# [88. Merge Sorted Array (Easy)](https://leetcode.com/problems/merge-sorted-array/)
<p>You are given two integer arrays <code>nums1</code> and <code>nums2</code>, sorted in <strong>non-decreasing order</strong>, and two integers <code>m</code> and <code>n</code>, representing the number of elements in <code>nums1</code> and <code>nums2</code> respectively.</p>
<p><strong>Merge</strong> <code>nums1</code> and <code>nums2</code> into a single array sorted in <strong>non-decreasing order</strong>.</p>
<p>The final sorted array should not be returned by the function, but instead be <em>stored inside the array </em><code>nums1</code>. To accommodate this, <code>nums1</code> has a length of <code>m + n</code>, where the first <code>m</code> elements denote the elements that should be merged, and the last <code>n</code> elements are set to <code>0</code> and should be ignored. <code>nums2</code> has a length of <code>n</code>.</p>
<p> </p>
<p><strong>Example 1:</strong></p>
<pre><strong>Input:</strong> nums1 = [1,2,3,0,0,0], m = 3, nums2 = [2,5,6], n = 3
<strong>Output:</strong> [1,2,2,3,5,6]
<strong>Explanation:</strong> The arrays we are merging are [1,2,3] and [2,5,6].
The result of the merge is [<u>1</u>,<u>2</u>,2,<u>3</u>,5,6] with the underlined elements coming from nums1.
</pre>
<p><strong>Example 2:</strong></p>
<pre><strong>Input:</strong> nums1 = [1], m = 1, nums2 = [], n = 0
<strong>Output:</strong> [1]
<strong>Explanation:</strong> The arrays we are merging are [1] and [].
The result of the merge is [1].
</pre>
<p><strong>Example 3:</strong></p>
<pre><strong>Input:</strong> nums1 = [0], m = 0, nums2 = [1], n = 1
<strong>Output:</strong> [1]
<strong>Explanation:</strong> The arrays we are merging are [] and [1].
The result of the merge is [1].
Note that because m = 0, there are no elements in nums1. The 0 is only there to ensure the merge result can fit in nums1.
</pre>
<p> </p>
<p><strong>Constraints:</strong></p>
<ul>
<li><code>nums1.length == m + n</code></li>
<li><code>nums2.length == n</code></li>
<li><code>0 <= m, n <= 200</code></li>
<li><code>1 <= m + n <= 200</code></li>
<li><code>-10<sup>9</sup> <= nums1[i], nums2[j] <= 10<sup>9</sup></code></li>
</ul>
<p> </p>
<p><strong>Follow up: </strong>Can you come up with an algorithm that runs in <code>O(m + n)</code> time?</p>
**Related Topics**:
[Array](https://leetcode.com/tag/array/), [Two Pointers](https://leetcode.com/tag/two-pointers/), [Sorting](https://leetcode.com/tag/sorting/)
**Similar Questions**:
* [Merge Two Sorted Lists (Easy)](https://leetcode.com/problems/merge-two-sorted-lists/)
* [Squares of a Sorted Array (Easy)](https://leetcode.com/problems/squares-of-a-sorted-array/)
* [Interval List Intersections (Medium)](https://leetcode.com/problems/interval-list-intersections/)
## Solution 1.
```cpp
// OJ: https://leetcode.com/problems/merge-sorted-array/
// Author: github.com/lzl124631x
// Time: O(M + N)
// Space: O(1)
class Solution {
public:
void merge(vector<int>& A, int m, vector<int>& B, int n) {
for (int i = m - 1, j = n - 1, k = m + n - 1; k >= 0; --k) {
if (j < 0 || (i >= 0 && A[i] > B[j])) A[k] = A[i--];
else A[k] = B[j--];
}
}
};
```

DdddJMs__135
- 粉丝: 3129
- 资源: 754
最新资源
- 【多智能体控制】基于matlab自适应领导者与追随者动态规划仿真【含Matlab源码 8003期】.mp4
- 压合半自动组装线体工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 移动式液压伸缩提升机工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- sgg慕尚花坊项目代码
- 折弯激光焊接设备工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 已生产的插针机工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 纸盒成型机工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 智能仓库穿梭车工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 纸盒六面包膜机覆膜机工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 自动Mylar贴合机工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 自动裁切机工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 自动调节双轴变位机单边基座工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 自动裁布机工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 自动焊管机工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 自制点胶系统工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 自动覆膜设备工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


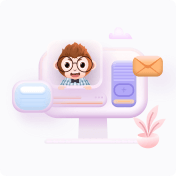