/*! ----------------------------------------------------------------------------
* @file config_options.c
* @brief Configuration options are defined here.
*
* @attention
*
* Copyright 2019 - 2021 (c) Decawave Ltd, Dublin, Ireland.
*
* All rights reserved.
*
*/
#include "config_options.h"
/* String used to display measured distance on LCD screen (16 characters maximum). */
char dist_str[16] = { 0 };
/*
* TX Power Configuration Settings
*/
/* Values for the PG_DELAY and TX_POWER registers reflect the bandwidth and power of the spectrum at the current
* temperature. These values can be calibrated prior to taking reference measurements. */
dwt_txconfig_t txconfig_options = {
0x34, /* PG delay. */
0xfdfdfdfd, /* TX power. */
0x0 /*PG count*/
};
dwt_txconfig_t txconfig_options_ch9 = {
0x34, /* PG delay. */
0xfefefefe, /* TX power. */
0x0 /*PG count*/
};
/*
* Configuration options for the following parameters:
* Channel: 5, 9
* PRF: 64
* Preamble Length: 64, 128, 512, 1024
* Preamble Code: 3/4 for 16MHz PRf, 9/10/11/12 for 64MHz PRF
* Data Rate: 0.85, 6.8
* STS: Length 64
*/
#ifdef CONFIG_OPTION_01
/* Configuration option 01.
* Channel 5, PRF 64M, Preamble Length 64, PAC 8, Preamble code 9, Data Rate 850k, STS Length 64
*/
dwt_config_t config_options = {
5, /* Channel number. */
DWT_PLEN_64, /* Preamble length. Used in TX only. */
DWT_PAC8, /* Preamble acquisition chunk size. Used in RX only. */
9, /* TX preamble code. Used in TX only. */
9, /* RX preamble code. Used in RX only. */
3, /* 0 to use standard 8 symbol SFD, 1 to use non-standard 8 symbol, 2 for non-standard 16 symbol SFD and 3 for 4z 8 symbol SDF type */
DWT_BR_850K, /* Data rate. */
DWT_PHRMODE_STD, /* PHY header mode. */
DWT_PHRRATE_STD, /* PHY header rate. */
(64 + 1 + 8 - 8), /* SFD timeout (preamble length + 1 + SFD length - PAC size). Used in RX only. */
DWT_STS_MODE_1, /* Mode 1 STS enabled */
DWT_STS_LEN_64, /* STS length*/
DWT_PDOA_M0 /* PDOA mode off */
};
#endif
#ifdef CONFIG_OPTION_02
/* Configuration option 02.
* Channel 9, PRF 64M, Preamble Length 64, PAC 8, Preamble code 9, Data Rate 850k, STS Length 64
*/
dwt_config_t config_options = {
9, /* Channel number. */
DWT_PLEN_64, /* Preamble length. Used in TX only. */
DWT_PAC8, /* Preamble acquisition chunk size. Used in RX only. */
9, /* TX preamble code. Used in TX only. */
9, /* RX preamble code. Used in RX only. */
3, /* 0 to use standard 8 symbol SFD, 1 to use non-standard 8 symbol, 2 for non-standard 16 symbol SFD and 3 for 4z 8 symbol SDF type */
DWT_BR_850K, /* Data rate. */
DWT_PHRMODE_STD, /* PHY header mode. */
DWT_PHRRATE_STD, /* PHY header rate. */
(64 + 1 + 8 - 8), /* SFD timeout (preamble length + 1 + SFD length - PAC size). Used in RX only. */
DWT_STS_MODE_1, /* Mode 1 STS enabled */
DWT_STS_LEN_64, /* STS length*/
DWT_PDOA_M0 /* PDOA mode off */
};
#endif
#ifdef CONFIG_OPTION_03
/* Configuration option 03.
* Channel 5, PRF 64M, Preamble Length 128, PAC 8, Preamble code 9, Data Rate 850k, STS Length 64
*/
dwt_config_t config_options = {
5, /* Channel number. */
DWT_PLEN_128, /* Preamble length. Used in TX only. */
DWT_PAC8, /* Preamble acquisition chunk size. Used in RX only. */
9, /* TX preamble code. Used in TX only. */
9, /* RX preamble code. Used in RX only. */
3, /* 0 to use standard 8 symbol SFD, 1 to use non-standard 8 symbol, 2 for non-standard 16 symbol SFD and 3 for 4z 8 symbol SDF type */
DWT_BR_850K, /* Data rate. */
DWT_PHRMODE_STD, /* PHY header mode. */
DWT_PHRRATE_STD, /* PHY header rate. */
(128 + 1 + 8 - 8), /* SFD timeout (preamble length + 1 + SFD length - PAC size). Used in RX only. */
DWT_STS_MODE_1, /* Mode 1 STS enabled */
DWT_STS_LEN_64, /* STS length*/
DWT_PDOA_M0 /* PDOA mode off */
};
#endif
#ifdef CONFIG_OPTION_04
/* Configuration option 04.
* Channel 9, PRF 64M, Preamble Length 128, PAC 8, Preamble code 9, Data Rate 850k, STS Length 64
*/
dwt_config_t config_options = {
9, /* Channel number. */
DWT_PLEN_128, /* Preamble length. Used in TX only. */
DWT_PAC8, /* Preamble acquisition chunk size. Used in RX only. */
9, /* TX preamble code. Used in TX only. */
9, /* RX preamble code. Used in RX only. */
3, /* 0 to use standard 8 symbol SFD, 1 to use non-standard 8 symbol, 2 for non-standard 16 symbol SFD and 3 for 4z 8 symbol SDF type */
DWT_BR_850K, /* Data rate. */
DWT_PHRMODE_STD, /* PHY header mode. */
DWT_PHRRATE_STD, /* PHY header rate. */
(128 + 1 + 8 - 8), /* SFD timeout (preamble length + 1 + SFD length - PAC size). Used in RX only. */
DWT_STS_MODE_1, /* Mode 1 STS enabled */
DWT_STS_LEN_64, /* STS length*/
DWT_PDOA_M0 /* PDOA mode off */
};
#endif
#ifdef CONFIG_OPTION_05
/* Configuration option 05.
* Channel 5, PRF 64M, Preamble Length 512, PAC 8, Preamble code 9, Data Rate 850k, STS Length 64
*/
dwt_config_t config_options = {
5, /* Channel number. */
DWT_PLEN_512, /* Preamble length. Used in TX only. */
DWT_PAC8, /* Preamble acquisition chunk size. Used in RX only. */
9, /* TX preamble code. Used in TX only. */
9, /* RX preamble code. Used in RX only. */
3, /* 0 to use standard 8 symbol SFD, 1 to use non-standard 8 symbol, 2 for non-standard 16 symbol SFD and 3 for 4z 8 symbol SDF type */
DWT_BR_850K, /* Data rate. */
DWT_PHRMODE_STD, /* PHY header mode. */
DWT_PHRRATE_STD, /* PHY header rate. */
(512 + 1 + 8 - 8), /* SFD timeout (preamble length + 1 + SFD length - PAC size). Used in RX only. */
DWT_STS_MODE_1, /* Mode 1 STS enabled */
DWT_STS_LEN_64, /* STS length*/
DWT_PDOA_M0 /* PDOA mode off */
};
#endif
#ifdef CONFIG_OPTION_06
/* Configuration option 06.
* Channel 9, PRF 64M, Preamble Length 512, PAC 8, Preamble code 9, Data Rate 850k, STS Length 64
*/
dwt_config_t config_options = {
9, /* Channel number. */
DWT_PLEN_512, /* Preamble length. Used in TX only. */
DWT_PAC8, /* Preamble acquisition chunk size. Used in RX only. */
9, /* TX preamble code. Used in TX only. */
9, /* RX preamble code. Used in RX only. */
3, /* 0 to use standard 8 symbol SFD, 1 to use non-standard 8 symbol, 2 for non-standard 16 symbol SFD and 3 for 4z 8 symbol SDF type */
DWT_BR_850K, /* Data rate. */
DWT_PHRMODE_STD, /* PHY header mode. */
DWT_PHRRATE_STD, /* PHY header rate. */
(512 + 1 + 8 - 8), /* SFD timeout (preamble length + 1 + SFD length - PAC size). Used in RX only. */
DWT_STS_MODE_1, /* Mode 1 STS enabled */
DWT_STS_LEN_64, /* STS length*/
DWT_PDOA_M0 /* PDOA mode off */
};
#endif
#ifdef CONFIG_OPTION_07
/* Configuration option 07.
* Channel 5, PRF 64M, Preamble Length 1024, PAC 8, Preamble code 9, Data Rate 850k, STS Length 64
*/
dwt_config_t config_options = {
5, /* Channel number. */
DWT_PLEN_1024, /* Preamble length. Used in TX only. */
DWT_PAC8, /* Preamble acquisition chunk size. Used in RX only. */
9, /* TX preamble code. Used in TX only. */
9, /* RX preamble code. Used in RX only. */
3, /* 0 to use standard 8 symbol SFD, 1 to use non-standard 8 symbol, 2 for non-standard 16 symbol SFD and 3 for 4z
没有合适的资源?快使用搜索试试~ 我知道了~
DW3000移植到Keil的驱动代码
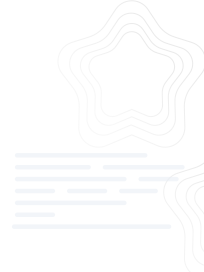
共78个文件
c:60个
h:14个
a:4个

需积分: 0 2 下载量 120 浏览量
2024-02-27
16:12:47
上传
评论
收藏 443KB RAR 举报
温馨提示
DW3000移植到Keil的驱动代码
资源推荐
资源详情
资源评论
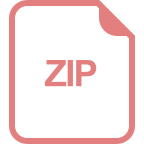
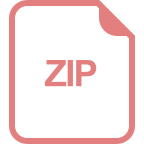
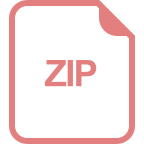
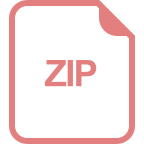
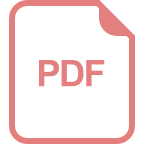
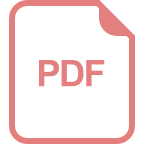
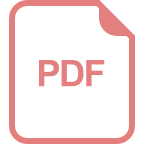
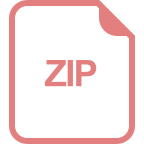
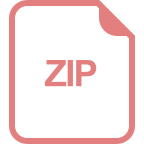
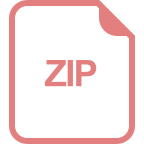
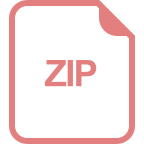
收起资源包目录


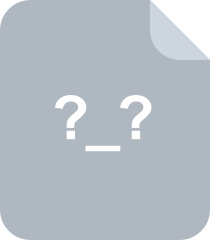
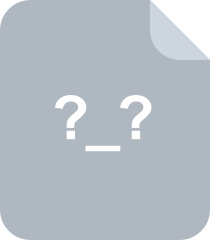
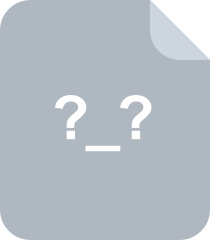


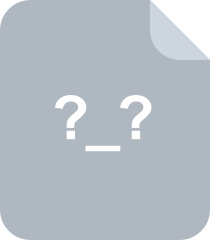
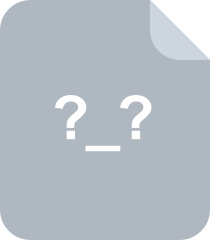
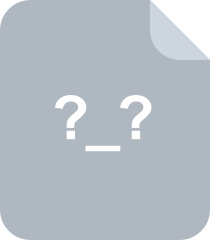
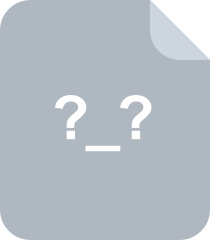

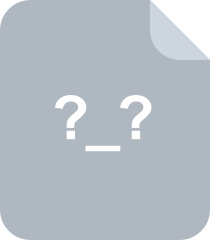
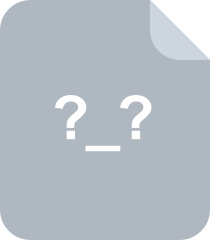
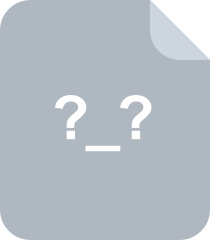
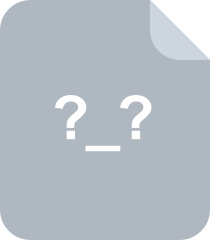

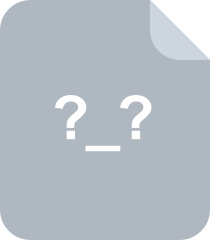

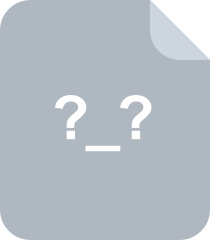
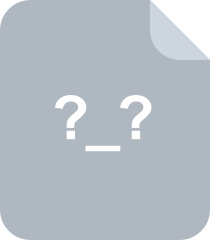

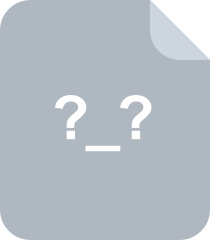
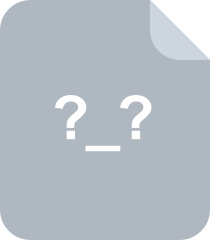


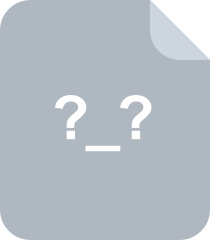

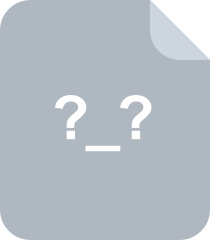

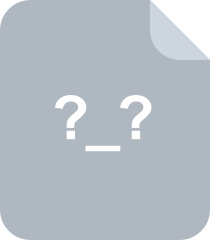

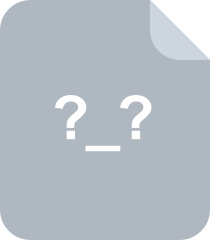

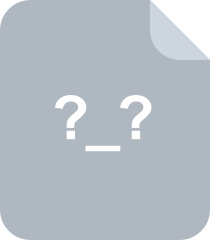
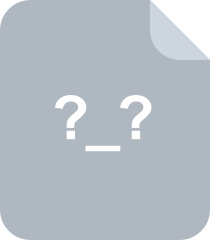
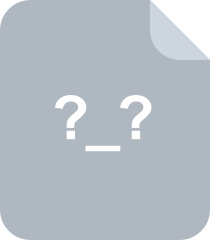

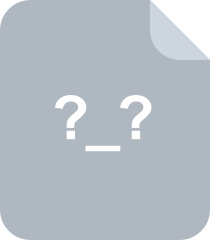
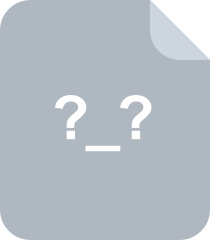
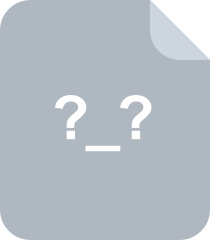

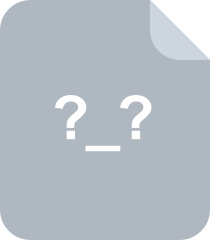

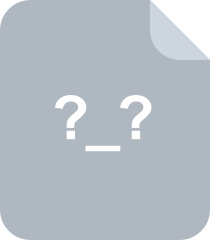

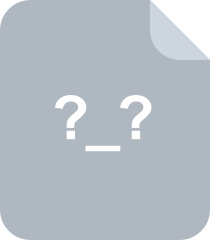

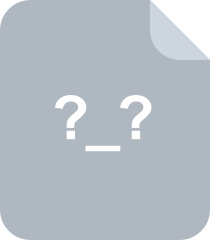

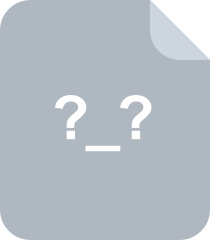

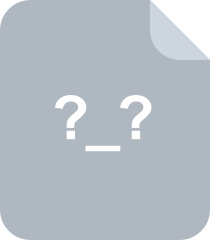

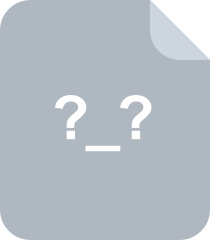
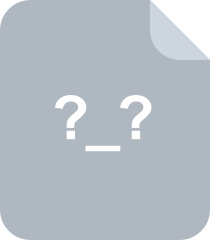

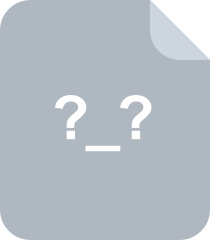

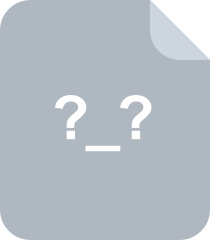

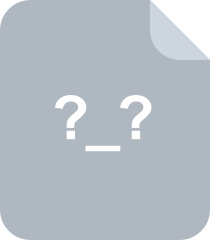
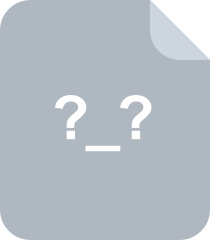
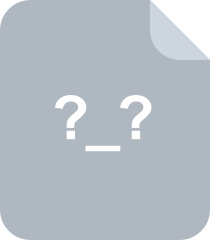

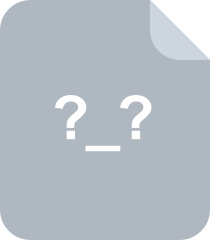

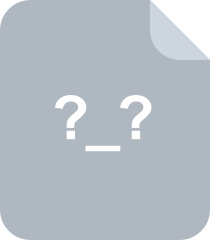

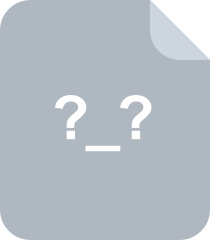

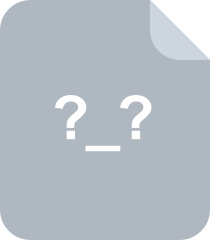

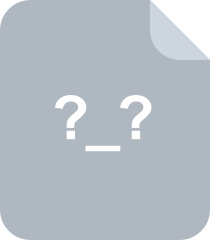

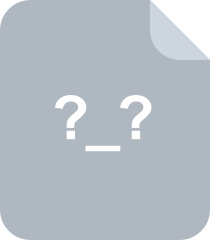

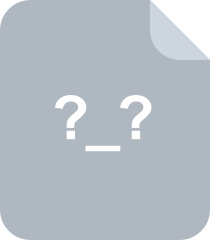

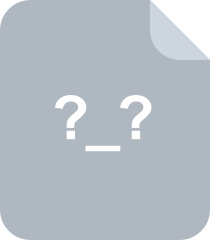

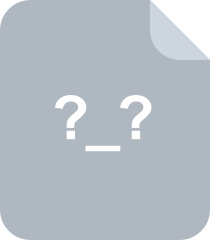
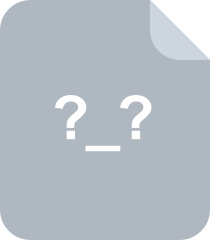

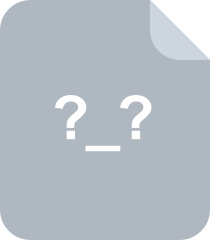

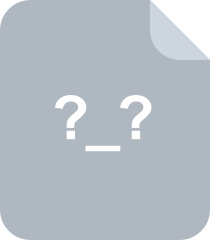

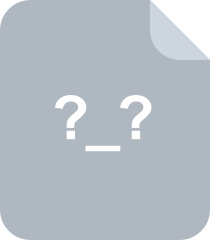

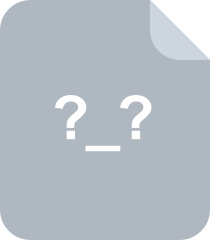

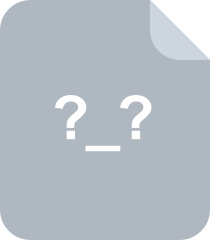

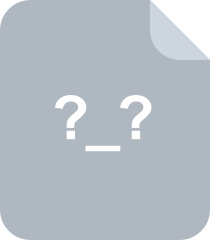
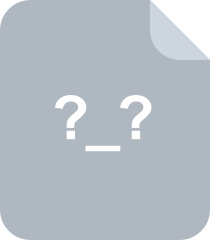

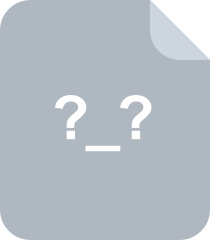

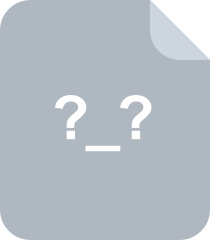
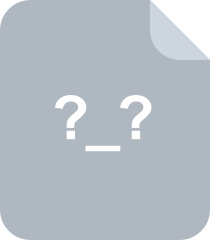

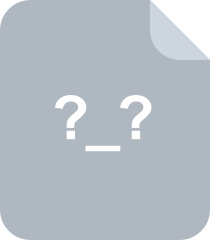

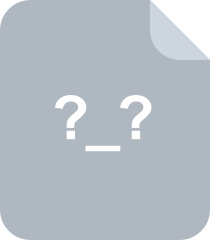

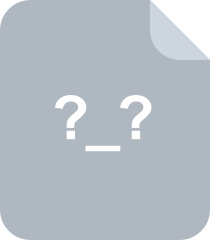

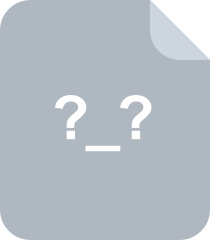
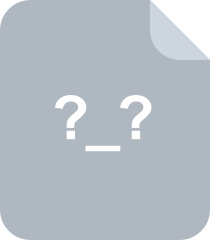

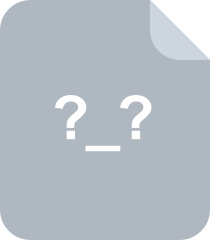

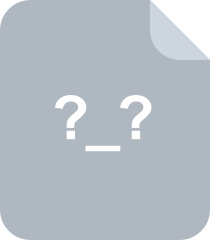

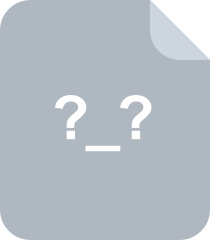

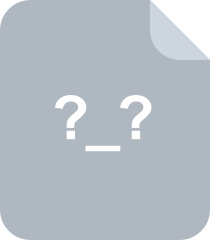

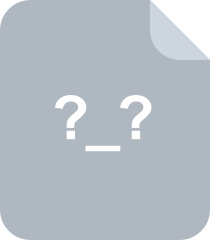

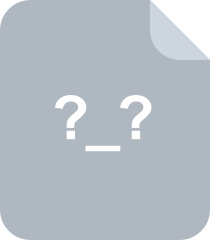
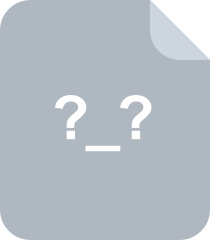
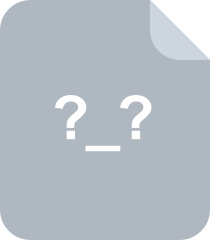
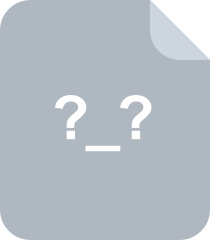
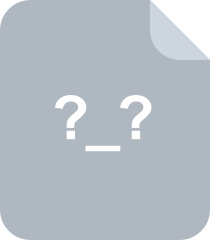
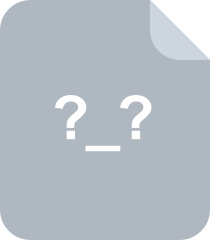
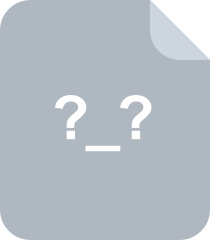
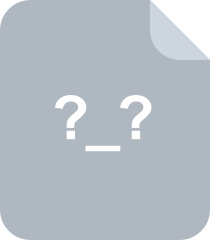
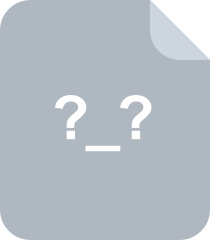
共 78 条
- 1
资源评论
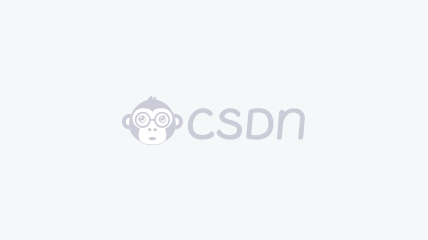

BIGGGG5718
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

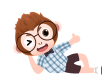
安全验证
文档复制为VIP权益,开通VIP直接复制
