MysqliDb -- Simple MySQLi wrapper and object mapper with prepared statements
<hr>
### Table of Contents
**[Initialization](#initialization)**
**[Objects mapping](#objects-mapping)**
**[Insert Query](#insert-query)**
**[Update Query](#update-query)**
**[Select Query](#select-query)**
**[Delete Query](#delete-query)**
**[Insert Data](#insert-data)**
**[Insert XML](#insert-xml)**
**[Pagination](#pagination)**
**[Running raw SQL queries](#running-raw-sql-queries)**
**[Query Keywords](#query-keywords)**
**[Where Conditions](#where--having-methods)**
**[Order Conditions](#ordering-method)**
**[Group Conditions](#grouping-method)**
**[Properties Sharing](#properties-sharing)**
**[Joining Tables](#join-method)**
**[Subqueries](#subqueries)**
**[EXISTS / NOT EXISTS condition](#exists--not-exists-condition)**
**[Has method](#has-method)**
**[Helper Methods](#helper-methods)**
**[Transaction Helpers](#transaction-helpers)**
**[Error Helpers](#error-helpers)**
**[Table Locking](#table-locking)**
## Support Me
This software is developed during my free time and I will be glad if somebody will support me.
Everyone's time should be valuable, so please consider donating.
[Donate with paypal](https://www.paypal.com/cgi-bin/webscr?cmd=_donations&business=a%2ebutenka%40gmail%2ecom&lc=DO&item_name=mysqlidb¤cy_code=USD&bn=PP%2dDonationsBF%3abtn_donateCC_LG%2egif%3aNonHosted)
### Installation
To utilize this class, first import MysqliDb.php into your project, and require it.
```php
require_once ('MysqliDb.php');
```
### Installation with composer
It is also possible to install library via composer
```
composer require thingengineer/mysqli-database-class:dev-master
```
### Initialization
Simple initialization with utf8 charset set by default:
```php
$db = new MysqliDb ('host', 'username', 'password', 'databaseName');
```
Advanced initialization:
```php
$db = new MysqliDb (Array (
'host' => 'host',
'username' => 'username',
'password' => 'password',
'db'=> 'databaseName',
'port' => 3306,
'prefix' => 'my_',
'charset' => 'utf8'));
```
table prefix, port and database charset params are optional.
If no charset should be set charset, set it to null
Also it is possible to reuse already connected mysqli object:
```php
$mysqli = new mysqli ('host', 'username', 'password', 'databaseName');
$db = new MysqliDb ($mysqli);
```
If no table prefix were set during object creation its possible to set it later with a separate call:
```php
$db->setPrefix ('my_');
```
If connection to mysql will be dropped Mysqlidb will try to automatically reconnect to the database once.
To disable this behavoir use
```php
$db->autoReconnect = false;
```
If you need to get already created mysqliDb object from another class or function use
```php
function init () {
// db staying private here
$db = new MysqliDb ('host', 'username', 'password', 'databaseName');
}
...
function myfunc () {
// obtain db object created in init ()
$db = MysqliDb::getInstance();
...
}
```
### Multiple database connection
If you need to connect to multiple databases use following method:
```php
$db->addConnection('slave', Array (
'host' => 'host',
'username' => 'username',
'password' => 'password',
'db'=> 'databaseName',
'port' => 3306,
'prefix' => 'my_',
'charset' => 'utf8')
);
```
To select database use connection() method
```php
$users = $db->connection('slave')->get('users');
```
### Objects mapping
dbObject.php is an object mapping library built on top of mysqliDb to provide model representation functionality.
See <a href='dbObject.md'>dbObject manual for more information</a>
### Insert Query
Simple example
```php
$data = Array ("login" => "admin",
"firstName" => "John",
"lastName" => 'Doe'
);
$id = $db->insert ('users', $data);
if($id)
echo 'user was created. Id=' . $id;
```
Insert with functions use
```php
$data = Array (
'login' => 'admin',
'active' => true,
'firstName' => 'John',
'lastName' => 'Doe',
'password' => $db->func('SHA1(?)',Array ("secretpassword+salt")),
// password = SHA1('secretpassword+salt')
'createdAt' => $db->now(),
// createdAt = NOW()
'expires' => $db->now('+1Y')
// expires = NOW() + interval 1 year
// Supported intervals [s]econd, [m]inute, [h]hour, [d]day, [M]onth, [Y]ear
);
$id = $db->insert ('users', $data);
if ($id)
echo 'user was created. Id=' . $id;
else
echo 'insert failed: ' . $db->getLastError();
```
Insert with on duplicate key update
```php
$data = Array ("login" => "admin",
"firstName" => "John",
"lastName" => 'Doe',
"createdAt" => $db->now(),
"updatedAt" => $db->now(),
);
$updateColumns = Array ("updatedAt");
$lastInsertId = "id";
$db->onDuplicate($updateColumns, $lastInsertId);
$id = $db->insert ('users', $data);
```
Insert multiple datasets at once
```php
$data = Array(
Array ("login" => "admin",
"firstName" => "John",
"lastName" => 'Doe'
),
Array ("login" => "other",
"firstName" => "Another",
"lastName" => 'User',
"password" => "very_cool_hash"
)
);
$ids = $db->insertMulti('users', $data);
if(!$ids) {
echo 'insert failed: ' . $db->getLastError();
} else {
echo 'new users inserted with following id\'s: ' . implode(', ', $ids);
}
```
If all datasets only have the same keys, it can be simplified
```php
$data = Array(
Array ("admin", "John", "Doe"),
Array ("other", "Another", "User")
);
$keys = Array("login", "firstName", "lastName");
$ids = $db->insertMulti('users', $data, $keys);
if(!$ids) {
echo 'insert failed: ' . $db->getLastError();
} else {
echo 'new users inserted with following id\'s: ' . implode(', ', $ids);
}
```
### Replace Query
<a href='https://dev.mysql.com/doc/refman/5.0/en/replace.html'>Replace()</a> method implements same API as insert();
### Update Query
```php
$data = Array (
'firstName' => 'Bobby',
'lastName' => 'Tables',
'editCount' => $db->inc(2),
// editCount = editCount + 2;
'active' => $db->not()
// active = !active;
);
$db->where ('id', 1);
if ($db->update ('users', $data))
echo $db->count . ' records were updated';
else
echo 'update failed: ' . $db->getLastError();
```
`update()` also support limit parameter:
```php
$db->update ('users', $data, 10);
// Gives: UPDATE users SET ... LIMIT 10
```
### Select Query
After any select/get function calls amount or returned rows is stored in $count variable
```php
$users = $db->get('users'); //contains an Array of all users
$users = $db->get('users', 10); //contains an Array 10 users
```
or select with custom columns set. Functions also could be used
```php
$cols = Array ("id", "name", "email");
$users = $db->get ("users", null, $cols);
if ($db->count > 0)
foreach ($users as $user) {
print_r ($user);
}
```
or select just one row
```php
$db->where ("id", 1);
$user = $db->getOne ("users");
echo $user['id'];
$stats = $db->getOne ("users", "sum(id), count(*) as cnt");
echo "total ".$stats['cnt']. "users found";
```
or select one column value or function result
```php
$count = $db->getValue ("users", "count(*)");
echo "{$count} users found";
```
select one column value or function result from multiple rows:
```php
$logins = $db->getValue ("users", "login", null);
// select login from users
$logins = $db->getValue ("users", "login", 5);
// select login from users limit 5
foreach ($logins as $login)
echo $login;
```
### Insert Data
You can also load .CSV or .XML data into a specific table.
To insert .csv data, use the following syntax:
```php
$path_to_file = "/home/john/file.csv";
$db->loadData("users", $path_to_file);
```
This will load a .cs
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
仿Twitter源代码 社交网络源码 基于脉聊二开版本这是一款类似于Twitter网站的源代码。利用原代码,你可以快速搭建自己的社交网络平台。程序语言是PHP。数据库采用Mysql。与此同时系统带有注册,评论,转发等功能。注册用户可以分享他 仿Twitter源代码 社交网络源码 基于脉聊二开版本 这是一款类似于Twitter网站的源代码。利用原代码,你可以快速搭建自己的社交网络平台。程序语言是PHP。数据库采用Mysql。与此同时系统带有注册,评论,转发等功能。注册用户可以分享他们的图片视频,或者创建投票的。同时系统自带ffmpeg功能上传视频会自动获取封面。还自带域名解析功;分享网址会抓取网站的描述和标题。配合后台管理面板,你可以快速设置所有的一切。 内附详细安装说明。
资源推荐
资源详情
资源评论
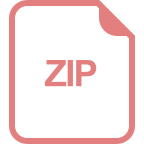
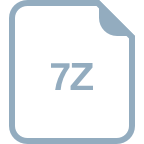
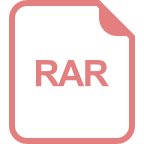
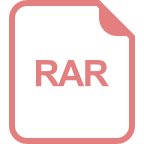
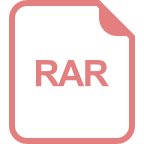
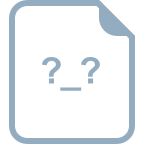
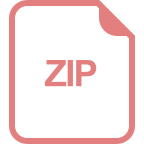
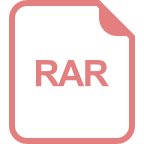
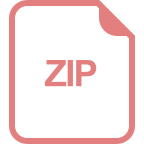
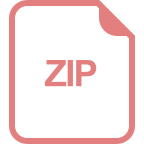
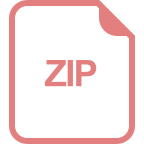
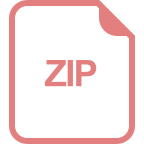
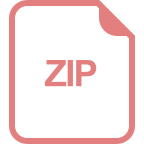
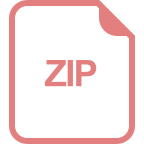
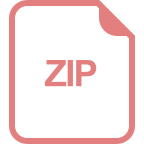
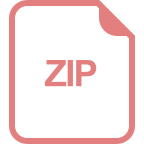
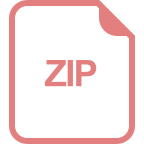
收起资源包目录

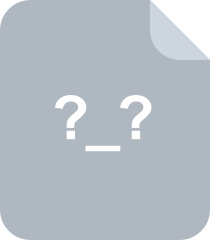
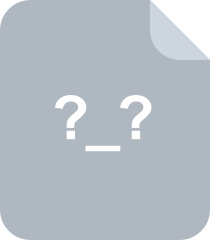
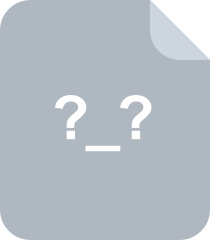
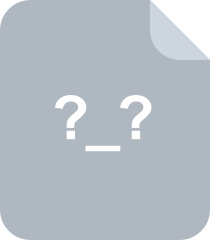
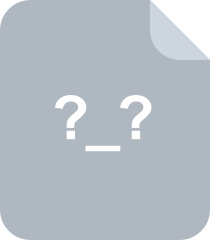
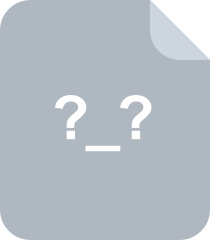
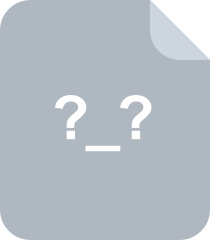
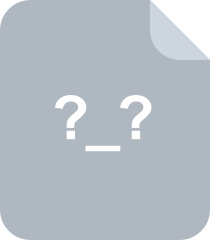
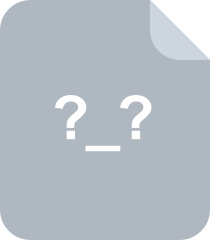
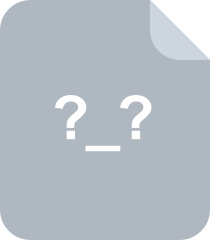
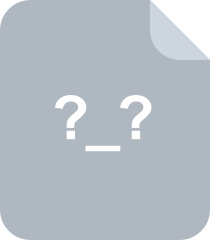
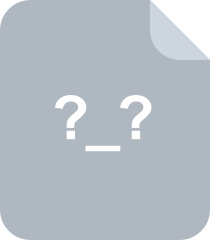
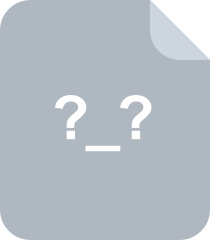
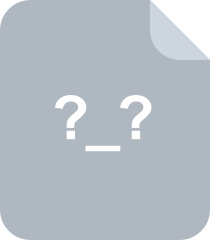
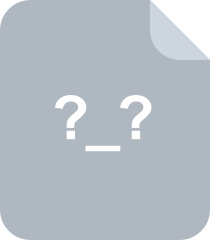
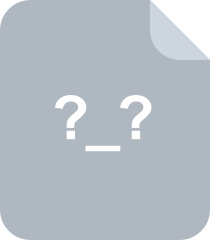
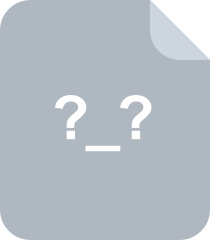
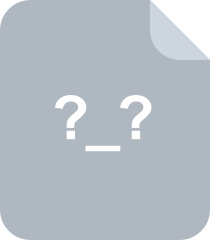
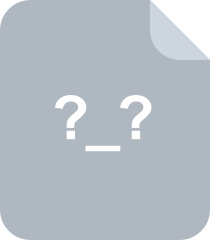
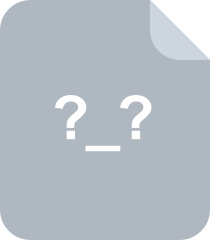
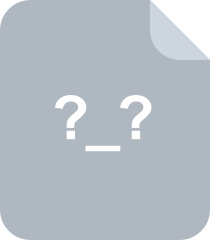
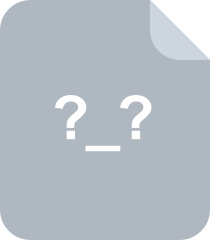
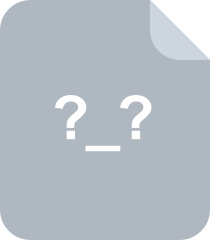
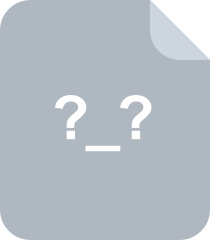
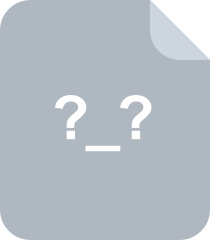
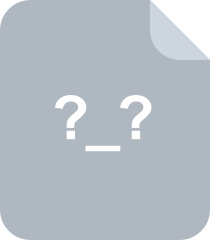
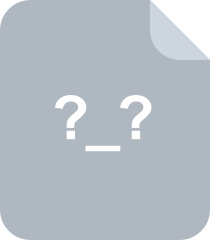
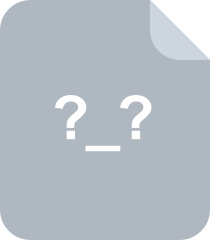
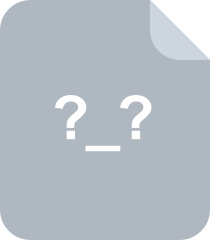
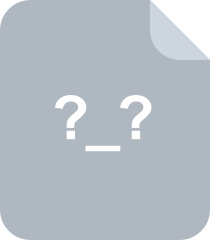
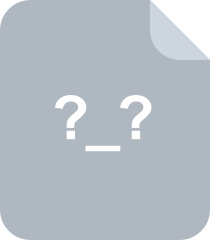
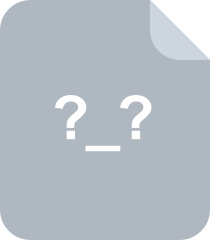
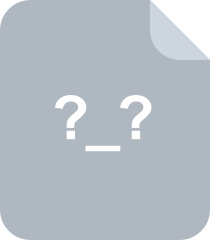
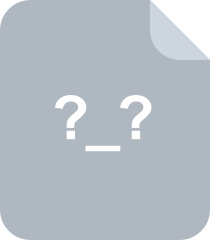
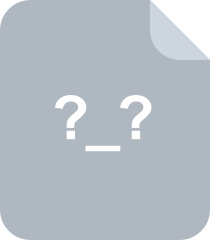
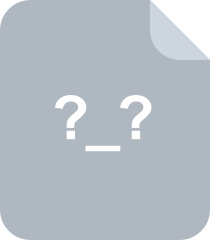
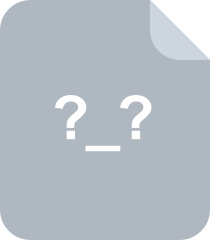
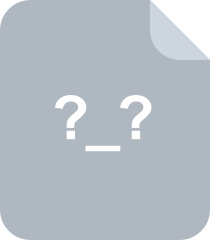
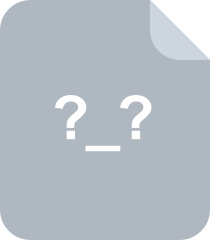
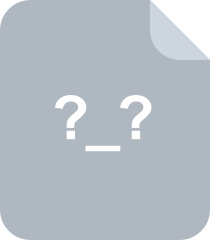
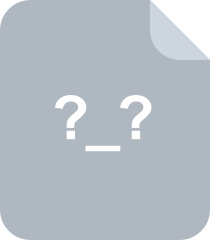
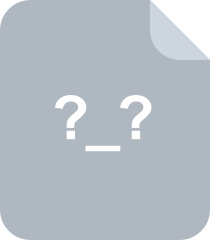
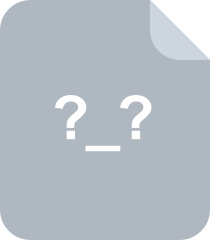
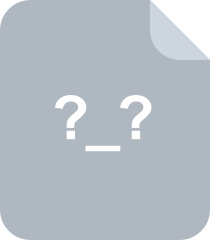
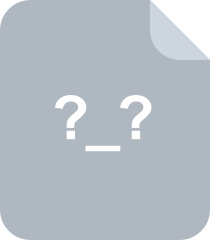
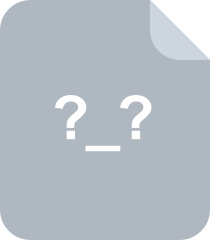
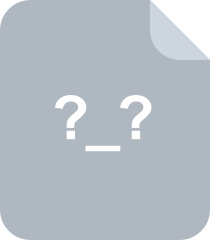
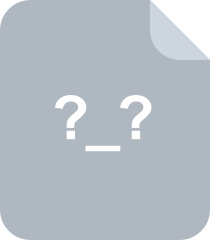
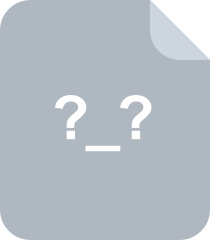
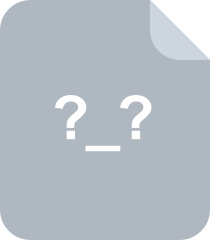
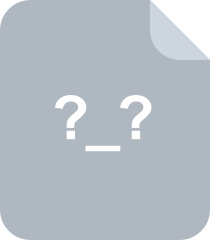
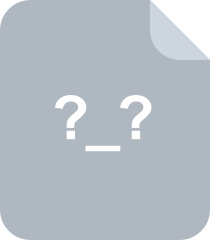
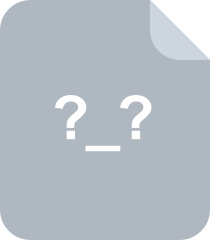
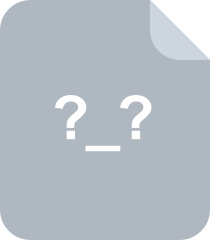
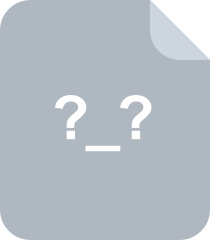
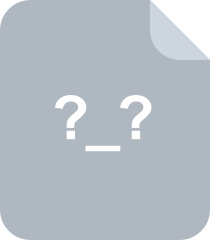
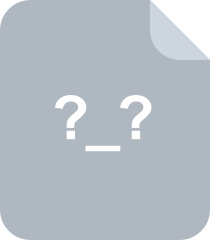
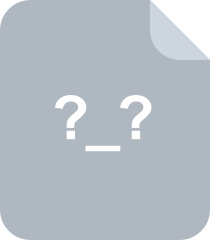
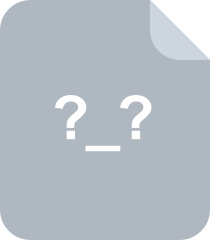
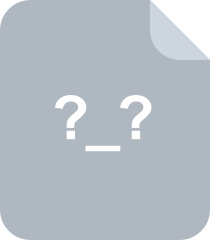
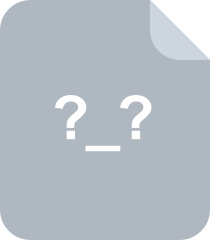
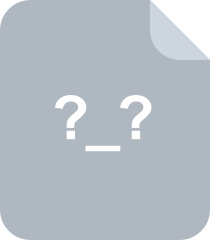
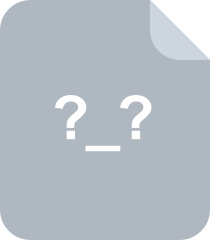
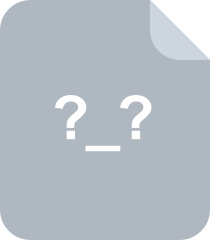
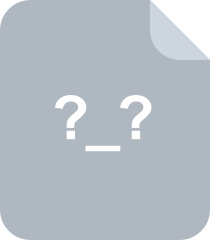
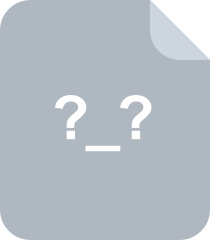
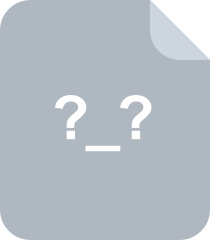
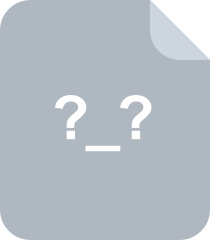
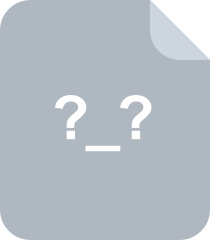
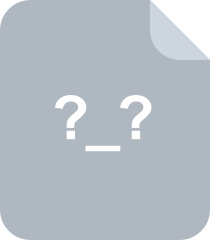
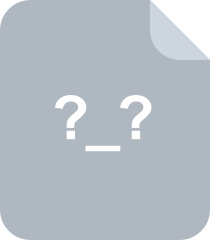
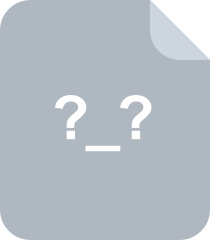
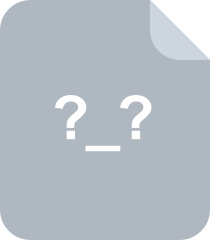
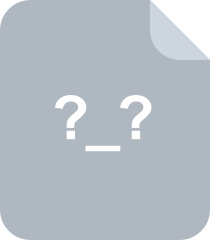
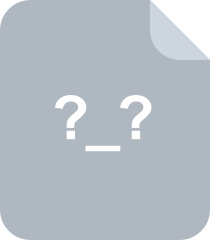
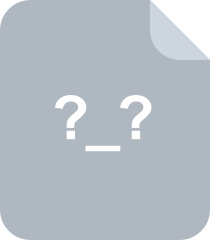
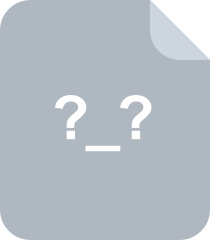
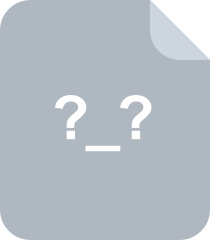
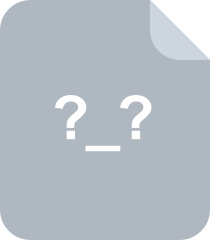
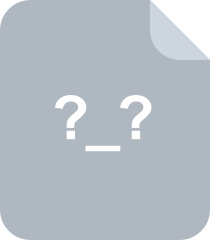
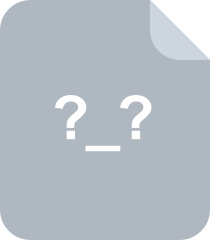
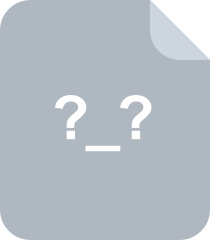
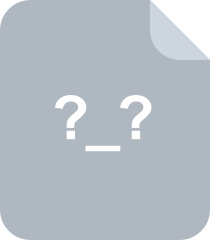
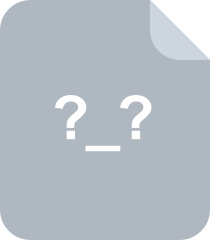
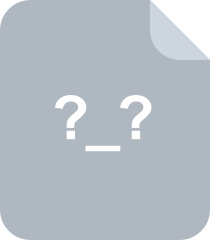
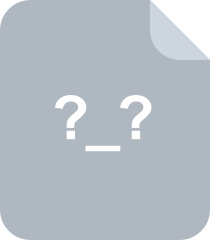
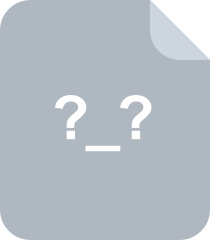
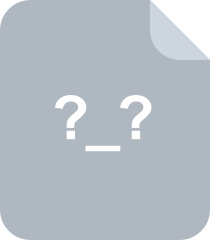
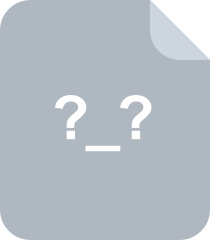
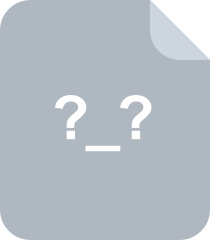
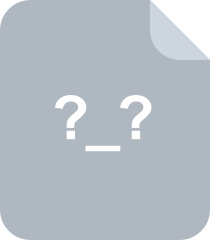
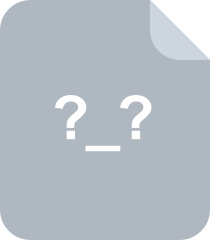
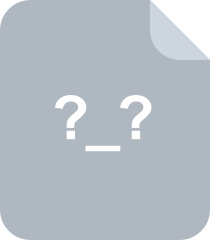
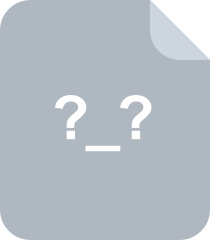
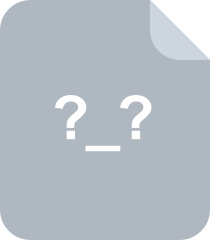
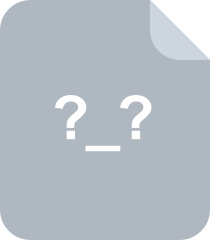
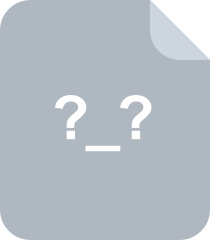
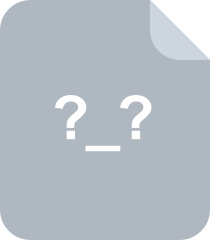
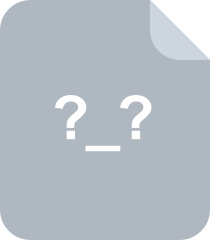
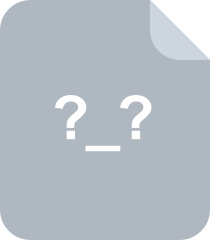
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
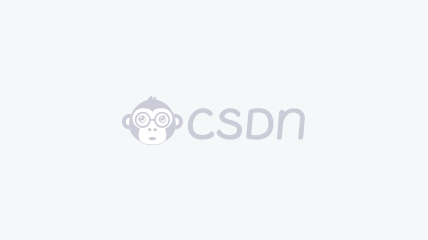

破碎的天堂鸟
- 粉丝: 9482
- 资源: 2723
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

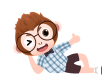
最新资源
- 录的CRUISE M热管理视频,有文档解说,没有模型,可用来学习了解
- 在win32汇编环境中如何生成richedit控件
- 学号-姓名-实验13NAT配置.doc
- 学号-姓名-实验12ACL包过滤.doc
- 学号-姓名-实验14广域网基础.docx
- 学号-姓名-实验10配置RIP.doc
- 学号-姓名-实验11配置OSPF.doc
- 学号-姓名-实验09路由配置+IPv6.doc
- 学号-姓名-实验08配置DHCP服务.doc
- 学号-姓名-实验07ARP.doc
- 学号-姓名-实验05VLAN配置.doc
- 学号-姓名-实验03文件操作与设备调试.doc
- 学号-姓名-实验01常用操作.doc
- 学号-姓名-实验00模拟器HCL.doc
- 2225060346-汤岚淇-实验12ACL包过滤.doc
- 2225060346-汤岚淇-实验06生成树协议.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


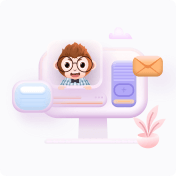
安全验证
文档复制为VIP权益,开通VIP直接复制
