package com.tong.myshiro.config;
import at.pollux.thymeleaf.shiro.dialect.ShiroDialect;
import com.tong.myshiro.listener.MySessionListener;
import com.tong.myshiro.realm.MyRealm;
import com.tong.myshiro.realm.MyRealm2;
import org.apache.shiro.authc.credential.HashedCredentialsMatcher;
import org.apache.shiro.authc.pam.AllSuccessfulStrategy;
import org.apache.shiro.authc.pam.ModularRealmAuthenticator;
import org.apache.shiro.cache.ehcache.EhCacheManager;
import org.apache.shiro.codec.Base64;
import org.apache.shiro.realm.Realm;
import org.apache.shiro.session.SessionListener;
import org.apache.shiro.session.mgt.SessionManager;
import org.apache.shiro.spring.security.interceptor.AuthorizationAttributeSourceAdvisor;
import org.apache.shiro.spring.web.ShiroFilterFactoryBean;
import org.apache.shiro.web.mgt.CookieRememberMeManager;
import org.apache.shiro.web.mgt.DefaultWebSecurityManager;
import org.apache.shiro.web.servlet.SimpleCookie;
import org.apache.shiro.web.session.mgt.DefaultWebSessionManager;
import org.springframework.aop.framework.autoproxy.DefaultAdvisorAutoProxyCreator;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Configuration
public class ShiroConfig {
// Realm管理者
@Bean
public ModularRealmAuthenticator modularRealmAuthenticator(){
ModularRealmAuthenticator modularRealmAuthenticator = new ModularRealmAuthenticator();
// 设置认证策略
modularRealmAuthenticator.setAuthenticationStrategy(new AllSuccessfulStrategy());
return modularRealmAuthenticator;
}
@Bean
public DefaultWebSecurityManager securityManager(MyRealm myRealm,
MyRealm2 myRealm2,
SessionManager sessionManager,
CookieRememberMeManager rememberMeManager,
EhCacheManager ehCacheManager){
DefaultWebSecurityManager securityManager = new DefaultWebSecurityManager();
// 自定义Realm放入SecurityManager中
// securityManager.setRealm(myRealm);
// 设置Realm管理者(需要设置在Realm之前)
securityManager.setAuthenticator(modularRealmAuthenticator());
List<Realm> realms = new ArrayList();
realms.add(myRealm);
// realms.add(myRealm2);
securityManager.setRealms(realms);
securityManager.setSessionManager(sessionManager);
securityManager.setRememberMeManager(rememberMeManager);
securityManager.setCacheManager(ehCacheManager);
return securityManager;
}
// 配置加密算法
@Bean
public HashedCredentialsMatcher hashedCredentialsMatcher(){
HashedCredentialsMatcher hashedCredentialsMatcher = new HashedCredentialsMatcher();
hashedCredentialsMatcher.setHashAlgorithmName("md5");
// 加密次数
hashedCredentialsMatcher.setHashIterations(5);
return hashedCredentialsMatcher;
}
@Bean
public MyRealm myRealm(HashedCredentialsMatcher hashedCredentialsMatcher){
MyRealm myRealm = new MyRealm();
myRealm.setCredentialsMatcher(hashedCredentialsMatcher);
return myRealm;
}
@Bean
public MyRealm2 myRealm2(){
return new MyRealm2();
}
// 配置过滤器
@Bean
public ShiroFilterFactoryBean shiroFilterFactoryBean(DefaultWebSecurityManager securityManager){
// 1.创建过滤器工厂
ShiroFilterFactoryBean filterFactory = new ShiroFilterFactoryBean();
// 2.过滤器工厂设置SecurityManager
filterFactory.setSecurityManager(securityManager);
// 3.设置Shiro的拦截规则
Map<String,String> filterMap = new HashMap();
// 不拦截的资源
filterMap.put("/login.html","anon");
filterMap.put("/fail.html","anon");
filterMap.put("/user/login2","anon");
filterMap.put("/css/**","anon");
// 鉴权过滤器,要写在/**之前
filterMap.put("/reportform/find","perms[/reportform/find]");
filterMap.put("/salary/find","perms[/salary/find]");
filterMap.put("/staff/find","perms[/staff/find]");
// 其余资源都拦截,authc表示需要登录认证才能访问,user表示登录认证和记住我认证都能访问
// filterMap.put("/**","authc");
filterMap.put("/user/pay","authc");
// filterMap.put("/**","user");
// 4.将拦截规则设置给过滤器工厂
filterFactory.setFilterChainDefinitionMap(filterMap);
// 5.配置登录页面
filterFactory.setLoginUrl("/login.html");
// 6.配置权限不足访问的页面
filterFactory.setUnauthorizedUrl("/noPermission.html");
return filterFactory;
}
// 会话管理器
@Bean
public SessionManager sessionManager(MySessionListener sessionListener){
// 创建会话管理器
DefaultWebSessionManager sessionManager = new DefaultWebSessionManager();
// 创建会话监听器集合
List<SessionListener> listeners = new ArrayList();
listeners.add(sessionListener);
// 将监听器集合设置到会话管理器中
sessionManager.setSessionListeners(listeners);
// 全局会话超时时间
// sessionManager.setGlobalSessionTimeout(5000);
// 是否删除无效的session对象,默认为true
sessionManager.setDeleteInvalidSessions(true);
// 是否开启定时调度器检测过期session,默认为true
sessionManager.setSessionValidationSchedulerEnabled(true);
return sessionManager;
}
// Cookie生成器
@Bean
public SimpleCookie simpleCookie(){
SimpleCookie simpleCookie = new SimpleCookie("rememberMe");
// Cookie的有效时间
simpleCookie.setMaxAge(20);
return simpleCookie;
}
// 记住我管理器
@Bean
public CookieRememberMeManager cookieRememberMeManager(SimpleCookie simpleCookie){
CookieRememberMeManager cookieRememberMeManager = new CookieRememberMeManager();
cookieRememberMeManager.setCookie(simpleCookie);
// Cookie加密的密钥
cookieRememberMeManager.setCipherKey(Base64.decode("6ZmI6I2j3Y+R1aSn5BOlAA=="));
return cookieRememberMeManager;
}
// 开启Shiro注解的支持
@Bean
public AuthorizationAttributeSourceAdvisor authorizationAttributeSourceAdvisor(DefaultWebSecurityManager securityManager){
AuthorizationAttributeSourceAdvisor authorizationAttributeSourceAdvisor = new AuthorizationAttributeSourceAdvisor();
authorizationAttributeSourceAdvisor.setSecurityManager(securityManager);
return authorizationAttributeSourceAdvisor;
}
// 开启AOP注解支持
@Bean
public DefaultAdvisorAutoProxyCreator defaultAdvisorAutoProxyCreator(){
DefaultAdvisorAutoProxyCreator defaultAdvisorAutoProxyCreator = new DefaultAdvisorAutoProxyCreator();
defaultAdvisorAutoProxyCreator.setProxyTargetClass(true);
return defaultAdvisorAutoProxyCreator;
}
// shiro整合thymeleaf
@Bean
public ShiroDialect shiroDialect(){
return new ShiroDialect();
}
// CacheManager
@Bean
public EhCacheManager ehCacheManager(){
EhCacheManager ehCacheManager = new EhCacheManager();
ehCacheManager.setCacheManagerConfigFile("classpath:shiro-ehcache.xml");
return ehCacheManager;
}
// @Bean
// public JdbcRealm jdbcRealm(DataSource dataSource){
// JdbcRealm jdbcRealm = new JdbcRealm();
//
没有合适的资源?快使用搜索试试~ 我知道了~
最详细Shiro学习资料(源码)
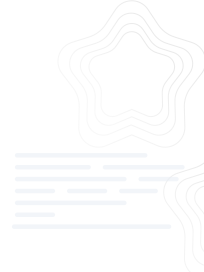
共172个文件
xml:112个
class:19个
java:19个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 21 浏览量
2023-11-16
21:15:17
上传
评论
收藏 151KB RAR 举报
温馨提示
Shiro(Apache Shiro)是一个强大且易于使用的Java安全框架,用于身份验证、授权、加密和会话管理等安全功能。它提供了简单的API和灵活的配置选项,可以方便地集成到现有的Java应用程序中。 Shiro的主要特点包括: 身份验证:Shiro支持多种身份验证方式,包括用户名/密码验证、基于角色的验证、基于证书的验证等。开发者可以根据具体需求选择适合的身份验证方式。 授权:Shiro提供了细粒度的授权机制,可以对用户进行角色和权限的控制。通过简单的注解或编程方式,开发者可以定义哪些用户可以访问哪些资源。 加密:Shiro支持常见的加密算法和哈希函数,可以确保用户密码等敏感信息的安全存储。 会话管理:Shiro提供了会话管理功能,可以管理用户的会话状态,并支持分布式会话管理。 Web集成:Shiro可以无缝集成到Java Web应用程序中,提供了过滤器和标签库等工具,简化了权限控制和身份验证的实现。 容器友好:Shiro可以与常见的Java容器(如Spring、Guice)以及其他框架(如Apache Struts、Apache Wicket)进行集成,方便开发者
资源推荐
资源详情
资源评论
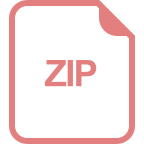
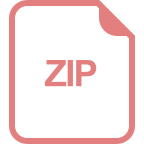
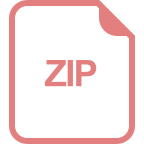
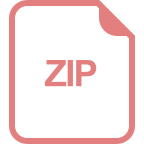
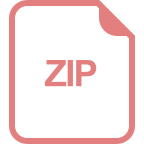
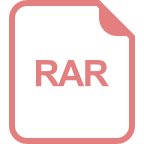
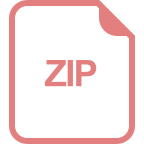
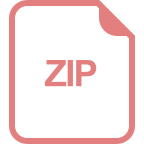
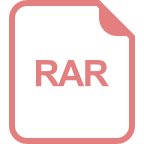
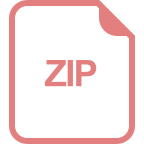
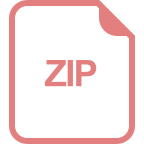
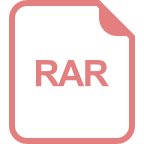
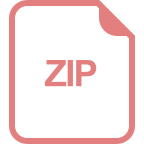
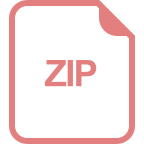
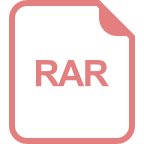
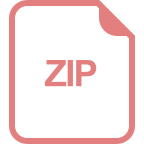
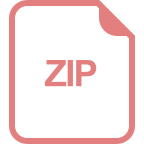
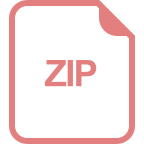
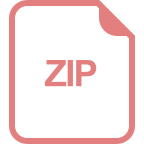
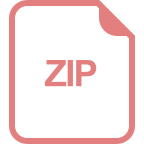
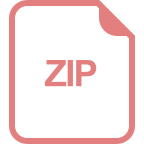
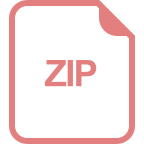
收起资源包目录

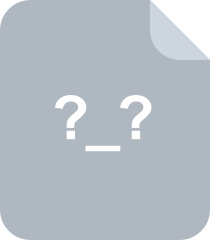
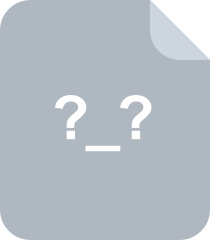
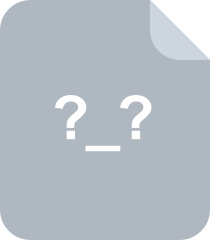
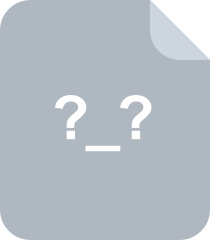
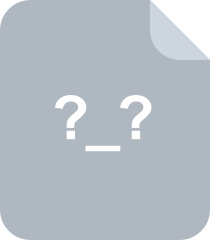
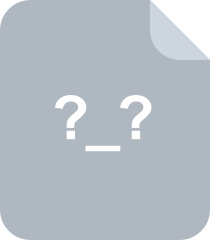
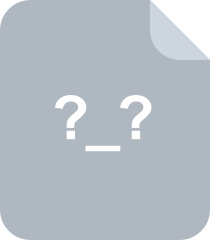
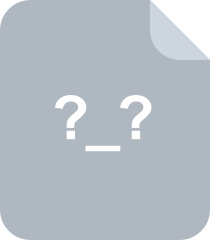
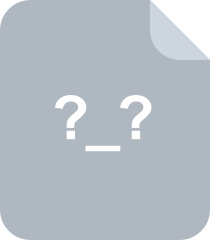
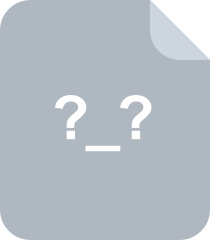
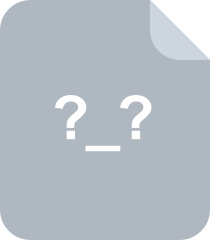
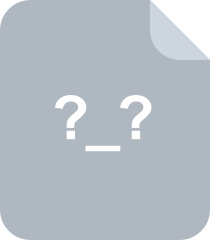
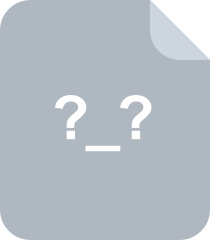
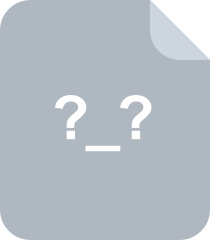
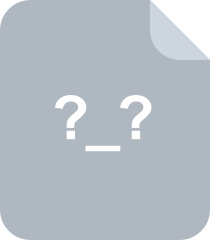
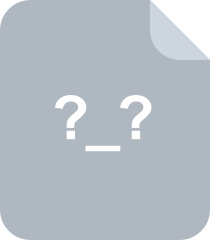
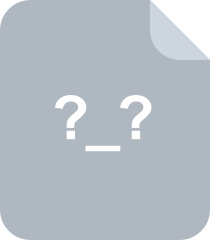
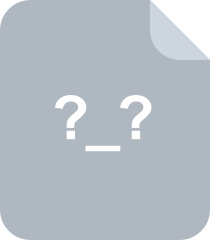
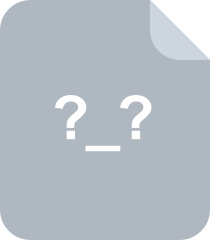
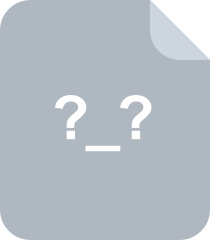
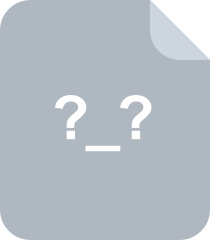
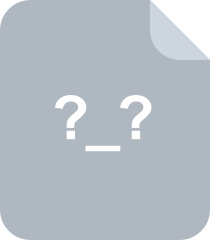
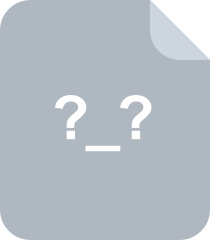
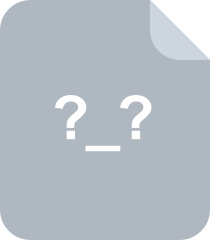
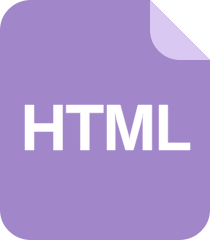
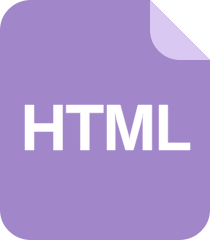
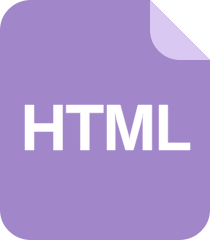
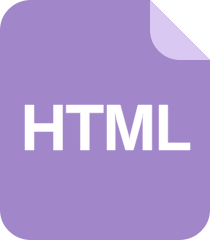
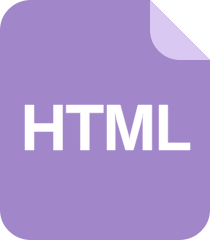
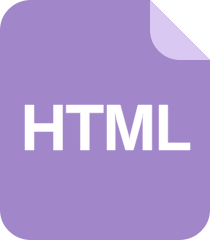
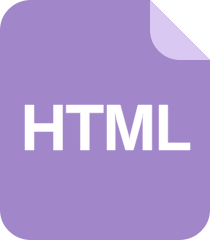
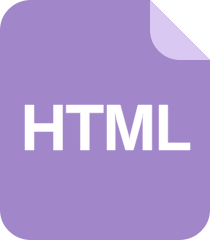
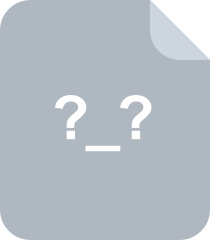
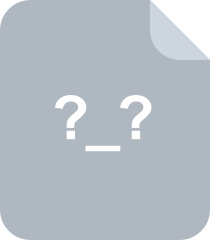
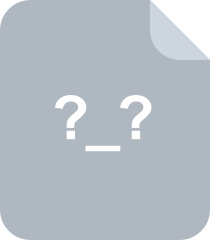
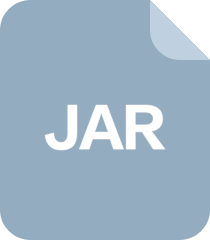
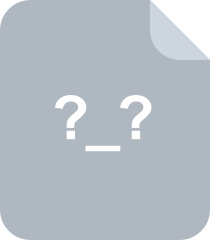
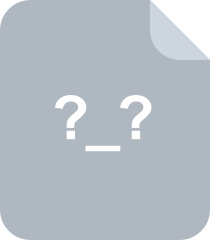
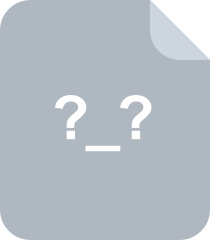
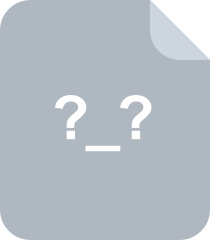
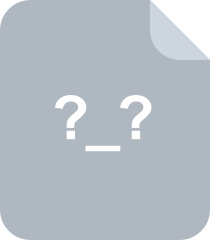
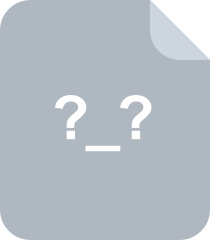
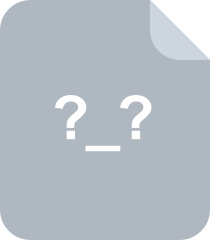
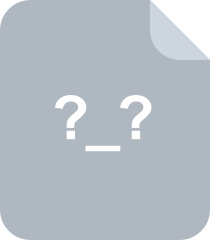
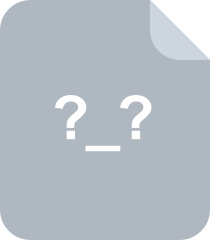
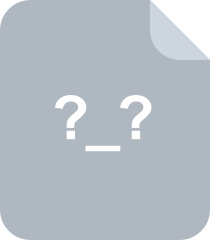
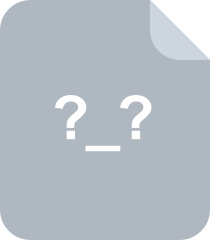
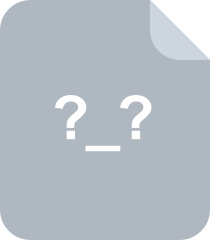
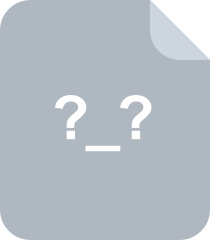
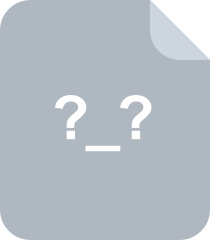
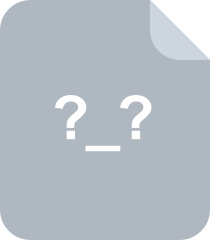
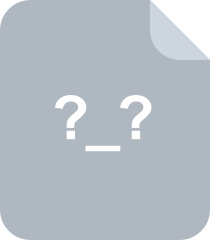
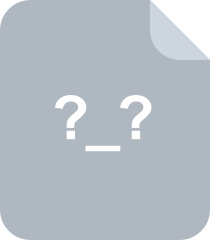
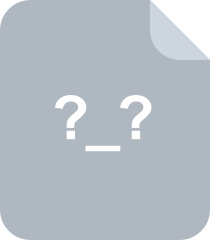
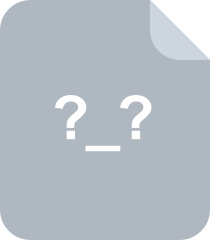
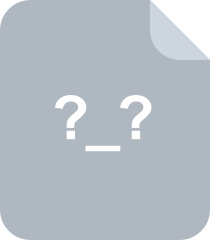
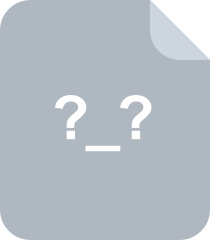
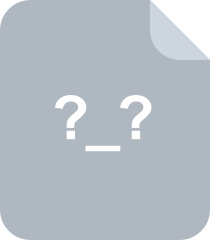
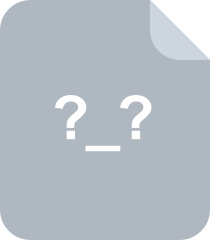
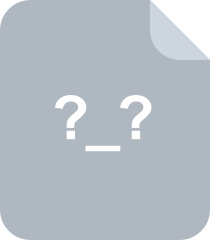
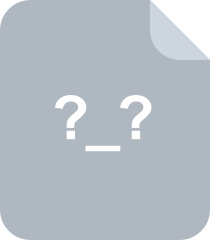
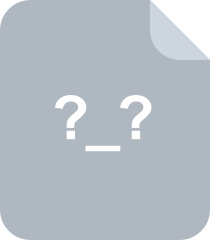
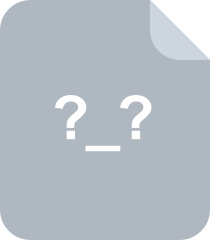
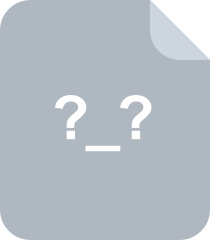
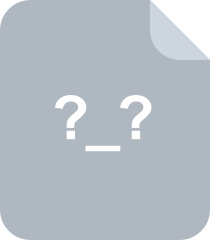
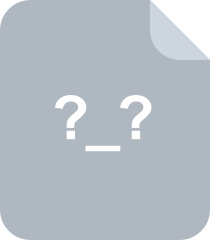
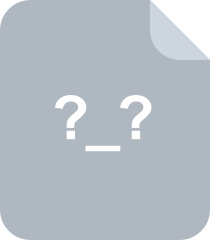
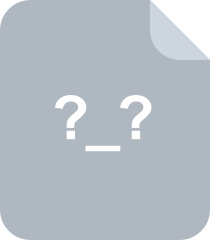
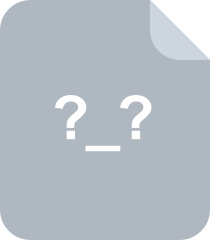
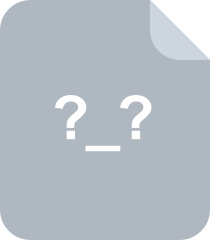
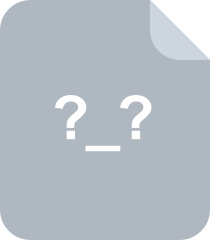
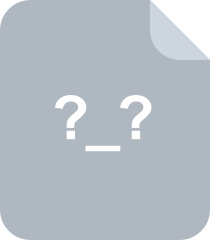
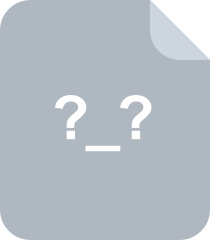
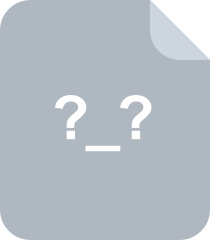
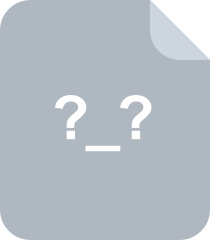
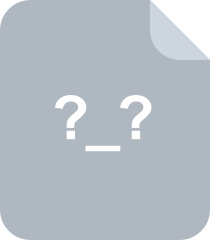
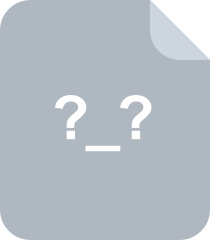
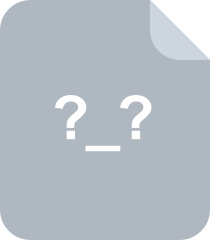
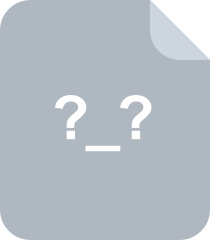
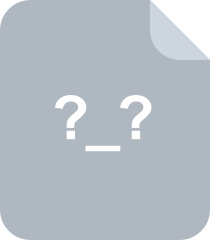
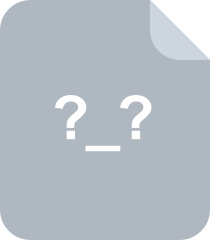
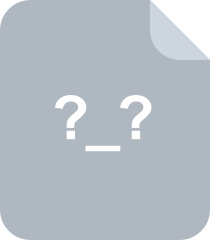
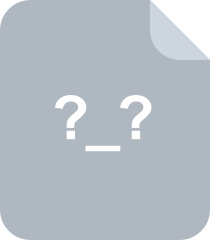
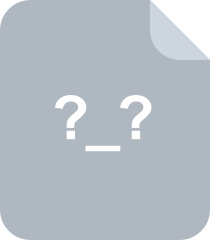
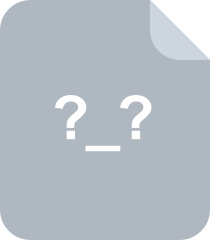
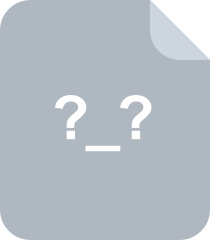
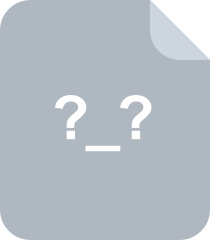
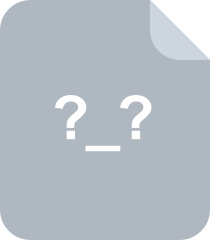
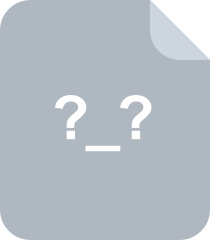
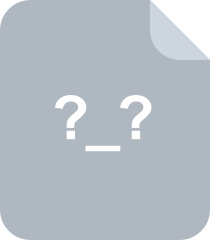
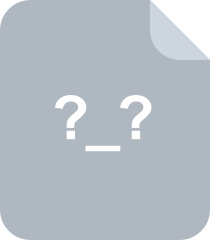
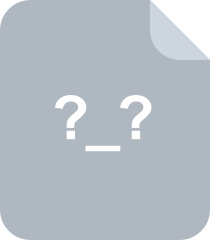
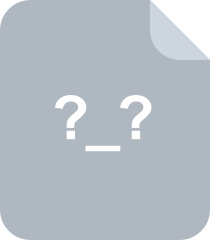
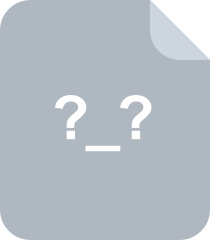
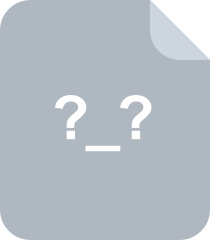
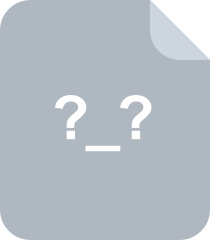
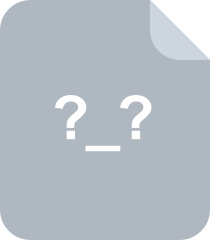
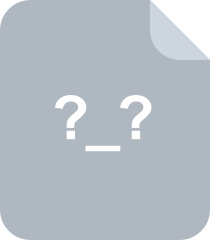
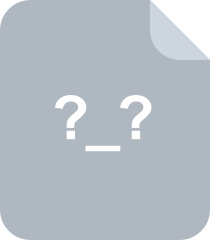
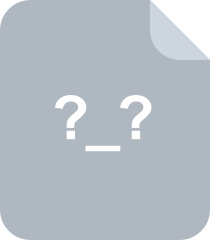
共 172 条
- 1
- 2
资源评论
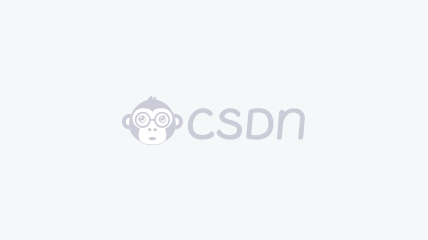

童小纯
- 粉丝: 3w+
- 资源: 289
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

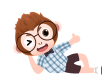
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


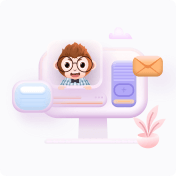
安全验证
文档复制为VIP权益,开通VIP直接复制
