
A PHP string manipulation library with multibyte support. Compatible with PHP
5.4+, PHP 7+, and HHVM.
``` php
s('string')->toTitleCase()->ensureRight('y') == 'Stringy'
```
Refer to the [1.x branch](https://github.com/danielstjules/Stringy/tree/1.x) or
[2.x branch](https://github.com/danielstjules/Stringy/tree/2.x) for older
documentation.
[](https://travis-ci.org/danielstjules/Stringy)
[](https://packagist.org/packages/danielstjules/stringy)
[](https://packagist.org/packages/danielstjules/stringy)
* [Why?](#why)
* [Installation](#installation)
* [OO and Chaining](#oo-and-chaining)
* [Implemented Interfaces](#implemented-interfaces)
* [PHP 5.6 Creation](#php-56-creation)
* [StaticStringy](#staticstringy)
* [Class methods](#class-methods)
* [create](#createmixed-str--encoding-)
* [Instance methods](#instance-methods)
<table>
<tr>
<td><a href="#appendstring-string">append</a></td>
<td><a href="#atint-index">at</a></td>
<td><a href="#betweenstring-start-string-end--int-offset">between</a></td>
<td><a href="#camelize">camelize</a></td>
</tr>
<tr>
<td><a href="#chars">chars</a></td>
<td><a href="#collapsewhitespace">collapseWhitespace</a></td>
<td><a href="#containsstring-needle--boolean-casesensitive--true-">contains</a></td>
<td><a href="#containsallarray-needles--boolean-casesensitive--true-">containsAll</a></td>
</tr>
<tr>
<td><a href="#containsanyarray-needles--boolean-casesensitive--true-">containsAny</a></td>
<td><a href="#countsubstrstring-substring--boolean-casesensitive--true-">countSubstr</a></td>
<td><a href="#dasherize">dasherize</a></td>
<td><a href="#delimitint-delimiter">delimit</a></td>
</tr>
<tr>
<td><a href="#endswithstring-substring--boolean-casesensitive--true-">endsWith</a></td>
<td><a href="#endswithanystring-substrings--boolean-casesensitive--true-">endsWithAny</a></td>
<td><a href="#ensureleftstring-substring">ensureLeft</a></td>
<td><a href="#ensurerightstring-substring">ensureRight</a></td>
</tr>
<tr>
<td><a href="#firstint-n">first</a></td>
<td><a href="#getencoding">getEncoding</a></td>
<td><a href="#haslowercase">hasLowerCase</a></td>
<td><a href="#hasuppercase">hasUpperCase</a></td>
</tr>
<tr>
<td><a href="#htmldecode">htmlDecode</a></td>
<td><a href="#htmlencode">htmlEncode</a></td>
<td><a href="#humanize">humanize</a></td>
<td><a href="#indexofstring-needle--offset--0-">indexOf</a></td>
</tr>
<tr>
<td><a href="#indexoflaststring-needle--offset--0-">indexOfLast</a></td>
<td><a href="#insertint-index-string-substring">insert</a></td>
<td><a href="#isalpha">isAlpha</a></td>
<td><a href="#isalphanumeric">isAlphanumeric</a></td>
</tr>
<tr>
<td><a href="#isbase64">isBase64</a></td>
<td><a href="#isblank">isBlank</a></td>
<td><a href="#ishexadecimal">isHexadecimal</a></td>
<td><a href="#isjson">isJson</a></td>
</tr>
<tr>
<td><a href="#islowercase">isLowerCase</a></td>
<td><a href="#isserialized">isSerialized</a></td>
<td><a href="#isuppercase">isUpperCase</a></td>
<td><a href="#lastint-n">last</a></td>
</tr>
<tr>
<td><a href="#length">length</a></td>
<td><a href="#lines">lines</a></td>
<td><a href="#longestcommonprefixstring-otherstr">longestCommonPrefix</a></td>
<td><a href="#longestcommonsuffixstring-otherstr">longestCommonSuffix</a></td>
</tr>
<tr>
<td><a href="#longestcommonsubstringstring-otherstr">longestCommonSubstring</a></td>
<td><a href="#lowercasefirst">lowerCaseFirst</a></td>
<td><a href="#padint-length--string-padstr-----string-padtype--right-">pad</a></td>
<td><a href="#padbothint-length--string-padstr----">padBoth</a></td>
</tr>
<tr>
<td><a href="#padleftint-length--string-padstr----">padLeft</a></td>
<td><a href="#padrightint-length--string-padstr----">padRight</a></td>
<td><a href="#prependstring-string">prepend</a></td>
<td><a href="#regexreplacestring-pattern-string-replacement--string-options--msr">regexReplace</a></td>
</tr>
<tr>
<td><a href="#removeleftstring-substring">removeLeft</a></td>
<td><a href="#removerightstring-substring">removeRight</a></td>
<td><a href="#repeatint-multiplier">repeat</a></td>
<td><a href="#replacestring-search-string-replacement">replace</a></td>
</tr>
<tr>
<td><a href="#reverse">reverse</a></td>
<td><a href="#safetruncateint-length--string-substring---">safeTruncate</a></td>
<td><a href="#shuffle">shuffle</a></td>
<td><a href="#slugify-string-replacement-----string-language--en">slugify</a></td>
</tr>
<tr>
<td><a href="#sliceint-start--int-end-">slice</a></td>
<td><a href="#splitstring-pattern--int-limit-">split</a></td>
<td><a href="#startswithstring-substring--boolean-casesensitive--true-">startsWith</a></td>
<td><a href="#startswithanystring-substrings--boolean-casesensitive--true-">startsWithAny</a></td>
</tr>
<tr>
<td><a href="#stripwhitespace">stripWhitespace</a></td>
<td><a href="#substrint-start--int-length-">substr</a></td>
<td><a href="#surroundstring-substring">surround</a></td>
<td><a href="#swapcase">swapCase</a></td>
</tr>
<tr>
<td><a href="#tidy">tidy</a></td>
<td><a href="#titleize-array-ignore">titleize</a></td>
<td><a href="#toascii-string-language--en--bool-removeunsupported--true-">toAscii</a></td>
<td><a href="#toboolean">toBoolean</a></td>
</tr>
<tr>
<td><a href="#tolowercase">toLowerCase</a></td>
<td><a href="#tospaces-tablength--4-">toSpaces</a></td>
<td><a href="#totabs-tablength--4-">toTabs</a></td>
<td><a href="#totitlecase">toTitleCase</a></td>
</tr>
<tr>
<td><a href="#touppercase">toUpperCase</a></td>
<td><a href="#trim-string-chars">trim</a></td>
<td><a href="#trimleft-string-chars">trimLeft</a></td>
<td><a href="#trimright-string-chars">trimRight</a></td>
</tr>
<tr>
<td><a href="#truncateint-length--string-substring---">truncate</a></td>
<td><a href="#underscored">underscored</a></td>
<td><a href="#uppercamelize">upperCamelize</a></td>
<td><a href="#uppercasefirst">upperCaseFirst</a></td>
</tr>
</table>
* [Extensions](#extensions)
* [Tests](#tests)
* [License](#license)
## Why?
In part due to a lack of multibyte support (including UTF-8) across many of
PHP's standard string functions. But also to offer an OO wrapper around the
`mbstring` module's multibyte-compatible functions. Stringy handles some quirks,
provides additional functionality, and hopefully makes strings a little easier
to work with!
```php
// Standard library
strtoupper('fòôbàř'); // 'FòôBàř'
strlen('fòôbàř'); // 10
// mbstring
mb_strtoupper('fòôbàř'); // 'FÒÔBÀŘ'
mb_strlen('fòôbàř'); // '6'
// Stringy
s('fòôbàř')->toUpperCase(); // 'FÒÔBÀŘ'
s('fòôbàř')->length(); // '6'
```
## Installation
If you're using Composer to manage dependencies, you can include the following
in your composer.json file:
```json
"require": {
"danielstjules/stringy": "~3.1.0"
}
```
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
思乐直播系统,集直播、短视频等功能,根据市场趋势开发并推出思乐直播APP,APP功能丰富且可在后台管理系统进行配置,做到按需求来开启功能。APP使用起来方便快捷,随时随地开启直播、分享短视频。 整个系统具备非常完善、丰富的功能,后台系统具有会员管理、直播管理、短视频管理、音乐管理、礼物管理、贵族管理、资金管理、举报管理、文章管理、日志管理、平台管理、靓号管理、活动管理功能,可通过后台系统对直播APP进行方面、全方位的管理。 后台环境:Linux系统 PHP7以上+Nginx+Mysql 安装步骤:安装PHP、Nginx、Mysql 1、把sl_onlive.sql导入到数据库中; 2、修改sile_onlive\config中db.config.php的相应设置; 3、将sile_onlive文件夹放至PHP的www目录下,设置域名根目录为WWW/sile_onlive/public即可
资源推荐
资源详情
资源评论
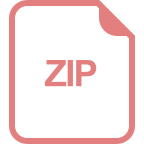
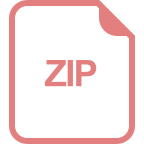
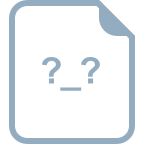
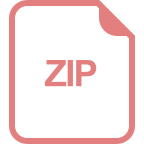
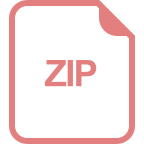
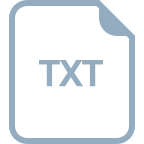
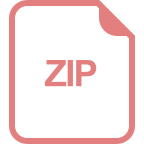
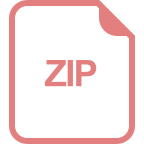
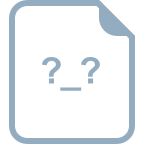
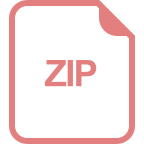
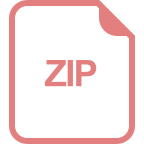
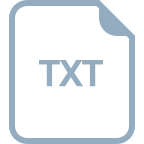
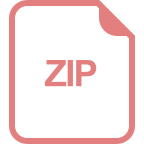
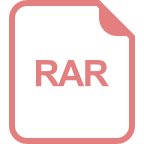
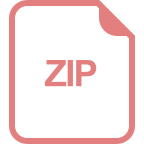
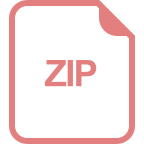
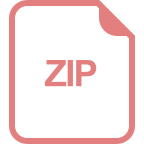
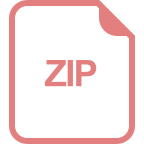
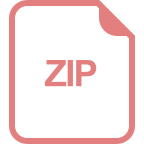
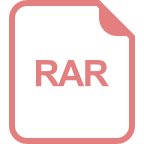
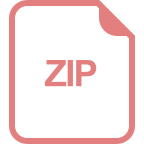
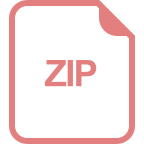
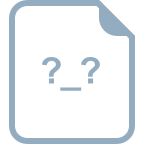
收起资源包目录

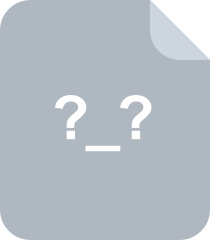
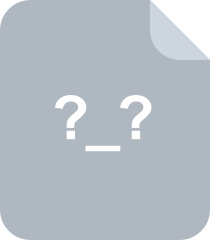
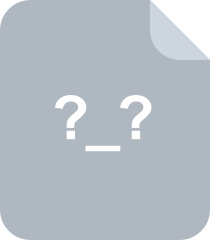
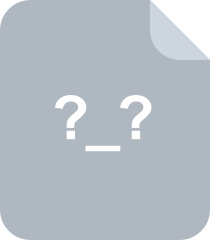
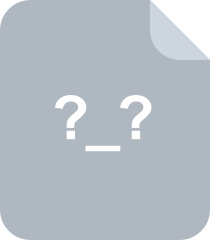
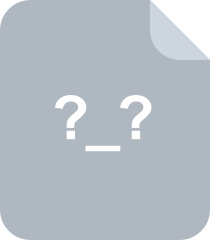
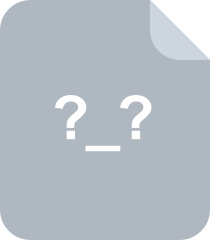
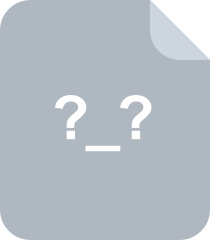
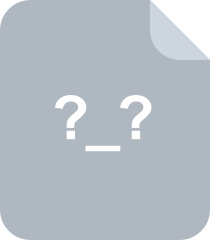
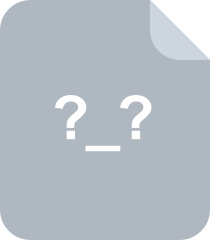
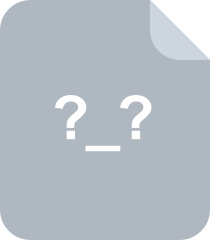
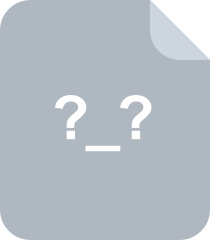
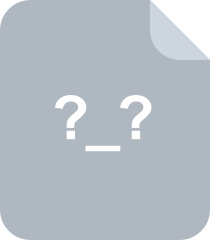
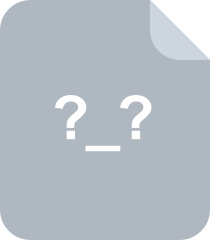
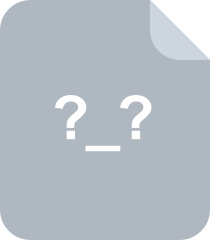
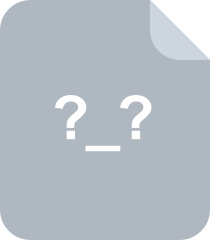
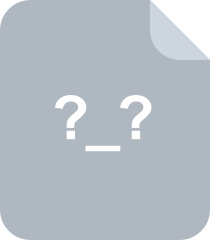
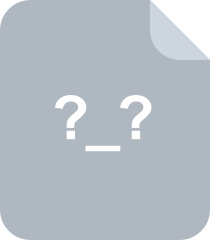
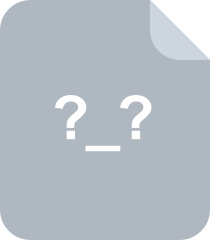
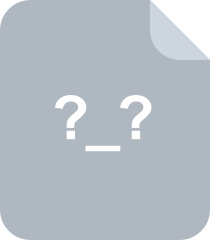
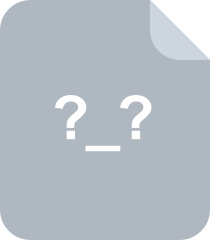
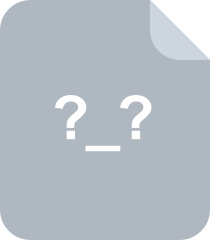
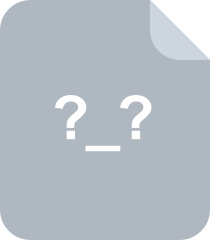
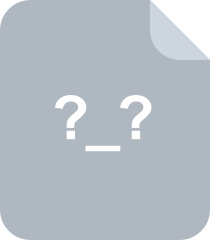
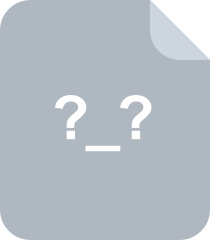
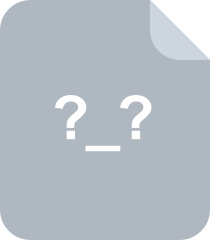
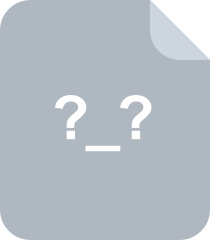
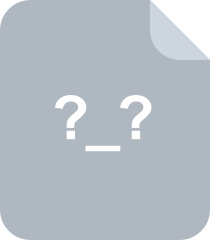
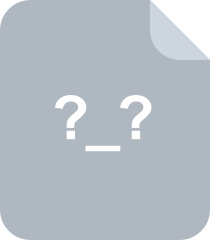
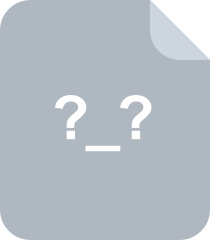
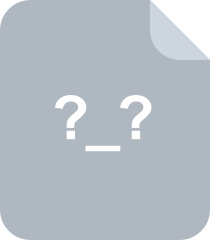
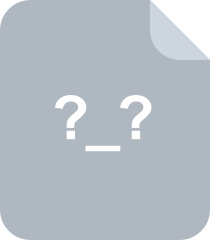
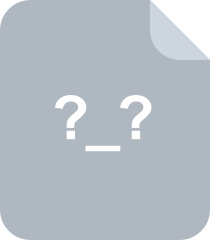
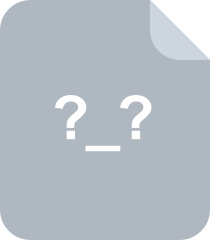
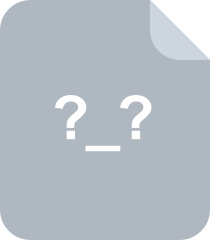
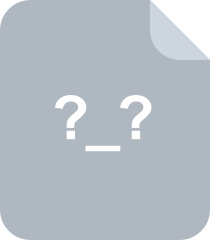
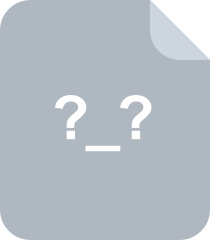
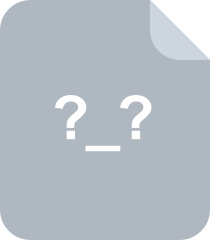
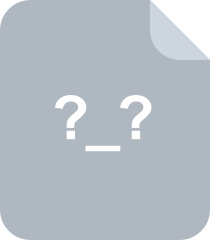
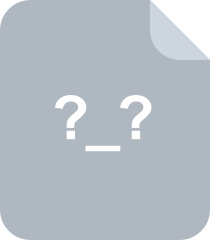
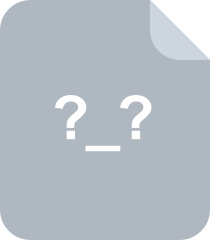
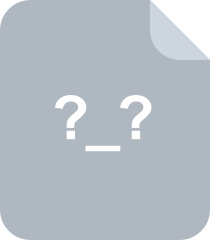
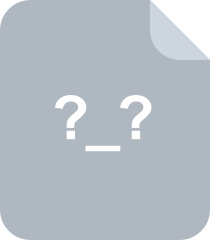
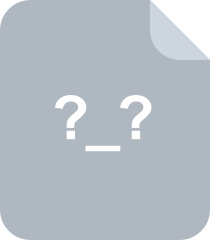
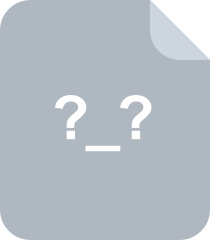
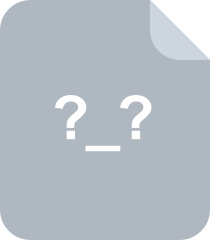
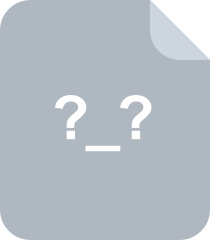
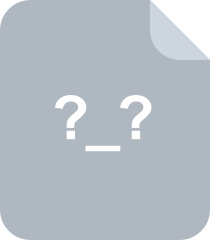
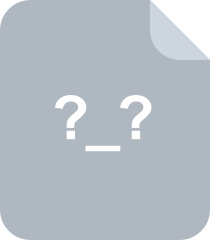
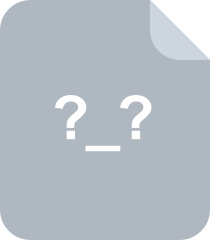
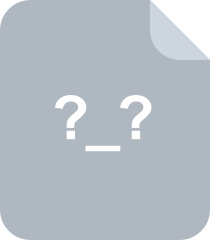
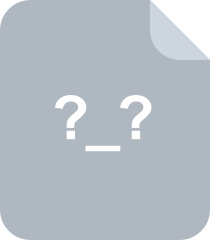
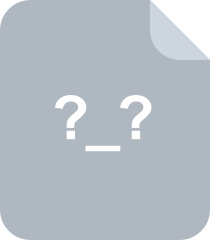
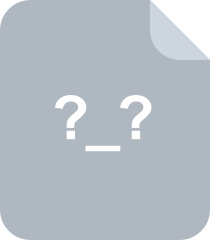
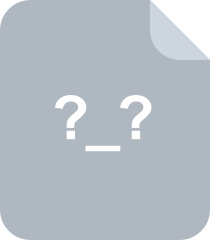
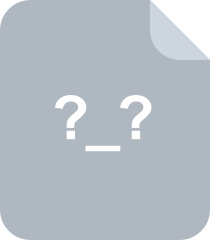
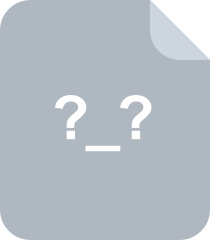
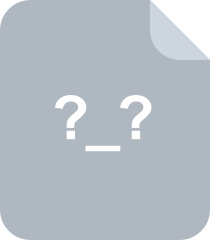
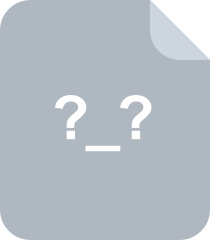
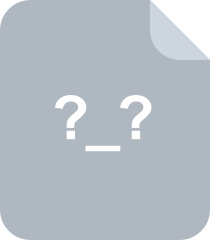
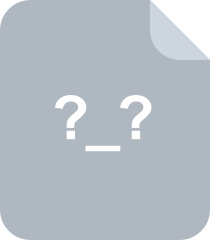
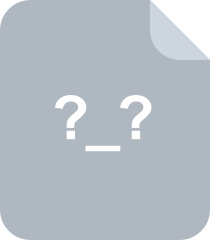
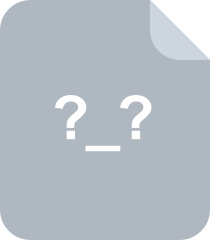
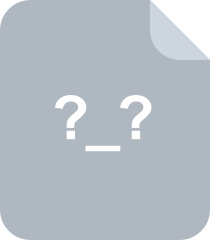
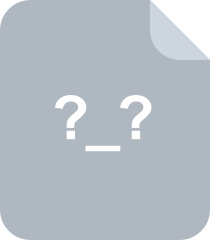
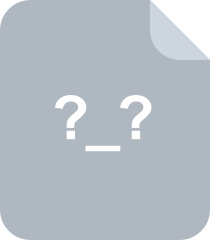
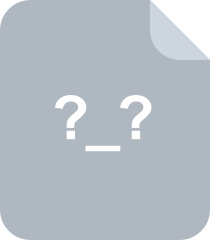
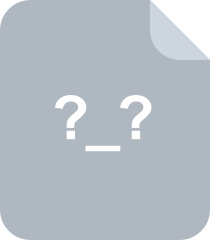
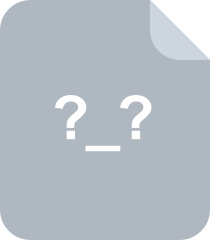
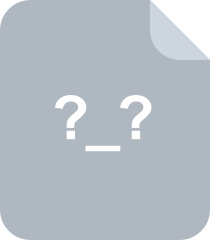
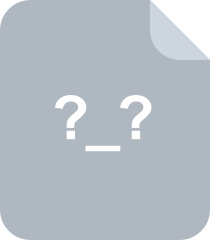
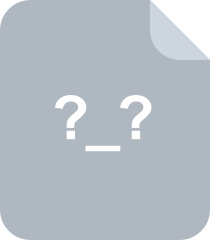
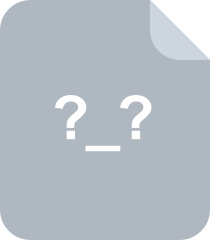
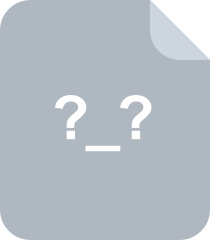
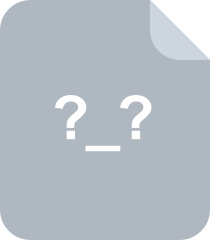
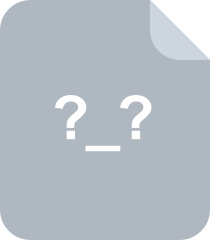
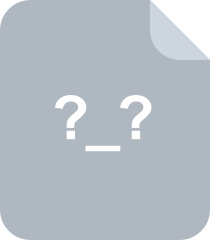
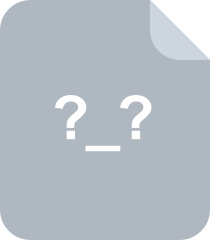
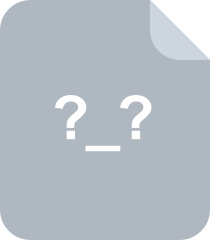
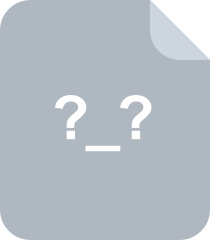
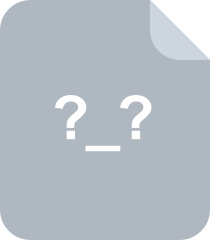
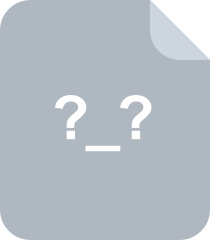
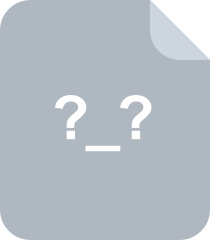
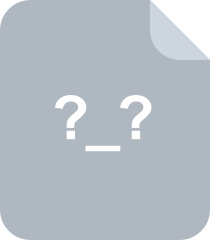
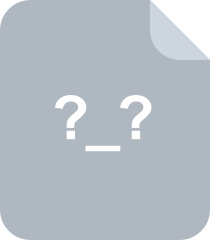
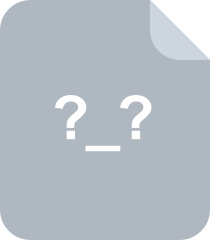
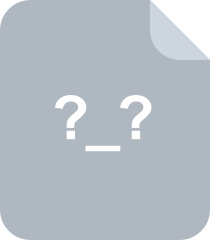
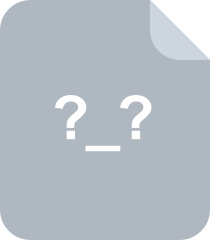
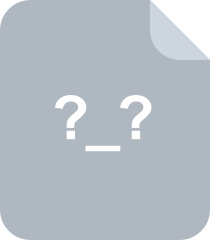
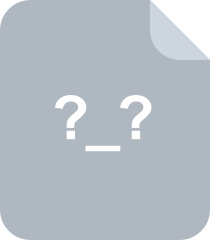
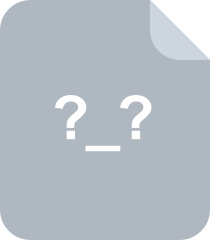
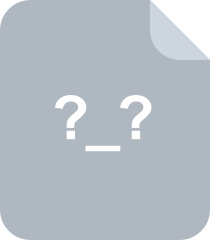
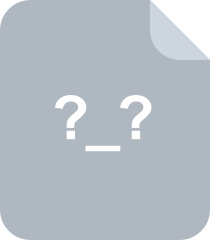
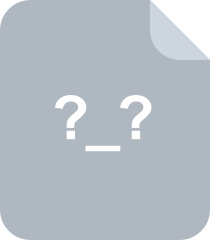
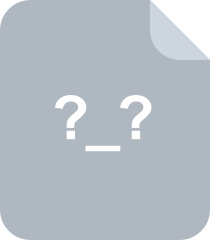
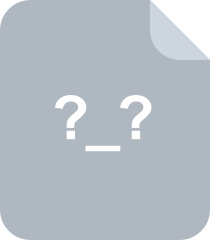
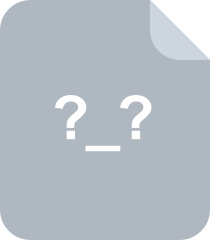
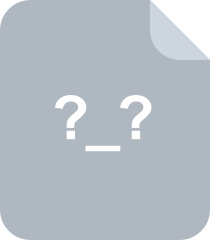
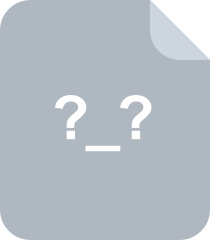
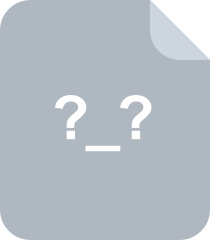
共 2002 条
- 1
- 2
- 3
- 4
- 5
- 6
- 21
资源评论
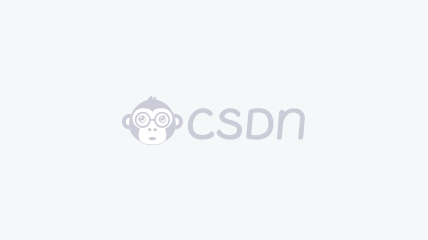

破碎的天堂鸟
- 粉丝: 8554
- 资源: 2343
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

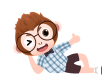
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


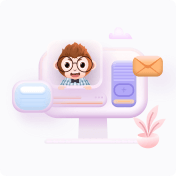
安全验证
文档复制为VIP权益,开通VIP直接复制
