/*
This code is a Java Server Pages (JSP) scriptlet that generates HTML output to display the schedule of courses for students. The key points about this code are:
1. **Source Code is from**: `.\jw-project\target\omcat\work\Tomcat\localhost\_org\.apache\.jsp.WEB-INF\pages\student\schedule.jsp`
2. **Scriptlet Utilizes**:
- **Taglibs** (`<c:forEach>`, `<c:if>`), which allow iteration and conditional rendering of content.
- **JSP Expression Language (EL)**, used to dynamically evaluate expressions in the JSP page.
3. **Key Components**:
- **`<c:forEach>`**: Used extensively for iterating over a list (`allCourse`) of course details (`c.course`). The iteration is done across different parts of the HTML template to customize sections based on course details.
- **Conditional Rendering** (`<c:if>`): Determines what content is displayed or hidden depending on specific conditions related to course section IDs.
4. **HTML and JS Output**:
This code outputs dynamic HTML that includes:
- Course name, week section number, teacher's name, classroom information for each course.
- Schedule display is organized into rows representing days of the week and columns representing time slots (morning, afternoon, evening).
5. **Customization Based on Conditions**:
The output changes based on conditions specified through `<c:if>` tags, which conditionally display or hide certain parts of the schedule for specific courses.
6. **Printing Functions**:
- A JavaScript function `print()` is included to trigger printing action when requested.
- There are also functions like `funFD` that manage a feature possibly related to expanding and collapsing details about individual courses.
7. **HTML Formatting**:
- The HTML output includes custom CSS styles for formatting the schedule table appearance, such as cell alignments (`font-size`, `text-color`, etc.).
In summary, this JSP code dynamically generates an HTML representation of course schedules based on data bound to variables like `allCourse`. It's organized into a grid-like structure that allows for customization and display of individual courses' details according to specific criteria.
*/
package org.apache.jsp.WEB_002dINF.pages.student;
import javax.servlet.*;
import javax.servlet.http.*;
import javax.servlet.jsp.*;
public final class schedule_jsp extends org.apache.jasper.runtime.HttpJspBase
implements org.apache.jasper.runtime.JspSourceDependent {
private static final javax.servlet.jsp.JspFactory _jspxFactory =
javax.servlet.jsp.JspFactory.getDefaultFactory();
private static java.util.Map<java.lang.String,java.lang.Long> _jspx_dependants;
private org.apache.jasper.runtime.TagHandlerPool _005fjspx_005ftagPool_005fc_005fforEach_0026_005fvar_005fitems;
private org.apache.jasper.runtime.TagHandlerPool _005fjspx_005ftagPool_005fc_005fif_0026_005ftest;
private javax.el.ExpressionFactory _el_expressionfactory;
private org.apache.tomcat.InstanceManager _jsp_instancemanager;
public java.util.Map<java.lang.String,java.lang.Long> getDependants() {
return _jspx_dependants;
}
public void _jspInit() {
_005fjspx_005ftagPool_005fc_005fforEach_0026_005fvar_005fitems = org.apache.jasper.runtime.TagHandlerPool.getTagHandlerPool(getServletConfig());
_005fjspx_005ftagPool_005fc_005fif_0026_005ftest = org.apache.jasper.runtime.TagHandlerPool.getTagHandlerPool(getServletConfig());
_el_expressionfactory = _jspxFactory.getJspApplicationContext(getServletConfig().getServletContext()).getExpressionFactory();
_jsp_instancemanager = org.apache.jasper.runtime.InstanceManagerFactory.getInstanceManager(getServletConfig());
}
public void _jspDestroy() {
_005fjspx_005ftagPool_005fc_005fforEach_0026_005fvar_005fitems.release();
_005fjspx_005ftagPool_005fc_005fif_0026_005ftest.release();
}
public void _jspService(final javax.servlet.http.HttpServletRequest request, final javax.servlet.http.HttpServletResponse response)
throws java.io.IOException, javax.servlet.ServletException {
final javax.servlet.jsp.PageContext pageContext;
javax.servlet.http.HttpSession session = null;
final javax.servlet.ServletContext application;
final javax.servlet.ServletConfig config;
javax.servlet.jsp.JspWriter out = null;
final java.lang.Object page = this;
javax.servlet.jsp.JspWriter _jspx_out = null;
javax.servlet.jsp.PageContext _jspx_page_context = null;
try {
response.setContentType("text/html;charset=UTF-8");
pageContext = _jspxFactory.getPageContext(this, request, response,
null, true, 8192, true);
_jspx_page_context = pageContext;
application = pageContext.getServletContext();
config = pageContext.getServletConfig();
session = pageContext.getSession();
out = pageContext.getOut();
_jspx_out = out;
out.write("\r\n");
out.write("\r\n");
out.write("<html>\r\n");
out.write("<head id=\"headerid1\">\r\n");
out.write("<title>学期理论课表</title>\r\n");
out.write("</head>\r\n");
out.write("<style type=\"text/css\">\r\n");
out.write("body {\r\n");
out.write("\ttext-align: center;\r\n");
out.write("}\r\n");
out.write("\r\n");
out.write("tr, td {\r\n");
out.write("\tfont-size: 12px;\r\n");
out.write("\tcolor: #000000;\r\n");
out.write("\tbackground: #ffffff\r\n");
out.write("}\r\n");
out.write("</style>\r\n");
out.write("<iframe id=\"notSession\" name=\"notSession\" style=\"display: none;\" src=\"\"></iframe>\r\n");
out.write("<script type=\"text/javascript\">\r\n");
out.write("\tjQuery(document)\r\n");
out.write("\t\t\t.ready(\r\n");
out.write("\t\t\t\t\tfunction() {\r\n");
out.write("\t\t\t\t\t\twindow\r\n");
out.write("\t\t\t\t\t\t\t\t.setInterval(\r\n");
out.write("\t\t\t\t\t\t\t\t\t\tfunction() {\r\n");
out.write("\t\t\t\t\t\t\t\t\t\t\tdocument\r\n");
out.write("\t\t\t\t\t\t\t\t\t\t\t\t\t.getElementById(\"notSession\").src = \"/jsxsd/framework/blankPage.jsp\";\r\n");
out.write("\t\t\t\t\t\t\t\t\t\t}, 1000 * 60 * 10);\r\n");
out.write("\t\t\t\t\t});\r\n");
out.write("</script>\r\n");
out.write("<body>\r\n");
out.write("\t<div class=\"Nsb_pw\" style=\"font-size: 5px\">\r\n");
out.write("\t\t<iframe style=\"display: none;\" name=\"ifrmPrint\" id=\"ifrmPrint\"></iframe>\r\n");
out.write("\t\t<form action=\"\" name=\"FormPrint\" id=\"FormPrint\" method=\"post\"\r\n");
out.write("\t\t\ttarget=\"ifrmPrint\">\r\n");
out.write("\t\t\t<table id=\"kbtable\" border=\"1\" width=\"100%\" cellspacing=\"0\"\r\n");
out.write("\t\t\t\tcellpadding=\"0\">\r\n");
out.write("\t\t\t\t<tr>\r\n");
out.write("\t\t\t\t\t<td align=\"center\" colspan=\"2\" width=\"1%\" height=\"25px\">时间</td>\r\n");
out.write("\t\t\t\t\t<td width=\"17%\" align=\"center\">星期一</td>\r\n");
out.write("\t\t\t\t\t<td width=\"123\" height=\"10\" align=\"center\">星期二</td>\r\n");
out.write("\t\t\t\t\t<td width=\"123\" height=\"10\" align=\"center\">星期三</td>\r\n");
out.write("\t\t\t\t\t<td width=\"124\" height=\"10\" align=\"center\">星期四</td>\r\n");
out.write("\t\t\t\t\t<td width=\"124\" height=\"10\" align=\"center\">星期五</td>\r\n");
out.write("\t\t\t\t\t<td width=\"124\" height=\"10\" align=\"center\">星期六</td>\r\n");
out.write("\t\t\t\t\t<td width=\"124\" height=\"10\" align=\"center\">星期日</td>\r\n");
out.write("\t\t\t\t</tr>\r\n");
out.write("\t\t\t\t<tr>\r\n");
out.write("\t\t\t\t\t<td align=\"center\" colspan=\"2\" width=\"1%\" height=\"20px\">早晨</td>\r\n");
out.write("\t\t\t\t\t<td width=\"123\" height=\"10\" align=\"center\"></td>\r\n");
out.
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
# 基于Spring Boot的教务管理系统 ## 项目概述 本项目是一个基于Spring Boot框架的教务管理系统,旨在提供一个高效、易用的平台来管理学生、教师、课程、成绩等教务相关信息。系统集成了多种功能,包括用户管理、课程管理、成绩管理、权限管理等,以满足不同用户的需求。 ## 主要功能 ### 用户管理 - **学生管理**:添加、删除、查询学生信息,包括学号、姓名、性别等。 - **教师管理**:管理教师信息,包括教师编号、姓名、所属学院等。 - **角色管理**:定义不同角色(如管理员、教师、学生)及其权限。 ### 课程管理 - **课程信息管理**:添加、编辑、删除课程信息,包括课程名称、学分、上课时间等。 - **课程安排**:为学生和教师安排课程,支持分页查询和批量操作。 ### 成绩管理 - **成绩录入**:教师可以录入学生成绩,支持批量导入和导出。 - **成绩查询**:学生和教师可以查询成绩,支持按课程、学期等条件筛选。 ### 权限管理 - **权限分配**:为不同角色分配权限,确保系统的安全性和稳定性。 - **权限验证**:在系统操作中
资源推荐
资源详情
资源评论
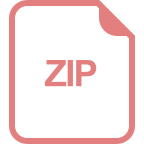
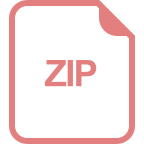
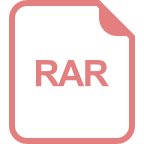
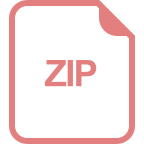
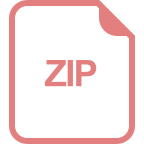
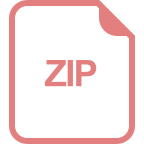
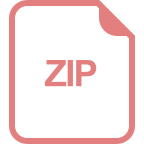
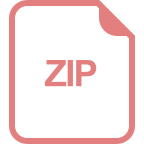
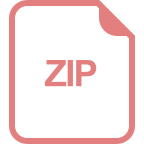
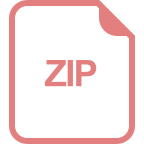
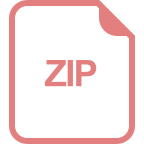
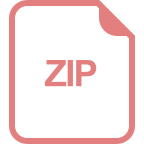
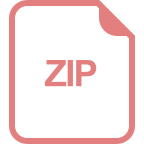
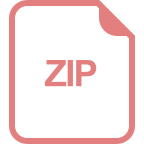
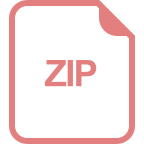
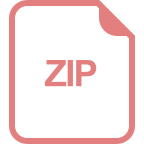
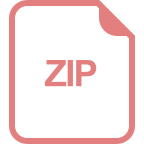
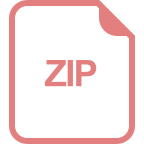
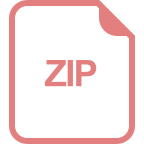
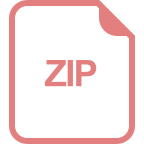
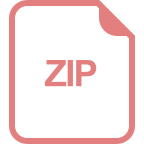
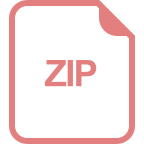
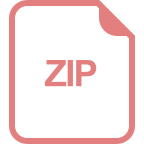
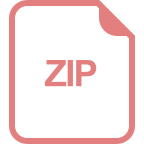
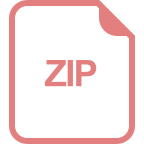
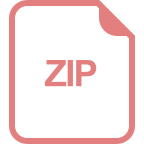
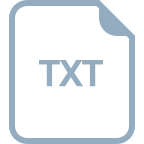
收起资源包目录

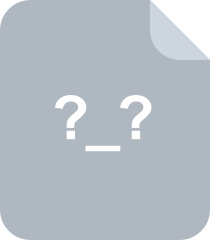
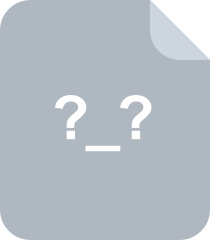
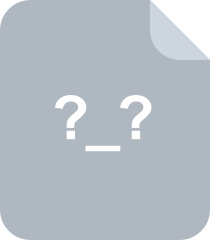
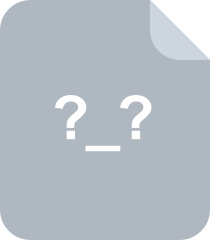
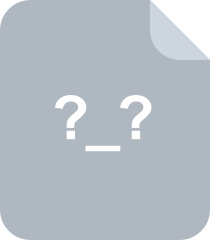
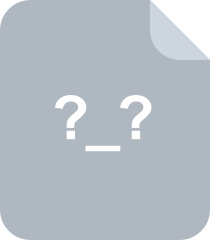
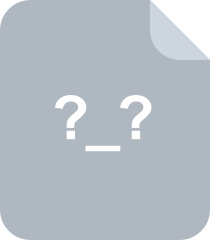
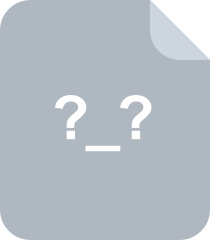
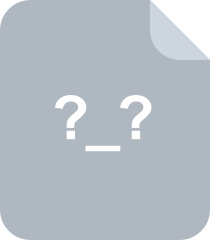
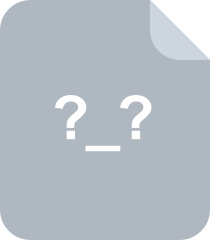
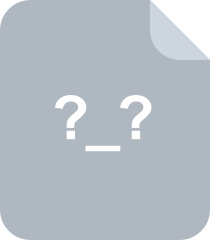
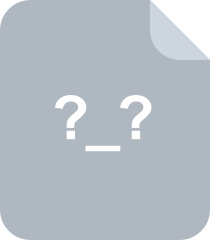
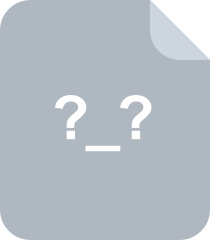
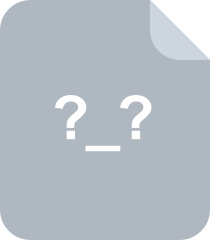
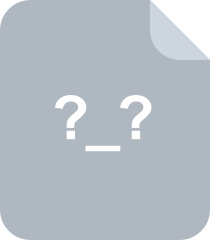
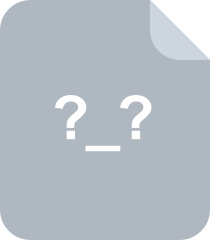
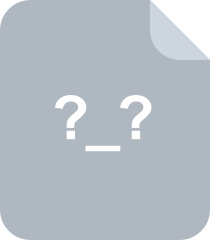
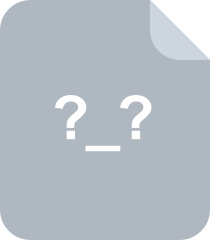
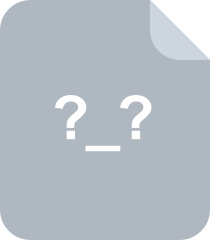
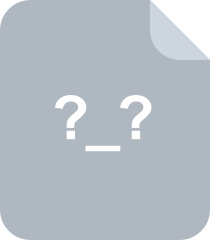
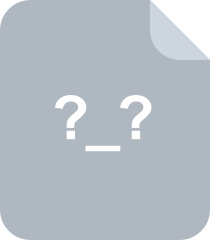
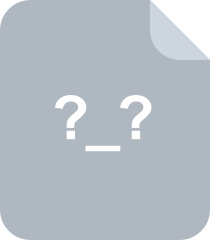
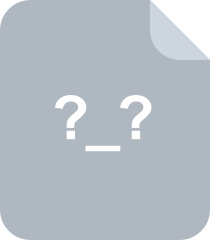
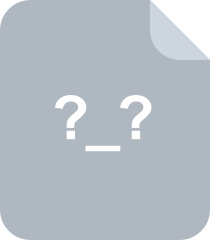
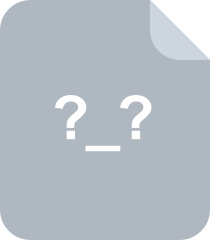
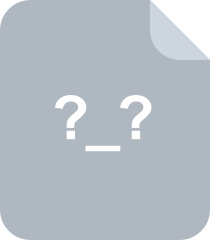
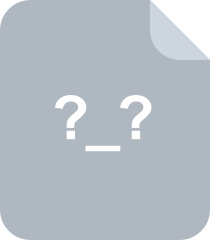
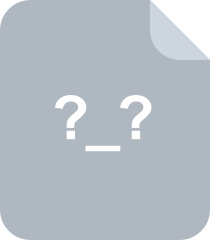
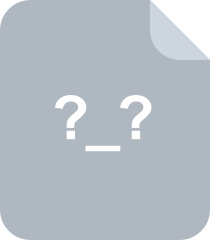
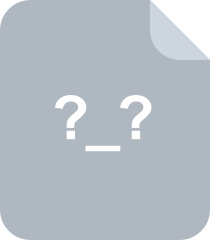
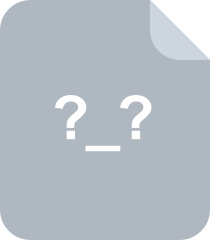
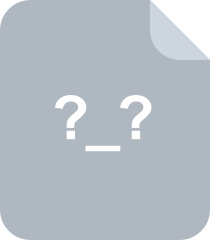
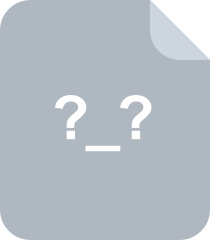
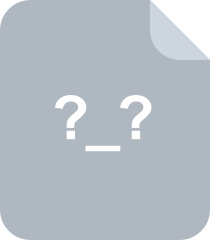
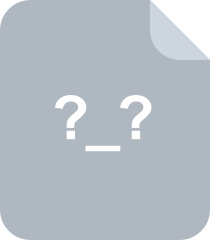
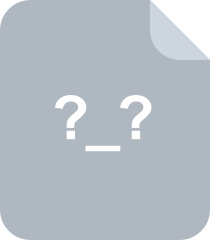
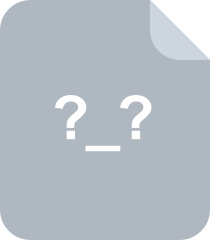
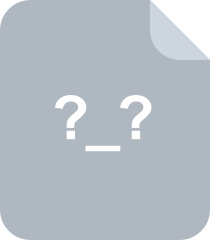
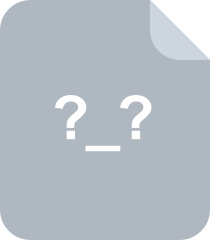
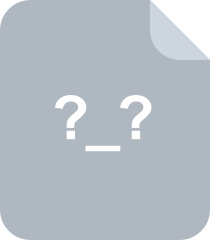
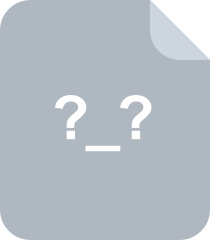
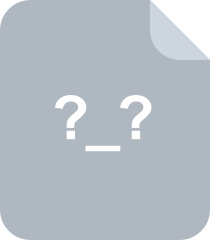
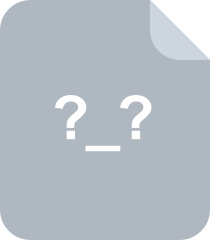
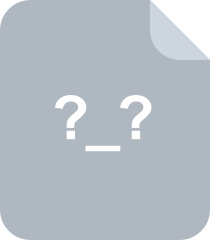
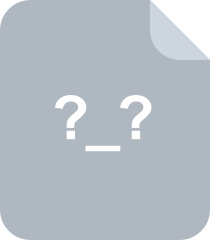
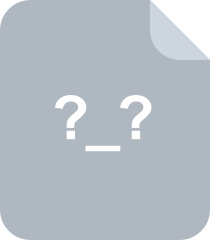
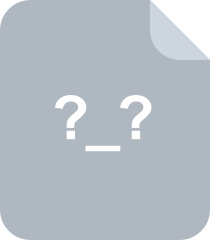
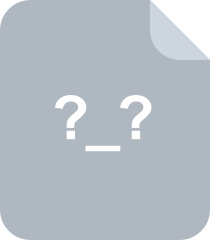
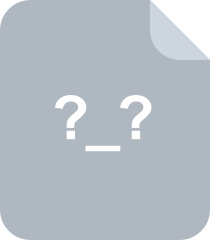
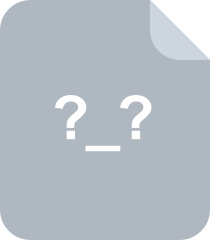
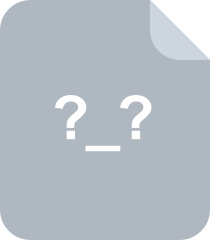
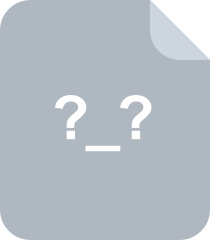
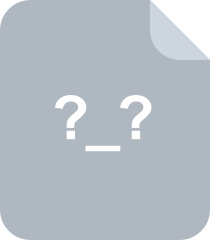
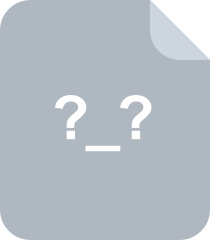
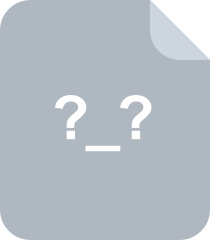
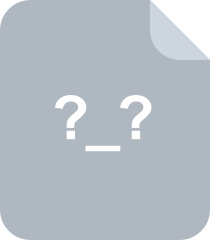
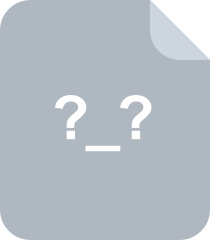
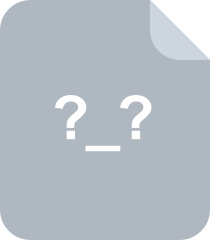
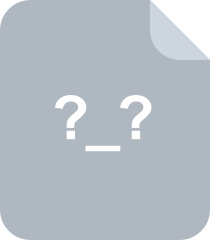
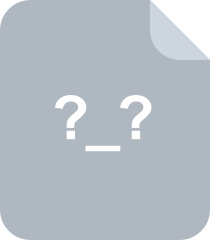
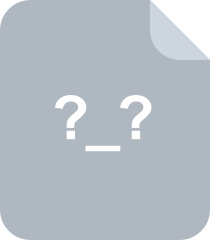
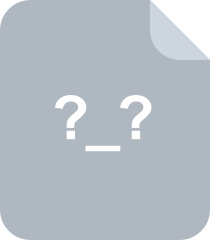
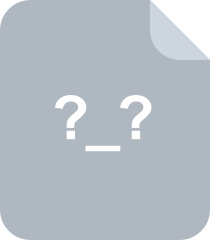
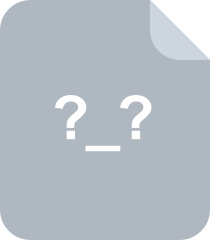
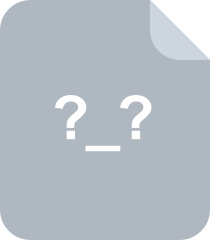
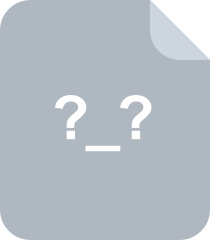
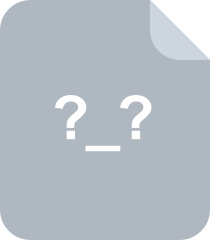
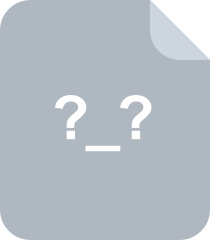
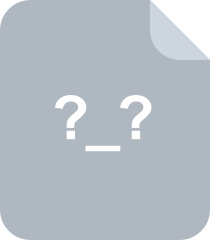
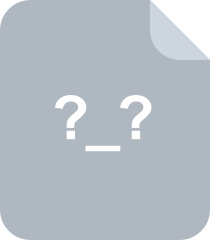
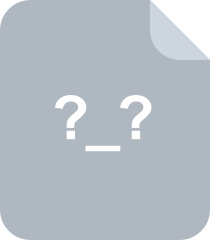
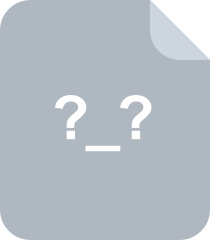
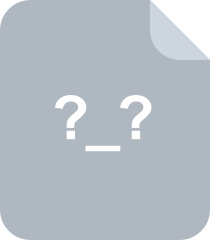
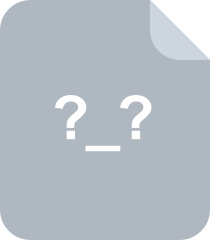
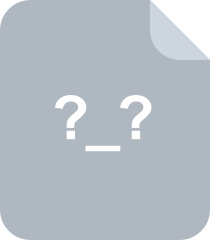
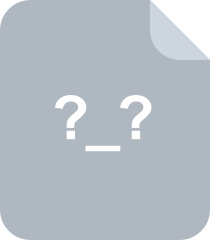
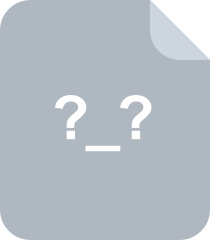
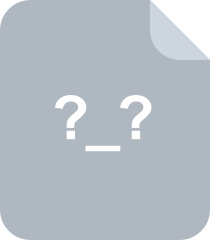
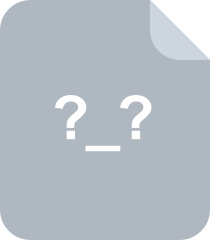
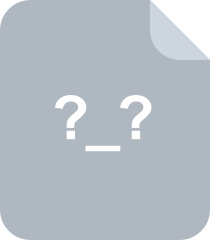
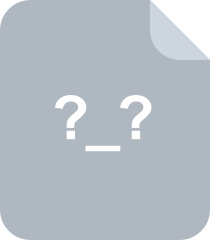
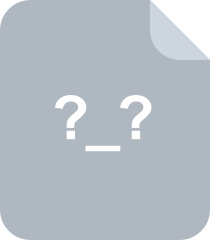
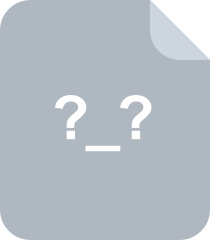
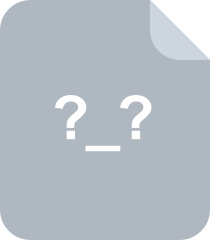
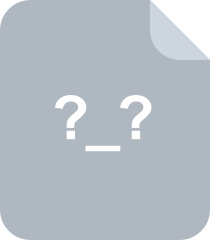
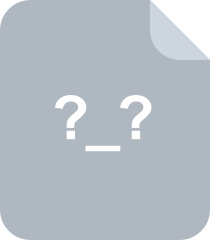
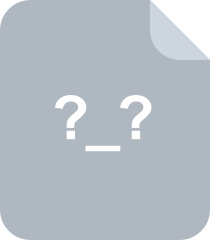
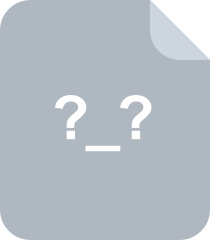
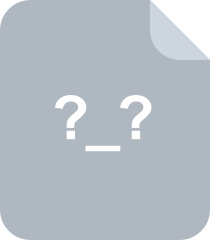
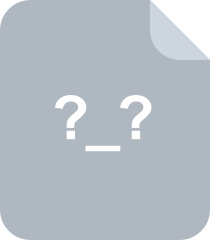
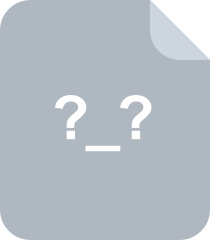
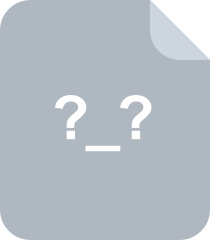
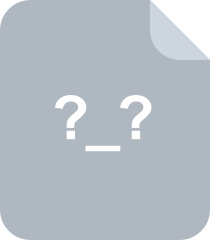
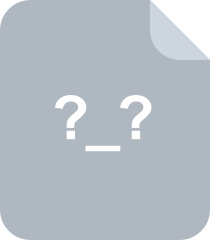
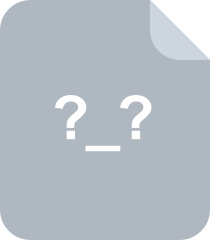
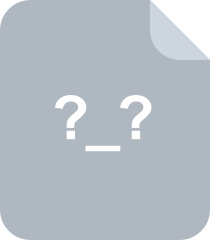
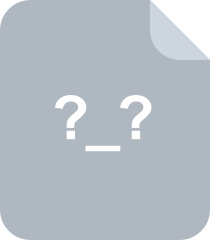
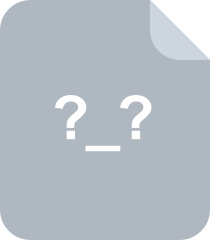
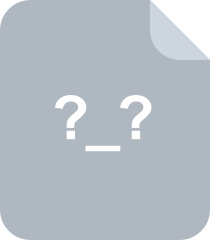
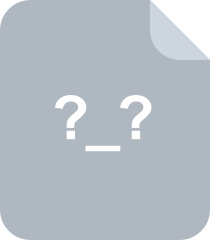
共 1215 条
- 1
- 2
- 3
- 4
- 5
- 6
- 13
资源评论
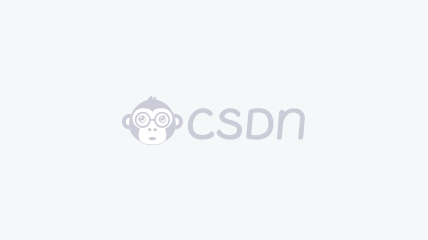

t0_54coder
- 粉丝: 2376
- 资源: 1629

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

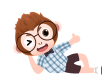
最新资源
- 基于MPC的非线性摆锤系统轨迹跟踪控制matlab仿真,包括程序中文注释,仿真操作步骤
- 基于MATLAB的ITS信道模型数值模拟仿真,包括程序中文注释,仿真操作步骤
- 基于Java、JavaScript、CSS的电子产品商城设计与实现源码
- 基于Vue 2的zjc项目设计源码,适用于赶项目需求
- 基于跨语言统一的C++头文件设计源码开发方案
- 基于MindSpore 1.3的T-GCNTemporal Graph Convolutional Network设计源码
- 基于Java的贝塞尔曲线绘制酷炫轮廓背景设计源码
- 基于Vue框架的Oracle数据库实训大作业设计与实现源码
- 基于SpringBoot和Vue的共享单车管理系统设计源码
- python基础学习(Part 1)的作业
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


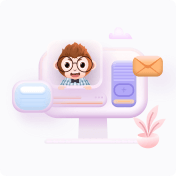
安全验证
文档复制为VIP权益,开通VIP直接复制
