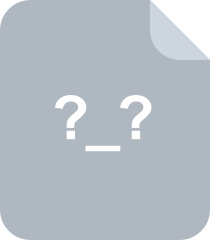
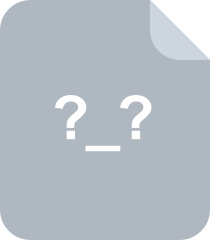
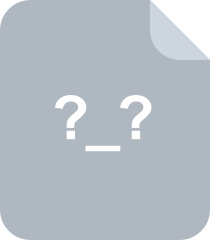
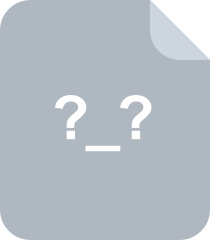
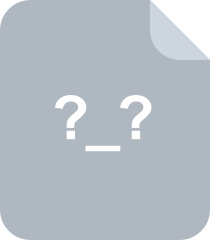
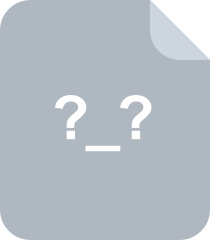
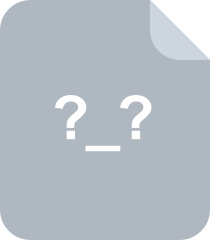
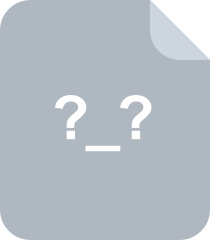
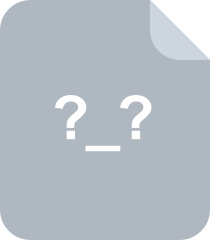
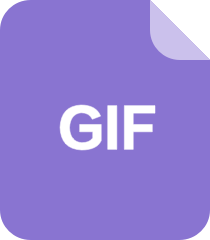
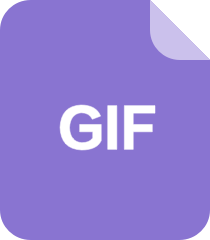
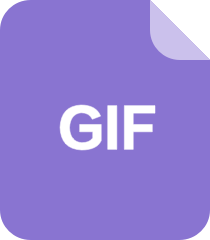
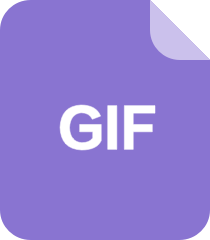
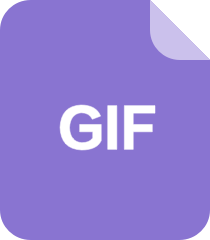
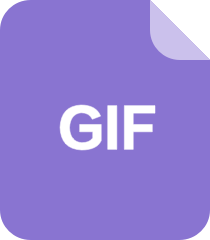
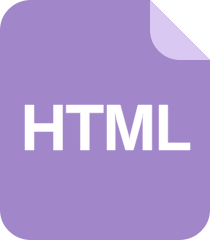
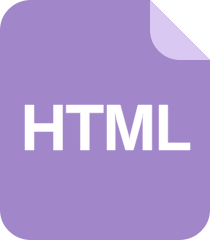
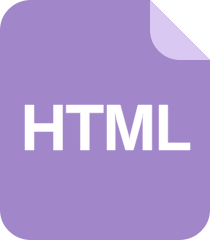
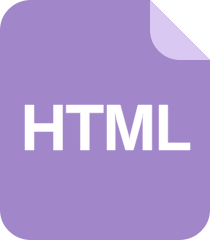
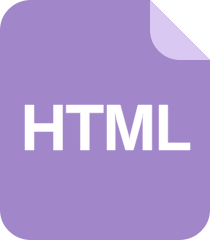
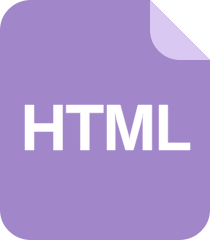
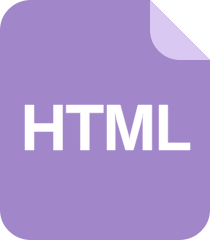
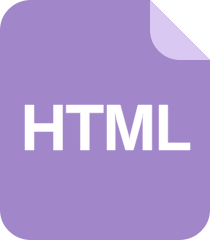
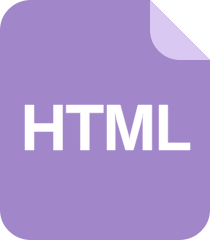
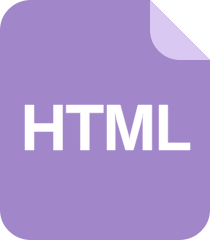
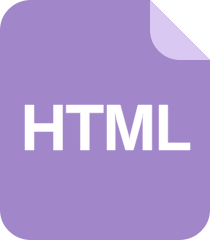
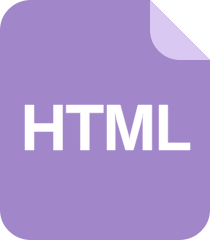
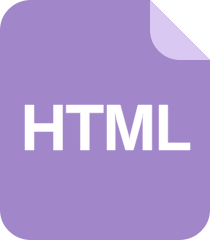
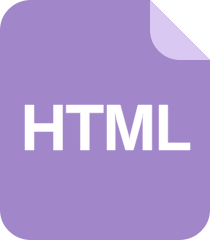
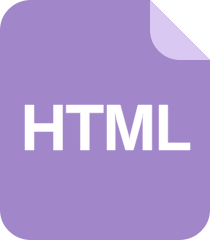
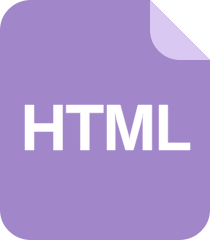
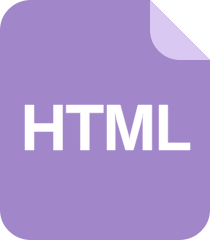
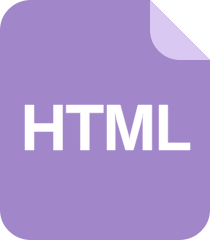
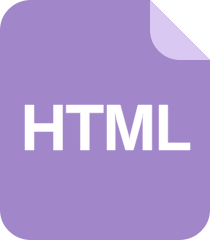
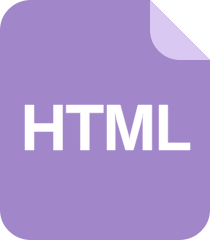
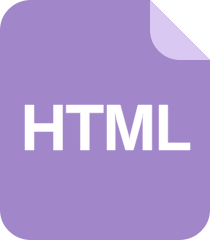
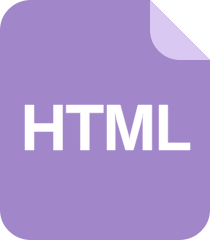
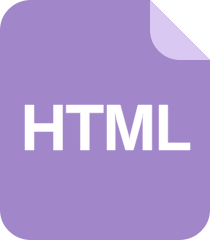
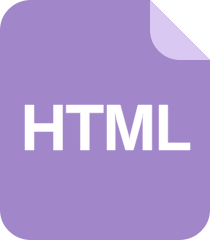
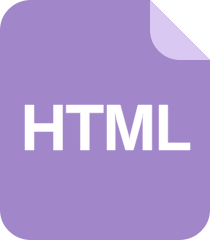
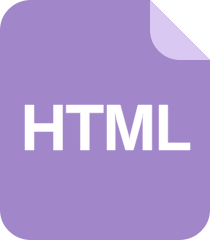
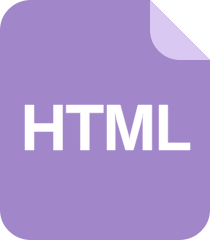
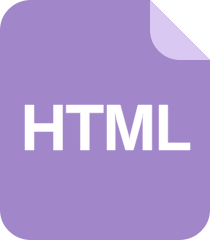
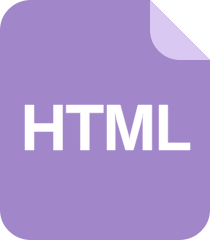
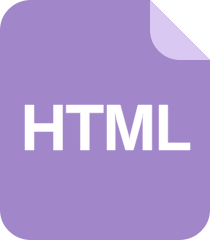
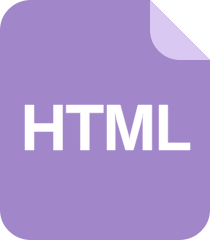
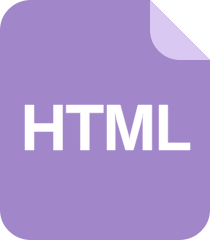
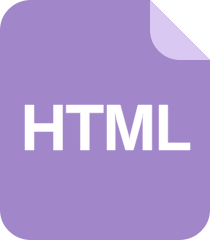
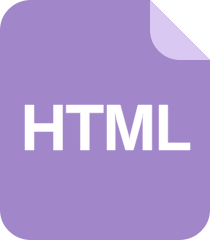
共 485 条
- 1
- 2
- 3
- 4
- 5
资源评论
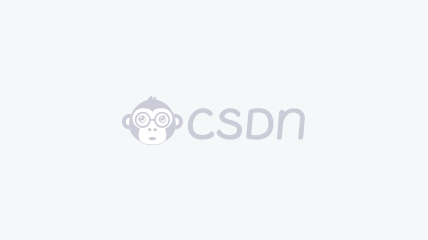

t0_54coder
- 粉丝: 3200
- 资源: 5642

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

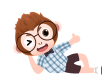
最新资源
- 2016中国社交媒体影响报告.pdf
- cq08m5kszc3d.pdf
- 2016中国数字营销行动报告.pdf
- 201601 - 凯络十大趋势报告 - 2016.pdf
- App Annie 全球移动应用市场 2015 年回顾.pdf
- Dive_Mobile-OMD.pdf
- IDC:2016全球数据中心预测.pdf
- go1.23.4.linux-amd64
- 山东春季高考C语言练习题目
- 基于单片机八人数字抢答器仿真、程序、设计报告 (1)抢答器同时供 8 名选手比赛,分别用8个按钮 s1-s8表示 (5)选手在设定的时间内进行抢答,抢答有效,定时器停止工作,蜂鸣器报警,数码管显示选手
- go1.23.4.src
- 水泵控制程序,跟随压力加减机,定时轮,故障自动投入,水泵相互备用 1.模式为0,先停泵,然后启动水泵 2.模式为1, 先启泵,然后在停泵 3.故障自动切水泵 4.当切泵时,启动运行时间最短的泵 5.当
- MATLAB界面版本- BP神经网络的火焰识别.zip
- 基于JAVA实现的五子棋人机对弈游戏
- MATLAB界面版本- GUI的水果识别.zip
- 5560m5nd6n7z.pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


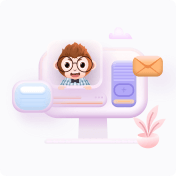
安全验证
文档复制为VIP权益,开通VIP直接复制
