<?php
// +----------------------------------------------------------------------
// | ThinkPHP [ WE CAN DO IT JUST THINK ]
// +----------------------------------------------------------------------
// | Copyright (c) 2006~2021 http://thinkphp.cn All rights reserved.
// +----------------------------------------------------------------------
// | Licensed ( http://www.apache.org/licenses/LICENSE-2.0 )
// +----------------------------------------------------------------------
// | Author: liu21st <liu21st@gmail.com>
// +----------------------------------------------------------------------
declare (strict_types = 1);
namespace think;
use ArrayAccess;
use think\facade\Lang;
use think\file\UploadedFile;
use think\route\Rule;
/**
* 请求管理类
* @package think
*/
class Request implements ArrayAccess
{
/**
* 兼容PATH_INFO获取
* @var array
*/
protected $pathinfoFetch = ['ORIG_PATH_INFO', 'REDIRECT_PATH_INFO', 'REDIRECT_URL'];
/**
* PATHINFO变量名 用于兼容模式
* @var string
*/
protected $varPathinfo = 's';
/**
* 请求类型
* @var string
*/
protected $varMethod = '_method';
/**
* 表单ajax伪装变量
* @var string
*/
protected $varAjax = '_ajax';
/**
* 表单pjax伪装变量
* @var string
*/
protected $varPjax = '_pjax';
/**
* 域名根
* @var string
*/
protected $rootDomain = '';
/**
* HTTPS代理标识
* @var string
*/
protected $httpsAgentName = '';
/**
* 前端代理服务器IP
* @var array
*/
protected $proxyServerIp = [];
/**
* 前端代理服务器真实IP头
* @var array
*/
protected $proxyServerIpHeader = ['HTTP_X_REAL_IP', 'HTTP_X_FORWARDED_FOR', 'HTTP_CLIENT_IP', 'HTTP_X_CLIENT_IP', 'HTTP_X_CLUSTER_CLIENT_IP'];
/**
* 请求类型
* @var string
*/
protected $method;
/**
* 域名(含协议及端口)
* @var string
*/
protected $domain;
/**
* HOST(含端口)
* @var string
*/
protected $host;
/**
* 子域名
* @var string
*/
protected $subDomain;
/**
* 泛域名
* @var string
*/
protected $panDomain;
/**
* 当前URL地址
* @var string
*/
protected $url;
/**
* 基础URL
* @var string
*/
protected $baseUrl;
/**
* 当前执行的文件
* @var string
*/
protected $baseFile;
/**
* 访问的ROOT地址
* @var string
*/
protected $root;
/**
* pathinfo
* @var string
*/
protected $pathinfo;
/**
* pathinfo(不含后缀)
* @var string
*/
protected $path;
/**
* 当前请求的IP地址
* @var string
*/
protected $realIP;
/**
* 当前控制器名
* @var string
*/
protected $controller;
/**
* 当前操作名
* @var string
*/
protected $action;
/**
* 当前请求参数
* @var array
*/
protected $param = [];
/**
* 当前GET参数
* @var array
*/
protected $get = [];
/**
* 当前POST参数
* @var array
*/
protected $post = [];
/**
* 当前REQUEST参数
* @var array
*/
protected $request = [];
/**
* 当前路由对象
* @var Rule
*/
protected $rule;
/**
* 当前ROUTE参数
* @var array
*/
protected $route = [];
/**
* 中间件传递的参数
* @var array
*/
protected $middleware = [];
/**
* 当前PUT参数
* @var array
*/
protected $put;
/**
* SESSION对象
* @var Session
*/
protected $session;
/**
* COOKIE数据
* @var array
*/
protected $cookie = [];
/**
* ENV对象
* @var Env
*/
protected $env;
/**
* 当前SERVER参数
* @var array
*/
protected $server = [];
/**
* 当前FILE参数
* @var array
*/
protected $file = [];
/**
* 当前HEADER参数
* @var array
*/
protected $header = [];
/**
* 资源类型定义
* @var array
*/
protected $mimeType = [
'xml' => 'application/xml,text/xml,application/x-xml',
'json' => 'application/json,text/x-json,application/jsonrequest,text/json',
'js' => 'text/javascript,application/javascript,application/x-javascript',
'css' => 'text/css',
'rss' => 'application/rss+xml',
'yaml' => 'application/x-yaml,text/yaml',
'atom' => 'application/atom+xml',
'pdf' => 'application/pdf',
'text' => 'text/plain',
'image' => 'image/png,image/jpg,image/jpeg,image/pjpeg,image/gif,image/webp,image/*',
'csv' => 'text/csv',
'html' => 'text/html,application/xhtml+xml,*/*',
];
/**
* 当前请求内容
* @var string
*/
protected $content;
/**
* 全局过滤规则
* @var array
*/
protected $filter;
/**
* php://input内容
* @var string
*/
// php://input
protected $input;
/**
* 请求安全Key
* @var string
*/
protected $secureKey;
/**
* 是否合并Param
* @var bool
*/
protected $mergeParam = false;
/**
* 架构函数
* @access public
*/
public function __construct()
{
// 保存 php://input
$this->input = file_get_contents('php://input');
}
public static function __make(App $app)
{
$request = new static();
if (function_exists('apache_request_headers') && $result = apache_request_headers()) {
$header = $result;
} else {
$header = [];
$server = $_SERVER;
foreach ($server as $key => $val) {
if (0 === strpos($key, 'HTTP_')) {
$key = str_replace('_', '-', strtolower(substr($key, 5)));
$header[$key] = $val;
}
}
if (isset($server['CONTENT_TYPE'])) {
$header['content-type'] = $server['CONTENT_TYPE'];
}
if (isset($server['CONTENT_LENGTH'])) {
$header['content-length'] = $server['CONTENT_LENGTH'];
}
}
$request->header = array_change_key_case($header);
$request->server = $_SERVER;
$request->env = $app->env;
$inputData = $request->getInputData($request->input);
$request->get = $_GET;
$request->post = $_POST ?: $inputData;
$request->put = $inputData;
$request->request = $_REQUEST;
$request->cookie = $_COOKIE;
$request->file = $_FILES ?? [];
return $request;
}
/**
* 设置当前包含协议的域名
* @access public
* @param string $domain 域名
* @return $this
*/
public function setDomain(string $domain)
{
$this->domain = $domain;
return $this;
}
/**
* 获取当前包含协议的域名
* @access public
* @param bool $port 是否需要去除端口号
* @return string
*/
public function domain(bool $port = false): string
{
return $this->scheme() . '://' . $this->host($port);
}
/**
* 获取当前根域名
* @access public
* @return string
*/
public function rootDomain(): string
{
$root = $this->rootDomain;
if (!$root) {
$item = explode('.', $this->host());
$count = count($item);
$root = $count > 1 ? $item[$count - 2] . '.' . $item[$count - 1] : $item[0];
}
return $root;
}
/**
* 设置当前泛域名的值
* @access public
* @param string $domain 域名
* @
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
这是一款基于ThinkPHP6.0框架的微信公众号多域名回调系统。微信公众号后台默认只能授权2个网页域名,用本系统可突破这个限制,用同一个公众号对接无限多个网站。网站后台支持回调域名白名单的管理,以及登录记录的查看。 本系统还有微信access_token的获取功能,可让当前站点作为中控服务器统一获取和刷新access_token,其他业务逻辑站点所使用的access_token均调用当前站点获取,这样可避免各自刷新造成冲突,导致access_token覆盖而影响业务。
资源推荐
资源详情
资源评论
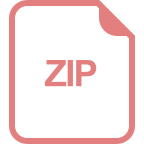
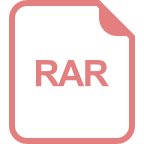
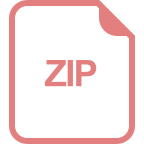
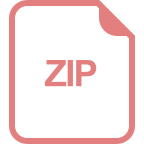
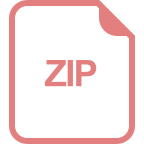
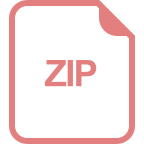
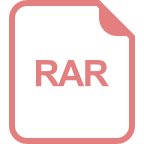
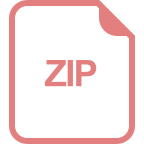
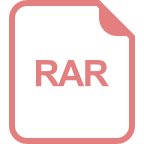
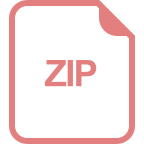
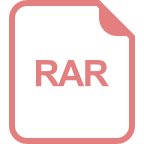
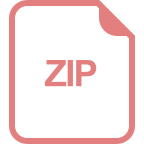
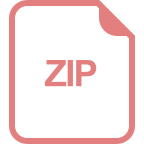
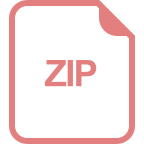
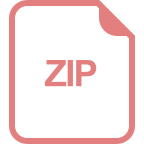
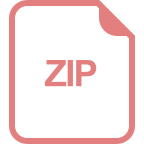
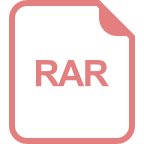
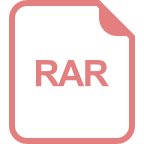
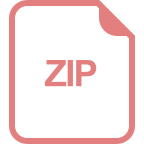
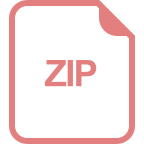
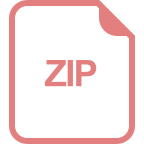
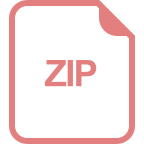
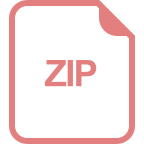
收起资源包目录

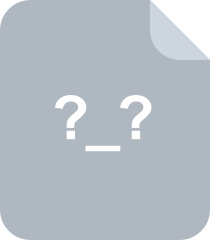
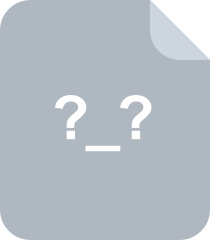
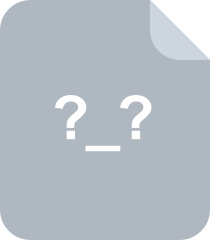
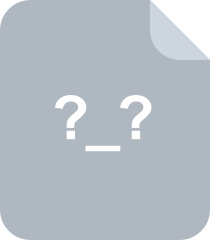
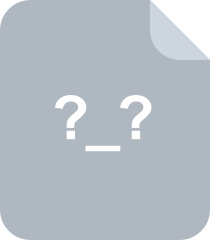
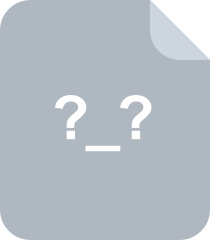
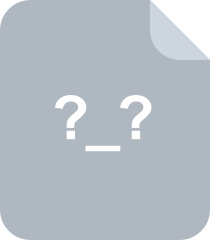
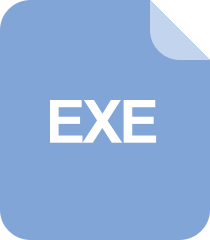
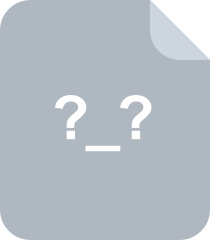
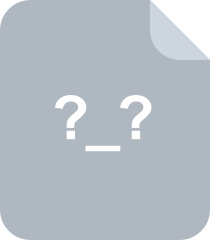
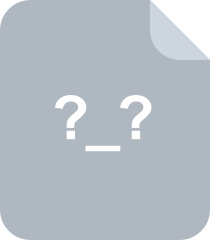
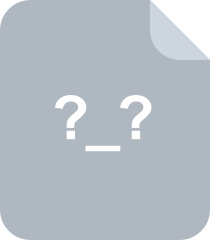
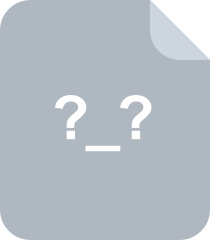
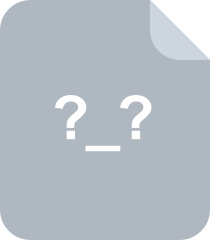
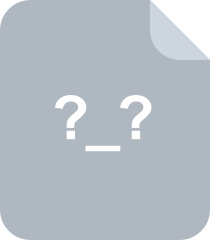
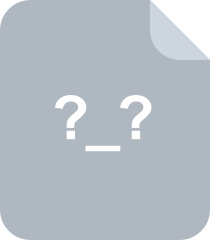
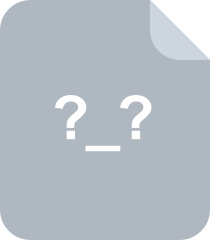
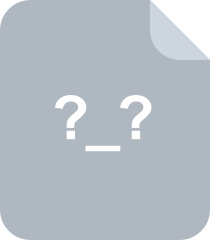
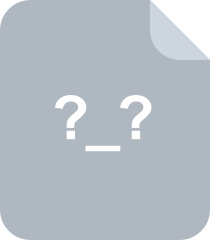
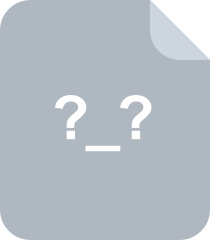
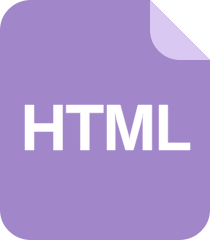
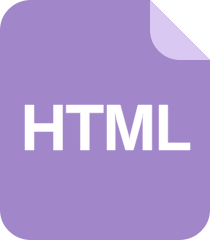
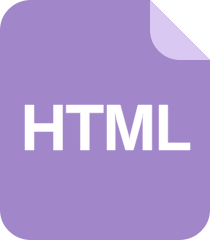
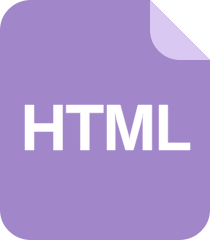
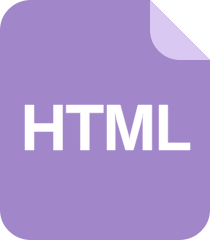
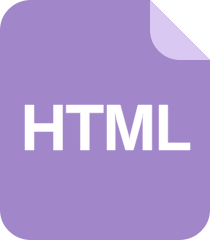
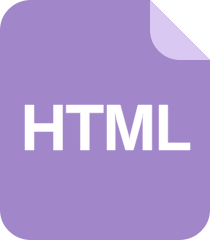
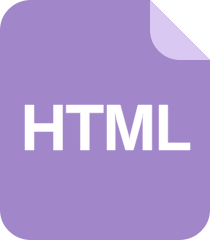
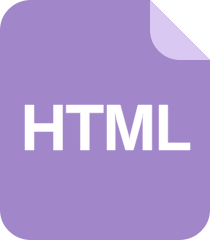
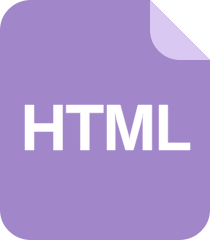
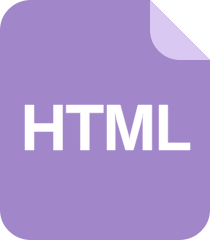
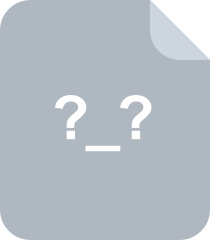
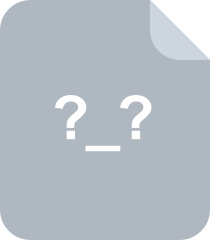
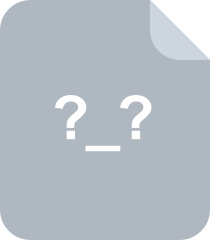
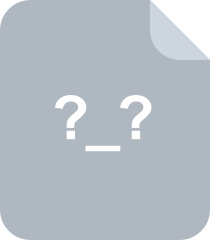
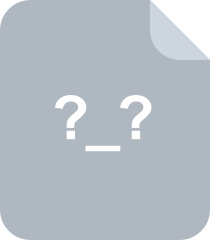
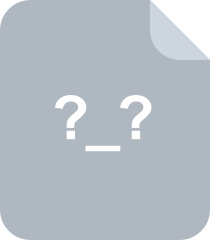
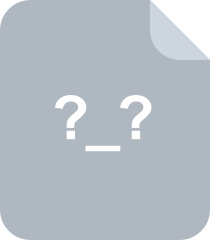
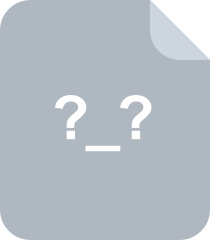
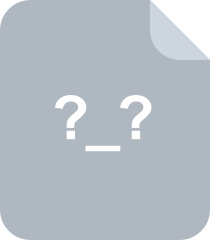
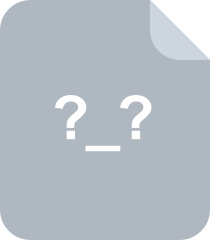
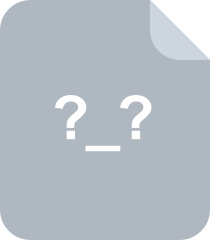
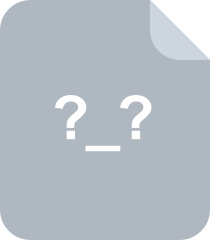
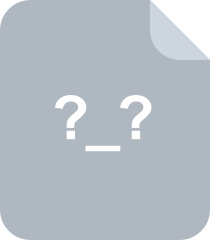
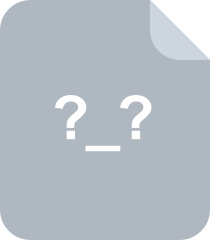
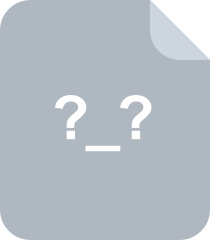
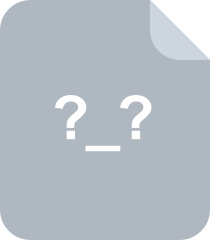
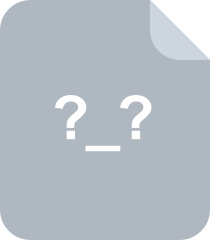
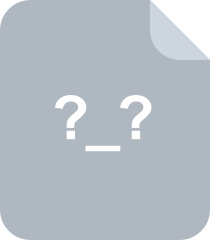
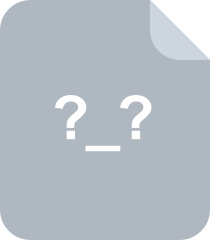
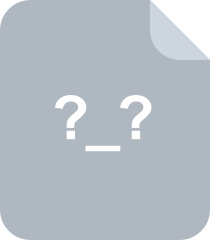
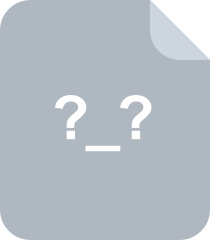
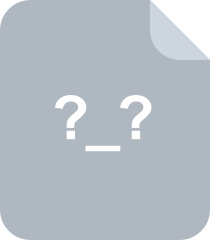
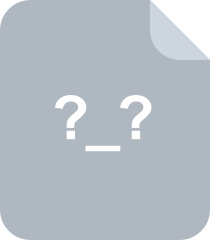
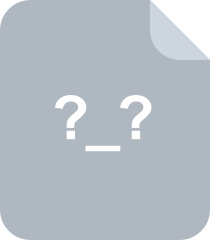
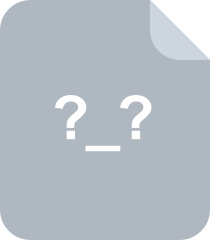
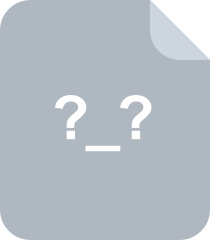
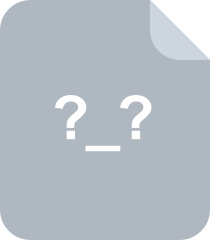
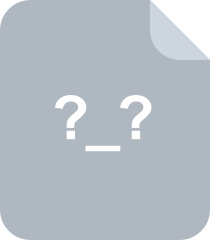
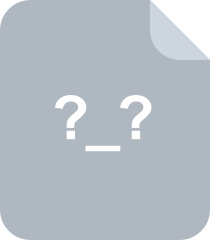
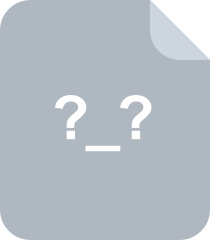
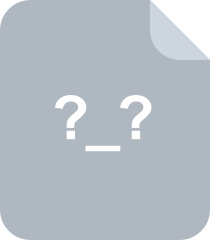
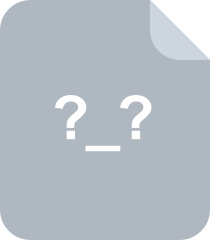
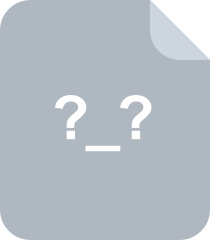
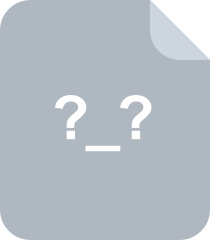
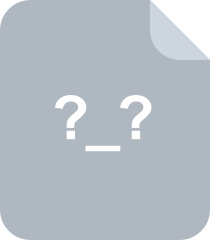
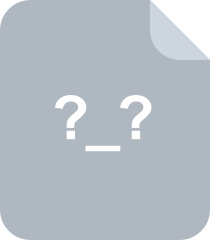
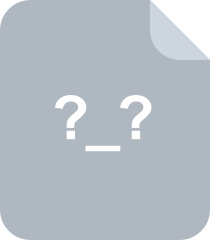
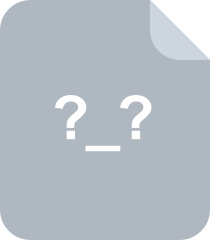
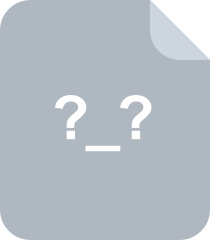
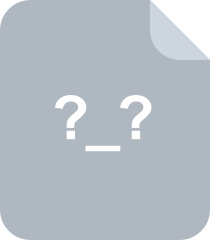
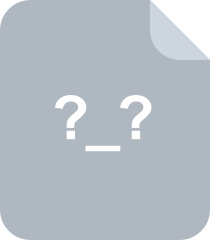
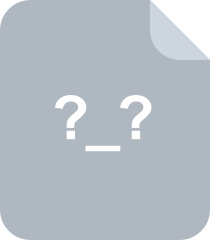
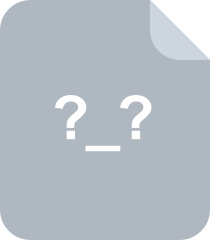
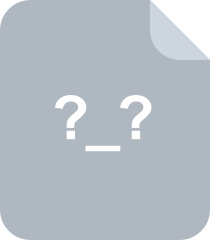
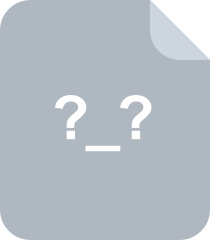
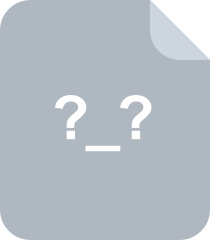
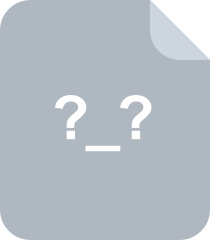
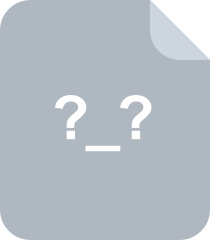
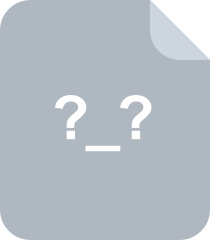
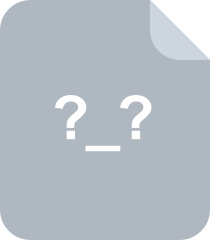
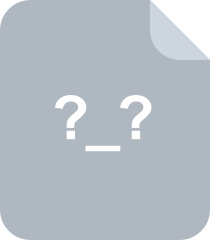
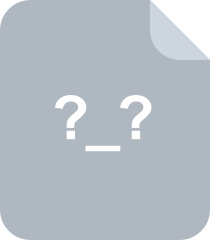
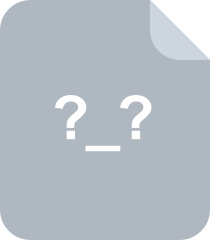
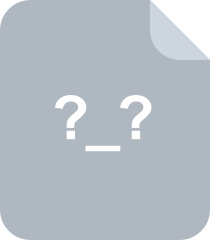
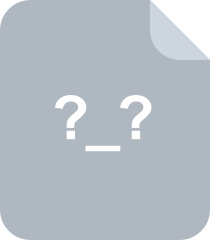
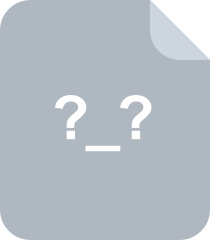
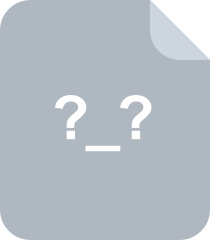
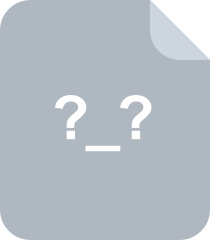
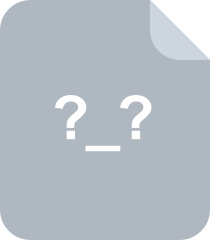
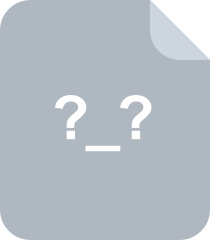
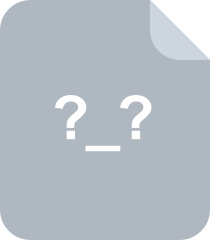
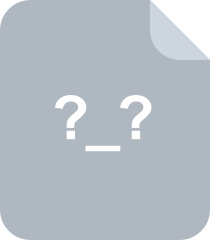
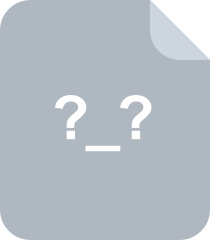
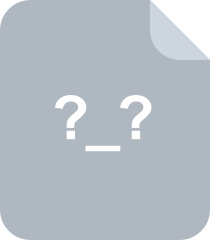
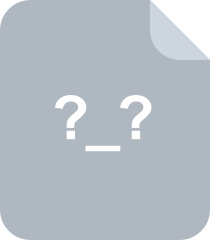
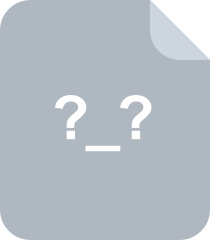
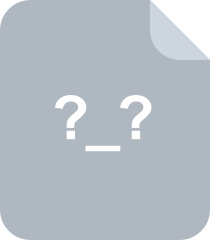
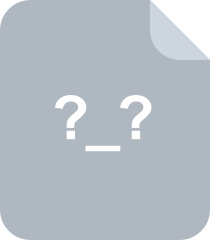
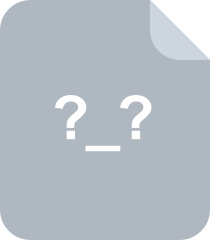
共 532 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
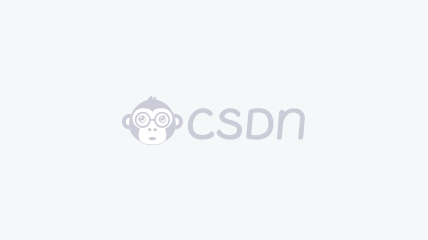

破碎的天堂鸟
- 粉丝: 9230
- 资源: 2586

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

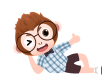
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


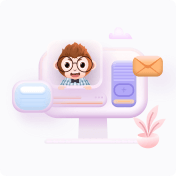
安全验证
文档复制为VIP权益,开通VIP直接复制
