package com.controller;
import java.io.File;
import java.math.BigDecimal;
import java.net.URL;
import java.text.SimpleDateFormat;
import com.alibaba.fastjson.JSONObject;
import java.util.*;
import org.springframework.beans.BeanUtils;
import javax.servlet.http.HttpServletRequest;
import org.springframework.web.context.ContextLoader;
import javax.servlet.ServletContext;
import com.service.TokenService;
import com.utils.*;
import java.lang.reflect.InvocationTargetException;
import com.service.DictionaryService;
import org.apache.commons.lang3.StringUtils;
import com.annotation.IgnoreAuth;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.entity.*;
import com.entity.view.*;
import com.service.*;
import com.utils.PageUtils;
import com.utils.R;
import com.alibaba.fastjson.*;
/**
* 试卷选题
* 后端接口
* @author
* @email
*/
@RestController
@Controller
@RequestMapping("/exampapertopic")
public class ExampapertopicController {
private static final Logger logger = LoggerFactory.getLogger(ExampapertopicController.class);
private static final String TABLE_NAME = "exampapertopic";
@Autowired
private ExampapertopicService exampapertopicService;
@Autowired
private TokenService tokenService;
@Autowired
private BaoxiuService baoxiuService;//报修
@Autowired
private BianminService bianminService;//便民服务
@Autowired
private ChatService chatService;//投诉管理
@Autowired
private CheweiService cheweiService;//车位
@Autowired
private CheweiOrderService cheweiOrderService;//车位订单
@Autowired
private DictionaryService dictionaryService;//字典
@Autowired
private ExampaperService exampaperService;//试卷
@Autowired
private ExamquestionService examquestionService;//试题表
@Autowired
private ExamrecordService examrecordService;//考试记录表
@Autowired
private ExamredetailsService examredetailsService;//答题详情表
@Autowired
private ExamrewrongquestionService examrewrongquestionService;//错题表
@Autowired
private FangwuService fangwuService;//房屋
@Autowired
private GonggaoService gonggaoService;//公告
@Autowired
private JiaofeiService jiaofeiService;//缴费
@Autowired
private WeixuiService weixuiService;//维修指派
@Autowired
private YonghuService yonghuService;//用户
@Autowired
private YuangongService yuangongService;//员工
@Autowired
private UsersService usersService;//管理员
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params, HttpServletRequest request){
logger.debug("page方法:,,Controller:{},,params:{}",this.getClass().getName(),JSONObject.toJSONString(params));
String role = String.valueOf(request.getSession().getAttribute("role"));
if(false)
return R.error(511,"永不会进入");
else if("用户".equals(role))
params.put("yonghuId",request.getSession().getAttribute("userId"));
else if("员工".equals(role))
params.put("yuangongId",request.getSession().getAttribute("userId"));
CommonUtil.checkMap(params);
PageUtils page = exampapertopicService.queryPage(params);
//字典表数据转换
List<ExampapertopicView> list =(List<ExampapertopicView>)page.getList();
for(ExampapertopicView c:list){
//修改对应字典表字段
dictionaryService.dictionaryConvert(c, request);
}
return R.ok().put("data", page);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id, HttpServletRequest request){
logger.debug("info方法:,,Controller:{},,id:{}",this.getClass().getName(),id);
ExampapertopicEntity exampapertopic = exampapertopicService.selectById(id);
if(exampapertopic !=null){
//entity转view
ExampapertopicView view = new ExampapertopicView();
BeanUtils.copyProperties( exampapertopic , view );//把实体数据重构到view中
//级联表 试卷
//级联表
ExampaperEntity exampaper = exampaperService.selectById(exampapertopic.getExampaperId());
if(exampaper != null){
BeanUtils.copyProperties( exampaper , view ,new String[]{ "id", "createTime", "insertTime", "updateTime", "username", "password", "newMoney"});//把级联的数据添加到view中,并排除id和创建时间字段,当前表的级联注册表
view.setExampaperId(exampaper.getId());
}
//级联表 试题表
//级联表
ExamquestionEntity examquestion = examquestionService.selectById(exampapertopic.getExamquestionId());
if(examquestion != null){
BeanUtils.copyProperties( examquestion , view ,new String[]{ "id", "createTime", "insertTime", "updateTime", "username", "password", "newMoney"});//把级联的数据添加到view中,并排除id和创建时间字段,当前表的级联注册表
view.setExamquestionId(examquestion.getId());
}
//修改对应字典表字段
dictionaryService.dictionaryConvert(view, request);
return R.ok().put("data", view);
}else {
return R.error(511,"查不到数据");
}
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody ExampapertopicEntity exampapertopic, HttpServletRequest request){
logger.debug("save方法:,,Controller:{},,exampapertopic:{}",this.getClass().getName(),exampapertopic.toString());
String role = String.valueOf(request.getSession().getAttribute("role"));
if(false)
return R.error(511,"永远不会进入");
Wrapper<ExampapertopicEntity> queryWrapper = new EntityWrapper<ExampapertopicEntity>()
.eq("exampaper_id", exampapertopic.getExampaperId())
.eq("examquestion_id", exampapertopic.getExamquestionId())
.eq("exampapertopic_number", exampapertopic.getExampapertopicNumber())
;
logger.info("sql语句:"+queryWrapper.getSqlSegment());
ExampapertopicEntity exampapertopicEntity = exampapertopicService.selectOne(queryWrapper);
if(exampapertopicEntity==null){
exampapertopic.setCreateTime(new Date());
exampapertopicService.insert(exampapertopic);
return R.ok();
}else {
return R.error(511,"表中有相同数据");
}
}
/**
* 后端修改
*/
@RequestMapping("/update")
public R update(@RequestBody ExampapertopicEntity exampapertopic, HttpServletRequest request) throws NoSuchFieldException, ClassNotFoundException, IllegalAccessException, InstantiationException {
logger.debug("update方法:,,Controller:{},,exampapertopic:{}",this.getClass().getName(),exampapertopic.toString());
ExampapertopicEntity oldExampapertopicEntity = exampapertopicService.selectById(exampapertopic.getId());//查询原先数据
String role = String.valueOf(request.getSession().getAttribute("role"));
// if(false)
// return R.error(511,"永远不会进入");
exampapertopicService.updateById(exampapertopic);//根据id更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@Requ
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
SSM项目小程序“智慧物业平台修改5.5.zip”是一个基于Spring、Spring MVC和MyBatis(SSM)框架开发的微信小程序,专为物业管理公司及其服务的社区居民设计。该项目利用微信小程序的普及性和便利性结合SSM框架的技术实力,提供一个智能化、综合性的物业服务平台。 以下是该智慧物业平台小程序的核心功能和特点: 1. **物业费用管理**:允许用户在线查询和管理物业费用缴纳情况,包括水电煤等公共事业账单。 2. **报修服务**:居民可以通过小程序直接提交家庭维修需求,并实时跟踪处理进度。 3. **访客管理**:集成访客登记系统,提高社区安全性,同时方便居民管理来访者信息。 4. **社区通知**:物业管理者可以通过小程序发布最新的社区通知和公告,确保信息及时传达。 5. **环境监控**:提供社区环境监控数据,如公共区域的空气质量、噪音水平等,提升居住体验。 6. **投诉建议**:居民可以通过小程序提交对物业服务的投诉或建议,增强物业管理的透明度和互动性。 7. **生活服务**:集成多种生活服务功能,如预约洗衣、保洁、团购等,为居民提供便利。 8. **智能门禁**:与智能门禁系统对接,居民可通过小程序远程控制小区门禁,提高出入便利性。 整个系统以微信小程序为前端,便于用户随时随地访问和使用;后端采用SSM框架,确保了数据处理的效率和稳定性。它不仅提供了一个方便的物业管理和社区服务平台,还通过综合化的服务和智能化的管理,提升了社区管理的现代化水平和居民的生活质量,是现代城市社区管理的重要工具。
资源推荐
资源详情
资源评论
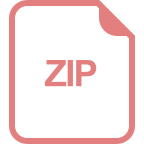
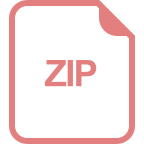
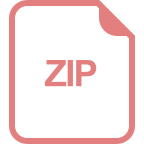
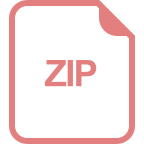
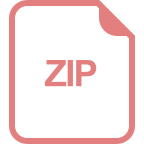
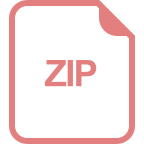
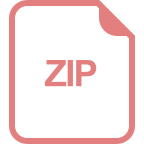
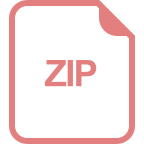
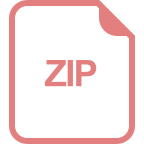
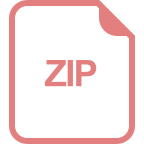
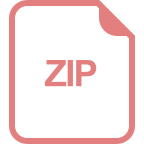
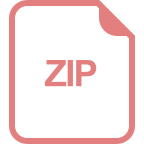
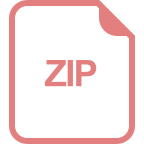
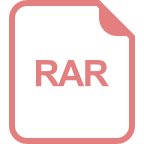
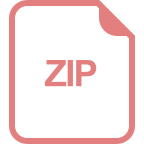
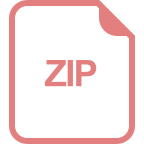
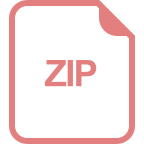
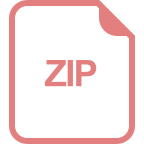
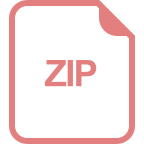
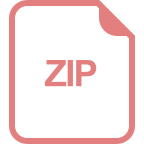
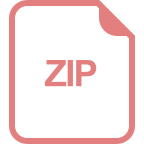
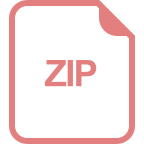
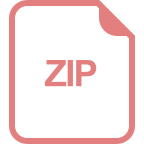
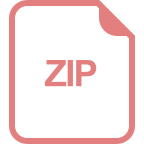
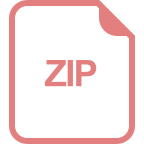
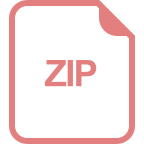
收起资源包目录

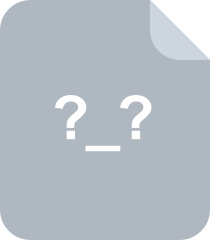
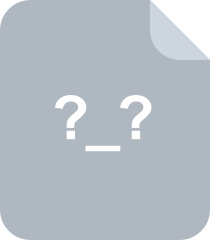
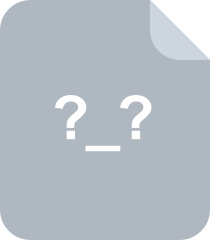
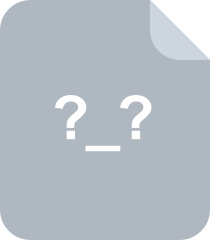
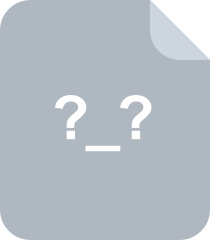
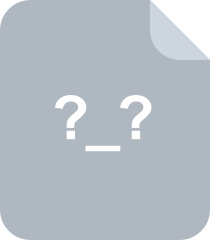
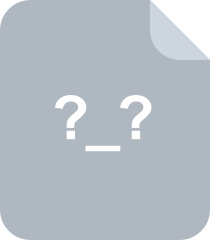
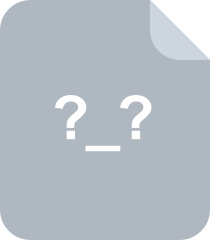
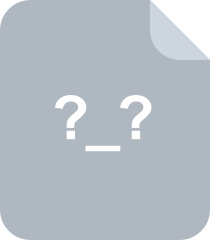
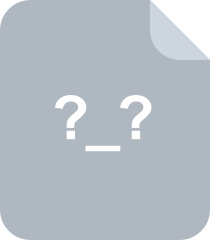
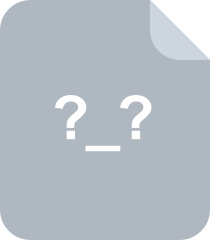
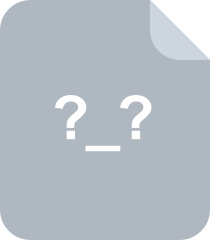
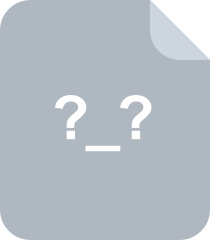
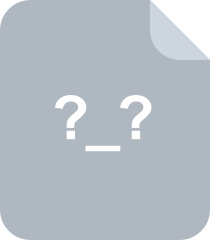
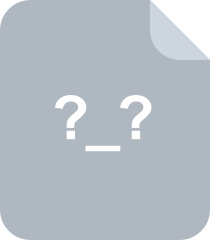
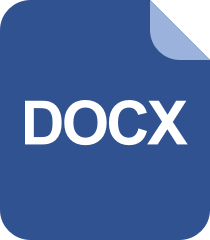
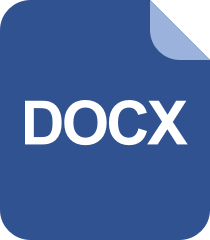
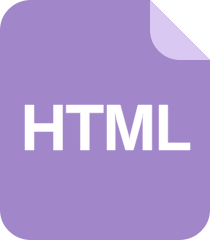
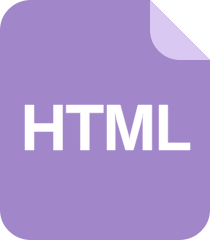
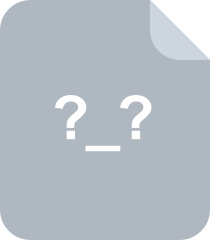
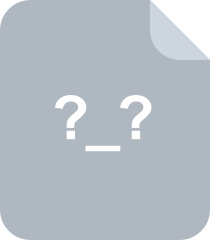
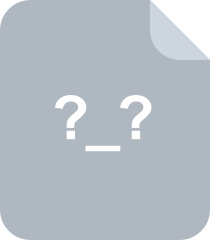
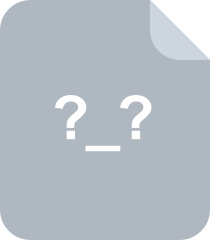
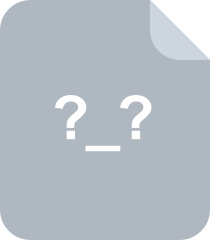
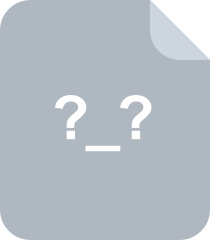
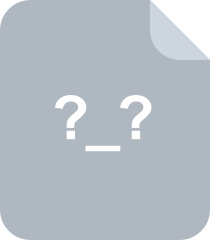
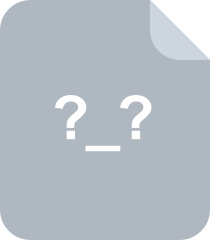
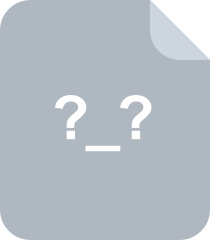
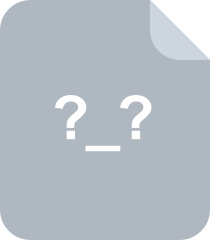
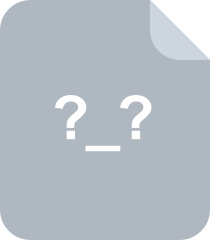
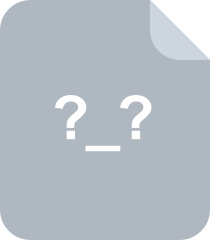
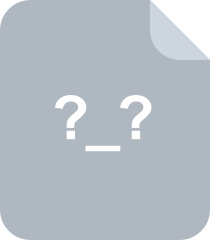
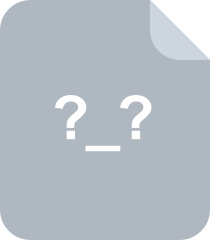
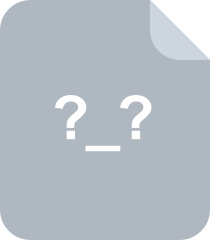
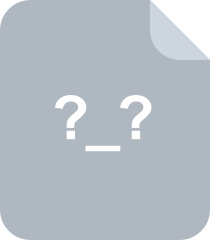
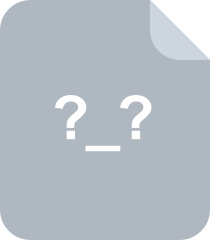
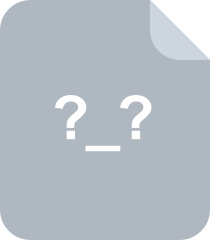
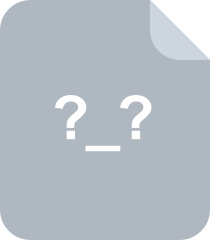
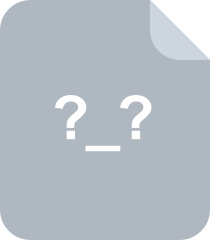
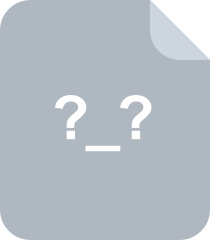
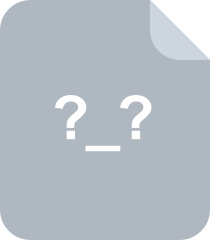
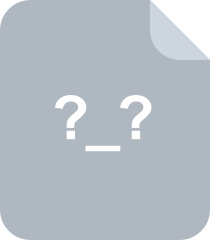
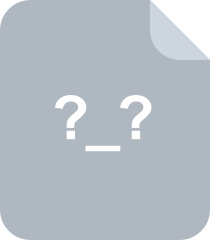
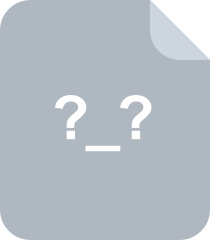
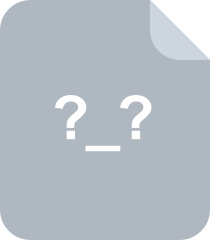
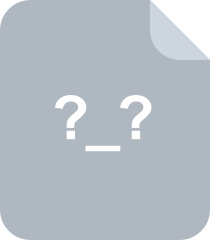
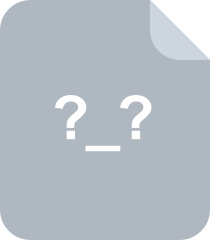
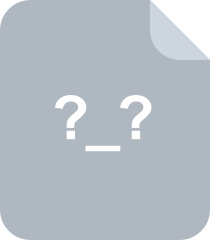
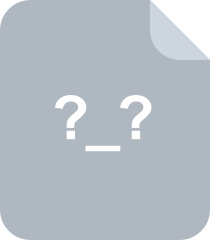
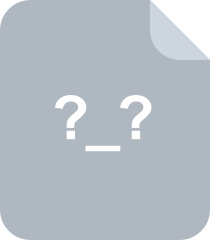
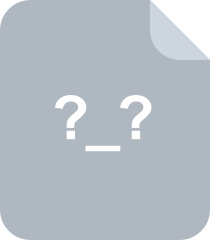
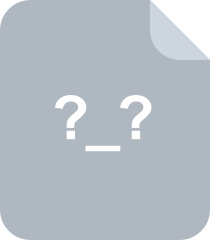
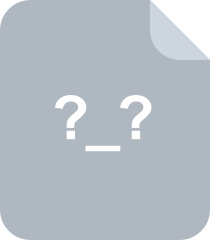
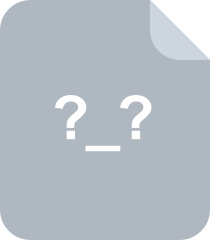
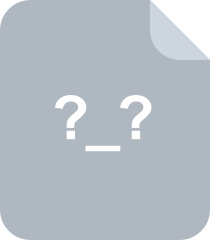
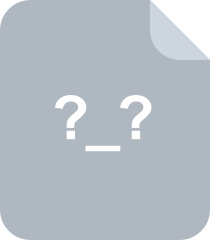
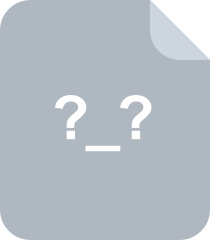
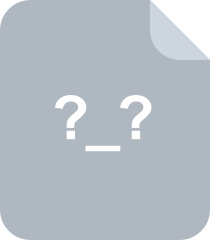
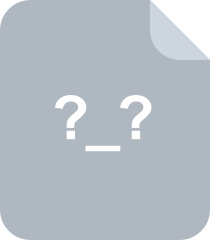
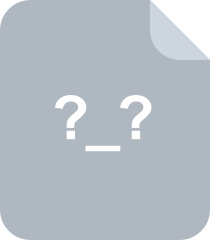
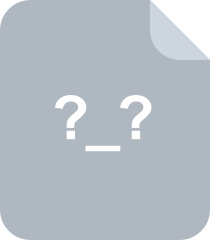
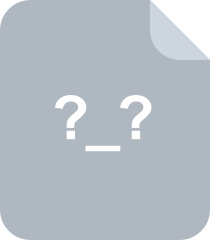
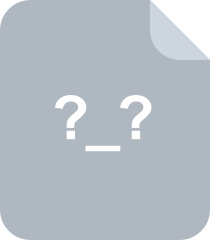
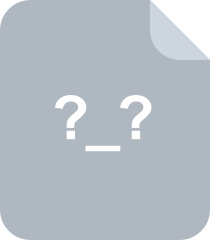
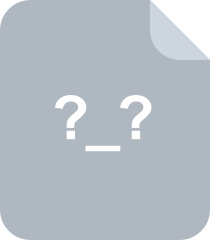
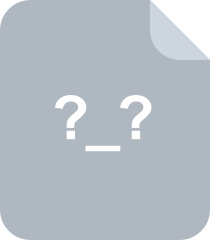
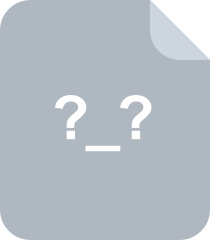
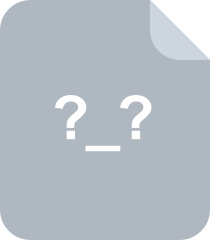
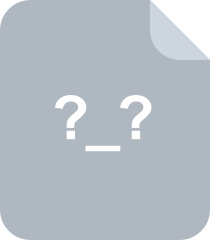
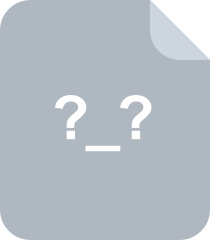
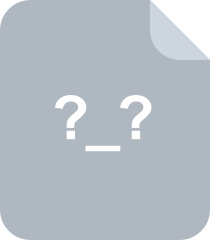
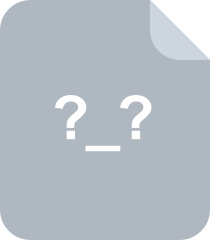
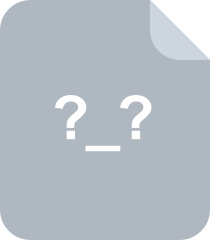
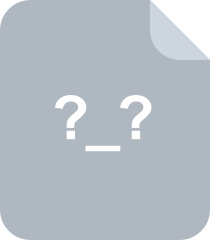
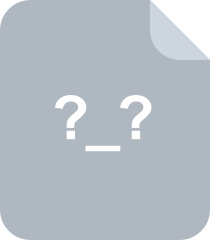
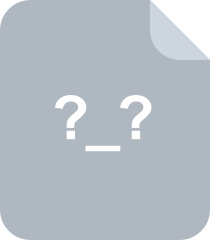
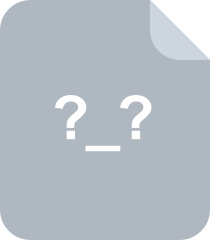
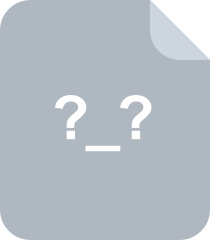
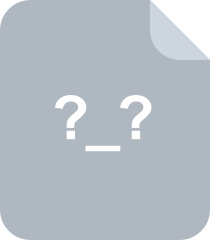
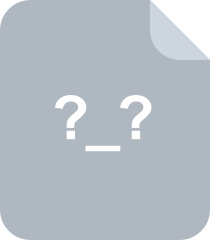
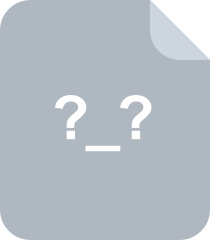
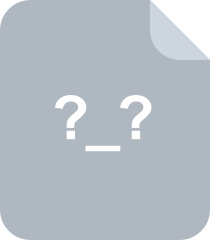
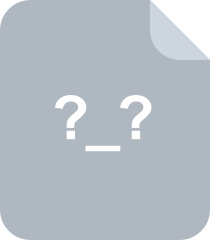
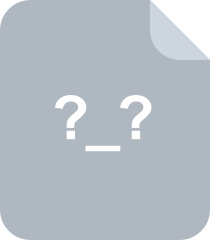
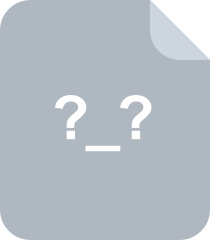
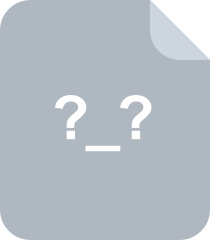
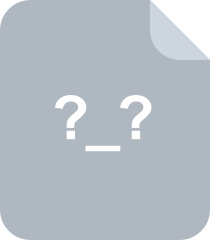
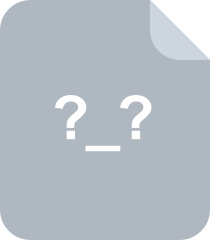
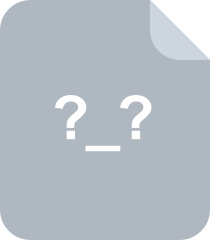
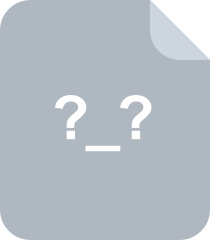
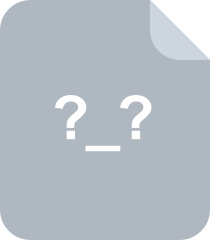
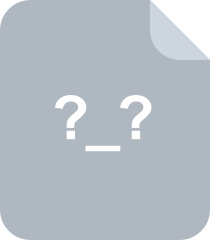
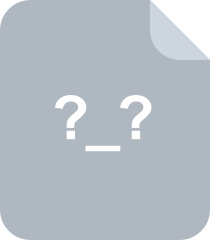
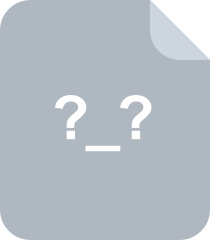
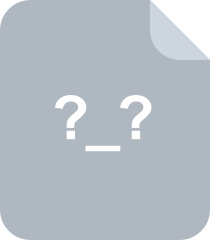
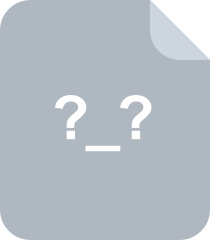
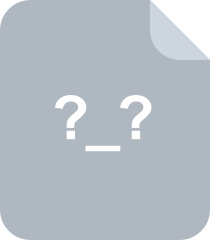
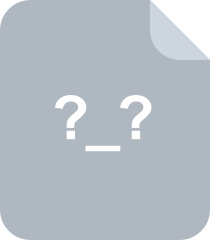
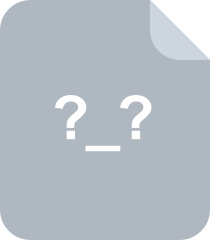
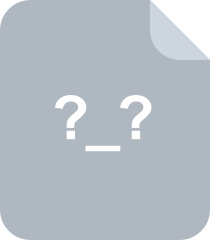
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
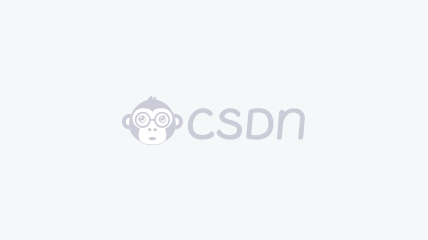

程序媛小刘
- 粉丝: 2849
- 资源: 1322
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

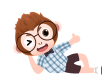
最新资源
- 基于Java语言实现养老院信息管理系统(SQL Server数据库)
- 社区居民诊疗健康-JAVA-基于SpringBoot的社区居民诊疗健康管理系统设计与实现(毕业论文)
- ChromeSetup.zip
- 大黄蜂V14旋翼机3D
- 体育购物商城-JAVA-基于springboot的体育购物商城设计与实现(毕业论文)
- 三保一评关系与区别分析
- 星形发动机3D 星形发动机
- 机考样例(学生).zip
- Day-05 Vue22222222222
- 经过数据增强后番茄叶片病害识别,约45000张数据,已标注
- 商用密码技术及产品介绍
- CC2530无线zigbee裸机代码实现WIFI ESP8266上传数据到服务器.zip
- 文物管理系统-JAVA-基于springboot的文物管理系统的设计与实现(毕业论文)
- 店铺数据采集系统项目全套技术资料.zip
- 数据安全基础介绍;数据安全概念
- 目标检测数据集: 果树上的tomato西红柿图像检测数据【VOC标注格式、包含数据和标签】
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


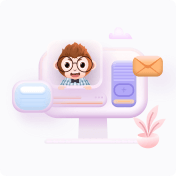
安全验证
文档复制为VIP权益,开通VIP直接复制
