/* Implement classes and message passing for Objective C.
Copyright (C) 1992, 1993, 1994, 1995, 1997, 1998, 1999, 2000, 2001,
2002, 2003, 2004, 2005, 2007, 2008, 2009, 2010, 2011
Free Software Foundation, Inc.
Contributed by Steve Naroff.
This file is part of GCC.
GCC is free software; you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; either version 3, or (at your option)
any later version.
GCC is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with GCC; see the file COPYING3. If not see
<http://www.gnu.org/licenses/>. */
#include "config.h"
#include "system.h"
#include "coretypes.h"
#include "tm.h"
#include "tree.h"
#ifdef OBJCPLUS
#include "cp-tree.h"
#else
#include "c-tree.h"
#include "c-lang.h"
#endif
#include "c-family/c-common.h"
#include "c-family/c-objc.h"
#include "c-family/c-pragma.h"
#include "c-family/c-format.h"
#include "flags.h"
#include "langhooks.h"
#include "objc-act.h"
#include "input.h"
#include "function.h"
#include "output.h"
#include "toplev.h"
#include "ggc.h"
#include "debug.h"
#include "target.h"
#include "diagnostic-core.h"
#include "intl.h"
#include "cgraph.h"
#include "tree-iterator.h"
#include "hashtab.h"
#include "langhooks-def.h"
/* Different initialization, code gen and meta data generation for each
runtime. */
#include "objc-runtime-hooks.h"
/* Routines used mainly by the runtimes. */
#include "objc-runtime-shared-support.h"
/* For default_tree_printer (). */
#include "tree-pretty-print.h"
/* For enum gimplify_status */
#include "gimple.h"
static unsigned int should_call_super_dealloc = 0;
/* When building Objective-C++, we are not linking against the C front-end
and so need to replicate the C tree-construction functions in some way. */
#ifdef OBJCPLUS
#define OBJCP_REMAP_FUNCTIONS
#include "objcp-decl.h"
#endif /* OBJCPLUS */
/* This is the default way of generating a method name. */
/* This has the problem that "test_method:argument:" and
"test:method_argument:" will generate the same name
("_i_Test__test_method_argument_" for an instance method of the
class "Test"), so you can't have them both in the same class!
Moreover, the demangling (going from
"_i_Test__test_method_argument" back to the original name) is
undefined because there are two correct ways of demangling the
name. */
#ifndef OBJC_GEN_METHOD_LABEL
#define OBJC_GEN_METHOD_LABEL(BUF, IS_INST, CLASS_NAME, CAT_NAME, SEL_NAME, NUM) \
do { \
char *temp; \
sprintf ((BUF), "_%s_%s_%s_%s", \
((IS_INST) ? "i" : "c"), \
(CLASS_NAME), \
((CAT_NAME)? (CAT_NAME) : ""), \
(SEL_NAME)); \
for (temp = (BUF); *temp; temp++) \
if (*temp == ':') *temp = '_'; \
} while (0)
#endif
/* These need specifying. */
#ifndef OBJC_FORWARDING_STACK_OFFSET
#define OBJC_FORWARDING_STACK_OFFSET 0
#endif
#ifndef OBJC_FORWARDING_MIN_OFFSET
#define OBJC_FORWARDING_MIN_OFFSET 0
#endif
/* Set up for use of obstacks. */
#include "obstack.h"
/* This obstack is used to accumulate the encoding of a data type. */
struct obstack util_obstack;
/* This points to the beginning of obstack contents, so we can free
the whole contents. */
char *util_firstobj;
/*** Private Interface (procedures) ***/
/* Init stuff. */
static void synth_module_prologue (void);
/* Code generation. */
static tree start_class (enum tree_code, tree, tree, tree, tree);
static tree continue_class (tree);
static void finish_class (tree);
static void start_method_def (tree);
static tree start_protocol (enum tree_code, tree, tree, tree);
static tree build_method_decl (enum tree_code, tree, tree, tree, bool);
static tree objc_add_method (tree, tree, int, bool);
static tree add_instance_variable (tree, objc_ivar_visibility_kind, tree);
static tree build_ivar_reference (tree);
static tree is_ivar (tree, tree);
/* We only need the following for ObjC; ObjC++ will use C++'s definition
of DERIVED_FROM_P. */
#ifndef OBJCPLUS
static bool objc_derived_from_p (tree, tree);
#define DERIVED_FROM_P(PARENT, CHILD) objc_derived_from_p (PARENT, CHILD)
#endif
/* Property. */
static void objc_gen_property_data (tree, tree);
static void objc_synthesize_getter (tree, tree, tree);
static void objc_synthesize_setter (tree, tree, tree);
static char *objc_build_property_setter_name (tree);
static int match_proto_with_proto (tree, tree, int);
static tree lookup_property (tree, tree);
static tree lookup_property_in_list (tree, tree);
static tree lookup_property_in_protocol_list (tree, tree);
static void build_common_objc_property_accessor_helpers (void);
static void objc_xref_basetypes (tree, tree);
static tree get_class_ivars (tree, bool);
static void build_fast_enumeration_state_template (void);
#ifdef OBJCPLUS
static void objc_generate_cxx_cdtors (void);
#endif
/* objc attribute */
static void objc_decl_method_attributes (tree*, tree, int);
static tree build_keyword_selector (tree);
/* Hash tables to manage the global pool of method prototypes. */
static void hash_init (void);
hash *nst_method_hash_list = 0;
hash *cls_method_hash_list = 0;
/* Hash tables to manage the global pool of class names. */
hash *cls_name_hash_list = 0;
hash *als_name_hash_list = 0;
hash *ivar_offset_hash_list = 0;
static void hash_class_name_enter (hash *, tree, tree);
static hash hash_class_name_lookup (hash *, tree);
static hash hash_lookup (hash *, tree);
static tree lookup_method (tree, tree);
static tree lookup_method_static (tree, tree, int);
static tree add_class (tree, tree);
static void add_category (tree, tree);
static inline tree lookup_category (tree, tree);
/* Protocols. */
static tree lookup_protocol (tree, bool, bool);
static tree lookup_and_install_protocols (tree, bool);
/* Type encoding. */
static void encode_type_qualifiers (tree);
static void encode_type (tree, int, int);
#ifdef OBJCPLUS
static void really_start_method (tree, tree);
#else
static void really_start_method (tree, struct c_arg_info *);
#endif
static int comp_proto_with_proto (tree, tree, int);
static tree objc_decay_parm_type (tree);
/* Utilities for debugging and error diagnostics. */
static char *gen_type_name (tree);
static char *gen_type_name_0 (tree);
static char *gen_method_decl (tree);
static char *gen_declaration (tree);
/* Everything else. */
static void generate_struct_by_value_array (void) ATTRIBUTE_NORETURN;
static void mark_referenced_methods (void);
static bool objc_type_valid_for_messaging (tree type, bool allow_classes);
static tree check_duplicates (hash, int, int);
/*** Private Interface (data) ***/
/* Flags for lookup_method_static(). */
/* Look for class methods. */
#define OBJC_LOOKUP_CLASS 1
/* Do not examine superclasses. */
#define OBJC_LOOKUP_NO_SUPER 2
/* Disable returning an instance method of a root class when a class
method can't be found. */
#define OBJC_LOOKUP_NO_INSTANCE_METHODS_OF_ROOT_CLASS 4
/* The OCTI_... enumeration itself is in objc/objc-act.h. */
tree objc_global_trees[OCTI_MAX];
struct imp_entry *imp_list = 0;
int imp_count = 0; /* `@implementation' */
int cat_count = 0; /* `@category' */
objc_ivar_visibility_kind objc_ivar_visibility;
/* Use to generate method labels. */
static int method_slot = 0;
/* Flag to say whether methods in a protocol are optional or
required. */
static bool objc_method_optional_flag = false;
static int objc_collecting_ivars = 0;
/* Flag that is set to 'true' while we are processing a class
extension. Since a class extension just "reopens" the main
@interface, this can be used to determine if we are in the main
@interface, or in a class extension. */
static bool objc_in_class_
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
gcc编译器是一款由GNU打造的编程语言编译器软件,支持处理C语言、Fortran、Pascal、Objective-C、Java等多种语言,实用性强,能够帮助用户进行高效的编译工作。gcc编译器是一套以 GPL 及 LGPL许可证所发行的自由软件,适用于windows的各个操作系统。 【gcc编译器使用说明】 1、在gcc--4.9.1的平级目录建一个编译目录 2、配置 ../gcc-4.9.1/configure --prefix=/usr/local/gcc-4.9.1 --enable-threads=posix --enable-stage1-checking=release --enable-stage1-languages=c,c++ --disable-multilib 3、编译 make 4、安装 make install 5、修改环境变量 export PATH=/usr/local/gcc-4.9.1/bin/:$PATH 6、查看gcc版本 gcc -v
资源详情
资源评论
资源推荐
收起资源包目录

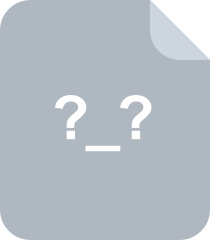
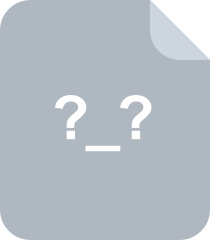
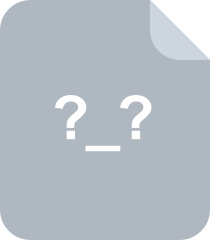
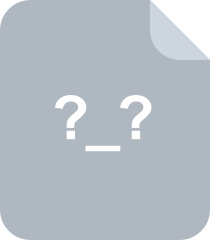
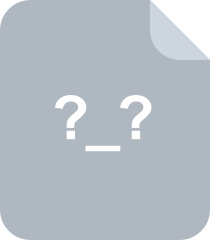
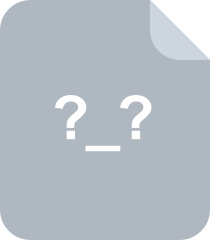
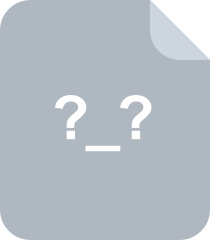
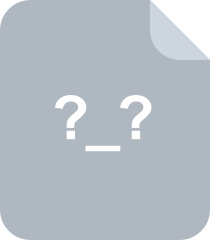
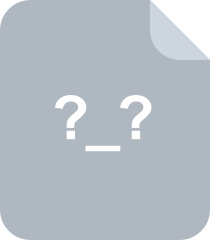
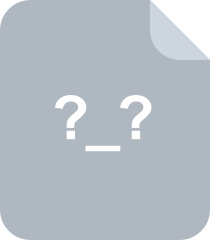
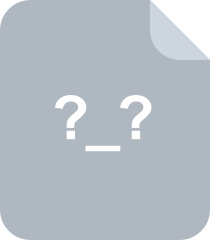
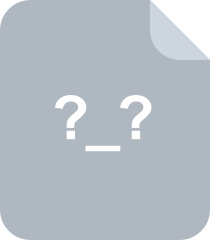
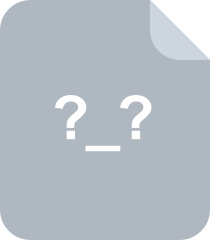
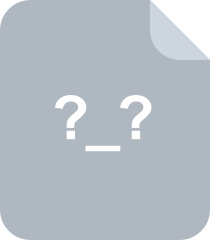
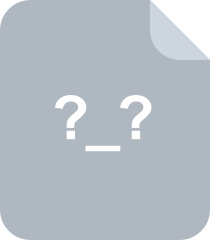
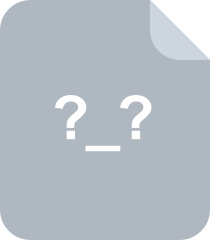
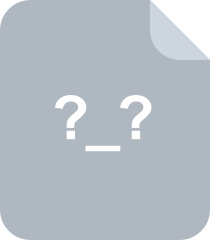
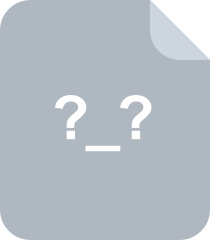
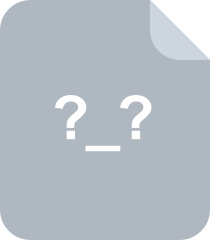
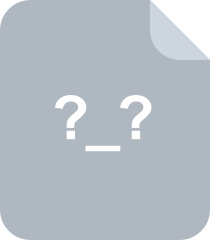
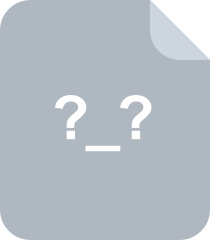
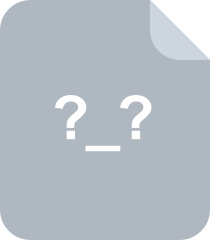
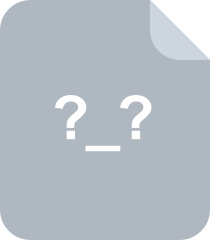
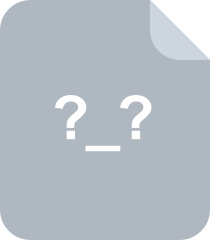
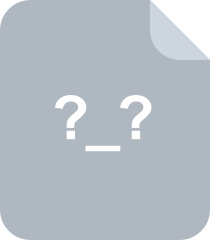
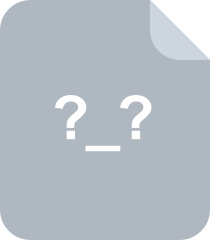
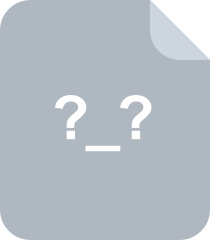
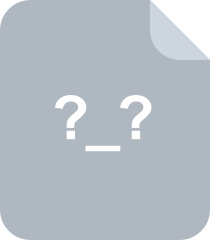
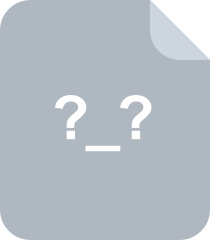
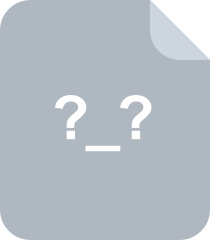
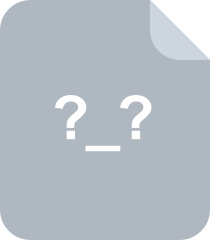
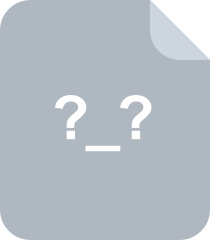
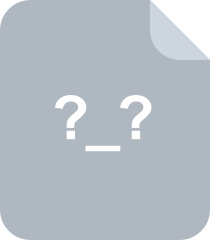
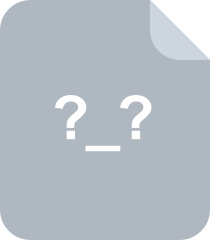
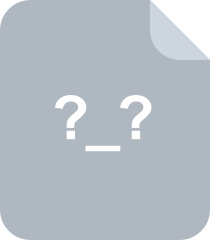
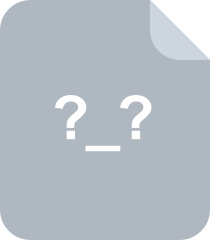
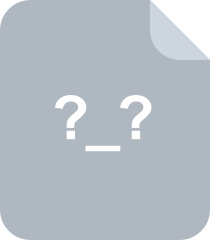
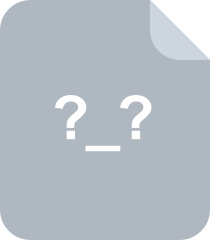
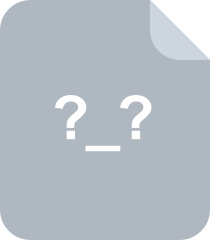
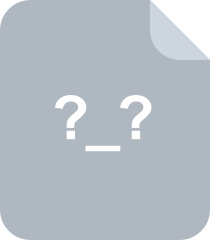
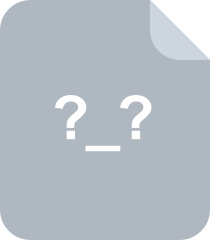
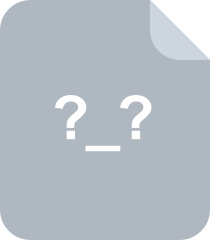
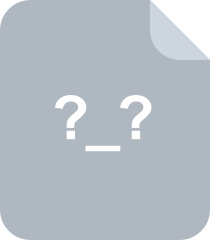
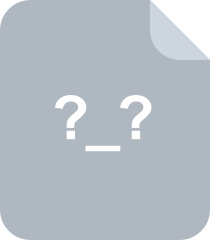
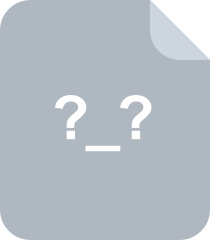
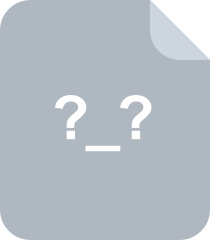
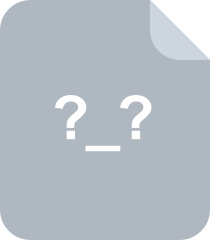
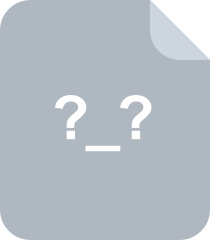
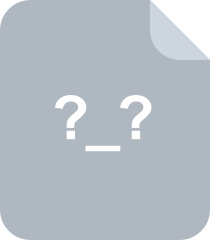
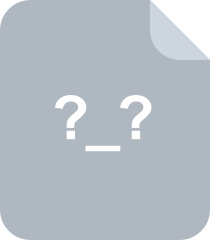
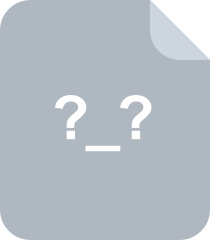
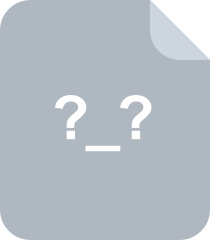
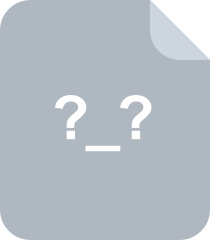
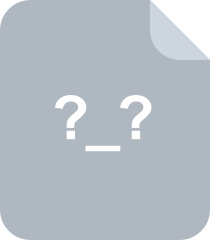
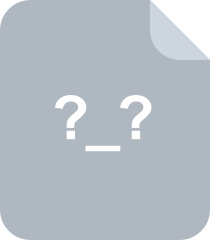
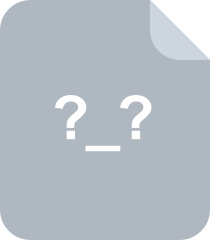
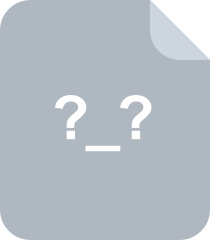
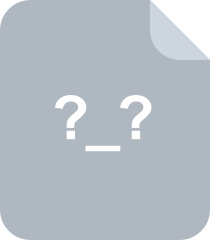
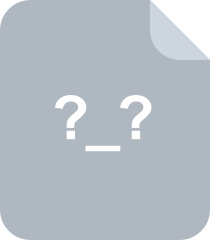
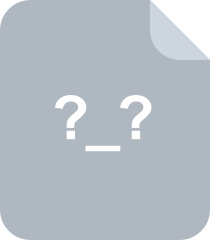
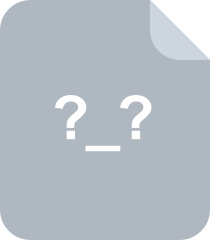
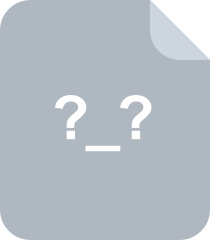
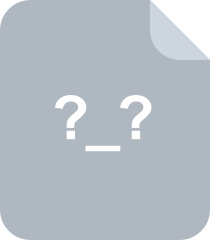
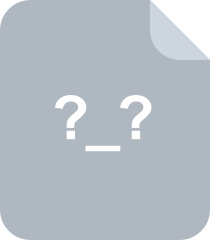
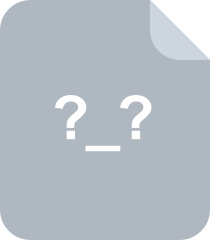
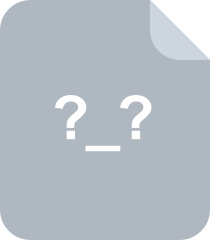
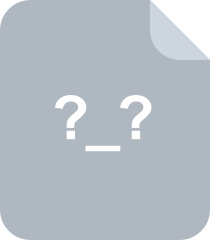
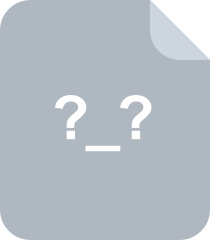
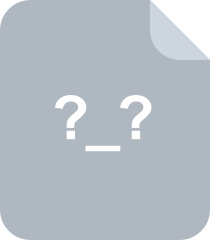
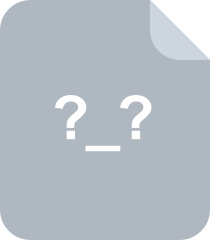
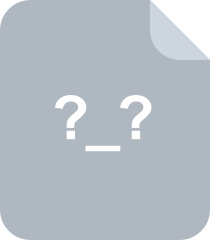
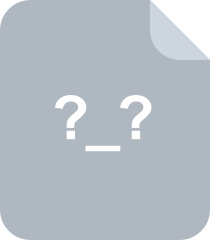
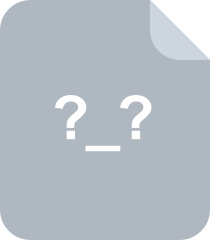
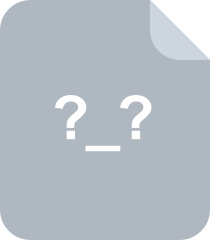
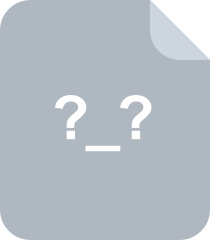
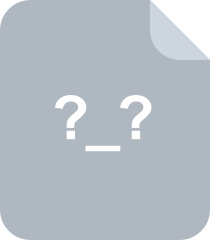
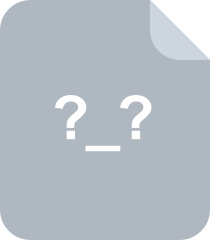
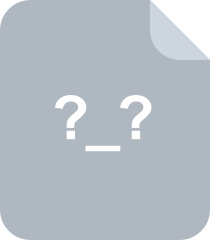
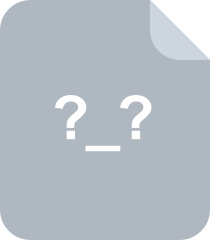
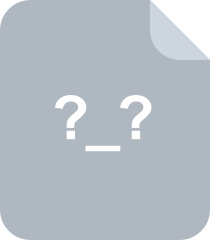
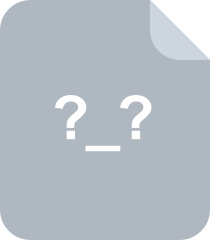
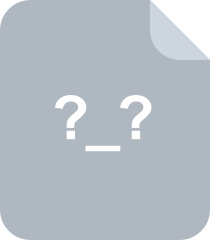
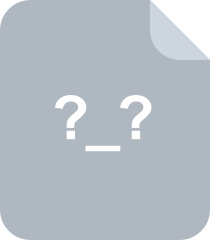
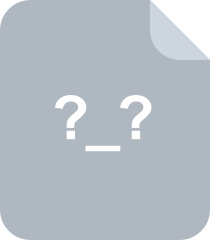
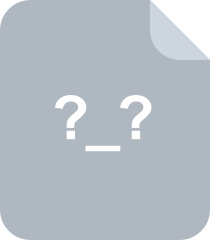
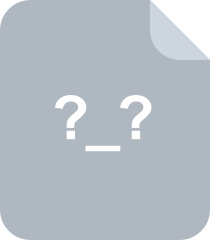
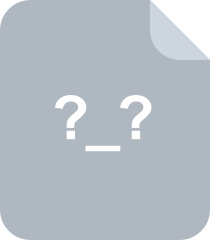
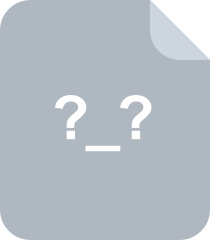
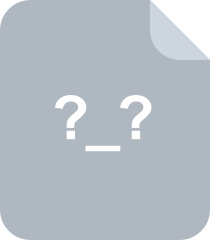
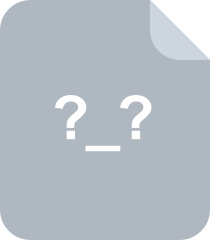
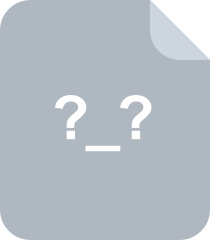
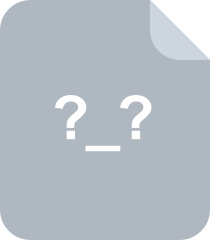
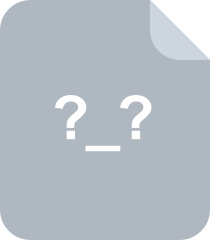
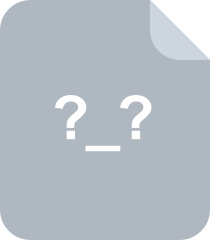
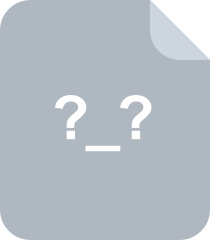
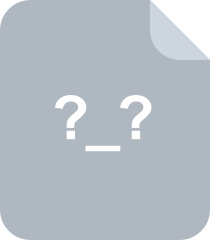
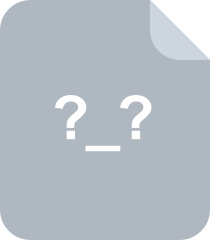
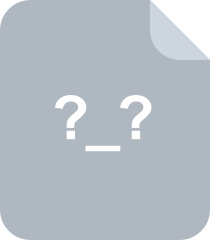
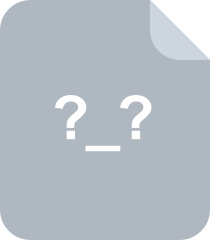
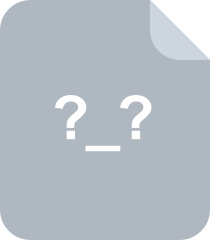
共 115 条
- 1
- 2
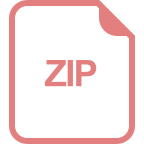
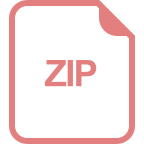
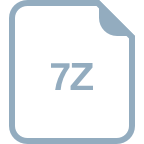
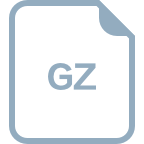
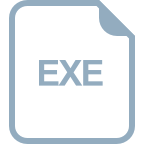
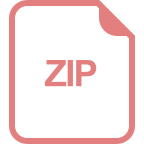
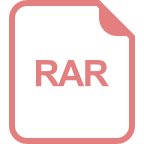
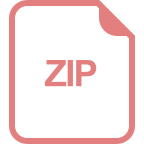
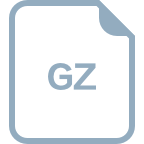
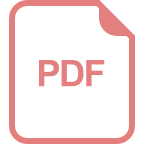
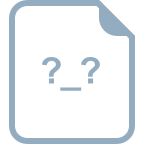
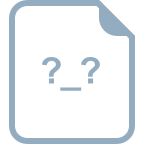
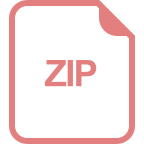
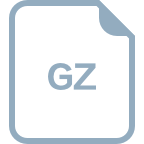
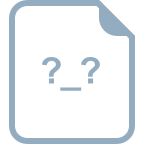
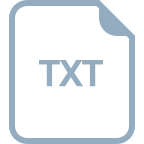
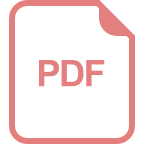
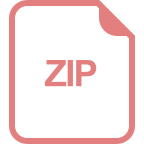

人来人往rlrw
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

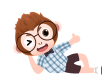
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


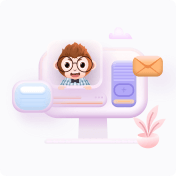
安全验证
文档复制为VIP权益,开通VIP直接复制

评论10