Driver Model
============
This README contains high-level information about driver model, a unified
way of declaring and accessing drivers in U-Boot. The original work was done
by:
Marek Vasut <marex@denx.de>
Pavel Herrmann <morpheus.ibis@gmail.com>
Viktor Křivák <viktor.krivak@gmail.com>
Tomas Hlavacek <tmshlvck@gmail.com>
This has been both simplified and extended into the current implementation
by:
Simon Glass <sjg@chromium.org>
Terminology
-----------
Uclass - a group of devices which operate in the same way. A uclass provides
a way of accessing individual devices within the group, but always
using the same interface. For example a GPIO uclass provides
operations for get/set value. An I2C uclass may have 10 I2C ports,
4 with one driver, and 6 with another.
Driver - some code which talks to a peripheral and presents a higher-level
interface to it.
Device - an instance of a driver, tied to a particular port or peripheral.
How to try it
-------------
Build U-Boot sandbox and run it:
make sandbox_defconfig
make
./u-boot -d u-boot.dtb
(type 'reset' to exit U-Boot)
There is a uclass called 'demo'. This uclass handles
saying hello, and reporting its status. There are two drivers in this
uclass:
- simple: Just prints a message for hello, doesn't implement status
- shape: Prints shapes and reports number of characters printed as status
The demo class is pretty simple, but not trivial. The intention is that it
can be used for testing, so it will implement all driver model features and
provide good code coverage of them. It does have multiple drivers, it
handles parameter data and platdata (data which tells the driver how
to operate on a particular platform) and it uses private driver data.
To try it, see the example session below:
=>demo hello 1
Hello '@' from 07981110: red 4
=>demo status 2
Status: 0
=>demo hello 2
g
r@
e@@
e@@@
n@@@@
g@@@@@
=>demo status 2
Status: 21
=>demo hello 4 ^
y^^^
e^^^^^
l^^^^^^^
l^^^^^^^
o^^^^^
w^^^
=>demo status 4
Status: 36
=>
Running the tests
-----------------
The intent with driver model is that the core portion has 100% test coverage
in sandbox, and every uclass has its own test. As a move towards this, tests
are provided in test/dm. To run them, try:
./test/dm/test-dm.sh
You should see something like this:
<...U-Boot banner...>
Running 53 driver model tests
Test: dm_test_autobind
Test: dm_test_autoprobe
Test: dm_test_bus_child_post_bind
Test: dm_test_bus_child_post_bind_uclass
Test: dm_test_bus_child_pre_probe_uclass
Test: dm_test_bus_children
Device 'c-test@0': seq 0 is in use by 'd-test'
Device 'c-test@1': seq 1 is in use by 'f-test'
Test: dm_test_bus_children_funcs
Test: dm_test_bus_children_iterators
Test: dm_test_bus_parent_data
Test: dm_test_bus_parent_data_uclass
Test: dm_test_bus_parent_ops
Test: dm_test_bus_parent_platdata
Test: dm_test_bus_parent_platdata_uclass
Test: dm_test_children
Test: dm_test_device_get_uclass_id
Test: dm_test_eth
Using eth@10002000 device
Using eth@10003000 device
Using eth@10004000 device
Test: dm_test_eth_alias
Using eth@10002000 device
Using eth@10004000 device
Using eth@10002000 device
Using eth@10003000 device
Test: dm_test_eth_prime
Using eth@10003000 device
Using eth@10002000 device
Test: dm_test_eth_rotate
Error: eth@10004000 address not set.
Error: eth@10004000 address not set.
Using eth@10002000 device
Error: eth@10004000 address not set.
Error: eth@10004000 address not set.
Using eth@10004000 device
Test: dm_test_fdt
Test: dm_test_fdt_offset
Test: dm_test_fdt_pre_reloc
Test: dm_test_fdt_uclass_seq
Test: dm_test_gpio
extra-gpios: get_value: error: gpio b5 not reserved
Test: dm_test_gpio_anon
Test: dm_test_gpio_copy
Test: dm_test_gpio_leak
extra-gpios: get_value: error: gpio b5 not reserved
Test: dm_test_gpio_phandles
Test: dm_test_gpio_requestf
Test: dm_test_i2c_bytewise
Test: dm_test_i2c_find
Test: dm_test_i2c_offset
Test: dm_test_i2c_offset_len
Test: dm_test_i2c_probe_empty
Test: dm_test_i2c_read_write
Test: dm_test_i2c_speed
Test: dm_test_leak
Test: dm_test_lifecycle
Test: dm_test_net_retry
Using eth@10004000 device
Using eth@10002000 device
Using eth@10004000 device
Test: dm_test_operations
Test: dm_test_ordering
Test: dm_test_pci_base
Test: dm_test_pci_swapcase
Test: dm_test_platdata
Test: dm_test_pre_reloc
Test: dm_test_remove
Test: dm_test_spi_find
Invalid chip select 0:0 (err=-19)
SF: Failed to get idcodes
SF: Detected M25P16 with page size 256 Bytes, erase size 64 KiB, total 2 MiB
Test: dm_test_spi_flash
2097152 bytes written in 0 ms
SF: Detected M25P16 with page size 256 Bytes, erase size 64 KiB, total 2 MiB
SPI flash test:
0 erase: 0 ticks, 65536000 KiB/s 524288.000 Mbps
1 check: 0 ticks, 65536000 KiB/s 524288.000 Mbps
2 write: 0 ticks, 65536000 KiB/s 524288.000 Mbps
3 read: 0 ticks, 65536000 KiB/s 524288.000 Mbps
Test passed
0 erase: 0 ticks, 65536000 KiB/s 524288.000 Mbps
1 check: 0 ticks, 65536000 KiB/s 524288.000 Mbps
2 write: 0 ticks, 65536000 KiB/s 524288.000 Mbps
3 read: 0 ticks, 65536000 KiB/s 524288.000 Mbps
Test: dm_test_spi_xfer
SF: Detected M25P16 with page size 256 Bytes, erase size 64 KiB, total 2 MiB
Test: dm_test_uclass
Test: dm_test_uclass_before_ready
Test: dm_test_usb_base
Test: dm_test_usb_flash
USB-1: scanning bus 1 for devices... 2 USB Device(s) found
Failures: 0
What is going on?
-----------------
Let's start at the top. The demo command is in common/cmd_demo.c. It does
the usual command processing and then:
struct udevice *demo_dev;
ret = uclass_get_device(UCLASS_DEMO, devnum, &demo_dev);
UCLASS_DEMO means the class of devices which implement 'demo'. Other
classes might be MMC, or GPIO, hashing or serial. The idea is that the
devices in the class all share a particular way of working. The class
presents a unified view of all these devices to U-Boot.
This function looks up a device for the demo uclass. Given a device
number we can find the device because all devices have registered with
the UCLASS_DEMO uclass.
The device is automatically activated ready for use by uclass_get_device().
Now that we have the device we can do things like:
return demo_hello(demo_dev, ch);
This function is in the demo uclass. It takes care of calling the 'hello'
method of the relevant driver. Bearing in mind that there are two drivers,
this particular device may use one or other of them.
The code for demo_hello() is in drivers/demo/demo-uclass.c:
int demo_hello(struct udevice *dev, int ch)
{
const struct demo_ops *ops = device_get_ops(dev);
if (!ops->hello)
return -ENOSYS;
return ops->hello(dev, ch);
}
As you can see it just calls the relevant driver method. One of these is
in drivers/demo/demo-simple.c:
static int simple_hello(struct udevice *dev, int ch)
{
const struct dm_demo_pdata *pdata = dev_get_platdata(dev);
printf("Hello from %08x: %s %d\n", map_to_sysmem(dev),
pdata->colour, pdata->sides);
return 0;
}
So that is a trip from top (command execution) to bottom (driver action)
but it leaves a lot of topics to address.
Declaring Drivers
-----------------
A driver declaration looks something like this (see
drivers/demo/demo-shape.c):
static const struct demo_ops shape_ops = {
.hello = shape_hello,
.status = shape_status,
};
U_BOOT_DRIVER(demo_shape_drv) = {
.name = "demo_shape_drv",
.id = UCLASS_DEMO,
.ops = &shape_ops,
.priv_data_size = sizeof(struct shape_data),
};
This driver has two methods (hello and status) and requires a bit of
private data (accessible through dev_get_priv(dev) once the driver has
been probed). It is a member of UCLASS_DEMO so will reg
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
嵌入式优质项目,资源经过严格测试可直接运行成功且功能正常的情况才上传,可轻松copy复刻,拿到资料包后可轻松复现出一样的项目。 本人单片机开发经验充足,深耕嵌入式领域,有任何使用问题欢迎随时与我联系,我会及时为你解惑,提供帮助。 【资源内容】:包含完整源码+工程文件+说明,项目具体内容可查看下方的资源详情。 【附带帮助】: 若还需要嵌入式物联网单片机相关领域开发工具、学习资料等,我会提供帮助,提供资料,鼓励学习进步。 【本人专注嵌入式领域】: 有任何使用问题欢迎随时与我联系,我会及时解答,第一时间为你提供帮助,CSDN博客端可私信,为你解惑,欢迎交流。 【建议小白】: 在所有嵌入式开发中硬件部分若不会画PCB/电路,可选择根据引脚定义将其代替为面包板+杜邦线+外设模块的方式,只需轻松简单连线,下载源码烧录进去便可轻松复刻出一样的项目 【适合场景】: 相关项目设计中,皆可应用在项目开发、毕业设计、课程设计、期末/期中/大作业、工程实训、大创等学科竞赛比赛、初期项目立项、学习/练手等方面中 可借鉴此优质项目实现复刻,也可以基于此项目进行扩展来开发出更多功能
资源推荐
资源详情
资源评论
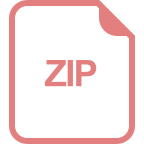
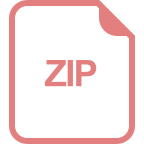
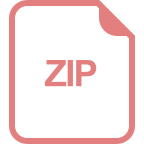
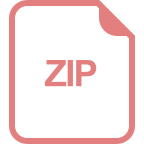
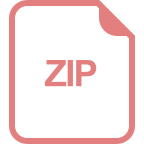
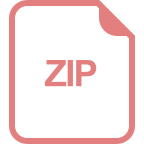
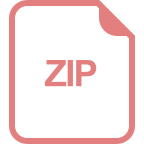
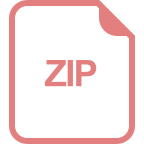
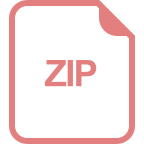
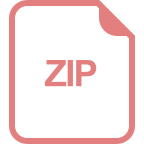
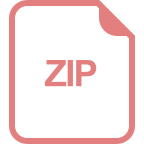
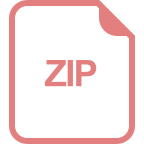
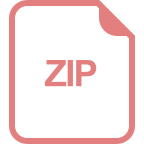
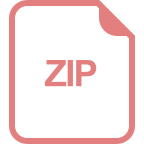
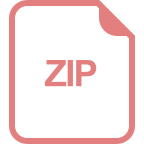
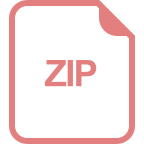
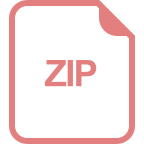
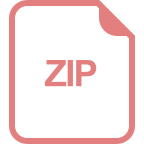
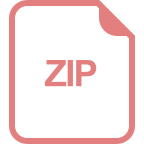
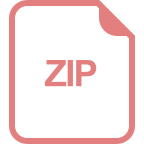
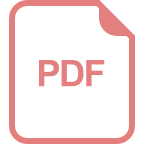
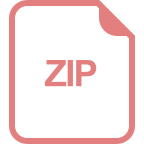
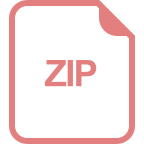
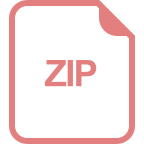
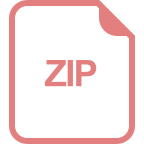
收起资源包目录

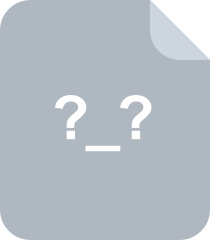
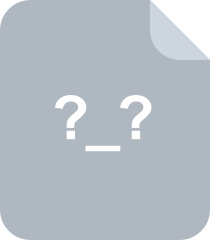
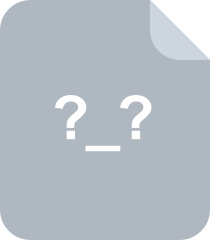
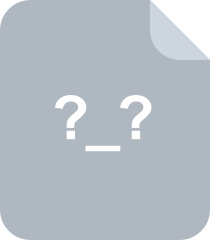
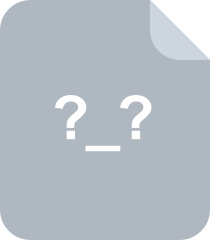
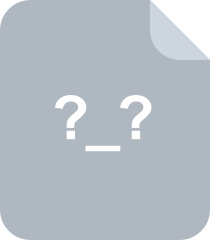
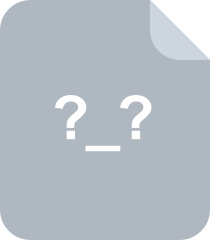
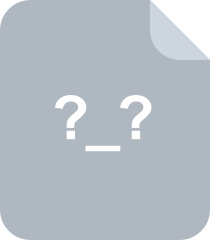
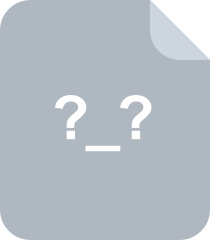
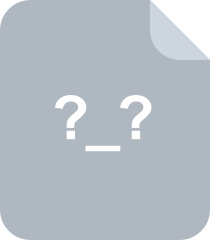
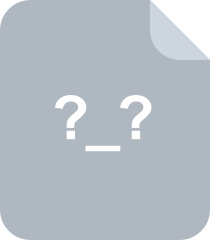
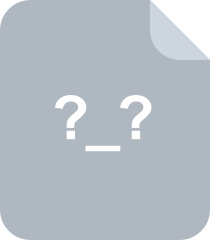
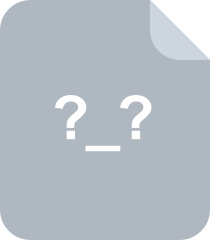
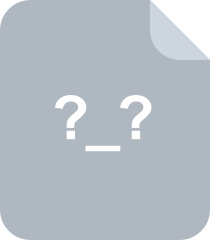
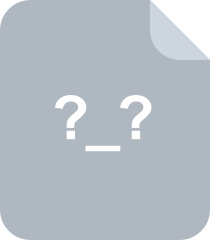
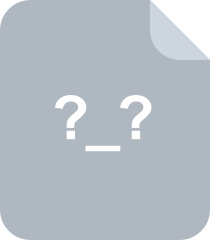
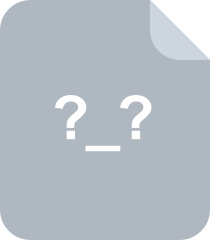
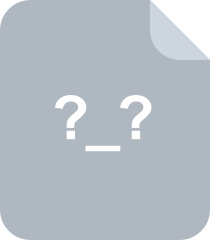
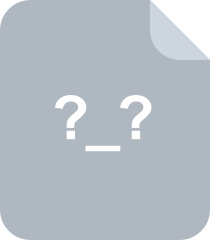
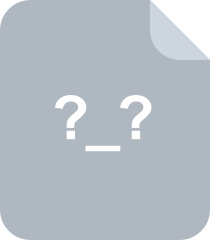
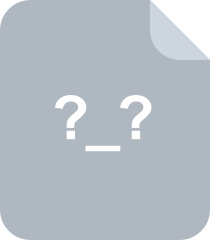
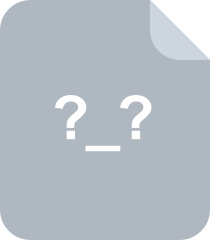
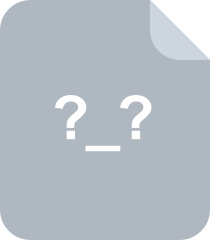
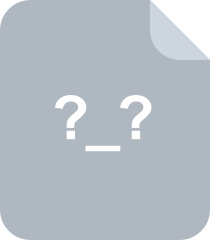
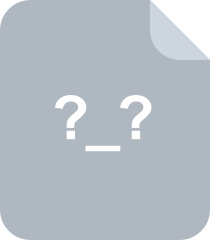
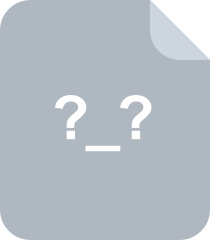
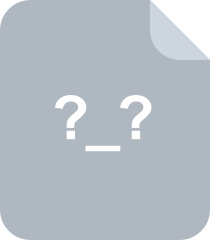
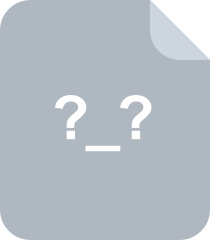
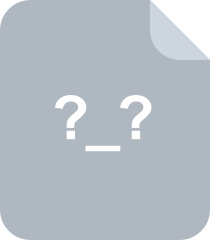
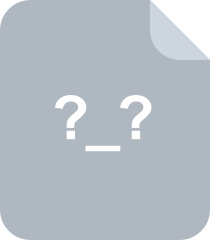
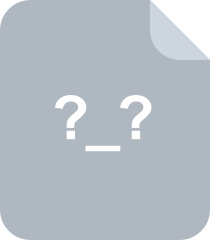
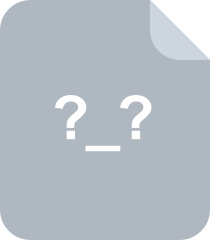
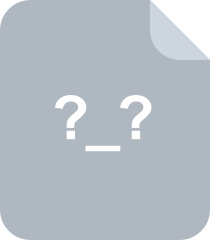
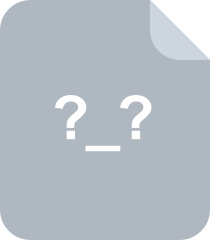
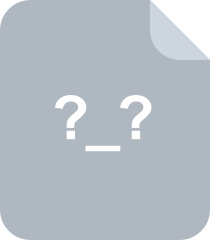
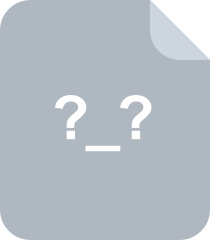
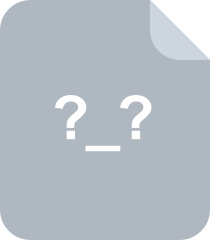
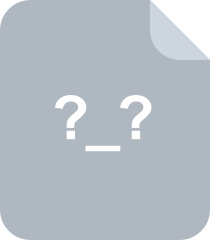
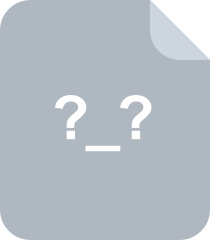
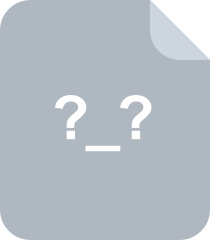
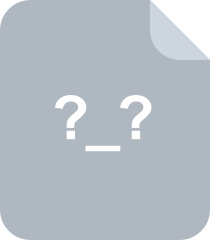
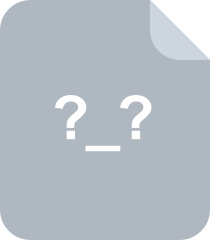
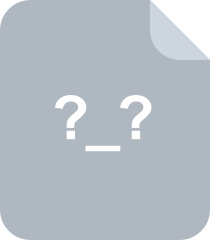
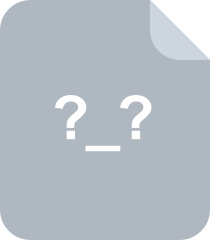
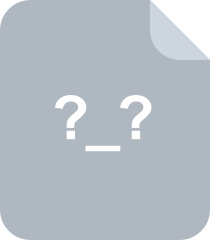
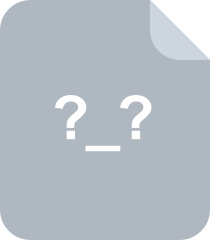
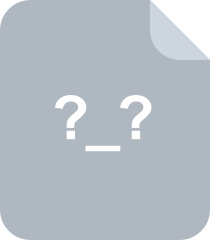
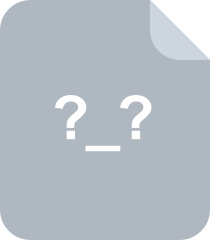
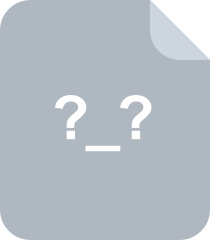
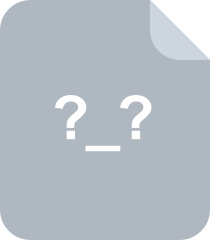
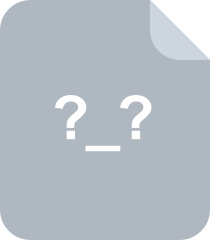
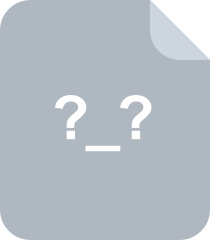
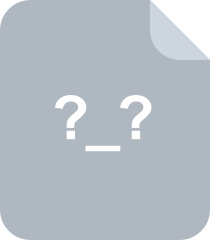
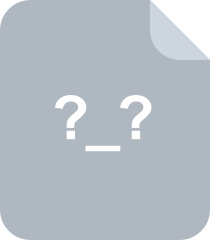
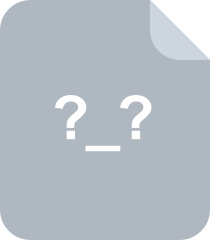
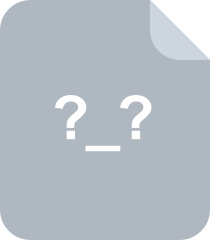
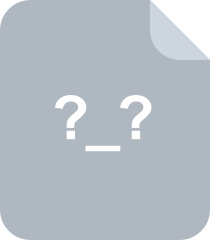
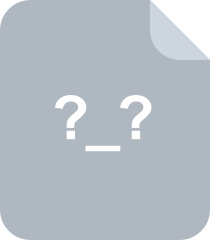
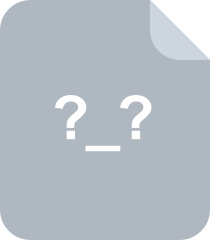
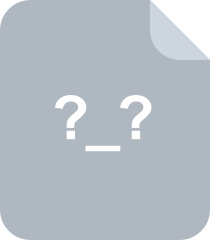
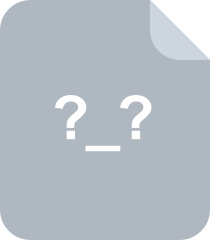
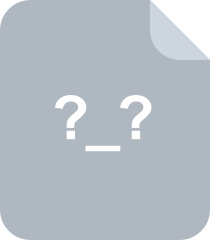
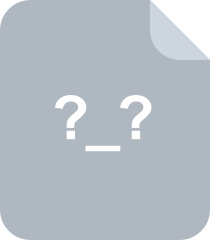
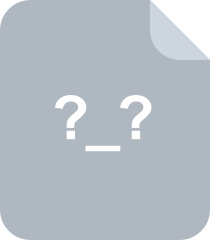
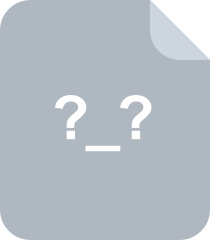
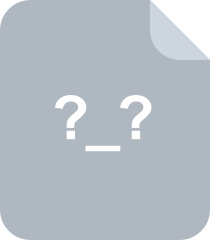
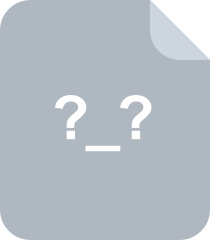
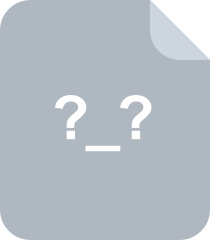
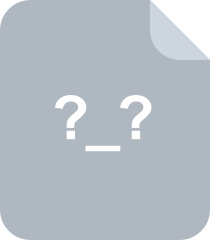
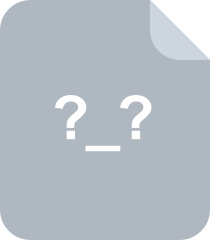
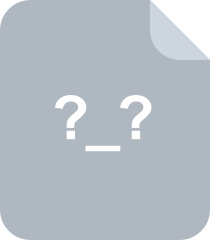
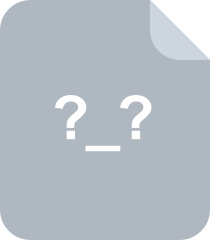
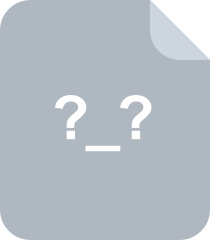
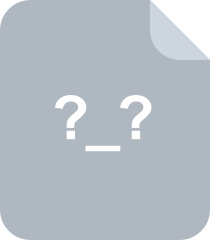
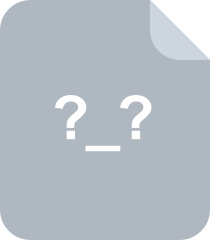
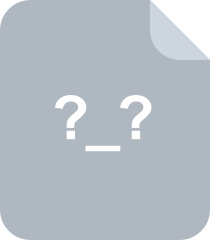
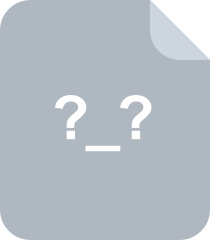
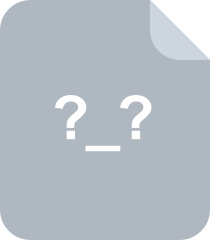
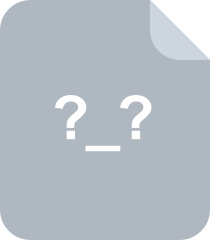
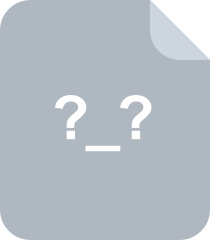
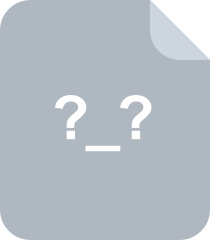
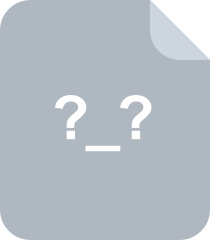
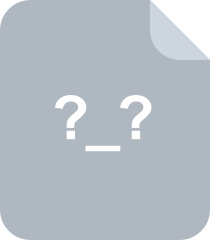
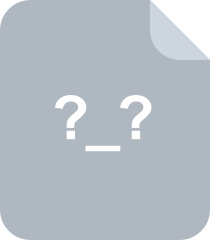
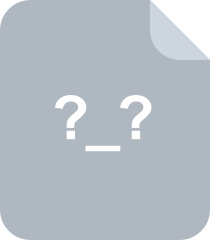
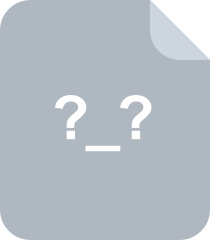
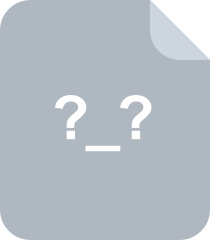
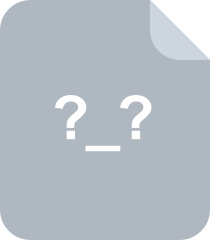
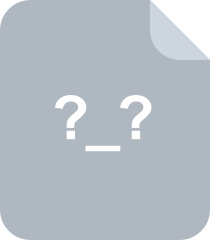
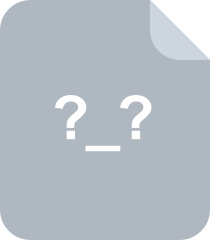
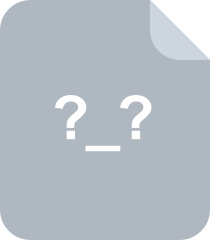
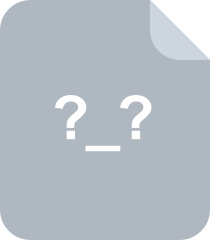
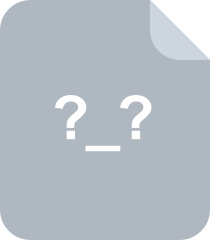
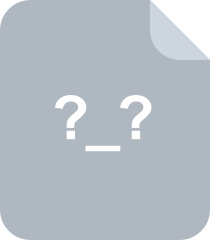
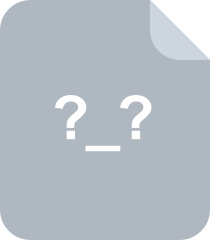
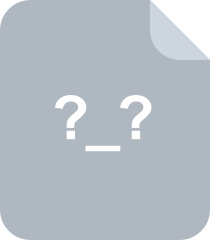
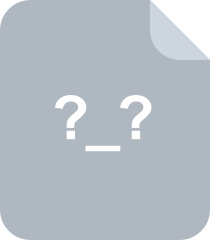
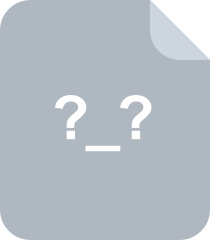
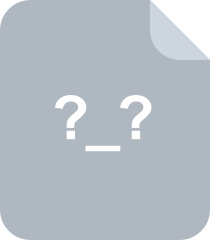
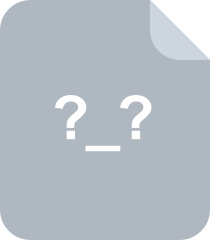
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
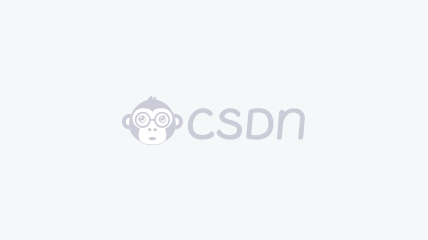

阿齐Archie
- 粉丝: 3w+
- 资源: 2474
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

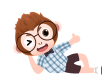
最新资源
- 白色扁平风格的银行业务企业网站源码下载.zip
- 白色扁平风格的新闻资讯娱乐整站网站源码下载.zip
- 白色扁平风格的优雅时尚英文整站网站源码下载.zip
- 白色扁平风格的阅读书店整站网站模板.zip
- 白色扁平风格的原创素材类企业网站源码下载.zip
- 白色扁平化的国际物流运输企业网站模板下载.zip
- 白色扁平化的绿色花艺花店企业网站模板下载.zip
- 白色扁平化的牛排汉堡西餐厅企业网站模板.zip
- 白色扁平化的网络博客整站网站模板下载.zip
- 白色扁平化风格的城市规划设计企业网站模板.rar
- 白色扁平化的在线课堂补习班模板下载.zip
- 白色扁平化风格的法律律师在线咨询企业网站模板.zip
- 白色扁平化风格的灯饰灯具销售企业网站模板.zip
- 白色扁平化风格的房地产开发公司模板下载.zip
- 白色扁平化风格的个人摄影博客模板下载.zip
- 白色扁平化风格的航空公司官网企业网站模板.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


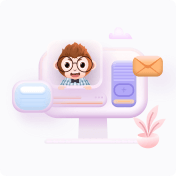
安全验证
文档复制为VIP权益,开通VIP直接复制
