/**
******************************************************************************
* @file stm32h7xx_hal_tim.c
* @author MCD Application Team
* @brief TIM HAL module driver.
* This file provides firmware functions to manage the following
* functionalities of the Timer (TIM) peripheral:
* + TIM Time Base Initialization
* + TIM Time Base Start
* + TIM Time Base Start Interruption
* + TIM Time Base Start DMA
* + TIM Output Compare/PWM Initialization
* + TIM Output Compare/PWM Channel Configuration
* + TIM Output Compare/PWM Start
* + TIM Output Compare/PWM Start Interruption
* + TIM Output Compare/PWM Start DMA
* + TIM Input Capture Initialization
* + TIM Input Capture Channel Configuration
* + TIM Input Capture Start
* + TIM Input Capture Start Interruption
* + TIM Input Capture Start DMA
* + TIM One Pulse Initialization
* + TIM One Pulse Channel Configuration
* + TIM One Pulse Start
* + TIM Encoder Interface Initialization
* + TIM Encoder Interface Start
* + TIM Encoder Interface Start Interruption
* + TIM Encoder Interface Start DMA
* + Commutation Event configuration with Interruption and DMA
* + TIM OCRef clear configuration
* + TIM External Clock configuration
******************************************************************************
* @attention
*
* Copyright (c) 2017 STMicroelectronics.
* All rights reserved.
*
* This software is licensed under terms that can be found in the LICENSE file
* in the root directory of this software component.
* If no LICENSE file comes with this software, it is provided AS-IS.
*
******************************************************************************
@verbatim
==============================================================================
##### TIMER Generic features #####
==============================================================================
[..] The Timer features include:
(#) 16-bit up, down, up/down auto-reload counter.
(#) 16-bit programmable prescaler allowing dividing (also on the fly) the
counter clock frequency either by any factor between 1 and 65536.
(#) Up to 4 independent channels for:
(++) Input Capture
(++) Output Compare
(++) PWM generation (Edge and Center-aligned Mode)
(++) One-pulse mode output
(#) Synchronization circuit to control the timer with external signals and to interconnect
several timers together.
(#) Supports incremental encoder for positioning purposes
##### How to use this driver #####
==============================================================================
[..]
(#) Initialize the TIM low level resources by implementing the following functions
depending on the selected feature:
(++) Time Base : HAL_TIM_Base_MspInit()
(++) Input Capture : HAL_TIM_IC_MspInit()
(++) Output Compare : HAL_TIM_OC_MspInit()
(++) PWM generation : HAL_TIM_PWM_MspInit()
(++) One-pulse mode output : HAL_TIM_OnePulse_MspInit()
(++) Encoder mode output : HAL_TIM_Encoder_MspInit()
(#) Initialize the TIM low level resources :
(##) Enable the TIM interface clock using __HAL_RCC_TIMx_CLK_ENABLE();
(##) TIM pins configuration
(+++) Enable the clock for the TIM GPIOs using the following function:
__HAL_RCC_GPIOx_CLK_ENABLE();
(+++) Configure these TIM pins in Alternate function mode using HAL_GPIO_Init();
(#) The external Clock can be configured, if needed (the default clock is the
internal clock from the APBx), using the following function:
HAL_TIM_ConfigClockSource, the clock configuration should be done before
any start function.
(#) Configure the TIM in the desired functioning mode using one of the
Initialization function of this driver:
(++) HAL_TIM_Base_Init: to use the Timer to generate a simple time base
(++) HAL_TIM_OC_Init and HAL_TIM_OC_ConfigChannel: to use the Timer to generate an
Output Compare signal.
(++) HAL_TIM_PWM_Init and HAL_TIM_PWM_ConfigChannel: to use the Timer to generate a
PWM signal.
(++) HAL_TIM_IC_Init and HAL_TIM_IC_ConfigChannel: to use the Timer to measure an
external signal.
(++) HAL_TIM_OnePulse_Init and HAL_TIM_OnePulse_ConfigChannel: to use the Timer
in One Pulse Mode.
(++) HAL_TIM_Encoder_Init: to use the Timer Encoder Interface.
(#) Activate the TIM peripheral using one of the start functions depending from the feature used:
(++) Time Base : HAL_TIM_Base_Start(), HAL_TIM_Base_Start_DMA(), HAL_TIM_Base_Start_IT()
(++) Input Capture : HAL_TIM_IC_Start(), HAL_TIM_IC_Start_DMA(), HAL_TIM_IC_Start_IT()
(++) Output Compare : HAL_TIM_OC_Start(), HAL_TIM_OC_Start_DMA(), HAL_TIM_OC_Start_IT()
(++) PWM generation : HAL_TIM_PWM_Start(), HAL_TIM_PWM_Start_DMA(), HAL_TIM_PWM_Start_IT()
(++) One-pulse mode output : HAL_TIM_OnePulse_Start(), HAL_TIM_OnePulse_Start_IT()
(++) Encoder mode output : HAL_TIM_Encoder_Start(), HAL_TIM_Encoder_Start_DMA(), HAL_TIM_Encoder_Start_IT().
(#) The DMA Burst is managed with the two following functions:
HAL_TIM_DMABurst_WriteStart()
HAL_TIM_DMABurst_ReadStart()
*** Callback registration ***
=============================================
[..]
The compilation define USE_HAL_TIM_REGISTER_CALLBACKS when set to 1
allows the user to configure dynamically the driver callbacks.
[..]
Use Function HAL_TIM_RegisterCallback() to register a callback.
HAL_TIM_RegisterCallback() takes as parameters the HAL peripheral handle,
the Callback ID and a pointer to the user callback function.
[..]
Use function HAL_TIM_UnRegisterCallback() to reset a callback to the default
weak function.
HAL_TIM_UnRegisterCallback takes as parameters the HAL peripheral handle,
and the Callback ID.
[..]
These functions allow to register/unregister following callbacks:
(+) Base_MspInitCallback : TIM Base Msp Init Callback.
(+) Base_MspDeInitCallback : TIM Base Msp DeInit Callback.
(+) IC_MspInitCallback : TIM IC Msp Init Callback.
(+) IC_MspDeInitCallback : TIM IC Msp DeInit Callback.
(+) OC_MspInitCallback : TIM OC Msp Init Callback.
(+) OC_MspDeInitCallback : TIM OC Msp DeInit Callback.
(+) PWM_MspInitCallback : TIM PWM Msp Init Callback.
(+) PWM_MspDeInitCallback : TIM PWM Msp DeInit Callback.
(+) OnePulse_MspInitCallback : TIM One Pulse Msp Init Callback.
(+) OnePulse_MspDeInitCallback : TIM One Pulse Msp DeInit Callback.
(+) Encoder_MspInitCallback : TIM Encoder Msp Init Callback.
(+) Encoder_MspDeInitCallback : TIM Encoder Msp DeInit Callback.
(+) HallSensor_MspInitCallback : TIM Hall Sensor Msp Init Callback.
(+) HallSensor_MspDeInitCallback : TIM Hall Sensor Msp DeInit Callback.
(+) PeriodElapsedCallback : TIM Period Elapsed Callback.
(+) PeriodElapsedHalfCpltCallback : TIM Period Elapsed half complete Callback.
(+) TriggerCallback : TIM Trigger Callback.
(+) TriggerHalfCpltCallback : TIM Trigger half complete Callback.
(+) IC_CaptureCallback : TIM Input Capture Callback.
(+) IC_CaptureHalfCpltCallback : TIM Input Capture half complete Callback.
(+) OC_DelayElapsedCallback
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
嵌入式优质项目,资源经过严格测试可直接运行成功且功能正常的情况才上传,可轻松copy复刻,拿到资料包后可轻松复现出一样的项目。 本人单片机开发经验充足,深耕嵌入式领域,有任何使用问题欢迎随时与我联系,我会及时为你解惑,提供帮助。 【资源内容】:包含完整源码+工程文件+说明,项目具体内容可查看下方的资源详情。 【附带帮助】: 若还需要嵌入式物联网单片机相关领域开发工具、学习资料等,我会提供帮助,提供资料,鼓励学习进步。 【本人专注嵌入式领域】: 有任何使用问题欢迎随时与我联系,我会及时解答,第一时间为你提供帮助,CSDN博客端可私信,为你解惑,欢迎交流。 【建议小白】: 在所有嵌入式开发中硬件部分若不会画PCB/电路,可选择根据引脚定义将其代替为面包板+杜邦线+外设模块的方式,只需轻松简单连线,下载源码烧录进去便可轻松复刻出一样的项目 【适合场景】: 相关项目设计中,皆可应用在项目开发、毕业设计、课程设计、期末/期中/大作业、工程实训、大创等学科竞赛比赛、初期项目立项、学习/练手等方面中 可借鉴此优质项目实现复刻,也可以基于此项目进行扩展来开发出更多功能
资源推荐
资源详情
资源评论
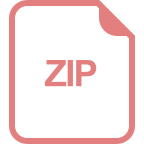
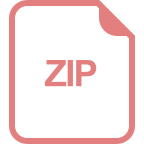
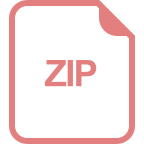
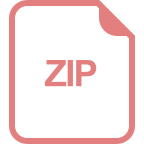
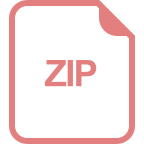
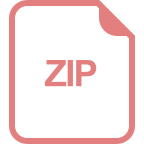
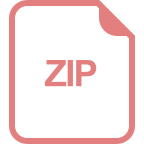
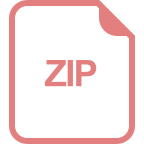
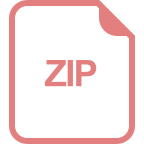
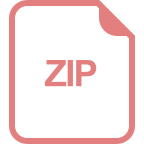
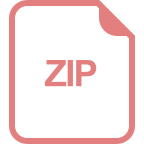
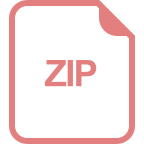
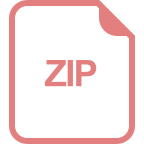
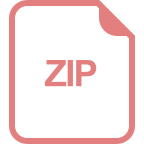
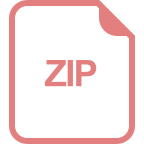
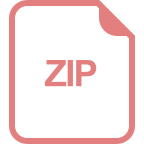
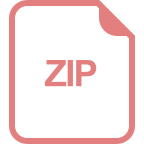
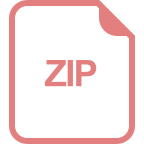
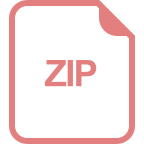
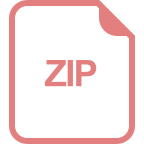
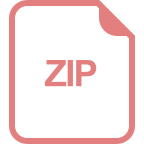
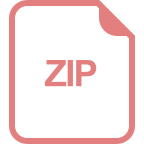
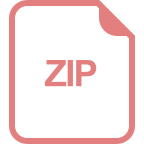
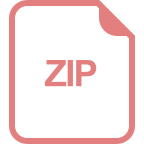
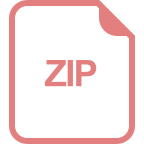
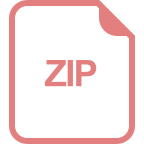
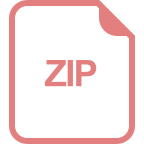
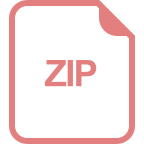
收起资源包目录

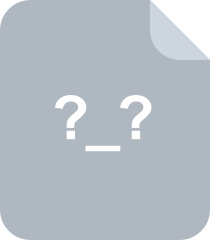
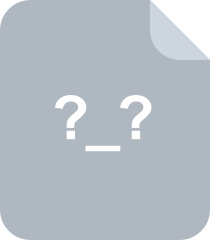
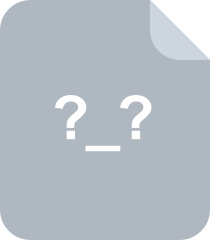
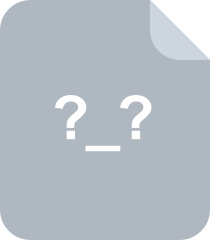
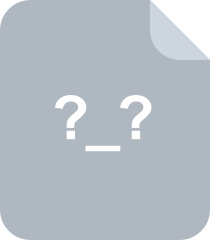
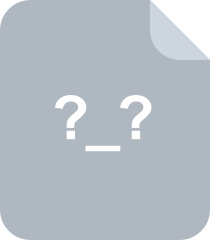
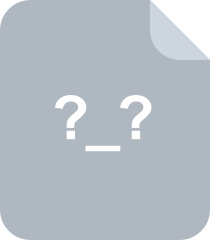
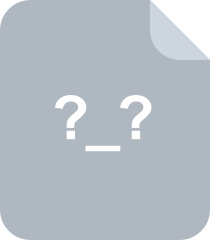
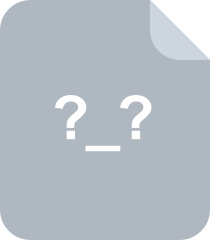
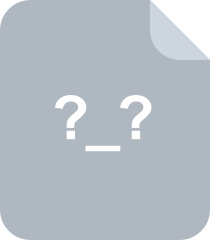
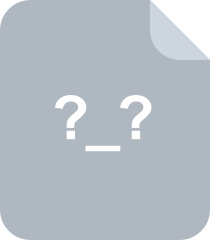
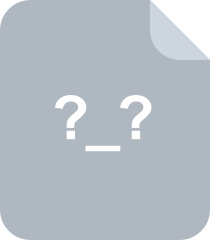
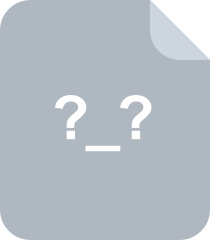
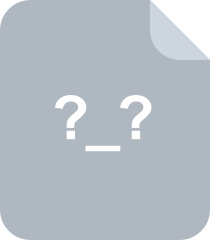
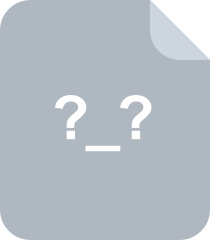
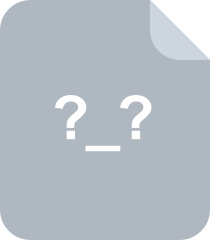
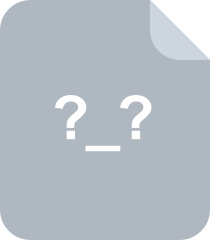
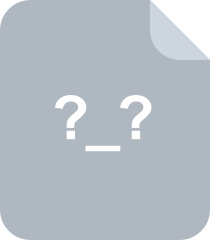
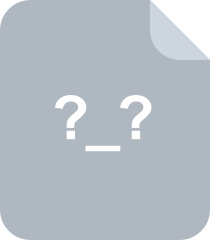
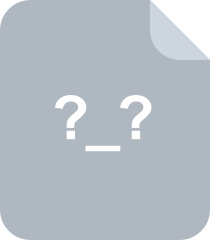
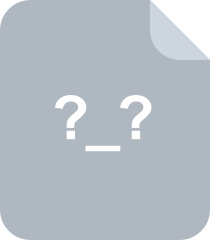
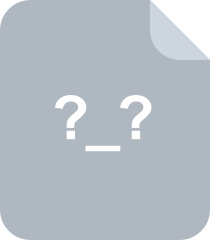
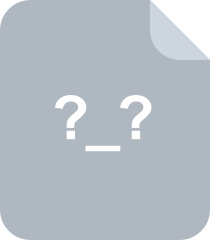
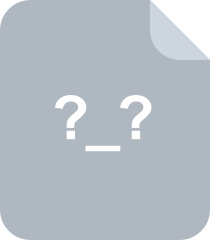
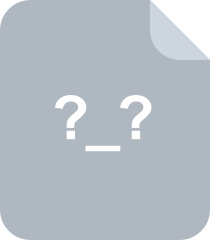
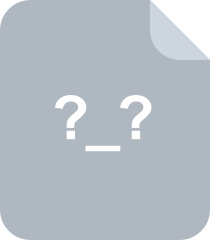
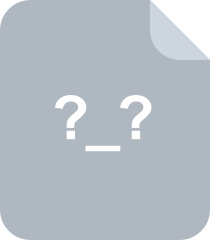
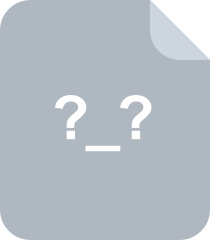
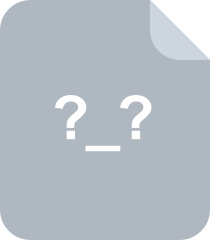
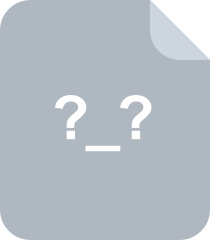
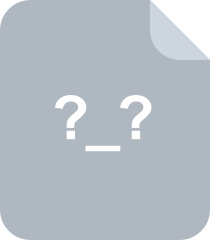
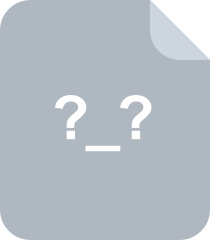
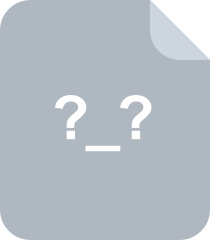
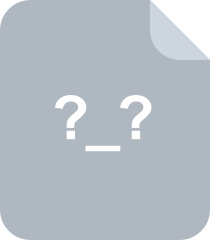
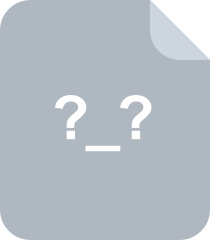
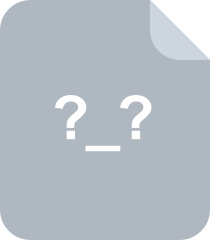
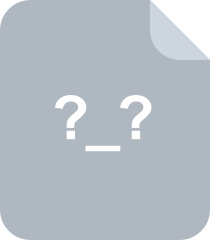
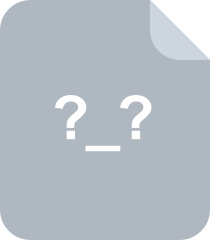
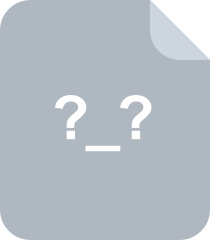
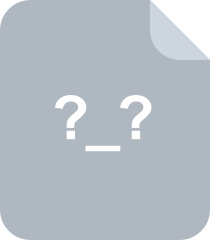
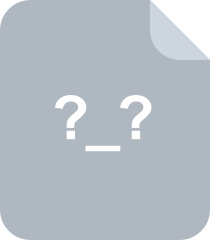
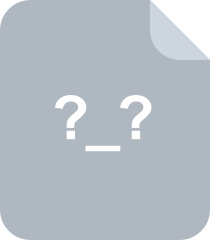
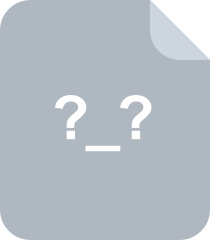
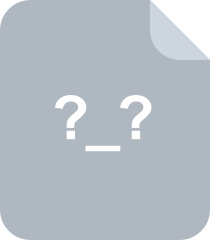
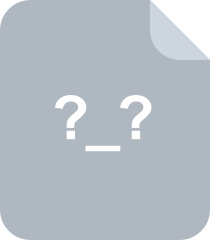
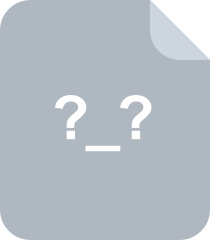
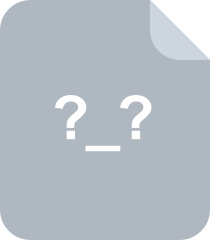
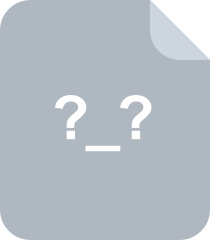
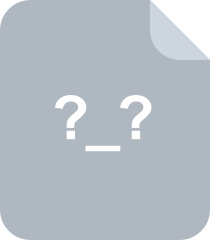
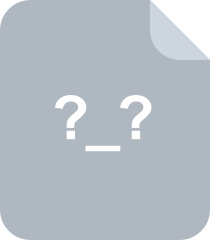
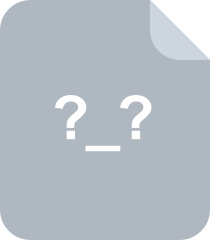
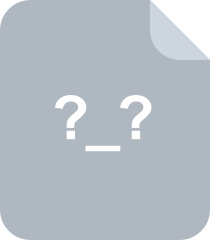
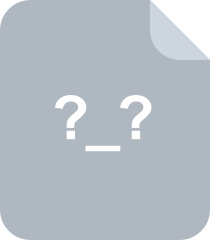
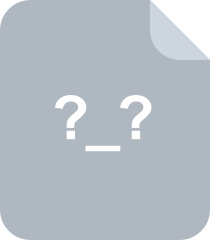
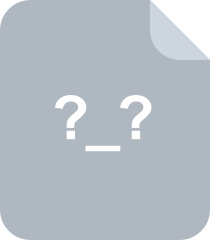
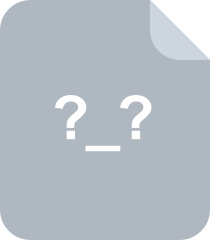
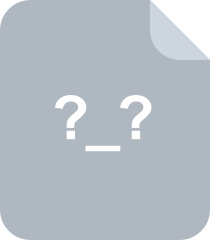
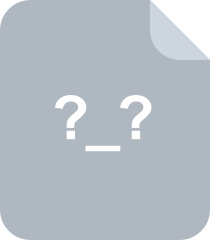
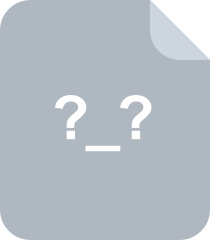
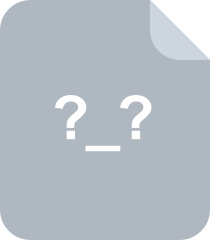
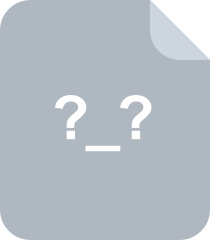
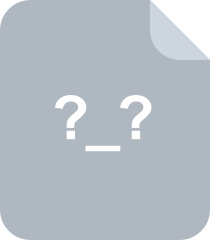
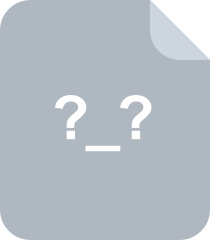
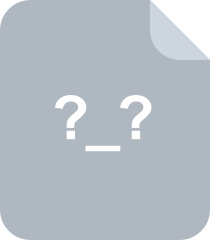
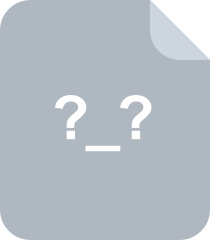
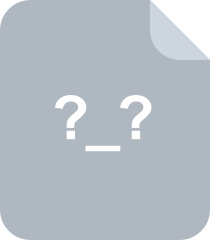
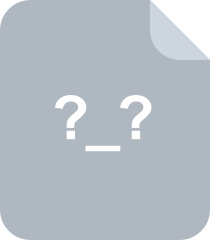
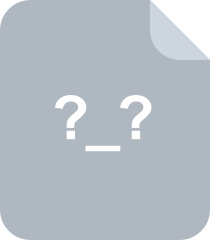
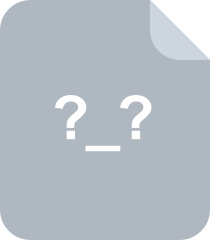
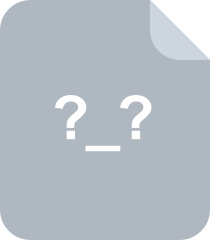
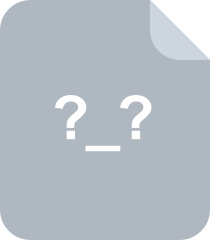
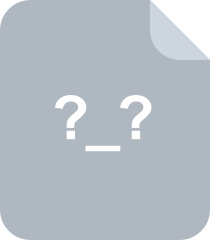
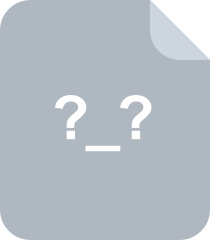
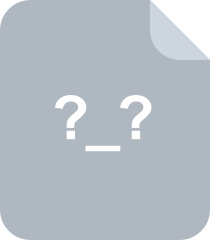
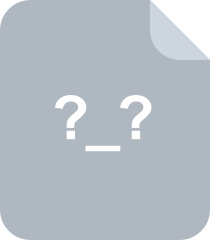
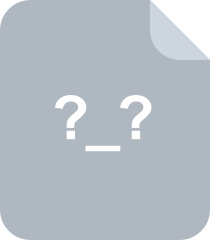
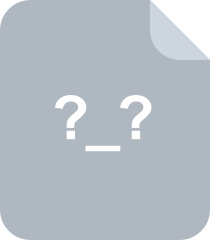
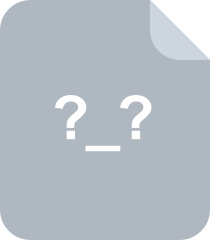
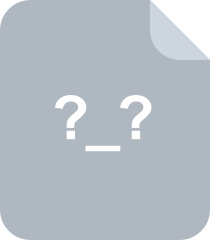
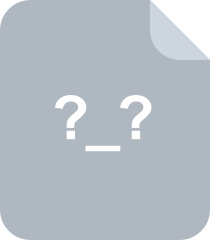
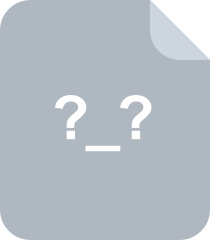
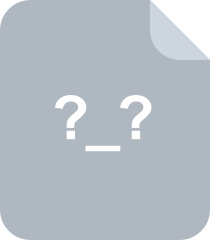
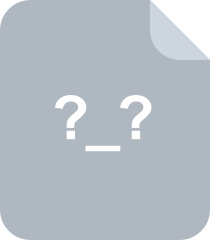
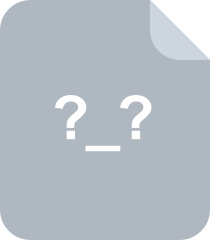
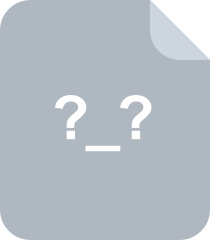
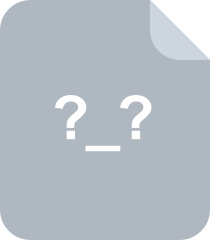
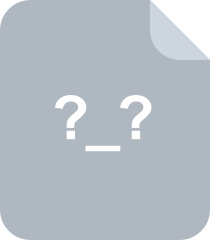
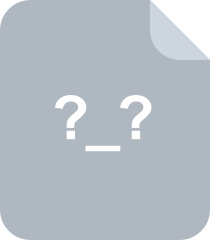
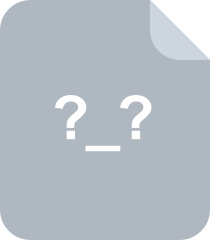
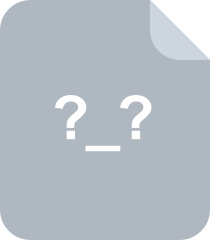
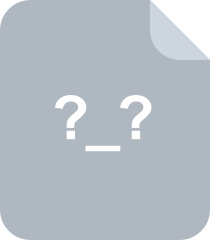
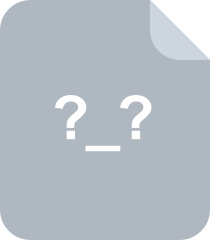
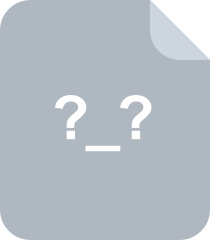
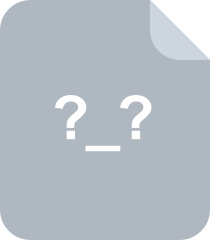
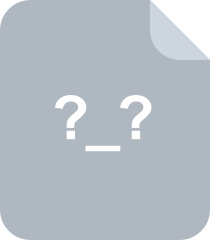
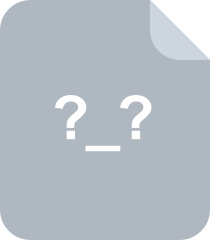
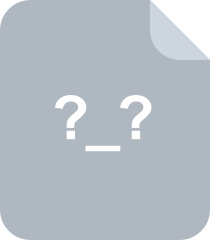
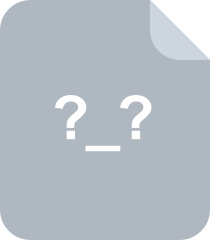
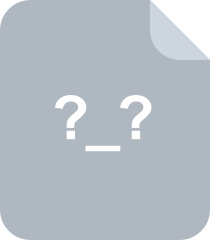
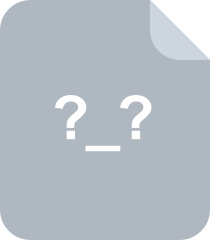
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
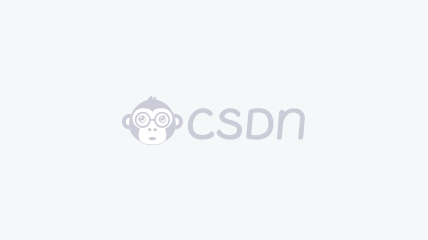

阿齐Archie
- 粉丝: 3w+
- 资源: 2463

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

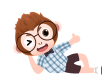
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


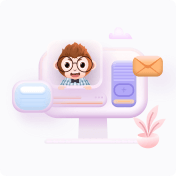
安全验证
文档复制为VIP权益,开通VIP直接复制
