package com.crm.info;
import java.util.Date;
import java.util.HashSet;
import java.util.Set;
/**
* HrEmployee entity. @author MyEclipse Persistence Tools
*/
public class HrEmployee implements java.io.Serializable {
// Fields
private Integer id;
private HrPosition hrPosition;
private ParamSysparam paramSysparam;
private HrDepartment hrDepartment;
private SysRole sysRole;
private HrPost hrPost;
private String uid;
private String pwd;
private String name;
private String idcard;
private Date birthday;
private String email;
private String sex;
private String telephone;
private String status;
private Integer sort;
private Date entrydate;
private String address;
private String remarks;
private String education;
private String professional;
private String schools;
private String title;
private Integer isdelete;
private Date deleteTime;
private String portal;
private String theme;
private Integer canlogin;
private String layout;
private Date loginoutdate;
private Integer ispost;
private Set crmReceivesForCEmpid = new HashSet(0);
private Set paramCitiesForUpdateId = new HashSet(0);
private Set paramCitiesForCreateId = new HashSet(0);
private Set crmReceivesForCreateId = new HashSet(0);
private Set sysLogins = new HashSet(0);
private Set crmContractsForCEmpid = new HashSet(0);
private Set sysRolesForUpdateid = new HashSet(0);
private Set sysRolesForCreateid = new HashSet(0);
private Set paramSysparamsForUpdateId = new HashSet(0);
private Set publicNotices = new HashSet(0);
private Set hrPosts = new HashSet(0);
private Set crmFollows = new HashSet(0);
private Set paramSysparamsForCreateId = new HashSet(0);
private Set crmInvoicesForCreateId = new HashSet(0);
private Set crmContacts = new HashSet(0);
private Set crmOrdersForCreateId = new HashSet(0);
private Set crmOrdersForFEmpId = new HashSet(0);
private Set crmProductCategories = new HashSet(0);
private Set crmOrdersForCEmpId = new HashSet(0);
private Set personalNoteses = new HashSet(0);
private Set crmInvoicesForCEmpid = new HashSet(0);
private Set publicChatRooms = new HashSet(0);
private Set personalCalendars = new HashSet(0);
private Set hrPositions = new HashSet(0);
private Set mailFlowsForReceiveId = new HashSet(0);
private Set personalEvents = new HashSet(0);
private Set mailFlowsForSenderId = new HashSet(0);
private Set crmCustomersForCreateId = new HashSet(0);
private Set crmCustomersForEmployeeId = new HashSet(0);
private Set publicNewses = new HashSet(0);
private Set crmContractsForCreaterId = new HashSet(0);
private Set crmContractsForOurContractorId = new HashSet(0);
// Constructors
/** default constructor */
public HrEmployee() {
}
/** full constructor */
public HrEmployee(HrPosition hrPosition, ParamSysparam paramSysparam,
HrDepartment hrDepartment, SysRole sysRole, HrPost hrPost,
String uid, String pwd, String name, String idcard, Date birthday,
String email, String sex, String telephone, String status,
Integer sort, Date entrydate, String address, String remarks,
String education, String professional, String schools,
String title, Integer isdelete, Date deleteTime, String portal,
String theme, Integer canlogin, String layout, Date loginoutdate,
Integer ispost, Set crmReceivesForCEmpid,
Set paramCitiesForUpdateId, Set paramCitiesForCreateId,
Set crmReceivesForCreateId, Set sysLogins,
Set crmContractsForCEmpid, Set sysRolesForUpdateid,
Set sysRolesForCreateid, Set paramSysparamsForUpdateId,
Set publicNotices, Set hrPosts, Set crmFollows,
Set paramSysparamsForCreateId, Set crmInvoicesForCreateId,
Set crmContacts, Set crmOrdersForCreateId, Set crmOrdersForFEmpId,
Set crmProductCategories, Set crmOrdersForCEmpId,
Set personalNoteses, Set crmInvoicesForCEmpid, Set publicChatRooms,
Set personalCalendars, Set hrPositions, Set mailFlowsForReceiveId,
Set personalEvents, Set mailFlowsForSenderId,
Set crmCustomersForCreateId, Set crmCustomersForEmployeeId,
Set publicNewses, Set crmContractsForCreaterId,
Set crmContractsForOurContractorId) {
this.hrPosition = hrPosition;
this.paramSysparam = paramSysparam;
this.hrDepartment = hrDepartment;
this.sysRole = sysRole;
this.hrPost = hrPost;
this.uid = uid;
this.pwd = pwd;
this.name = name;
this.idcard = idcard;
this.birthday = birthday;
this.email = email;
this.sex = sex;
this.telephone = telephone;
this.status = status;
this.sort = sort;
this.entrydate = entrydate;
this.address = address;
this.remarks = remarks;
this.education = education;
this.professional = professional;
this.schools = schools;
this.title = title;
this.isdelete = isdelete;
this.deleteTime = deleteTime;
this.portal = portal;
this.theme = theme;
this.canlogin = canlogin;
this.layout = layout;
this.loginoutdate = loginoutdate;
this.ispost = ispost;
this.crmReceivesForCEmpid = crmReceivesForCEmpid;
this.paramCitiesForUpdateId = paramCitiesForUpdateId;
this.paramCitiesForCreateId = paramCitiesForCreateId;
this.crmReceivesForCreateId = crmReceivesForCreateId;
this.sysLogins = sysLogins;
this.crmContractsForCEmpid = crmContractsForCEmpid;
this.sysRolesForUpdateid = sysRolesForUpdateid;
this.sysRolesForCreateid = sysRolesForCreateid;
this.paramSysparamsForUpdateId = paramSysparamsForUpdateId;
this.publicNotices = publicNotices;
this.hrPosts = hrPosts;
this.crmFollows = crmFollows;
this.paramSysparamsForCreateId = paramSysparamsForCreateId;
this.crmInvoicesForCreateId = crmInvoicesForCreateId;
this.crmContacts = crmContacts;
this.crmOrdersForCreateId = crmOrdersForCreateId;
this.crmOrdersForFEmpId = crmOrdersForFEmpId;
this.crmProductCategories = crmProductCategories;
this.crmOrdersForCEmpId = crmOrdersForCEmpId;
this.personalNoteses = personalNoteses;
this.crmInvoicesForCEmpid = crmInvoicesForCEmpid;
this.publicChatRooms = publicChatRooms;
this.personalCalendars = personalCalendars;
this.hrPositions = hrPositions;
this.mailFlowsForReceiveId = mailFlowsForReceiveId;
this.personalEvents = personalEvents;
this.mailFlowsForSenderId = mailFlowsForSenderId;
this.crmCustomersForCreateId = crmCustomersForCreateId;
this.crmCustomersForEmployeeId = crmCustomersForEmployeeId;
this.publicNewses = publicNewses;
this.crmContractsForCreaterId = crmContractsForCreaterId;
this.crmContractsForOurContractorId = crmContractsForOurContractorId;
}
// Property accessors
public Integer getId() {
return this.id;
}
public void setId(Integer id) {
this.id = id;
}
public HrPosition getHrPosition() {
return this.hrPosition;
}
public void setHrPosition(HrPosition hrPosition) {
this.hrPosition = hrPosition;
}
public ParamSysparam getParamSysparam() {
return this.paramSysparam;
}
public void setParamSysparam(ParamSysparam paramSysparam) {
this.paramSysparam = paramSysparam;
}
public HrDepartment getHrDepartment() {
return this.hrDepartment;
}
public void setHrDepartment(HrDepartment hrDepartment) {
this.hrDepartment = hrDepartment;
}
public SysRole getSysRole() {
return this.sysRole;
}
public void setSysRole(SysRole sysRole) {
this.sysRole = sysRole;
}
public HrPost getHrPost() {
return this.hrPost;
}
public void setHrPost(HrPost hrPost) {
this.hrPost = hrPost;
}
public String getUid() {
return this.uid;
}
public void setUid(String uid) {
this.uid = uid;
}
public String getPwd() {
return this.pwd;
}
public void setPwd(String pwd) {
this.pwd = pwd;
}
public String getName() {
return this.name;
}
public void setName(String name) {
this.name = name;
}
public String getIdcard() {
return this.idcard;
}
public void setIdcard(String idcard) {
this.idcard = idcard;
}
public Date getBirthday() {
return this.birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
public String getEmail() {
return this.email;
}
public void setEmail(S
没有合适的资源?快使用搜索试试~ 我知道了~
METRO客户关系管理系统源码CRM整站源码 java源码 CRM源码 企业客户关系管理系统源码
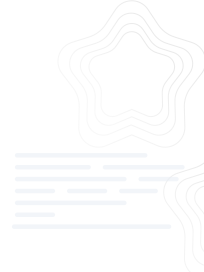
共1175个文件
png:265个
js:240个
class:127个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 182 浏览量
2024-09-04
20:09:13
上传
评论
收藏 9.95MB ZIP 举报
温馨提示
某java客户关系管理系统源码CRM源码,系统包括:个人工作、信息中心、客户管理、合同订单、财务管理、产品管理、人事管理以及数据回收站等8个模块。另包括权限管理模块用于系统的用户、角色和相关权限,收发邮件功能用于获得客户的详细需求,文档管理功能用于客户信息文件的存储。 安装说明: 详细见压缩包,不懂JAVA的不要下
资源推荐
资源详情
资源评论
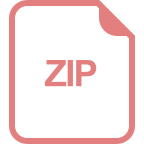
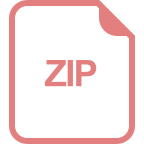
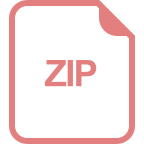
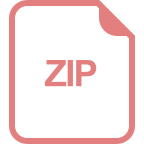
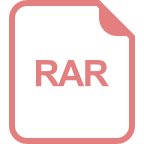
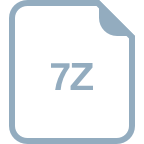
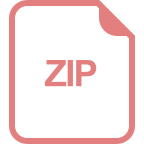
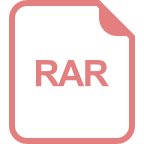
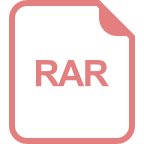
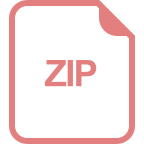
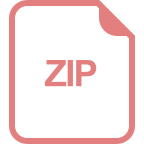
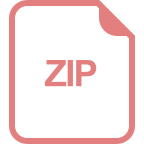
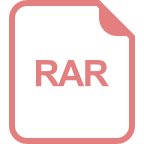
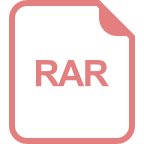
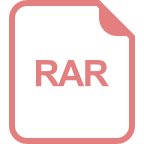
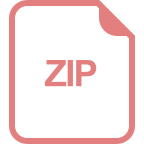
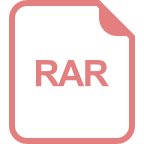
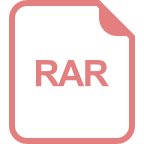
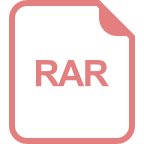
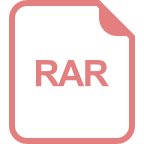
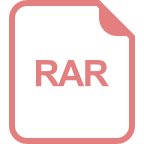
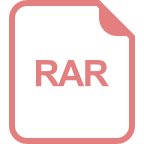
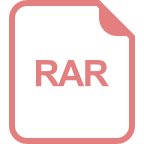
收起资源包目录

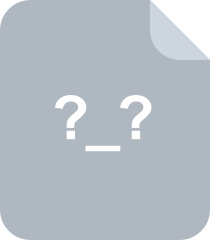
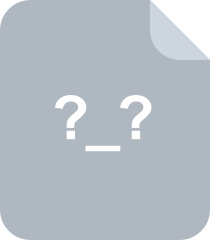
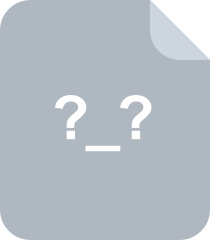
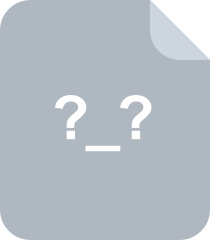
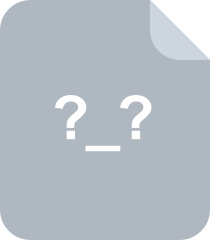
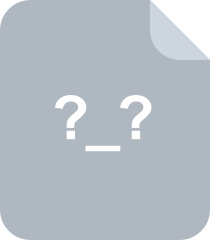
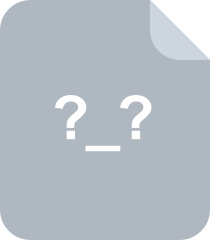
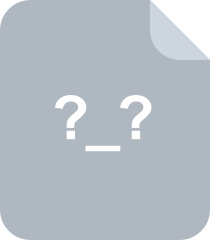
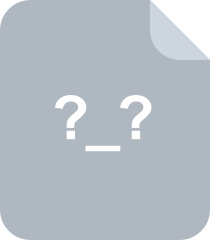
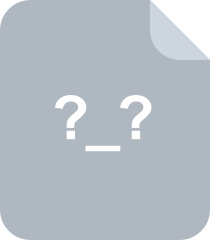
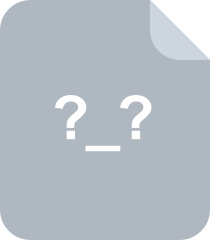
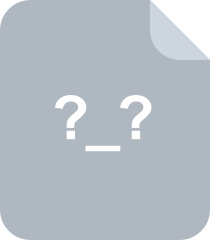
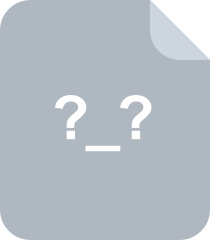
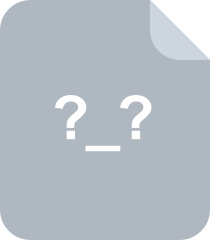
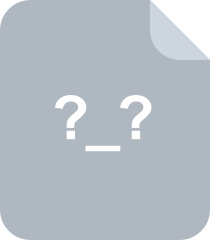
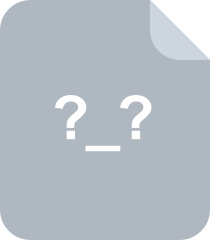
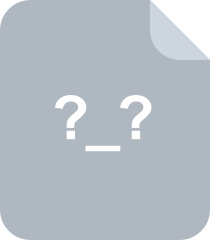
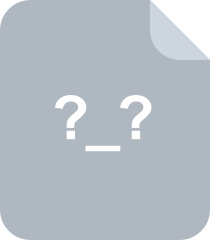
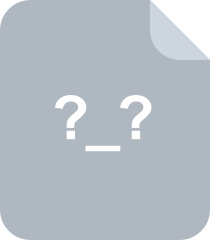
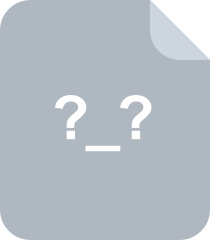
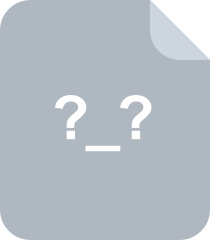
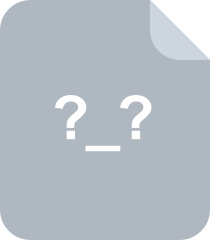
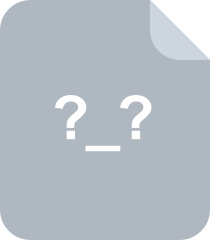
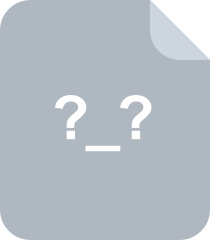
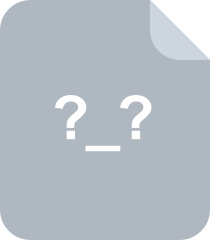
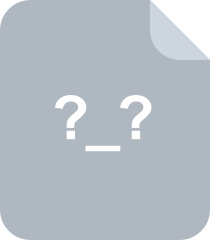
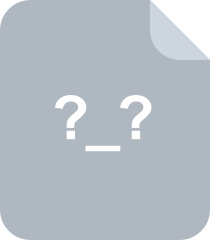
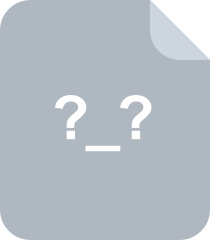
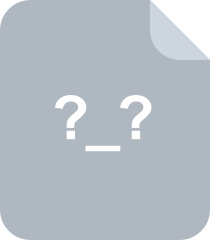
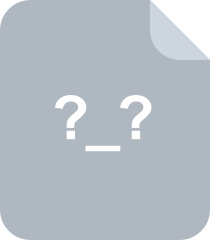
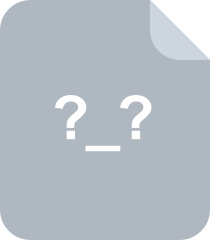
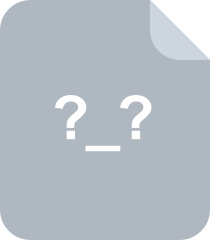
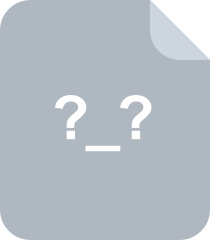
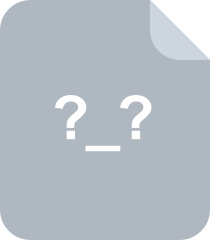
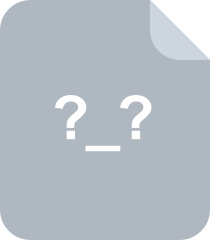
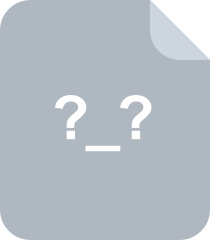
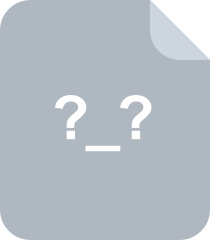
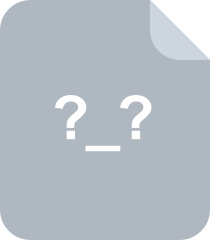
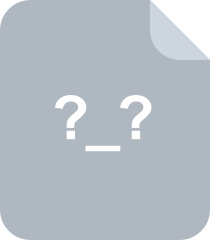
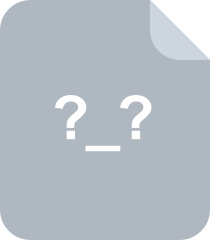
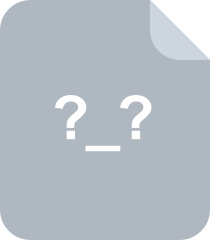
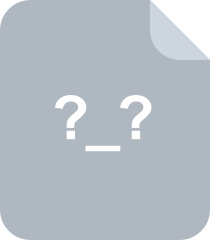
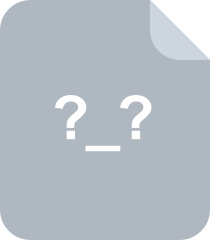
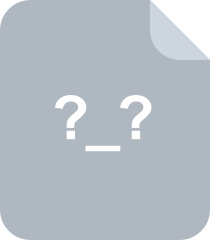
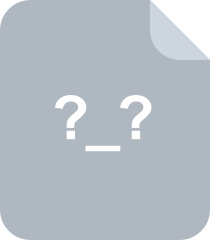
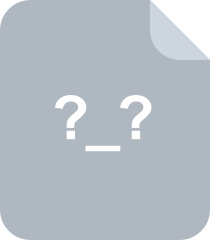
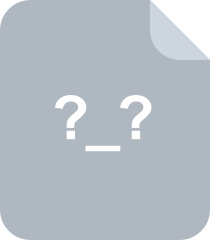
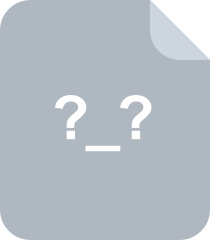
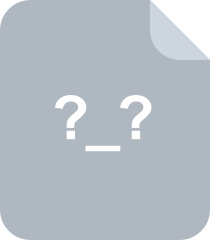
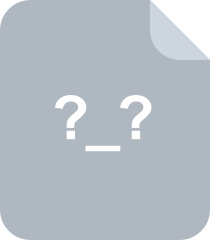
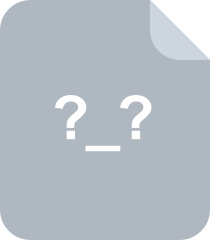
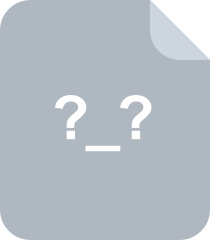
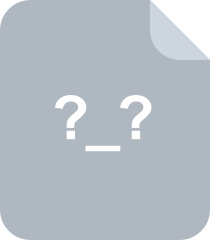
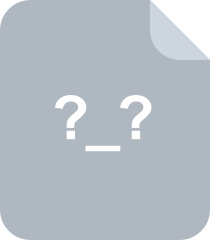
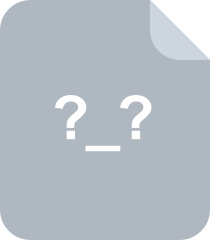
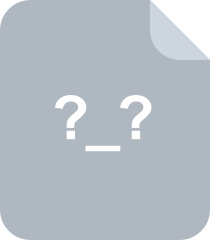
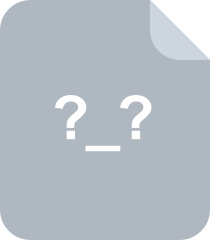
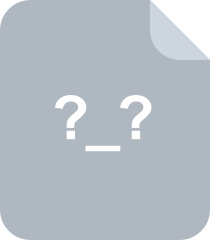
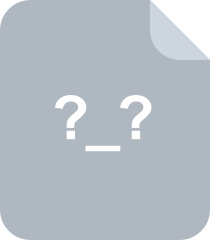
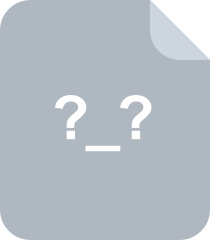
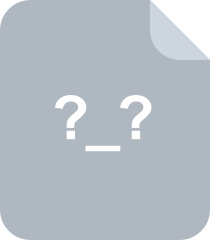
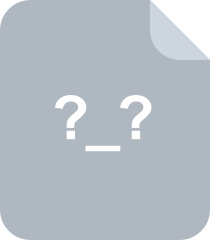
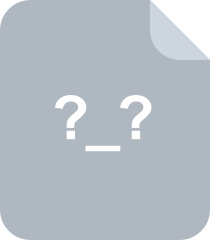
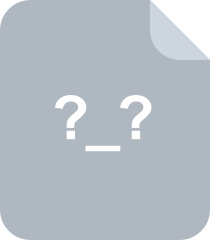
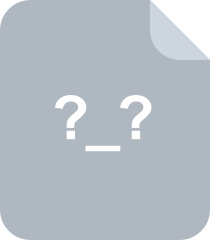
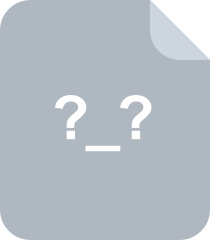
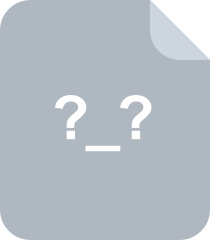
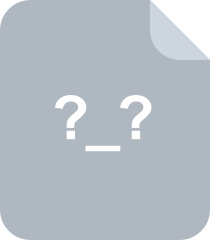
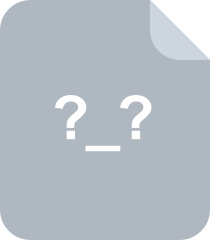
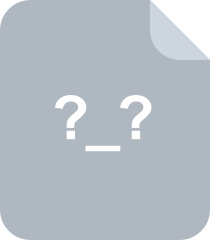
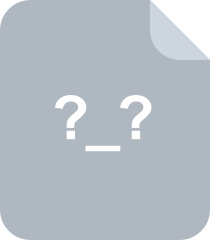
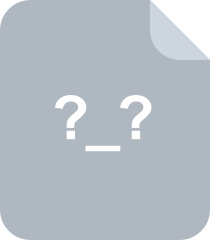
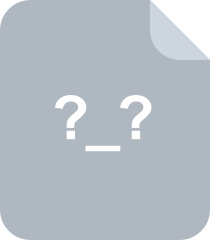
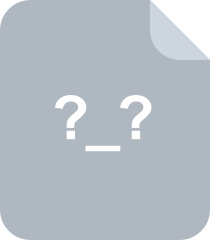
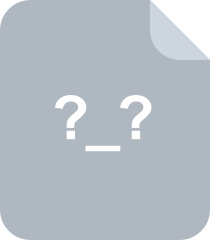
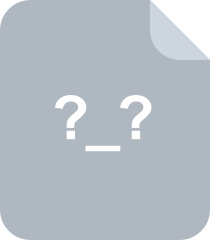
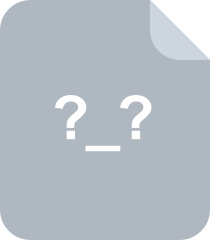
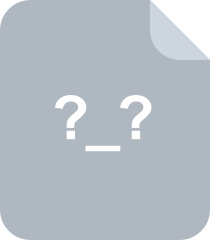
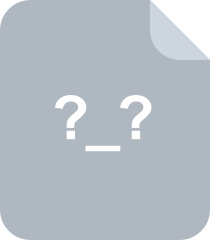
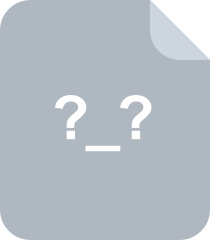
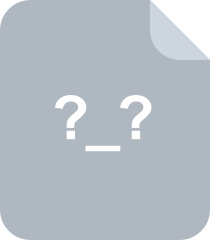
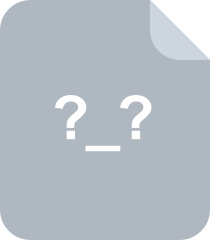
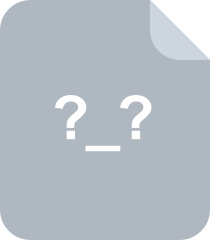
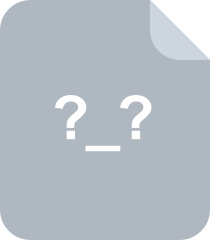
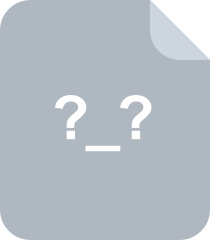
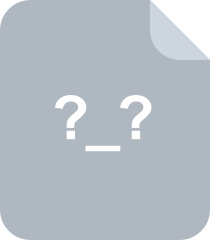
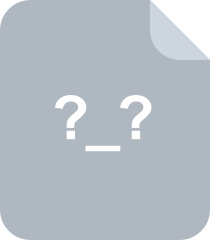
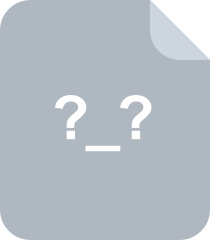
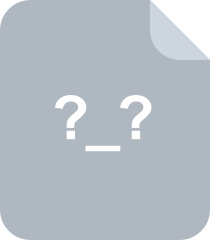
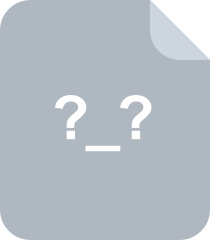
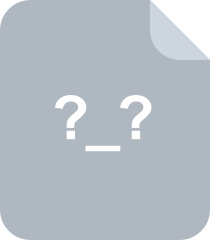
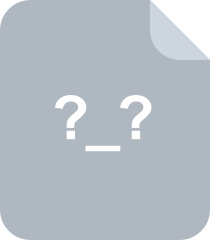
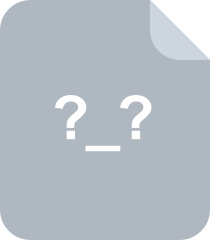
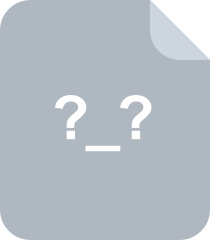
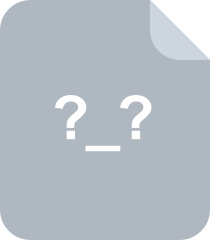
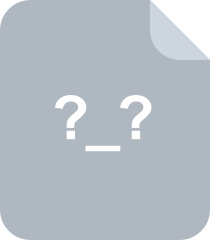
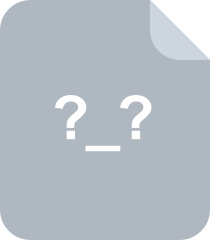
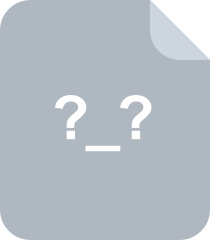
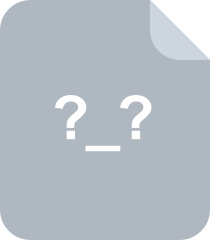
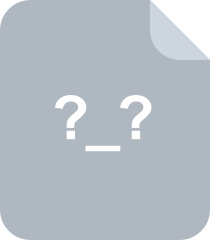
共 1175 条
- 1
- 2
- 3
- 4
- 5
- 6
- 12
资源评论
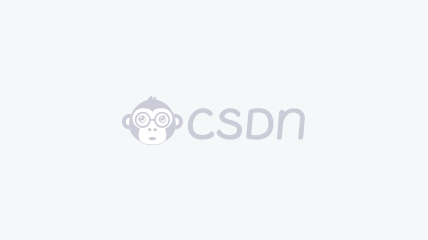

破碎的天堂鸟
- 粉丝: 9729
- 资源: 2845

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

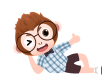
最新资源
- 机械设计晶圆切割铺展机x_t全套技术资料100%好用.zip
- 新能源汽车转向系统设计计算书,excel格式
- matlab simulink仿真,蓄电池超级电容器协调控制,完美跟踪给定功率曲线,功率变化快的部分由超级电容出力,功率变化慢的地方由蓄电池出力
- 机械设计开关电源适配器电路自动点焊生产线sw17可编辑全套技术资料100%好用.zip
- 机械设计快递柜电机驱动锁sw18可编辑全套技术资料100%好用.zip
- 分享一批广州的美食数据.zip
- 350-401 Exam.PDF
- FDTD方法在90度楔形波散射模拟中的应用及其数值优化
- 计算机视觉领域:YOLO与SAM在飞机甲板目标分割应用的技术实现与比较
- 机械设计垃圾桶提升机sw16可编辑全套技术资料100%好用.zip
- 机械设计蓝牙锁机械驱动结构设计sw17可编辑全套技术资料100%好用.zip
- 直方图均衡化_QQ浏览器压缩包.zip
- 机械设计矿车自冷电池包sw17可编辑全套技术资料100%好用.zip
- AZ-103-Certking.pdf
- 机械设计两叉固定机sw13可编辑全套技术资料100%好用.zip
- AZ-900 - 186q.vce
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


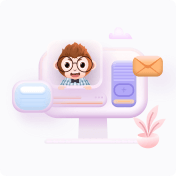
安全验证
文档复制为VIP权益,开通VIP直接复制
