%OFDM Simulation in MATLAB
%The intial code is from Jordan Street's work.
close all;
clc
clear all;
%% Simulation Parameters
%Moduluation method: BPSK, QPSK, 8PSK, 16QAM, 32QAM, 64QAM,
%Assume we use 14 channels of 256. 1,2 and 3 we use 128QAM, 4 and 5 we use 64QAM, 6,7,8 we use 32QAM
mod_methods = {'BPSK', 'QPSK', '8QAM', '16QAM', '32QAM', '64QAM','128QAM','256QAM'};
%Channel Information we use
Sub_channel_num_used = 80;
Channel_bit = [10,10,10,10,10,10,10,10];%Channel number of each modulation scheme
Total_bit_num_in_one_ofdm_symbol = 8*Channel_bit(8) + 7*Channel_bit(7) + 6*Channel_bit(6) + 5*Channel_bit(5) + 4*Channel_bit(4) + 3*Channel_bit(3) + 2*Channel_bit(2) + 1*Channel_bit(1);
%fft size
nfft = 512;
%size of cycle prefix extension
n_cpe = 16;
%Channel estimation method
ch_est_method = 'LS';
%ch_est_method = 'none';
%SNR in dB
snr = 10;%The unit is Db, the ratio of average power of signal and noise
%Number of channel taps
n_taps = 8;%this is the same as the example, I think it is the pulse response
%Check whether the parameter is right or not?
if Sub_channel_num_used ~= sum(Channel_bit)
disp('error: The summary of bit allocated channel is not equal to total used channel');
exit;
end
if Sub_channel_num_used >= nfft/2 - 1%dc cannot be used in the simulation
disp('error: The number of used subchannel is larger than fft number');
exit;
end
%%Read the image and convert to binary format, we use one picture as our signal source
im = imread('baboon.png');
im_bin_1 = im(:);
im_bin_2 = dec2bin(im_bin_1,8)';
im_bin = im_bin_2(:); %transfer the matrix to bit sequence
%% Transmisstor
%Binary Stream to Symbol
%We assume we just use 20 subchannel, the first five we use first five channel as
%Step 1: Padding source signal
sys_rem = mod(Total_bit_num_in_one_ofdm_symbol-mod(length(im_bin),Total_bit_num_in_one_ofdm_symbol),Total_bit_num_in_one_ofdm_symbol);%calculate the bit not enought for one OFDM frame
padding = repmat('0',sys_rem,1);
im_bin_padded = [im_bin; padding];%combining in row
ofdm_symbol_total_num = length(im_bin_padded)/Total_bit_num_in_one_ofdm_symbol;
cons_data = reshape(im_bin_padded,Total_bit_num_in_one_ofdm_symbol,ofdm_symbol_total_num);
%Mapping source bit to symbol
%Step 2: Devide the signal source into different groups according modulation scheme, offline processing method
cons_data_1bit = cons_data(1:1*Channel_bit(1),:);
cons_data_2bit = cons_data(1*Channel_bit(1)+1:1*Channel_bit(1)+2*Channel_bit(2),:);
cons_data_3bit = cons_data(1*Channel_bit(1)+2*Channel_bit(2)+1:1*Channel_bit(1)+2*Channel_bit(2)+3*Channel_bit(3),:);
cons_data_4bit = cons_data(1*Channel_bit(1)+2*Channel_bit(2)+3*Channel_bit(3)+1:1*Channel_bit(1)+2*Channel_bit(2)+3*Channel_bit(3)+4*Channel_bit(4),:);
cons_data_5bit = cons_data(1*Channel_bit(1)+2*Channel_bit(2)+3*Channel_bit(3)+4*Channel_bit(4)+1:1*Channel_bit(1)+2*Channel_bit(2)+3*Channel_bit(3)+4*Channel_bit(4)+5*Channel_bit(5),:);
cons_data_6bit = cons_data(1*Channel_bit(1)+2*Channel_bit(2)+3*Channel_bit(3)+4*Channel_bit(4)+5*Channel_bit(5)+1:1*Channel_bit(1)+2*Channel_bit(2)+3*Channel_bit(3)+4*Channel_bit(4)+5*Channel_bit(5)+6*Channel_bit(6),:);
cons_data_7bit = cons_data(1*Channel_bit(1)+2*Channel_bit(2)+3*Channel_bit(3)+4*Channel_bit(4)+5*Channel_bit(5)+6*Channel_bit(6)+1:1*Channel_bit(1)+2*Channel_bit(2)+3*Channel_bit(3)+4*Channel_bit(4)+5*Channel_bit(5)+6*Channel_bit(6)+7*Channel_bit(7),:);
cons_data_8bit = cons_data(1*Channel_bit(1)+2*Channel_bit(2)+3*Channel_bit(3)+4*Channel_bit(4)+5*Channel_bit(5)+6*Channel_bit(6)+7*Channel_bit(7)+1:1*Channel_bit(1)+2*Channel_bit(2)+3*Channel_bit(3)+4*Channel_bit(4)+5*Channel_bit(5)+6*Channel_bit(6)+7*Channel_bit(7)+8*Channel_bit(8),:);
%Step 3: reshaping the each group for symbol mapping, and do the tranposition operation for mapping
cons_data_1bit_reshape = reshape(cons_data_1bit,1,ofdm_symbol_total_num*Channel_bit(1))';
cons_data_2bit_reshape = reshape(cons_data_2bit,2,ofdm_symbol_total_num*Channel_bit(2))';
cons_data_3bit_reshape = reshape(cons_data_3bit,3,ofdm_symbol_total_num*Channel_bit(3))';
cons_data_4bit_reshape = reshape(cons_data_4bit,4,ofdm_symbol_total_num*Channel_bit(4))';
cons_data_5bit_reshape = reshape(cons_data_5bit,5,ofdm_symbol_total_num*Channel_bit(5))';
cons_data_6bit_reshape = reshape(cons_data_6bit,6,ofdm_symbol_total_num*Channel_bit(6))';
cons_data_7bit_reshape = reshape(cons_data_7bit,7,ofdm_symbol_total_num*Channel_bit(7))';
cons_data_8bit_reshape = reshape(cons_data_8bit,8,ofdm_symbol_total_num*Channel_bit(8))';
%Step 4: transform from bit to dec for symbol mapping
cons_data_1bit_sys_id = bin2dec(cons_data_1bit_reshape);
cons_data_2bit_sys_id = bin2dec(cons_data_2bit_reshape);
cons_data_3bit_sys_id = bin2dec(cons_data_3bit_reshape);
cons_data_4bit_sys_id = bin2dec(cons_data_4bit_reshape);
cons_data_5bit_sys_id = bin2dec(cons_data_5bit_reshape);
cons_data_6bit_sys_id = bin2dec(cons_data_6bit_reshape);
cons_data_7bit_sys_id = bin2dec(cons_data_7bit_reshape);
cons_data_8bit_sys_id = bin2dec(cons_data_8bit_reshape);
%Adding this step to calculate the symbol error rate in each subchannel
cons_data_1bit_sys_id_reshape = reshape(cons_data_1bit_sys_id,Channel_bit(1),ofdm_symbol_total_num);
cons_data_2bit_sys_id_reshape = reshape(cons_data_2bit_sys_id,Channel_bit(2),ofdm_symbol_total_num);
cons_data_3bit_sys_id_reshape = reshape(cons_data_3bit_sys_id,Channel_bit(3),ofdm_symbol_total_num);
cons_data_4bit_sys_id_reshape = reshape(cons_data_4bit_sys_id,Channel_bit(4),ofdm_symbol_total_num);
cons_data_5bit_sys_id_reshape = reshape(cons_data_5bit_sys_id,Channel_bit(5),ofdm_symbol_total_num);
cons_data_6bit_sys_id_reshape = reshape(cons_data_6bit_sys_id,Channel_bit(6),ofdm_symbol_total_num);
cons_data_7bit_sys_id_reshape = reshape(cons_data_7bit_sys_id,Channel_bit(7),ofdm_symbol_total_num);
cons_data_8bit_sys_id_reshape = reshape(cons_data_8bit_sys_id,Channel_bit(8),ofdm_symbol_total_num);
%Step 4: Mapping and judging whether the matrix is empty or not
not_empty_flag = zeros(8,1);
if Channel_bit(1)~=0
cons_data_1bit_symbol = qammod(cons_data_1bit_sys_id,2^1,'UnitAveragePower',true);
cons_data_1bit_symbol_reshape = reshape(cons_data_1bit_symbol,Channel_bit(1),ofdm_symbol_total_num);
not_empty_flag(1) = 1;
else
cons_data_1bit_symbol_reshape = [];
end
if Channel_bit(2)~=0
cons_data_2bit_symbol = qammod(cons_data_2bit_sys_id,2^2,'UnitAveragePower',true);
cons_data_2bit_symbol_reshape = reshape(cons_data_2bit_symbol,Channel_bit(2),ofdm_symbol_total_num);
not_empty_flag(2) = 1;
else
cons_data_2bit_symbol_reshape = [];
end
if Channel_bit(3)~=0
cons_data_3bit_symbol = qammod(cons_data_3bit_sys_id,2^3,'UnitAveragePower',true);
cons_data_3bit_symbol_reshape = reshape(cons_data_3bit_symbol,Channel_bit(3),ofdm_symbol_total_num);
not_empty_flag(3) = 1;
else
cons_data_3bit_symbol_reshape = [];
end
if Channel_bit(4)~=0
cons_data_4bit_symbol = qammod(cons_data_4bit_sys_id,2^4,'UnitAveragePower',true);
cons_data_4bit_symbol_reshape = reshape(cons_data_4bit_symbol,Channel_bit(4),ofdm_symbol_total_num);
not_empty_flag(4) = 1;
else
cons_data_4bit_symbol_reshape = [];
end
if Channel_bit(5)~=0
cons_data_5bit_symbol = qammod(cons_data_5bit_sys_id,2^5,'UnitAveragePower',true);
cons_data_5bit_symbol_reshape = reshape(cons_data_5bit_symbol,Channel_bit(5),ofdm_symbol_total_num);
not_empty_flag(5) = 1;
else
cons_data_5bit_symbol_reshape = [];
end
if Channel_bit(6)~=0
cons_data_6bit_symbol = qammod(cons_data_6bit_sys_id,2^6,'UnitAveragePower',true);
cons_data_6bit_symbol_reshape = reshape(cons_data_6bit_symbol,Channel_bit(6),ofdm_symbol_total_num);
not_empty_flag(6) = 1;
else
cons_data_6bit_symbol_reshape = [];
end
if Channel_bit(7)~=0
cons_data_7bit_symbol = qammod(cons_data_7bit_sys_id,2^7,'UnitAveragePower',true);
cons_data_7bit_symbol_reshape = reshape(cons_data_7bit_symbol,Channel_bit(7),ofdm_symbol_total_num);
not_empty_flag(7) = 1;
else
cons_data_7bit_symbol_reshape = [];
end
if Channel_bit(8)~=0
cons_data_8bit_symbol = qammod(cons_data_8
没有合适的资源?快使用搜索试试~ 我知道了~
VLC-OFDM-Simulation-original
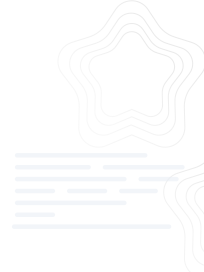
共11个文件
jpg:3个
mat:2个
png:2个

0 下载量 200 浏览量
2024-08-28
21:17:53
上传
评论
收藏 694KB ZIP 举报
温馨提示
VLC_OFDM_Simulation_original
资源推荐
资源详情
资源评论
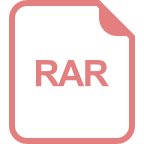
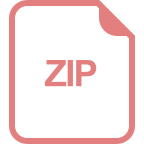
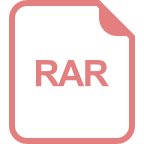
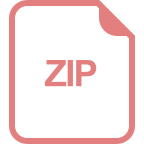
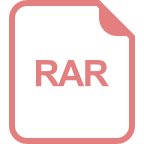
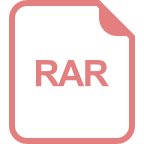
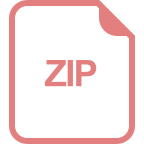
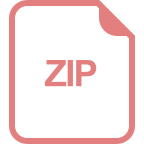
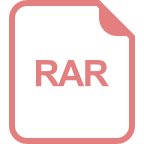
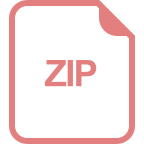
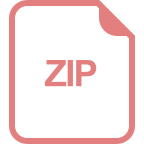
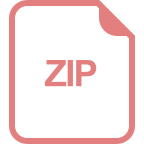
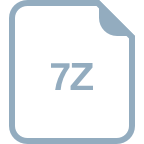
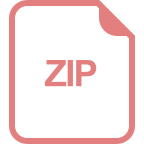
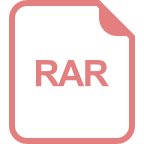
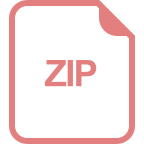
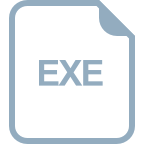
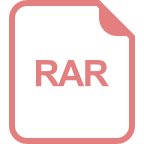
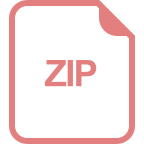
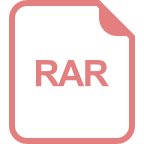
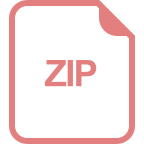
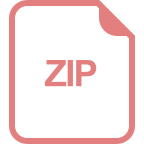
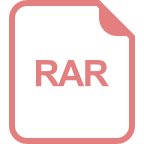
收起资源包目录


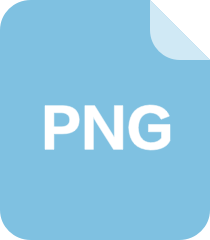
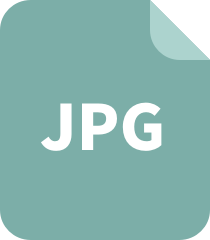




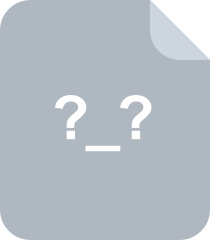
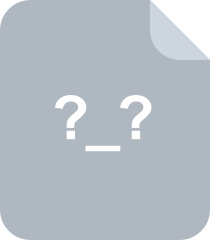

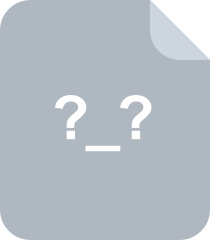
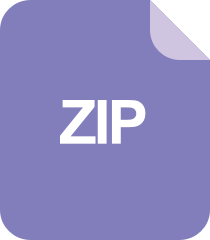
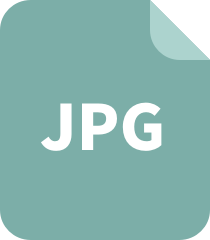
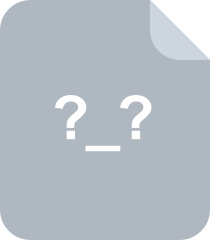
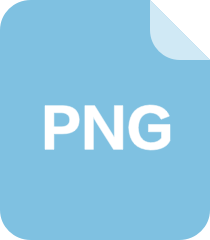
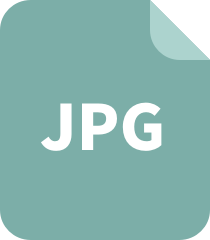
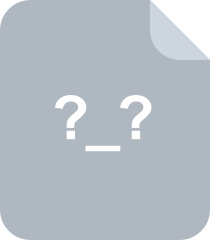
共 11 条
- 1
资源评论
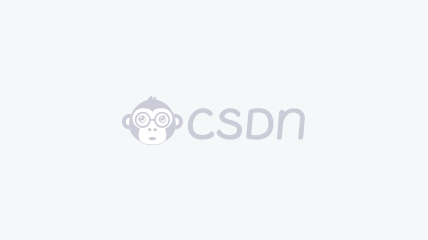

潦草通信狗
- 粉丝: 338
- 资源: 215
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

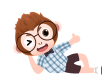
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


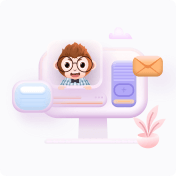
安全验证
文档复制为VIP权益,开通VIP直接复制
