# utest: Asynchronous C++ Test Harness
This test harness allows you to execute a specified series of (asynchronous) C++ test cases with sensible default reporting and useful customization options.
Please note that this is a purposefully lean test harness, only dealing with test execution and providing default reporting handlers. It specifically does not support auto-discovery of test cases and does not provide you with test macros or other convenience functions.
Instead, the macros in the [unity module](https://github.com/ARMmbed/mbed-os/tree/master/features/frameworks/unity) can be used for this purpose. However, you are not required to use these, and can use your own macros if you wish.
Furthermore, test failure recovery through the use of exceptions or `longjmp` is not supported; the test will either continue and ignore failures or die by busy-waiting.
## Theory of Operation
A test specification contains a setup handler, several test cases and a teardown handler.
Each test case contains a textual description, setup, teardown and failure handler as well as the actual test handler.
The order of handler execution is:
1. Test setup handler.
1. For each test case:
1. Test case setup handler.
1. Test case execution handler.
1. (wait for timeout or callback validation in case of an asynchronous test case.)
1. (repeat test case execution handler if specified.)
1. Test case teardown handler.
1. Test teardown handler.
## Example
The following example showcases a lot of functionality and proper integration with the [Greentea test tool](https://github.com/ARMmbed/mbed-os-tools/tree/master/packages/mbed-greentea), while making use of the [unity test macros](https://github.com/ARMmbed/mbed-os/tree/master/features/frameworks/unity):
```cpp
#include "mbed.h"
#include "greentea-client/test_env.h"
#include "utest/utest.h"
#include "unity/unity.h"
using namespace utest::v1;
void test_simple() {
TEST_ASSERT_EQUAL(0, 0);
printf("Simple test called\n");
}
status_t test_repeats_setup(const Case *const source, const size_t index_of_case) {
// Call the default handler for proper reporting
status_t status = greentea_case_setup_handler(source, index_of_case);
printf("Setting up for '%s'\n", source->get_description());
return status;
}
control_t test_repeats(const size_t call_count) {
printf("Called for the %u. time\n", call_count);
TEST_ASSERT_NOT_EQUAL(3, call_count);
// Specify how often this test is repeated ie. n total calls
return (call_count < 2) ? CaseRepeatAll : CaseNext;
}
void test_callback_validate() {
// You may also use assertions here!
TEST_ASSERT_EQUAL_PTR(0, 0);
// Validate the callback
Harness::validate_callback();
}
control_t test_asynchronous() {
TEST_ASSERT_TRUE_MESSAGE(true, "(true == false) o_O");
// Set up a callback in the future. This may also be an interrupt!
EventQueue *queue = mbed_event_queue();
queue->call_in(100, test_callback_validate);
// Set a 200ms timeout starting from now
return CaseTimeout(200);
}
control_t test_asynchronous_timeout(const size_t call_count) {
TEST_ASSERT_TRUE_MESSAGE(true, "(true == false) o_O");
// Set a 200ms timeout starting from now,
// but automatically repeat only this handler on timeout.
if (call_count >= 5) {
// but after the 5th call, the callback finally gets validated
EventQueue *queue = mbed_event_queue();
queue->call_in(100, test_callback_validate);
}
return CaseRepeatHandlerOnTimeout(200);
}
// Custom setup handler required for proper Greentea support
status_t greentea_setup(const size_t number_of_cases) {
GREENTEA_SETUP(20, "default_auto");
// Call the default reporting function
return greentea_test_setup_handler(number_of_cases);
}
// Specify all your test cases here
Case cases[] = {
Case("Simple Test", test_simple),
Case("Repeating Test", test_repeats_setup, test_repeats),
Case("Asynchronous Test (200ms timeout)", test_asynchronous),
Case("Asynchronous Timeout Repeat", test_asynchronous_timeout)
};
// Declare your test specification with a custom setup handler
Specification specification(greentea_setup, cases);
int main()
{ // Run the test specification
Harness::run(specification);
}
```
Running this test will output the following:
```
{{timeout;20}}
{{host_test_name;default_auto}}
{{description;utest greentea example}}
{{test_id;MBED_OS}}
{{start}}
>>> Running 4 test cases...
>>> Running case #1: 'Simple Test'...
Simple test called
>>> 'Simple Test': 1 passed, 0 failed
>>> Running case #2: 'Repeating Test'...
Setting up for 'Repeating Test'
Called for the 1. time
>>> 'Repeating Test': 1 passed, 0 failed
>>> Running case #2: 'Repeating Test'...
Setting up for 'Repeating Test'
Called for the 2. time
>>> 'Repeating Test': 2 passed, 0 failed
>>> Running case #3: 'Asynchronous Test (200ms timeout)'...
>>> 'Asynchronous Test (200ms timeout)': 1 passed, 0 failed
>>> Running case #4: 'Asynchronous Timeout Repeat'...
>>> failure with reason 'Ignored: Timed Out'
>>> failure with reason 'Ignored: Timed Out'
>>> failure with reason 'Ignored: Timed Out'
>>> failure with reason 'Ignored: Timed Out'
>>> failure with reason 'Ignored: Timed Out'
>>> 'Asynchronous Timeout Repeat': 1 passed, 0 failed
>>> Test cases: 4 passed, 0 failed
{{success}}
{{end}}
```
## Detailed Description
### Handlers
There are five handler types you can, but do not have to, override to customize operation.
Please see the `utest/types.h` file for a detailed description.
1. `status_t test_setup_handler_t(const size_t number_of_cases)`: called before execution of any test case.
1. `void test_teardown_handler_t(const size_t passed, const size_t failed, const failure_t failure)`: called after execution of all test cases, and if testing is aborted.
1. `void test_failure_handler_t(const failure_t failure)`: called whenever a failure occurs anywhere in the specification.
1. `status_t case_setup_handler_t(const Case *const source, const size_t index_of_case)`: called before execution of each test case.
1. `status_t case_teardown_handler_t(const Case *const source, const size_t passed, const size_t failed, const failure_t reason)`: called after execution of each test case, and if testing is aborted.
1. `status_t case_failure_handler_t(const Case *const source, const failure_t reason)`: called whenever a failure occurs during the execution of a test case.
All handlers are defaulted for integration with the [Greentea testing tool](https://github.com/ARMmbed/mbed-os-tools/tree/master/packages/mbed-greentea).
### Test Case Handlers
There are three test case handlers:
1. `void case_handler_t(void)`: executes once, if the case setup succeeded.
1. `control_t case_control_handler_t(void)`: executes (asynchronously) as many times as you specify, if the case setup succeeded.
1. `control_t case_call_count_handler_t(const size_t call_count)`: executes (asynchronously) as many times as you specify, if the case setup succeeded.
To specify a test case you must wrap it into a `Case` class: `Case("mandatory description", case_handler)`. You may override the setup, teardown and failure handlers in this wrapper class as well.
The `Case` constructor is overloaded to allow you a comfortable declaration of all your callbacks and the order of arguments is:
1. Description (required).
1. Setup handler (optional).
1. Test case handler (required).
1. Teardown handler (optional).
1. Failure handler (optional).
#### Test Case Attributes
You can modify test case behavior by returning `control_t` modifiers:
- `CaseNext`: never repeats and immediately moves to next test case
- `CaseNoRepeat`: never repeats.
- `CaseRepeatAll`: repeats test case **with** setup and teardown handlers.
没有合适的资源?快使用搜索试试~ 我知道了~
(源码)基于mbed OS和utest库的嵌入式系统HAL测试框架.zip
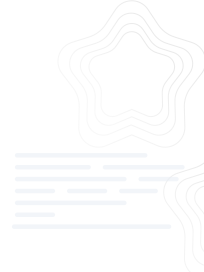
共342个文件
h:97个
cpp:67个
txt:53个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 109 浏览量
2024-11-13
13:58:07
上传
评论
收藏 1.03MB ZIP 举报
温馨提示
# 基于mbed OS和utest库的嵌入式系统HAL测试框架 ## 项目简介 本项目是一个用于测试嵌入式系统硬件抽象层(HAL)及其相关功能的单元测试框架。该框架基于mbed OS和utest库,旨在验证HAL中的不同模块和功能,如GPIO、ADC、PWM、UART、I2C和SPI等。通过编写和运行一系列的测试用例,可以确保HAL的正确性和性能。 ## 项目的主要特性和功能 1. 测试环境设置通过greenteasetup函数初始化测试环境,包括硬件平台、串行通信和其他必要的资源。 2. 测试用例定义使用utest框架定义各种测试用例,每个用例都对应HAL中的一个特定功能。测试用例通常包括设置、执行和清理过程,使用断言来验证预期结果。 3. 测试执行在main函数中,通过Harness::run(specification)运行所有的测试用例。测试规格(Specification)对象包含了测试用例列表、测试环境的设置和清理函数。
资源推荐
资源详情
资源评论
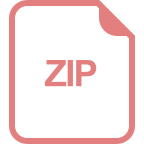
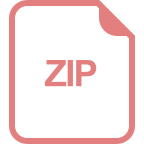
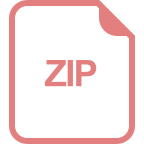
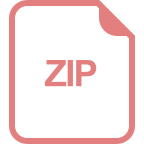
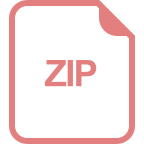
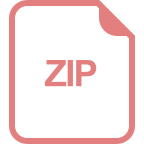
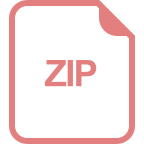
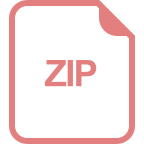
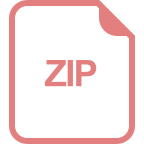
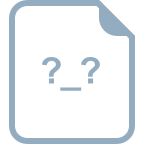
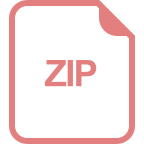
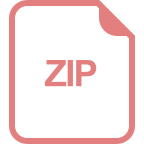
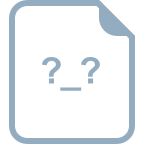
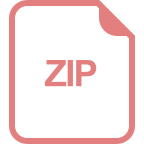
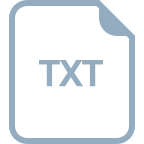
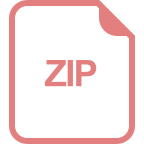
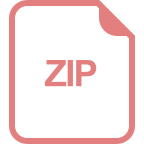
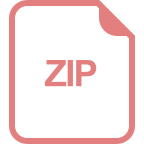
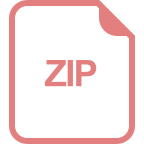
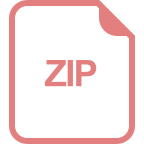
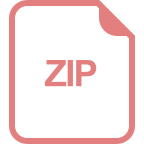
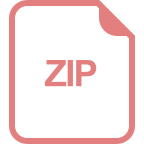
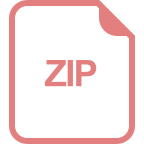
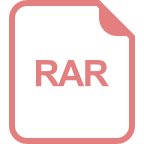
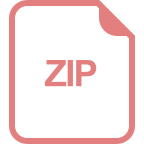
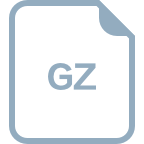
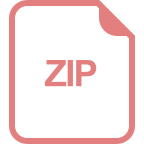
收起资源包目录

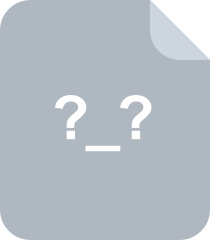
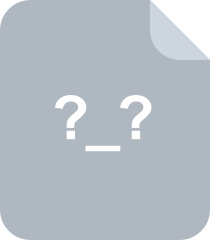
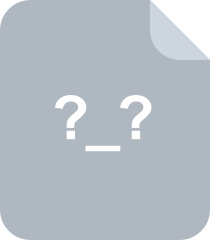
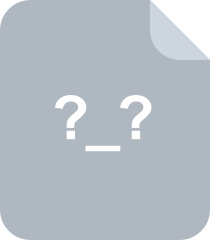
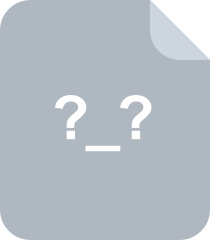
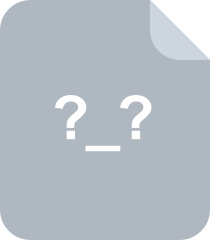
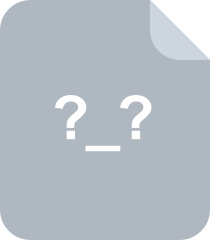
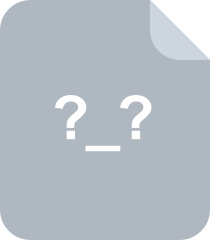
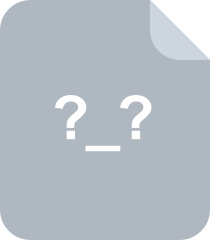
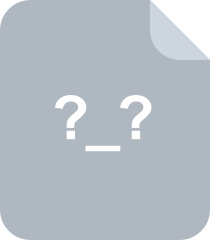
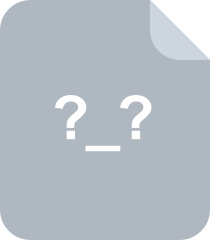
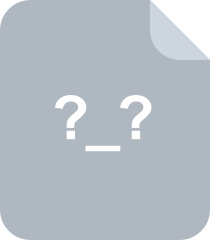
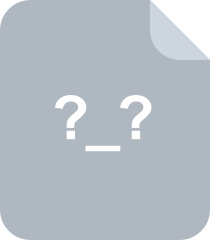
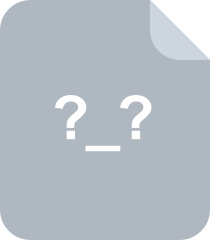
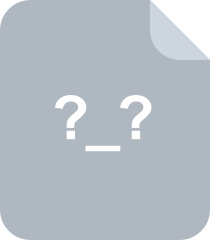
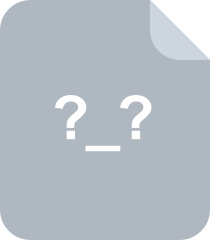
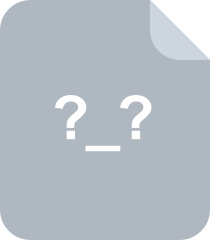
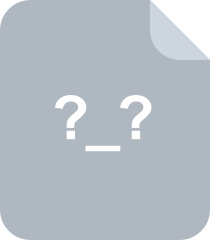
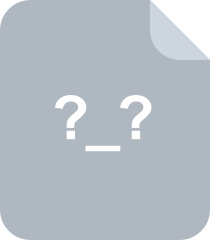
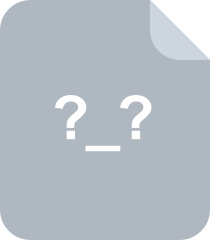
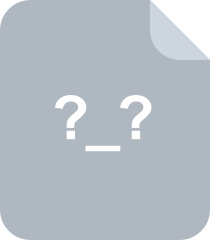
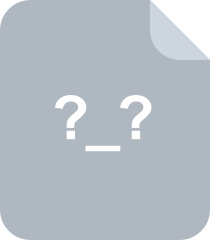
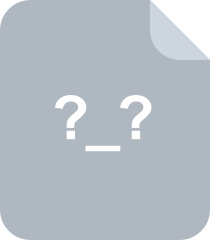
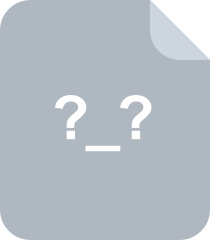
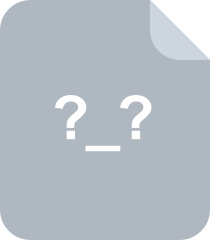
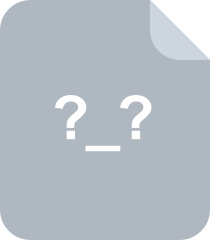
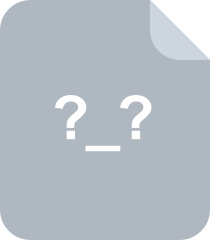
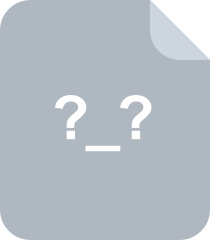
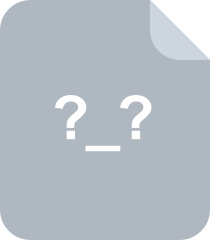
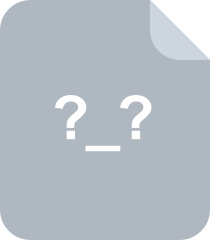
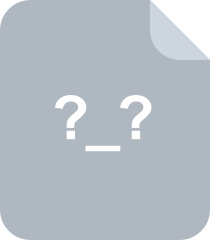
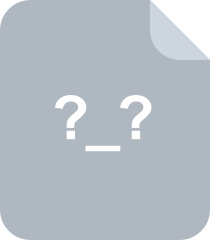
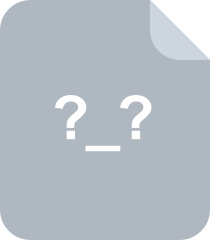
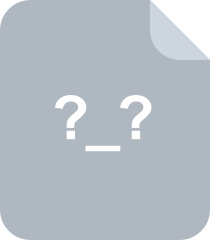
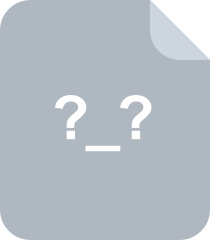
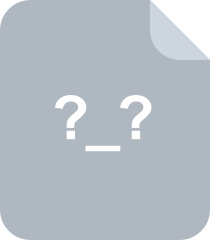
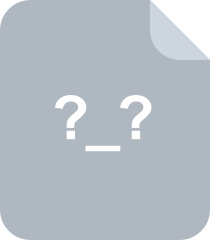
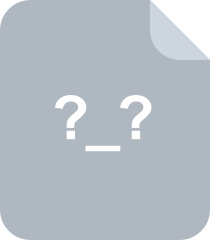
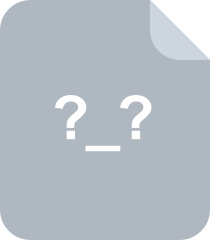
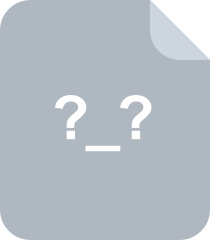
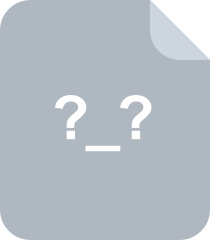
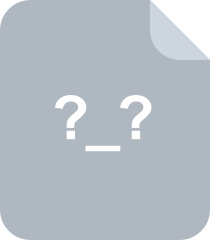
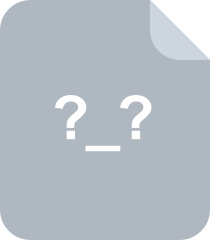
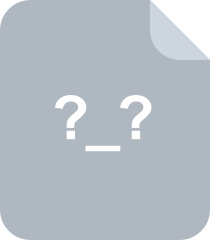
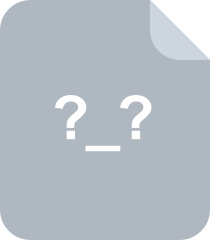
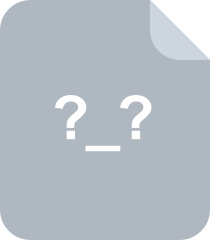
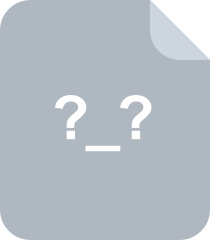
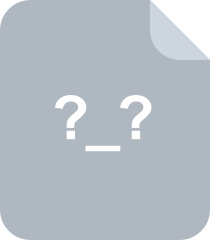
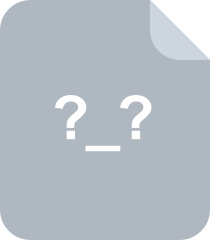
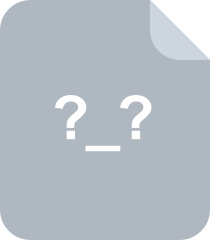
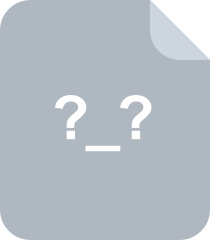
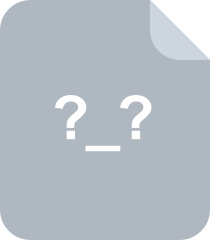
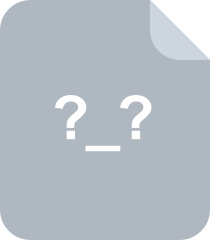
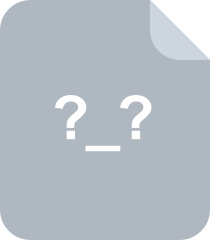
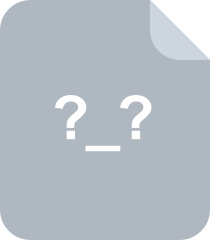
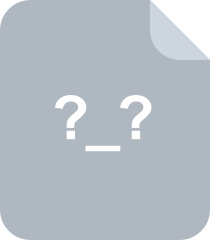
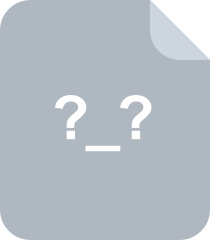
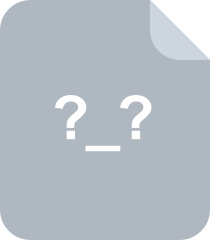
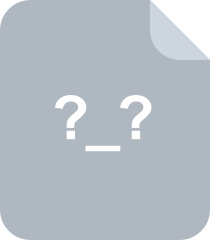
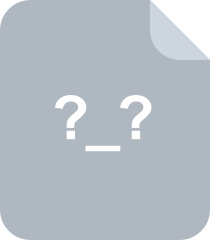
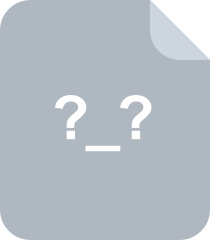
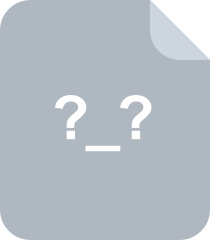
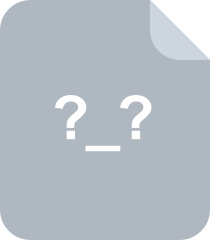
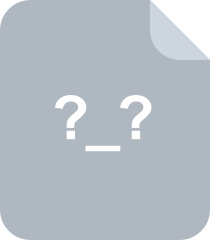
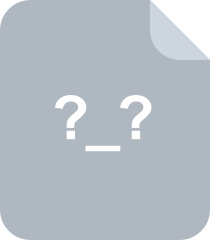
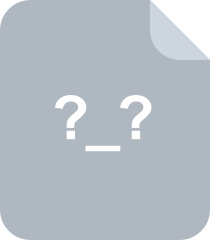
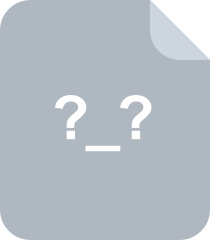
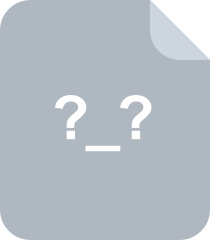
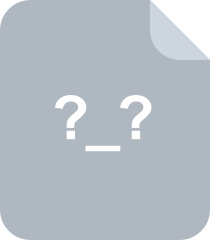
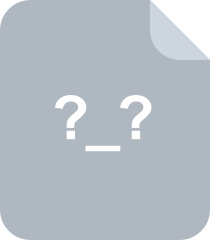
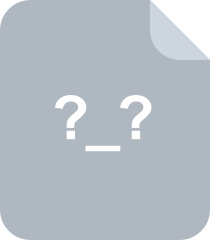
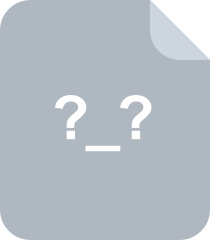
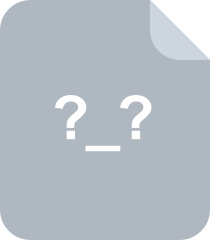
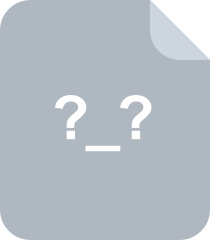
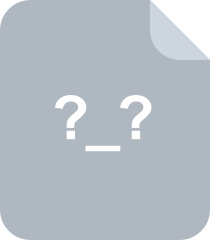
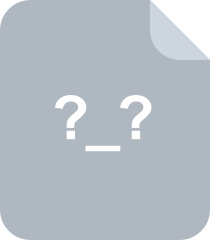
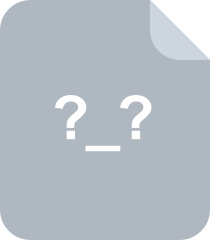
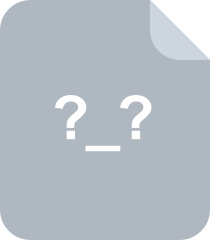
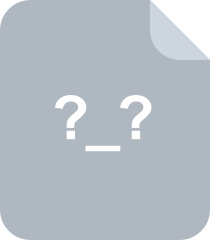
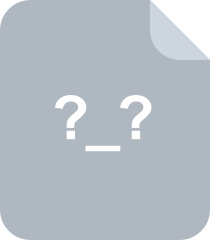
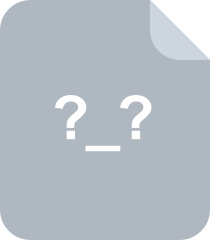
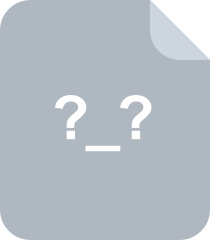
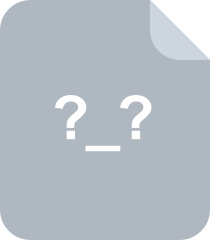
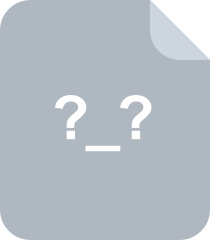
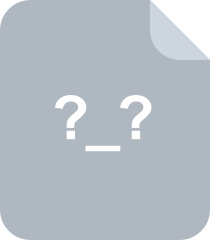
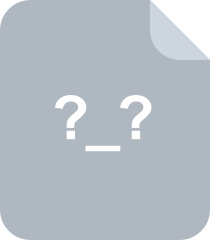
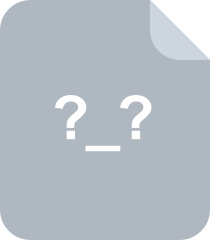
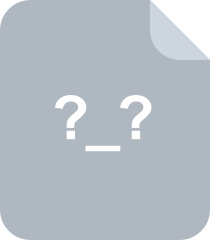
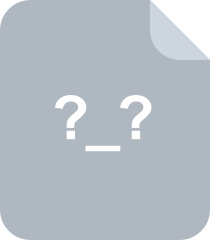
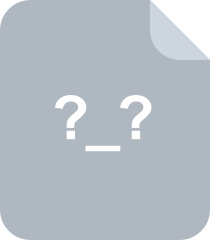
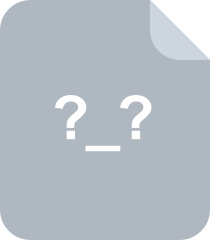
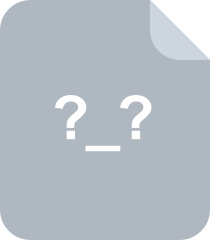
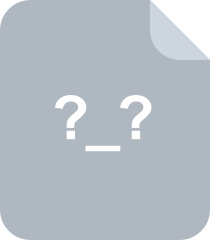
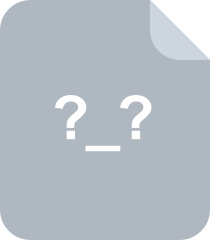
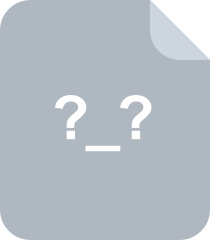
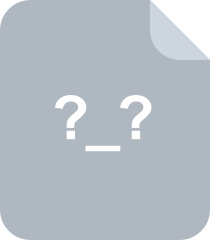
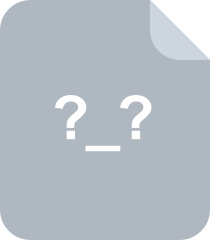
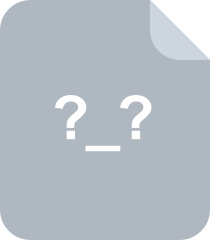
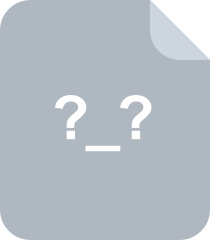
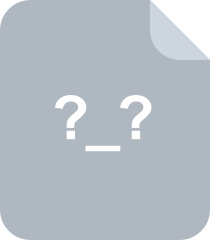
共 342 条
- 1
- 2
- 3
- 4
资源评论
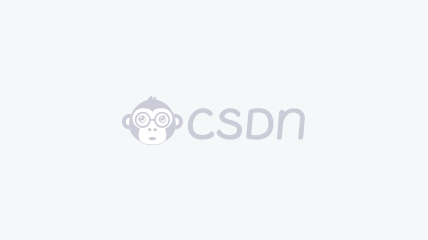

t0_54coder
- 粉丝: 3148
- 资源: 5642

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

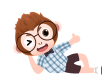
最新资源
- 电子学习资料设计作品全资料数字温度计资料
- 基于Django框架的IT资产维修管理系统设计源码
- 基于SpringBoot与Vue框架的宿舍管理系统设计源码
- 基于C#语言的预警监测系统设计源码
- yolo将xml文件转换为txt文件
- 电子学习资料设计作品全资料水库控制系统资料
- 基于PHP+MySQL架构的FoxCMS黔狐内容管理系统设计源码
- 基于Go语言的MySQL Binlog Exporter设计源码,实现Prometheus监控MySQL Binlog事件及主从同步监控
- 电子学习资料设计作品全资料同步电机模型的MATLAB仿真资料
- 基于Go语言的校园云互助跑腿小程序设计源码
- 基于SSM框架的JSP/Java精品课程在线学习系统设计源码
- 电子学习资料设计作品全资料危险气体泄露报警器设计资料
- 基于Vue2+Vant2与Vue2+Element Ui的宠物寄养平台前后台管理系统设计源码
- 基于Vue框架的居家上门服务系统移动端设计源码
- 基于Vue3与Node的SSR旅游住宿信息服务平台设计源码-爱此迎
- 卧式摆线螺杆机带仿真视频sw19可编辑全套技术资料100%好用.zip.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


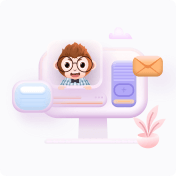
安全验证
文档复制为VIP权益,开通VIP直接复制
