<?php
/**
* CodeIgniter
*
* An open source application development framework for PHP
*
* This content is released under the MIT License (MIT)
*
* Copyright (c) 2014 - 2019, British Columbia Institute of Technology
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*
* @package CodeIgniter
* @author EllisLab Dev Team
* @copyright Copyright (c) 2008 - 2014, EllisLab, Inc. (https://ellislab.com/)
* @copyright Copyright (c) 2014 - 2019, British Columbia Institute of Technology (https://bcit.ca/)
* @license https://opensource.org/licenses/MIT MIT License
* @link https://codeigniter.com
* @since Version 1.0.0
* @filesource
*/
defined('BASEPATH') OR exit('No direct script access allowed');
/**
* Query Builder Class
*
* This is the platform-independent base Query Builder implementation class.
*
* @package CodeIgniter
* @subpackage Drivers
* @category Database
* @author EllisLab Dev Team
* @link https://codeigniter.com/user_guide/database/
*/
abstract class CI_DB_query_builder extends CI_DB_driver {
/**
* Return DELETE SQL flag
*
* @var bool
*/
protected $return_delete_sql = FALSE;
/**
* Reset DELETE data flag
*
* @var bool
*/
protected $reset_delete_data = FALSE;
/**
* QB SELECT data
*
* @var array
*/
protected $qb_select = array();
/**
* QB DISTINCT flag
*
* @var bool
*/
protected $qb_distinct = FALSE;
/**
* QB FROM data
*
* @var array
*/
protected $qb_from = array();
/**
* QB JOIN data
*
* @var array
*/
protected $qb_join = array();
/**
* QB WHERE data
*
* @var array
*/
protected $qb_where = array();
/**
* QB GROUP BY data
*
* @var array
*/
protected $qb_groupby = array();
/**
* QB HAVING data
*
* @var array
*/
protected $qb_having = array();
/**
* QB keys
*
* @var array
*/
protected $qb_keys = array();
/**
* QB LIMIT data
*
* @var int
*/
protected $qb_limit = FALSE;
/**
* QB OFFSET data
*
* @var int
*/
protected $qb_offset = FALSE;
/**
* QB ORDER BY data
*
* @var array
*/
protected $qb_orderby = array();
/**
* QB data sets
*
* @var array
*/
protected $qb_set = array();
/**
* QB data set for update_batch()
*
* @var array
*/
protected $qb_set_ub = array();
/**
* QB aliased tables list
*
* @var array
*/
protected $qb_aliased_tables = array();
/**
* QB WHERE group started flag
*
* @var bool
*/
protected $qb_where_group_started = FALSE;
/**
* QB WHERE group count
*
* @var int
*/
protected $qb_where_group_count = 0;
// Query Builder Caching variables
/**
* QB Caching flag
*
* @var bool
*/
protected $qb_caching = FALSE;
/**
* QB Cache exists list
*
* @var array
*/
protected $qb_cache_exists = array();
/**
* QB Cache SELECT data
*
* @var array
*/
protected $qb_cache_select = array();
/**
* QB Cache FROM data
*
* @var array
*/
protected $qb_cache_from = array();
/**
* QB Cache JOIN data
*
* @var array
*/
protected $qb_cache_join = array();
/**
* QB Cache aliased tables list
*
* @var array
*/
protected $qb_cache_aliased_tables = array();
/**
* QB Cache WHERE data
*
* @var array
*/
protected $qb_cache_where = array();
/**
* QB Cache GROUP BY data
*
* @var array
*/
protected $qb_cache_groupby = array();
/**
* QB Cache HAVING data
*
* @var array
*/
protected $qb_cache_having = array();
/**
* QB Cache ORDER BY data
*
* @var array
*/
protected $qb_cache_orderby = array();
/**
* QB Cache data sets
*
* @var array
*/
protected $qb_cache_set = array();
/**
* QB No Escape data
*
* @var array
*/
protected $qb_no_escape = array();
/**
* QB Cache No Escape data
*
* @var array
*/
protected $qb_cache_no_escape = array();
// --------------------------------------------------------------------
/**
* Select
*
* Generates the SELECT portion of the query
*
* @param string
* @param mixed
* @return CI_DB_query_builder
*/
public function select($select = '*', $escape = NULL)
{
if (is_string($select))
{
$select = explode(',', $select);
}
// If the escape value was not set, we will base it on the global setting
is_bool($escape) OR $escape = $this->_protect_identifiers;
foreach ($select as $val)
{
$val = trim($val);
if ($val !== '')
{
$this->qb_select[] = $val;
$this->qb_no_escape[] = $escape;
if ($this->qb_caching === TRUE)
{
$this->qb_cache_select[] = $val;
$this->qb_cache_exists[] = 'select';
$this->qb_cache_no_escape[] = $escape;
}
}
}
return $this;
}
// --------------------------------------------------------------------
/**
* Select Max
*
* Generates a SELECT MAX(field) portion of a query
*
* @param string the field
* @param string an alias
* @return CI_DB_query_builder
*/
public function select_max($select = '', $alias = '')
{
return $this->_max_min_avg_sum($select, $alias, 'MAX');
}
// --------------------------------------------------------------------
/**
* Select Min
*
* Generates a SELECT MIN(field) portion of a query
*
* @param string the field
* @param string an alias
* @return CI_DB_query_builder
*/
public function select_min($select = '', $alias = '')
{
return $this->_max_min_avg_sum($select, $alias, 'MIN');
}
// --------------------------------------------------------------------
/**
* Select Average
*
* Generates a SELECT AVG(field) portion of a query
*
* @param string the field
* @param string an alias
* @return CI_DB_query_builder
*/
public function select_avg($select = '', $alias = '')
{
return $this->_max_min_avg_sum($select, $alias, 'AVG');
}
// --------------------------------------------------------------------
/**
* Select Sum
*
* Generates a SELECT SUM(field) portion of a query
*
* @param string the field
* @param string an alias
* @return CI_DB_query_builder
*/
public function select_sum($select = '', $alias = '')
{
return $this->_max_min_avg_sum($select, $alias, 'SUM');
}
// --------------------------------------------------------------------
/**
* SELECT [MAX|MIN|AVG|SUM]()
*
* @used-by select_max()
* @used-by select_min()
* @used-by select_avg()
* @used-by select_sum()
*
* @param string $select Field name
* @param string $alias
* @param string $type
* @return CI_DB_query_builder
*/
protected function _max_min_avg_sum($select = '', $alias = '', $type = 'MAX')
{
if ( ! is_string($select) OR $select === '')
{
$this->display_error('db_invalid_query');
}
$type = strtoupper($type);
if ( ! in_array($type, array('MAX', 'MIN', 'AVG', 'SUM')))
{
show_error('Invalid function type: '.$type);
}
if ($alias === '')
{
$alias = $this->_create_alias_from_table(trim($select));
}
$sql = $type.
没有合适的资源?快使用搜索试试~ 我知道了~
PHP幼儿园管理系统.zip
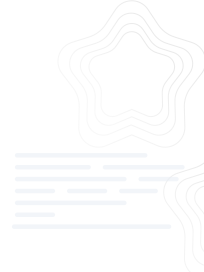
共700个文件
php:224个
html:217个
js:157个

0 下载量 54 浏览量
2024-08-28
13:51:57
上传
评论
收藏 5.13MB ZIP 举报
温馨提示
PHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统.zipPHP幼儿园管理系统
资源推荐
资源详情
资源评论
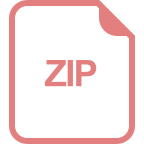
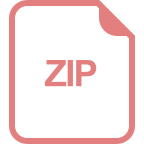
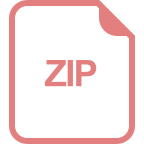
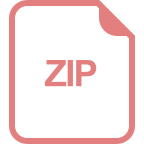
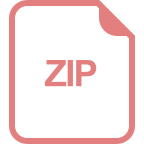
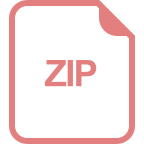
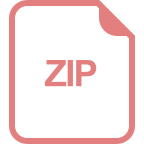
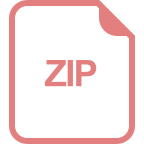
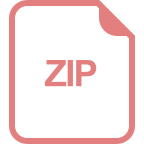
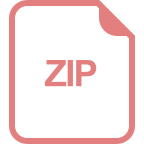
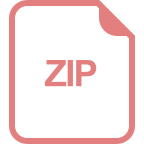
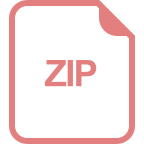
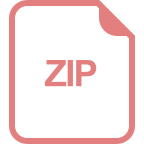
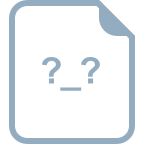
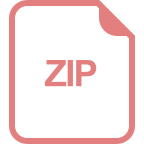
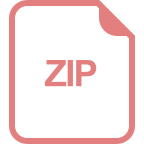
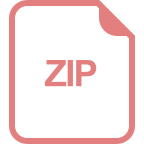
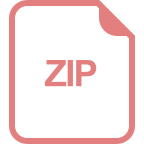
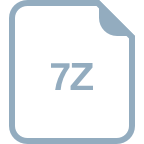
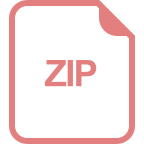
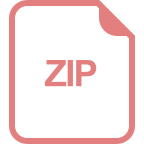
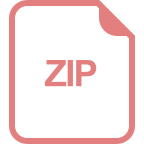
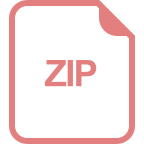
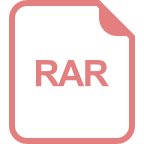
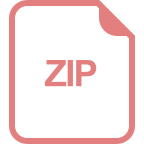
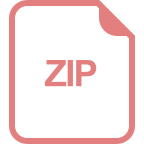
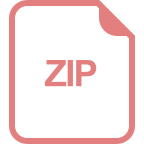
收起资源包目录

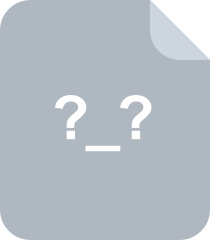
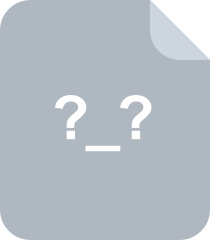
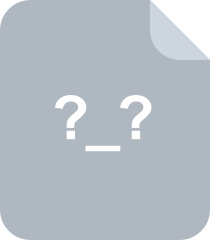
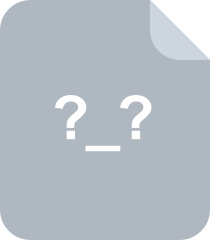
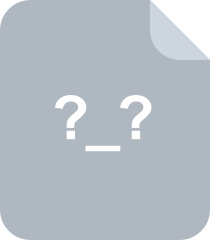
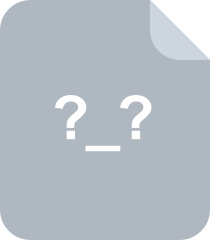
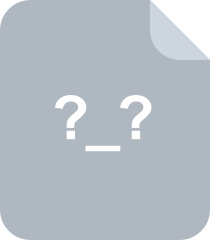
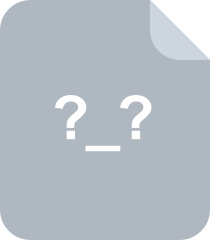
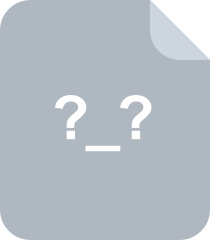
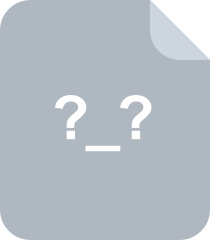
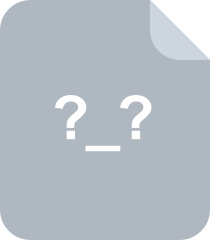
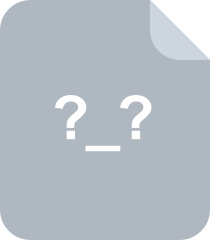
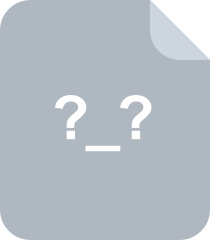
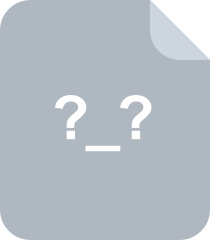
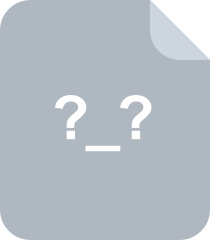
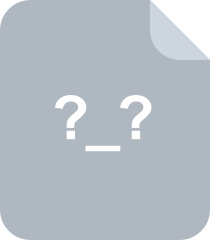
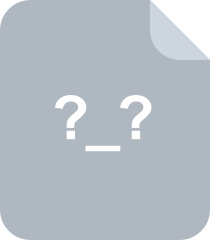
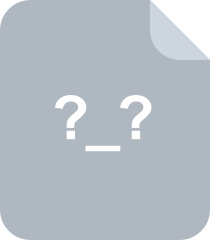
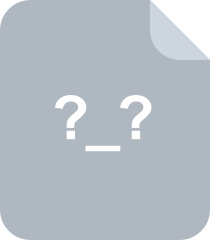
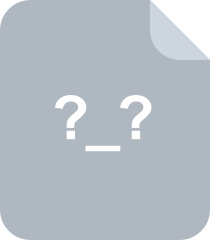
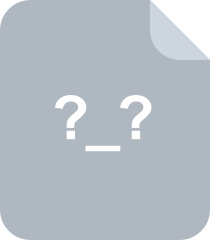
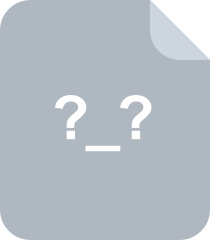
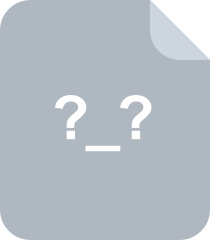
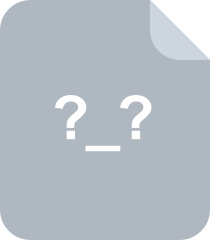
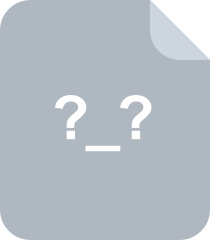
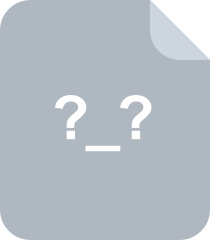
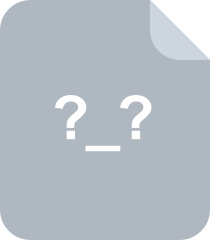
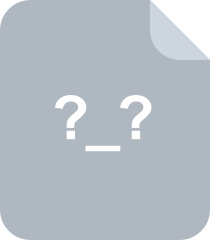
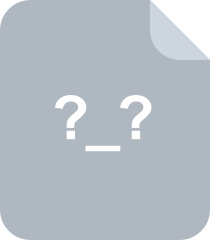
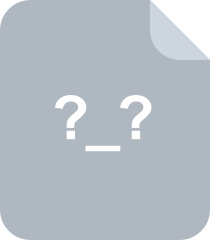
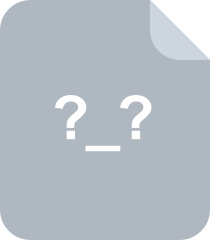
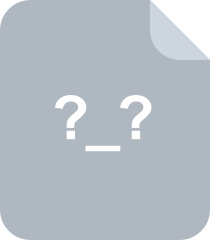
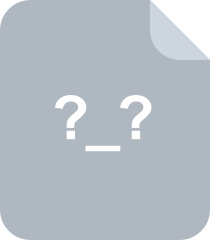
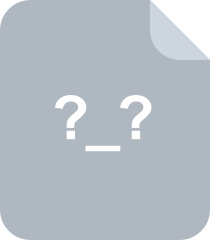
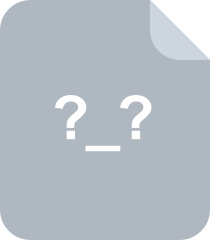
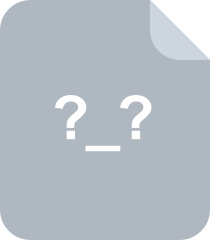
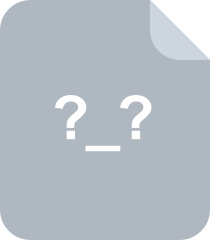
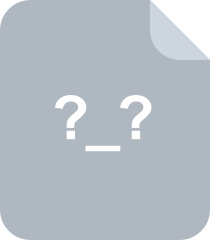
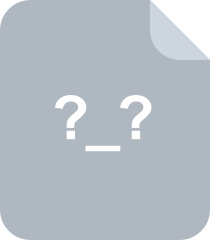
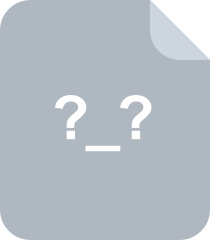
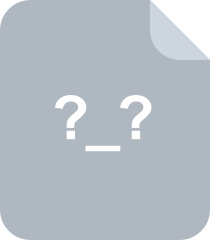
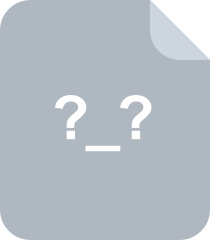
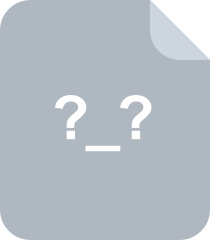
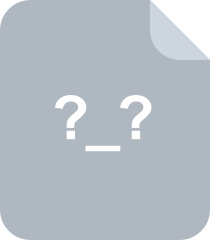
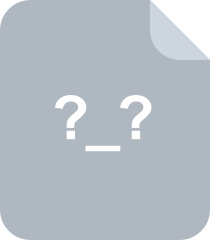
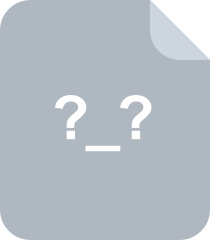
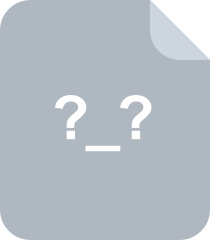
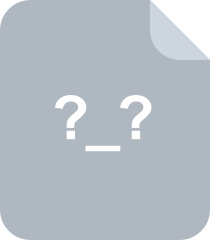
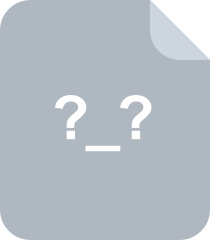
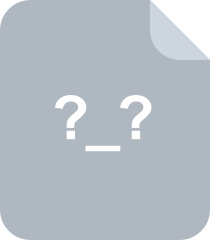
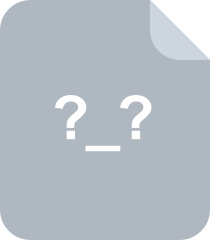
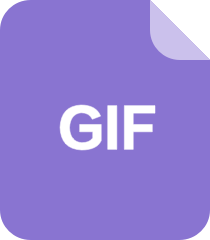
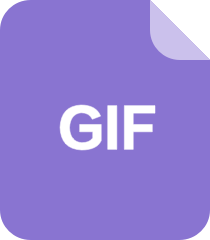
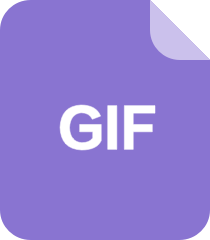
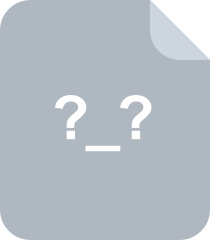
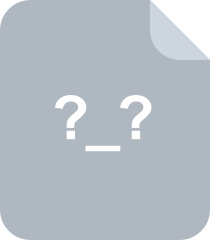
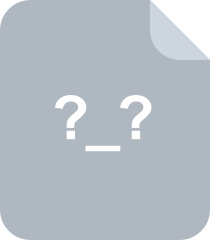
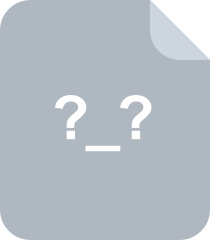
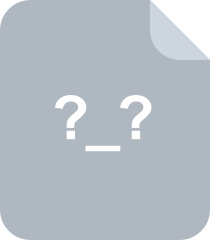
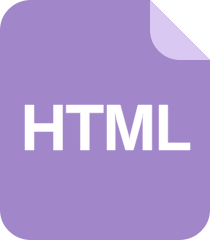
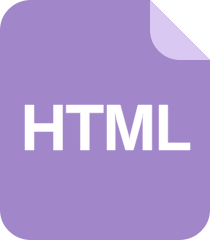
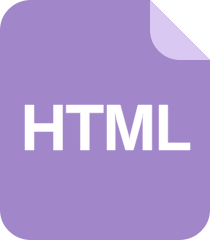
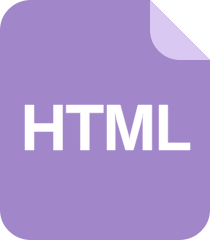
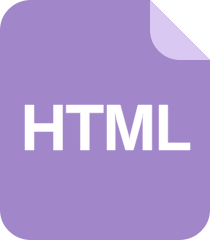
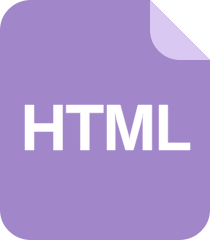
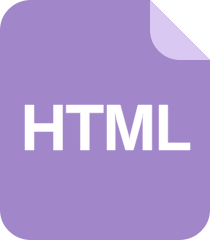
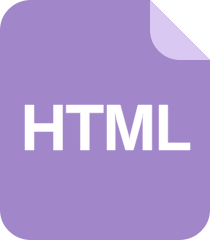
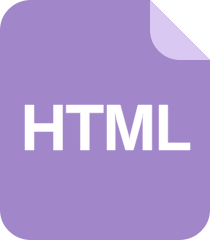
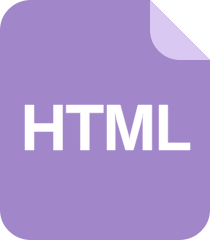
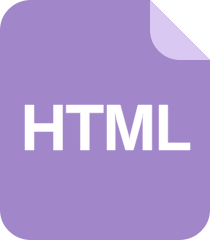
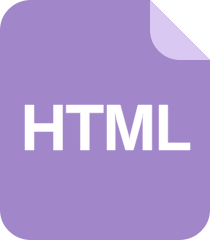
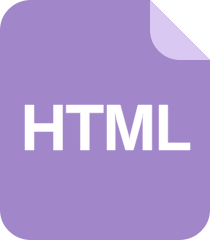
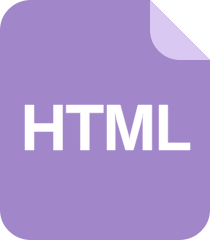
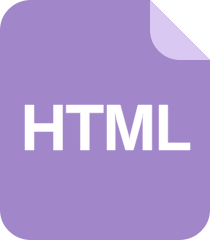
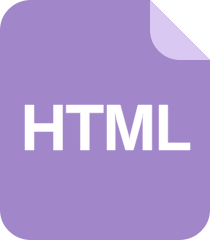
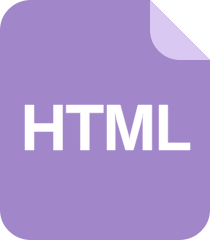
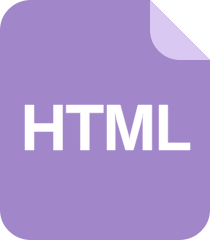
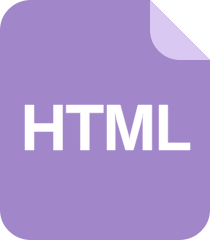
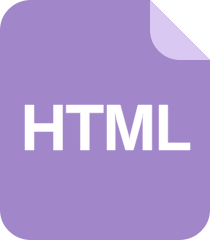
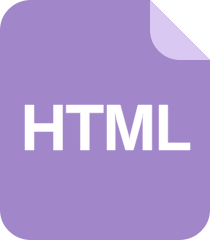
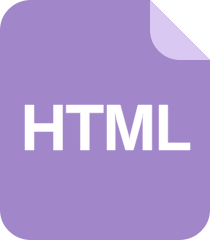
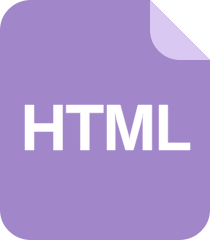
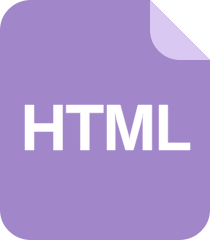
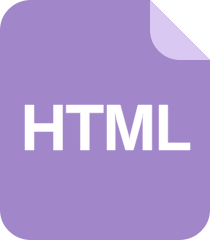
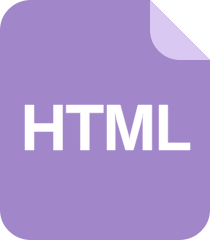
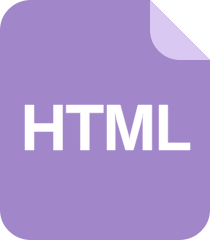
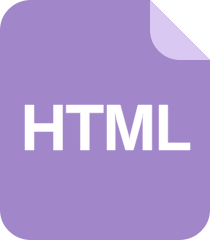
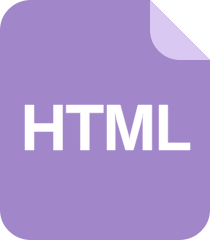
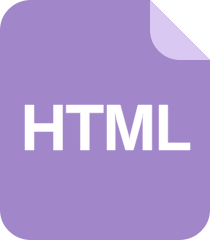
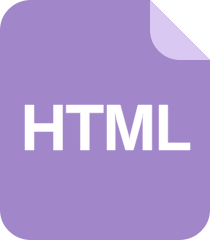
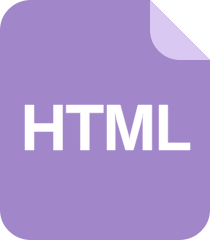
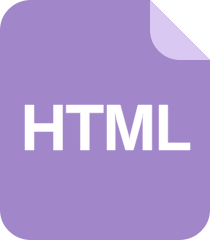
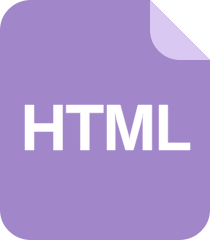
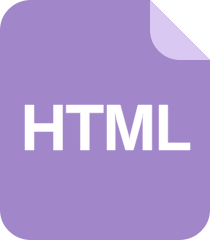
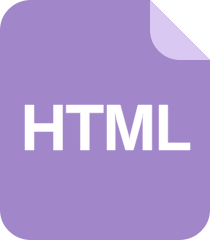
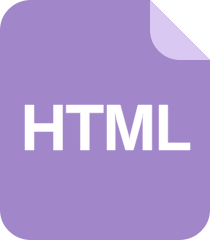
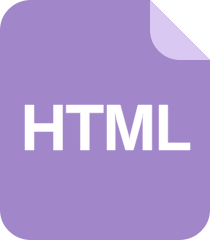
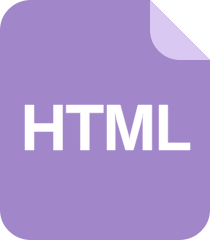
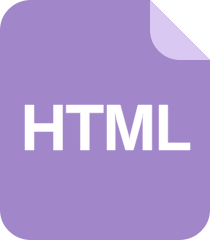
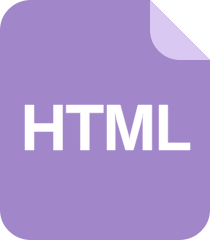
共 700 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
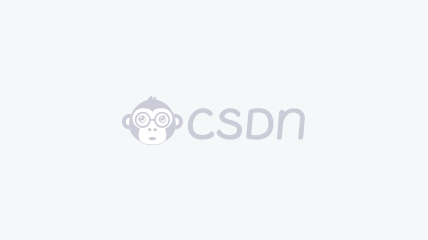

阿齐Archie
- 粉丝: 3w+
- 资源: 2474
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

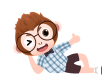
最新资源
- 三菱FX系列上位机源码 C#上位机FX系列源码, 串口485.232通讯, 可读X Y M S D,可写Y M S D,FX系列,有备注 本上位机使用用pchmi库
- azkaban执行job相关文件
- 基于ssm的高校校园招聘服务系统源码(java毕业设计完整源码+LW).zip
- 飞剪程序、追剪程序plc程序伺服程序 几年前的飞剪追剪程序,用的都是汇川系列 包含详细的注释、触摸屏程序、plc程序、伺服参数设置和图纸,实际当中的应用
- 不同颜色机器护垫检测14-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 西门子S7-1200控制5轴伺服程序加维纶触摸屏画面案例 1.PTO伺服轴脉冲定位控制功能应用+速度模式应用+扭矩模式应用 2.程序为结构化编程,每一功能为模块化设计,具有一个项目都有的功能:
- 深度学习,pytorch,四种天气图片数据集
- 潮乎盲盒小程序,盲盒全开源源码,潮玩盲盒功能,推广海报,多级分销,小程序源码
- 锅炉控制系统,西门子200smartPLC程序,昆仑触摸屏程序,带CAD电气图纸
- 大学计算机专业课程学习报告-深入探索编程与技术前沿
- MMC七电平整流器模型 MATLAB,2019及以下版本 带单相接地故障设置
- Dreamweaver CS6
- 冒泡排序算法详解与Python实现
- C++图书管理系统源代码+设计文档(高分项目)
- 书籍学习配套源码:TCPIP服务器客户端 和 UDP服务器客户端 程序
- 基于Python的网络流量分析控制台.py
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


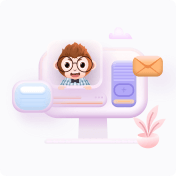
安全验证
文档复制为VIP权益,开通VIP直接复制
