<?php
/**
* display errors
*/
if( is_super_admin() ){
// error_reporting(E_ALL);
// ini_set("display_errors", 1);
}else{
// exit;
}
/**
* remove actions from wp_head
*/
remove_action( 'wp_head', 'feed_links_extra', 3 );
remove_action( 'wp_head', 'rsd_link' );
remove_action( 'wp_head', 'wlwmanifest_link' );
remove_action( 'wp_head', 'index_rel_link' );
remove_action( 'wp_head', 'start_post_rel_link', 10, 0 );
remove_action( 'wp_head', 'wp_generator' );
/**
* WordPress Emoji Delete
*/
remove_action( 'admin_print_scripts' , 'print_emoji_detection_script');
remove_action( 'admin_print_styles' , 'print_emoji_styles');
remove_action( 'wp_head' , 'print_emoji_detection_script', 7);
remove_action( 'wp_print_styles' , 'print_emoji_styles');
remove_filter( 'the_content_feed' , 'wp_staticize_emoji');
remove_filter( 'comment_text_rss' , 'wp_staticize_emoji');
remove_filter( 'wp_mail' , 'wp_staticize_emoji_for_email');
/**
* wp-json delete
*/
add_filter('rest_enabled', '_return_false');
add_filter('rest_jsonp_enabled', '_return_false');
remove_action( 'wp_head', 'rest_output_link_wp_head', 10 );
remove_action( 'wp_head', 'wp_oembed_add_discovery_links', 10 );
/**
* post formats
*/
add_theme_support( 'post-formats', array( 'gallery', 'image', 'video' ) );
add_post_type_support( 'page', 'post-formats' );
// add link manager
add_filter( 'pre_option_link_manager_enabled', '__return_true' );
/**
* add theme thumbnail
*/
if ( function_exists( 'add_theme_support' ) ) {
add_theme_support( 'post-thumbnails' );
}
/**
* register menus
*/
if (function_exists('register_nav_menus')){
register_nav_menus( array(
'nav' => __('导航'),
'bomnav' => __('底部菜单')
));
}
/**
* register sidebar
*/
if (function_exists('register_sidebar')){
$sidebars = array(
'single' => '文章页侧栏',
'page' => '页面侧栏',
);
foreach ($sidebars as $key => $value) {
register_sidebar(array(
'name' => $value,
'id' => $key,
'before_widget' => '<div class="widget %2$s">',
'after_widget' => '</div>',
'before_title' => '<h3>',
'after_title' => '</h3>'
));
};
}
/**
* the theme
*/
$current_theme = wp_get_theme();
function _the_theme_name(){
global $current_theme;
return $current_theme->get( 'Name' );
}
function _the_theme_version(){
global $current_theme;
return $current_theme->get( 'Version' );
}
function _the_theme_thumb(){
return _hui('post_default_thumb') ? _hui('post_default_thumb') : get_stylesheet_directory_uri() . '/img/thumb.png';
}
function _the_theme_avatar(){
return get_stylesheet_directory_uri() . '/img/avatar.png';
}
function _get_description_max_length(){
return 200;
}
function _get_delimiter(){
return _hui('connector') ? _hui('connector') : '-';
}
/**
* get theme option
*/
function _hui( $name, $default = false ) {
$option_name = _the_theme_name();
$options = get_option( $option_name );
if ( isset( $options[$name] ) ) {
return $options[$name];
}
return $default;
}
/**
* Widgets
*/
require_once (get_stylesheet_directory() . '/functions-widgets.php');
/**
* Functions for admin
*/
if( is_admin() ){
require_once (get_stylesheet_directory() . '/functions-admin.php');
}
/**
* target blank
*/
function _target_blank(){
return _hui('target_blank') ? ' target="_blank"' : '';
}
/**
* Disable embeds
*/
if ( !function_exists( 'disable_embeds_init' ) ) :
function disable_embeds_init(){
global $wp;
$wp->public_query_vars = array_diff($wp->public_query_vars, array('embed'));
remove_action('rest_api_init', 'wp_oembed_register_route');
add_filter('embed_oembed_discover', '__return_false');
remove_filter('oembed_dataparse', 'wp_filter_oembed_result', 10);
remove_action('wp_head', 'wp_oembed_add_discovery_links');
remove_action('wp_head', 'wp_oembed_add_host_js');
add_filter('tiny_mce_plugins', 'disable_embeds_tiny_mce_plugin');
add_filter('rewrite_rules_array', 'disable_embeds_rewrites');
}
add_action('init', 'disable_embeds_init', 9999);
function disable_embeds_tiny_mce_plugin($plugins){
return array_diff($plugins, array('wpembed'));
}
function disable_embeds_rewrites($rules){
foreach ($rules as $rule => $rewrite) {
if (false !== strpos($rewrite, 'embed=true')) {
unset($rules[$rule]);
}
}
return $rules;
}
function disable_embeds_remove_rewrite_rules(){
add_filter('rewrite_rules_array', 'disable_embeds_rewrites');
flush_rewrite_rules();
}
register_activation_hook(__FILE__, 'disable_embeds_remove_rewrite_rules');
function disable_embeds_flush_rewrite_rules(){
remove_filter('rewrite_rules_array', 'disable_embeds_rewrites');
flush_rewrite_rules();
}
register_deactivation_hook(__FILE__, 'disable_embeds_flush_rewrite_rules');
endif;
/**
* SSL Gravatar
*/
if (!function_exists('_get_ssl2_avatar')) :
function _get_ssl2_avatar($avatar) {
$avatar = preg_replace('/.*\/avatar\/(.*)\?s=([\d]+)&.*/','<img src="https://secure.gravatar.com/avatar/$1?s=$2&d=mm" class="avatar avatar-$2" height="50" width="50">',$avatar);
return $avatar;
}
add_filter('get_avatar', '_get_ssl2_avatar');
endif;
/**
* Remove Open Sans that WP adds from frontend
*/
if (!function_exists('remove_wp_open_sans')) :
function remove_wp_open_sans() {
wp_deregister_style( 'open-sans' );
wp_register_style( 'open-sans', false );
}
add_action('wp_enqueue_scripts', 'remove_wp_open_sans');
endif;
if (!function_exists('remove_open_sans')) :
function remove_open_sans() {
wp_deregister_style( 'open-sans' );
wp_register_style( 'open-sans', false );
wp_enqueue_style('open-sans','');
}
add_action( 'init', 'remove_open_sans' );
endif;
/**
* title
*/
function _title() {
global $paged;
$html = '';
$t = trim(wp_title('', false));
if ($t) {
$html .= $t . _get_delimiter();
}
if ( get_query_var('page') ) {
$html .= '第' . get_query_var('page') . '页' . _get_delimiter();
}
$html .= get_bloginfo('name');
if (is_home()) {
if ($paged > 1) {
$html .= _get_delimiter() . '最新发布';
}elseif( get_option('blogdescription') ){
$html .= _get_delimiter() . get_option('blogdescription');
}
}
if( is_category() ){
global $wp_query;
$cat_ID = get_query_var('cat');
$cat_tit = _get_tax_meta($cat_ID, 'title');
if( $cat_tit ){
$html = $cat_tit;
}
}
if ($paged > 1) {
$html .= _get_delimiter() . '第' . $paged . '页';
}
return $html;
}
/**
* menu
*/
function _the_menu($location = 'nav') {
echo str_replace("</ul></div>", "", preg_replace("/<div[^>]*><ul[^>]*>/", "", wp_nav_menu(array('theme_location' => $location, 'echo' => false))));
}
/**
* logo
*/
function _the_logo() {
$tag = is_home() ? 'h1' : 'div';
$src = _hui('logo_src');
if( wp_is_mobile() && _hui('logo_src_m') ){
$src = _hui('logo_src_m');
}
echo '<' . $tag . ' class="logo"><a href="' . get_bloginfo('url') . '" title="' . get_bloginfo('name') . ( get_bloginfo('description')?_get_delimiter() . get_bloginfo('
没有合适的资源?快使用搜索试试~ 我知道了~
wordpress高端多功能图片主题tob1.0无限制版
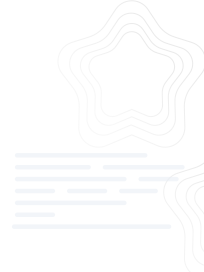
共90个文件
php:47个
gif:23个
png:7个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
1 下载量 200 浏览量
2024-10-07
20:41:09
上传
评论
收藏 239KB ZIP 举报
温馨提示
tob主题是由国内知名WP主题制作团队themebetter出品,适用于各种图片展示网站、新闻站、电影站、美图站、资源站等等,扁平化设计、公众号展示、打赏功能、列表无限加载、相册功能等。 tob主题1.0版本更新内容: 新增首页置顶文章,默认开启无需设置,置顶标记文字可自定义,主题设置-首页中可修改 新增文章评论整体开关,主题设置-基本中可设置 新增手机端列表布局三种选择:新闻列表、一图一行、两图一行 新增图片、视频、相册类型文章显示标签和上下文章 新增主题全面兼容PHP 7.3 新增对WordPress5.0新版编辑器的兼容支持 调整底部友情链接选择只在首页显示时只在首页第一页显示,不显示在首页分页 调整视频类型文章在列表中的展示图标风格 调整分类下子分类的显示顺序按名称排序 优化小工具画廊的展示 优化缩略图细节展示 修复文章中相册图片可能存在的点击失效的问题 修复当设置某些分类的文章不显示在首页时的分页页码错误 修复WordPress5.1后Ajax评论回复功能失效 修复开启debug后的各种错误提示 删除首页智能列表功能,使用置顶文章更加简单更加高效 删除JS文件托管的选项
资源推荐
资源详情
资源评论
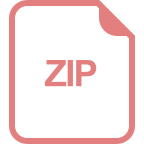
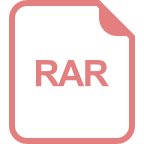
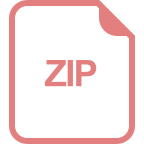
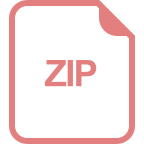
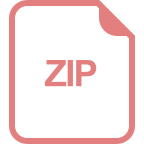
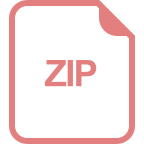
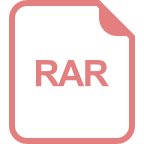
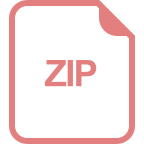
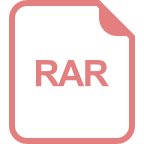
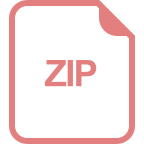
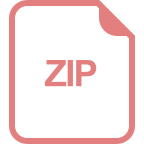
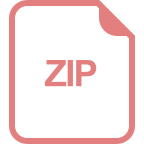
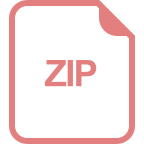
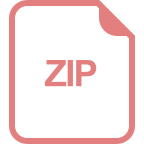
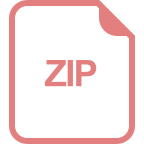
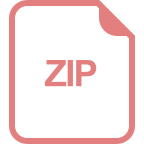
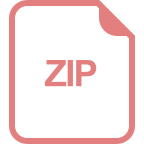
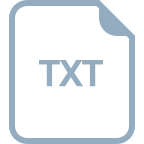
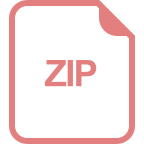
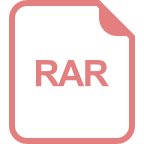
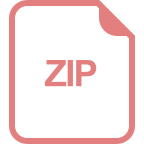
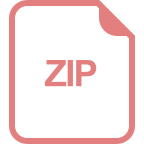
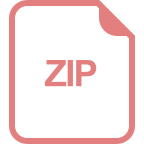
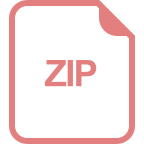
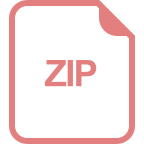
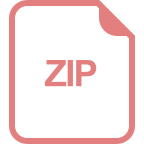
收起资源包目录



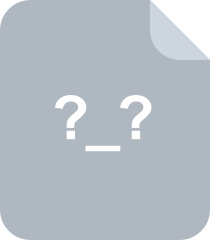
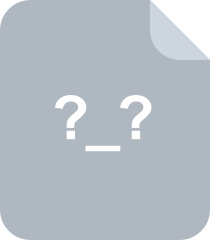

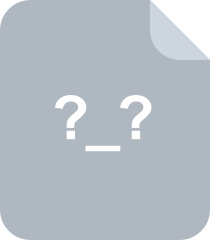
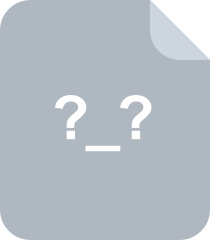
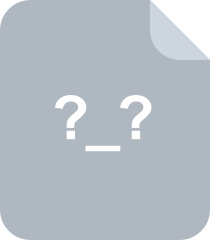
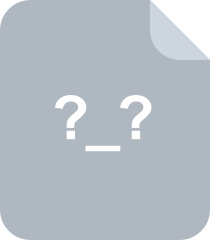
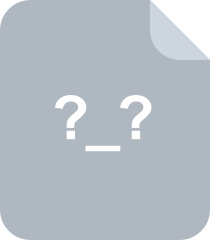
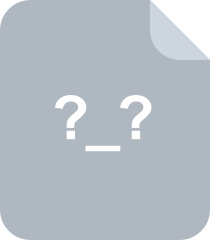
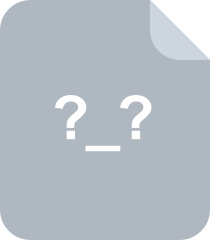
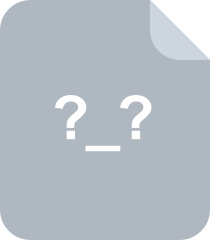


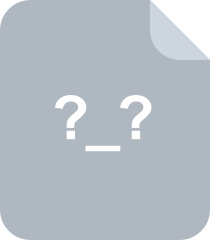
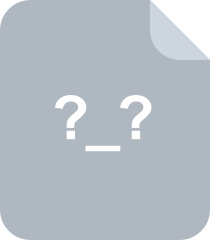
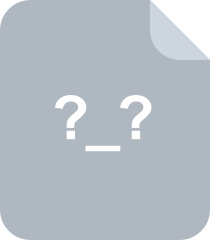
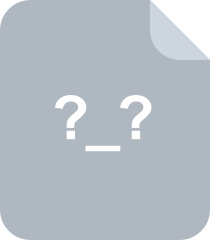
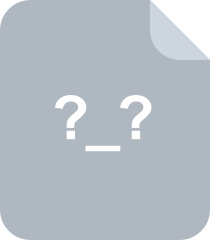

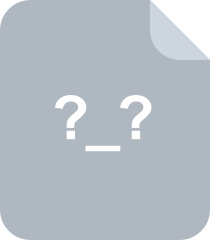
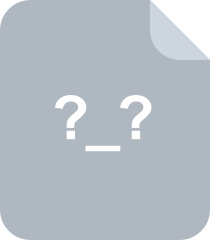
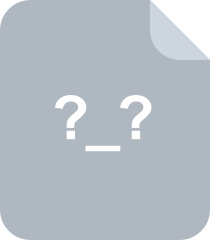
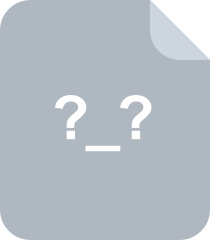
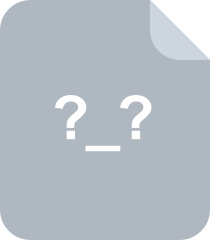

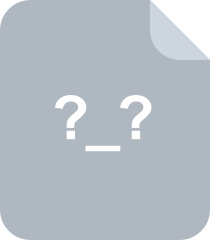

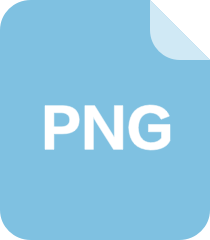
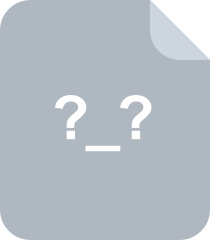
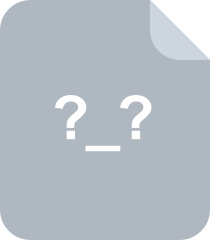
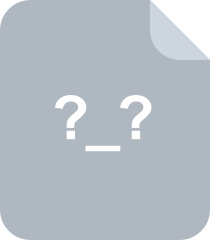
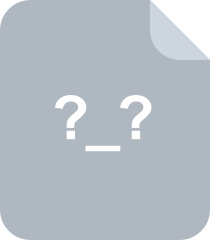
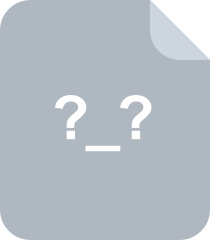
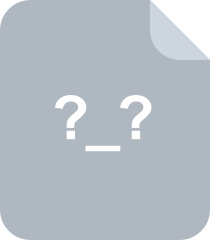
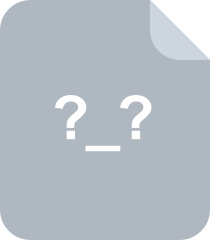

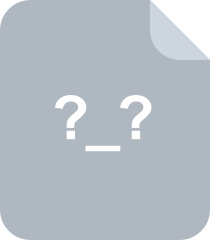
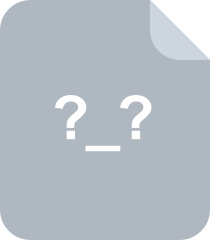
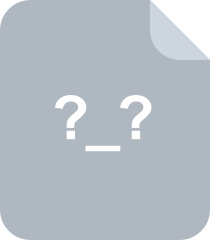
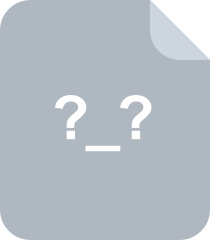
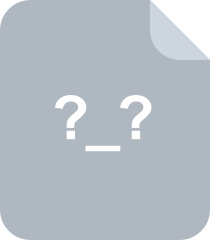
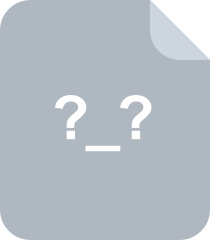
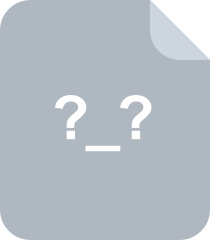

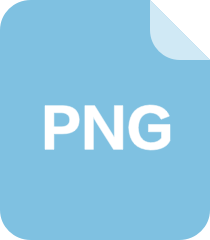
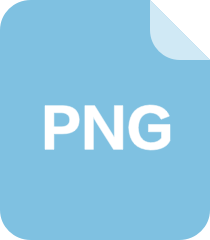
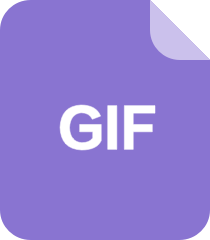
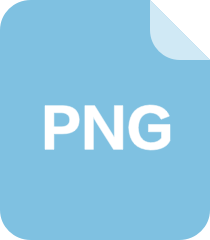
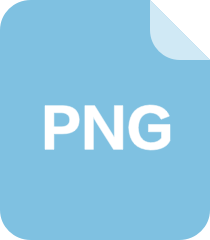
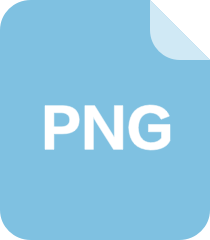
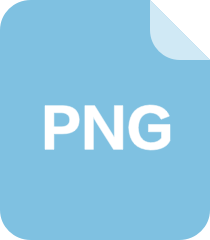

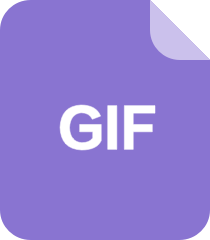
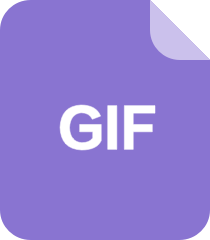
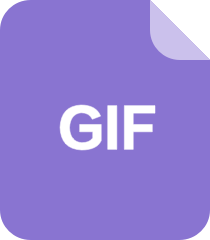
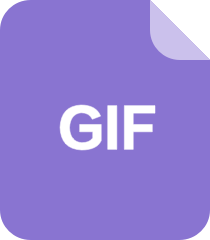
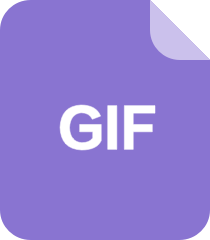
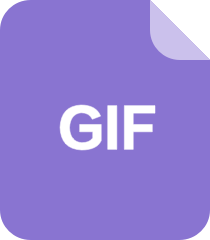
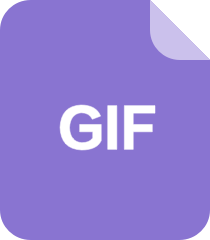
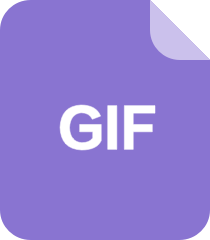
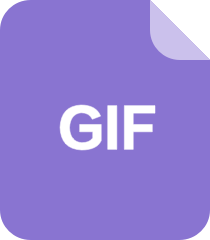
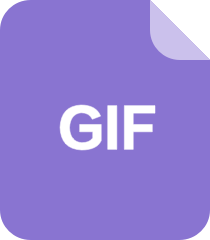
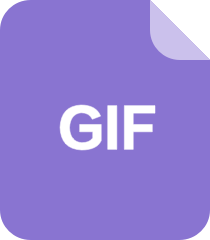
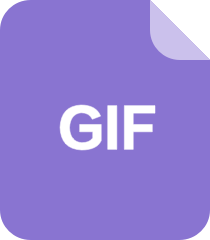
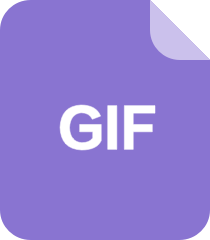
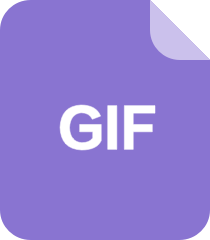
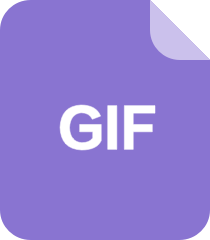
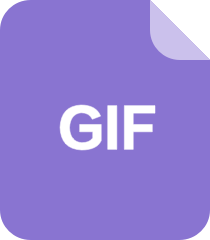
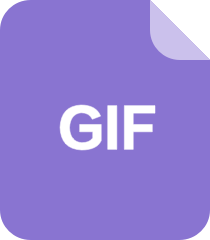
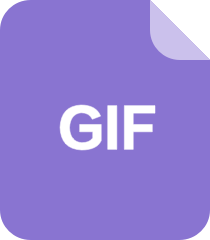
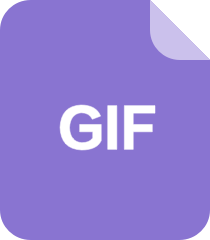
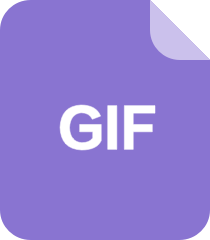
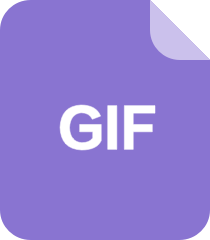
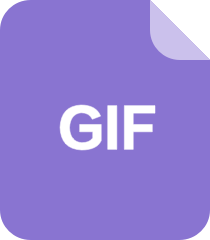
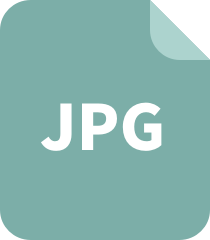
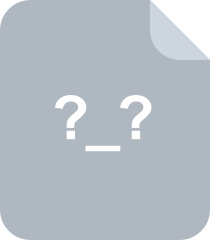
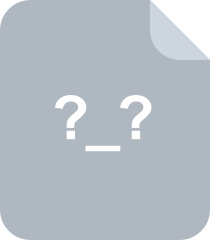
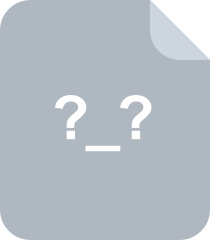
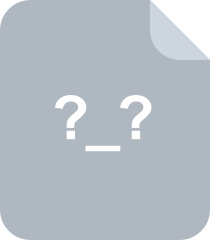
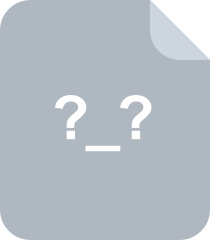
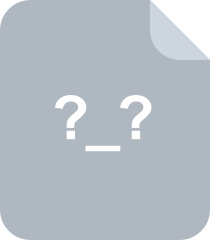
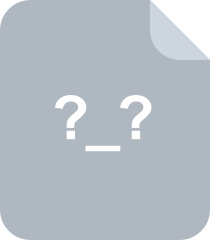
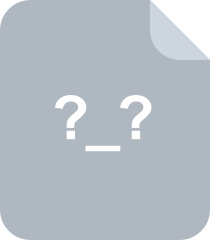
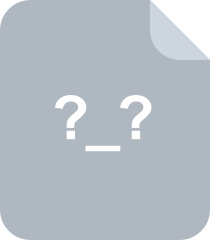
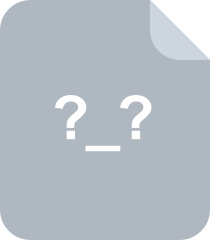
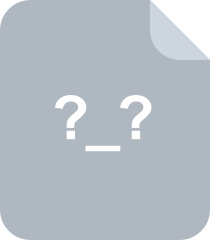
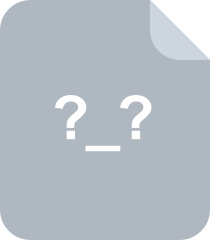
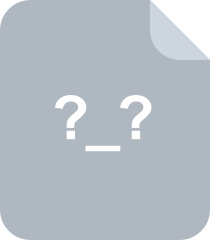
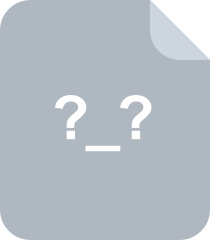
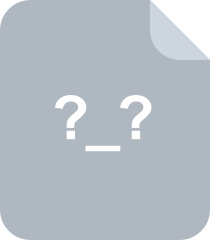
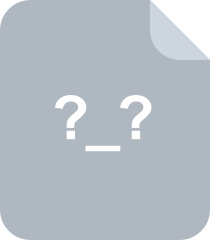
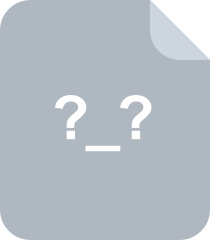

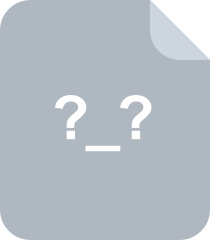
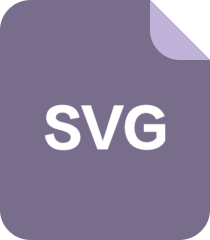
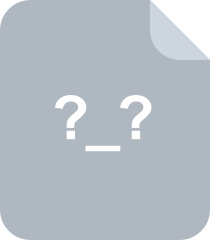
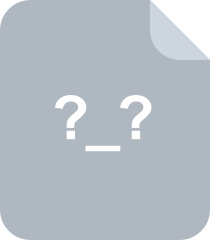
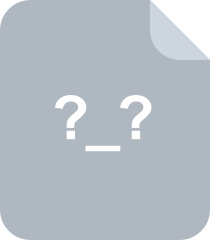

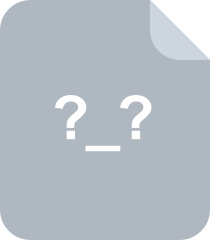
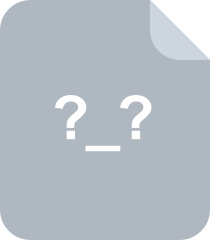
共 90 条
- 1
资源评论
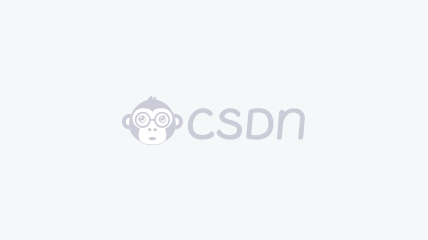

破碎的天堂鸟
- 粉丝: 9420
- 资源: 2680
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

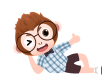
最新资源
- 毕设和企业适用springboot众筹平台类及医疗诊断系统源码+论文+视频.zip
- 毕设和企业适用springboot众筹平台类及在线订餐系统源码+论文+视频.zip
- 毕设和企业适用springboot众筹平台类及远程医疗平台源码+论文+视频.zip
- 毕设和企业适用springboot众筹平台类及智能农业解决方案源码+论文+视频.zip
- 毕设和企业适用springboot众筹平台类及智能交通管理平台源码+论文+视频.zip
- 毕设和企业适用springboot众筹平台类及智能物流调度平台源码+论文+视频.zip
- 毕设和企业适用springboot自动化仓库管理平台类及电商产品推荐平台源码+论文+视频.zip
- 毕设和企业适用springboot自动化仓库管理平台类及环境监控平台源码+论文+视频.zip
- 毕设和企业适用springboot自动化仓库管理平台类及活动管理平台源码+论文+视频.zip
- 毕设和企业适用springboot自动化仓库管理平台类及技术文档管理平台源码+论文+视频.zip
- 毕设和企业适用springboot自动化仓库管理平台类及教育信息平台源码+论文+视频.zip
- 毕设和企业适用springboot自动化仓库管理平台类及全渠道电商平台源码+论文+视频.zip
- 毕设和企业适用springboot自动化仓库管理平台类及物联网监控平台源码+论文+视频.zip
- 毕设和企业适用springboot自动化仓库管理平台类及无线通信平台源码+论文+视频.zip
- 毕设和企业适用springboot自动化仓库管理平台类及招聘管理平台源码+论文+视频.zip
- 毕设和企业适用springboot自动化仓库管理平台类及新闻传播平台源码+论文+视频.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


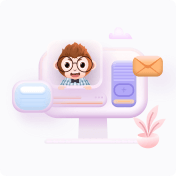
安全验证
文档复制为VIP权益,开通VIP直接复制
