<?php if (!defined('ABSPATH')) {die;} // Cannot access directly.
//
// Set a unique slug-like ID
//
$prefix = '_prefix_my_options';
//
// Create options
//
CSF::createOptions($prefix, array(
'menu_title' => 'CSF Demo',
'menu_slug' => 'csf-demo',
'theme' => 'light',
));
//
// Create a section
//
CSF::createSection($prefix, array(
'title' => 'Overview',
'icon' => 'fas fa-rocket',
'fields' => array(
//
// A text field
//
array(
'id' => 'opt-text',
'type' => 'text',
'title' => 'Text',
),
array(
'id' => 'opt-textarea',
'type' => 'textarea',
'title' => 'Textarea',
'help' => 'The help text of the field.',
),
array(
'id' => 'opt-upload',
'type' => 'upload',
'title' => 'Upload',
),
array(
'id' => 'opt-switcher',
'type' => 'switcher',
'title' => 'Switcher',
'label' => 'The label text of the switcher.',
),
array(
'id' => 'opt-color',
'type' => 'color',
'title' => 'Color',
'default' => '#3498db',
),
array(
'id' => 'opt-checkbox',
'type' => 'checkbox',
'title' => 'Checkbox',
'label' => 'The label text of the checkbox.',
),
array(
'id' => 'opt-radio',
'type' => 'radio',
'title' => 'Radio',
'options' => array(
'yes' => 'Yes, Please.',
'no' => 'No, Thank you.',
),
'default' => 'yes',
),
array(
'id' => 'opt-select',
'type' => 'select',
'title' => 'Select',
'placeholder' => 'Select an option',
'options' => array(
'opt-1' => 'Option 1',
'opt-2' => 'Option 2',
'opt-3' => 'Option 3',
),
),
array(
'id' => 'opt-image-select',
'type' => 'image_select',
'title' => 'Image Select',
'options' => array(
'opt-1' => 'http://codestarframework.com/assets/images/placeholder/100x80-2ecc71.gif',
'opt-2' => 'http://codestarframework.com/assets/images/placeholder/100x80-e74c3c.gif',
'opt-3' => 'http://codestarframework.com/assets/images/placeholder/100x80-ffbc00.gif',
'opt-4' => 'http://codestarframework.com/assets/images/placeholder/100x80-3498db.gif',
'opt-5' => 'http://codestarframework.com/assets/images/placeholder/100x80-555555.gif',
),
'default' => 'opt-1',
),
array(
'id' => 'opt-background',
'type' => 'background',
'title' => 'Background',
),
array(
'type' => 'notice',
'style' => 'success',
'content' => 'A <strong>notice</strong> field with <strong>success</strong> style.',
),
array(
'id' => 'opt-icon',
'type' => 'icon',
'title' => 'Icon',
),
array(
'id' => 'opt-alt-text',
'type' => 'text',
'title' => 'Text',
),
array(
'id' => 'opt-alt-textarea',
'type' => 'textarea',
'title' => 'Textarea',
'subtitle' => 'A textarea with shortcoder.',
'shortcoder' => 'csf_demo_shortcodes',
),
),
));
//
// Basic Fields
//
CSF::createSection($prefix, array(
'id' => 'basic_fields',
'title' => 'Basic Fields',
'icon' => 'fas fa-plus-circle',
));
//
// Field: text
//
CSF::createSection($prefix, array(
'parent' => 'basic_fields',
'title' => 'Text',
'icon' => 'far fa-square',
'description' => 'Visit documentation for more details on this field: <a href="http://codestarframework.com/documentation/#/fields?id=text" target="_blank">Field: text</a>',
'fields' => array(
array(
'id' => 'opt-text-1',
'type' => 'text',
'title' => 'Text',
),
array(
'id' => 'opt-text-2',
'type' => 'text',
'title' => 'Text with default',
'default' => 'This is default value bla bla bla',
),
array(
'id' => 'opt-text-3',
'type' => 'text',
'title' => 'Text field ingenuity',
'subtitle' => 'The field of subtitle text.',
'help' => 'The field of help text.',
'before' => '<p>The field of before text.</p>',
'after' => '<p>The field of after text.</p>',
),
array(
'id' => 'opt-text-4',
'type' => 'text',
'title' => 'Text with placeholder',
'placeholder' => 'Typed something...',
),
array(
'id' => 'opt-text-5',
'type' => 'text',
'title' => 'Text readonly',
'attributes' => array(
'readonly' => 'readonly',
),
'default' => 'readonly text field, can not be changed',
),
array(
'id' => 'opt-text-6',
'type' => 'text',
'title' => 'Text with maxlength (5)',
'attributes' => array(
'maxlength' => '5',
),
'default' => 'abc',
),
array(
'id' => 'opt-text-7',
'type' => 'text',
'title' => 'Text usign custom styles',
'attributes' => array(
'style' => 'width: 100%; height: 40px; border-color: #93C054;',
),
),
array(
'id' => 'opt-text-8',
'type' => 'text',
'after' => '<p>It shows full width if there is no field of title.</p>',
),
),
));
//
// Field: textarea
//
CSF::createSection($prefix, array(
'parent' => 'basic_fields',
'title' => 'Textarea',
'icon' => 'far fa-square',
'description' => 'Visit documentation for more details on this field: <a href="http://codestarframework.com/documentation/#/fields?id=textarea" target="_blank">Field: textrea</a>',
'fields' => array(
array(
'id' => 'opt-textarea-1',
'type' => 'textarea',
'title' => 'Textarea',
),
array(
'id' => 'opt-textarea-2',
'type' => 'textarea',
'title' => 'Textarea wtih default',
'default' => 'This is default value bla bla bla',
),
array(
'id' => 'opt-textarea-3',
'type' => 'textarea',
'title' => 'Text with placeholder',
'placeholder' => 'Typed something...',
),
array(
'id' => 'opt-textarea-4',
'type' => 'textarea',
'title' => 'Textarea with shortcoder',
'shortcoder' => 'csf_demo_shortcodes',
),
array(
'id' => 'opt-textarea-5',
'type' => 'textarea',
'title' => 'Textarea field ingenuity',
'subtitle' => 'The field of subtitle text.',
'help' => 'The field of help text.',
'before' => '<p>The field of before text.</p>',
'after' => '<p>The field of after text.</p>',
),
array(
'id' => 'opt-textarea-6',
'type' => 'textarea',
'after' => '<p>It shows full
没有合适的资源?快使用搜索试试~ 我知道了~
子比主题美化 付费区块下载美化插件(开源版)
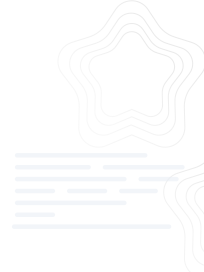
共164个文件
php:92个
po:20个
mo:20个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 43 浏览量
2024-10-25
11:47:53
上传
评论
收藏 505KB ZIP 举报
温馨提示
美化子比主题付费区块及下载区块,付费图片,付费视频不支持,此插件原作者只写了未购买样式,已购买和免费资源样式没有写,有能力可以自己二开,已经把已购买和免费资源函数写进去了,内包含非常详细的注释亦可以当插件基础框架学习.
资源推荐
资源详情
资源评论
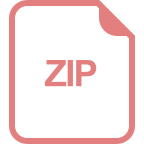
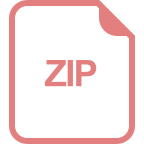
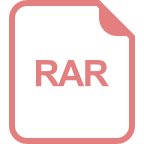
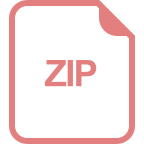
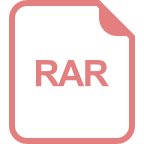
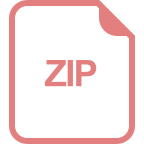
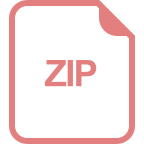
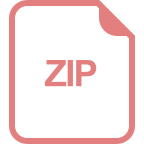
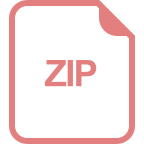
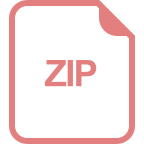
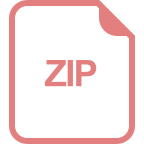
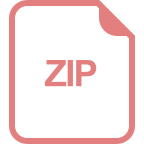
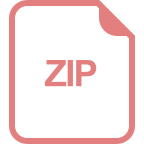
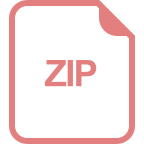
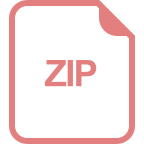
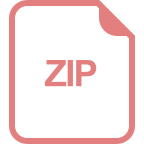
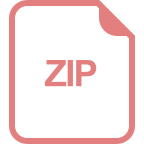
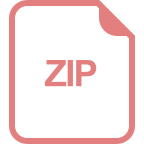
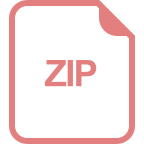
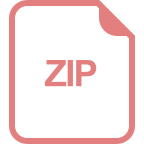
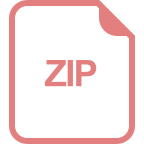
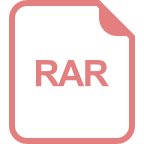
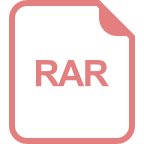
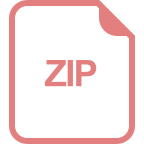
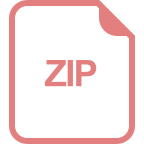
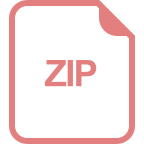
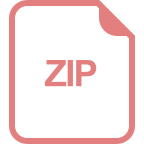
收起资源包目录

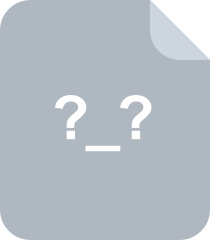
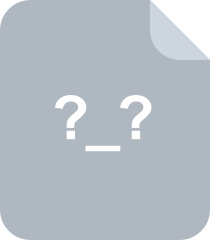
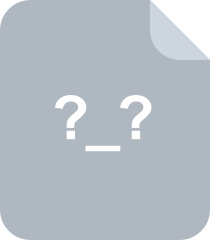
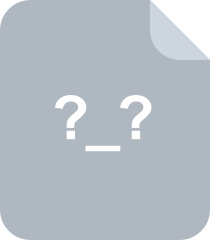
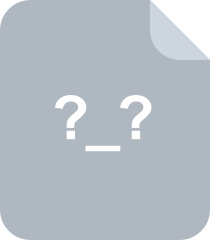
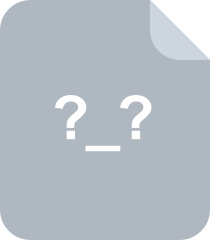
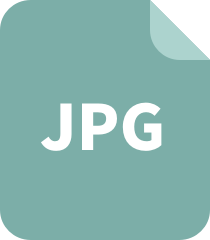
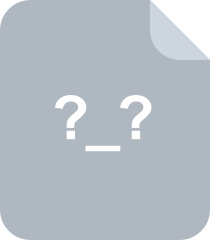
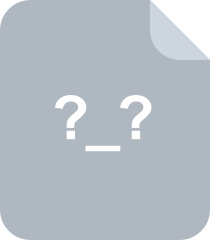
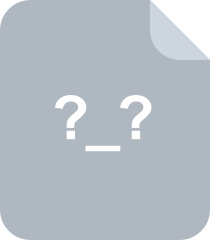
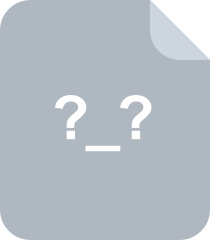
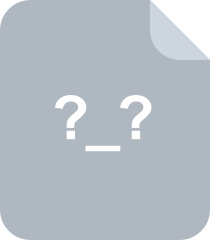
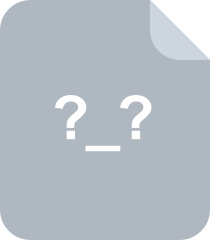
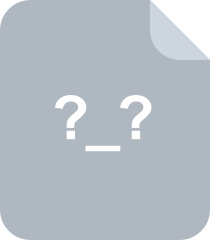
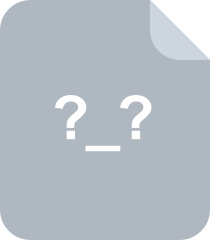
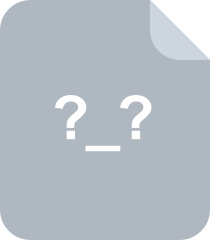
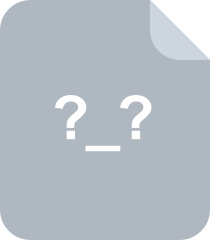
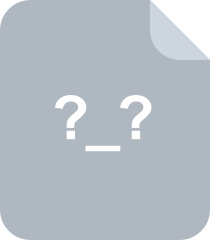
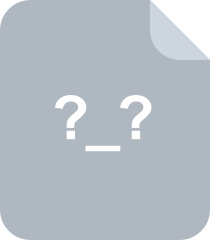
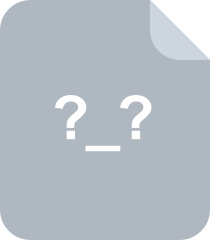
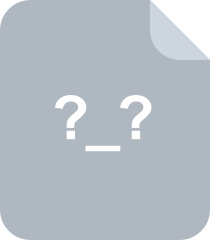
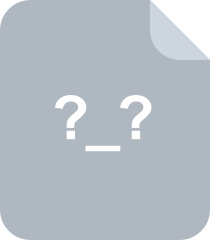
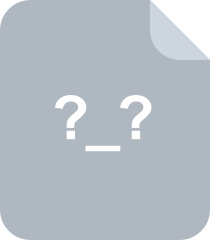
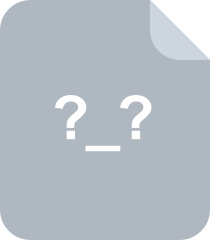
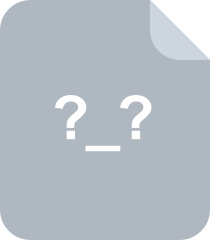
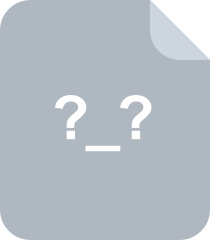
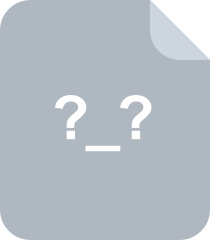
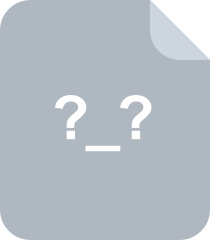
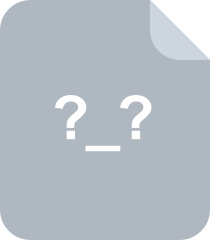
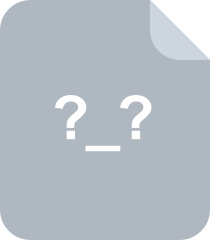
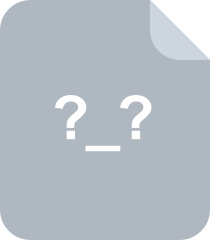
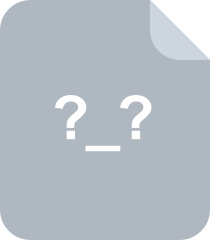
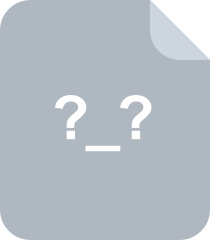
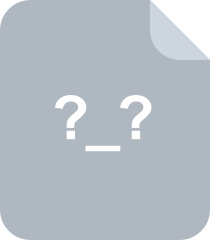
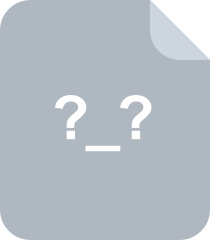
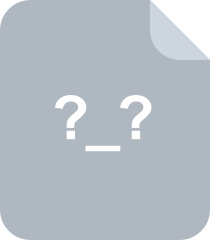
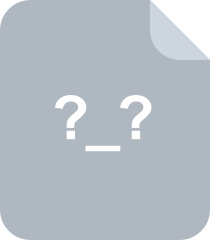
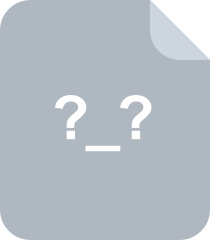
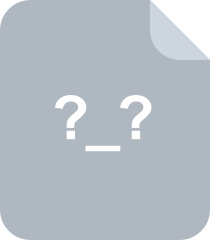
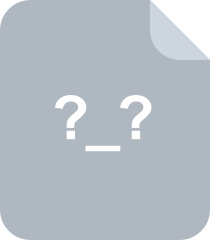
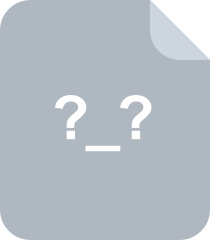
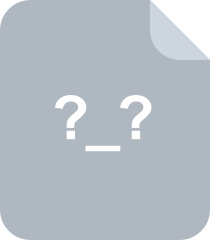
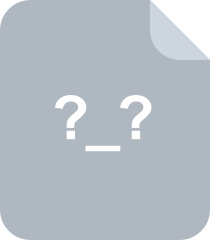
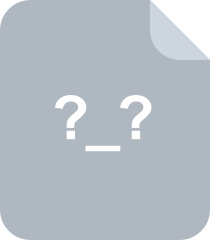
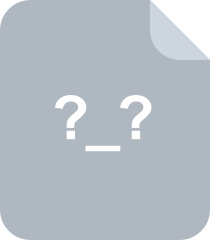
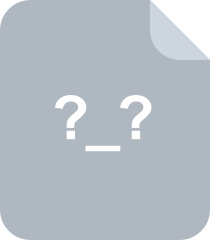
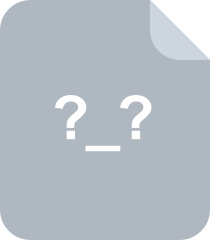
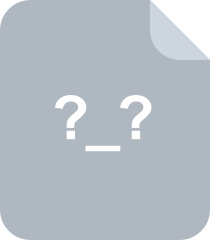
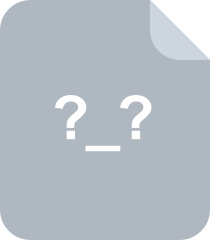
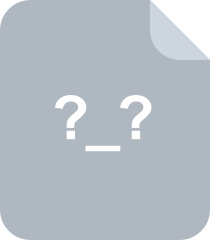
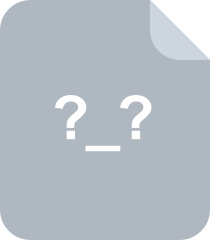
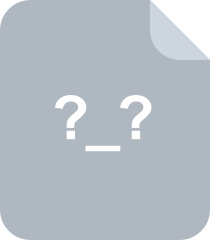
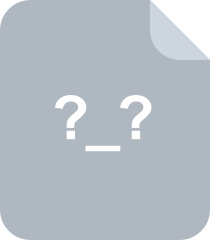
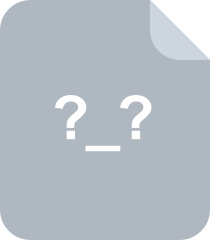
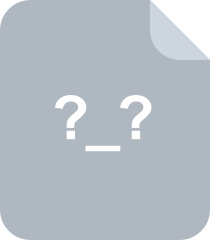
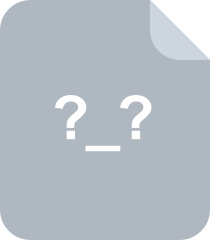
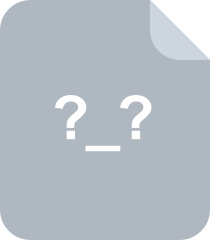
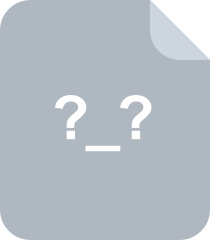
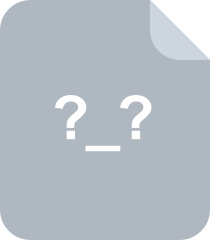
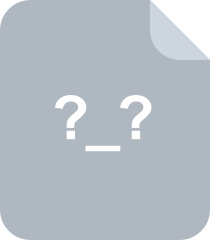
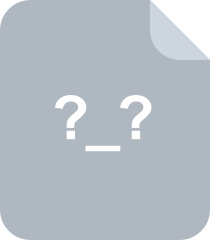
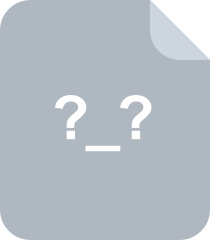
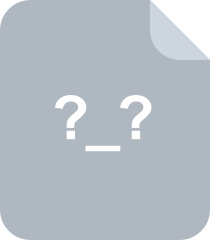
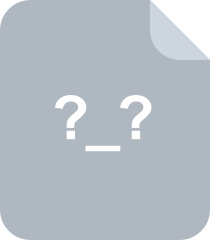
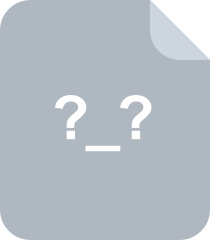
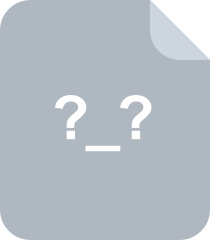
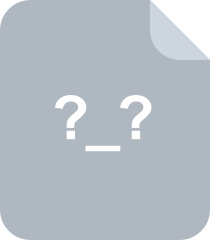
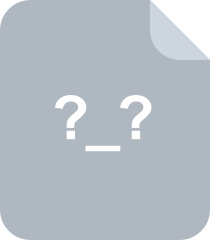
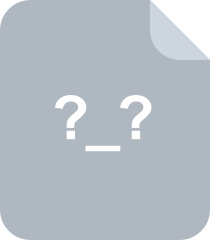
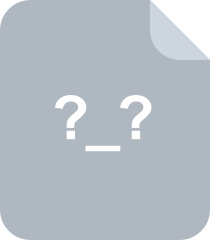
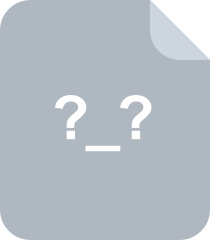
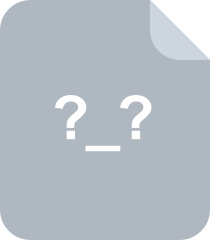
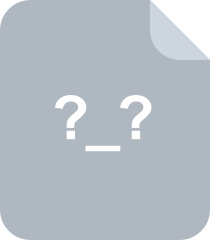
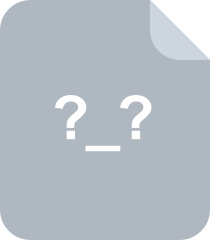
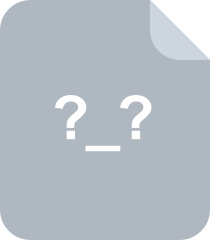
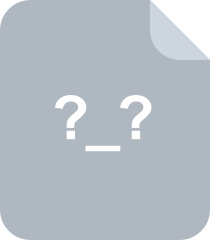
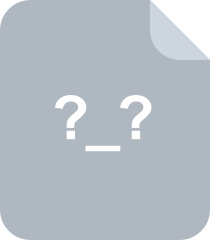
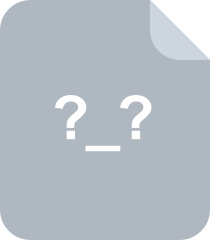
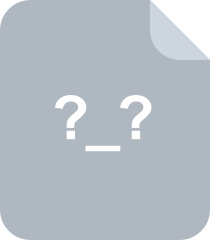
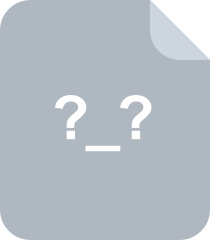
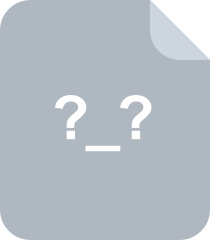
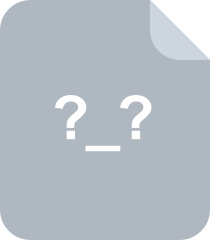
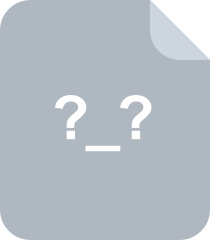
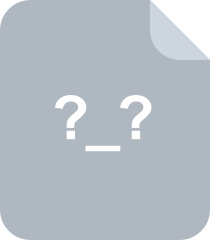
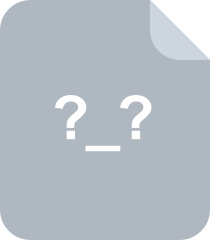
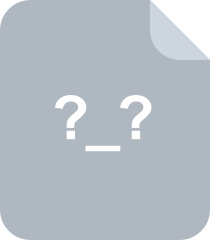
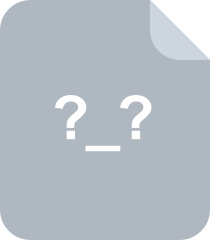
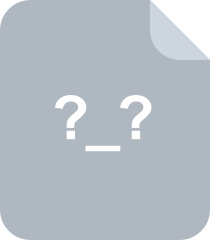
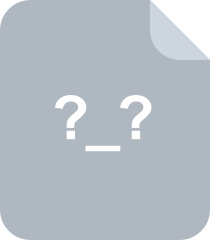
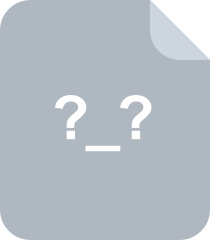
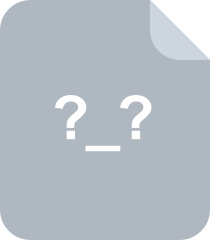
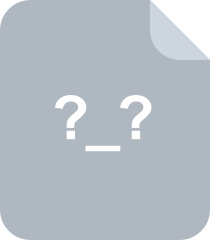
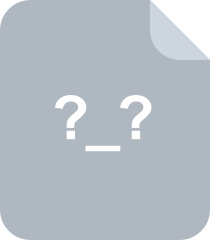
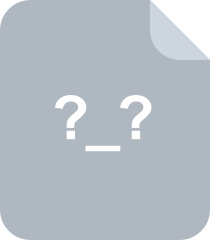
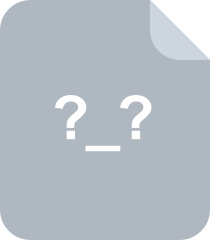
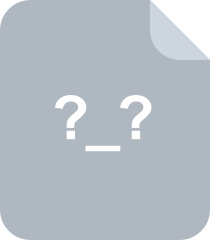
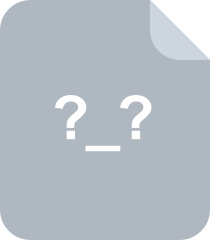
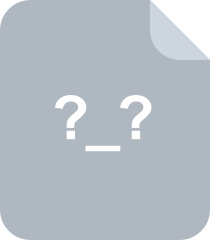
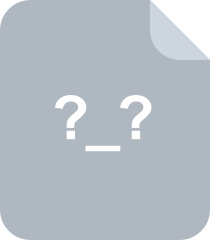
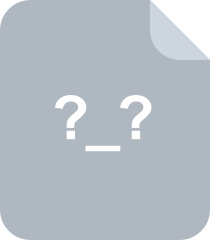
共 164 条
- 1
- 2
资源评论
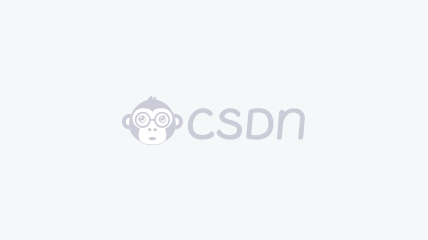

破碎的天堂鸟
- 粉丝: 8449
- 资源: 2304

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

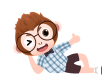
最新资源
- Cocos2d-x教程视频使用Eclipse在Ubuntu下搭建Cocos2d-x 3集成开发环境
- java实现飞机大战的游戏
- 安捷伦的噪声系数基础应用笔记
- MISRA-C工业标准的C编程规范(中文版).pdf
- Cocos2d-x教程视频粒子系统初级应用
- Cocos2d-x教程视频彩虹糖粒子特效
- Cocos2d-x教程视频Windows平台下在VS2013中为Cocos2d-x3工程添加Box2D物理引擎支持库
- rpi4b基于uboot通过nfs挂载最新主线Linux内核的注意事项
- Cocos2d-x教程视频TMX地图解析
- Cocos2d-x教程视频CocosStudio 2.0 文件格式解析
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


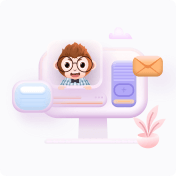
安全验证
文档复制为VIP权益,开通VIP直接复制
