`PhpZip`
========
`PhpZip` is a php-library for extended work with ZIP-archives.
[](https://travis-ci.org/Ne-Lexa/php-zip)
[](https://scrutinizer-ci.com/g/Ne-Lexa/php-zip/?branch=master)
[](https://packagist.org/packages/nelexa/zip)
[](https://packagist.org/packages/nelexa/zip)
[](https://php.net/)
[](https://packagist.org/packages/nelexa/zip)
[Russian Documentation](README.RU.md)
Table of contents
-----------------
- [Features](#Features)
- [Requirements](#Requirements)
- [Installation](#Installation)
- [Examples](#Examples)
- [Glossary](#Glossary)
- [Documentation](#Documentation)
+ [Overview of methods of the class `\PhpZip\ZipFile`](#Documentation-Overview)
+ [Creation/Opening of ZIP-archive](#Documentation-Open-Zip-Archive)
+ [Reading entries from the archive](#Documentation-Open-Zip-Entries)
+ [Iterating entries](#Documentation-Zip-Iterate)
+ [Getting information about entries](#Documentation-Zip-Info)
+ [Adding entries to the archive](#Documentation-Add-Zip-Entries)
+ [Deleting entries from the archive](#Documentation-Remove-Zip-Entries)
+ [Working with entries and archive](#Documentation-Entries)
+ [Working with passwords](#Documentation-Password)
+ [zipalign - alignment tool for Android (APK) files](#Documentation-ZipAlign-Usage)
+ [Undo changes](#Documentation-Unchanged)
+ [Saving a file or output to a browser](#Documentation-Save-Or-Output-Entries)
+ [Closing the archive](#Documentation-Close-Zip-Archive)
- [Running the tests](#Running-Tests)
- [Changelog](#Changelog)
- [Upgrade](#Upgrade)
+ [Upgrade version 2 to version 3.0](#Upgrade-v2-to-v3)
### <a name="Features"></a> Features
- Opening and unzipping zip files.
- Creating ZIP-archives.
- Modifying ZIP archives.
- Pure php (not require extension `php-zip` and class `\ZipArchive`).
- It supports saving the archive to a file, outputting the archive to the browser, or outputting it as a string without saving it to a file.
- Archival comments and comments of individual entry are supported.
- Get information about each entry in the archive.
- Only the following compression methods are supported:
+ No compressed (Stored).
+ Deflate compression.
+ BZIP2 compression with the extension `php-bz2`.
- Support for `ZIP64` (file size is more than 4 GB or the number of entries in the archive is more than 65535).
- Built-in support for aligning the archive to optimize Android packages (APK) [`zipalign`](https://developer.android.com/studio/command-line/zipalign.html).
- Working with passwords for PHP 5.5
> **Attention!**
>
> For 32-bit systems, the `Traditional PKWARE Encryption (ZipCrypto)` encryption method is not currently supported.
> Use the encryption method `WinZIP AES Encryption`, whenever possible.
+ Set the password to read the archive for all entries or only for some.
+ Change the password for the archive, including for individual entries.
+ Delete the archive password for all or individual entries.
+ Set the password and/or the encryption method, both for all, and for individual entries in the archive.
+ Set different passwords and encryption methods for different entries.
+ Delete the password for all or some entries.
+ Support `Traditional PKWARE Encryption (ZipCrypto)` and `WinZIP AES Encryption` encryption methods.
+ Set the encryption method for all or individual entries in the archive.
### <a name="Requirements"></a> Requirements
- `PHP` >= 5.5 (preferably 64-bit).
- Optional php-extension `bzip2` for BZIP2 compression.
- Optional php-extension `openssl` or `mcrypt` for `WinZip Aes Encryption` support.
### <a name="Installation"></a> Installation
`composer require nelexa/zip`
Latest stable version: [](https://packagist.org/packages/nelexa/zip)
### <a name="Examples"></a> Examples
```php
// create new archive
$zipFile = new \PhpZip\ZipFile();
try{
$zipFile
->addFromString('zip/entry/filename', 'Is file content') // add an entry from the string
->addFile('/path/to/file', 'data/tofile') // add an entry from the file
->addDir(__DIR__, 'to/path/') // add files from the directory
->saveAsFile($outputFilename) // save the archive to a file
->close(); // close archive
// open archive, extract, add files, set password and output to browser.
$zipFile
->openFile($outputFilename) // open archive from file
->extractTo($outputDirExtract) // extract files to the specified directory
->deleteFromRegex('~^\.~') // delete all hidden (Unix) files
->addFromString('dir/file.txt', 'Test file') // add a new entry from the string
->setPassword('password') // set password for all entries
->outputAsAttachment('library.jar'); // output to the browser without saving to a file
}
catch(\PhpZip\Exception\ZipException $e){
// handle exception
}
finally{
$zipFile->close();
}
```
Other examples can be found in the `tests/` folder
### <a name="Glossary"></a> Glossary
**Zip Entry** - file or folder in a ZIP-archive. Each entry in the archive has certain properties, for example: file name, compression method, encryption method, file size before compression, file size after compression, CRC32 and others.
### <a name="Documentation"></a> Documentation:
#### <a name="Documentation-Overview"></a> Overview of methods of the class `\PhpZip\ZipFile`
- [ZipFile::__construct](#Documentation-ZipFile-__construct) - initializes the ZIP archive.
- [ZipFile::addAll](#Documentation-ZipFile-addAll) - adds all entries from an array.
- [ZipFile::addDir](#Documentation-ZipFile-addDir) - adds files to the archive from the directory on the specified path without subdirectories.
- [ZipFile::addDirRecursive](#Documentation-ZipFile-addDirRecursive) - adds files to the archive from the directory on the specified path with subdirectories.
- [ZipFile::addEmptyDir](#Documentation-ZipFile-addEmptyDir) - add a new directory.
- [ZipFile::addFile](#Documentation-ZipFile-addFile) - adds a file to a ZIP archive from the given path.
- [ZipFile::addSplFile](#Documentation-ZipFile-addSplFile) - adds a `\SplFileInfo` to a ZIP archive.
- [ZipFile::addFromFinder](#Documentation-ZipFile-addFromFinder) - adds files from the `Symfony\Component\Finder\Finder` to a ZIP archive.
- [ZipFile::addFilesFromIterator](#Documentation-ZipFile-addFilesFromIterator) - adds files from the iterator of directories.
- [ZipFile::addFilesFromGlob](#Documentation-ZipFile-addFilesFromGlob) - adds files from a directory by glob pattern without subdirectories.
- [ZipFile::addFilesFromGlobRecursive](#Documentation-ZipFile-addFilesFromGlobRecursive) - adds files from a directory by glob pattern with subdirectories.
- [ZipFile::addFilesFromRegex](#Documentation-ZipFile-addFilesFromRegex) - adds files from a directory by PCRE pattern without subdirectories.
- [ZipFile::addFilesFromRegexRecursive](#Documentation-ZipFile-addFilesFromRegexRecursive) - adds files from a directory by PCRE pattern with subdirectories.
- [ZipFile::addFromStream](#Documentation-ZipFile-addFromStream) - adds a entry from the stream to the ZIP archive.
- [ZipFile::addFromString](#Documentation-ZipFile-addFromString) - adds a file to a ZIP archive using its contents.
- [ZipFile::close](#Documentation-ZipFile-close) - close the archive.
- [ZipFile::count](#Documentation-ZipFile-count) - returns the number of entries in the archive.
- [ZipFile::deleteFromName](#Documentation-ZipFile-deleteFromName) - deletes an entry in the archive using its name.
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
源支付V5版本今天找到了,源支付是什么想必就不用我来介绍了,你可以把它理解为只是改了个名字的码支付源码,功能跟码支付差不多,可以免签支付啥的。 程序的测试用的CentOS 7.6,Linux搭建Nginx环境,PHP7.2版,Mysql5.6版,还要安装一个扩展名为:Redis,我大致看了一下这5.0版本,他的后端UI样式跟3.0版本是完全不一样的,更加的美观了。
资源推荐
资源详情
资源评论
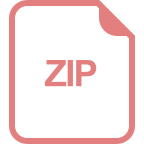
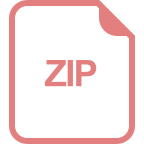
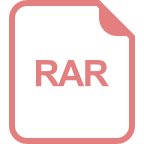
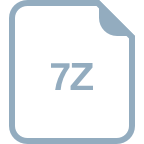
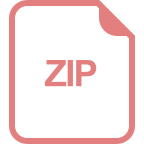
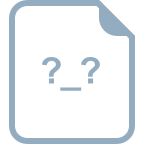
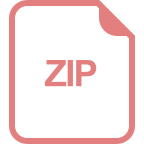
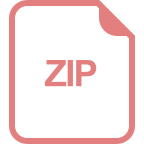
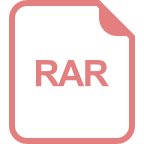
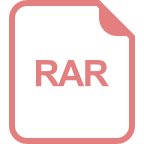
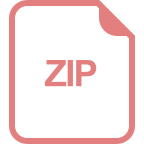
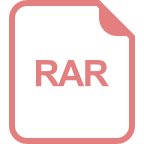
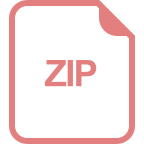
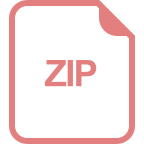
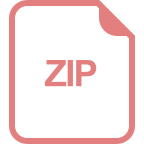
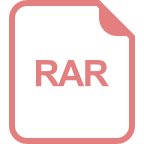
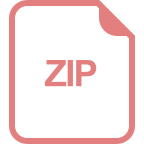
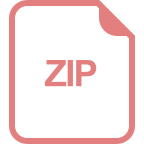
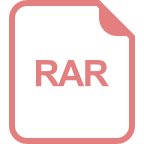
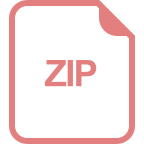
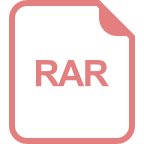
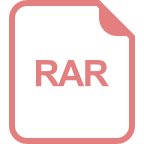
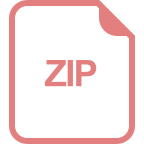
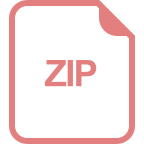
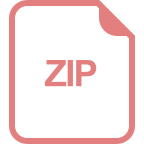
收起资源包目录

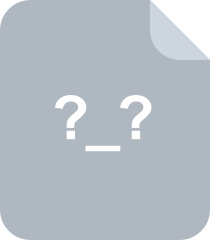
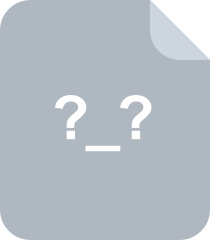
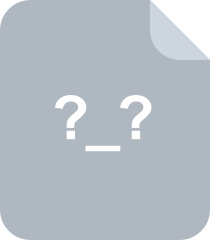
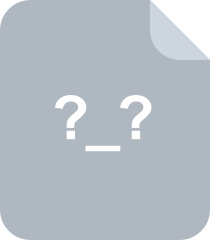
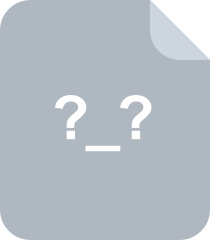
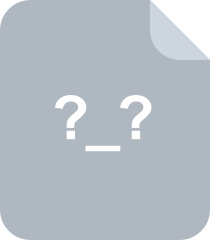
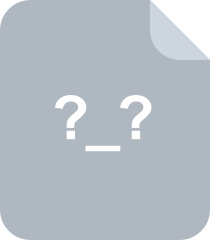
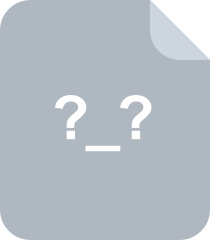
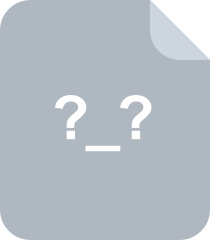
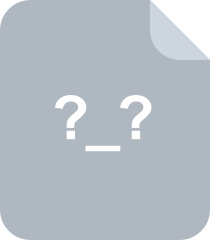
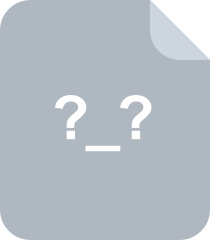
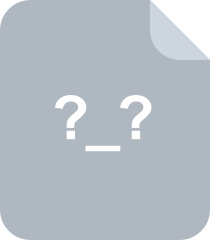
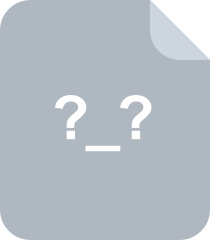
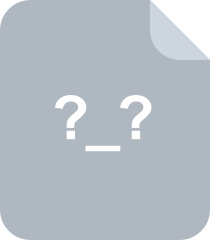
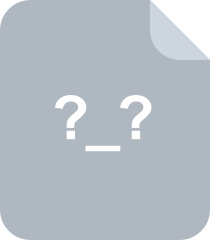
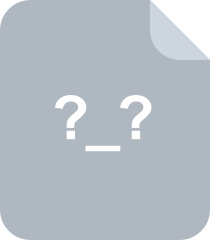
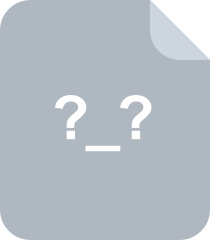
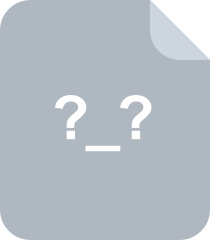
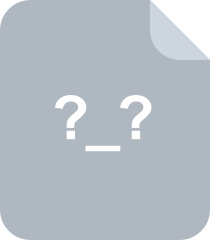
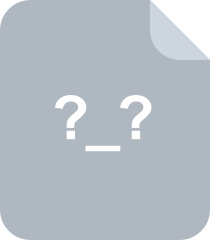
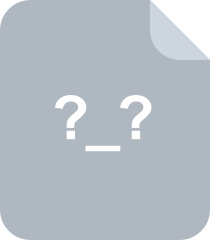
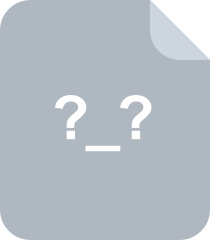
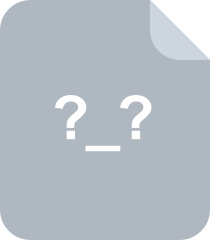
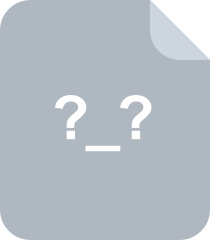
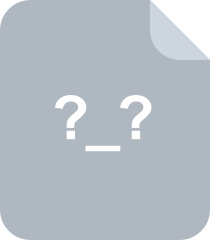
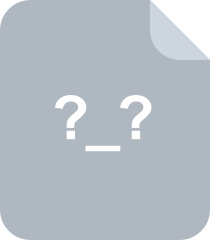
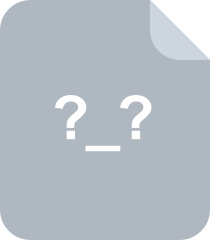
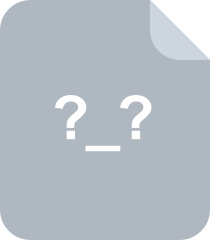
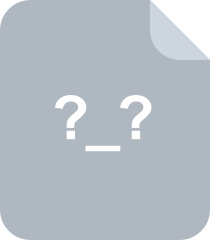
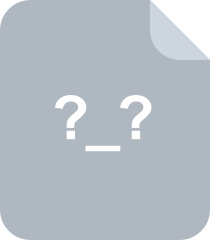
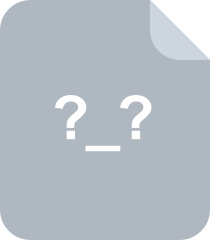
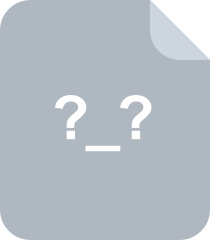
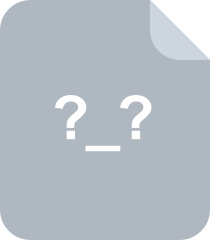
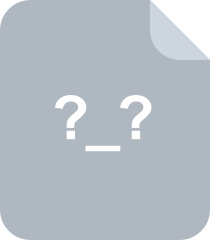
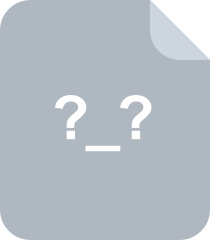
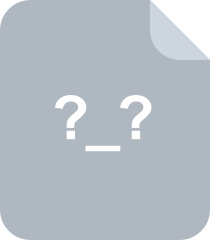
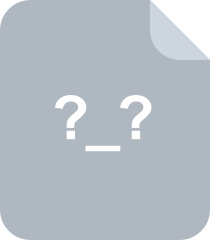
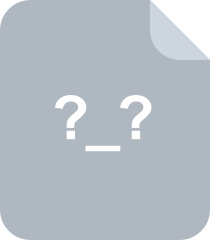
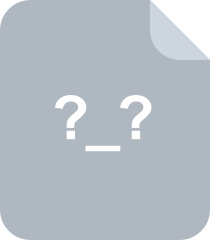
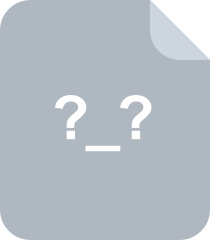
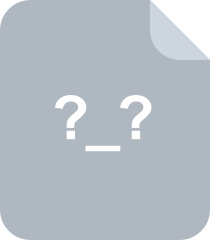
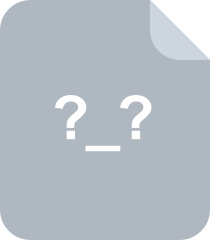
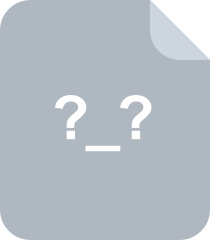
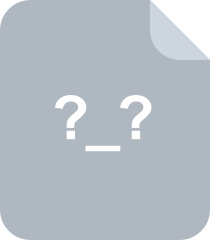
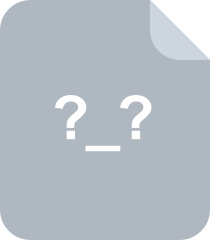
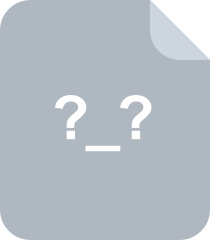
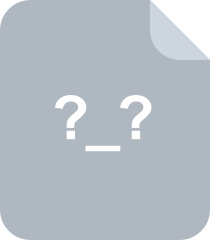
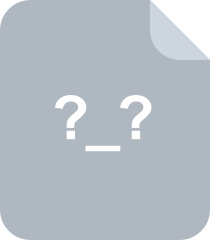
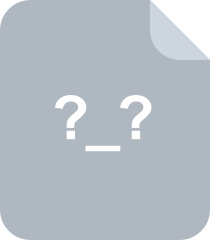
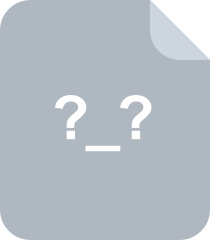
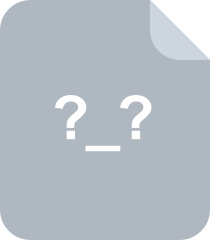
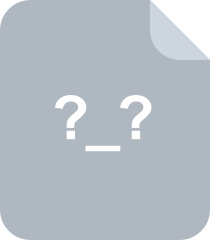
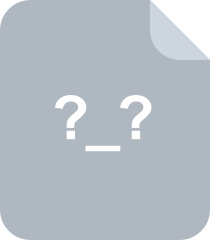
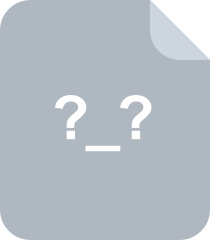
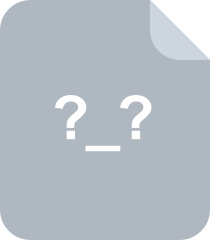
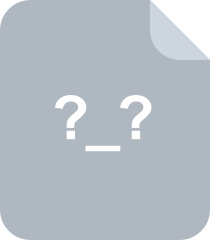
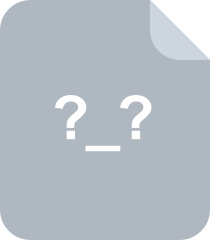
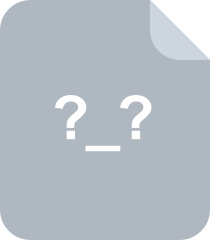
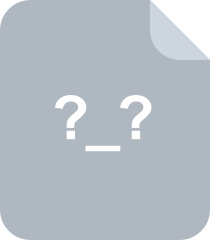
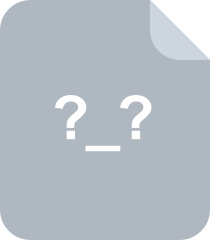
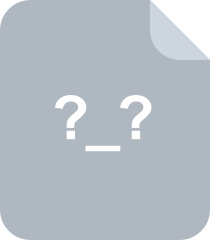
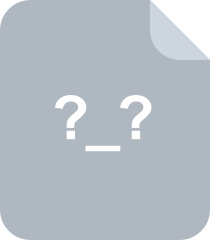
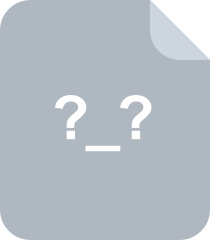
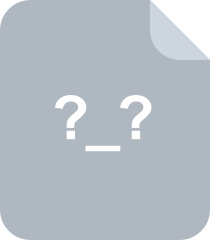
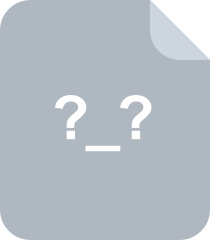
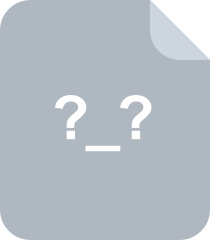
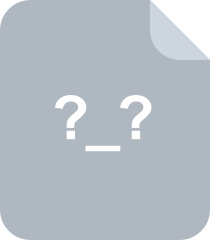
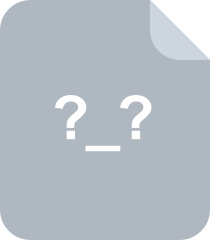
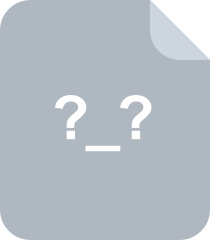
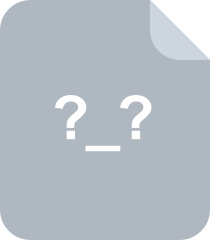
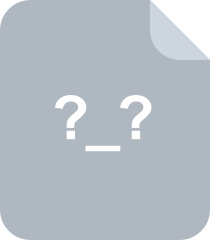
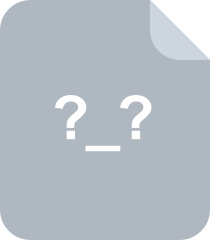
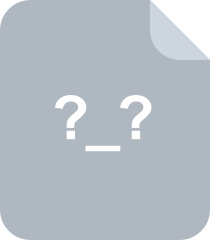
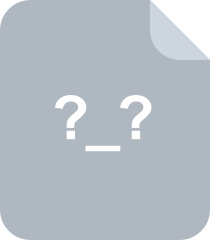
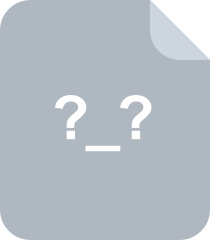
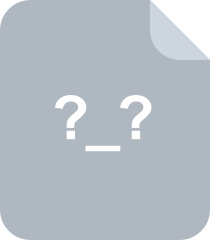
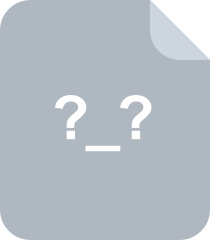
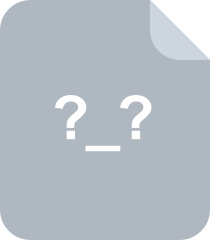
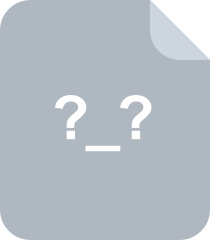
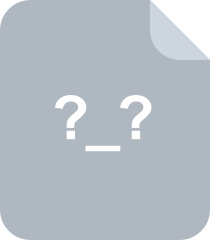
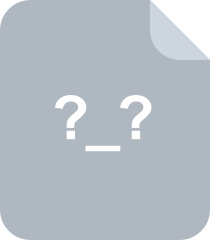
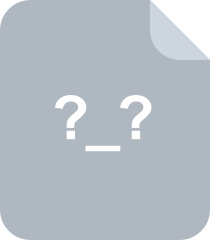
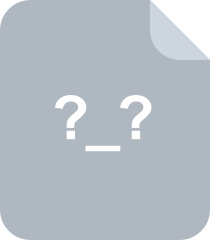
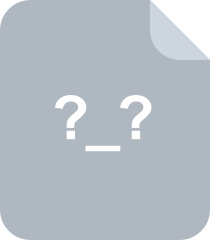
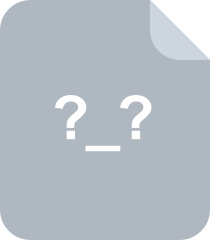
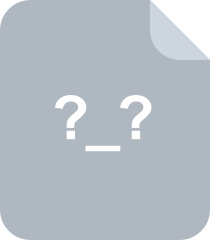
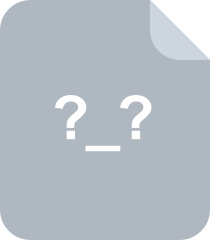
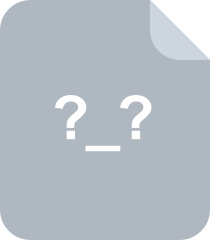
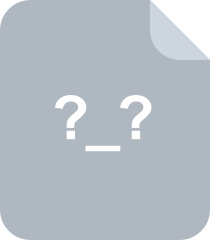
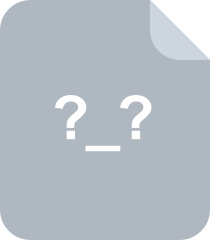
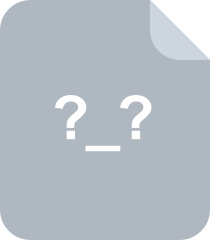
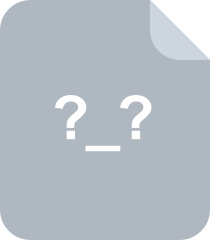
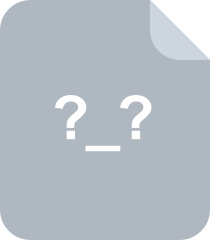
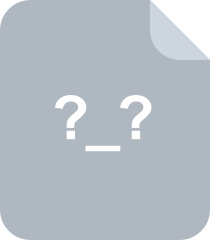
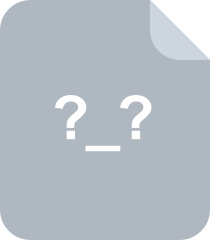
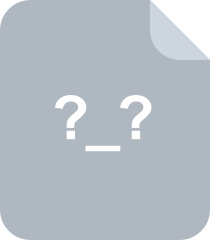
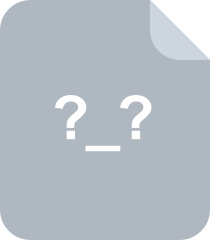
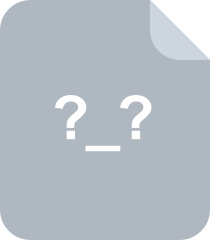
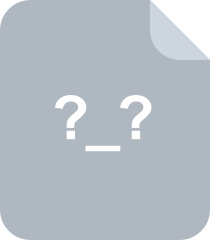
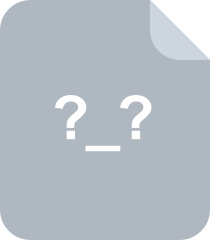
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
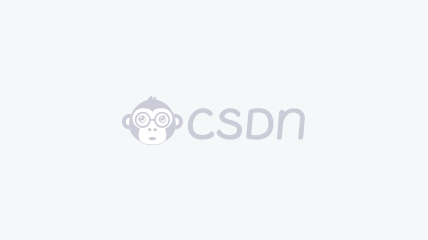

破碎的天堂鸟
- 粉丝: 8362
- 资源: 2285
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

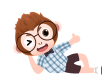
最新资源
- 【java毕业设计】仓储系统出入库模块设计源码(ssm+mysql+说明文档+LW).zip
- 最新整理!!!中国同一带一路国家海关进出口数据
- 【java毕业设计】毕业生生活用品出售网站系统源码(ssm+mysql+说明文档+LW).zip
- 最新整理-中国各、省、市、县、乡镇基尼系数数据(到2023年)
- 【java毕业设计】影院管理系统源码(ssm+mysql+说明文档+LW).zip
- Golang: 高效、简洁的Google开源编程语言
- 【java毕业设计】家用电器销售网站源码(ssm+mysql+说明文档+LW).zip
- 数维杯大学生数学建模竞赛概述及其价值解析
- 【java毕业设计】二手交易平台网站源码(ssm+mysql+说明文档+LW).zip
- HEVC Video Extensive
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


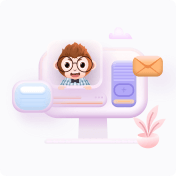
安全验证
文档复制为VIP权益,开通VIP直接复制
